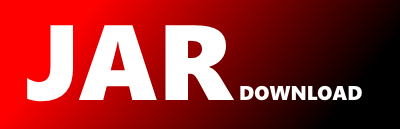
org.opencms.setup.xml.v8.CmsXmlAddADESearch Maven / Gradle / Ivy
Show all versions of opencms-setup Show documentation
/*
* This library is part of OpenCms -
* the Open Source Content Management System
*
* Copyright (c) Alkacon Software GmbH (http://www.alkacon.com)
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* For further information about Alkacon Software GmbH, please see the
* company website: http://www.alkacon.com
*
* For further information about OpenCms, please see the
* project website: http://www.opencms.org
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*/
package org.opencms.setup.xml.v8;
import org.opencms.configuration.CmsConfigurationManager;
import org.opencms.configuration.CmsSearchConfiguration;
import org.opencms.configuration.I_CmsXmlConfiguration;
import org.opencms.search.CmsVfsIndexer;
import org.opencms.search.fields.CmsSearchField;
import org.opencms.search.fields.CmsSearchFieldConfiguration;
import org.opencms.search.fields.CmsSearchFieldMapping;
import org.opencms.search.galleries.CmsGallerySearchAnalyzer;
import org.opencms.search.galleries.CmsGallerySearchFieldConfiguration;
import org.opencms.search.galleries.CmsGallerySearchFieldMapping;
import org.opencms.search.galleries.CmsGallerySearchIndex;
import org.opencms.setup.xml.A_CmsXmlSearch;
import org.opencms.setup.xml.CmsSetupXmlHelper;
import org.opencms.setup.xml.CmsXmlUpdateAction;
import java.io.StringReader;
import java.util.ArrayList;
import java.util.LinkedHashMap;
import java.util.List;
import java.util.Map;
import org.dom4j.Document;
import org.dom4j.DocumentException;
import org.dom4j.Element;
import org.dom4j.Node;
import org.dom4j.io.SAXReader;
/**
* Adds the gallery search nodes.
*
* @since 8.0.0
*/
public class CmsXmlAddADESearch extends A_CmsXmlSearch {
/**
* Action to add the gallery modules index source.
*/
public static class CmsAddGalleryModuleIndexSourceAction extends CmsXmlUpdateAction {
/**
* @see org.opencms.setup.xml.CmsXmlUpdateAction#executeUpdate(org.dom4j.Document, java.lang.String, boolean)
*/
@Override
public boolean executeUpdate(Document doc, String xpath, boolean forReal) {
Element node = (Element)doc.selectSingleNode("/opencms/search/indexsources");
if (!node.selectNodes("indexsource[name='gallery_modules_source']").isEmpty()) {
return false;
}
String galleryModulesSource = " \n"
+ " gallery_modules_source \n"
+ " \n"
+ " \n"
+ " /system/modules/ \n"
+ " \n"
+ " \n"
+ " xmlcontent-galleries \n"
+ " \n"
+ " \n";
try {
Element sourceElem = createElementFromXml(galleryModulesSource);
node.add(sourceElem);
return true;
} catch (DocumentException e) {
System.err.println("Failed to add gallery_modules_source");
return false;
}
}
}
/**
* An XML update action which adds the /system/galleries folder to the gallery index source.
*/
public static class CmsAddIndexSourceResourceAction extends CmsXmlUpdateAction {
/**
* @see org.opencms.setup.xml.CmsXmlUpdateAction#executeUpdate(org.dom4j.Document, java.lang.String, boolean)
*/
@Override
public boolean executeUpdate(Document doc, String xpath, boolean forReal) {
if (!forReal) {
CmsSetupXmlHelper.setValue(
doc,
"/opencms/search/indexsources/indexsource[name='gallery_source']/resources/resource[text()='/system/galleries/']",
"/system/galleries/");
return true;
}
Element indexSourceResources = (Element)doc.selectSingleNode("/opencms/search/indexsources/indexsource[name='gallery_source']/resources");
if (indexSourceResources == null) {
return false;
}
Element source = (Element)indexSourceResources.selectSingleNode("resource[text()='/system/galleries/']");
if (source != null) {
return false;
}
Element resourceElement = indexSourceResources.addElement("resource");
resourceElement.addText("/system/galleries/");
return true;
}
}
/**
* Action for updating the office document types in the index sources.
