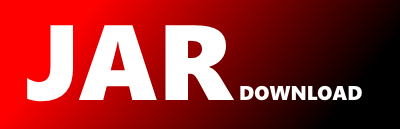
org.opencms.setup.CmsSetupDb Maven / Gradle / Ivy
Show all versions of opencms-test Show documentation
/*
* This library is part of OpenCms -
* the Open Source Content Management System
*
* Copyright (c) Alkacon Software GmbH & Co. KG (http://www.alkacon.com)
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* For further information about Alkacon Software GmbH & Co. KG, please see the
* company website: http://www.alkacon.com
*
* For further information about OpenCms, please see the
* project website: http://www.opencms.org
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*/
package org.opencms.setup;
import org.opencms.main.CmsException;
import org.opencms.main.CmsLog;
import org.opencms.util.CmsDataTypeUtil;
import org.opencms.util.CmsStringUtil;
import java.io.File;
import java.io.FileNotFoundException;
import java.io.FileReader;
import java.io.IOException;
import java.io.LineNumberReader;
import java.io.Reader;
import java.io.StringReader;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Statement;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.StringTokenizer;
import org.apache.commons.logging.Log;
/**
* Helper class to call database setup scripts.
*
* @since 6.0.0
*/
public class CmsSetupDb extends Object {
/** The folder where to read the setup data from. */
public static final String SETUP_DATA_FOLDER = "WEB-INF/setupdata/";
/** The folder where the setup wizard is located. */
public static final String SETUP_FOLDER = "setup/";
/** The log object for this class. */
private static final Log LOG = CmsLog.getLog(CmsSetupDb.class);
/** The setup base path. */
private String m_basePath;
/** A SQL connection. */
private Connection m_con;
/** A flag signaling if error logging is enabled. */
private boolean m_errorLogging;
/** A list to store error messages. */
private List m_errors;
/**
* Creates a new CmsSetupDb object.
*
* @param basePath the location of the setup scripts
*/
public CmsSetupDb(String basePath) {
m_errors = new ArrayList();
m_basePath = basePath;
m_errorLogging = true;
}
/**
* Returns an optional warning message if needed, null
if not.
*
* @param db the selected database key
*
* @return html warning, or null
if no warning
*/
public String checkVariables(String db) {
StringBuffer html = new StringBuffer(512);
if (m_con == null) {
return null; // prior error, trying to get a connection
}
Exception exception = null;
if (db.equals("mysql")) {
String statement = "SELECT @@max_allowed_packet;";
Statement stmt = null;
ResultSet rs = null;
long maxAllowedPacket = 0;
try {
stmt = m_con.createStatement();
rs = stmt.executeQuery(statement);
if (rs.next()) {
maxAllowedPacket = rs.getLong(1);
}
} catch (Exception e) {
exception = e;
} finally {
if (stmt != null) {
try {
stmt.close();
} catch (SQLException e) {
// ignore
}
}
}
if (exception == null) {
int megabyte = 1024 * 1024;
if (maxAllowedPacket > 0) {
html.append("
MySQL system variable 'max_allowed_packet'
is set to ");
html.append(maxAllowedPacket);
html.append(" Byte (");
html.append((maxAllowedPacket / megabyte) + "MB).
\n");
}
html.append(
"Please note that it will not be possible for OpenCms to handle files bigger than this value in the VFS.
\n");
int requiredMaxAllowdPacket = 16;
if (maxAllowedPacket < (requiredMaxAllowdPacket * megabyte)) {
m_errors.add(
"Your 'max_allowed_packet'
variable is set to less than "
+ (requiredMaxAllowdPacket * megabyte)
+ " Byte ("
+ requiredMaxAllowdPacket
+ "MB).
\n"
+ "The required value for running OpenCms is at least "
+ requiredMaxAllowdPacket
+ "MB."
+ "Please change your MySQL configuration (in the my.ini
or my.cnf
file).
\n");
}
} else {
html.append(
"OpenCms was not able to detect the value of your 'max_allowed_packet'
variable.
\n");
html.append(
"Please note that it will not be possible for OpenCms to handle files bigger than this value.
\n");
html.append(
"The recommended value for running OpenCms is 16MB, please set it in your MySQL configuration (in your my.ini
or my.cnf
file).
