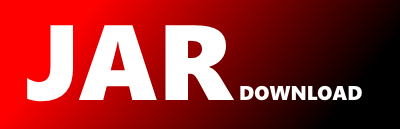
org.opencb.opencga.client.rest.clients.VariantClient Maven / Gradle / Ivy
/*
* Copyright 2015-2024 OpenCB
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.opencb.opencga.client.rest.clients;
import org.opencb.biodata.models.clinical.qc.Signature;
import org.opencb.biodata.models.variant.Variant;
import org.opencb.biodata.models.variant.avro.VariantAnnotation;
import org.opencb.biodata.models.variant.metadata.SampleVariantStats;
import org.opencb.biodata.models.variant.metadata.VariantMetadata;
import org.opencb.biodata.models.variant.metadata.VariantSetStats;
import org.opencb.commons.datastore.core.FacetField;
import org.opencb.commons.datastore.core.ObjectMap;
import org.opencb.commons.datastore.core.QueryResponse;
import org.opencb.opencga.client.config.ClientConfiguration;
import org.opencb.opencga.client.exceptions.ClientException;
import org.opencb.opencga.client.rest.*;
import org.opencb.opencga.core.models.analysis.knockout.KnockoutByGene;
import org.opencb.opencga.core.models.analysis.knockout.KnockoutByIndividual;
import org.opencb.opencga.core.models.clinical.ExomiserWrapperParams;
import org.opencb.opencga.core.models.job.Job;
import org.opencb.opencga.core.models.operations.variant.VariantIndexParams;
import org.opencb.opencga.core.models.operations.variant.VariantStatsExportParams;
import org.opencb.opencga.core.models.variant.CircosAnalysisParams;
import org.opencb.opencga.core.models.variant.CohortVariantStatsAnalysisParams;
import org.opencb.opencga.core.models.variant.FamilyQcAnalysisParams;
import org.opencb.opencga.core.models.variant.GatkWrapperParams;
import org.opencb.opencga.core.models.variant.GenomePlotAnalysisParams;
import org.opencb.opencga.core.models.variant.GwasAnalysisParams;
import org.opencb.opencga.core.models.variant.HRDetectAnalysisParams;
import org.opencb.opencga.core.models.variant.IndividualQcAnalysisParams;
import org.opencb.opencga.core.models.variant.InferredSexAnalysisParams;
import org.opencb.opencga.core.models.variant.KnockoutAnalysisParams;
import org.opencb.opencga.core.models.variant.MendelianErrorAnalysisParams;
import org.opencb.opencga.core.models.variant.MutationalSignatureAnalysisParams;
import org.opencb.opencga.core.models.variant.PlinkWrapperParams;
import org.opencb.opencga.core.models.variant.RelatednessAnalysisParams;
import org.opencb.opencga.core.models.variant.RvtestsWrapperParams;
import org.opencb.opencga.core.models.variant.SampleEligibilityAnalysisParams;
import org.opencb.opencga.core.models.variant.SampleQcAnalysisParams;
import org.opencb.opencga.core.models.variant.SampleVariantFilterParams;
import org.opencb.opencga.core.models.variant.SampleVariantStatsAnalysisParams;
import org.opencb.opencga.core.models.variant.VariantExportParams;
import org.opencb.opencga.core.models.variant.VariantStatsAnalysisParams;
import org.opencb.opencga.core.response.RestResponse;
/*
* WARNING: AUTOGENERATED CODE
*
* This code was generated by a tool.
*
* Manual changes to this file may cause unexpected behavior in your application.
* Manual changes to this file will be overwritten if the code is regenerated.
*/
/**
* This class contains methods for the Variant webservices.
* PATH: analysis/variant
*/
public class VariantClient extends AbstractParentClient {
public VariantClient(String token, ClientConfiguration configuration) {
super(token, configuration);
}
/**
* Calculate and fetch aggregation stats.
* @param params Map containing any of the following optional parameters.
* savedFilter: Use a saved filter at User level.
* region: List of regions, these can be just a single chromosome name or regions in the format chr:start-end, e.g.:
* 2,3:100000-200000.
* type: List of types, accepted values are SNV, MNV, INDEL, SV, COPY_NUMBER, COPY_NUMBER_LOSS, COPY_NUMBER_GAIN, INSERTION,
* DELETION, DUPLICATION, TANDEM_DUPLICATION, BREAKEND, e.g. SNV,INDEL.
* project: Project [organization@]project where project can be either the ID or the alias.
* study: Filter variants from the given studies, these can be either the numeric ID or the alias with the format
* organization@project:study.
* cohort: Select variants with calculated stats for the selected cohorts.
* cohortStatsRef: Reference Allele Frequency: [{study:}]{cohort}[<|>|<=|>=]{number}. e.g. ALL<=0.4.
* cohortStatsAlt: Alternate Allele Frequency: [{study:}]{cohort}[<|>|<=|>=]{number}. e.g. ALL<=0.4.
* cohortStatsMaf: Minor Allele Frequency: [{study:}]{cohort}[<|>|<=|>=]{number}. e.g. ALL<=0.4.
* cohortStatsMgf: Minor Genotype Frequency: [{study:}]{cohort}[<|>|<=|>=]{number}. e.g. ALL<=0.4.
* cohortStatsPass: Filter PASS frequency: [{study:}]{cohort}[<|>|<=|>=]{number}. e.g. ALL>0.8.
* missingAlleles: Number of missing alleles: [{study:}]{cohort}[<|>|<=|>=]{number}.
* missingGenotypes: Number of missing genotypes: [{study:}]{cohort}[<|>|<=|>=]{number}.
* score: Filter by variant score: [{study:}]{score}[<|>|<=|>=]{number}.
* annotationExists: Return only annotated variants.
* gene: List of genes, most gene IDs are accepted (HGNC, Ensembl gene, ...). This is an alias to 'xref' parameter.
* ct: List of SO consequence types, e.g. missense_variant,stop_lost or SO:0001583,SO:0001578. Accepts aliases 'loss_of_function'
* and 'protein_altering'.
* xref: List of any external reference, these can be genes, proteins or variants. Accepted IDs include HGNC, Ensembl genes,
* dbSNP, ClinVar, HPO, Cosmic, HGVS ...
* biotype: List of biotypes, e.g. protein_coding.
* proteinSubstitution: Protein substitution scores include SIFT and PolyPhen. You can query using the score
* {protein_score}[<|>|<=|>=]{number} or the description {protein_score}[~=|=]{description} e.g. polyphen>0.1,sift=tolerant.
* conservation: Filter by conservation score: {conservation_score}[<|>|<=|>=]{number} e.g. phastCons>0.5,phylop<0.1,gerp>0.1.
* populationFrequencyAlt: Alternate Population Frequency: {study}:{population}[<|>|<=|>=]{number}. e.g. 1000G:ALL<0.01.
* populationFrequencyRef: Reference Population Frequency: {study}:{population}[<|>|<=|>=]{number}. e.g. 1000G:ALL<0.01.
* populationFrequencyMaf: Population minor allele frequency: {study}:{population}[<|>|<=|>=]{number}. e.g. 1000G:ALL<0.01.
* transcriptFlag: List of transcript flags. e.g. canonical, CCDS, basic, LRG, MANE Select, MANE Plus Clinical, EGLH_HaemOnc,
* TSO500.
* geneTraitId: List of gene trait association id. e.g. "umls:C0007222" , "OMIM:269600".
* go: List of GO (Gene Ontology) terms. e.g. "GO:0002020".
* expression: List of tissues of interest. e.g. "lung".
* proteinKeyword: List of Uniprot protein variant annotation keywords.
* drug: List of drug names.
* functionalScore: Functional score: {functional_score}[<|>|<=|>=]{number} e.g. cadd_scaled>5.2 , cadd_raw<=0.3.
* clinical: Clinical source: clinvar, cosmic.
* clinicalSignificance: Clinical significance: benign, likely_benign, likely_pathogenic, pathogenic.
* clinicalConfirmedStatus: Clinical confirmed status.
* customAnnotation: Custom annotation: {key}[<|>|<=|>=]{number} or {key}[~=|=]{text}.
* trait: List of traits, based on ClinVar, HPO, COSMIC, i.e.: IDs, histologies, descriptions,...
