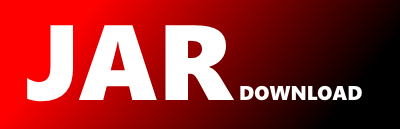
org.opencms.ade.containerpage.shared.CmsContainerElementData Maven / Gradle / Ivy
Show all versions of opencms-gwt Show documentation
/*
* This library is part of OpenCms -
* the Open Source Content Management System
*
* Copyright (c) Alkacon Software GmbH (http://www.alkacon.com)
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* For further information about Alkacon Software, please see the
* company website: http://www.alkacon.com
*
* For further information about OpenCms, please see the
* project website: http://www.opencms.org
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*/
package org.opencms.ade.containerpage.shared;
import org.opencms.gwt.shared.CmsAdditionalInfoBean;
import org.opencms.util.CmsStringUtil;
import org.opencms.xml.content.CmsXmlContentProperty;
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
import java.util.Set;
/**
* Bean holding all element information including it's formatted contents.
*
* @since 8.0.0
*/
public class CmsContainerElementData extends CmsContainerElement {
/** The contents by container type. */
private Map m_contents;
/** The group-container description. */
private String m_description;
/** The inheritance infos off all sub-items. */
private List m_inheritanceInfos = new ArrayList();
/** The inheritance name. */
private String m_inheritanceName;
/** The last user modifying the element. */
private String m_lastModifiedByUser;
/** The date of last modification. */
private long m_lastModifiedDate;
/** The element navText property. */
private String m_navText;
/** The settings for this container entry. */
private Map m_settings;
/** The contained sub-item id's. */
private List m_subItems = new ArrayList();
/** The element title property. */
private String m_title;
/** The supported container types of a group-container. */
private Set m_types;
/** The formatter configurations by container. */
private Map> m_formatters;
/**
* Returns if there are alternative formatters available for the given container.
*
* @param containerName the container name
*
* @return true
if there are alternative formatters available for the given container
*/
public boolean hasAlternativeFormatters(String containerName) {
return (m_formatters.get(containerName) != null) && (m_formatters.get(containerName).size() > 1);
}
/**
* Sets the formatter configurations.
*
* @param formatters the formatter configurations to set
*/
public void setFormatters(Map> formatters) {
m_formatters = formatters;
}
/**
* Returns the current formatter configuration.
*
* @param containerName the current container name
*
* @return the current formatter configuration
*/
public CmsFormatterConfig getFormatterConfig(String containerName) {
String formatterId = getSettings().get(CmsFormatterConfig.getSettingsKeyForContainer(containerName));
CmsFormatterConfig formatterConfig = null;
if ((formatterId != null)
&& getFormatters().containsKey(containerName)
&& getFormatters().get(containerName).containsKey(formatterId)) {
// if the settings contain the formatter id, use the matching config
formatterConfig = getFormatters().get(containerName).get(formatterId);
} else if (getFormatters().containsKey(containerName) && !getFormatters().get(containerName).isEmpty()) {
// otherwise use the first entry for the given container
formatterConfig = getFormatters().get(containerName).values().iterator().next();
}
return formatterConfig;
}
/**
* Returns the formatter configurations.
*
* @return the formatter configurations
*/
public Map> getFormatters() {
return m_formatters;
}
/**
* Returns the contents.
*
* @return the contents
*/
public Map getContents() {
return m_contents;
}
/**
* Returns the required css resources.
*
* @param containerName the current container name
*
* @return the required css resources
*/
public Set getCssResources(String containerName) {
CmsFormatterConfig formatterConfig = getFormatterConfig(containerName);
return formatterConfig != null ? formatterConfig.getCssResources() : Collections. emptySet();
}
/**
* Returns the description.
*
* @return the description
*/
public String getDescription() {
return m_description;
}
/**
* Returns the individual element settings formated with nice-names to be used as additional-info.
*
* @param containerId the container id
*
* @return the settings list
*/
public List getFormatedIndividualSettings(String containerId) {
List result = new ArrayList();
CmsFormatterConfig config = getFormatterConfig(containerId);
if ((m_settings != null) && (config != null)) {
for (Entry settingEntry : m_settings.entrySet()) {
String settingKey = settingEntry.getKey();
if (config.getSettingConfig().containsKey(settingEntry.getKey())) {
String niceName = config.getSettingConfig().get(settingEntry.getKey()).getNiceName();
if (CmsStringUtil.isNotEmptyOrWhitespaceOnly(config.getSettingConfig().get(settingEntry.getKey()).getNiceName())) {
settingKey = niceName;
}
}
result.add(new CmsAdditionalInfoBean(settingKey, settingEntry.getValue(), null));
}
}
return result;
}
/**
* Returns the inheritance infos off all sub-items.
