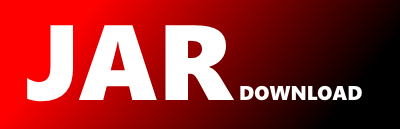
org.opendatadiscovery.client.api.OpenDataDiscoveryIngestionApi Maven / Gradle / Ivy
Show all versions of ingestion-contract-client Show documentation
package org.opendatadiscovery.client.api;
import org.opendatadiscovery.client.ApiClient;
import org.opendatadiscovery.client.model.CompactDataEntityList;
import org.opendatadiscovery.client.model.DataEntityList;
import org.opendatadiscovery.client.model.DataSourceList;
import org.opendatadiscovery.client.model.DatasetStatisticsList;
import org.opendatadiscovery.client.model.IngestionAlertList;
import org.opendatadiscovery.client.model.MetricSetList;
import java.util.HashMap;
import java.util.List;
import java.util.Locale;
import java.util.Map;
import java.util.stream.Collectors;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.util.LinkedMultiValueMap;
import org.springframework.util.MultiValueMap;
import org.springframework.core.ParameterizedTypeReference;
import org.springframework.web.reactive.function.client.WebClient.ResponseSpec;
import org.springframework.web.reactive.function.client.WebClientResponseException;
import org.springframework.core.io.FileSystemResource;
import org.springframework.http.HttpHeaders;
import org.springframework.http.HttpMethod;
import org.springframework.http.HttpStatus;
import org.springframework.http.MediaType;
import org.springframework.http.ResponseEntity;
import reactor.core.publisher.Mono;
import reactor.core.publisher.Flux;
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-04-17T12:42:16.563637609Z[Etc/UTC]")
public class OpenDataDiscoveryIngestionApi {
private ApiClient apiClient;
public OpenDataDiscoveryIngestionApi() {
this(new ApiClient());
}
@Autowired
public OpenDataDiscoveryIngestionApi(ApiClient apiClient) {
this.apiClient = apiClient;
}
public ApiClient getApiClient() {
return apiClient;
}
public void setApiClient(ApiClient apiClient) {
this.apiClient = apiClient;
}
/**
* Alerts target catalog about ingestion issues
* Alerts target catalog about ingestion issues
* 201 - Created
* @param ingestionAlertList The ingestionAlertList parameter
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
private ResponseSpec createAlertsRequestCreation(IngestionAlertList ingestionAlertList) throws WebClientResponseException {
Object postBody = ingestionAlertList;
// create path and map variables
final Map pathParams = new HashMap();
final MultiValueMap queryParams = new LinkedMultiValueMap();
final HttpHeaders headerParams = new HttpHeaders();
final MultiValueMap cookieParams = new LinkedMultiValueMap();
final MultiValueMap formParams = new LinkedMultiValueMap();
final String[] localVarAccepts = { };
final List localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final MediaType localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { };
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return apiClient.invokeAPI("/ingestion/alerts", HttpMethod.POST, pathParams, queryParams, postBody, headerParams, cookieParams, formParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Alerts target catalog about ingestion issues
* Alerts target catalog about ingestion issues
* 201 - Created
* @param ingestionAlertList The ingestionAlertList parameter
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public Mono createAlerts(IngestionAlertList ingestionAlertList) throws WebClientResponseException {
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return createAlertsRequestCreation(ingestionAlertList).bodyToMono(localVarReturnType);
}
/**
* Alerts target catalog about ingestion issues
* Alerts target catalog about ingestion issues
* 201 - Created
* @param ingestionAlertList The ingestionAlertList parameter
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public Mono> createAlertsWithHttpInfo(IngestionAlertList ingestionAlertList) throws WebClientResponseException {
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return createAlertsRequestCreation(ingestionAlertList).toEntity(localVarReturnType);
}
/**
* Alerts target catalog about ingestion issues
* Alerts target catalog about ingestion issues
* 201 - Created
* @param ingestionAlertList The ingestionAlertList parameter
* @return ResponseSpec
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public ResponseSpec createAlertsWithResponseSpec(IngestionAlertList ingestionAlertList) throws WebClientResponseException {
return createAlertsRequestCreation(ingestionAlertList);
}
/**
* Creates data sources in the target catalog
* Creates data sources in the target catalog
*
201 - Created