* field: List of facet fields separated by semicolons, e.g.: studies;type. For nested faceted fields use >>, e.g.:
* chromosome>>type;percentile(gerp).
* @return a RestResponse object.
* @throws ClientException ClientException if there is any server error.
*/
public RestResponse aggregationStats(ObjectMap params) throws ClientException {
params = params != null ? params : new ObjectMap();
return execute("analysis", null, "variant", null, "aggregationStats", params, GET, QueryResponse.class);
}
/**
* Read variant annotations metadata from any saved versions.
* @param params Map containing any of the following optional parameters.
* annotationId: Annotation identifier.
* project: Project [organization@]project where project can be either the ID or the alias.
* @return a RestResponse object.
* @throws ClientException ClientException if there is any server error.
*/
public RestResponse metadataAnnotation(ObjectMap params) throws ClientException {
params = params != null ? params : new ObjectMap();
return execute("analysis", null, "variant/annotation", null, "metadata", params, GET, ObjectMap.class);
}
/**
* Query variant annotations from any saved versions.
* @param params Map containing any of the following optional parameters.
* id: List of variant IDs in the format chrom:start:ref:alt, e.g. 19:7177679:C:T.
* region: List of regions, these can be just a single chromosome name or regions in the format chr:start-end, e.g.:
* 2,3:100000-200000.
* include: Fields included in the response, whole JSON path must be provided.
* exclude: Fields excluded in the response, whole JSON path must be provided.
* limit: Number of results to be returned.
* skip: Number of results to skip.
* annotationId: Annotation identifier.
* @return a RestResponse object.
* @throws ClientException ClientException if there is any server error.
*/
public RestResponse queryAnnotation(ObjectMap params) throws ClientException {
params = params != null ? params : new ObjectMap();
return execute("analysis", null, "variant/annotation", null, "query", params, GET, VariantAnnotation.class);
}
/**
* Generate a Circos plot for a given sample.
* @param data Circos analysis params to customize the plot. These parameters include the title, the plot density (i.e., the number of
* points to display), the general query and the list of tracks. Currently, the supported track types are: COPY-NUMBER, INDEL,
* REARRANGEMENT and SNV. In addition, each track can contain a specific query.
* @param params Map containing any of the following optional parameters.
* study: study.
* @return a RestResponse object.
* @throws ClientException ClientException if there is any server error.
*/
public RestResponse runCircos(CircosAnalysisParams data, ObjectMap params) throws ClientException {
params = params != null ? params : new ObjectMap();
params.put("body", data);
return execute("analysis", null, "variant/circos", null, "run", params, POST, String.class);
}
/**
* Delete cohort variant stats from a cohort.
* @param params Map containing any of the following optional parameters.
* study: study.
* cohort: Cohort ID or UUID.
* @return a RestResponse object.
* @throws ClientException ClientException if there is any server error.
*/
public RestResponse deleteCohortStats(ObjectMap params) throws ClientException {
params = params != null ? params : new ObjectMap();
return execute("analysis", null, "variant/cohort/stats", null, "delete", params, DELETE, SampleVariantStats.class);
}
/**
* Read cohort variant stats from list of cohorts.
* @param cohort Comma separated list of cohort IDs or UUIDs up to a maximum of 100.
* @param params Map containing any of the following optional parameters.
* study: study.
* @return a RestResponse object.
* @throws ClientException ClientException if there is any server error.
*/
public RestResponse infoCohortStats(String cohort, ObjectMap params) throws ClientException {
params = params != null ? params : new ObjectMap();
params.putIfNotNull("cohort", cohort);
return execute("analysis", null, "variant/cohort/stats", null, "info", params, GET, VariantSetStats.class);
}
/**
* Compute cohort variant stats for the selected list of samples.
* @param data Cohort variant stats params.
* @param params Map containing any of the following optional parameters.
* study: Study [[organization@]project:]study where study and project can be either the ID or UUID.
* jobId: Job ID. It must be a unique string within the study. An ID will be autogenerated automatically if not provided.
* jobDescription: Job description.
* jobDependsOn: Comma separated list of existing job IDs the job will depend on.
* jobTags: Job tags.
* jobScheduledStartTime: Time when the job is scheduled to start.
* jobPriority: Priority of the job.
* jobDryRun: Flag indicating that the job will be executed in dry-run mode. In this mode, OpenCGA will validate that all
* parameters and prerequisites are correctly set for successful execution, but the job will not actually run.
* @return a RestResponse object.
* @throws ClientException ClientException if there is any server error.
*/
public RestResponse runCohortStats(CohortVariantStatsAnalysisParams data, ObjectMap params) throws ClientException {
params = params != null ? params : new ObjectMap();
params.put("body", data);
return execute("analysis", null, "variant/cohort/stats", null, "run", params, POST, Job.class);
}
/**
* The Exomiser is a Java program that finds potential disease-causing variants from whole-exome or whole-genome sequencing data.
* @param data Exomiser parameters.
* @param params Map containing any of the following optional parameters.
* study: study.
* jobId: Job ID. It must be a unique string within the study. An ID will be autogenerated automatically if not provided.
* jobDependsOn: Comma separated list of existing job IDs the job will depend on.
* jobDescription: Job description.
* jobTags: Job tags.
* jobScheduledStartTime: Time when the job is scheduled to start.
* jobPriority: Priority of the job.
* jobDryRun: Flag indicating that the job will be executed in dry-run mode. In this mode, OpenCGA will validate that all
* parameters and prerequisites are correctly set for successful execution, but the job will not actually run.
* @return a RestResponse object.
* @throws ClientException ClientException if there is any server error.
*/
public RestResponse runExomiser(ExomiserWrapperParams data, ObjectMap params) throws ClientException {
params = params != null ? params : new ObjectMap();
params.put("body", data);
return execute("analysis", null, "variant/exomiser", null, "run", params, POST, Job.class);
}
/**
* Filter and export variants from the variant storage to a file.
* @param data Variant export params.
* @param params Map containing any of the following optional parameters.
* include: Fields included in the response, whole JSON path must be provided.
* exclude: Fields excluded in the response, whole JSON path must be provided.
* project: Project [organization@]project where project can be either the ID or the alias.
* study: Study [[organization@]project:]study where study and project can be either the ID or UUID.
* jobId: Job ID. It must be a unique string within the study. An ID will be autogenerated automatically if not provided.
* jobDescription: Job description.
* jobDependsOn: Comma separated list of existing job IDs the job will depend on.
* jobTags: Job tags.
* jobScheduledStartTime: Time when the job is scheduled to start.
* jobPriority: Priority of the job.
* jobDryRun: Flag indicating that the job will be executed in dry-run mode. In this mode, OpenCGA will validate that all
* parameters and prerequisites are correctly set for successful execution, but the job will not actually run.
* @return a RestResponse object.
* @throws ClientException ClientException if there is any server error.
*/
public RestResponse runExport(VariantExportParams data, ObjectMap params) throws ClientException {
params = params != null ? params : new ObjectMap();
params.put("body", data);
return execute("analysis", null, "variant/export", null, "run", params, POST, Job.class);
}
/**
* Calculate the possible genotypes for the members of a family.
* @param modeOfInheritance Mode of inheritance.
* @param params Map containing any of the following optional parameters.
* study: Study [[organization@]project:]study where study and project can be either the ID or UUID.
* family: Family id.
* clinicalAnalysis: Clinical analysis id.
* penetrance: Penetrance.
* disorder: Disorder id.
* @return a RestResponse object.
* @throws ClientException ClientException if there is any server error.
*/
public RestResponse genotypesFamily(String modeOfInheritance, ObjectMap params) throws ClientException {
params = params != null ? params : new ObjectMap();
params.putIfNotNull("modeOfInheritance", modeOfInheritance);
return execute("analysis", null, "variant/family", null, "genotypes", params, GET, ObjectMap.class);
}
/**
* Run quality control (QC) for a given family. It computes the relatedness scores among the family members.
* @param data Family QC analysis params. Family ID. Relatedness method, by default 'PLINK/IBD'. Minor allele frequence (MAF) is used
* to filter variants before computing relatedness, e.g.: 1000G:CEU>0.35 or cohort:ALL>0.05.
* @param params Map containing any of the following optional parameters.
* study: Study [[organization@]project:]study where study and project can be either the ID or UUID.
