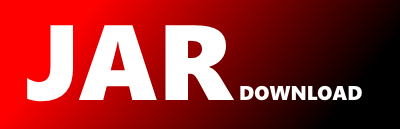
org.opencms.workplace.CmsWorkplaceManager Maven / Gradle / Ivy
Show all versions of opencms-test Show documentation
/*
* This library is part of OpenCms -
* the Open Source Content Management System
*
* Copyright (c) Alkacon Software GmbH (http://www.alkacon.com)
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* For further information about Alkacon Software GmbH, please see the
* company website: http://www.alkacon.com
*
* For further information about OpenCms, please see the
* project website: http://www.opencms.org
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*/
package org.opencms.workplace;
import org.opencms.ade.configuration.CmsElementView;
import org.opencms.ade.galleries.shared.CmsGallerySearchScope;
import org.opencms.configuration.CmsDefaultUserSettings;
import org.opencms.db.CmsExportPoint;
import org.opencms.db.CmsUserSettings;
import org.opencms.db.I_CmsProjectDriver;
import org.opencms.file.CmsFolder;
import org.opencms.file.CmsObject;
import org.opencms.file.CmsProject;
import org.opencms.file.CmsPropertyDefinition;
import org.opencms.file.CmsRequestContext;
import org.opencms.file.CmsResource;
import org.opencms.file.CmsResourceFilter;
import org.opencms.file.CmsUser;
import org.opencms.file.CmsVfsResourceNotFoundException;
import org.opencms.file.types.CmsResourceTypeFolder;
import org.opencms.file.types.CmsResourceTypeFolderExtended;
import org.opencms.file.types.I_CmsResourceType;
import org.opencms.i18n.CmsAcceptLanguageHeaderParser;
import org.opencms.i18n.CmsEncoder;
import org.opencms.i18n.CmsI18nInfo;
import org.opencms.i18n.CmsLocaleComparator;
import org.opencms.i18n.CmsLocaleManager;
import org.opencms.i18n.I_CmsLocaleHandler;
import org.opencms.loader.CmsLoaderException;
import org.opencms.main.CmsEvent;
import org.opencms.main.CmsException;
import org.opencms.main.CmsLog;
import org.opencms.main.I_CmsEventListener;
import org.opencms.main.OpenCms;
import org.opencms.module.CmsModule;
import org.opencms.module.CmsModuleManager;
import org.opencms.relations.CmsCategoryService;
import org.opencms.security.CmsOrganizationalUnit;
import org.opencms.security.CmsPermissionSet;
import org.opencms.security.CmsPermissionViolationException;
import org.opencms.security.CmsRole;
import org.opencms.security.CmsRoleViolationException;
import org.opencms.security.I_CmsPrincipal;
import org.opencms.util.CmsRfsFileViewer;
import org.opencms.util.CmsStringUtil;
import org.opencms.util.CmsUUID;
import org.opencms.workplace.editors.CmsEditorDisplayOptions;
import org.opencms.workplace.editors.CmsEditorHandler;
import org.opencms.workplace.editors.CmsWorkplaceEditorManager;
import org.opencms.workplace.editors.I_CmsEditorActionHandler;
import org.opencms.workplace.editors.I_CmsEditorCssHandler;
import org.opencms.workplace.editors.I_CmsEditorHandler;
import org.opencms.workplace.editors.I_CmsPreEditorActionDefinition;
import org.opencms.workplace.editors.directedit.CmsDirectEditDefaultProvider;
import org.opencms.workplace.editors.directedit.I_CmsDirectEditProvider;
import org.opencms.workplace.explorer.CmsExplorerContextMenu;
import org.opencms.workplace.explorer.CmsExplorerTypeAccess;
import org.opencms.workplace.explorer.CmsExplorerTypeSettings;
import org.opencms.workplace.explorer.menu.CmsMenuRule;
import org.opencms.workplace.galleries.A_CmsAjaxGallery;
import org.opencms.workplace.tools.CmsToolManager;
import java.io.UnsupportedEncodingException;
import java.util.ArrayList;
import java.util.Collections;
import java.util.HashMap;
import java.util.HashSet;
import java.util.Iterator;
import java.util.List;
import java.util.Locale;
import java.util.Map;
import java.util.Set;
import java.util.concurrent.TimeUnit;
import java.util.regex.Pattern;
import java.util.regex.PatternSyntaxException;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpSession;
import org.apache.commons.logging.Log;
import com.google.common.cache.Cache;
import com.google.common.cache.CacheBuilder;
import com.google.common.collect.Lists;
import com.google.common.collect.Maps;
import com.google.common.collect.Sets;
/**
* Manages the global OpenCms workplace settings for all users.
