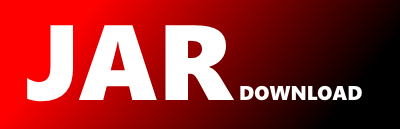
org.opencms.workplace.CmsWorkplace Maven / Gradle / Ivy
Show all versions of opencms-test Show documentation
/*
* This library is part of OpenCms -
* the Open Source Content Management System
*
* Copyright (c) Alkacon Software GmbH & Co. KG (http://www.alkacon.com)
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* For further information about Alkacon Software GmbH & Co. KG, please see the
* company website: http://www.alkacon.com
*
* For further information about OpenCms, please see the
* project website: http://www.opencms.org
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*/
package org.opencms.workplace;
import org.opencms.db.CmsDbEntryNotFoundException;
import org.opencms.db.CmsUserSettings;
import org.opencms.file.CmsObject;
import org.opencms.file.CmsProject;
import org.opencms.file.CmsRequestContext;
import org.opencms.file.CmsResource;
import org.opencms.file.CmsResourceFilter;
import org.opencms.file.CmsUser;
import org.opencms.i18n.CmsEncoder;
import org.opencms.i18n.CmsMessages;
import org.opencms.i18n.CmsMultiMessages;
import org.opencms.jsp.CmsJspActionElement;
import org.opencms.lock.CmsLock;
import org.opencms.lock.CmsLockType;
import org.opencms.main.CmsBroadcast;
import org.opencms.main.CmsContextInfo;
import org.opencms.main.CmsException;
import org.opencms.main.CmsIllegalStateException;
import org.opencms.main.CmsLog;
import org.opencms.main.CmsSessionInfo;
import org.opencms.main.CmsStaticResourceHandler;
import org.opencms.main.OpenCms;
import org.opencms.security.CmsRole;
import org.opencms.security.CmsRoleViolationException;
import org.opencms.site.CmsSite;
import org.opencms.site.CmsSiteManagerImpl;
import org.opencms.synchronize.CmsSynchronizeSettings;
import org.opencms.util.CmsMacroResolver;
import org.opencms.util.CmsRequestUtil;
import org.opencms.util.CmsStringUtil;
import org.opencms.util.CmsUUID;
import java.io.IOException;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
import java.util.ArrayList;
import java.util.Collection;
import java.util.HashMap;
import java.util.Iterator;
import java.util.List;
import java.util.Locale;
import java.util.Map;
import java.util.Map.Entry;
import javax.servlet.ServletException;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.http.HttpSession;
import javax.servlet.jsp.PageContext;
import org.apache.commons.collections.Buffer;
import org.apache.commons.fileupload.FileItem;
import org.apache.commons.logging.Log;
/**
* Master class for the JSP based workplace which provides default methods and
* session handling for all JSP workplace classes.
*
* @since 6.0.0
*/
public abstract class CmsWorkplace {
/** The debug flag. */
public static final boolean DEBUG = false;
/** Path to the JSP workplace frame loader file. */
public static final String JSP_WORKPLACE_URI = CmsWorkplace.VFS_PATH_VIEWS + "workplace.jsp";
/** Request parameter name for the model file. */
public static final String PARAM_MODELFILE = "modelfile";
/** Request parameter name prefix for the preferred editors. */
public static final String INPUT_DEFAULT = "default";
/** Request parameter name for the resource list. */
public static final String PARAM_RESOURCELIST = "resourcelist";
/** Request parameter name for no settings in start galleries. */
public static final String INPUT_NONE = "none";
/** Path to system folder. */
public static final String VFS_PATH_SYSTEM = "/system/";
/** Path to the workplace. */
public static final String VFS_PATH_WORKPLACE = VFS_PATH_SYSTEM + "workplace/";
/** Constant for the JSP dialogs path. */
public static final String PATH_DIALOGS = VFS_PATH_WORKPLACE + "commons/";
/** Parameter for the default locale. */
public static final Locale DEFAULT_LOCALE = Locale.ENGLISH;
/** Parameter for the default language. */
public static final String DEFAULT_LANGUAGE = DEFAULT_LOCALE.getLanguage();
/** Constant for the JSP common files (e.g. error page) path. */
public static final String DIALOG_PATH_COMMON = PATH_DIALOGS + "includes/";
/** Constant for the JSP common close dialog page. */
public static final String FILE_DIALOG_CLOSE = DIALOG_PATH_COMMON + "closedialog.jsp";
/** Constant for the JSP common confirmation dialog. */
public static final String FILE_DIALOG_SCREEN_CONFIRM = DIALOG_PATH_COMMON + "confirmation.jsp";
/** Constant for the JSP common error dialog. */
public static final String FILE_DIALOG_SCREEN_ERROR = DIALOG_PATH_COMMON + "error.jsp";