* jobId: Job ID. It must be a unique string within the study. An ID will be autogenerated automatically if not provided.
* jobDescription: Job description.
* jobDependsOn: Comma separated list of existing job IDs the job will depend on.
* jobTags: Job tags.
* jobScheduledStartTime: Time when the job is scheduled to start.
* jobPriority: Priority of the job.
* jobDryRun: Flag indicating that the job will be executed in dry-run mode. In this mode, OpenCGA will validate that all
* parameters and prerequisites are correctly set for successful execution, but the job will not actually run.
* @return a RestResponse object.
* @throws ClientException ClientException if there is any server error.
*/
public RestResponse runFamilyQc(FamilyQcAnalysisParams data, ObjectMap params) throws ClientException {
params = params != null ? params : new ObjectMap();
params.put("body", data);
return execute("analysis", null, "variant/family/qc", null, "run", params, POST, Job.class);
}
/**
* [DEPRECATED] Use operation/variant/delete.
* @param params Map containing any of the following optional parameters.
* jobId: Job ID. It must be a unique string within the study. An ID will be autogenerated automatically if not provided.
* jobDescription: Job description.
* jobDependsOn: Comma separated list of existing job IDs the job will depend on.
* jobTags: Job tags.
* jobScheduledStartTime: Time when the job is scheduled to start.
* jobPriority: Priority of the job.
* jobDryRun: Flag indicating that the job will be executed in dry-run mode. In this mode, OpenCGA will validate that all
* parameters and prerequisites are correctly set for successful execution, but the job will not actually run.
* study: Study [[organization@]project:]study where study and project can be either the ID or UUID.
* file: Files to remove.
* resume: Resume a previously failed indexation.
* @return a RestResponse object.
* @throws ClientException ClientException if there is any server error.
*/
public RestResponse deleteFile(ObjectMap params) throws ClientException {
params = params != null ? params : new ObjectMap();
return execute("analysis", null, "variant/file", null, "delete", params, DELETE, Job.class);
}
/**
* GATK is a Genome Analysis Toolkit for variant discovery in high-throughput sequencing data. Supported Gatk commands: HaplotypeCaller.
* @param data Gatk parameters. Supported Gatk commands: HaplotypeCaller.
* @param params Map containing any of the following optional parameters.
* study: study.
* jobId: Job ID. It must be a unique string within the study. An ID will be autogenerated automatically if not provided.
* jobDescription: Job description.
* jobDependsOn: Comma separated list of existing job IDs the job will depend on.
* jobTags: Job tags.
* jobScheduledStartTime: Time when the job is scheduled to start.
* jobPriority: Priority of the job.
* jobDryRun: Flag indicating that the job will be executed in dry-run mode. In this mode, OpenCGA will validate that all
* parameters and prerequisites are correctly set for successful execution, but the job will not actually run.
* @return a RestResponse object.
* @throws ClientException ClientException if there is any server error.
*/
public RestResponse runGatk(GatkWrapperParams data, ObjectMap params) throws ClientException {
params = params != null ? params : new ObjectMap();
params.put("body", data);
return execute("analysis", null, "variant/gatk", null, "run", params, POST, Job.class);
}
/**
* Generate a genome plot for a given sample.
* @param data Genome plot analysis params to customize the plot. The configuration file includes the title, the plot density (i.e.,
* the number of points to display), the general query and the list of tracks. Currently, the supported track types are:
* COPY-NUMBER, INDEL, REARRANGEMENT and SNV. In addition, each track can contain a specific query.
* @param params Map containing any of the following optional parameters.
* study: Study [[organization@]project:]study where study and project can be either the ID or UUID.
* jobId: Job ID. It must be a unique string within the study. An ID will be autogenerated automatically if not provided.
* jobDescription: Job description.
* jobDependsOn: Comma separated list of existing job IDs the job will depend on.
* jobTags: Job tags.
* jobScheduledStartTime: Time when the job is scheduled to start.
* jobPriority: Priority of the job.
* jobDryRun: Flag indicating that the job will be executed in dry-run mode. In this mode, OpenCGA will validate that all
* parameters and prerequisites are correctly set for successful execution, but the job will not actually run.
* @return a RestResponse object.
* @throws ClientException ClientException if there is any server error.
*/
public RestResponse runGenomePlot(GenomePlotAnalysisParams data, ObjectMap params) throws ClientException {
params = params != null ? params : new ObjectMap();
params.put("body", data);
return execute("analysis", null, "variant/genomePlot", null, "run", params, POST, Job.class);
}
/**
* Run a Genome Wide Association Study between two cohorts.
* @param data Gwas analysis params.
* @param params Map containing any of the following optional parameters.
* study: Study [[organization@]project:]study where study and project can be either the ID or UUID.
* jobId: Job ID. It must be a unique string within the study. An ID will be autogenerated automatically if not provided.
* jobDescription: Job description.
* jobDependsOn: Comma separated list of existing job IDs the job will depend on.
* jobTags: Job tags.
* jobScheduledStartTime: Time when the job is scheduled to start.
* jobPriority: Priority of the job.
* jobDryRun: Flag indicating that the job will be executed in dry-run mode. In this mode, OpenCGA will validate that all
* parameters and prerequisites are correctly set for successful execution, but the job will not actually run.
* @return a RestResponse object.
* @throws ClientException ClientException if there is any server error.
*/
public RestResponse runGwas(GwasAnalysisParams data, ObjectMap params) throws ClientException {
params = params != null ? params : new ObjectMap();
params.put("body", data);
return execute("analysis", null, "variant/gwas", null, "run", params, POST, Job.class);
}
/**
* Run HRDetect analysis for a given somatic sample.
* @param data HRDetect analysis parameters.
* @param params Map containing any of the following optional parameters.
* study: Study [[organization@]project:]study where study and project can be either the ID or UUID.
* jobId: Job ID. It must be a unique string within the study. An ID will be autogenerated automatically if not provided.
* jobDescription: Job description.
* jobDependsOn: Comma separated list of existing job IDs the job will depend on.
* jobTags: Job tags.
* jobScheduledStartTime: Time when the job is scheduled to start.
* jobPriority: Priority of the job.
* jobDryRun: Flag indicating that the job will be executed in dry-run mode. In this mode, OpenCGA will validate that all
* parameters and prerequisites are correctly set for successful execution, but the job will not actually run.
* @return a RestResponse object.
* @throws ClientException ClientException if there is any server error.
*/
public RestResponse runHrDetect(HRDetectAnalysisParams data, ObjectMap params) throws ClientException {
params = params != null ? params : new ObjectMap();
params.put("body", data);
return execute("analysis", null, "variant/hrDetect", null, "run", params, POST, Job.class);
}
/**
* [DEPRECATED] Use operation/variant/index.
* @param data Variant index params.
* @param params Map containing any of the following optional parameters.
* study: Study [[organization@]project:]study where study and project can be either the ID or UUID.
* jobId: Job ID. It must be a unique string within the study. An ID will be autogenerated automatically if not provided.
* jobDependsOn: Comma separated list of existing job IDs the job will depend on.
* jobDescription: Job description.
* jobTags: Job tags.
* jobScheduledStartTime: Time when the job is scheduled to start.
* jobPriority: Priority of the job.
* jobDryRun: Flag indicating that the job will be executed in dry-run mode. In this mode, OpenCGA will validate that all
* parameters and prerequisites are correctly set for successful execution, but the job will not actually run.
* @return a RestResponse object.
* @throws ClientException ClientException if there is any server error.
*/
public RestResponse runIndex(VariantIndexParams data, ObjectMap params) throws ClientException {
params = params != null ? params : new ObjectMap();
params.put("body", data);
return execute("analysis", null, "variant/index", null, "run", params, POST, Job.class);
}
/**
* Run quality control (QC) for a given individual. It includes inferred sex and mendelian errors (UDP).
* @param data Individual QC analysis params.
* @param params Map containing any of the following optional parameters.
* study: Study [[organization@]project:]study where study and project can be either the ID or UUID.
* jobId: Job ID. It must be a unique string within the study. An ID will be autogenerated automatically if not provided.
* jobDescription: Job description.
* jobDependsOn: Comma separated list of existing job IDs the job will depend on.
* jobTags: Job tags.
* jobScheduledStartTime: Time when the job is scheduled to start.