*
* @return the inheritance infos off all sub-items.
*/
public List getInheritanceInfos() {
if (isInheritContainer()) {
return m_inheritanceInfos;
} else {
throw new UnsupportedOperationException("Only inherit containers have inheritance infos");
}
}
/**
* Returns the inheritance name.
*
* @return the inheritance name
*/
public String getInheritanceName() {
return m_inheritanceName;
}
/**
* Returns the last modifying user.
*
* @return the last modifying user
*/
public String getLastModifiedByUser() {
return m_lastModifiedByUser;
}
/**
* Returns the last modification date.
*
* @return the last modification date
*/
public long getLastModifiedDate() {
return m_lastModifiedDate;
}
/**
* Returns the navText.
*
* @return the navText
*/
public String getNavText() {
return m_navText;
}
/**
* Gets the setting configuration for this container element.
*
* @param containerName the current container name
*
* @return the setting configuration map
*/
public Map getSettingConfig(String containerName) {
CmsFormatterConfig formatterConfig = getFormatterConfig(containerName);
return formatterConfig != null
? formatterConfig.getSettingConfig()
: Collections. emptyMap();
}
/**
* Returns the settings for this container element.
*
* @return a map of settings
*/
public Map getSettings() {
return m_settings;
}
/**
* Returns the sub-items.
*
* @return the sub-items
*/
public List getSubItems() {
if (isGroupContainer()) {
return m_subItems;
} else if (isInheritContainer()) {
List result = new ArrayList();
for (CmsInheritanceInfo info : m_inheritanceInfos) {
if (info.isVisible()) {
result.add(info.getClientId());
}
}
return result;
} else {
throw new UnsupportedOperationException("Only group or inherit containers have sub-items");
}
}
/**
* Returns the title.
*
* @return the title
*/
public String getTitle() {
return m_title;
}
/**
* Returns the supported container types.
*
* @return the supported container types
*/
public Set getTypes() {
return m_types;
}
/**
* @see org.opencms.ade.containerpage.shared.CmsContainerElement#hasSettings(java.lang.String)
*/
@Override
public boolean hasSettings(String containerId) {
CmsFormatterConfig config = getFormatterConfig(containerId);
return (config != null) && (!config.getSettingConfig().isEmpty() || hasAlternativeFormatters(containerId));
}
/**
* Sets the contents.
*
* @param contents the contents to set
*/
public void setContents(Map contents) {
m_contents = contents;
}
/**
* Sets the description.
*
* @param description the description to set
*/
public void setDescription(String description) {
m_description = description;
}
/**
* Sets the inheritance infos.
*
* @param inheritanceInfos the inheritance infos to set
*/
public void setInheritanceInfos(List inheritanceInfos) {
m_inheritanceInfos = inheritanceInfos;
}
/**
* Sets the inheritance name.
*
* @param inheritanceName the inheritance name to set
*/
public void setInheritanceName(String inheritanceName) {
m_inheritanceName = inheritanceName;
}
/**
* Sets the modifying userdByUser.
*
* @param lastModifiedByUser the last modifying user to set
*/
public void setLastModifiedByUser(String lastModifiedByUser) {
m_lastModifiedByUser = lastModifiedByUser;
}
/**
* Sets the last modification date.
*
* @param lastModifiedDate the last modification date to set
*/
public void setLastModifiedDate(long lastModifiedDate) {
m_lastModifiedDate = lastModifiedDate;
}
/**
* Sets the navText.
*
* @param navText the navText to set
*/
public void setNavText(String navText) {
m_navText = navText;
}
/**
* Sets the settings for this container element.
*
* @param settings the new settings
*/
public void setSettings(Map settings) {
m_settings = settings;
}
/**
* Sets the sub-items.
*
* @param subItems the sub-items to set
*/
public void setSubItems(List subItems) {
m_subItems = subItems;
}
/**
* Sets the title.
*
* @param title the title to set
*/
public void setTitle(String title) {
m_title = title;
}
/**
* Sets the supported container types.
*
* @param types the supported container types to set
*/
public void setTypes(Set types) {
m_types = types;
}
/**
* @see java.lang.Object#toString()
*/
@Override
public String toString() {
StringBuffer result = new StringBuffer();
result.append("Title: ").append(m_title).append(" File: ").append(getSitePath()).append(" ClientId: ").append(
getClientId());
return result.toString();
}
}