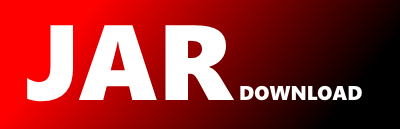
org.opencms.util.TestCmsStringUtil Maven / Gradle / Ivy
Show all versions of opencms-test Show documentation
/*
* This library is part of OpenCms -
* the Open Source Content Management System
*
* Copyright (c) Alkacon Software GmbH & Co. KG (http://www.alkacon.com)
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* For further information about Alkacon Software GmbH & Co. KG, please see the
* company website: http://www.alkacon.com
*
* For further information about OpenCms, please see the
* project website: http://www.opencms.org
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*/
package org.opencms.util;
import org.opencms.i18n.CmsEncoder;
import org.opencms.test.OpenCmsTestCase;
import java.util.Arrays;
import java.util.List;
import com.google.common.base.Optional;
/**
* Test cases for {@link org.opencms.util.CmsStringUtil}.
*/
public class TestCmsStringUtil extends OpenCmsTestCase {
/**
* Tests content replacement during import.
*/
public void testCmsContentReplacement() {
String content, result, context, search, replace;
content = "
\n"
+ "See \n"
+ "http://www.opencms.org/opencms/opencms/opencms/index.html\n"
+ "or \n"
+ "/opencms/opencms/opencms/index.html\n"
+ "
\n"
+ "
\n"
+ "Some URL in the Text: http://www.thirdsite.org/opencms/opencms/some/url.html.\n"
+ "Another URL in the Text: /opencms/opencms/some/url.html.\n"
+ "\n";
result = "\n"
+ "See \n"
+ "http://www.opencms.org/opencms/opencms/opencms/index.html\n"
+ "or \n"
+ CmsStringUtil.MACRO_OPENCMS_CONTEXT
+ "/opencms/index.html\n"
+ "
\n"
+ "
\n"
+ "Some URL in the Text: http://www.thirdsite.org/opencms/opencms/some/url.html.\n"
+ "Another URL in the Text: "
+ CmsStringUtil.MACRO_OPENCMS_CONTEXT
+ "/some/url.html.\n"
+ "\n";
context = "/opencms/opencms/";
// search = "([>\"']\\s*)" + context;
search = "([^\\w/])" + context;
replace = "$1" + CmsStringUtil.escapePattern(CmsStringUtil.MACRO_OPENCMS_CONTEXT) + "/";
String test = CmsStringUtil.substitutePerl(content, search, replace, "g");
System.err.println(this.getClass().getName() + ".testCmsContentReplacement():");
System.err.println(test);
assertEquals(test, result);
test = CmsStringUtil.substituteContextPath(content, context);
assertEquals(test, result);
}
/**
* Combined tests.
*/
public void testCombined() {
String test;
String content = "
A paragraph with text...
\n";
String search = "/opencms/opencms/";
String replace = "${path}";
test = CmsStringUtil.substitute(content, search, replace);
assertEquals(
test,
"A paragraph with text...
\n");
test = CmsStringUtil.substitute(test, replace, search);
assertEquals(
test,
"A paragraph with text...
\n");
}
/**
* Tests for complext import patterns.
*/
public void testComplexPatternForImport() {
String content = "/pics/test.gif
script = '/pics/test.gif' /pics/othertest.gif \n"
+ "/mymodule/pics/test.gif
script = '/mymodule/pics/test.gif' /mymodule/system/galleries/pics/othertest.gif ";
String search = "([>\"']\\s*)/pics/";
String replace = "$1/system/galleries/pics/";
String test = CmsStringUtil.substitutePerl(content, search, replace, "g");
assertEquals(
test,
"/system/galleries/pics/test.gif
script = '/system/galleries/pics/test.gif' /system/galleries/pics/othertest.gif \n"
+ "/mymodule/pics/test.gif
script = '/mymodule/pics/test.gif' /mymodule/system/galleries/pics/othertest.gif ");
}
/**
* Tests the parseDuration method.
*/
public void testDuration() {
long second = 1000;
long minute = 60 * second;
long hour = 60 * minute;
long day = 24 * hour;
assertEquals(day + (5 * minute), CmsStringUtil.parseDuration(" 1d 5m ", 0));
assertEquals(hour + 5, CmsStringUtil.parseDuration("1h5ms", 0));
assertEquals(3 * hour, CmsStringUtil.parseDuration("4x3h5y", 0));
assertEquals((5 * second) + 16, CmsStringUtil.parseDuration("5s16ms", 0));
// check default value
assertEquals(0, CmsStringUtil.parseDuration("0s", 10));
assertEquals(10, CmsStringUtil.parseDuration("4440", 10));
}
/**
* Tests for the escape patterns.
*/
public void testEscapePattern() {
String test;
test = CmsStringUtil.escapePattern("/opencms/opencms");
assertEquals(test, "\\/opencms\\/opencms");
test = CmsStringUtil.escapePattern("/opencms/$");
assertEquals(test, "\\/opencms\\/\\$");
}
/**
* Tests the body tag extraction.
*/
public void testExtractHtmlBody() {
String content, result;
String innerContent = "This is body content in the body\n
A headline
\nSome text in the body\n";
content = "" + innerContent + "";
result = CmsStringUtil.extractHtmlBody(content);
assertEquals(result, innerContent);
content = "" + innerContent + "";
result = CmsStringUtil.extractHtmlBody(content);
assertEquals(result, innerContent);
content = "\nTest \n"
+ innerContent
+ "\n";
result = CmsStringUtil.extractHtmlBody(content);
assertEquals(result, innerContent);
content = "< body style='css' background-color:#ffffff>" + innerContent + " BODY>";
result = CmsStringUtil.extractHtmlBody(content);
assertEquals(result, innerContent);
content = "" + innerContent + "