* jobPriority: Priority of the job.
* jobDryRun: Flag indicating that the job will be executed in dry-run mode. In this mode, OpenCGA will validate that all
* parameters and prerequisites are correctly set for successful execution, but the job will not actually run.
* @return a RestResponse object.
* @throws ClientException ClientException if there is any server error.
*/
public RestResponse runIndividualQc(IndividualQcAnalysisParams data, ObjectMap params) throws ClientException {
params = params != null ? params : new ObjectMap();
params.put("body", data);
return execute("analysis", null, "variant/individual/qc", null, "run", params, POST, Job.class);
}
/**
* Infer sex from chromosome mean coverages.
* @param data Inferred sex analysis params.
* @param params Map containing any of the following optional parameters.
* study: Study [[organization@]project:]study where study and project can be either the ID or UUID.
* jobId: Job ID. It must be a unique string within the study. An ID will be autogenerated automatically if not provided.
* jobDescription: Job description.
* jobDependsOn: Comma separated list of existing job IDs the job will depend on.
* jobTags: Job tags.
* jobScheduledStartTime: Time when the job is scheduled to start.
* jobPriority: Priority of the job.
* jobDryRun: Flag indicating that the job will be executed in dry-run mode. In this mode, OpenCGA will validate that all
* parameters and prerequisites are correctly set for successful execution, but the job will not actually run.
* @return a RestResponse object.
* @throws ClientException ClientException if there is any server error.
*/
public RestResponse runInferredSex(InferredSexAnalysisParams data, ObjectMap params) throws ClientException {
params = params != null ? params : new ObjectMap();
params.put("body", data);
return execute("analysis", null, "variant/inferredSex", null, "run", params, POST, Job.class);
}
/**
* Fetch values from KnockoutAnalysis result, by genes.
* @param params Map containing any of the following optional parameters.
* limit: Number of results to be returned.
* skip: Number of results to skip.
* study: study.
* job: Job ID or UUID.
* @return a RestResponse object.
* @throws ClientException ClientException if there is any server error.
*/
public RestResponse queryKnockoutGene(ObjectMap params) throws ClientException {
params = params != null ? params : new ObjectMap();
return execute("analysis", null, "variant/knockout/gene", null, "query", params, GET, KnockoutByGene.class);
}
/**
* Fetch values from KnockoutAnalysis result, by individuals.
* @param params Map containing any of the following optional parameters.
* limit: Number of results to be returned.
* skip: Number of results to skip.
* study: study.
* job: Job ID or UUID.
* @return a RestResponse object.
* @throws ClientException ClientException if there is any server error.
*/
public RestResponse queryKnockoutIndividual(ObjectMap params) throws ClientException {
params = params != null ? params : new ObjectMap();
return execute("analysis", null, "variant/knockout/individual", null, "query", params, GET, KnockoutByIndividual.class);
}
/**
* Obtains the list of knocked out genes for each sample.
* @param data Gene knockout analysis params.
* @param params Map containing any of the following optional parameters.
* study: study.
* jobId: Job ID. It must be a unique string within the study. An ID will be autogenerated automatically if not provided.
* jobDescription: Job description.
* jobDependsOn: Comma separated list of existing job IDs the job will depend on.
* jobTags: Job tags.
* jobScheduledStartTime: Time when the job is scheduled to start.
* jobPriority: Priority of the job.
* jobDryRun: Flag indicating that the job will be executed in dry-run mode. In this mode, OpenCGA will validate that all
* parameters and prerequisites are correctly set for successful execution, but the job will not actually run.
* @return a RestResponse object.
* @throws ClientException ClientException if there is any server error.
*/
public RestResponse runKnockout(KnockoutAnalysisParams data, ObjectMap params) throws ClientException {
params = params != null ? params : new ObjectMap();
params.put("body", data);
return execute("analysis", null, "variant/knockout", null, "run", params, POST, Job.class);
}
/**
* Run mendelian error analysis to infer uniparental disomy regions.
* @param data Mendelian error analysis params.
* @param params Map containing any of the following optional parameters.
* study: Study [[organization@]project:]study where study and project can be either the ID or UUID.
* jobId: Job ID. It must be a unique string within the study. An ID will be autogenerated automatically if not provided.
* jobDescription: Job description.
* jobDependsOn: Comma separated list of existing job IDs the job will depend on.
* jobTags: Job tags.
* jobScheduledStartTime: Time when the job is scheduled to start.
* jobPriority: Priority of the job.
* jobDryRun: Flag indicating that the job will be executed in dry-run mode. In this mode, OpenCGA will validate that all
* parameters and prerequisites are correctly set for successful execution, but the job will not actually run.
* @return a RestResponse object.
* @throws ClientException ClientException if there is any server error.
*/
public RestResponse runMendelianError(MendelianErrorAnalysisParams data, ObjectMap params) throws ClientException {
params = params != null ? params : new ObjectMap();
params.put("body", data);
return execute("analysis", null, "variant/mendelianError", null, "run", params, POST, Job.class);
}
/**
* .
* @param params Map containing any of the following optional parameters.
* project: Project [organization@]project where project can be either the ID or the alias.
* study: Filter variants from the given studies, these can be either the numeric ID or the alias with the format
* organization@project:study.
* file: Filter variants from the files specified. This will set includeFile parameter when not provided.
* sample: Filter variants by sample genotype. This will automatically set 'includeSample' parameter when not provided. This
* filter accepts multiple 3 forms: 1) List of samples: Samples that contain the main variant. Accepts AND (;) and OR (,)
* operators. e.g. HG0097,HG0098 . 2) List of samples with genotypes: {sample}:{gt1},{gt2}. Accepts AND (;) and OR (,)
* operators. e.g. HG0097:0/0;HG0098:0/1,1/1 . Unphased genotypes (e.g. 0/1, 1/1) will also include phased genotypes (e.g.
* 0|1, 1|0, 1|1), but not vice versa. When filtering by multi-allelic genotypes, any secondary allele will match,
* regardless of its position e.g. 1/2 will match with genotypes 1/2, 1/3, 1/4, .... Genotype aliases accepted: HOM_REF,
* HOM_ALT, HET, HET_REF, HET_ALT, HET_MISS and MISS e.g. HG0097:HOM_REF;HG0098:HET_REF,HOM_ALT . 3) Sample with
* segregation mode: {sample}:{segregation}. Only one sample accepted.Accepted segregation modes: [ autosomalDominant,
* autosomalRecessive, XLinkedDominant, XLinkedRecessive, YLinked, mitochondrial, deNovo, deNovoStrict, mendelianError,
* compoundHeterozygous ]. Value is case insensitive. e.g. HG0097:DeNovo Sample must have parents defined and indexed. .
* includeStudy: List of studies to include in the result. Accepts 'all' and 'none'.
* includeFile: List of files to be returned. Accepts 'all' and 'none'. If undefined, automatically includes files used for
* filtering. If none, no file is included.
* includeSample: List of samples to be included in the result. Accepts 'all' and 'none'. If undefined, automatically includes
* samples used for filtering. If none, no sample is included.
* include: Fields included in the response, whole JSON path must be provided.
* exclude: Fields excluded in the response, whole JSON path must be provided.
* @return a RestResponse object.
* @throws ClientException ClientException if there is any server error.
*/
public RestResponse metadata(ObjectMap params) throws ClientException {
params = params != null ? params : new ObjectMap();
return execute("analysis", null, "variant", null, "metadata", params, GET, VariantMetadata.class);
}
/**
* Run mutational signature analysis for a given sample. Use context index.
* @param params Map containing any of the following optional parameters.
* study: Filter variants from the given studies, these can be either the numeric ID or the alias with the format
* organization@project:study.
* sample: Sample name.
* type: Variant type. Valid values: SNV, SV.
* ct: List of SO consequence types, e.g. missense_variant,stop_lost or SO:0001583,SO:0001578. Accepts aliases 'loss_of_function'
* and 'protein_altering'.
* biotype: List of biotypes, e.g. protein_coding.
* fileData: Filter by file data (i.e. FILTER, QUAL and INFO columns from VCF file). [{file}:]{key}{op}{value}[,;]* . If no file
* is specified, will use all files from "file" filter. e.g. AN>200 or file_1.vcf:AN>200;file_2.vcf:AN<10 . Many fields can
* be combined. e.g. file_1.vcf:AN>200;DB=true;file_2.vcf:AN<10,FILTER=PASS,LowDP.
