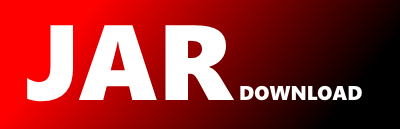
org.opencms.gwt.client.rpc.CmsRpcAction Maven / Gradle / Ivy
Show all versions of opencms-gwt Show documentation
/*
* This library is part of OpenCms -
* the Open Source Content Management System
*
* Copyright (c) Alkacon Software GmbH (http://www.alkacon.com)
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* For further information about Alkacon Software, please see the
* company website: http://www.alkacon.com
*
* For further information about OpenCms, please see the
* project website: http://www.opencms.org
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*/
package org.opencms.gwt.client.rpc;
import org.opencms.gwt.client.Messages;
import org.opencms.gwt.client.ui.CmsErrorDialog;
import org.opencms.gwt.client.ui.CmsNotification;
import com.google.gwt.core.client.GWT;
import com.google.gwt.event.shared.UmbrellaException;
import com.google.gwt.user.client.Timer;
import com.google.gwt.user.client.rpc.AsyncCallback;
import com.google.gwt.user.client.rpc.StatusCodeException;
/**
* Consistently manages RPCs errors and 'loading' state.
*
* @param The type of the expected return value
*
* @since 8.0
*/
public abstract class CmsRpcAction implements AsyncCallback {
/** The message displayed when loading. */
private String m_loadingMessage = Messages.get().key(Messages.GUI_LOADING_0);
/** The result, used only for synchronized request. */
private T m_result;
/** The timer to control the display of the 'loading' state, if the action takes too long. */
private Timer m_timer;
/**
* Executes the current RPC call.
*
* Initializes client-server communication and will
*/
public abstract void execute();
/**
* Executes a synchronized request.
*
* @return the RPC result
*
* @see #execute()
*/
public T executeSync() {
execute();
return m_result;
}
/**
* Handle errors.
*
* @see com.google.gwt.user.client.rpc.AsyncCallback#onFailure(java.lang.Throwable)
*/
public void onFailure(Throwable t) {
// a status code exception indicates the session is no longer valid
if ((t instanceof StatusCodeException) && (((StatusCodeException)t).getStatusCode() == 500)) {
CmsErrorDialog dialog = new CmsErrorDialog(Messages.get().key(Messages.GUI_SESSION_EXPIRED_0), null);
dialog.center();
} else {
CmsErrorDialog.handleException(t);
}
// remove the overlay
stop(false);
}
/**
* @see com.google.gwt.user.client.rpc.AsyncCallback#onSuccess(java.lang.Object)
*/
public void onSuccess(T value) {
try {
m_result = value;
onResponse(value);
} catch (UmbrellaException exception) {
Throwable wrappedException = exception.getCauses().iterator().next();
onFailure(wrappedException);
if (!GWT.isProdMode()) {
throw exception;
}
} catch (RuntimeException error) {
onFailure(error);
if (!GWT.isProdMode()) {
throw error;
}
}
}
/**
* Sets the loading message.
*
* @param loadingMessage the loading message to set
*/
public void setLoadingMessage(String loadingMessage) {
m_loadingMessage = loadingMessage;
}
/**
* Starts the timer for showing the 'loading' state.
*
* Note: Has to be called manually before calling the RPC service.
*
* @param delay the delay in milliseconds
* @param blocking shows an blocking overlay if true
*/
public void start(int delay, final boolean blocking) {
if (delay <= 0) {
show(blocking);
return;
}
m_timer = new Timer() {
/**
* @see com.google.gwt.user.client.Timer#run()
*/
@Override
public void run() {
show(blocking);
}
};
m_timer.schedule(delay);
}
/**
* Stops the timer.
*
* Note: Has to be called manually on success.
*
* @param displayDone true
if you want to tell the user that the operation was successful
*/
public void stop(boolean displayDone) {
if (m_timer != null) {
m_timer.cancel();
m_timer = null;
}
CmsNotification.get().hide();
if (displayDone) {
CmsNotification.get().send(CmsNotification.Type.NORMAL, Messages.get().key(Messages.GUI_DONE_0));
}
}
/**
* Handles the result when received from server.
*
* @param result the result from server
*
* @see AsyncCallback#onSuccess(Object)
*/
protected abstract void onResponse(T result);
/**
* Shows the 'loading message'.
*
* Overwrite to customize the message.
*
* @param blocking shows an blocking overlay if true
*/
protected void show(boolean blocking) {
if (blocking) {
CmsNotification.get().sendBlocking(CmsNotification.Type.NORMAL, m_loadingMessage);
} else {
CmsNotification.get().sendSticky(CmsNotification.Type.NORMAL, m_loadingMessage);
}
}
}