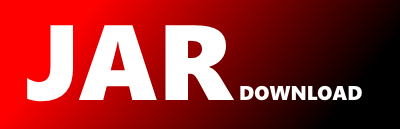
org.opencms.ade.containerpage.shared.CmsFormatterConfig Maven / Gradle / Ivy
Show all versions of opencms-gwt Show documentation
/*
* This library is part of OpenCms -
* the Open Source Content Management System
*
* Copyright (c) Alkacon Software GmbH (http://www.alkacon.com)
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* For further information about Alkacon Software, please see the
* company website: http://www.alkacon.com
*
* For further information about OpenCms, please see the
* project website: http://www.opencms.org
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*/
package org.opencms.ade.containerpage.shared;
import org.opencms.util.CmsStringUtil;
import org.opencms.xml.content.CmsXmlContentProperty;
import java.util.Map;
import java.util.Set;
import com.google.gwt.user.client.rpc.IsSerializable;
/**
* Formatter configuration data.
*/
public class CmsFormatterConfig implements IsSerializable {
/** Key for the formatter configuration id setting. Append the container name to the key, to store container depending values. */
public static final String FORMATTER_SETTINGS_KEY = "formatterSettings#";
/** Id used for schema based formatters. */
public static final String SCHEMA_FORMATTER_ID = "schema_formatter";
/** The required css resources. */
private Set m_cssResources;
/** The formatter configuration id. */
private String m_id;
/** The inline CSS. */
private String m_inlineCss;
/** The formatter root path. */
private String m_jspRootPath;
/** The formatter label. */
private String m_label;
/** The setting for this container element. */
private Map m_settingConfig;
/**
* Constructor.
*
* @param id the formatter id
*/
public CmsFormatterConfig(String id) {
m_id = id;
}
/**
* Constructor for serialization only.
*/
protected CmsFormatterConfig() {
// nothing to to
}
/**
* Returns the formatter configuration settings key for the given container name.
*
* @param containerName the container name
*
* @return the settings key
*/
public static String getSettingsKeyForContainer(String containerName) {
return FORMATTER_SETTINGS_KEY + containerName;
}
/**
* Returns the required CSS resources.
*
* @return the CSS resources
*/
public Set getCssResources() {
return m_cssResources;
}
/**
* Returns the formatter configuration id.
*
* @return the configuration id
*/
public String getId() {
return m_id;
}
/**
* Returns the inline CSS.
*
* @return the inline CSS
*/
public String getInlineCss() {
return m_inlineCss;
}
/**
* Returns the formatter root path.
*
* @return the formatter root path
*/
public String getJspRootPath() {
return m_jspRootPath;
}
/**
* Returns the formatter label.
*
* @return the label
*/
public String getLabel() {
return m_label;
}
/**
* Returns the settings configuration.
*
* @return the settings configuration
*/
public Map getSettingConfig() {
return m_settingConfig;
}
/**
* Returns if the formatter has inline CSS.
*
* @return true
if the formatter has inline CSS
*/
public boolean hasInlineCss() {
return CmsStringUtil.isNotEmptyOrWhitespaceOnly(m_inlineCss);
}
/**
* Sets the required CSS resources.
*
* @param cssResources the CSS resources
*/
public void setCssResources(Set cssResources) {
m_cssResources = cssResources;
}
/**
* Sets the inline CSS.
*
* @param inlineCss the inline CSS
*/
public void setInlineCss(String inlineCss) {
m_inlineCss = inlineCss;
}
/**
* Sets the formatter root path.
*
* @param jspRootPath the formatter root path
*/
public void setJspRootPath(String jspRootPath) {
m_jspRootPath = jspRootPath;
}
/**
* Sets the formatter label.
*
* @param label the label
*/
public void setLabel(String label) {
m_label = label;
}
/**
* Sets the settings configuration.
*
* @param settingConfig the settings configuration
*/
public void setSettingConfig(Map settingConfig) {
m_settingConfig = settingConfig;
}
}