*/
public static final class CmsIndexSourceTypeUpdateAction extends CmsXmlUpdateAction {
/**
* @see org.opencms.setup.xml.CmsXmlUpdateAction#executeUpdate(org.dom4j.Document, java.lang.String, boolean)
*/
@SuppressWarnings("unchecked")
@Override
public boolean executeUpdate(Document doc, String xpath, boolean forReal) {
List nodes = doc.selectNodes("/opencms/search/indexsources/indexsource");
boolean result = false;
for (Node node : nodes) {
if (containsOldType(node)) {
result = true;
removeTypes(node);
addNewTypes(node);
}
}
return result;
}
/**
* Adds new office document types.
*
* @param node the node to add the document types to
*/
protected void addNewTypes(Node node) {
Element element = (Element)(node.selectSingleNode("documenttypes-indexed"));
for (String type : new String[] {"openoffice", "msoffice-ole2", "msoffice-ooxml"}) {
element.addElement("name").addText(type);
}
}
/**
* Checks whether a node contains the old office document types.
*
* @param node the node which should be checked
*
* @return true if the node contains old office document types
*/
protected boolean containsOldType(Node node) {
@SuppressWarnings("unchecked")
List nodes = node.selectNodes("documenttypes-indexed/name[text()='msword' or text()='msexcel' or text()='mspowerpoint']");
return !nodes.isEmpty();
}
/**
* Removes the office document types from a node.
*
* @param node the node from which to remove the document types
*/
protected void removeTypes(Node node) {
@SuppressWarnings("unchecked")
List nodes = node.selectNodes("documenttypes-indexed/name[text()='msword' or text()='msexcel' or text()='mspowerpoint' or text()='msoffice-ooxml' or text()='openoffice' or text()='msoffice-ole2']");
for (Node nodeToRemove : nodes) {
nodeToRemove.detach();
}
}
}
/**
* An XML update action which replaces an element given by an XPath with some other XML element.
*/
class ElementReplaceAction extends CmsXmlUpdateAction {
/**
* The XML which should be used as a replacement (as a string).
*/
private String m_replacementXml;
/**
* The xpath of the element to replace.
*/
private String m_xpath;
/**
* Creates a new instance.
*
* @param xpath the xpath of the element to replace
* @param replacementXml the replacement xml
*/
public ElementReplaceAction(String xpath, String replacementXml) {
m_xpath = xpath;
m_replacementXml = replacementXml;
}
/**
* @see org.opencms.setup.xml.CmsXmlUpdateAction#executeUpdate(org.dom4j.Document, java.lang.String, boolean)
*/
@Override
public boolean executeUpdate(Document doc, String xpath, boolean forReal) {
if (!forReal) {
return true;
}
Node node = doc.selectSingleNode(m_xpath);
if (node != null) {
Element parent = node.getParent();
node.detach();
try {
Element element = createElementFromXml(m_replacementXml);
parent.add(element);
return true;
} catch (DocumentException e) {
e.printStackTrace(System.out);
return false;
}
} else {
return false;
}
}
}
/** A map from xpaths to XML update actions.
*/
private Map m_actions;
/**
* Creates a dom4j element from an XML string.
