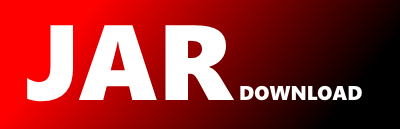
org.opencms.setup.CmsSetupBean Maven / Gradle / Ivy
/*
* This library is part of OpenCms -
* the Open Source Content Management System
*
* Copyright (c) Alkacon Software GmbH & Co. KG (http://www.alkacon.com)
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* For further information about Alkacon Software GmbH & Co. KG, please see the
* company website: http://www.alkacon.com
*
* For further information about OpenCms, please see the
* project website: http://www.opencms.org
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*/
package org.opencms.setup;
import org.opencms.configuration.CmsConfigurationException;
import org.opencms.configuration.CmsConfigurationManager;
import org.opencms.configuration.CmsImportExportConfiguration;
import org.opencms.configuration.CmsModuleConfiguration;
import org.opencms.configuration.CmsParameterConfiguration;
import org.opencms.configuration.CmsSearchConfiguration;
import org.opencms.configuration.CmsSitesConfiguration;
import org.opencms.configuration.CmsSystemConfiguration;
import org.opencms.configuration.CmsVfsConfiguration;
import org.opencms.configuration.CmsWorkplaceConfiguration;
import org.opencms.configuration.I_CmsXmlConfiguration;
import org.opencms.db.CmsDbPoolV11;
import org.opencms.db.CmsUserSettings;
import org.opencms.file.CmsObject;
import org.opencms.file.CmsResource;
import org.opencms.file.CmsUser;
import org.opencms.i18n.CmsEncoder;
import org.opencms.i18n.CmsMessageContainer;
import org.opencms.importexport.CmsImportParameters;
import org.opencms.loader.CmsImageLoader;
import org.opencms.main.CmsException;
import org.opencms.main.CmsLog;
import org.opencms.main.CmsRuntimeException;
import org.opencms.main.CmsShell;
import org.opencms.main.CmsSystemInfo;
import org.opencms.main.I_CmsEventListener;
import org.opencms.main.I_CmsShellCommands;
import org.opencms.main.OpenCms;
import org.opencms.main.OpenCmsServlet;
import org.opencms.module.CmsModule;
import org.opencms.module.CmsModuleManager;
import org.opencms.report.CmsShellReport;
import org.opencms.setup.comptest.CmsSetupTestResult;
import org.opencms.setup.comptest.CmsSetupTestSimapi;
import org.opencms.setup.comptest.I_CmsSetupTest;
import org.opencms.setup.xml.CmsSetupXmlHelper;
import org.opencms.util.CmsCollectionsGenericWrapper;
import org.opencms.util.CmsFileUtil;
import org.opencms.util.CmsStringUtil;
import org.opencms.workplace.tools.CmsIdentifiableObjectContainer;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.FileReader;
import java.io.FileWriter;
import java.io.IOException;
import java.io.InputStream;
import java.io.LineNumberReader;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Collections;
import java.util.Comparator;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Properties;
import java.util.Set;
import java.util.SortedMap;
import java.util.TreeMap;
import java.util.zip.ZipEntry;
import java.util.zip.ZipFile;
import javax.servlet.ServletConfig;
import javax.servlet.ServletContext;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpSession;
import javax.servlet.jsp.JspWriter;
import javax.servlet.jsp.PageContext;
import org.apache.commons.logging.Log;
import com.google.common.collect.Maps;
/**
* A java bean as a controller for the OpenCms setup wizard.
*
* It is not allowed to customize this bean with methods for a specific database server setup!
*
* Database server specific settings should be set/read using get/setDbProperty, as for example like:
*
*
* setDbProperty("oracle.defaultTablespace", value);
*
*
*
* @since 6.0.0
*/
public class CmsSetupBean implements I_CmsShellCommands {
/** Prefix for 'marker' properties in opencms.properties where additional properties should be inserted. */
public static final String ADDITIONAL_PREFIX = "additional.";
/** DB provider constant for as400. */
public static final String AS400_PROVIDER = "as400";
/** The name of the components properties file. */
public static final String COMPONENTS_PROPERTIES = "components.properties";
/** DB provider constant for db2. */
public static final String DB2_PROVIDER = "db2";
/** Folder constant name.
*/
public static final String FOLDER_BACKUP = "backup" + File.separatorChar;
/** Folder constant name.
*/
public static final String FOLDER_DATABASE = "database" + File.separatorChar;
/** Folder constant name.
*/
public static final String FOLDER_LIB = "lib" + File.separatorChar;
/** Folder constant name.
*/
public static final String FOLDER_SETUP = "WEB-INF/setupdata" + File.separatorChar;
/** DB provider constant. */
public static final String GENERIC_PROVIDER = "generic";
/** DB provider constant for hsqldb. */
public static final String HSQLDB_PROVIDER = "hsqldb";
/** Name of the property file containing HTML fragments for setup wizard and error dialog. */
public static final String HTML_MESSAGE_FILE = "org/opencms/setup/htmlmsg.properties";
/** DB provider constant for maxdb. */
public static final String MAXDB_PROVIDER = "maxdb";
/** DB provider constant for mssql. */
public static final String MSSQL_PROVIDER = "mssql";
/** DB provider constant for mysql. */
public static final String MYSQL_PROVIDER = "mysql";
/** DB provider constant for oracle. */
public static final String ORACLE_PROVIDER = "oracle";
/** DB provider constant for postgresql. */
public static final String POSTGRESQL_PROVIDER = "postgresql";
/** The default component position, is missing. */
protected static final int DEFAULT_POSITION = 9999;
/** Properties file key constant post fix. */
protected static final String PROPKEY_CHECKED = ".checked";
/** Properties file key constant prefix. */
protected static final String PROPKEY_COMPONENT = "component.";
/** Properties file key constant. */
protected static final String PROPKEY_COMPONENTS = "components";
/** Properties file key constant post fix. */
protected static final String PROPKEY_DEPENDENCIES = ".dependencies";
/** Properties file key constant post fix. */
protected static final String PROPKEY_DESCRIPTION = ".description";
/** Properties file key constant post fix. */
protected static final String PROPKEY_MODULES = ".modules";
/** Properties file key constant post fix. */
protected static final String PROPKEY_NAME = ".name";
/** Properties file key constant post fix. */
protected static final String PROPKEY_POSITION = ".position";
/** The log object for this class. */
private static final Log LOG = CmsLog.getLog(CmsSetupBean.class);
/** Contains HTML fragments for the output in the JSP pages of the setup wizard. */
private static Properties m_htmlProps;
/** Required files per database setup (for sql supported dbs). */
