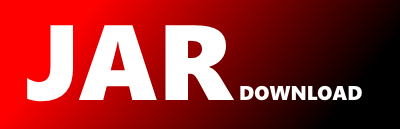
org.opencms.workplace.commons.CmsChacc Maven / Gradle / Ivy
Show all versions of opencms-test Show documentation
/*
* This library is part of OpenCms -
* the Open Source Content Management System
*
* Copyright (c) Alkacon Software GmbH & Co. KG (http://www.alkacon.com)
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* For further information about Alkacon Software GmbH & Co. KG, please see the
* company website: http://www.alkacon.com
*
* For further information about OpenCms, please see the
* project website: http://www.opencms.org
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*/
package org.opencms.workplace.commons;
import org.opencms.db.CmsDbEntryNotFoundException;
import org.opencms.file.CmsGroup;
import org.opencms.file.CmsObject;
import org.opencms.file.CmsResource;
import org.opencms.file.CmsResourceFilter;
import org.opencms.file.CmsUser;
import org.opencms.file.history.CmsHistoryPrincipal;
import org.opencms.jsp.CmsJspActionElement;
import org.opencms.lock.CmsLockFilter;
import org.opencms.main.CmsException;
import org.opencms.main.CmsLog;
import org.opencms.main.OpenCms;
import org.opencms.security.CmsAccessControlEntry;
import org.opencms.security.CmsAccessControlList;
import org.opencms.security.CmsOrganizationalUnit;
import org.opencms.security.CmsPermissionSet;
import org.opencms.security.CmsPrincipal;
import org.opencms.security.CmsRole;
import org.opencms.security.I_CmsPrincipal;
import org.opencms.util.CmsStringUtil;
import org.opencms.util.CmsUUID;
import org.opencms.widgets.CmsPrincipalWidget;
import org.opencms.workplace.CmsDialog;
import org.opencms.workplace.CmsWorkplace;
import org.opencms.workplace.CmsWorkplaceSettings;
import org.opencms.workplace.explorer.CmsResourceUtil;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.HashMap;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Set;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.jsp.PageContext;
import org.apache.commons.logging.Log;
/**
* Provides methods for building the permission settings dialog.
*
* The following files use this class:
*
* - /commons/chacc.jsp
*
*
*
* @since 6.0.0
*/
public class CmsChacc extends CmsDialog {
/** Value for the action: add an access control entry. */
public static final int ACTION_ADDACE = 300;
/** Value for the action: delete the permissions. */
public static final int ACTION_DELETE = 200;
/** Value for the action: set the internal use flag. */
public static final int ACTION_INTERNALUSE = 400;
/** Request parameter value for the action: add an access control entry. */
public static final String DIALOG_ADDACE = "addace";
/** Request parameter value for the action: delete the permissions. */
public static final String DIALOG_DELETE = "delete";
/** Request parameter value for the action: set the internal use flag. */
public static final String DIALOG_INTERNALUSE = "internaluse";
/** The dialog type. */
public static final String DIALOG_TYPE = "chacc";
/** Request parameter name for the inherit permissions parameter. */
public static final String PARAM_INHERIT = "inherit";
/** Request parameter name for the internal use only flag. */
public static final String PARAM_INTERNAL = "internal";
/** Request parameter name for the name parameter. */
public static final String PARAM_NAME = "name";
/** Request parameter name for the overwrite inherited permissions parameter. */
public static final String PARAM_OVERWRITEINHERITED = "overwriteinherited";
/** Request parameter name for the responsible parameter. */
public static final String PARAM_RESPONSIBLE = "responsible";
/** Request parameter name for the type parameter. */
public static final String PARAM_TYPE = "type";
/** Request parameter name for the view parameter. */
public static final String PARAM_VIEW = "view";
/** Constant for the request parameters suffix: allow. */
public static final String PERMISSION_ALLOW = "allow";
/** Constant for the request parameters suffix: deny. */
public static final String PERMISSION_DENY = "deny";
/** The log object for this class. */
private static final Log LOG = CmsLog.getLog(CmsChacc.class);
/** Constant for unknown type. */
private static final String UNKNOWN_TYPE = "Unknown";
/** PermissionSet of the current user for the resource. */