*
* @param xml the xml string
* @return the dom4j element
*
* @throws DocumentException if the XML parsing fails
*/
public static org.dom4j.Element createElementFromXml(String xml) throws DocumentException {
SAXReader reader = new SAXReader();
Document newNodeDocument = reader.read(new StringReader(xml));
return newNodeDocument.getRootElement();
}
/**
* @see org.opencms.setup.xml.I_CmsSetupXmlUpdate#getName()
*/
public String getName() {
return "Add the ADE containerpage and gallery search nodes";
}
/**
* @see org.opencms.setup.xml.A_CmsSetupXmlUpdate#executeUpdate(org.dom4j.Document, java.lang.String, boolean)
*/
@Override
protected boolean executeUpdate(Document document, String xpath, boolean forReal) {
CmsXmlUpdateAction action = m_actions.get(xpath);
if (action == null) {
return false;
}
return action.executeUpdate(document, xpath, forReal);
}
/**
* @see org.opencms.setup.xml.A_CmsSetupXmlUpdate#getCommonPath()
*/
@Override
protected String getCommonPath() {
// /opencms/search
return new StringBuffer("/").append(CmsConfigurationManager.N_ROOT).append("/").append(
CmsSearchConfiguration.N_SEARCH).toString();
}
/**
* @see org.opencms.setup.xml.A_CmsSetupXmlUpdate#getXPathsToUpdate()
*/
@Override
protected List getXPathsToUpdate() {
if (m_actions == null) {
initActions();
}
return new ArrayList(m_actions.keySet());
}
/**
* Builds the xpath for the documenttypes node.
*
* @return the xpath for the documenttypes node
*/
private String buildXpathForDoctypes() {
return getCommonPath() + "/" + CmsSearchConfiguration.N_DOCUMENTTYPES;
}
/**
* Builds an xpath for a document type node in an index source.
*
* @param source the name of the index source
* @param doctype the document type
*
* @return the xpath
*/
private String buildXpathForIndexedDocumentType(String source, String doctype) {
StringBuffer xp = new StringBuffer(256);
xp.append(getCommonPath());
xp.append("/");
xp.append(CmsSearchConfiguration.N_INDEXSOURCES);
xp.append("/");
xp.append(CmsSearchConfiguration.N_INDEXSOURCE);
xp.append("[");
xp.append(I_CmsXmlConfiguration.N_NAME);
xp.append("='" + source + "']");
xp.append("/");
xp.append(CmsSearchConfiguration.N_DOCUMENTTYPES_INDEXED);
xp.append("/");
xp.append(I_CmsXmlConfiguration.N_NAME);
xp.append("[text()='" + doctype + "']");
return xp.toString();
}
/**
* Creates an action which adds an indexed type to an index source.
*
* @param type the type which should be indexed
*
* @return the update action
*/
private CmsXmlUpdateAction createIndexedTypeAction(final String type) {
return new CmsXmlUpdateAction() {
@Override
public boolean executeUpdate(Document doc, String xpath, boolean forReal) {
Node node = doc.selectSingleNode(xpath);
if (node != null) {
return false;
}
CmsSetupXmlHelper.setValue(doc, xpath + "/text()", type);
return true;
}
};
}
/**
* Initializes the map of XML update actions.
*/
private void initActions() {
m_actions = new LinkedHashMap();
StringBuffer xp;
CmsXmlUpdateAction action0 = new CmsXmlUpdateAction() {
@SuppressWarnings("unchecked")
@Override
public boolean executeUpdate(Document doc, String xpath, boolean forReal) {
Node node = doc.selectSingleNode(xpath);
org.dom4j.Element parent = node.getParent();
int position = parent.indexOf(node);
parent.remove(node);
try {
parent.elements().add(
position,
createElementFromXml(" \n"
+ " \n"
+ " generic \n"
+ " org.opencms.search.documents.CmsDocumentGeneric \n"
+ " \n"
+ " \n"
+ " * \n"
+ " \n"
+ " \n"
+ " \n"
+ " html \n"
+ " org.opencms.search.documents.CmsDocumentHtml \n"
+ " \n"
+ " text/html \n"
+ " \n"
+ " \n"
+ " plain \n"
+ " \n"
+ " \n"
+ " \n"
+ " image \n"
+ " org.opencms.search.documents.CmsDocumentGeneric \n"