private static final String[] REQUIRED_SQL_DB_SETUP_FILES = {"database.properties"};
/** A map with all available modules. */
protected Map m_availableModules;
/** A CmsObject to execute shell commands. */
protected CmsObject m_cms;
/** Contains all defined components. */
protected CmsIdentifiableObjectContainer m_components;
/** A list with the package names of the modules to be installed .*/
protected List m_installModules;
/** Location for log file. */
protected String m_logFile = OpenCms.getSystemInfo().getLogFileRfsFolder() + "setup.log";
/** A map with lists of dependent module package names keyed by module package names. */
protected Map> m_moduleDependencies;
/** A map with all available modules filenames. */
protected Map m_moduleFilenames;
/** Location for module archives relative to the webapp folder. */
protected String m_modulesFolder = CmsSystemInfo.FOLDER_WEBINF
+ CmsSystemInfo.FOLDER_PACKAGES
+ CmsSystemInfo.FOLDER_MODULES;
/** The new logging offset in the workplace import thread. */
protected int m_newLoggingOffset;
/** The lod logging offset in the workplace import thread. */
protected int m_oldLoggingOffset;
/** The absolute path to the home directory of the OpenCms webapp. */
protected String m_webAppRfsPath;
/** Additional property sets to be inserted into opencms.properties during setup. */
private Map m_additionalProperties = Maps.newHashMap();
/** Signals whether the setup is executed in the auto mode or in the wizard mode. */
private boolean m_autoMode;
/** The absolute path to the config sub directory of the OpenCms web application. */
private String m_configRfsPath;
/** Contains the properties of "opencms.properties". */
private CmsParameterConfiguration m_configuration;
private String m_contextPath;
/** Key of the selected database server (e.g. "mysql", "generic" or "oracle") */
private String m_databaseKey;
/** List of keys of all available database server setups (e.g. "mysql", "generic" or "oracle") */
private List m_databaseKeys;
/** Map of database setup properties of all available database server setups keyed by their database keys. */
private Map m_databaseProperties;
/** Password used for the JDBC connection when the OpenCms database is created. */
private String m_dbCreatePwd;
/** The name of the default web application (in web.xml). */
private String m_defaultWebApplication;
/** Contains the error messages to be displayed in the setup wizard. */
private List m_errors;
/** The ethernet address. */
private String m_ethernetAddress;
/** The full key of the selected database including the "_jpa" or "_sql" information. */
private String m_fullDatabaseKey;
/** Flag which is set to true after module import if there is an index.html file in the default site. */
private boolean m_hasIndexHtml;
/** The Database Provider used in setup. */
private String m_provider;
/** A map with tokens ${...} to be replaced in SQL scripts. */
private Map m_replacer;
/** The initial servlet configuration. */
private ServletConfig m_servletConfig;
/** The servlet mapping (in web.xml). */
private String m_servletMapping;
private CmsShell m_shell;
/** List of sorted keys by ranking of all available database server setups (e.g. "mysql", "generic" or "oracle") */
private List m_sortedDatabaseKeys;
/** The workplace import thread. */
private CmsSetupWorkplaceImportThread m_workplaceImportThread;
/** Xml read/write helper object. */
private CmsSetupXmlHelper m_xmlHelper;
/**
* Default constructor.
*/
public CmsSetupBean() {
initHtmlParts();
}
/**
* Restores the opencms.xml either to or from a backup file, depending
* whether the setup wizard is executed the first time (the backup
* does not exist) or not (the backup exists).
*
* @param filename something like e.g. "opencms.xml"
* @param originalFilename the configurations real file name, e.g. "opencms.xml.ori"
*/
public void backupConfiguration(String filename, String originalFilename) {
// ensure backup folder exists
File backupFolder = new File(m_configRfsPath + FOLDER_BACKUP);
if (!backupFolder.exists()) {
backupFolder.mkdirs();
}
// copy file to (or from) backup folder
originalFilename = FOLDER_BACKUP + originalFilename;
File file = new File(m_configRfsPath + originalFilename);
if (file.exists()) {
copyFile(originalFilename, filename);
} else {
copyFile(filename, originalFilename);
}
}
/**
* Returns a map of dependencies.
*
* The component dependencies are get from the setup and module components.properties files found.
*
* @return a Map of component ids as keys and a list of dependency names as values
*/
public Map> buildDepsForAllComponents() {
Map> ret = new HashMap>();
Iterator itComponents = CmsCollectionsGenericWrapper. list(
m_components.elementList()).iterator();
while (itComponents.hasNext()) {
CmsSetupComponent component = itComponents.next();
// if component a depends on component b, and component c depends also on component b:
// build a map with a list containing "a" and "c" keyed by "b" to get a
// list of components depending on component "b"...
Iterator itDeps = component.getDependencies().iterator();
while (itDeps.hasNext()) {
String dependency = itDeps.next();
// get the list of dependent modules
List componentDependencies = ret.get(dependency);
if (componentDependencies == null) {
// build a new list if "b" has no dependent modules yet
componentDependencies = new ArrayList();
ret.put(dependency, componentDependencies);
}
// add "a" as a module depending on "b"
componentDependencies.add(component.getId());
}
}
itComponents = CmsCollectionsGenericWrapper. list(m_components.elementList()).iterator();
while (itComponents.hasNext()) {
CmsSetupComponent component = itComponents.next();
if (ret.get(component.getId()) == null) {
ret.put(component.getId(), new ArrayList());
}
}
return ret;
}
/**
* Checks the ethernet address value and generates a dummy address, if necessary. *
*/
public void checkEthernetAddress() {
// check the ethernet address in order to generate a random address, if not available
if (CmsStringUtil.isEmpty(getEthernetAddress())) {
setEthernetAddress(CmsStringUtil.getEthernetAddress());
}
}
/**
* Clears the cache.
*/
public void clearCache() {
OpenCms.getEventManager().fireEvent(I_CmsEventListener.EVENT_CLEAR_CACHES);
}
/**
* Copies a given file.
*
* @param source the source file
* @param target the destination file
*/
public void copyFile(String source, String target) {
try {
CmsFileUtil.copy(m_configRfsPath + source, m_configRfsPath + target);
} catch (IOException e) {
m_errors.add("Could not copy " + source + " to " + target + " \n");
m_errors.add(e.toString() + "\n");
}
}
/**
* Returns html code to display an error.