private CmsPermissionSet m_curPermissions;
/** Indicates if forms are editable by current user. */
private boolean m_editable;
/** Stores eventual error message Strings. */
private List m_errorMessages = new ArrayList();
/** Indicates if inheritance flags are set as hidden fields for resource folders. */
private boolean m_inherit;
/** The name parameter. */
private String m_paramName;
/** The type parameter. */
private String m_paramType;
/** UUID paramter as String. */
private String m_paramUuid;
/** Stores all possible permission keys of a permission set. */
private Set m_permissionKeys = CmsPermissionSet.getPermissionKeys();
/** Marks if the inherited permissions information should be displayed. */
private boolean m_showInheritedPermissions;
/** The possible types of new access control entries. */
private String[] m_types = {
I_CmsPrincipal.PRINCIPAL_GROUP,
I_CmsPrincipal.PRINCIPAL_USER,
CmsRole.PRINCIPAL_ROLE,
CmsAccessControlEntry.PRINCIPAL_ALL_OTHERS_NAME,
CmsAccessControlEntry.PRINCIPAL_OVERWRITE_ALL_NAME};
/** The possible type values of access control entries. */
private int[] m_typesInt = {
CmsAccessControlEntry.ACCESS_FLAGS_GROUP,
CmsAccessControlEntry.ACCESS_FLAGS_USER,
CmsAccessControlEntry.ACCESS_FLAGS_ROLE,
CmsAccessControlEntry.ACCESS_FLAGS_ALLOTHERS,
CmsAccessControlEntry.ACCESS_FLAGS_OVERWRITE_ALL};
/** The possible localized types of new access control entries. */
private String[] m_typesLocalized = new String[5];
/**
* Public constructor.
*
* @param jsp an initialized JSP action element
*/
public CmsChacc(CmsJspActionElement jsp) {
super(jsp);
m_errorMessages.clear();
}
/**
* Public constructor with JSP variables.
*
* @param context the JSP page context
* @param req the JSP request
* @param res the JSP response
*/
public CmsChacc(PageContext context, HttpServletRequest req, HttpServletResponse res) {
this(new CmsJspActionElement(context, req, res));
}
/**
* Builds a detail view selector.
*
* @param wp the dialog object
* @return the HTML code for the detail view selector
*/
public static String buildSummaryDetailsButtons(CmsDialog wp) {
StringBuffer result = new StringBuffer(512);
// create detail view selector
result.append("
\n\n\t");
result.append(wp.key(Messages.GUI_PERMISSION_SELECT_VIEW_0));
result.append(" \n");
String selectedView = wp.getSettings().getPermissionDetailView();
result.append("\t\n\t\n");
result.append(" \n
\n");
return result.toString();
}
/**
* Adds a new access control entry to the resource.
*
* @return true if a new ace was created, otherwise false
*/
public boolean actionAddAce() {
String file = getParamResource();
String name = getParamName();
String type = getParamType();
int arrayPosition = -1;
try {
arrayPosition = Integer.parseInt(type);
} catch (Exception e) {
// can usually be ignored
if (LOG.isInfoEnabled()) {
LOG.info(e.getLocalizedMessage());
}
}
if (checkNewEntry(name, arrayPosition)) {
String permissionString = "";
if (getInheritOption() && getSettings().getUserSettings().getDialogPermissionsInheritOnFolder()) {
// inherit permissions on folders if setting is enabled
permissionString = "+i";
}
try {
// lock resource if autolock is enabled
checkLock(getParamResource());
if (name.equals(key(Messages.GUI_LABEL_ALLOTHERS_0))) {
getCms().chacc(
file,
getTypes(false)[arrayPosition],
CmsAccessControlEntry.PRINCIPAL_ALL_OTHERS_NAME,
permissionString);
} else if (name.equals(key(Messages.GUI_LABEL_OVERWRITEALL_0))) {
getCms().chacc(
file,
getTypes(false)[arrayPosition],
CmsAccessControlEntry.PRINCIPAL_OVERWRITE_ALL_NAME,
permissionString);
} else {
if (getTypes(false)[arrayPosition].equalsIgnoreCase(CmsRole.PRINCIPAL_ROLE)) {
// if role, first check if we have to translate the role name
CmsRole role = CmsRole.valueOfRoleName(name);
if (role == null) {
// we need translation
Iterator it = CmsRole.getSystemRoles().iterator();
while (it.hasNext()) {
role = it.next();
if (role.getName(getLocale()).equalsIgnoreCase(name)) {
name = role.getRoleName();
break;
}
}
}
}
getCms().chacc(file, getTypes(false)[arrayPosition], name, permissionString);
}
return true;
} catch (CmsException e) {
m_errorMessages.add(e.getMessage());
if (LOG.isErrorEnabled()) {
LOG.error(e.getLocalizedMessage(), e);
}
}
}
return false;
}
/**
* Modifies the Internal Use flag of a resource.