+ " \n"
+ " \n"
+ " image \n"
+ " \n"
+ " \n"
+ " \n"
+ " jsp \n"
+ " org.opencms.search.documents.CmsDocumentPlainText \n"
+ " \n"
+ " \n"
+ " jsp \n"
+ " \n"
+ " \n"
+ " \n"
+ " pdf \n"
+ " org.opencms.search.documents.CmsDocumentPdf \n"
+ " \n"
+ " application/pdf \n"
+ " \n"
+ " \n"
+ " binary \n"
+ " plain \n"
+ " \n"
+ " \n"
+ " \n"
+ " rtf \n"
+ " org.opencms.search.documents.CmsDocumentRtf \n"
+ " \n"
+ " text/rtf \n"
+ " application/rtf \n"
+ " \n"
+ " \n"
+ " binary \n"
+ " plain \n"
+ " \n"
+ " \n"
+ " \n"
+ " text \n"
+ " org.opencms.search.documents.CmsDocumentPlainText \n"
+ " \n"
+ " text/html \n"
+ " text/plain \n"
+ " \n"
+ " \n"
+ " plain \n"
+ " \n"
+ " \n"
+ " \n"
+ " xmlcontent \n"
+ " org.opencms.search.documents.CmsDocumentXmlContent \n"
+ " \n"
+ " \n"
+ " * \n"
+ " \n"
+ " \n"
+ " \n"
+ " containerpage \n"
+ " org.opencms.search.documents.CmsDocumentContainerPage \n"
+ " \n"
+ " text/html \n"
+ " \n"
+ " \n"
+ " containerpage \n"
+ " \n"
+ " \n"
+ " \n"
+ " xmlpage \n"
+ " org.opencms.search.documents.CmsDocumentXmlPage \n"
+ " \n"
+ " text/html \n"
+ " \n"
+ " \n"
+ " xmlpage \n"
+ " \n"
+ " \n"
+ " \n"
+ " xmlcontent-galleries \n"
+ " org.opencms.search.galleries.CmsGalleryDocumentXmlContent \n"
+ " \n"
+ " \n"
+ " xmlcontent-galleries \n"
+ " \n"
+ " \n"
+ " \n"
+ " xmlpage-galleries \n"
+ " org.opencms.search.galleries.CmsGalleryDocumentXmlPage \n"
+ " \n"
+ " \n"
+ " xmlpage-galleries \n"
+ " \n"
+ " \n"
+ " \n"
+ " msoffice-ole2 \n"
+ " org.opencms.search.documents.CmsDocumentMsOfficeOLE2 \n"
+ " \n"
+ " application/vnd.ms-powerpoint \n"
+ " application/msword \n"
+ " application/vnd.ms-excel \n"
+ " \n"
+ " \n"
+ " binary \n"
+ " plain \n"
+ " \n"
+ " \n"
+ " \n"
+ " msoffice-ooxml \n"
+ " org.opencms.search.documents.CmsDocumentMsOfficeOOXML \n"
+ " \n"
+ " application/vnd.openxmlformats-officedocument.wordprocessingml.document \n"
+ " application/vnd.openxmlformats-officedocument.spreadsheetml.sheet \n"
+ " application/vnd.openxmlformats-officedocument.presentationml.presentation \n"
+ " \n"
+ " \n"
+ " binary \n"
+ " plain \n"
+ " \n"
+ " \n"
+ " \n"
+ " openoffice \n"
+ " org.opencms.search.documents.CmsDocumentOpenOffice \n"
+ " \n"
+ " application/vnd.oasis.opendocument.text \n"
+ " application/vnd.oasis.opendocument.spreadsheet \n"
+ " \n"
+ " \n"
+ " binary \n"
+ " plain \n"
+ " \n"
+ " \n"
+ " \n"));
} catch (DocumentException e) {
System.out.println("failed to update document types.");
}
return true;
}
};
m_actions.put(buildXpathForDoctypes(), action0);
//
//=============================================================================================================
//
CmsXmlUpdateAction action1 = new CmsXmlUpdateAction() {
@SuppressWarnings("synthetic-access")
@Override
public boolean executeUpdate(Document doc, String xpath, boolean forReal) {
Node node = doc.selectSingleNode(xpath);
if (node == null) {
createAnalyzer(doc, xpath, CmsGallerySearchAnalyzer.class, "all");
return true;
}
return false;
}
};
xp = new StringBuffer(256);
xp.append(getCommonPath());
xp.append("/");
xp.append(CmsSearchConfiguration.N_ANALYZERS);
xp.append("/");
xp.append(CmsSearchConfiguration.N_ANALYZER);
xp.append("[");
xp.append(CmsSearchConfiguration.N_CLASS);
xp.append("='").append(CmsGallerySearchAnalyzer.class.getName()).append("']");
m_actions.put(xp.toString(), action1);
//
//=============================================================================================================
//
CmsXmlUpdateAction action2 = new CmsXmlUpdateAction() {
@SuppressWarnings("synthetic-access")
@Override
public boolean executeUpdate(Document doc, String xpath, boolean forReal) {
Node node = doc.selectSingleNode(xpath);
if (node != null) {
node.detach();
}
createIndex(
doc,
xpath,
CmsGallerySearchIndex.class,
CmsGallerySearchIndex.GALLERY_INDEX_NAME,
"offline",
"Offline",
"all",
"gallery_fields",
new String[] {"gallery_source_all"});
return true;
}
};
xp = new StringBuffer(256);
xp.append(getCommonPath());
xp.append("/");
xp.append(CmsSearchConfiguration.N_INDEXES);
xp.append("/");
xp.append(CmsSearchConfiguration.N_INDEX);
xp.append("[");
xp.append(I_CmsXmlConfiguration.N_NAME);
xp.append("='").append(CmsGallerySearchIndex.GALLERY_INDEX_NAME).append("']");
m_actions.put(xp.toString(), action2);
//
//=============================================================================================================
//
CmsXmlUpdateAction action3 = new CmsXmlUpdateAction() {
@SuppressWarnings("synthetic-access")
@Override
public boolean executeUpdate(Document doc, String xpath, boolean forReal) {
Node node = doc.selectSingleNode(xpath);
if (node != null) {
return false;
}
// create doc type
createIndexSource(doc, xpath, "gallery_source", CmsVfsIndexer.class, new String[] {
"/sites/",
"/shared/",
"/system/galleries/"}, new String[] {
"xmlpage-galleries",
"xmlcontent-galleries",
"jsp",
"text",
"pdf",
"rtf",
"html",
"image",
"generic",
"openoffice",
"msoffice-ole2",
"msoffice-ooxml"});
return true;
}
};
xp = new StringBuffer(256);
xp.append(getCommonPath());
xp.append("/");
xp.append(CmsSearchConfiguration.N_INDEXSOURCES);
xp.append("/");
xp.append(CmsSearchConfiguration.N_INDEXSOURCE);
xp.append("[");
xp.append(I_CmsXmlConfiguration.N_NAME);
xp.append("='gallery_source']");
m_actions.put(xp.toString(), action3);
//
//=============================================================================================================
//
CmsXmlUpdateAction action4 = new CmsXmlUpdateAction() {
@Override
public boolean executeUpdate(Document document, String xpath, boolean forReal) {
Node node = document.selectSingleNode(xpath);
if (node != null) {
node.detach();
}
// create field config
CmsSearchFieldConfiguration fieldConf = new CmsSearchFieldConfiguration();
fieldConf.setName("gallery_fields");
fieldConf.setDescription("The standard OpenCms search index field configuration.");
CmsSearchField field = new CmsSearchField();
//
field.setName("content");
field.setStored("compress");
field.setIndexed("true");
field.setInExcerpt("true");
field.setDisplayNameForConfiguration("%(key.field.content)");
//
CmsSearchFieldMapping mapping = new CmsSearchFieldMapping();
mapping.setType("content");
field.addMapping(mapping);
fieldConf.addField(field);
//
field = new CmsSearchField();
field.setName("title-key");
field.setStored("true");
field.setIndexed("untokenized");
field.setBoost("0.0");
// Title
mapping = new CmsSearchFieldMapping();
mapping.setType("property");
mapping.setParam("Title");
field.addMapping(mapping);
fieldConf.addField(field);
//
field = new CmsSearchField();
field.setName("title");
field.setStored("false");
field.setIndexed("true");
field.setDisplayNameForConfiguration("%(key.field.title)");
// Title
mapping = new CmsSearchFieldMapping();
mapping.setType("property");
mapping.setParam("Title");
field.addMapping(mapping);
fieldConf.addField(field);
//
field = new CmsSearchField();
field.setName("description");
field.setStored("true");
field.setIndexed("true");
field.setDisplayNameForConfiguration("%(key.field.description)");
// Description
mapping = new CmsSearchFieldMapping();
mapping.setType("property");
mapping.setParam("Description");
field.addMapping(mapping);
fieldConf.addField(field);
//
field = new CmsSearchField();
field.setName("meta");