*
* This class reads the settings from the "opencms.properties" and stores them in member variables.
* For each setting one or more get methods are provided.
*
* @since 6.0.0
*/
public final class CmsWorkplaceManager implements I_CmsLocaleHandler, I_CmsEventListener {
/**
* Helper class used to easily define default view mappings for standard resource types.
*/
static class ViewRules {
/**
* Internal view map.
*/
private Map m_viewMap = Maps.newHashMap();
/**
* Creates a new instance.
*
* @param rules an array of strings of the form 'foo,bar,baz:view1', where foo, ... are type names and view1 is a view name (explorertype)
*/
public ViewRules(String... rules) {
for (String rule : rules) {
int colIndex = rule.indexOf(':');
if (colIndex != -1) {
String before = rule.substring(0, colIndex);
String after = rule.substring(colIndex + 1);
for (String token : CmsStringUtil.splitAsList(before, ",")) {
m_viewMap.put(token.trim(), after);
}
}
}
}
/**
* Gets the view configured for the given type.
*
* @param type a resource type name
* @return the view explorer type for the given resource type
*/
public String getViewForType(String type) {
return m_viewMap.get(type);
}
}
/** The logger for this class. */
private static final Log LOG = CmsLog.getLog(CmsWorkplaceManager.class);
/** The default encoding for the workplace (UTF-8). */
public static final String DEFAULT_WORKPLACE_ENCODING = CmsEncoder.ENCODING_UTF_8;
/** The id of the "requestedResource" parameter for the OpenCms login form. */
public static final String PARAM_LOGIN_REQUESTED_RESOURCE = "requestedResource";
/** Key name for the session workplace settings. */
public static final String SESSION_WORKPLACE_SETTINGS = "__CmsWorkplace.WORKPLACE_SETTINGS";
/** Default view configuration. */
static ViewRules m_defaultViewRules = new ViewRules(
"folder,plain,jsp,htmlredirect,containerpage:view_basic",
"imagegallery,downloadgallery,linkgallery,subsitemap,content_folder:view_folders",
"formatter_config,xmlvfsbundle,propertyvfsbundle,sitemap_config,sitemap_master_config,module_config,elementview,seo_file,containerpage_template,inheritance_config,macro_formatter:view_configs",
"xmlcontent,pointer:view_other");
/** Value of the acacia-unlock configuration option (may be null if not set). */
private String m_acaciaUnlock;
/** The admin cms context. */
private CmsObject m_adminCms;
/** Indicates if auto-locking of resources is enabled or disabled. */
private boolean m_autoLockResources;
/** The name of the local category folder(s). */
private String m_categoryFolder;
/** The customized workplace foot. */
private CmsWorkplaceCustomFoot m_customFoot;
/** The default access for explorer types. */
private CmsExplorerTypeAccess m_defaultAccess;
/** The configured default locale of the workplace. */
private Locale m_defaultLocale;
/** The default property setting for setting new property values. */
private boolean m_defaultPropertiesOnStructure;
/** The default user settings. */
private CmsDefaultUserSettings m_defaultUserSettings;
/** The configured dialog handlers. */
private Map m_dialogHandler;
/** The configured direct edit provider. */
private I_CmsDirectEditProvider m_directEditProvider;
/** The edit action handler. */
private I_CmsEditorActionHandler m_editorAction;
/** The editor CSS handlers. */
private List m_editorCssHandlers;
/** The workplace editor display options. */
private CmsEditorDisplayOptions m_editorDisplayOptions;
/** The editor handler. */
private I_CmsEditorHandler m_editorHandler;
/** The editor manager. */
private CmsWorkplaceEditorManager m_editorManager;
/** The flag if switching tabs in the advanced property dialog is enabled. */
private boolean m_enableAdvancedPropertyTabs;
/** The configured encoding of the workplace. */
private String m_encoding;
/** The explorer type settings. */
private List m_explorerTypeSettings;
/** The explorer type settings from the configured modules. */
private List m_explorerTypeSettingsFromModules;
/** The explorer type settings from the XML configuration. */
private List m_explorerTypeSettingsFromXml;