* @param request the Http servlet request
*
* @return true if the operation was was successfully removed, otherwise false
*/
public boolean actionInternalUse(HttpServletRequest request) {
String internal = request.getParameter(PARAM_INTERNAL);
CmsResource resource;
boolean internalValue = false;
if (internal != null) {
internalValue = true;
}
try {
resource = getCms().readResource(getParamResource(), CmsResourceFilter.ALL);
int flags = resource.getFlags();
if (internalValue) {
flags |= CmsResource.FLAG_INTERNAL;
} else {
flags &= ~CmsResource.FLAG_INTERNAL;
}
getCms().lockResource(getParamResource());
getCms().chflags(getParamResource(), flags);
} catch (CmsException e) {
m_errorMessages.add(key(Messages.ERR_MODIFY_INTERNAL_FLAG_0));
if (LOG.isErrorEnabled()) {
LOG.error(e.getLocalizedMessage(), e);
}
return false;
}
return true;
}
/**
* Modifies a present access control entry for a resource.
*
* @param request the Http servlet request
* @return true if the modification worked, otherwise false
*/
public boolean actionModifyAce(HttpServletRequest request) {
String file = getParamResource();
// get request parameters
String name = getParamName();
String type = getParamType();
String inherit = request.getParameter(PARAM_INHERIT);
String overWriteInherited = request.getParameter(PARAM_OVERWRITEINHERITED);
String responsible = request.getParameter(PARAM_RESPONSIBLE);
// get the new permissions
Set permissionKeys = CmsPermissionSet.getPermissionKeys();
int allowValue = 0;
int denyValue = 0;
String key, param;
int value, paramInt;
Iterator i = permissionKeys.iterator();
// loop through all possible permissions
while (i.hasNext()) {
key = i.next();
value = CmsPermissionSet.getPermissionValue(key);
// set the right allowed and denied permissions from request parameters
try {
param = request.getParameter(value + PERMISSION_ALLOW);
paramInt = Integer.parseInt(param);
allowValue |= paramInt;
} catch (Exception e) {
// can usually be ignored
if (LOG.isInfoEnabled()) {
LOG.info(e.getLocalizedMessage());
}
}
try {
param = request.getParameter(value + PERMISSION_DENY);
paramInt = Integer.parseInt(param);
denyValue |= paramInt;
} catch (Exception e) {
// can usually be ignored
if (LOG.isInfoEnabled()) {
LOG.info(e.getLocalizedMessage());
}
}
}
// get the current Ace to get the current ace flags
try {
List allEntries = getCms().getAccessControlEntries(file, false);
int flags = 0;
for (int k = 0; k < allEntries.size(); k++) {
CmsAccessControlEntry curEntry = allEntries.get(k);
String curType = getEntryType(curEntry.getFlags(), false);
I_CmsPrincipal p;
try {
p = CmsPrincipal.readPrincipalIncludingHistory(getCms(), curEntry.getPrincipal());
} catch (CmsException e) {
p = null;
}
if (((p != null) && p.getName().equals(name) && curType.equals(type))) {
flags = curEntry.getFlags();
break;
} else if (p == null) {
// check if it is the case of a role
CmsRole role = CmsRole.valueOfId(curEntry.getPrincipal());
if ((role != null) && name.equals(role.getRoleName())) {
flags = curEntry.getFlags();
break;
} else if ((curEntry.getPrincipal().equals(CmsAccessControlEntry.PRINCIPAL_ALL_OTHERS_ID)
&& name.equals(CmsAccessControlEntry.PRINCIPAL_ALL_OTHERS_NAME))
|| (curEntry.getPrincipal().equals(CmsAccessControlEntry.PRINCIPAL_OVERWRITE_ALL_ID)
&& name.equals(CmsAccessControlEntry.PRINCIPAL_OVERWRITE_ALL_NAME))) {
flags = curEntry.getFlags();
break;
}
}
}
// modify the ace flags to determine inheritance of the current ace
if (Boolean.valueOf(inherit).booleanValue()) {
flags |= CmsAccessControlEntry.ACCESS_FLAGS_INHERIT;
