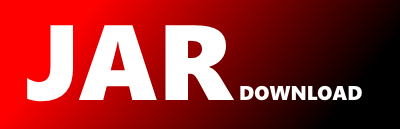
org.opencms.xml.containerpage.I_CmsFormatterBean Maven / Gradle / Ivy
Show all versions of opencms-test Show documentation
/*
* This library is part of OpenCms -
* the Open Source Content Management System
*
* Copyright (c) Alkacon Software GmbH & Co. KG (http://www.alkacon.com)
*
* This library is free software; you can redistribute it and/or
* modify it under the terms of the GNU Lesser General Public
* License as published by the Free Software Foundation; either
* version 2.1 of the License, or (at your option) any later version.
*
* This library is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* For further information about Alkacon Software, please see the
* company website: http://www.alkacon.com
*
* For further information about OpenCms, please see the
* project website: http://www.opencms.org
*
* You should have received a copy of the GNU Lesser General Public
* License along with this library; if not, write to the Free Software
* Foundation, Inc., 59 Temple Place, Suite 330, Boston, MA 02111-1307 USA
*/
package org.opencms.xml.containerpage;
import org.opencms.util.CmsUUID;
import org.opencms.xml.content.CmsXmlContentProperty;
import java.util.Collection;
import java.util.List;
import java.util.Locale;
import java.util.Map;
import java.util.Set;
/**
* Interface representing a configured formatter.
*/
public interface I_CmsFormatterBean {
/**
* Returns the formatter container type.
*
* If this is "*", then the formatter is a width based formatter.
*
* @return the formatter container type
*/
Set getContainerTypes();
/**
* Gets the CSS head includes.
*
* @return the CSS head includes
*/
Set getCssHeadIncludes();
/**
* Returns the id of this formatter.
*
* This method may return null because the id is not always defined for formatters, e.g. for those formatters declared in a schema.
*
* @return the formatter id
*/
String getId();
/**
* Gets the inline CSS snippets.
*
* @return the inline CSS snippets
*/
String getInlineCss();
/**
* Gets the inline JS snippets.
*
* @return the inline JS snippets
*/
String getInlineJavascript();
/**
* Gets the Javascript head includes.
*
* @return the head includes
*/
List getJavascriptHeadIncludes();
/**
* Returns the root path of the formatter JSP in the OpenCms VFS.
*
* @return the root path of the formatter JSP in the OpenCms VFS.
*/
String getJspRootPath();
/**
* Returns the structure id of the JSP resource for this formatter.
*
* @return the structure id of the JSP resource for this formatter
*/
CmsUUID getJspStructureId();
/**
* Returns the location this formatter was defined in.
*
* This will be an OpenCms VFS root path, either to the XML schema XSD, or the
* configuration file this formatter was defined in, or to the JSP that
* makes up this formatter.
*
* @return the location this formatter was defined in
*/
String getLocation();
/**
* Returns the maximum formatter width.
*
* If this is not set, then {@link Integer#MAX_VALUE} is returned.
*
* @return the maximum formatter width
*/
int getMaxWidth();
/**
* Returns the minimum formatter width.
*
* If this is not set, then -1
is returned.
*
* @return the minimum formatter width
*/
int getMinWidth();
/**
* Gets the nice name for this formatter.
*
* @param locale the locale
*
* @return the nice name for this formatter
*/
String getNiceName(Locale locale);
/**
* Gets the rank.
*
* @return the rank
*/
int getRank();
/**
* Gets the resource type names.
*
* @return the resource type names
*/
Collection getResourceTypeNames();
/**
* Gets the defined settings.
*
* @return the defined settings
*/
Map getSettings();
/**
* Returns if this formatter has nested containers.
*
* @return true
if this formatter has nested containers
*/
boolean hasNestedContainers();
/**
* Returns true if the formatter is automatically enabled.
*
* @return true if the formatter is automatically enabled
*/
boolean isAutoEnabled();
/**
* Returns true if the formatter can be used for detail views.
*
* @return true if the formatter can be used for detail views
*/
boolean isDetailFormatter();
/**
* Returns whether this formatter should be used by the 'display' tag.
*
* @return true
if this formatter should be used by the 'display' tag
*/
boolean isDisplayFormatter();
/**
* Returns true if the formatter is from a formatter configuration file.
*
* @return formatter f
*/
boolean isFromFormatterConfigFile();
/**
* Returns true if this formatter should match all type/width combinations.
*
* @return true if this formatter should match all type/width combinations
*/
boolean isMatchAll();
/**
* Indicates if this formatter is to be used as preview in the ADE gallery GUI.
*
* @return true
if this formatter is to be used as preview in the ADE gallery GUI
*/
boolean isPreviewFormatter();
/**
* Returns true
in case an XML content formatted with this formatter should be included in the
* online full text search.
*
* @return true
in case an XML content formatted with this formatter should be included in the
* online full text search
*/
boolean isSearchContent();
/**
* Returns true
in case this formatter is based on type information.
*
* @return true
in case this formatter is based on type information
*/
boolean isTypeFormatter();
/**
* Sets the JSP structure id.
*
* @param structureId the jsp structure id
*/
void setJspStructureId(CmsUUID structureId);
}