/** The explorer type settings as Map with resource type name as key. */
private Map m_explorerTypeSettingsMap;
/** The element views generated from explorer types. */
private Map m_explorerTypeViews = Maps.newHashMap();
/** The workplace export points. */
private Set m_exportPoints;
/** Maximum size of an upload file. */
private int m_fileMaxUploadSize;
/** The instance used for reading portions of lines of a file to choose. */
private CmsRfsFileViewer m_fileViewSettings;
/** The configured workplace galleries. */
private Map m_galleries;
/** The configured gallery default scope. */
private String m_galleryDefaultScope;
/** The group translation. */
private I_CmsGroupNameTranslation m_groupNameTranslation;
/** The configured group translation class name. */
private String m_groupTranslationClass;
/** Keep-alive flag. */
private Boolean m_keepAlive;
/** Contains all folders that should be labeled if siblings exist. */
private List m_labelSiteFolders;
/** List of installed workplace locales, sorted ascending. */
private List m_locales;
/** The configured list of localized workplace folders. */
private List m_localizedFolders;
/** The configured list of menu rule sets. */
private List m_menuRules;
/** The configured menu rule sets as Map with the rule name as key. */
private Map m_menuRulesMap;
/** The workplace localized messages (mapped to the locales). */
private Map m_messages;
/** The configured multi context menu. */
private CmsExplorerContextMenu m_multiContextMenu;
/** The condition definitions for the resource types which are triggered before opening the editor. */
private List m_preEditorConditionDefinitions;
/** The repository folder handler. */
private I_CmsRepositoryFolderHandler m_repositoryFolderHandler;
/** Indicates if the user management icon should be displayed in the workplace. */
private boolean m_showUserGroupIcon;
/** Exclude patterns for synchronization. */
private ArrayList m_synchronizeExcludePatterns;
/** The temporary file project used by the editors. */
private CmsProject m_tempFileProject;
/** The tool manager. */
private CmsToolManager m_toolManager;
/** The user additional information configuration. */
private CmsWorkplaceUserInfoManager m_userInfoManager;
/** The user list mode. */
private String m_userListMode;
/** The configured workplace views. */
private List m_views;
/** Expiring cache used to limit the number of notifications sent because of invalid workplace server names. */
private Cache m_workplaceServerUserChecks;
/** The XML content auto correction flag. */
private boolean m_xmlContentAutoCorrect;
/**
* Creates a new instance for the workplace manager, will be called by the workplace configuration manager.
*/
public CmsWorkplaceManager() {
if (CmsLog.INIT.isInfoEnabled()) {
CmsLog.INIT.info(Messages.get().getBundle().key(Messages.INIT_WORKPLACE_INITIALIZE_START_0));
}
m_locales = new ArrayList();
m_labelSiteFolders = new ArrayList();
m_localizedFolders = new ArrayList();
m_autoLockResources = true;
m_categoryFolder = CmsCategoryService.REPOSITORY_BASE_FOLDER;
m_xmlContentAutoCorrect = true;
m_showUserGroupIcon = true;
m_dialogHandler = new HashMap();
m_views = new ArrayList();
m_exportPoints = new HashSet();
m_editorHandler = new CmsEditorHandler();
m_fileMaxUploadSize = -1;
m_fileViewSettings = new CmsRfsFileViewer();
m_explorerTypeSettingsFromXml = new ArrayList();
m_explorerTypeSettingsFromModules = new ArrayList();
m_defaultPropertiesOnStructure = true;
m_enableAdvancedPropertyTabs = true;
m_defaultUserSettings = new CmsDefaultUserSettings();
m_defaultAccess = new CmsExplorerTypeAccess();
m_galleries = new HashMap();
m_menuRules = new ArrayList();
m_menuRulesMap = new HashMap();
flushMessageCache();
m_multiContextMenu = new CmsExplorerContextMenu();
m_multiContextMenu.setMultiMenu(true);
m_preEditorConditionDefinitions = new ArrayList();
m_editorCssHandlers = new ArrayList();
m_customFoot = new CmsWorkplaceCustomFoot();
m_synchronizeExcludePatterns = new ArrayList();
// important to set this to null to avoid unnecessary overhead during configuration phase
m_explorerTypeSettings = null;
CacheBuilder