*
* @param pathPrefix to adjust the path
*
* @return html code
*/
public String displayError(String pathPrefix) {
if (pathPrefix == null) {
pathPrefix = "";
}
StringBuffer html = new StringBuffer(512);
html.append("
");
html.append("\t");
html.append("\t\t");
html.append(getHtmlPart("C_BLOCK_START", "Error"));
html.append("\t\t\t");
html.append("\t\t\t\t");
html.append("\t\t\t\t\t.append(pathPrefix).append("resources/error.png)
");
html.append("\t\t\t\t\t ");
html.append("\t\t\t\t\t");
html.append("\t\t\t\t\t\tThe Alkacon OpenCms setup wizard has not been started correctly!
");
html.append("\t\t\t\t\t\tPlease click here to restart the wizard.");
html.append("\t\t\t\t\t ");
html.append("\t\t\t\t ");
html.append("\t\t\t
");
html.append(getHtmlPart("C_BLOCK_END"));
html.append("\t\t ");
html.append("\t ");
html.append("
");
return html.toString();
}
/**
* Returns html code to display the errors occurred.
*
* @param pathPrefix to adjust the path
*
* @return html code
*/
public String displayErrors(String pathPrefix) {
if (pathPrefix == null) {
pathPrefix = "";
}
StringBuffer html = new StringBuffer(512);
html.append("
");
html.append("\t");
html.append("\t\t");
html.append(getHtmlPart("C_BLOCK_START", "Error"));
html.append("\t\t\t");
html.append("\t\t\t\t");
html.append("\t\t\t\t\t.append(pathPrefix).append("resources/error.png)
");
html.append("\t\t\t\t\t ");
html.append("\t\t\t\t\t");
Iterator iter = getErrors().iterator();
while (iter.hasNext()) {
String msg = iter.next();
html.append("\t\t\t\t\t\t");
html.append(msg);
html.append("
");
}
html.append("\t\t\t\t\t ");
html.append("\t\t\t\t ");
html.append("\t\t\t
");
html.append(getHtmlPart("C_BLOCK_END"));
html.append("\t\t ");
html.append("\t ");
html.append("
");
return html.toString();
}
/**
* Returns a map with all available modules.
*
* The map contains maps keyed by module package names. Each of these maps contains various
* information about the module such as the module name, version, description, and a list of
* it's dependencies. You should refer to the source code of this method to understand the data
* structure of the map returned by this method!
*
* @return a map with all available modules
*/
public Map getAvailableModules() {
if ((m_availableModules == null) || m_availableModules.isEmpty()) {
m_availableModules = new HashMap();
m_moduleDependencies = new HashMap>();
m_moduleFilenames = new HashMap();
m_components = new CmsIdentifiableObjectContainer(true, true);
try {
addComponentsFromPath(m_webAppRfsPath + FOLDER_SETUP);
Map modules = CmsModuleManager.getAllModulesFromPath(getModuleFolder());
Iterator> itMods = modules.entrySet().iterator();
while (itMods.hasNext()) {
Map.Entry entry = itMods.next();
CmsModule module = entry.getKey();
// put the module information into a map keyed by the module packages names
m_availableModules.put(module.getName(), module);
m_moduleFilenames.put(module.getName(), entry.getValue());
addComponentsFromPath(getModuleFolder() + entry.getValue());
}
} catch (CmsConfigurationException e) {
throw new CmsRuntimeException(e.getMessageContainer());
}
initializeComponents(new HashSet(m_availableModules.keySet()));
}
return m_availableModules;
}
/**
* Returns the components.
*
* @return the components
*/
public CmsIdentifiableObjectContainer getComponents() {
return m_components;
}
/**
* Returns the "config" path in the OpenCms web application.
*
* @return the config path
*/
public String getConfigRfsPath() {
return m_configRfsPath;
}
public String getContextPath() {
return m_contextPath;
}
/**
* Returns the key of the selected database server (e.g. "mysql", "generic" or "oracle").
*
* @return the key of the selected database server (e.g. "mysql", "generic" or "oracle")
*/
public String getDatabase() {
if (m_databaseKey == null) {
m_databaseKey = getExtProperty("db.name");
}
if (CmsStringUtil.isEmpty(m_databaseKey)) {
m_databaseKey = getSortedDatabases().get(0);
}
return m_databaseKey;
}
/**
* Returns a list of needed jar filenames for a database server setup specified by a database key (e.g. "mysql", "generic" or "oracle").
*
* @param databaseKey a database key (e.g. "mysql", "generic" or "oracle")
*
* @return a list of needed jar filenames
*/
public List getDatabaseLibs(String databaseKey) {
List lst;
lst = CmsStringUtil.splitAsList(
getDatabaseProperties().get(databaseKey).getProperty(databaseKey + ".libs"),
',',
true);
return lst;
}
/**
* Returns the clear text name for a database server setup specified by a database key (e.g. "mysql", "generic" or "oracle").
*
* @param databaseKey a database key (e.g. "mysql", "generic" or "oracle")
* @return the clear text name for a database server setup
*/
public String getDatabaseName(String databaseKey) {
return getDatabaseProperties().get(databaseKey).getProperty(databaseKey + PROPKEY_NAME);
}
/**
* Returns a map with the database properties of *all* available database configurations keyed
* by their database keys (e.g. "mysql", "generic" or "oracle").
*
* @return a map with the database properties of *all* available database configurations
*/
public Map getDatabaseProperties() {
if (m_databaseProperties == null) {
readDatabaseConfig();
}
return m_databaseProperties;
}
/**
* Returns a list with they keys (e.g. "mysql", "generic" or "oracle") of all available
* database server setups found in "/setup/database/".
*
* @return a list with they keys (e.g. "mysql", "generic" or "oracle") of all available database server setups
*/
public List getDatabases() {
if (m_databaseKeys == null) {
readDatabaseConfig();
}
return m_databaseKeys;
}
/**
* Returns the database name.
*
* @return the database name
*/
public String getDb() {
return getDbProperty(m_databaseKey + ".dbname");
}
/**
* Returns the JDBC connect URL parameters.
*
* @return the JDBC connect URL parameters
*/
public String getDbConStrParams() {
return getDbProperty(m_databaseKey + ".constr.params");
}
/**
* Returns the database create statement.
*
* @return the database create statement
*/
public String getDbCreateConStr() {
return getDbProperty(m_databaseKey + ".constr");
}
/**
* Returns the password used for database creation.
*
* @return the password used for database creation
*/
public String getDbCreatePwd() {
return (m_dbCreatePwd != null) ? m_dbCreatePwd : "";
}
/**
* Returns the database user that is used to connect to the database.