field.setStored("false");
field.setIndexed("true");
// Title
mapping = new CmsSearchFieldMapping();
mapping.setType("property");
mapping.setParam("Title");
field.addMapping(mapping);
// Description
mapping = new CmsSearchFieldMapping();
mapping.setType("property");
mapping.setParam("Description");
field.addMapping(mapping);
// name
mapping = new CmsSearchFieldMapping();
mapping.setType("attribute");
mapping.setParam("name");
field.addMapping(mapping);
fieldConf.addField(field);
//
field = new CmsSearchField();
field.setName("res_dateExpired");
field.setStored("true");
field.setIndexed("untokenized");
// dateExpired
mapping = new CmsSearchFieldMapping();
mapping.setType("attribute");
mapping.setParam("dateExpired");
field.addMapping(mapping);
fieldConf.addField(field);
//
field = new CmsSearchField();
field.setName("res_dateReleased");
field.setStored("true");
field.setIndexed("untokenized");
// dateReleased
mapping = new CmsSearchFieldMapping();
mapping.setType("attribute");
mapping.setParam("dateReleased");
field.addMapping(mapping);
fieldConf.addField(field);
//
field = new CmsSearchField();
field.setName("res_length");
field.setStored("true");
field.setIndexed("untokenized");
// length
mapping = new CmsSearchFieldMapping();
mapping.setType("attribute");
mapping.setParam("length");
field.addMapping(mapping);
fieldConf.addField(field);
//
field = new CmsSearchField();
field.setName("res_state");
field.setStored("true");
field.setIndexed("untokenized");
// state
mapping = new CmsSearchFieldMapping();
mapping.setType("attribute");
mapping.setParam("state");
field.addMapping(mapping);
fieldConf.addField(field);
//
field = new CmsSearchField();
field.setName("res_structureId");
field.setStored("true");
field.setIndexed("untokenized");
// structureId
mapping = new CmsSearchFieldMapping();
mapping.setType("attribute");
mapping.setParam("structureId");
field.addMapping(mapping);
fieldConf.addField(field);
//
field = new CmsSearchField();
field.setName("res_userCreated");
field.setStored("true");
field.setIndexed("untokenized");
// userCreated
mapping = new CmsSearchFieldMapping();
mapping.setType("attribute");
mapping.setParam("userCreated");
field.addMapping(mapping);
fieldConf.addField(field);
//
field = new CmsSearchField();
field.setName("res_userLastModified");
field.setStored("true");
field.setIndexed("untokenized");
// userLastModified
mapping = new CmsSearchFieldMapping();
mapping.setType("attribute");
mapping.setParam("userLastModified");
field.addMapping(mapping);
fieldConf.addField(field);
//
field = new CmsSearchField();
field.setName("res_locales");
field.setStored("true");
field.setIndexed("true");
try {
field.setAnalyzer("WhitespaceAnalyzer");
} catch (Exception e) {
// ignore
e.printStackTrace();
}
// res_locales
mapping = new CmsGallerySearchFieldMapping();
mapping.setType("dynamic");
mapping.setParam("res_locales");
field.addMapping(mapping);
fieldConf.addField(field);
//
field = new CmsSearchField();
field.setName("additional_info");
field.setStored("true");
field.setIndexed("false");
// additional_info
mapping = new CmsGallerySearchFieldMapping();
mapping.setType("dynamic");
mapping.setParam("additional_info");
field.addMapping(mapping);
fieldConf.addField(field);
//
field = new CmsSearchField();
field.setName("container_types");
field.setStored("true");
field.setIndexed("true");
try {
field.setAnalyzer("WhitespaceAnalyzer");
} catch (Exception e) {
// ignore
e.printStackTrace();
}
// container_types
mapping = new CmsGallerySearchFieldMapping();
mapping.setType("dynamic");
mapping.setParam("container_types");
field.addMapping(mapping);
fieldConf.addField(field);