* @param dataSourceList The dataSourceList parameter
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
private ResponseSpec createDataSourceRequestCreation(DataSourceList dataSourceList) throws WebClientResponseException {
Object postBody = dataSourceList;
// create path and map variables
final Map pathParams = new HashMap();
final MultiValueMap queryParams = new LinkedMultiValueMap();
final HttpHeaders headerParams = new HttpHeaders();
final MultiValueMap cookieParams = new LinkedMultiValueMap();
final MultiValueMap formParams = new LinkedMultiValueMap();
final String[] localVarAccepts = { };
final List localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final MediaType localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { };
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return apiClient.invokeAPI("/ingestion/datasources", HttpMethod.POST, pathParams, queryParams, postBody, headerParams, cookieParams, formParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Creates data sources in the target catalog
* Creates data sources in the target catalog
* 201 - Created
* @param dataSourceList The dataSourceList parameter
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public Mono createDataSource(DataSourceList dataSourceList) throws WebClientResponseException {
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return createDataSourceRequestCreation(dataSourceList).bodyToMono(localVarReturnType);
}
/**
* Creates data sources in the target catalog
* Creates data sources in the target catalog
* 201 - Created
* @param dataSourceList The dataSourceList parameter
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public Mono> createDataSourceWithHttpInfo(DataSourceList dataSourceList) throws WebClientResponseException {
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return createDataSourceRequestCreation(dataSourceList).toEntity(localVarReturnType);
}
/**
* Creates data sources in the target catalog
* Creates data sources in the target catalog
* 201 - Created
* @param dataSourceList The dataSourceList parameter
* @return ResponseSpec
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public ResponseSpec createDataSourceWithResponseSpec(DataSourceList dataSourceList) throws WebClientResponseException {
return createDataSourceRequestCreation(dataSourceList);
}
/**
* Get data entity by oddrn in Data Entity Groups
* Searches for data entities in the target catalog by oddrn of a DEG they are currently part of
*
200 - OK
* @param oddrn The oddrn parameter
* @return CompactDataEntityList
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
private ResponseSpec getDataEntitiesByDEGOddrnRequestCreation(String oddrn) throws WebClientResponseException {
Object postBody = null;
// verify the required parameter 'oddrn' is set
if (oddrn == null) {
throw new WebClientResponseException("Missing the required parameter 'oddrn' when calling getDataEntitiesByDEGOddrn", HttpStatus.BAD_REQUEST.value(), HttpStatus.BAD_REQUEST.getReasonPhrase(), null, null, null);
}
// create path and map variables
final Map pathParams = new HashMap();
final MultiValueMap queryParams = new LinkedMultiValueMap();
final HttpHeaders headerParams = new HttpHeaders();
final MultiValueMap cookieParams = new LinkedMultiValueMap();
final MultiValueMap formParams = new LinkedMultiValueMap();
queryParams.putAll(apiClient.parameterToMultiValueMap(null, "oddrn", oddrn));
final String[] localVarAccepts = {
"application/json"
};
final List localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = { };
final MediaType localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { };
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return apiClient.invokeAPI("/ingestion/entities/degs/children", HttpMethod.GET, pathParams, queryParams, postBody, headerParams, cookieParams, formParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Get data entity by oddrn in Data Entity Groups
* Searches for data entities in the target catalog by oddrn of a DEG they are currently part of
* 200 - OK
* @param oddrn The oddrn parameter
* @return CompactDataEntityList
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public Mono getDataEntitiesByDEGOddrn(String oddrn) throws WebClientResponseException {
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return getDataEntitiesByDEGOddrnRequestCreation(oddrn).bodyToMono(localVarReturnType);
}
/**
* Get data entity by oddrn in Data Entity Groups
* Searches for data entities in the target catalog by oddrn of a DEG they are currently part of
* 200 - OK
