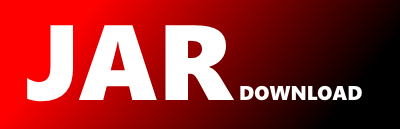
R.Organization-methods.R Maven / Gradle / Ivy
# WARNING: AUTOGENERATED CODE
#
# This code was generated by a tool.
#
# Manual changes to this file may cause unexpected behavior in your application.
# Manual changes to this file will be overwritten if the code is regenerated.
# ##############################################################################
#' OrganizationClient methods
#' @include AllClasses.R
#' @include AllGenerics.R
#' @include commons.R
#' @description This function implements the OpenCGA calls for managing Organizations.
#' The following table summarises the available *actions* for this client:
#'
#' | endpointName | Endpoint WS | parameters accepted |
#' | -- | :-- | --: |
#' | create | /{apiVersion}/organizations/create | include, exclude, includeResult, body[*] |
#' | createNotes | /{apiVersion}/organizations/notes/create | include, exclude, includeResult, body[*] |
#' | searchNotes | /{apiVersion}/organizations/notes/search | include, exclude, creationDate, modificationDate, id, scope, visibility, uuid, userId, tags, version |
#' | deleteNotes | /{apiVersion}/organizations/notes/{id}/delete | id[*], includeResult |
#' | updateNotes | /{apiVersion}/organizations/notes/{id}/update | include, exclude, id[*], tagsAction, includeResult, body[*] |
#' | userUpdateStatus | /{apiVersion}/organizations/user/{user}/status/update | include, exclude, user[*], organization, includeResult, body[*] |
#' | updateUser | /{apiVersion}/organizations/user/{user}/update | include, exclude, user[*], organization, includeResult, body[*] |
#' | updateConfiguration | /{apiVersion}/organizations/{organization}/configuration/update | include, exclude, organization[*], includeResult, authenticationOriginsAction, body[*] |
#' | info | /{apiVersion}/organizations/{organization}/info | include, exclude, organization[*] |
#' | update | /{apiVersion}/organizations/{organization}/update | include, exclude, organization[*], includeResult, adminsAction, body[*] |
#'
#' @md
#' @seealso \url{http://docs.opencb.org/display/opencga/Using+OpenCGA} and the RESTful API documentation
#' \url{http://bioinfo.hpc.cam.ac.uk/opencga-prod/webservices/}
#' [*]: Required parameter
#' @export
setMethod("organizationClient", "OpencgaR", function(OpencgaR, id, organization, user, endpointName, params=NULL, ...) {
switch(endpointName,
#' @section Endpoint /{apiVersion}/organizations/create:
#' Create a new organization.
#' @param include Fields included in the response, whole JSON path must be provided.
#' @param exclude Fields excluded in the response, whole JSON path must be provided.
#' @param includeResult Flag indicating to include the created or updated document result in the response.
#' @param data JSON containing the organization to be created.
create=fetchOpenCGA(object=OpencgaR, category="organizations", categoryId=NULL, subcategory=NULL,
subcategoryId=NULL, action="create", params=params, httpMethod="POST", as.queryParam=NULL, ...),
#' @section Endpoint /{apiVersion}/organizations/notes/create:
#' Create a new note.
#' @param include Fields included in the response, whole JSON path must be provided.
#' @param exclude Fields excluded in the response, whole JSON path must be provided.
#' @param includeResult Flag indicating to include the created or updated document result in the response.
#' @param data JSON containing the Note to be added.
createNotes=fetchOpenCGA(object=OpencgaR, category="organizations", categoryId=NULL, subcategory="notes",
subcategoryId=NULL, action="create", params=params, httpMethod="POST", as.queryParam=NULL, ...),
#' @section Endpoint /{apiVersion}/organizations/notes/search:
#' Search for notes of scope ORGANIZATION.
#' @param include Fields included in the response, whole JSON path must be provided.
#' @param exclude Fields excluded in the response, whole JSON path must be provided.
#' @param creationDate Creation date. Format: yyyyMMddHHmmss. Examples: >2018, 2017-2018, <201805.
#' @param modificationDate Modification date. Format: yyyyMMddHHmmss. Examples: >2018, 2017-2018, <201805.
#' @param id Note unique identifier.
#' @param scope Scope of the Note.
#' @param visibility Visibility of the Note.
#' @param uuid Unique 32-character identifier assigned automatically by OpenCGA.
#' @param userId User that wrote that Note.
#' @param tags Note tags.