* filter: Specify the FILTER for any of the files. If 'file' filter is provided, will match the file and the filter. e.g.:
* PASS,LowGQX.
* qual: Specify the QUAL for any of the files. If 'file' filter is provided, will match the file and the qual. e.g.: >123.4.
* region: List of regions, these can be just a single chromosome name or regions in the format chr:start-end, e.g.:
* 2,3:100000-200000.
* gene: List of genes, most gene IDs are accepted (HGNC, Ensembl gene, ...). This is an alias to 'xref' parameter.
* panel: Filter by genes from the given disease panel.
* panelModeOfInheritance: Filter genes from specific panels that match certain mode of inheritance. Accepted values : [
* autosomalDominant, autosomalRecessive, XLinkedDominant, XLinkedRecessive, YLinked, mitochondrial, deNovo, mendelianError,
* compoundHeterozygous ].
* panelConfidence: Filter genes from specific panels that match certain confidence. Accepted values : [ high, medium, low,
* rejected ].
* panelFeatureType: Filter elements from specific panels by type. Accepted values : [ gene, region, str, variant ].
* panelRoleInCancer: Filter genes from specific panels that match certain role in cancer. Accepted values : [ both, oncogene,
* tumorSuppressorGene, fusion ].
* panelIntersection: Intersect panel genes and regions with given genes and regions from que input query. This will prevent
* returning variants from regions out of the panel.
* msId: Signature ID.
* msDescription: Signature description.
* @return a RestResponse object.
* @throws ClientException ClientException if there is any server error.
*/
public RestResponse queryMutationalSignature(ObjectMap params) throws ClientException {
params = params != null ? params : new ObjectMap();
return execute("analysis", null, "variant/mutationalSignature", null, "query", params, GET, Signature.class);
}
/**
* Run mutational signature analysis for a given sample.
* @param data Mutational signature analysis parameters to index the genome context for that sample, and to compute both catalogue
* counts and signature fitting. In order to skip one of them, , use the following keywords: , catalogue, fitting.
* @param params Map containing any of the following optional parameters.
* study: Study [[organization@]project:]study where study and project can be either the ID or UUID.
* jobId: Job ID. It must be a unique string within the study. An ID will be autogenerated automatically if not provided.
* jobDescription: Job description.
* jobDependsOn: Comma separated list of existing job IDs the job will depend on.
* jobTags: Job tags.
* jobScheduledStartTime: Time when the job is scheduled to start.
* jobPriority: Priority of the job.
* jobDryRun: Flag indicating that the job will be executed in dry-run mode. In this mode, OpenCGA will validate that all
* parameters and prerequisites are correctly set for successful execution, but the job will not actually run.
* @return a RestResponse object.
* @throws ClientException ClientException if there is any server error.
*/
public RestResponse runMutationalSignature(MutationalSignatureAnalysisParams data, ObjectMap params) throws ClientException {
params = params != null ? params : new ObjectMap();
params.put("body", data);
return execute("analysis", null, "variant/mutationalSignature", null, "run", params, POST, Job.class);
}
/**
* Plink is a whole genome association analysis toolset, designed to perform a range of basic, large-scale analyses.
* @param data Plink params.
* @param params Map containing any of the following optional parameters.
* study: study.
* jobId: Job ID. It must be a unique string within the study. An ID will be autogenerated automatically if not provided.
* jobDescription: Job description.
* jobDependsOn: Comma separated list of existing job IDs the job will depend on.
* jobTags: Job tags.
* jobScheduledStartTime: Time when the job is scheduled to start.
* jobPriority: Priority of the job.
* jobDryRun: Flag indicating that the job will be executed in dry-run mode. In this mode, OpenCGA will validate that all
* parameters and prerequisites are correctly set for successful execution, but the job will not actually run.
* @return a RestResponse object.
* @throws ClientException ClientException if there is any server error.
*/
public RestResponse runPlink(PlinkWrapperParams data, ObjectMap params) throws ClientException {
params = params != null ? params : new ObjectMap();
params.put("body", data);
return execute("analysis", null, "variant/plink", null, "run", params, POST, Job.class);
}
/**
* Filter and fetch variants from indexed VCF files in the variant storage.
* @param params Map containing any of the following optional parameters.
* include: Fields included in the response, whole JSON path must be provided.
* exclude: Fields excluded in the response, whole JSON path must be provided.
* limit: Number of results to be returned.
* skip: Number of results to skip.
* count: Get the total number of results matching the query. Deactivated by default.
* sort: Sort the results.
* summary: Fast fetch of main variant parameters.
* approximateCount: Get an approximate count, instead of an exact total count. Reduces execution time.
* approximateCountSamplingSize: Sampling size to get the approximate count. Larger values increase accuracy but also increase
* execution time.
* savedFilter: Use a saved filter at User level.
* id: List of variant IDs in the format chrom:start:ref:alt, e.g. 19:7177679:C:T.
* region: List of regions, these can be just a single chromosome name or regions in the format chr:start-end, e.g.:
* 2,3:100000-200000.
* type: List of types, accepted values are SNV, MNV, INDEL, SV, COPY_NUMBER, COPY_NUMBER_LOSS, COPY_NUMBER_GAIN, INSERTION,
* DELETION, DUPLICATION, TANDEM_DUPLICATION, BREAKEND, e.g. SNV,INDEL.
* reference: Reference allele.
* alternate: Main alternate allele.
* project: Project [organization@]project where project can be either the ID or the alias.
* study: Filter variants from the given studies, these can be either the numeric ID or the alias with the format
* organization@project:study.
* file: Filter variants from the files specified. This will set includeFile parameter when not provided.
* filter: Specify the FILTER for any of the files. If 'file' filter is provided, will match the file and the filter. e.g.:
* PASS,LowGQX.
* qual: Specify the QUAL for any of the files. If 'file' filter is provided, will match the file and the qual. e.g.: >123.4.
* fileData: Filter by file data (i.e. FILTER, QUAL and INFO columns from VCF file). [{file}:]{key}{op}{value}[,;]* . If no file
* is specified, will use all files from "file" filter. e.g. AN>200 or file_1.vcf:AN>200;file_2.vcf:AN<10 . Many fields can
* be combined. e.g. file_1.vcf:AN>200;DB=true;file_2.vcf:AN<10,FILTER=PASS,LowDP.
* sample: Filter variants by sample genotype. This will automatically set 'includeSample' parameter when not provided. This
* filter accepts multiple 3 forms: 1) List of samples: Samples that contain the main variant. Accepts AND (;) and OR (,)
* operators. e.g. HG0097,HG0098 . 2) List of samples with genotypes: {sample}:{gt1},{gt2}. Accepts AND (;) and OR (,)
* operators. e.g. HG0097:0/0;HG0098:0/1,1/1 . Unphased genotypes (e.g. 0/1, 1/1) will also include phased genotypes (e.g.
* 0|1, 1|0, 1|1), but not vice versa. When filtering by multi-allelic genotypes, any secondary allele will match,
* regardless of its position e.g. 1/2 will match with genotypes 1/2, 1/3, 1/4, .... Genotype aliases accepted: HOM_REF,
* HOM_ALT, HET, HET_REF, HET_ALT, HET_MISS and MISS e.g. HG0097:HOM_REF;HG0098:HET_REF,HOM_ALT . 3) Sample with
* segregation mode: {sample}:{segregation}. Only one sample accepted.Accepted segregation modes: [ autosomalDominant,
* autosomalRecessive, XLinkedDominant, XLinkedRecessive, YLinked, mitochondrial, deNovo, deNovoStrict, mendelianError,
* compoundHeterozygous ]. Value is case insensitive. e.g. HG0097:DeNovo Sample must have parents defined and indexed. .
* genotype: Samples with a specific genotype: {samp_1}:{gt_1}(,{gt_n})*(;{samp_n}:{gt_1}(,{gt_n})*)* e.g.
