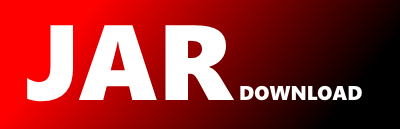
R.User-methods.R Maven / Gradle / Ivy
# WARNING: AUTOGENERATED CODE
#
# This code was generated by a tool.
#
# Manual changes to this file may cause unexpected behavior in your application.
# Manual changes to this file will be overwritten if the code is regenerated.
# ##############################################################################
#' UserClient methods
#' @include AllClasses.R
#' @include AllGenerics.R
#' @include commons.R
#' @description This function implements the OpenCGA calls for managing Users.
#' The following table summarises the available *actions* for this client:
#'
#' | endpointName | Endpoint WS | parameters accepted |
#' | -- | :-- | --: |
#' | anonymous | /{apiVersion}/users/anonymous | organization[*] |
#' | create | /{apiVersion}/users/create | body[*] |
#' | login | /{apiVersion}/users/login | body |
#' | password | /{apiVersion}/users/password | body[*] |
#' | search | /{apiVersion}/users/search | include, exclude, limit, skip, count, organization, id, authenticationId |
#' | info | /{apiVersion}/users/{users}/info | include, exclude, organization, users[*] |
#' | configs | /{apiVersion}/users/{user}/configs | user[*], name |
#' | updateConfigs | /{apiVersion}/users/{user}/configs/update | user[*], action, body[*] |
#' | filters | /{apiVersion}/users/{user}/filters | user[*], id |
#' | updateFilters | /{apiVersion}/users/{user}/filters/update | user[*], action, body[*] |
#' | updateFilter | /{apiVersion}/users/{user}/filters/{filterId}/update | user[*], filterId[*], body[*] |
#' | resetPassword | /{apiVersion}/users/{user}/password/reset | user[*] |
#' | update | /{apiVersion}/users/{user}/update | include, exclude, user[*], includeResult, body[*] |
#'
#' @md
#' @seealso \url{http://docs.opencb.org/display/opencga/Using+OpenCGA} and the RESTful API documentation
#' \url{http://bioinfo.hpc.cam.ac.uk/opencga-prod/webservices/}
#' [*]: Required parameter
#' @export
setMethod("userClient", "OpencgaR", function(OpencgaR, filterId, user, users, endpointName, params=NULL, ...) {
switch(endpointName,
#' @section Endpoint /{apiVersion}/users/anonymous:
#' Get an anonymous token to gain access to the system.
#' @param organization Organization id.
anonymous=fetchOpenCGA(object=OpencgaR, category="users", categoryId=NULL, subcategory=NULL,
subcategoryId=NULL, action="anonymous", params=params, httpMethod="POST",
as.queryParam=c("organization"), ...),
#' @section Endpoint /{apiVersion}/users/create:
#' Create a new user.
#' @param data JSON containing the parameters.
create=fetchOpenCGA(object=OpencgaR, category="users", categoryId=NULL, subcategory=NULL, subcategoryId=NULL,
action="create", params=params, httpMethod="POST", as.queryParam=NULL, ...),
#' @section Endpoint /{apiVersion}/users/login:
#' Get identified and gain access to the system.
#' @param data JSON containing the authentication parameters.
login=fetchOpenCGA(object=OpencgaR, category="users", categoryId=NULL, subcategory=NULL, subcategoryId=NULL,
action="login", params=params, httpMethod="POST", as.queryParam=NULL, ...),
#' @section Endpoint /{apiVersion}/users/password:
#' Change the password of a user.
#' @param data JSON containing the change of password parameters.
password=fetchOpenCGA(object=OpencgaR, category="users", categoryId=NULL, subcategory=NULL, subcategoryId=NULL,
action="password", params=params, httpMethod="POST", as.queryParam=NULL, ...),
#' @section Endpoint /{apiVersion}/users/search:
#' User search method.
#' @param include Fields included in the response, whole JSON path must be provided.
#' @param exclude Fields excluded in the response, whole JSON path must be provided.
#' @param limit Number of results to be returned.
#' @param skip Number of results to skip.
#' @param count Get the total number of results matching the query. Deactivated by default.
#' @param organization Organization id.