*
* @return the database user
*/
public String getDbCreateUser() {
return getDbProperty(m_databaseKey + ".user");
}
/**
* Returns the database driver belonging to the database
* from the default configuration.
*
* @return name of the database driver
*/
public String getDbDriver() {
return getDbProperty(m_databaseKey + ".driver");
}
/**
* Returns the value for a given key from the database properties.
*
* @param key the property key
*
* @return the string value for a given key
*/
public String getDbProperty(String key) {
// extract the database key out of the entire key
String databaseKey = key.substring(0, key.indexOf('.'));
Properties databaseProperties = getDatabaseProperties().get(databaseKey);
return databaseProperties.getProperty(key, "");
}
/**
* Returns the validation query belonging to the database
* from the default configuration .
*
* @return query used to validate connections
*/
public String getDbTestQuery() {
return getDbProperty(m_databaseKey + ".testQuery");
}
/**
* Returns a connection string.
*
* @return the connection string used by the OpenCms core
*/
public String getDbWorkConStr() {
if (m_provider.equals(POSTGRESQL_PROVIDER)) {
return getDbProperty(m_databaseKey + ".constr.newDb");
} else {
return getExtProperty(CmsDbPoolV11.KEY_DATABASE_POOL + '.' + getPool() + ".jdbcUrl");
}
}
/**
* Returns the password of the database from the properties .
*
* @return the password for the OpenCms database user
*/
public String getDbWorkPwd() {
return getExtProperty(CmsDbPoolV11.KEY_DATABASE_POOL + '.' + getPool() + ".password");
}
/**
* Returns the user of the database from the properties.
*
* @return the database user used by the opencms core
*/
public String getDbWorkUser() {
String user = getExtProperty(CmsDbPoolV11.KEY_DATABASE_POOL + '.' + getPool() + ".user");
if (CmsStringUtil.isEmptyOrWhitespaceOnly(user)) {
return getDbCreateUser();
}
return user;
}
/**
* Returns the default content encoding.
* @return String
*/
public String getDefaultContentEncoding() {
return getExtProperty("defaultContentEncoding");
}
/**
* Returns the name of the default web application, configured in web.xml
.
*
* By default this is "ROOT"
.
*
* @return the name of the default web application, configured in web.xml
*/
public String getDefaultWebApplication() {
return m_defaultWebApplication;
}
/**
* Returns the display string for a given module.
*
* @param module a module
*
* @return the display string for the given module
*/
public String getDisplayForModule(CmsModule module) {
String name = module.getNiceName();
String group = module.getGroup();
String version = module.getVersion().getVersion();
String display = name;
if (CmsStringUtil.isNotEmptyOrWhitespaceOnly(group)) {
display = group + ": " + display;
}
if (CmsStringUtil.isNotEmptyOrWhitespaceOnly(version)) {
display += " (" + version + ")";
}
return display;
}
/**
* Returns the error messages.
*
* @return a vector of error messages
*/
public List getErrors() {
return m_errors;
}
/**
* Returns the mac ethernet address.
*
* @return the mac ethernet addess
*/
public String getEthernetAddress() {
if (m_ethernetAddress == null) {
String address = getExtProperty("server.ethernet.address");
m_ethernetAddress = CmsStringUtil.isNotEmpty(address) ? address : CmsStringUtil.getEthernetAddress();
}
return m_ethernetAddress;
}
/**
* Returns the fullDatabaseKey.
*
* @return the fullDatabaseKey
*/
public String getFullDatabaseKey() {
if (m_fullDatabaseKey == null) {
m_fullDatabaseKey = getExtProperty("db.name") + "_sql";
}
if (CmsStringUtil.isEmpty(m_fullDatabaseKey) || m_fullDatabaseKey.equals("_sql")) {
m_fullDatabaseKey = getSortedDatabases().get(0) + "_sql";
}
return m_fullDatabaseKey;
}
/**
* Generates the HTML code for the drop down for db selection.
*
* @return the generated HTML
*/
public String getHtmlForDbSelection() {
StringBuffer buf = new StringBuffer(2048);
buf.append(
"");
return buf.toString();
}
/**
* Returns a help image icon tag to display a help text in the setup wizard.
*
* @param id the id of the desired help div
* @param pathPrefix the path prefix to the image
* @return the HTML part for the help icon or an empty String, if the part was not found
*/
public String getHtmlHelpIcon(String id, String pathPrefix) {
String value = m_htmlProps.getProperty("C_HELP_IMG");
if (value == null) {
return "";
} else {
value = CmsStringUtil.substitute(value, "$replace$", id);
return CmsStringUtil.substitute(value, "$path$", pathPrefix);
}
}
/**
* Returns the specified HTML part of the HTML property file to create the output.
*
* @param part the name of the desired part
* @return the HTML part or an empty String, if the part was not found
*/
public String getHtmlPart(String part) {
return getHtmlPart(part, "");
}
/**
* Returns the specified HTML part of the HTML property file to create the output.
*
* @param part the name of the desired part
* @param replaceString String which is inserted in the found HTML part at the location of "$replace$"
* @return the HTML part or an empty String, if the part was not found
*/
public String getHtmlPart(String part, String replaceString) {
String value = m_htmlProps.getProperty(part);
if (value == null) {
return "";
} else {
return CmsStringUtil.substitute(value, "$replace$", replaceString);
}
}
/**
* Returns the path to the /WEB-INF/lib folder.
*
* @return the path to the /WEB-INF/lib folder
*/
public String getLibFolder() {
return getWebAppRfsPath() + CmsSystemInfo.FOLDER_WEBINF + FOLDER_LIB;
}
/**
* Returns the name of the log file.
*
* @return the name of the log file
*/
public String getLogName() {
return m_logFile;
}
/**
* Returns a map with lists of dependent module package names keyed by module package names.
*
* @return a map with lists of dependent module package names keyed by module package names
*/
public Map> getModuleDependencies() {
if ((m_moduleDependencies == null) || m_moduleDependencies.isEmpty()) {
try {
// open the folder "/WEB-INF/packages/modules/"
m_moduleDependencies = CmsModuleManager.buildDepsForAllModules(getModuleFolder(), true);
} catch (CmsConfigurationException e) {
throw new CmsRuntimeException(e.getMessageContainer());
}
}
return m_moduleDependencies;
}
/**
* Returns the absolute path to the module root folder.
