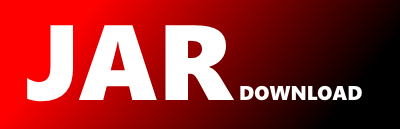
org.opencms.ui.shared.CmsContextMenuState Maven / Gradle / Ivy
Show all versions of opencms-test Show documentation
package org.opencms.ui.shared;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
import com.vaadin.shared.AbstractComponentState;
/**
* The context menu state.
*/
public class CmsContextMenuState extends AbstractComponentState {
/**
* The menu item state.
*/
public static class ContextMenuItemState implements Serializable {
/** The serial version id. */
private static final long serialVersionUID = 3836772122928080543L;
/** The caption. */
private String m_caption;
/** The item children. */
private List m_children;
/** The description, used as tooltip. */
private String m_description;
/** The enabled flag. */
private boolean m_enabled = true;
/** The item id. */
private String m_id;
/** The separator flag. */
private boolean m_separator;
/** The styles. */
private Set m_styles;
/**
* Constructor.
*/
public ContextMenuItemState() {
m_children = new ArrayList();
m_styles = new HashSet();
}
/**
* Adds a child item.
*
* @param caption the caption
* @param id the id
*
* @return the child item state
*/
public ContextMenuItemState addChild(String caption, String id) {
ContextMenuItemState child = new ContextMenuItemState();
child.setCaption(caption);
child.m_id = id;
m_children.add(child);
return child;
}
/**
* @see java.lang.Object#equals(java.lang.Object)
*/
@Override
public boolean equals(Object obj) {
if (this == obj) {
return true;
}
if (obj instanceof ContextMenuItemState) {
return m_id.equals(((ContextMenuItemState)obj).m_id);
}
return false;
}
/**
* Returns the caption.
*
* @return the caption
*/
public String getCaption() {
return m_caption;
}
/**
* Returns the child items.
*
* @return the child items
*/
public List getChildren() {
return m_children;
}
/**
* Returns the description.
*
* @return the description
*/
public String getDescription() {
return m_description;
}
/**
* Returns the id.
*
* @return the id
*/
public String getId() {
return m_id;
}
/**
* Returns the styles.
*
* @return the styles
*/
public Set getStyles() {
return m_styles;
}
/**
* @see java.lang.Object#hashCode()
*/
@Override
public int hashCode() {
return m_id.hashCode();
}
/**
* Returns whether a separator should be displayed.
*
* @return true
if a separator should be displayed
*/
public boolean isSeparator() {
return m_separator;
}
/**
* Returns whether the item is enabled.
*
* @return true
if the item is enabled
*/
public boolean isEnabled() {
return m_enabled;
}
/**
* Removes the given child.
*
* @param child the child to remove
*/
public void removeChild(ContextMenuItemState child) {
m_children.remove(child);
}
/**
* Sets the caption.
*
* @param caption the caption
*/
public void setCaption(String caption) {
m_caption = caption;
}
/**
* Sets the child items.
*
* @param children the children
*/
public void setChildren(List children) {
m_children = children;
}
/**
* Sets the description.
*
* @param description the description to set
*/
public void setDescription(String description) {
m_description = description;
}
/**
* Sets the item enabled.
*
* @param enabled true
to enable the item
*/
public void setEnabled(boolean enabled) {
m_enabled = enabled;
}
/**
* Sets the id.
*
* @param id the id to set
*/
public void setId(String id) {
m_id = id;
}
/**
* Sets whether a separator should be displayed.
*
* @param separator true
if a separator should be displayed
*/
public void setSeparator(boolean separator) {
m_separator = separator;
}
/**
* Sets the styles.
*
* @param styleNames the styles
*/
public void setStyles(Set styleNames) {
m_styles = styleNames;
}
}
/** The serial version id. */
private static final long serialVersionUID = -247856391284942254L;
/** The hides automatically flag. */
private boolean m_hideAutomatically;
/** The opens automatically flag. */
private boolean m_openAutomatically;
/** The root items. */
private List m_rootItems;
/**
* Constructor.
*/
public CmsContextMenuState() {
m_rootItems = new ArrayList();
}
/**
* Adds a child item.
*
* @param itemCaption the caption
* @param itemId the id
*
* @return the item state
*/
public ContextMenuItemState addChild(String itemCaption, String itemId) {
ContextMenuItemState rootItem = new ContextMenuItemState();
rootItem.setCaption(itemCaption);
rootItem.setId(itemId);
m_rootItems.add(rootItem);
return rootItem;
}
/**
* Returns the root items.
*
* @return the root items
*/
public List getRootItems() {
return m_rootItems;
}
/**
* Returns whether the menu is set to hide automatically.
*
* @return true
if context menu is hidden automatically
*/
public boolean isHideAutomatically() {
return m_hideAutomatically;
}
/**
* Returns whether the menu is set to open automatically.
*
* @return true
if open automatically is on. If open automatically is on, it
* means that context menu will always be opened when it's host
* component is right clicked. If automatic opening is turned off,
* context menu will only open when server side open(x, y) is
* called.
*/
public boolean isOpenAutomatically() {
return m_openAutomatically;
}
/**
* Enables or disables automatic hiding of context menu.
*
* @param hideAutomatically the hide automatically flag
*/
public void setHideAutomatically(boolean hideAutomatically) {
this.m_hideAutomatically = hideAutomatically;
}
/**
* Enables or disables open automatically feature. If open automatically is
* on, it means that context menu will always be opened when it's host
* component is right clicked. If automatic opening is turned off, context
* menu will only open when server side open(x, y) is called.
*
* @param openAutomatically the open automatically flag
*/
public void setOpenAutomatically(boolean openAutomatically) {
this.m_openAutomatically = openAutomatically;
}
/**
* Sets the root items.
*
* @param rootItems the root items
*/
public void setRootItems(List rootItems) {
this.m_rootItems = rootItems;
}
}