#' @param id Comma separated list user IDs up to a maximum of 100. Also admits basic regular expressions using the operator '~', i.e. '~{perl-regex}' e.g. '~value' for case sensitive, '~/value/i' for case insensitive search.
#' @param authenticationId Authentication origin ID.
search=fetchOpenCGA(object=OpencgaR, category="users", categoryId=NULL, subcategory=NULL, subcategoryId=NULL,
action="search", params=params, httpMethod="GET", as.queryParam=NULL, ...),
#' @section Endpoint /{apiVersion}/users/{users}/info:
#' Return the user information including its projects and studies.
#' @param include Fields included in the response, whole JSON path must be provided.
#' @param exclude Fields excluded in the response, whole JSON path must be provided.
#' @param organization Organization id.
#' @param users Comma separated list of user IDs.
info=fetchOpenCGA(object=OpencgaR, category="users", categoryId=users, subcategory=NULL, subcategoryId=NULL,
action="info", params=params, httpMethod="GET", as.queryParam=NULL, ...),
#' @section Endpoint /{apiVersion}/users/{user}/configs:
#' Fetch a user configuration.
#' @param user User ID.
#' @param name Unique name (typically the name of the application).
configs=fetchOpenCGA(object=OpencgaR, category="users", categoryId=user, subcategory=NULL, subcategoryId=NULL,
action="configs", params=params, httpMethod="GET", as.queryParam=NULL, ...),
#' @section Endpoint /{apiVersion}/users/{user}/configs/update:
#' Add or remove a custom user configuration.
#' @param user User ID.
#' @param action Action to be performed: ADD or REMOVE a group. Allowed values: ['ADD REMOVE']
#' @param data JSON containing anything useful for the application such as user or default preferences. When removing, only the id will be necessary.
updateConfigs=fetchOpenCGA(object=OpencgaR, category="users", categoryId=user, subcategory="configs",
subcategoryId=NULL, action="update", params=params, httpMethod="POST", as.queryParam=NULL, ...),
#' @section Endpoint /{apiVersion}/users/{user}/filters:
#' Fetch user filters.
#' @param user User ID.
#' @param id Filter id. If provided, it will only fetch the specified filter.
filters=fetchOpenCGA(object=OpencgaR, category="users", categoryId=user, subcategory=NULL, subcategoryId=NULL,
action="filters", params=params, httpMethod="GET", as.queryParam=NULL, ...),
#' @section Endpoint /{apiVersion}/users/{user}/filters/update:
#' Add or remove a custom user filter.
#' @param user User ID.
#' @param action Action to be performed: ADD or REMOVE a group. Allowed values: ['ADD REMOVE']
#' @param data Filter parameters. When removing, only the 'name' of the filter will be necessary.
updateFilters=fetchOpenCGA(object=OpencgaR, category="users", categoryId=user, subcategory="filters",
subcategoryId=NULL, action="update", params=params, httpMethod="POST", as.queryParam=NULL, ...),
#' @section Endpoint /{apiVersion}/users/{user}/filters/{filterId}/update:
#' Update a custom filter.
#' @param user User ID.
#' @param filterId Filter id.
#' @param data Filter parameters.
updateFilter=fetchOpenCGA(object=OpencgaR, category="users", categoryId=user, subcategory="filters",
subcategoryId=filterId, action="update", params=params, httpMethod="POST", as.queryParam=NULL, ...),
#' @section Endpoint /{apiVersion}/users/{user}/password/reset:
#' Reset password.
#' @param user User ID.
resetPassword=fetchOpenCGA(object=OpencgaR, category="users", categoryId=user, subcategory="password",
subcategoryId=NULL, action="reset", params=params, httpMethod="GET", as.queryParam=NULL, ...),
#' @section Endpoint /{apiVersion}/users/{user}/update:
#' Update some user attributes.
#' @param include Fields included in the response, whole JSON path must be provided.
#' @param exclude Fields excluded in the response, whole JSON path must be provided.
#' @param user User ID.
#' @param includeResult Flag indicating to include the created or updated document result in the response.
#' @param data JSON containing the params to be updated.
update=fetchOpenCGA(object=OpencgaR, category="users", categoryId=user, subcategory=NULL, subcategoryId=NULL,
action="update", params=params, httpMethod="POST", as.queryParam=NULL, ...),
)
})
© 2015 - 2025 Weber Informatics LLC | Privacy Policy