} else {
flags &= ~CmsAccessControlEntry.ACCESS_FLAGS_INHERIT;
}
// modify the ace flags to determine overwriting of inherited ace
if (Boolean.valueOf(overWriteInherited).booleanValue()) {
flags |= CmsAccessControlEntry.ACCESS_FLAGS_OVERWRITE;
} else {
flags &= ~CmsAccessControlEntry.ACCESS_FLAGS_OVERWRITE;
}
if (Boolean.valueOf(responsible).booleanValue()) {
flags |= CmsAccessControlEntry.ACCESS_FLAGS_RESPONSIBLE;
} else {
flags &= ~CmsAccessControlEntry.ACCESS_FLAGS_RESPONSIBLE;
}
// lock resource if autolock is enabled
checkLock(getParamResource());
// try to change the access entry
if (name.equals(CmsAccessControlEntry.PRINCIPAL_ALL_OTHERS_ID.toString())) {
getCms().chacc(
file,
type,
CmsAccessControlEntry.PRINCIPAL_ALL_OTHERS_NAME,
allowValue,
denyValue,
flags);
} else if (name.equals(CmsAccessControlEntry.PRINCIPAL_OVERWRITE_ALL_ID.toString())) {
getCms().chacc(
file,
type,
CmsAccessControlEntry.PRINCIPAL_OVERWRITE_ALL_NAME,
allowValue,
denyValue,
flags);
} else {
getCms().chacc(file, type, name, allowValue, denyValue, flags);
}
return true;
} catch (CmsException e) {
m_errorMessages.add(key(Messages.ERR_CHACC_MODIFY_ENTRY_0));
if (LOG.isErrorEnabled()) {
LOG.error(e.getLocalizedMessage(), e);
}
return false;
}
}
/**
* Removes a present access control entry from the resource.
*
* @return true if the ace was successfully removed, otherwise false
*/
public boolean actionRemoveAce() {
String file = getParamResource();
String name = getParamName();
String type = getParamType();
String uuid = getParamUuid();
try {
// lock resource if autolock is enabled
checkLock(getParamResource());
// check if it is the case of a role
CmsRole role = CmsRole.valueOfGroupName(name);
if (role != null) {
// translate the internal group name to a role name
name = role.getFqn();
}
try {
getCms().rmacc(file, type, uuid);
} catch (CmsException e) {
LOG.warn(e.getLocalizedMessage(), e);
getCms().rmacc(file, type, name);
}
return true;
} catch (CmsException e) {
m_errorMessages.add(key(Messages.ERR_CHACC_DELETE_ENTRY_0));
if (LOG.isErrorEnabled()) {
LOG.error(e.getLocalizedMessage(), e);
}
return false;
}
}
/**
* Builds a String with HTML code to display the users access rights for the current resource.
*
* @return HTML String with the access rights of the current user
*/
public String buildCurrentPermissions() {
StringBuffer result = new StringBuffer(
dialogToggleStart(
key(Messages.GUI_PERMISSION_USER_0),
"userpermissions",
getSettings().getUserSettings().getDialogExpandUserPermissions()));
result.append(dialogWhiteBoxStart());
try {
result.append(buildPermissionEntryForm(
getSettings().getUser().getId(),
buildPermissionsForCurrentUser(),
false,
false));
} catch (CmsException e) {
// should never happen
if (LOG.isErrorEnabled()) {
LOG.error(e.getLocalizedMessage(), e);
}
}
result.append(dialogWhiteBoxEnd());
result.append("
* * @return all error messages */ public String buildErrorMessages() { StringBuffer result = new StringBuffer(8); String errorMessages = getErrorMessagesString(); if (!"".equals(errorMessages)) { result.append(dialogBlock(HTML_START, key(Messages.GUI_PERMISSION_ERROR_0), true)); result.append("").append(errorMessages).append(""); result.append(dialogBlockEnd()); } return result.toString(); } /** * Builds a String with HTML code to display the responsible users of a resource.
*
* @param show true the responsible list is open
* @return HTML code for the responsible users of the current resource
*/
public String buildResponsibleList(boolean show) {
List