#' @param version Autoincremental version assigned to the registered entry. By default, updates does not create new versions. To enable versioning, users must set the `incVersion` flag from the /update web service when updating the document.
searchNotes=fetchOpenCGA(object=OpencgaR, category="organizations", categoryId=NULL, subcategory="notes",
subcategoryId=NULL, action="search", params=params, httpMethod="GET", as.queryParam=NULL, ...),
#' @section Endpoint /{apiVersion}/organizations/notes/{id}/delete:
#' Delete note.
#' @param id Note unique identifier.
#' @param includeResult Flag indicating to include the created or updated document result in the response.
deleteNotes=fetchOpenCGA(object=OpencgaR, category="organizations", categoryId=NULL, subcategory="notes",
subcategoryId=id, action="delete", params=params, httpMethod="DELETE", as.queryParam=NULL, ...),
#' @section Endpoint /{apiVersion}/organizations/notes/{id}/update:
#' Update a note.
#' @param include Fields included in the response, whole JSON path must be provided.
#' @param exclude Fields excluded in the response, whole JSON path must be provided.
#' @param id Note unique identifier.
#' @param tagsAction Action to be performed if the array of tags is being updated. Allowed values: ['ADD SET REMOVE']
#' @param includeResult Flag indicating to include the created or updated document result in the response.
#' @param data JSON containing the Note fields to be updated.
updateNotes=fetchOpenCGA(object=OpencgaR, category="organizations", categoryId=NULL, subcategory="notes",
subcategoryId=id, action="update", params=params, httpMethod="POST", as.queryParam=NULL, ...),
#' @section Endpoint /{apiVersion}/organizations/user/{user}/status/update:
#' Update the user status.
#' @param include Fields included in the response, whole JSON path must be provided.
#' @param exclude Fields excluded in the response, whole JSON path must be provided.
#' @param user User ID.
#' @param organization Organization id.
#' @param includeResult Flag indicating to include the created or updated document result in the response.
#' @param data JSON containing the User fields to be updated.
userUpdateStatus=fetchOpenCGA(object=OpencgaR, category="organizations/user", categoryId=user,
subcategory="status", subcategoryId=NULL, action="update", params=params, httpMethod="POST",
as.queryParam=NULL, ...),
#' @section Endpoint /{apiVersion}/organizations/user/{user}/update:
#' Update the user information.
#' @param include Fields included in the response, whole JSON path must be provided.
#' @param exclude Fields excluded in the response, whole JSON path must be provided.
#' @param user User ID.
#' @param organization Organization id.
#' @param includeResult Flag indicating to include the created or updated document result in the response.
#' @param data JSON containing the User fields to be updated.
updateUser=fetchOpenCGA(object=OpencgaR, category="organizations", categoryId=NULL, subcategory="user",
subcategoryId=user, action="update", params=params, httpMethod="POST", as.queryParam=NULL, ...),
#' @section Endpoint /{apiVersion}/organizations/{organization}/configuration/update:
#' Update the Organization configuration attributes.
#' @param include Fields included in the response, whole JSON path must be provided.
#' @param exclude Fields excluded in the response, whole JSON path must be provided.
#' @param organization Organization id.
#' @param includeResult Flag indicating to include the created or updated document result in the response.
#' @param authenticationOriginsAction Action to be performed if the array of authenticationOrigins is being updated. Allowed values: ['ADD SET REMOVE REPLACE']
#' @param data JSON containing the params to be updated.
updateConfiguration=fetchOpenCGA(object=OpencgaR, category="organizations", categoryId=organization,
subcategory="configuration", subcategoryId=NULL, action="update", params=params, httpMethod="POST",
as.queryParam=NULL, ...),
#' @section Endpoint /{apiVersion}/organizations/{organization}/info:
#' Return the organization information.
#' @param include Fields included in the response, whole JSON path must be provided.
#' @param exclude Fields excluded in the response, whole JSON path must be provided.
#' @param organization Organization id.
info=fetchOpenCGA(object=OpencgaR, category="organizations", categoryId=organization, subcategory=NULL,
subcategoryId=NULL, action="info", params=params, httpMethod="GET", as.queryParam=NULL, ...),
#' @section Endpoint /{apiVersion}/organizations/{organization}/update:
#' Update some organization attributes.
#' @param include Fields included in the response, whole JSON path must be provided.
#' @param exclude Fields excluded in the response, whole JSON path must be provided.
#' @param organization Organization id.
#' @param includeResult Flag indicating to include the created or updated document result in the response.
#' @param adminsAction Action to be performed if the array of admins is being updated. Allowed values: ['ADD REMOVE']
#' @param data JSON containing the params to be updated.
update=fetchOpenCGA(object=OpencgaR, category="organizations", categoryId=organization, subcategory=NULL,
subcategoryId=NULL, action="update", params=params, httpMethod="POST", as.queryParam=NULL, ...),
)
})
© 2015 - 2025 Weber Informatics LLC | Privacy Policy