*
* @return the absolute path to the module root folder
*/
public String getModuleFolder() {
return new StringBuffer(m_webAppRfsPath).append(m_modulesFolder).toString();
}
/**
* Returns A list with the package names of the modules to be installed.
*
* @return A list with the package names of the modules to be installed
*/
public List getModulesToInstall() {
if ((m_installModules == null) || m_installModules.isEmpty()) {
return Collections.emptyList();
}
return Collections.unmodifiableList(m_installModules);
}
/**
* Gets the default pool.
*
* @return name of the default pool
*/
public String getPool() {
return CmsStringUtil.splitAsArray(getExtProperty("db.pools"), ",")[0];
}
/**
* Returns the parameter configuration.
*
* @return the parameter configuration
*/
public CmsParameterConfiguration getProperties() {
return m_configuration;
}
/**
* Returns the replacer.
*
* @return the replacer
*/
public Map getReplacer() {
return m_replacer;
}
/**
* Return the OpenCms server name.
*
* @return the OpenCms server name
*/
public String getServerName() {
return getExtProperty("server.name");
}
/**
* Returns the initial servlet configuration.
*
* @return the initial servlet configuration
*/
public ServletConfig getServletConfig() {
return m_servletConfig;
}
/**
* Returns the OpenCms servlet mapping, configured in web.xml
.
*
* By default this is "/opencms/*"
.
*
* @return the OpenCms servlet mapping, configured in web.xml
*/
public String getServletMapping() {
return m_servletMapping;
}
/**
* Returns a sorted list with they keys (e.g. "mysql", "generic" or "oracle") of all available
* database server setups found in "/setup/database/" sorted by their ranking property.
*
* @return a sorted list with they keys (e.g. "mysql", "generic" or "oracle") of all available database server setups
*/
public List getSortedDatabases() {
if (m_sortedDatabaseKeys == null) {
List databases = m_databaseKeys;
List sortedDatabases = new ArrayList(databases.size());
SortedMap mappedDatabases = new TreeMap();
for (int i = 0; i < databases.size(); i++) {
String key = databases.get(i);
Integer ranking = Integer.valueOf(0);
try {
ranking = Integer.valueOf(getDbProperty(key + ".ranking"));
} catch (Exception e) {
// ignore
}
mappedDatabases.put(ranking, key);
}
while (mappedDatabases.size() > 0) {
// get database with highest ranking
Integer key = mappedDatabases.lastKey();
String database = mappedDatabases.get(key);
sortedDatabases.add(database);
mappedDatabases.remove(key);
}
m_sortedDatabaseKeys = sortedDatabases;
}
return m_sortedDatabaseKeys;
}
/**
* Returns the absolute path to the OpenCms home directory.
*
* @return the path to the OpenCms home directory
*/
public String getWebAppRfsPath() {
return m_webAppRfsPath;
}
/**
* Checks if the setup wizard is enabled.
*
* @return true if the setup wizard is enables, false otherwise
*/
public boolean getWizardEnabled() {
return Boolean.valueOf(getExtProperty("wizard.enabled")).booleanValue();
}
/**
* Returns the workplace import thread.
*
* @return the workplace import thread
*/
public CmsSetupWorkplaceImportThread getWorkplaceImportThread() {
return m_workplaceImportThread;
}
/**
* Return the OpenCms workplace site.
*
* @return the OpenCms workplace site
*/
public String getWorkplaceSite() {
return getExtProperty("site.workplace");
}
/**
* Returns the xml Helper object.
*
* @return the xml Helper object
*/
public CmsSetupXmlHelper getXmlHelper() {
if (m_xmlHelper == null) {
// lazzy initialization
m_xmlHelper = new CmsSetupXmlHelper(getConfigRfsPath());
}
return m_xmlHelper;
}
/**
* Returns true if there is an index.html file in the default site after the module import.
*
* @return true if there is an index.html file
*/
public boolean hasIndexHtml() {
return m_hasIndexHtml;
}
/**
* Returns the html code for component selection.
*
* @return html code
*/
public String htmlComponents() {
StringBuffer html = new StringBuffer(1024);
Iterator itComponents = CmsCollectionsGenericWrapper. list(
m_components.elementList()).iterator();
while (itComponents.hasNext()) {
CmsSetupComponent component = itComponents.next();
html.append(htmlComponent(component));
}
return html.toString();
}
/**
* Returns html code for the module descriptions in help ballons.
*
* @return html code
*/
public String htmlModuleHelpDescriptions() {
StringBuffer html = new StringBuffer(1024);
Iterator itModules = sortModules(getAvailableModules().values()).iterator();
for (int i = 0; itModules.hasNext(); i++) {
String moduleName = itModules.next();
CmsModule module = getAvailableModules().get(moduleName);
if (CmsStringUtil.isNotEmptyOrWhitespaceOnly(module.getDescription())) {
html.append(getHtmlPart("C_HELP_START", "" + i));
html.append(module.getDescription());
html.append("\n");
html.append(getHtmlPart("C_HELP_END"));
html.append("\n");
}
}
return html.toString();
}
/**
* Returns html for displaying a module selection box.
*
* @return html code
*/
public String htmlModules() {
StringBuffer html = new StringBuffer(1024);
Iterator itModules = sortModules(getAvailableModules().values()).iterator();
for (int i = 0; itModules.hasNext(); i++) {
String moduleName = itModules.next();
CmsModule module = getAvailableModules().get(moduleName);
html.append(htmlModule(module, i));
}
return html.toString();
}
/**
* Installed all modules that have been set using {@link #setInstallModules(String)}.
*
* This method is invoked as a shell command.
*
* @throws Exception if something goes wrong
*/
public void importModulesFromSetupBean() throws Exception {
// read here how the list of modules to be installed is passed from the setup bean to the
// setup thread, and finally to the shell process that executes the setup script:
// 1) the list with the package names of the modules to be installed is saved by setInstallModules
// 2) the setup thread gets initialized in a JSP of the setup wizard
// 3) the instance of the setup bean is passed to the setup thread by setAdditionalShellCommand
// 4) the setup bean is passed to the shell by startSetup
// 5) because the setup bean implements I_CmsShellCommands, the shell constructor can pass the shell's CmsObject back to the setup bean
// 6) thus, the setup bean can do things with the Cms
if ((m_cms != null) && (m_installModules != null)) {
for (int i = 0; i < m_installModules.size(); i++) {
String filename = m_moduleFilenames.get(m_installModules.get(i));
try {
importModuleFromDefault(filename);
} catch (Exception e) {
// log a exception during module import, but make sure the next module is still imported
e.printStackTrace(System.err);
}
}
m_hasIndexHtml = false;
try {
m_cms.readResource("/index.html");
m_hasIndexHtml = true;
} catch (Exception e) {
}
}
}
/**
* Creates a new instance of the setup Bean from a JSP page.