* @param oddrn The oddrn parameter
* @return ResponseEntity<CompactDataEntityList>
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public Mono> getDataEntitiesByDEGOddrnWithHttpInfo(String oddrn) throws WebClientResponseException {
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return getDataEntitiesByDEGOddrnRequestCreation(oddrn).toEntity(localVarReturnType);
}
/**
* Get data entity by oddrn in Data Entity Groups
* Searches for data entities in the target catalog by oddrn of a DEG they are currently part of
* 200 - OK
* @param oddrn The oddrn parameter
* @return ResponseSpec
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public ResponseSpec getDataEntitiesByDEGOddrnWithResponseSpec(String oddrn) throws WebClientResponseException {
return getDataEntitiesByDEGOddrnRequestCreation(oddrn);
}
/**
* Ingests list of metrics
* Ingests list of metrics
*
201 - Created
* @param metricSetList The metricSetList parameter
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
private ResponseSpec ingestMetricsRequestCreation(MetricSetList metricSetList) throws WebClientResponseException {
Object postBody = metricSetList;
// verify the required parameter 'metricSetList' is set
if (metricSetList == null) {
throw new WebClientResponseException("Missing the required parameter 'metricSetList' when calling ingestMetrics", HttpStatus.BAD_REQUEST.value(), HttpStatus.BAD_REQUEST.getReasonPhrase(), null, null, null);
}
// create path and map variables
final Map pathParams = new HashMap();
final MultiValueMap queryParams = new LinkedMultiValueMap();
final HttpHeaders headerParams = new HttpHeaders();
final MultiValueMap cookieParams = new LinkedMultiValueMap();
final MultiValueMap formParams = new LinkedMultiValueMap();
final String[] localVarAccepts = { };
final List localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final MediaType localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { };
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return apiClient.invokeAPI("/ingestion/metrics", HttpMethod.POST, pathParams, queryParams, postBody, headerParams, cookieParams, formParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Ingests list of metrics
* Ingests list of metrics
* 201 - Created
* @param metricSetList The metricSetList parameter
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public Mono ingestMetrics(MetricSetList metricSetList) throws WebClientResponseException {
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return ingestMetricsRequestCreation(metricSetList).bodyToMono(localVarReturnType);
}
/**
* Ingests list of metrics
* Ingests list of metrics
* 201 - Created
* @param metricSetList The metricSetList parameter
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public Mono> ingestMetricsWithHttpInfo(MetricSetList metricSetList) throws WebClientResponseException {
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return ingestMetricsRequestCreation(metricSetList).toEntity(localVarReturnType);
}
/**
* Ingests list of metrics
* Ingests list of metrics
* 201 - Created
* @param metricSetList The metricSetList parameter
* @return ResponseSpec
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public ResponseSpec ingestMetricsWithResponseSpec(MetricSetList metricSetList) throws WebClientResponseException {
return ingestMetricsRequestCreation(metricSetList);
}
/**
* Ingests list of data entities
* Ingests list of data entities
*
201 - Created
* @param dataEntityList The dataEntityList parameter
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
private ResponseSpec postDataEntityListRequestCreation(DataEntityList dataEntityList) throws WebClientResponseException {
Object postBody = dataEntityList;
// verify the required parameter 'dataEntityList' is set
if (dataEntityList == null) {
throw new WebClientResponseException("Missing the required parameter 'dataEntityList' when calling postDataEntityList", HttpStatus.BAD_REQUEST.value(), HttpStatus.BAD_REQUEST.getReasonPhrase(), null, null, null);
}
// create path and map variables
final Map pathParams = new HashMap();
final MultiValueMap queryParams = new LinkedMultiValueMap();
final HttpHeaders headerParams = new HttpHeaders();
final MultiValueMap cookieParams = new LinkedMultiValueMap();
final MultiValueMap formParams = new LinkedMultiValueMap();
final String[] localVarAccepts = { };
final List localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final MediaType localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { };
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return apiClient.invokeAPI("/ingestion/entities", HttpMethod.POST, pathParams, queryParams, postBody, headerParams, cookieParams, formParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Ingests list of data entities
* Ingests list of data entities
* 201 - Created
* @param dataEntityList The dataEntityList parameter
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public Mono postDataEntityList(DataEntityList dataEntityList) throws WebClientResponseException {
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return postDataEntityListRequestCreation(dataEntityList).bodyToMono(localVarReturnType);
}
/**
* Ingests list of data entities
* Ingests list of data entities
* 201 - Created
* @param dataEntityList The dataEntityList parameter
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public Mono> postDataEntityListWithHttpInfo(DataEntityList dataEntityList) throws WebClientResponseException {
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return postDataEntityListRequestCreation(dataEntityList).toEntity(localVarReturnType);
}
/**
* Ingests list of data entities
* Ingests list of data entities
* 201 - Created
* @param dataEntityList The dataEntityList parameter
* @return ResponseSpec
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public ResponseSpec postDataEntityListWithResponseSpec(DataEntityList dataEntityList) throws WebClientResponseException {
return postDataEntityListRequestCreation(dataEntityList);
}
/**
* Ingests list of stats for data sets
* Ingests list of stats for data sets
*
201 - Created
* @param datasetStatisticsList The datasetStatisticsList parameter
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
private ResponseSpec postDataSetStatsListRequestCreation(DatasetStatisticsList datasetStatisticsList) throws WebClientResponseException {
Object postBody = datasetStatisticsList;
// verify the required parameter 'datasetStatisticsList' is set
if (datasetStatisticsList == null) {
throw new WebClientResponseException("Missing the required parameter 'datasetStatisticsList' when calling postDataSetStatsList", HttpStatus.BAD_REQUEST.value(), HttpStatus.BAD_REQUEST.getReasonPhrase(), null, null, null);
}
// create path and map variables
final Map pathParams = new HashMap();
final MultiValueMap queryParams = new LinkedMultiValueMap();
final HttpHeaders headerParams = new HttpHeaders();
final MultiValueMap cookieParams = new LinkedMultiValueMap();
final MultiValueMap formParams = new LinkedMultiValueMap();
final String[] localVarAccepts = { };
final List localVarAccept = apiClient.selectHeaderAccept(localVarAccepts);
final String[] localVarContentTypes = {
"application/json"
};
final MediaType localVarContentType = apiClient.selectHeaderContentType(localVarContentTypes);
String[] localVarAuthNames = new String[] { };
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return apiClient.invokeAPI("/ingestion/entities/datasets/stats", HttpMethod.POST, pathParams, queryParams, postBody, headerParams, cookieParams, formParams, localVarAccept, localVarContentType, localVarAuthNames, localVarReturnType);
}
/**
* Ingests list of stats for data sets
* Ingests list of stats for data sets
* 201 - Created
* @param datasetStatisticsList The datasetStatisticsList parameter
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public Mono postDataSetStatsList(DatasetStatisticsList datasetStatisticsList) throws WebClientResponseException {
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return postDataSetStatsListRequestCreation(datasetStatisticsList).bodyToMono(localVarReturnType);
}
/**
* Ingests list of stats for data sets
* Ingests list of stats for data sets
* 201 - Created
* @param datasetStatisticsList The datasetStatisticsList parameter
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public Mono> postDataSetStatsListWithHttpInfo(DatasetStatisticsList datasetStatisticsList) throws WebClientResponseException {
ParameterizedTypeReference localVarReturnType = new ParameterizedTypeReference() {};
return postDataSetStatsListRequestCreation(datasetStatisticsList).toEntity(localVarReturnType);
}
/**
* Ingests list of stats for data sets
* Ingests list of stats for data sets
* 201 - Created
* @param datasetStatisticsList The datasetStatisticsList parameter
* @return ResponseSpec
* @throws WebClientResponseException if an error occurs while attempting to invoke the API
*/
public ResponseSpec postDataSetStatsListWithResponseSpec(DatasetStatisticsList datasetStatisticsList) throws WebClientResponseException {
return postDataSetStatsListRequestCreation(datasetStatisticsList);
}
}