* HG0097:0/0;HG0098:0/1,1/1. Unphased genotypes (e.g. 0/1, 1/1) will also include phased genotypes (e.g. 0|1, 1|0, 1|1),
* but not vice versa. When filtering by multi-allelic genotypes, any secondary allele will match, regardless of its
* position e.g. 1/2 will match with genotypes 1/2, 1/3, 1/4, .... Genotype aliases accepted: HOM_REF, HOM_ALT, HET,
* HET_REF, HET_ALT, HET_MISS and MISS e.g. HG0097:HOM_REF;HG0098:HET_REF,HOM_ALT. This will automatically set
* 'includeSample' parameter when not provided.
* sampleData: Filter by any SampleData field from samples. [{sample}:]{key}{op}{value}[,;]* . If no sample is specified, will
* use all samples from "sample" or "genotype" filter. e.g. DP>200 or HG0097:DP>200,HG0098:DP<10 . Many FORMAT fields can be
* combined. e.g. HG0097:DP>200;GT=1/1,0/1,HG0098:DP<10.
* sampleAnnotation: Selects some samples using metadata information from Catalog. e.g.
* age>20;phenotype=hpo:123,hpo:456;name=smith.
* sampleMetadata: Return the samples metadata group by study. Sample names will appear in the same order as their corresponding
* genotypes.
* unknownGenotype: Returned genotype for unknown genotypes. Common values: [0/0, 0|0, ./.].
* sampleLimit: Limit the number of samples to be included in the result.
* sampleSkip: Skip some samples from the result. Useful for sample pagination.
* cohort: Select variants with calculated stats for the selected cohorts.
* cohortStatsRef: Reference Allele Frequency: [{study:}]{cohort}[<|>|<=|>=]{number}. e.g. ALL<=0.4.
* cohortStatsAlt: Alternate Allele Frequency: [{study:}]{cohort}[<|>|<=|>=]{number}. e.g. ALL<=0.4.
* cohortStatsMaf: Minor Allele Frequency: [{study:}]{cohort}[<|>|<=|>=]{number}. e.g. ALL<=0.4.
* cohortStatsMgf: Minor Genotype Frequency: [{study:}]{cohort}[<|>|<=|>=]{number}. e.g. ALL<=0.4.
* cohortStatsPass: Filter PASS frequency: [{study:}]{cohort}[<|>|<=|>=]{number}. e.g. ALL>0.8.
* missingAlleles: Number of missing alleles: [{study:}]{cohort}[<|>|<=|>=]{number}.
* missingGenotypes: Number of missing genotypes: [{study:}]{cohort}[<|>|<=|>=]{number}.
* score: Filter by variant score: [{study:}]{score}[<|>|<=|>=]{number}.
* family: Filter variants where any of the samples from the given family contains the variant (HET or HOM_ALT).
* familyDisorder: Specify the disorder to use for the family segregation.
* familySegregation: Filter by segregation mode from a given family. Accepted values: [ autosomalDominant, autosomalRecessive,
* XLinkedDominant, XLinkedRecessive, YLinked, mitochondrial, deNovo, deNovoStrict, mendelianError, compoundHeterozygous ].
* familyMembers: Sub set of the members of a given family.
* familyProband: Specify the proband child to use for the family segregation.
* includeStudy: List of studies to include in the result. Accepts 'all' and 'none'.
* includeFile: List of files to be returned. Accepts 'all' and 'none'. If undefined, automatically includes files used for
* filtering. If none, no file is included.
* includeSample: List of samples to be included in the result. Accepts 'all' and 'none'. If undefined, automatically includes
* samples used for filtering. If none, no sample is included.
* includeSampleData: List of Sample Data keys (i.e. FORMAT column from VCF file) from Sample Data to include in the output. e.g:
* DP,AD. Accepts 'all' and 'none'.
* includeGenotype: Include genotypes, apart of other formats defined with includeFormat.
* includeSampleId: Include sampleId on each result.
* annotationExists: Return only annotated variants.
* gene: List of genes, most gene IDs are accepted (HGNC, Ensembl gene, ...). This is an alias to 'xref' parameter.
* ct: List of SO consequence types, e.g. missense_variant,stop_lost or SO:0001583,SO:0001578. Accepts aliases 'loss_of_function'
* and 'protein_altering'.
* xref: List of any external reference, these can be genes, proteins or variants. Accepted IDs include HGNC, Ensembl genes,
* dbSNP, ClinVar, HPO, Cosmic, HGVS ...
* biotype: List of biotypes, e.g. protein_coding.
* proteinSubstitution: Protein substitution scores include SIFT and PolyPhen. You can query using the score
* {protein_score}[<|>|<=|>=]{number} or the description {protein_score}[~=|=]{description} e.g. polyphen>0.1,sift=tolerant.
* conservation: Filter by conservation score: {conservation_score}[<|>|<=|>=]{number} e.g. phastCons>0.5,phylop<0.1,gerp>0.1.
* populationFrequencyAlt: Alternate Population Frequency: {study}:{population}[<|>|<=|>=]{number}. e.g. 1000G:ALL<0.01.
* populationFrequencyRef: Reference Population Frequency: {study}:{population}[<|>|<=|>=]{number}. e.g. 1000G:ALL<0.01.
* populationFrequencyMaf: Population minor allele frequency: {study}:{population}[<|>|<=|>=]{number}. e.g. 1000G:ALL<0.01.
* transcriptFlag: List of transcript flags. e.g. canonical, CCDS, basic, LRG, MANE Select, MANE Plus Clinical, EGLH_HaemOnc,
* TSO500.
* geneTraitId: List of gene trait association id. e.g. "umls:C0007222" , "OMIM:269600".
* go: List of GO (Gene Ontology) terms. e.g. "GO:0002020".
* expression: List of tissues of interest. e.g. "lung".
* proteinKeyword: List of Uniprot protein variant annotation keywords.
* drug: List of drug names.
* functionalScore: Functional score: {functional_score}[<|>|<=|>=]{number} e.g. cadd_scaled>5.2 , cadd_raw<=0.3.
* clinical: Clinical source: clinvar, cosmic.
* clinicalSignificance: Clinical significance: benign, likely_benign, likely_pathogenic, pathogenic.
* clinicalConfirmedStatus: Clinical confirmed status.
* customAnnotation: Custom annotation: {key}[<|>|<=|>=]{number} or {key}[~=|=]{text}.
* panel: Filter by genes from the given disease panel.
* panelModeOfInheritance: Filter genes from specific panels that match certain mode of inheritance. Accepted values : [
* autosomalDominant, autosomalRecessive, XLinkedDominant, XLinkedRecessive, YLinked, mitochondrial, deNovo, mendelianError,
* compoundHeterozygous ].
* panelConfidence: Filter genes from specific panels that match certain confidence. Accepted values : [ high, medium, low,
* rejected ].
* panelRoleInCancer: Filter genes from specific panels that match certain role in cancer. Accepted values : [ both, oncogene,
* tumorSuppressorGene, fusion ].
* panelFeatureType: Filter elements from specific panels by type. Accepted values : [ gene, region, str, variant ].
* panelIntersection: Intersect panel genes and regions with given genes and regions from que input query. This will prevent
* returning variants from regions out of the panel.
* trait: List of traits, based on ClinVar, HPO, COSMIC, i.e.: IDs, histologies, descriptions,...
* @return a RestResponse object.
* @throws ClientException ClientException if there is any server error.
*/
public RestResponse query(ObjectMap params) throws ClientException {
params = params != null ? params : new ObjectMap();
return execute("analysis", null, "variant", null, "query", params, GET, Variant.class);
}
/**
* Compute a score to quantify relatedness between samples.
* @param data Relatedness analysis params.
* @param params Map containing any of the following optional parameters.
* study: Study [[organization@]project:]study where study and project can be either the ID or UUID.
* jobId: Job ID. It must be a unique string within the study. An ID will be autogenerated automatically if not provided.
* jobDescription: Job description.
* jobDependsOn: Comma separated list of existing job IDs the job will depend on.
* jobTags: Job tags.
* jobScheduledStartTime: Time when the job is scheduled to start.
* jobPriority: Priority of the job.
* jobDryRun: Flag indicating that the job will be executed in dry-run mode. In this mode, OpenCGA will validate that all
* parameters and prerequisites are correctly set for successful execution, but the job will not actually run.
* @return a RestResponse object.
* @throws ClientException ClientException if there is any server error.