/** Constant for the JSP common error dialog. */
public static final String FILE_DIALOG_SCREEN_ERRORPAGE = DIALOG_PATH_COMMON + "errorpage.jsp";
/** Constant for the JSP common wait screen. */
public static final String FILE_DIALOG_SCREEN_WAIT = DIALOG_PATH_COMMON + "wait.jsp";
/** Path to workplace views. */
public static final String VFS_PATH_VIEWS = VFS_PATH_WORKPLACE + "views/";
/** Constant for the JSP explorer filelist file. */
public static final String FILE_EXPLORER_FILELIST = VFS_PATH_VIEWS + "explorer/explorer_files.jsp";
/** Constant for the JSP common report page. */
public static final String FILE_REPORT_OUTPUT = DIALOG_PATH_COMMON + "report.jsp";
/** Helper variable to deliver the html end part. */
public static final int HTML_END = 1;
/** Helper variable to deliver the html start part. */
public static final int HTML_START = 0;
/** The request parameter for the workplace project selection. */
public static final String PARAM_WP_EXPLORER_RESOURCE = "wpExplorerResource";
/** The request parameter for the workplace project selection. */
public static final String PARAM_WP_PROJECT = "wpProject";
/** The request parameter for the workplace site selection. */
public static final String PARAM_WP_SITE = "wpSite";
/** Constant for the JSP workplace path. */
public static final String PATH_WORKPLACE = VFS_PATH_WORKPLACE;
/** Path for file type icons relative to the resources folder. */
public static final String RES_PATH_FILETYPES = "filetypes/";
/** Path to exported system image folder. */
public static final String RFS_PATH_RESOURCES = "/resources/";
/** Directory name of content default_bodies folder. */
public static final String VFS_DIR_DEFAULTBODIES = "default_bodies/";
/** Directory name of content templates folder. */
public static final String VFS_DIR_TEMPLATES = "templates/";
/** Path to commons. */
public static final String VFS_PATH_COMMONS = VFS_PATH_WORKPLACE + "commons/";
/** Path to the workplace editors. */
public static final String VFS_PATH_EDITORS = VFS_PATH_WORKPLACE + "editors/";
/** Path to the galleries. */
public static final String VFS_PATH_GALLERIES = VFS_PATH_SYSTEM + "galleries/";
/** Path to locales. */
public static final String VFS_PATH_LOCALES = VFS_PATH_WORKPLACE + "locales/";
/** Path to modules folder. */
public static final String VFS_PATH_MODULES = VFS_PATH_SYSTEM + "modules/";
/** Path to system image folder. */
public static final String VFS_PATH_RESOURCES = VFS_PATH_WORKPLACE + "resources/";
/** Constant for the direct edit view JSP. */
public static final String VIEW_DIRECT_EDIT = VFS_PATH_VIEWS + "explorer/directEdit.jsp";
/** Constant for the explorer view JSP. */
public static final String VIEW_WORKPLACE = VFS_PATH_VIEWS + "explorer/explorer_fs.jsp";
/** Constant for the admin view JSP. */
public static final String VIEW_ADMIN = CmsWorkplace.VFS_PATH_VIEWS + "admin/admin-fs.jsp";
/** Key name for the request attribute to indicate a multipart request was already parsed. */
protected static final String REQUEST_ATTRIBUTE_MULTIPART = "__CmsWorkplace.MULTIPART";
/** Key name for the request attribute to reload the folder tree view. */
protected static final String REQUEST_ATTRIBUTE_RELOADTREE = "__CmsWorkplace.RELOADTREE";
/** Key name for the session workplace class. */
protected static final String SESSION_WORKPLACE_CLASS = "__CmsWorkplace.WORKPLACE_CLASS";
/** The "explorerview" view selection. */
public static final String VIEW_EXPLORER = "explorerview";
/** The "galleryview" view selection. */
public static final String VIEW_GALLERY = "galleryview";
/** The "list" view selection. */
public static final String VIEW_LIST = "listview";
/** Request parameter name for the directpublish parameter. */
public static final String PARAM_DIRECTPUBLISH = "directpublish";
/** Request parameter name for the publishsiblings parameter. */
public static final String PARAM_PUBLISHSIBLINGS = "publishsiblings";
/** Request parameter name for the relatedresources parameter. */
public static final String PARAM_RELATEDRESOURCES = "relatedresources";
/** Request parameter name for the subresources parameter. */
public static final String PARAM_SUBRESOURCES = "subresources";
/** Default value for date last modified, the release and expire date. */
public static final String DEFAULT_DATE_STRING = "-";
/** Absolute path to the model file dialog. */
