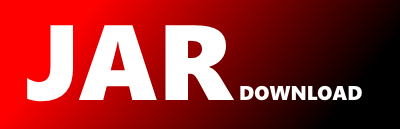
org.opencypher.tools.xml.Reference Maven / Gradle / Ivy
/*
* Copyright (c) 2015-2019 "Neo Technology,"
* Network Engine for Objects in Lund AB [http://neotechnology.com]
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
* Attribution Notice under the terms of the Apache License 2.0
*
* This work was created by the collective efforts of the openCypher community.
* Without limiting the terms of Section 6, any Derivative Work that is not
* approved by the public consensus process of the openCypher Implementers Group
* should not be described as “Cypher” (and Cypher® is a registered trademark of
* Neo4j Inc.) or as "openCypher". Extensions by implementers or prototypes or
* proposals for change that have been documented or implemented should only be
* described as "implementation extensions to Cypher" or as "proposed changes to
* Cypher that are not yet approved by the openCypher community".
*/
package org.opencypher.tools.xml;
import java.io.Serializable;
import java.lang.invoke.MethodHandle;
import java.lang.invoke.MethodHandles;
import static java.lang.invoke.MethodHandles.filterReturnValue;
import static org.opencypher.tools.Reflection.methodHandle;
/**
* DSL for getting {@linkplain MethodHandle method handles} from method references.
*/
interface Reference extends Serializable
{
static Function function( Function reference )
{
return reference;
}
static BiFunction biFunction( BiFunction reference )
{
return reference;
}
static ToIntFunction toInt( ToIntFunction reference )
{
return reference;
}
static ToLongFunction toLong( ToLongFunction reference )
{
return reference;
}
static ToBoolFunction toBool( ToBoolFunction reference )
{
return reference;
}
static ToDoubleFunction toDouble( ToDoubleFunction reference )
{
return reference;
}
interface Function extends Reference
{
R apply( T t );
default Function then( Function super R, ? extends V> after )
{
return new Function()
{
@Override
public V apply( T value )
{
return after.apply( Function.this.apply( value ) );
}
@Override
public MethodHandle mh()
{
return filterReturnValue( Function.this.mh(), after.mh() );
}
};
}
}
interface IntFunction extends Reference
{
R apply( int value );
}
interface ToIntFunction extends Reference
{
int applyAsInt( T value );
default Function then( IntFunction after )
{
return new Function()
{
@Override
public R apply( T value )
{
return after.apply( applyAsInt( value ) );
}
@Override
public MethodHandle mh()
{
return filterReturnValue( ToIntFunction.this.mh(), after.mh() );
}
};
}
}
interface LongFunction extends Reference
{
R apply( long value );
}
interface ToLongFunction extends Reference
{
long applyAsLong( T value );
default Function then( LongFunction after )
{
return new Function()
{
@Override
public R apply( T value )
{
return after.apply( applyAsLong( value ) );
}
@Override
public MethodHandle mh()
{
return filterReturnValue( ToLongFunction.this.mh(), after.mh() );
}
};
}
}
interface BoolFunction extends Reference
{
R apply( boolean value );
}
interface ToBoolFunction extends Reference
{
boolean applyAsBool( T value );
default Function then( BoolFunction after )
{
return new Function()
{
@Override
public R apply( T value )
{
return after.apply( applyAsBool( value ) );
}
@Override
public MethodHandle mh()
{
return filterReturnValue( ToBoolFunction.this.mh(), after.mh() );
}
};
}
}
interface DoubleFunction extends Reference
{
R apply( double value );
}
interface ToDoubleFunction extends Reference
{
double applyAsDouble( T value );
default Function then( DoubleFunction after )
{
return new Function()
{
@Override
public R apply( T value )
{
return after.apply( applyAsDouble( value ) );
}
@Override
public MethodHandle mh()
{
return filterReturnValue( ToDoubleFunction.this.mh(), after.mh() );
}
};
}
}
interface BiFunction extends Reference
{
R apply( T t, U u );
}
default MethodHandle mh()
{
return methodHandle( MethodHandles.lookup(), this );
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy