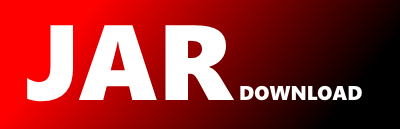
org.opencms.ade.galleries.shared.I_CmsGalleryConfiguration Maven / Gradle / Ivy
Show all versions of opencms-test Show documentation
/*
* This library is part of OpenCms -
* the Open Source Content Management System
*
* Copyright (c) Alkacon Software GmbH & Co. KG (http://www.alkacon.com)
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* For further information about Alkacon Software, please see the
* company website: http://www.alkacon.com
*
* For further information about OpenCms, please see the
* project website: http://www.opencms.org
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*/
package org.opencms.ade.galleries.shared;
import org.opencms.ade.galleries.shared.I_CmsGalleryProviderConstants.GalleryMode;
import java.util.List;
/**
* The gallery configuration interface.
*/
public interface I_CmsGalleryConfiguration {
/**
* Returns the currently selected element.
*
* @return the currently selected element
*/
String getCurrentElement();
/**
* Returns the gallery mode.
*
* @return the gallery mode
*/
GalleryMode getGalleryMode();
/**
* Returns the start gallery path.
*
* @return the start gallery path
*/
String getGalleryPath();
/**
* Gets the prefix for the key used to store the last selected gallery.
*
* @return the prefix for the key used to store the last selected gallery
*/
String getGalleryStoragePrefix();
/**
* Returns the gallery type name.
*
* @return the gallery type name
*/
String getGalleryTypeName();
/**
* Returns the available gallery types.
*
* @return the available gallery types
*/
String[] getGalleryTypes();
/**
* Returns the image format names.
*
* @return the image format names
*/
String getImageFormatNames();
/**
* Returns the image formats.
*
* @return the image formats
*/
String getImageFormats();
/**
* Returns the content locale.
*
* @return the content locale
*/
String getLocale();
/**
* Returns the path of the edited resource.
*
* @return the path of the edited resource
*/
String getReferencePath();
/**
* Returns the available resource types.
*
* @return the available resource types
*/
List getResourceTypes();
/**
* Returns the searchable types.
*
* @return the searchable types
*/
List getSearchTypes();
/**
* Returns the start folder.
*
* @return the start folder
*/
String getStartFolder();
/**
* Returns the start site.
*
* @return the start site
*/
String getStartSite();
/**
* Gets the tab configuration.
*
* @return the gallery tab configuration
*/
CmsGalleryTabConfiguration getTabConfiguration();
/**
* Gets the tree token.
*
* The tree token is used to save/load tree opening states for tree tabs in the gallery
* dialog. If two widget instances use different tree tokens, opening or closing tree entries
* in one will not effect the tree opening state of the other.
*
* @return the tree token
*/
String getTreeToken();
/**
* Returns the upload folder.
*
* @return the upload folder
*/
String getUploadFolder();
/**
* Returns true if the galleries should be selectable.
*
* @return true if the galleries should be selectable
*/
boolean isGalleriesSelectable();
/**
* Returns false if the results should not be selectable.
*
* @return false if the results should not be selectable
*/
boolean isResultsSelectable();
/**
* Returns if files are selectable.
*
* @return true
if files are selectable
*/
boolean isIncludeFiles();
/**
* Returns if the site selector should be shown.
*
* @return true
if the site selector should be shown
*/
boolean isShowSiteSelector();
/**
* Returns if image formats should be used in preview.
*
* @return true
if image format should be used in preview
*/
boolean isUseFormats();
/**
* Sets the currentElement.
*
* @param currentElement the currentElement to set
*/
void setCurrentElement(String currentElement);
/**
* Sets the start folder.
*
* @param startFolder the start folder
*/
void setStartFolder(String startFolder);
}