*
* @param pageContext the JSP's page context
*/
public void init(PageContext pageContext) {
ServletContext servCtx = pageContext.getServletContext();
ServletConfig servConfig = pageContext.getServletConfig();
init(servCtx, servConfig);
}
public void init(ServletContext servCtx, ServletConfig servConfig) {
// check for OpenCms installation directory path
String webAppRfsPath = servConfig.getServletContext().getRealPath("/");
// read the the OpenCms servlet mapping from the servlet context parameters
String servletMapping = servCtx.getInitParameter(OpenCmsServlet.SERVLET_PARAM_OPEN_CMS_SERVLET);
// read the the default context name from the servlet context parameters
String defaultWebApplication = servCtx.getInitParameter(OpenCmsServlet.SERVLET_PARAM_DEFAULT_WEB_APPLICATION);
m_servletConfig = servConfig;
m_contextPath = servCtx.getContextPath();
init(webAppRfsPath, servletMapping, defaultWebApplication);
}
/**
* Creates a new instance of the setup Bean.
*
* @param webAppRfsPath path to the OpenCms web application
* @param servletMapping the OpenCms servlet mapping
* @param defaultWebApplication the name of the default web application
*
*/
public void init(String webAppRfsPath, String servletMapping, String defaultWebApplication) {
try {
// explicit set to null to overwrite exiting values from session
m_availableModules = null;
m_fullDatabaseKey = null;
m_databaseKey = null;
m_databaseKeys = null;
m_databaseProperties = null;
m_configuration = null;
m_installModules = null;
m_moduleDependencies = null;
m_sortedDatabaseKeys = null;
m_moduleFilenames = null;
if (servletMapping == null) {
servletMapping = "/opencms/*";
}
if (defaultWebApplication == null) {
defaultWebApplication = "ROOT";
}
m_servletMapping = servletMapping;
m_defaultWebApplication = defaultWebApplication;
setWebAppRfsPath(webAppRfsPath);
m_errors = new ArrayList();
if (CmsStringUtil.isNotEmptyOrWhitespaceOnly(webAppRfsPath)) {
m_configuration = new CmsParameterConfiguration(m_configRfsPath + CmsSystemInfo.FILE_PROPERTIES);
readDatabaseConfig();
}
if (m_workplaceImportThread != null) {
if (m_workplaceImportThread.isAlive()) {
m_workplaceImportThread.kill();
}
m_workplaceImportThread = null;
m_newLoggingOffset = 0;
m_oldLoggingOffset = 0;
}
} catch (Exception e) {
e.printStackTrace();
m_errors.add(e.toString());
}
}
/**
* This method reads the properties from the htmlmsg.property file
* and sets the HTML part properties with the matching values.
*/
public void initHtmlParts() {
if (m_htmlProps != null) {
// html already initialized
return;
}
try {
m_htmlProps = new Properties();
m_htmlProps.load(getClass().getClassLoader().getResourceAsStream(HTML_MESSAGE_FILE));
} catch (Exception e) {
e.printStackTrace();
m_errors.add(e.toString());
}
}
/**
* @see org.opencms.main.I_CmsShellCommands#initShellCmsObject(org.opencms.file.CmsObject, org.opencms.main.CmsShell)
*/
public void initShellCmsObject(CmsObject cms, CmsShell shell) {
m_cms = cms;
m_shell = shell;
}
/**
* Returns the autoMode.
*
* @return the autoMode
*/
public boolean isAutoMode() {
return m_autoMode;
}
/**
* Over simplistic helper to compare two strings to check radio buttons.
*
* @param value1 the first value
* @param value2 the second value
* @return "checked" if both values are equal, the empty String "" otherwise
*/
public String isChecked(String value1, String value2) {
if ((value1 == null) || (value2 == null)) {
return "";
}
if (value1.trim().equalsIgnoreCase(value2.trim())) {
return "checked";
}
return "";
}
/**
* Returns true if this setup bean is correctly initialized.
*
* @return true if this setup bean is correctly initialized
*/
public boolean isInitialized() {
return m_configuration != null;
}
/**
* Returns js code with array definition for the available component dependencies.
*
* @return js code
*/
public String jsComponentDependencies() {
List components = CmsCollectionsGenericWrapper.list(m_components.elementList());
Map> componentDependencies = buildDepsForAllComponents();
StringBuffer jsCode = new StringBuffer(1024);
jsCode.append("\t// an array holding the dependent components for the n-th component\n");
jsCode.append("\tvar componentDependencies = new Array(");
jsCode.append(components.size());
jsCode.append(");\n");
for (int i = 0; i < components.size(); i++) {
CmsSetupComponent component = components.get(i);
List dependencies = componentDependencies.get(component.getId());
jsCode.append("\tcomponentDependencies[" + i + "] = new Array(");
if (dependencies != null) {
for (int j = 0; j < dependencies.size(); j++) {
jsCode.append("\"" + dependencies.get(j) + "\"");
if (j < (dependencies.size() - 1)) {
jsCode.append(", ");
}
}
}
jsCode.append(");\n");
}
jsCode.append("\n\n");
return jsCode.toString();
}
/**
* Returns js code with array definition for the component modules.
*
* @return js code
*/
public String jsComponentModules() {
List components = CmsCollectionsGenericWrapper.list(m_components.elementList());
StringBuffer jsCode = new StringBuffer(1024);
jsCode.append("\t// an array holding the components modules\n");
jsCode.append("\tvar componentModules = new Array(");
jsCode.append(components.size());
jsCode.append(");\n");
for (int i = 0; i < components.size(); i++) {
CmsSetupComponent component = components.get(i);
jsCode.append("\tcomponentModules[" + i + "] = \"");
List modules = getComponentModules(component);
for (int j = 0; j < modules.size(); j++) {
jsCode.append(modules.get(j));
if (j < (modules.size() - 1)) {
jsCode.append("|");
}
}
jsCode.append("\";\n");
}
jsCode.append("\n\n");
return jsCode.toString();
}
/**
* Returns js code with array definition for the available components names.
*
* @return js code
*/
public String jsComponentNames() {
StringBuffer jsCode = new StringBuffer(1024);
jsCode.append("\t// an array from 1...n holding the component names\n");
jsCode.append("\tvar componentNames = new Array(");
jsCode.append(m_components.elementList().size());
jsCode.append(");\n");
for (int i = 0; i < m_components.elementList().size(); i++) {
CmsSetupComponent component = m_components.elementList().get(i);
jsCode.append("\tcomponentNames[" + i + "] = \"" + component.getId() + "\";\n");
}
jsCode.append("\n\n");
return jsCode.toString();
}
/**
* Returns js code with array definition for the available module dependencies.
*
* @return js code
*/
public String jsModuleDependencies() {
List moduleNames = sortModules(getAvailableModules().values());
StringBuffer jsCode = new StringBuffer(1024);
jsCode.append("\t// an array holding the dependent modules for the n-th module\n");
jsCode.append("\tvar moduleDependencies = new Array(");
jsCode.append(moduleNames.size());
jsCode.append(");\n");
for (int i = 0; i < moduleNames.size(); i++) {
String moduleName = moduleNames.get(i);
List dependencies = getModuleDependencies().get(moduleName);
jsCode.append("\tmoduleDependencies[" + i + "] = new Array(");
if (dependencies != null) {
for (int j = 0; j < dependencies.size(); j++) {
jsCode.append("\"" + dependencies.get(j) + "\"");
if (j < (dependencies.size() - 1)) {
jsCode.append(", ");
}
}
}
jsCode.append(");\n");
}
jsCode.append("\n\n");
return jsCode.toString();
}
/**
* Returns js code with array definition for the available module names.
*
* @return js code
*/
public String jsModuleNames() {
List moduleNames = sortModules(getAvailableModules().values());
StringBuffer jsCode = new StringBuffer(1024);
jsCode.append("\t// an array from 1...n holding the module package names\n");
jsCode.append("\tvar modulePackageNames = new Array(");
jsCode.append(moduleNames.size());
jsCode.append(");\n");
for (int i = 0; i < moduleNames.size(); i++) {
String moduleName = moduleNames.get(i);
jsCode.append("\tmodulePackageNames[" + i + "] = \"" + moduleName + "\";\n");
}
jsCode.append("\n\n");
return jsCode.toString();
}
/**
* Locks (i.e. disables) the setup wizard.
*
*/
public void lockWizard() {
setExtProperty("wizard.enabled", CmsStringUtil.FALSE);
}
/**
* Prepares step 10 of the setup wizard.
*/
public void prepareStep10() {
if (isInitialized()) {
// lock the wizard for further use
lockWizard();
// save Properties to file "opencms.properties"
saveProperties(getProperties(), CmsSystemInfo.FILE_PROPERTIES, false);
}
}
/**
* Prepares step 8 of the setup wizard.
*
* @return true if the workplace should be imported
*/
public boolean prepareStep8() {
if (isInitialized()) {
try {
checkEthernetAddress();
// backup the XML configuration
backupConfiguration(
CmsImportExportConfiguration.DEFAULT_XML_FILE_NAME,
CmsImportExportConfiguration.DEFAULT_XML_FILE_NAME + CmsConfigurationManager.POSTFIX_ORI);
backupConfiguration(
CmsModuleConfiguration.DEFAULT_XML_FILE_NAME,
CmsModuleConfiguration.DEFAULT_XML_FILE_NAME + CmsConfigurationManager.POSTFIX_ORI);
backupConfiguration(
CmsSearchConfiguration.DEFAULT_XML_FILE_NAME,
CmsSearchConfiguration.DEFAULT_XML_FILE_NAME + CmsConfigurationManager.POSTFIX_ORI);
backupConfiguration(
CmsSystemConfiguration.DEFAULT_XML_FILE_NAME,
CmsSystemConfiguration.DEFAULT_XML_FILE_NAME + CmsConfigurationManager.POSTFIX_ORI);
backupConfiguration(
CmsVfsConfiguration.DEFAULT_XML_FILE_NAME,
CmsVfsConfiguration.DEFAULT_XML_FILE_NAME + CmsConfigurationManager.POSTFIX_ORI);
backupConfiguration(
CmsWorkplaceConfiguration.DEFAULT_XML_FILE_NAME,
CmsWorkplaceConfiguration.DEFAULT_XML_FILE_NAME + CmsConfigurationManager.POSTFIX_ORI);
backupConfiguration(
CmsConfigurationManager.DEFAULT_XML_FILE_NAME,
CmsConfigurationManager.DEFAULT_XML_FILE_NAME + CmsConfigurationManager.POSTFIX_ORI);
// save Properties to file "opencms.properties"
setDatabase(m_databaseKey);
saveProperties(getProperties(), CmsSystemInfo.FILE_PROPERTIES, true);
// if has sql driver the sql scripts will be eventualy executed
CmsSetupTestResult testResult = new CmsSetupTestSimapi().execute(this);
if (testResult.getResult().equals(I_CmsSetupTest.RESULT_FAILED)) {
// "/opencms/vfs/resources/resourceloaders/loader[@class='org.opencms.loader.CmsImageLoader']/param[@name='image.scaling.enabled']";
StringBuffer xp = new StringBuffer(256);
xp.append("/").append(CmsConfigurationManager.N_ROOT);
xp.append("/").append(CmsVfsConfiguration.N_VFS);
xp.append("/").append(CmsVfsConfiguration.N_RESOURCES);
xp.append("/").append(CmsVfsConfiguration.N_RESOURCELOADERS);
xp.append("/").append(CmsVfsConfiguration.N_LOADER);
xp.append("[@").append(I_CmsXmlConfiguration.A_CLASS);
xp.append("='").append(CmsImageLoader.class.getName());
xp.append("']/").append(I_CmsXmlConfiguration.N_PARAM);
xp.append("[@").append(I_CmsXmlConfiguration.A_NAME);
xp.append("='").append(CmsImageLoader.CONFIGURATION_SCALING_ENABLED).append("']");
getXmlHelper().setValue(
CmsVfsConfiguration.DEFAULT_XML_FILE_NAME,
xp.toString(),
Boolean.FALSE.toString());
}
// /opencms/system/sites/workplace-server
StringBuffer xp = new StringBuffer(256);
xp.append("/").append(CmsConfigurationManager.N_ROOT);
xp.append("/").append(CmsSitesConfiguration.N_SITES);
xp.append("/").append(CmsSitesConfiguration.N_WORKPLACE_SERVER);
getXmlHelper().setValue(CmsSitesConfiguration.DEFAULT_XML_FILE_NAME, xp.toString(), getWorkplaceSite());
// /opencms/system/sites/site[@uri='/sites/default/']/@server
xp = new StringBuffer(256);
xp.append("/").append(CmsConfigurationManager.N_ROOT);
xp.append("/").append(CmsSitesConfiguration.N_SITES);
xp.append("/").append(I_CmsXmlConfiguration.N_SITE);
xp.append("[@").append(I_CmsXmlConfiguration.A_URI);
xp.append("='").append(CmsResource.VFS_FOLDER_SITES);
xp.append("/default/']/@").append(CmsSitesConfiguration.A_SERVER);
getXmlHelper().setValue(CmsSitesConfiguration.DEFAULT_XML_FILE_NAME, xp.toString(), getWorkplaceSite());
getXmlHelper().writeAll();
} catch (Exception e) {
if (LOG.isErrorEnabled()) {
LOG.error(e.getLocalizedMessage(), e);
}
}
}
return true;
}
/**
* Prepares step 8b of the setup wizard.