\n");
html.append(CmsException.getStackTraceAsString(exception));
}
}
if (html.length() == 0) {
return null;
}
return html.toString();
}
/**
* Clears the error messages stored internally.
*/
public void clearErrors() {
m_errors.clear();
}
/**
* Closes the internal connection to the database.
*/
public void closeConnection() {
try {
if (m_con != null) {
m_con.close();
}
} catch (Exception e) {
// ignore
}
m_con = null;
}
/**
* Calls the create database script for the given database.
*
* @param database the name of the database
* @param replacer the replacements to perform in the drop script
*/
public void createDatabase(String database, Map replacer) {
m_errorLogging = true;
executeSql(database, "create_db.sql", replacer, true);
}
/**
* Calls the create database script for the given database.
*
* @param database the name of the database
* @param replacer the replacements to perform in the drop script
* @param abortOnError indicates if the script is aborted if an error occurs
*/
public void createDatabase(String database, Map replacer, boolean abortOnError) {
m_errorLogging = true;
executeSql(database, "create_db.sql", replacer, abortOnError);
}
/**
* Calls the create tables script for the given database.
*
* @param database the name of the database
* @param replacer the replacements to perform in the drop script
*/
public void createTables(String database, Map replacer) {
m_errorLogging = true;
executeSql(database, "create_tables.sql", replacer, true);
}
/**
* Calls the create tables script for the given database.
*
* @param database the name of the database
* @param replacer the replacements to perform in the drop script
* @param abortOnError indicates if the script is aborted if an error occurs
*/
public void createTables(String database, Map replacer, boolean abortOnError) {
m_errorLogging = true;
executeSql(database, "create_tables.sql", replacer, abortOnError);
}
/**
* Calls the drop script for the given database.
*
* @param database the name of the database
* @param replacer the replacements to perform in the drop script
*/
public void dropDatabase(String database, Map replacer) {
m_errorLogging = true;
executeSql(database, "drop_db.sql", replacer, false);
}
/**
* Calls the drop script for the given database.
*
* @param database the name of the database
* @param replacer the replacements to perform in the drop script
* @param abortOnError indicates if the script is aborted if an error occurs
*/
public void dropDatabase(String database, Map replacer, boolean abortOnError) {
m_errorLogging = true;
executeSql(database, "drop_db.sql", replacer, abortOnError);
}
/**
* Calls the drop tables script for the given database.
*
* @param database the name of the database
*/
public void dropTables(String database) {
m_errorLogging = true;
executeSql(database, "drop_tables.sql", null, false);
}
/**
* Calls the drop tables script for the given database.
*
* @param database the name of the database
* @param replacer the replacements to perform in the drop script
*/
public void dropTables(String database, Map replacer) {
m_errorLogging = true;
executeSql(database, "drop_tables.sql", replacer, false);
}
/**
* Calls the drop tables script for the given database.
*
* @param database the name of the database
* @param replacer the replacements to perform in the drop script
* @param abortOnError indicates if the script is aborted if an error occurs
*/
public void dropTables(String database, Map replacer, boolean abortOnError) {
m_errorLogging = true;
executeSql(database, "drop_tables.sql", replacer, abortOnError);
}
/**
* Creates and executes a database statement from a String returning the result set.
*
* @param query the query to execute
* @param replacer the replacements to perform in the script
*
* @return the result set of the query
*
* @throws SQLException if something goes wrong
*/
public CmsSetupDBWrapper executeSqlStatement(String query, Map replacer) throws SQLException {
CmsSetupDBWrapper dbwrapper = new CmsSetupDBWrapper(m_con);
dbwrapper.createStatement();
String queryToExecute = query;
// Check if a map of replacements is given
if (replacer != null) {
queryToExecute = replaceTokens(query, replacer);
}
// do the query
dbwrapper.excecuteQuery(queryToExecute);
// return the result
return dbwrapper;
}
/** Creates and executes a database statement from a String returning the result set.
*
* @param query the query to execute
* @param replacer the replacements to perform in the script
* @param params the list of parameters for the statement
*
* @return the result set of the query
*
* @throws SQLException if something goes wrong
*/
public CmsSetupDBWrapper executeSqlStatement(String query, Map replacer, List