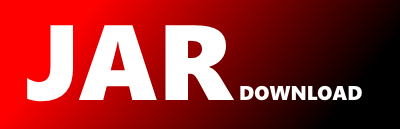
org.opencms.gwt.shared.CmsListInfoBean Maven / Gradle / Ivy
Show all versions of opencms-gwt Show documentation
/*
* This library is part of OpenCms -
* the Open Source Content Management System
*
* Copyright (c) Alkacon Software GmbH (http://www.alkacon.com)
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* For further information about Alkacon Software, please see the
* company website: http://www.alkacon.com
*
* For further information about OpenCms, please see the
* project website: http://www.opencms.org
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*/
package org.opencms.gwt.shared;
import org.opencms.db.CmsResourceState;
import java.util.ArrayList;
import java.util.List;
import com.google.gwt.user.client.rpc.IsSerializable;
/**
* A bean holding all info to be displayed in {@link org.opencms.gwt.client.ui.CmsListItemWidget}s.
*
* @see org.opencms.gwt.client.ui.CmsListItemWidget
*
* @since 8.0.0
*/
public class CmsListInfoBean implements IsSerializable {
/** Lock icons. */
public enum LockIcon {
/** Closed lock. */
CLOSED,
/** No lock. */
NONE,
/** Open lock. */
OPEN,
/** Shared closed lock. */
SHARED_CLOSED,
/** Shared open lock. */
SHARED_OPEN
}
/**
* Enum for the type of page icon which should be displayed.
*/
public enum StateIcon {
/** copy page icon. */
copy,
/** export page icon. */
export,
/** secure page icon. */
secure,
/** standard page icon. */
standard
}
/** CSS class for multi-line additional info's. */
public static final String CSS_CLASS_MULTI_LINE = "multiLineLabel";
/** The additional info. */
private List m_additionalInfo;
/** The lock icon. */
private LockIcon m_lockIcon;
/** The lock icon title. */
private String m_lockIconTitle;
/** Flag to control whether a resource state of 'changed' should be visualized with an overlay icon. */
private boolean m_markChangedState = true;
/** The resource state. */
private CmsResourceState m_resourceState;
/** The resource type name of the resource. */
private String m_resourceType;
/** The state icon information: for the type of state icon which should be displayed. The state icon indicates if a resource is exported, secure etc. */
private StateIcon m_stateIcon;
/** The sub-title. */
private String m_subTitle;
/** The title. */
private String m_title;
/**
* Default constructor.
*/
public CmsListInfoBean() {
// empty
}
/**
* Constructor.
*
* @param title the title
* @param subtitle the subtitle
* @param additionalInfo the additional info
*/
public CmsListInfoBean(String title, String subtitle, List additionalInfo) {
m_title = title;
m_subTitle = subtitle;
m_additionalInfo = additionalInfo;
}
/**
* Sets a new additional info.
*
* @param name the additional info name
* @param value the additional info value
*/
public void addAdditionalInfo(String name, String value) {
getAdditionalInfo().add(new CmsAdditionalInfoBean(name, value, null));
}
/**
* Sets a new additional info.
*
* @param name the additional info name
* @param value the additional info value
* @param style the CSS style to apply to the info
*/
public void addAdditionalInfo(String name, String value, String style) {
getAdditionalInfo().add(new CmsAdditionalInfoBean(name, value, style));
}
/**
* Returns the additional info.
*
* @return the additional info
*/
public List getAdditionalInfo() {
if (m_additionalInfo == null) {
m_additionalInfo = new ArrayList();
}
return m_additionalInfo;
}
/**
* Returns the lock icon.
*
* @return the lockIcon
*/
public LockIcon getLockIcon() {
return m_lockIcon;
}
/**
* Returns the lock icon title.
*
* @return the lock icon title
*/
public String getLockIconTitle() {
return m_lockIconTitle;
}
/**
* Returns the resourceState.
*
* @return the resourceState
*/
public CmsResourceState getResourceState() {
return m_resourceState;
}
/**
* Returns the resource type name.
*
* @return the resource type name
*/
public String getResourceType() {
return m_resourceType;
}
/**
* Returns the state icon.
*
* The state icon indicates if a resource is exported, secure etc.
*
* @return the state Icon
*/
public StateIcon getStateIcon() {
return m_stateIcon;
}
/**
* Returns the sub-title.
*
* @return the sub-title
*/
public String getSubTitle() {
return m_subTitle;
}
/**
* Returns the title.
*
* @return the title
*/
public String getTitle() {
return m_title;
}
/**
* Returns if the bean has additional info elements.
*
* @return true
if the bean has additional info elements
*/
public boolean hasAdditionalInfo() {
return (m_additionalInfo != null) && (m_additionalInfo.size() > 0);
}
/**
* Returns true if the 'changed' resource state should be marked by an icon.
*
* @return true if the 'changed' resource state should be marked by an icon.
*/
public boolean isMarkChangedState() {
return m_markChangedState;
}
/**
* Sets the additional info.
*
* @param additionalInfo the additional info to set
*/
public void setAdditionalInfo(List additionalInfo) {
m_additionalInfo = additionalInfo;
}
/**
* Sets the lock icon.
*
* @param lockIcon the lock icon to set
*/
public void setLockIcon(LockIcon lockIcon) {
m_lockIcon = lockIcon;
}
/**
* Sets the lock icon title.
*
* @param lockIconTitle the lock icon title to set
*/
public void setLockIconTitle(String lockIconTitle) {
m_lockIconTitle = lockIconTitle;
}
/**
* Enables or disables the display of the 'changed' icon for the 'changed' resource state.
*
* @param markChanged true if the 'changed' state should be displayed
*/
public void setMarkChangedState(boolean markChanged) {
m_markChangedState = markChanged;
}
/**
* Sets the resourceState.
*
* @param resourceState the resourceState to set
*/
public void setResourceState(CmsResourceState resourceState) {
m_resourceState = resourceState;
}
/**
* Sets the resource type name.
*
* @param resourceType the resource type name to set
*/
public void setResourceType(String resourceType) {
m_resourceType = resourceType;
}
/**
* Sets the state icon.
*
* The state icon indicates if a resource is exported, secure etc.
*
* @param stateIcon the state icon to set
*/
public void setStateIcon(StateIcon stateIcon) {
m_stateIcon = stateIcon;
}
/**
* Sets the sub-title.
*
* @param subTitle the sub-title to set
*/
public void setSubTitle(String subTitle) {
m_subTitle = subTitle;
}
/**
* Sets the title.
*
* @param title the title to set
*/
public void setTitle(String title) {
m_title = title;
}
}