public static final String VFS_PATH_MODELDIALOG = CmsWorkplace.VFS_PATH_COMMONS
+ "newresource_xmlcontent_modelfile.jsp";
/** Absolute path to thenew resource dialog. */
public static final String VFS_PATH_NEWRESOURCEDIALOG = CmsWorkplace.VFS_PATH_COMMONS
+ "newresource_xmlcontent.jsp";
/** Request parameter name for the new resource type. */
public static final String PARAM_NEWRESOURCETYPE = "newresourcetype";
/** The log object for this class. */
private static final Log LOG = CmsLog.getLog(CmsWorkplace.class);
/** The link to the explorer file list (cached for performance reasons). */
private static String m_file_explorer_filelist;
/** The URI to the skin resources (cached for performance reasons). */
private static String m_skinUri;
/** The URI to the stylesheet resources (cached for performance reasons). */
private static String m_styleUri;
/** The request parameter for the workplace view selection. */
public static final String PARAM_WP_VIEW = "wpView";
/** The request parameter for the workplace start selection. */
public static final String PARAM_WP_START = "wpStart";
/** The current users OpenCms context. */
private CmsObject m_cms;
/** Helper variable to store the id of the current project. */
private CmsUUID m_currentProjectId;
/** Flag for indicating that request forwarded was. */
private boolean m_forwarded;
/** The current JSP action element. */
private CmsJspActionElement m_jsp;
/** The macro resolver, this is cached to avoid multiple instance generation. */
private CmsMacroResolver m_macroResolver;
/** The currently used message bundle. */
private CmsMultiMessages m_messages;
/** The list of multi part file items (if available). */
private List m_multiPartFileItems;
/** The map of parameters read from the current request. */
private Map m_parameterMap;
/** The current resource URI. */
private String m_resourceUri;
/** The current OpenCms users http session. */
private HttpSession m_session;
/** The current OpenCms users workplace settings. */
private CmsWorkplaceSettings m_settings;
/**
* Public constructor.
*
* @param jsp the initialized JSP context
*/
public CmsWorkplace(CmsJspActionElement jsp) {
initWorkplaceMembers(jsp);
}
/**
* Constructor in case no page context is available.
*
* @param cms the current user context
* @param session the session
*/
public CmsWorkplace(CmsObject cms, HttpSession session) {
initWorkplaceMembers(cms, session);
}
/**
* Public constructor with JSP variables.
*
* @param context the JSP page context
* @param req the JSP request
* @param res the JSP response
*/
public CmsWorkplace(PageContext context, HttpServletRequest req, HttpServletResponse res) {
this(new CmsJspActionElement(context, req, res));
}
/**
* Generates a html select box out of the provided values.
*
* @param parameters a string that will be inserted into the initial select tag,
* if null no parameters will be inserted
* @param options the options
* @param values the option values, if null the select will have no value attributes
* @param selected the index of the pre-selected option, if -1 no option is pre-selected
* @param useLineFeed if true, adds some formatting "\n" to the output String
* @return a String representing a html select box
*/
public static String buildSelect(
String parameters,
List options,
List values,
int selected,
boolean useLineFeed) {
StringBuffer result = new StringBuffer(1024);
result.append("");
if (useLineFeed) {
result.append("\n");
}
return result.toString();
}
/**
* Checks if permissions for roles should be editable for the current user on the resource with the given path.
*
* @param cms the CMS context
* @param path the path of a resource
*
* @return true
if permissions for roles should be editable for the current user on the resource with the given path
*/
public static boolean canEditPermissionsForRoles(CmsObject cms, String path) {
return OpenCms.getRoleManager().hasRoleForResource(cms, CmsRole.VFS_MANAGER, path)
&& path.startsWith(VFS_PATH_SYSTEM);
}
/**
* Returns the style sheets for the report.
*
* @param cms the current users context
* @return the style sheets for the report
*/
public static String generateCssStyle(CmsObject cms) {
StringBuffer result = new StringBuffer(128);
result.append("\n");
return result.toString();
}
/**
* Generates the footer for the extended report view.
*
* @return html code
*/
public static String generatePageEndExtended() {
StringBuffer result = new StringBuffer(128);
result.append("