*/
public RestResponse runRelatedness(RelatednessAnalysisParams data, ObjectMap params) throws ClientException {
params = params != null ? params : new ObjectMap();
params.put("body", data);
return execute("analysis", null, "variant/relatedness", null, "run", params, POST, Job.class);
}
/**
* Rvtests is a flexible software package for genetic association studies. Supported RvTests commands: rvtest, vcf2kinship.
* @param data RvTests parameters. Supported RvTests commands: rvtest, vcf2kinship.
* @param params Map containing any of the following optional parameters.
* study: study.
* jobId: Job ID. It must be a unique string within the study. An ID will be autogenerated automatically if not provided.
* jobDescription: Job description.
* jobDependsOn: Comma separated list of existing job IDs the job will depend on.
* jobTags: Job tags.
* jobScheduledStartTime: Time when the job is scheduled to start.
* jobPriority: Priority of the job.
* jobDryRun: Flag indicating that the job will be executed in dry-run mode. In this mode, OpenCGA will validate that all
* parameters and prerequisites are correctly set for successful execution, but the job will not actually run.
* @return a RestResponse object.
* @throws ClientException ClientException if there is any server error.
*/
public RestResponse runRvtests(RvtestsWrapperParams data, ObjectMap params) throws ClientException {
params = params != null ? params : new ObjectMap();
params.put("body", data);
return execute("analysis", null, "variant/rvtests", null, "run", params, POST, Job.class);
}
/**
* Calculate and fetch sample aggregation stats.
* @param params Map containing any of the following optional parameters.
* savedFilter: Use a saved filter at User level.
* region: List of regions, these can be just a single chromosome name or regions in the format chr:start-end, e.g.:
* 2,3:100000-200000.
* type: List of types, accepted values are SNV, MNV, INDEL, SV, COPY_NUMBER, COPY_NUMBER_LOSS, COPY_NUMBER_GAIN, INSERTION,
* DELETION, DUPLICATION, TANDEM_DUPLICATION, BREAKEND, e.g. SNV,INDEL.
* project: Project [organization@]project where project can be either the ID or the alias.
* study: Filter variants from the given studies, these can be either the numeric ID or the alias with the format
* organization@project:study.
* file: Filter variants from the files specified. This will set includeFile parameter when not provided.
* filter: Specify the FILTER for any of the files. If 'file' filter is provided, will match the file and the filter. e.g.:
* PASS,LowGQX.
* sample: Filter variants by sample genotype. This will automatically set 'includeSample' parameter when not provided. This
* filter accepts multiple 3 forms: 1) List of samples: Samples that contain the main variant. Accepts AND (;) and OR (,)
* operators. e.g. HG0097,HG0098 . 2) List of samples with genotypes: {sample}:{gt1},{gt2}. Accepts AND (;) and OR (,)
* operators. e.g. HG0097:0/0;HG0098:0/1,1/1 . Unphased genotypes (e.g. 0/1, 1/1) will also include phased genotypes (e.g.
* 0|1, 1|0, 1|1), but not vice versa. When filtering by multi-allelic genotypes, any secondary allele will match,
* regardless of its position e.g. 1/2 will match with genotypes 1/2, 1/3, 1/4, .... Genotype aliases accepted: HOM_REF,
* HOM_ALT, HET, HET_REF, HET_ALT, HET_MISS and MISS e.g. HG0097:HOM_REF;HG0098:HET_REF,HOM_ALT . 3) Sample with
* segregation mode: {sample}:{segregation}. Only one sample accepted.Accepted segregation modes: [ autosomalDominant,
* autosomalRecessive, XLinkedDominant, XLinkedRecessive, YLinked, mitochondrial, deNovo, deNovoStrict, mendelianError,
* compoundHeterozygous ]. Value is case insensitive. e.g. HG0097:DeNovo Sample must have parents defined and indexed. .
* genotype: Samples with a specific genotype: {samp_1}:{gt_1}(,{gt_n})*(;{samp_n}:{gt_1}(,{gt_n})*)* e.g.
* HG0097:0/0;HG0098:0/1,1/1. Unphased genotypes (e.g. 0/1, 1/1) will also include phased genotypes (e.g. 0|1, 1|0, 1|1),
* but not vice versa. When filtering by multi-allelic genotypes, any secondary allele will match, regardless of its
* position e.g. 1/2 will match with genotypes 1/2, 1/3, 1/4, .... Genotype aliases accepted: HOM_REF, HOM_ALT, HET,
* HET_REF, HET_ALT, HET_MISS and MISS e.g. HG0097:HOM_REF;HG0098:HET_REF,HOM_ALT. This will automatically set
* 'includeSample' parameter when not provided.
* sampleAnnotation: Selects some samples using metadata information from Catalog. e.g.
* age>20;phenotype=hpo:123,hpo:456;name=smith.
* family: Filter variants where any of the samples from the given family contains the variant (HET or HOM_ALT).
* familyDisorder: Specify the disorder to use for the family segregation.
* familySegregation: Filter by segregation mode from a given family. Accepted values: [ autosomalDominant, autosomalRecessive,
* XLinkedDominant, XLinkedRecessive, YLinked, mitochondrial, deNovo, deNovoStrict, mendelianError, compoundHeterozygous ].
* familyMembers: Sub set of the members of a given family.
* familyProband: Specify the proband child to use for the family segregation.
* ct: List of SO consequence types, e.g. missense_variant,stop_lost or SO:0001583,SO:0001578. Accepts aliases 'loss_of_function'
* and 'protein_altering'.
* biotype: List of biotypes, e.g. protein_coding.
* populationFrequencyAlt: Alternate Population Frequency: {study}:{population}[<|>|<=|>=]{number}. e.g. 1000G:ALL<0.01.
* clinical: Clinical source: clinvar, cosmic.
* clinicalSignificance: Clinical significance: benign, likely_benign, likely_pathogenic, pathogenic.
* clinicalConfirmedStatus: Clinical confirmed status.
* field: List of facet fields separated by semicolons, e.g.: studies;type. For nested faceted fields use >>, e.g.:
* chromosome>>type . Accepted values: chromosome, type, genotype, consequenceType, biotype, clinicalSignificance, dp, qual,
* filter.
* @return a RestResponse object.
* @throws ClientException ClientException if there is any server error.
*/
public RestResponse aggregationStatsSample(ObjectMap params) throws ClientException {
params = params != null ? params : new ObjectMap();
return execute("analysis", null, "variant/sample", null, "aggregationStats", params, GET, FacetField.class);
}
/**
* Filter samples by a complex query involving metadata and variants data.
* @param data .
* @param params Map containing any of the following optional parameters.
* study: Study [[organization@]project:]study where study and project can be either the ID or UUID.
* jobId: Job ID. It must be a unique string within the study. An ID will be autogenerated automatically if not provided.
* jobDescription: Job description.
* jobDependsOn: Comma separated list of existing job IDs the job will depend on.
* jobTags: Job tags.
* jobScheduledStartTime: Time when the job is scheduled to start.
* jobPriority: Priority of the job.
* jobDryRun: Flag indicating that the job will be executed in dry-run mode. In this mode, OpenCGA will validate that all
* parameters and prerequisites are correctly set for successful execution, but the job will not actually run.
* @return a RestResponse object.
* @throws ClientException ClientException if there is any server error.
*/
public RestResponse runSampleEligibility(SampleEligibilityAnalysisParams data, ObjectMap params) throws ClientException {
params = params != null ? params : new ObjectMap();
params.put("body", data);
return execute("analysis", null, "variant/sample/eligibility", null, "run", params, POST, Job.class);
}
/**
* Run quality control (QC) for a given sample. It includes variant stats, and if the sample is somatic, mutational signature and
* genome plot are calculated.
* @param data Sample QC analysis params. Mutational signature and genome plot are calculated for somatic samples only. In order to
* skip some metrics, use the following keywords (separated by commas): variant-stats, signature, signature-catalogue,
* signature-fitting, genome-plot.
* @param params Map containing any of the following optional parameters.
* study: Study [[organization@]project:]study where study and project can be either the ID or UUID.
* jobId: Job ID. It must be a unique string within the study. An ID will be autogenerated automatically if not provided.
* jobDescription: Job description.
* jobDependsOn: Comma separated list of existing job IDs the job will depend on.
* jobTags: Job tags.