*/
public void prepareStep8b() {
if (!isInitialized()) {
return;
}
if ((m_workplaceImportThread != null) && (m_workplaceImportThread.isFinished())) {
// setup is already finished, just wait for client to collect final data
return;
}
if (m_workplaceImportThread == null) {
m_workplaceImportThread = new CmsSetupWorkplaceImportThread(this);
}
if (!m_workplaceImportThread.isAlive() && !m_workplaceImportThread.getState().equals(Thread.State.TERMINATED)) {
m_workplaceImportThread.start();
}
}
/**
* Generates the output for step 8b of the setup wizard.
*
* @param out the JSP print stream
* @throws IOException in case errors occur while writing to "out"
*/
public void prepareStep8bOutput(JspWriter out) throws IOException {
if ((m_workplaceImportThread == null) || (m_workplaceImportThread.getLoggingThread() == null)) {
return;
}
m_oldLoggingOffset = m_newLoggingOffset;
m_newLoggingOffset = m_workplaceImportThread.getLoggingThread().getMessages().size();
if (isInitialized()) {
for (int i = m_oldLoggingOffset; i < m_newLoggingOffset; i++) {
String str = m_workplaceImportThread.getLoggingThread().getMessages().get(i).toString();
str = CmsEncoder.escapeWBlanks(str, CmsEncoder.ENCODING_UTF_8);
out.println("output[" + (i - m_oldLoggingOffset) + "] = \"" + str + "\";");
}
} else {
out.println("output[0] = 'ERROR';");
}
boolean threadFinished = m_workplaceImportThread.isFinished();
boolean allWritten = m_oldLoggingOffset >= m_workplaceImportThread.getLoggingThread().getMessages().size();
out.println("function initThread() {");
if (isInitialized()) {
out.print("send();");
if (threadFinished && allWritten) {
out.println("setTimeout('top.display.finish()', 1000);");
} else {
int timeout = 5000;
if (getWorkplaceImportThread().getLoggingThread().getMessages().size() < 20) {
timeout = 2000;
}
out.println("setTimeout('location.reload()', " + timeout + ");");
}
}
out.println("}");
}
/**
* Saves properties to specified file.
*
* @param properties the properties to be saved
* @param file the file to save the properties to
* @param backup if true, create a backupfile
*/
public void saveProperties(CmsParameterConfiguration properties, String file, boolean backup) {
if (new File(m_configRfsPath + file).isFile()) {
String backupFile = file + CmsConfigurationManager.POSTFIX_ORI;
String tempFile = file + ".tmp";
m_errors.clear();
if (backup) {
// make a backup copy
copyFile(file, FOLDER_BACKUP + backupFile);
}
//save to temporary file
copyFile(file, tempFile);
// save properties
save(properties, tempFile, file, null);
// delete temp file
File temp = new File(m_configRfsPath + tempFile);
temp.delete();
} else {
m_errors.add("No valid file: " + file + "\n");
}
}
/**
* Saves properties to specified file.
*
* @param properties the properties to be saved
* @param file the file to save the properties to
* @param backup if true, create a backupfile
* @param forceWrite the keys for the properties which should always be written, even if they don't exist in the configuration file
*/
public void saveProperties(
CmsParameterConfiguration properties,
String file,
boolean backup,
Set forceWrite) {
if (new File(m_configRfsPath + file).isFile()) {
String backupFile = file + CmsConfigurationManager.POSTFIX_ORI;
String tempFile = file + ".tmp";
m_errors.clear();
if (backup) {
// make a backup copy
copyFile(file, FOLDER_BACKUP + backupFile);
}
//save to temporary file
copyFile(file, tempFile);
// save properties
save(properties, tempFile, file, forceWrite);
// delete temp file
File temp = new File(m_configRfsPath + tempFile);
temp.delete();
} else {
m_errors.add("No valid file: " + file + "\n");
}
}
/**
* Sets the autoMode.
*
* @param autoMode the autoMode to set
*/
public void setAutoMode(boolean autoMode) {
m_autoMode = autoMode;
}
/**
* Sets the database drivers to the given value.
*
* @param databaseKey the key of the selected database server (e.g. "mysql", "generic" or "oracle")
*/
public void setDatabase(String databaseKey) {
m_databaseKey = databaseKey;
String vfsDriver;
String userDriver;
String projectDriver;
String historyDriver;
String subscriptionDriver;
String sqlManager;
vfsDriver = getDbProperty(m_databaseKey + ".vfs.driver");
userDriver = getDbProperty(m_databaseKey + ".user.driver");
projectDriver = getDbProperty(m_databaseKey + ".project.driver");
historyDriver = getDbProperty(m_databaseKey + ".history.driver");
subscriptionDriver = getDbProperty(m_databaseKey + ".subscription.driver");
sqlManager = getDbProperty(m_databaseKey + ".sqlmanager");
// set the db properties
setExtProperty("db.name", m_databaseKey);
setExtProperty("db.vfs.driver", vfsDriver);
setExtProperty("db.vfs.sqlmanager", sqlManager);
setExtProperty("db.user.driver", userDriver);
setExtProperty("db.user.sqlmanager", sqlManager);
setExtProperty("db.project.driver", projectDriver);
setExtProperty("db.project.sqlmanager", sqlManager);
setExtProperty("db.history.driver", historyDriver);
setExtProperty("db.history.sqlmanager", sqlManager);
setExtProperty("db.subscription.driver", subscriptionDriver);
setExtProperty("db.subscription.sqlmanager", sqlManager);
Properties dbProps = getDatabaseProperties().get(databaseKey);
String prefix = "additional.";
String dbPropBlock = "";
for (Map.Entry