createFieldConfig(document, xpath, fieldConf, CmsGallerySearchFieldConfiguration.class);
return true;
}
};
xp = new StringBuffer(256);
xp.append(getCommonPath());
xp.append("/");
xp.append(CmsSearchConfiguration.N_FIELDCONFIGURATIONS);
xp.append("/");
xp.append(CmsSearchConfiguration.N_FIELDCONFIGURATION);
xp.append("[");
xp.append(I_CmsXmlConfiguration.N_NAME);
xp.append("='gallery_fields']");
m_actions.put(xp.toString(), action4);
//
//=============================================================================================================
//
m_actions.put(
"/opencms/search/fieldconfigurations/fieldconfiguration[name='gallery_fields']/field[@name='search_exclude']",
new CmsXmlUpdateAction() {
@Override
public boolean executeUpdate(Document document, String xpath, boolean forReal) {
if (document.selectSingleNode(xpath) == null) {
Element galleryFieldsElement = (Element)(document.selectSingleNode("/opencms/search/fieldconfigurations/fieldconfiguration[name='gallery_fields']/fields"));
Element fieldElement = galleryFieldsElement.addElement("field").addAttribute(
"name",
"search_exclude").addAttribute("store", "true").addAttribute("index", "true");
fieldElement.addElement("mapping").addAttribute("type", "property-search").addText(
"search.exclude");
return true;
} else {
return false;
}
}
});
//
//=============================================================================================================
//
m_actions.put("/opencms/search/indexsources", new CmsIndexSourceTypeUpdateAction());
// use dummy check [1=1] to make the xpaths unique
m_actions.put("/opencms/search/indexsources[1=1]", new CmsAddGalleryModuleIndexSourceAction());
m_actions.put(
buildXpathForIndexedDocumentType("source1", "containerpage"),
createIndexedTypeAction("containerpage"));
m_actions.put("/opencms/search/indexsource[2=2]", new CmsXmlUpdateAction() {
/**
* @see org.opencms.setup.xml.CmsXmlUpdateAction#executeUpdate(org.dom4j.Document, java.lang.String, boolean)
*/
@Override
public boolean executeUpdate(Document doc, String xpath, boolean forReal) {
Element node = (Element)doc.selectSingleNode("/opencms/search/indexsources");
if (!node.selectNodes("indexsource[name='gallery_source_all']").isEmpty()) {
return false;
}
String xml = " \n"
+ " gallery_source_all \n"
+ " \n"
+ " \n"
+ " / \n"
+ " \n"
+ " \n"
+ " generic \n"
+ " xmlpage-galleries \n"
+ " xmlcontent-galleries \n"
+ " jsp \n"
+ " text \n"
+ " pdf \n"
+ " rtf \n"
+ " html \n"
+ " image \n"
+ " generic \n"
+ " msoffice-ole2 \n"
+ " msoffice-ooxml \n"
+ " openoffice \n"
+ " containerpage \n"
+ " \n"
+ " \n"
+ "";
try {
Element sourceElem = createElementFromXml(xml);
node.add(sourceElem);
return true;
} catch (DocumentException e) {
System.err.println("Failed to add gallery_modules_source");
return false;
}
}
});
//=============================================================================================================
String analyzerEnPath = "/opencms/search/analyzers/analyzer[class='org.apache.lucene.analysis.standard.StandardAnalyzer'][locale='en']";
m_actions.put(analyzerEnPath, new ElementReplaceAction(analyzerEnPath, "\n"
+ " org.apache.lucene.analysis.en.EnglishAnalyzer \n"
+ " en \n"
+ " "));
String analyzerItPath = "/opencms/search/analyzers/analyzer[class='org.apache.lucene.analysis.snowball.SnowballAnalyzer'][stemmer='Italian']";
m_actions.put(analyzerItPath, new ElementReplaceAction(analyzerItPath, "\n"
+ " org.apache.lucene.analysis.it.ItalianAnalyzer \n"
+ " it \n"
+ " "));
m_actions.put(
"/opencms/search/indexsources/indexsource[name='gallery_source']/resources['systemgalleries'='systemgalleries']",
new CmsAddIndexSourceResourceAction());
}
}