* jobScheduledStartTime: Time when the job is scheduled to start.
* jobPriority: Priority of the job.
* jobDryRun: Flag indicating that the job will be executed in dry-run mode. In this mode, OpenCGA will validate that all
* parameters and prerequisites are correctly set for successful execution, but the job will not actually run.
* @return a RestResponse object.
* @throws ClientException ClientException if there is any server error.
*/
public RestResponse runSampleQc(SampleQcAnalysisParams data, ObjectMap params) throws ClientException {
params = params != null ? params : new ObjectMap();
params.put("body", data);
return execute("analysis", null, "variant/sample/qc", null, "run", params, POST, Job.class);
}
/**
* Get sample data of a given variant.
* @param params Map containing any of the following optional parameters.
* limit: Number of results to be returned.
* skip: Number of results to skip.
* variant: Variant.
* study: Study where all the samples belong to.
* genotype: Genotypes that the sample must have to be selected.
* @return a RestResponse object.
* @throws ClientException ClientException if there is any server error.
*/
public RestResponse querySample(ObjectMap params) throws ClientException {
params = params != null ? params : new ObjectMap();
return execute("analysis", null, "variant/sample", null, "query", params, GET, Variant.class);
}
/**
* Get samples given a set of variants.
* @param data Sample variant filter params.
* @param params Map containing any of the following optional parameters.
* study: Study [[organization@]project:]study where study and project can be either the ID or UUID.
* jobId: Job ID. It must be a unique string within the study. An ID will be autogenerated automatically if not provided.
* jobDescription: Job description.
* jobDependsOn: Comma separated list of existing job IDs the job will depend on.
* jobTags: Job tags.
* jobScheduledStartTime: Time when the job is scheduled to start.
* jobPriority: Priority of the job.
* jobDryRun: Flag indicating that the job will be executed in dry-run mode. In this mode, OpenCGA will validate that all
* parameters and prerequisites are correctly set for successful execution, but the job will not actually run.
* @return a RestResponse object.
* @throws ClientException ClientException if there is any server error.
*/
public RestResponse runSample(SampleVariantFilterParams data, ObjectMap params) throws ClientException {
params = params != null ? params : new ObjectMap();
params.put("body", data);
return execute("analysis", null, "variant/sample", null, "run", params, POST, Job.class);
}
/**
* Obtain sample variant stats from a sample.
* @param sample Sample ID.
* @param params Map containing any of the following optional parameters.
* region: List of regions, these can be just a single chromosome name or regions in the format chr:start-end, e.g.:
* 2,3:100000-200000.
* type: List of types, accepted values are SNV, MNV, INDEL, SV, COPY_NUMBER, COPY_NUMBER_LOSS, COPY_NUMBER_GAIN, INSERTION,
* DELETION, DUPLICATION, TANDEM_DUPLICATION, BREAKEND, e.g. SNV,INDEL.
* study: Study [[organization@]project:]study where study and project can be either the ID or UUID.
* file: Filter variants from the files specified. This will set includeFile parameter when not provided.
* filter: Specify the FILTER for any of the files. If 'file' filter is provided, will match the file and the filter. e.g.:
* PASS,LowGQX.
* sampleData: Filter by any SampleData field from samples. [{sample}:]{key}{op}{value}[,;]* . If no sample is specified, will
* use all samples from "sample" or "genotype" filter. e.g. DP>200 or HG0097:DP>200,HG0098:DP<10 . Many FORMAT fields can be
* combined. e.g. HG0097:DP>200;GT=1/1,0/1,HG0098:DP<10.
* ct: List of SO consequence types, e.g. missense_variant,stop_lost or SO:0001583,SO:0001578. Accepts aliases 'loss_of_function'
* and 'protein_altering'.
* biotype: List of biotypes, e.g. protein_coding.
* transcriptFlag: List of transcript flags. e.g. canonical, CCDS, basic, LRG, MANE Select, MANE Plus Clinical, EGLH_HaemOnc,
* TSO500.
* populationFrequencyAlt: Alternate Population Frequency: {study}:{population}[<|>|<=|>=]{number}. e.g. 1000G:ALL<0.01.
* clinical: Clinical source: clinvar, cosmic.
* clinicalSignificance: Clinical significance: benign, likely_benign, likely_pathogenic, pathogenic.
* clinicalConfirmedStatus: Clinical confirmed status.
* study: Study [[organization@]project:]study where study and project can be either the ID or UUID.
* filterTranscript: Do filter transcripts when obtaining transcript counts.
* @return a RestResponse object.
* @throws ClientException ClientException if there is any server error.
*/
public RestResponse querySampleStats(String sample, ObjectMap params) throws ClientException {
params = params != null ? params : new ObjectMap();
params.putIfNotNull("sample", sample);
return execute("analysis", null, "variant/sample/stats", null, "query", params, GET, SampleVariantStats.class);
}
/**
* Compute sample variant stats for the selected list of samples.
* @param data Sample variant stats params. Use index=true and indexId='' to store the result in catalog sample QC. indexId=ALL
* requires an empty query. Use sample=all to compute sample stats of all samples in the variant storage.
* @param params Map containing any of the following optional parameters.
* study: Study [[organization@]project:]study where study and project can be either the ID or UUID.
* jobId: Job ID. It must be a unique string within the study. An ID will be autogenerated automatically if not provided.
* jobDescription: Job description.
* jobDependsOn: Comma separated list of existing job IDs the job will depend on.
* jobTags: Job tags.
* jobScheduledStartTime: Time when the job is scheduled to start.
* jobPriority: Priority of the job.
* jobDryRun: Flag indicating that the job will be executed in dry-run mode. In this mode, OpenCGA will validate that all
* parameters and prerequisites are correctly set for successful execution, but the job will not actually run.
* @return a RestResponse object.
* @throws ClientException ClientException if there is any server error.
*/
public RestResponse runSampleStats(SampleVariantStatsAnalysisParams data, ObjectMap params) throws ClientException {
params = params != null ? params : new ObjectMap();
params.put("body", data);
return execute("analysis", null, "variant/sample/stats", null, "run", params, POST, Job.class);
}
/**
* Export calculated variant stats and frequencies.
* @param data Variant stats export params.
* @param params Map containing any of the following optional parameters.
* project: Project [organization@]project where project can be either the ID or the alias.
* study: Study [[organization@]project:]study where study and project can be either the ID or UUID.
* jobId: Job ID. It must be a unique string within the study. An ID will be autogenerated automatically if not provided.
* jobDescription: Job description.
* jobDependsOn: Comma separated list of existing job IDs the job will depend on.
* jobTags: Job tags.
* jobScheduledStartTime: Time when the job is scheduled to start.
* jobPriority: Priority of the job.
* jobDryRun: Flag indicating that the job will be executed in dry-run mode. In this mode, OpenCGA will validate that all
* parameters and prerequisites are correctly set for successful execution, but the job will not actually run.
* @return a RestResponse object.
* @throws ClientException ClientException if there is any server error.
*/
public RestResponse runStatsExport(VariantStatsExportParams data, ObjectMap params) throws ClientException {
params = params != null ? params : new ObjectMap();
params.put("body", data);
return execute("analysis", null, "variant/stats/export", null, "run", params, POST, Job.class);
}
/**
* Compute variant stats for any cohort and any set of variants.
* @param data Variant stats params.
* @param params Map containing any of the following optional parameters.
* study: Study [[organization@]project:]study where study and project can be either the ID or UUID.
* jobId: Job ID. It must be a unique string within the study. An ID will be autogenerated automatically if not provided.
* jobDescription: Job description.
* jobDependsOn: Comma separated list of existing job IDs the job will depend on.
* jobTags: Job tags.
* jobScheduledStartTime: Time when the job is scheduled to start.
* jobPriority: Priority of the job.
* jobDryRun: Flag indicating that the job will be executed in dry-run mode. In this mode, OpenCGA will validate that all
* parameters and prerequisites are correctly set for successful execution, but the job will not actually run.
* @return a RestResponse object.
* @throws ClientException ClientException if there is any server error.
*/
public RestResponse runStats(VariantStatsAnalysisParams data, ObjectMap params) throws ClientException {
params = params != null ? params : new ObjectMap();
params.put("body", data);
return execute("analysis", null, "variant/stats", null, "run", params, POST, Job.class);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy