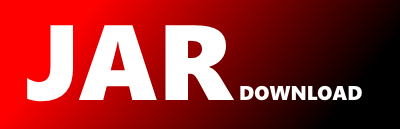
org.opendatadiscovery.client.model.DataEntity Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ingestion-contract-client Show documentation
Show all versions of ingestion-contract-client Show documentation
Ingestion Contract WebFlux Client defines OpenDataDiscovery APIs and models for Spring WebClient
/*
* OpenDataDiscovery API Contract
* OpenDataDiscovery API Contract
*
* The version of the OpenAPI document: 0.0.1
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package org.opendatadiscovery.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import java.time.OffsetDateTime;
import java.util.ArrayList;
import java.util.List;
import org.opendatadiscovery.client.model.DataConsumer;
import org.opendatadiscovery.client.model.DataEntityGroup;
import org.opendatadiscovery.client.model.DataEntityType;
import org.opendatadiscovery.client.model.DataInput;
import org.opendatadiscovery.client.model.DataQualityTest;
import org.opendatadiscovery.client.model.DataQualityTestRun;
import org.opendatadiscovery.client.model.DataRelationship;
import org.opendatadiscovery.client.model.DataSet;
import org.opendatadiscovery.client.model.DataTransformer;
import org.opendatadiscovery.client.model.DataTransformerRun;
import org.opendatadiscovery.client.model.MetadataExtension;
import org.opendatadiscovery.client.model.Tag;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonTypeName;
/**
* DataEntity
*/
@JsonPropertyOrder({
DataEntity.JSON_PROPERTY_ODDRN,
DataEntity.JSON_PROPERTY_NAME,
DataEntity.JSON_PROPERTY_VERSION,
DataEntity.JSON_PROPERTY_DESCRIPTION,
DataEntity.JSON_PROPERTY_OWNER,
DataEntity.JSON_PROPERTY_METADATA,
DataEntity.JSON_PROPERTY_TAGS,
DataEntity.JSON_PROPERTY_UPDATED_AT,
DataEntity.JSON_PROPERTY_CREATED_AT,
DataEntity.JSON_PROPERTY_TYPE,
DataEntity.JSON_PROPERTY_DATASET,
DataEntity.JSON_PROPERTY_DATA_TRANSFORMER,
DataEntity.JSON_PROPERTY_DATA_TRANSFORMER_RUN,
DataEntity.JSON_PROPERTY_DATA_QUALITY_TEST,
DataEntity.JSON_PROPERTY_DATA_QUALITY_TEST_RUN,
DataEntity.JSON_PROPERTY_DATA_INPUT,
DataEntity.JSON_PROPERTY_DATA_CONSUMER,
DataEntity.JSON_PROPERTY_DATA_ENTITY_GROUP,
DataEntity.JSON_PROPERTY_DATA_RELATIONSHIP
})
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-04-17T12:42:16.563637609Z[Etc/UTC]")
public class DataEntity {
public static final String JSON_PROPERTY_ODDRN = "oddrn";
private String oddrn;
public static final String JSON_PROPERTY_NAME = "name";
private String name;
public static final String JSON_PROPERTY_VERSION = "version";
private String version;
public static final String JSON_PROPERTY_DESCRIPTION = "description";
private String description;
public static final String JSON_PROPERTY_OWNER = "owner";
private String owner;
public static final String JSON_PROPERTY_METADATA = "metadata";
private List metadata;
public static final String JSON_PROPERTY_TAGS = "tags";
private List tags;
public static final String JSON_PROPERTY_UPDATED_AT = "updated_at";
private OffsetDateTime updatedAt;
public static final String JSON_PROPERTY_CREATED_AT = "created_at";
private OffsetDateTime createdAt;
public static final String JSON_PROPERTY_TYPE = "type";
private DataEntityType type;
public static final String JSON_PROPERTY_DATASET = "dataset";
private DataSet dataset;
public static final String JSON_PROPERTY_DATA_TRANSFORMER = "data_transformer";
private DataTransformer dataTransformer;
public static final String JSON_PROPERTY_DATA_TRANSFORMER_RUN = "data_transformer_run";
private DataTransformerRun dataTransformerRun;
public static final String JSON_PROPERTY_DATA_QUALITY_TEST = "data_quality_test";
private DataQualityTest dataQualityTest;
public static final String JSON_PROPERTY_DATA_QUALITY_TEST_RUN = "data_quality_test_run";
private DataQualityTestRun dataQualityTestRun;
public static final String JSON_PROPERTY_DATA_INPUT = "data_input";
private DataInput dataInput;
public static final String JSON_PROPERTY_DATA_CONSUMER = "data_consumer";
private DataConsumer dataConsumer;
public static final String JSON_PROPERTY_DATA_ENTITY_GROUP = "data_entity_group";
private DataEntityGroup dataEntityGroup;
public static final String JSON_PROPERTY_DATA_RELATIONSHIP = "data_relationship";
private DataRelationship dataRelationship;
public DataEntity() {
}
public DataEntity oddrn(String oddrn) {
this.oddrn = oddrn;
return this;
}
/**
* Get oddrn
* @return oddrn
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_ODDRN)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getOddrn() {
return oddrn;
}
@JsonProperty(JSON_PROPERTY_ODDRN)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setOddrn(String oddrn) {
this.oddrn = oddrn;
}
public DataEntity name(String name) {
this.name = name;
return this;
}
/**
* Get name
* @return name
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_NAME)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getName() {
return name;
}
@JsonProperty(JSON_PROPERTY_NAME)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setName(String name) {
this.name = name;
}
public DataEntity version(String version) {
this.version = version;
return this;
}
/**
* Get version
* @return version
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_VERSION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getVersion() {
return version;
}
@JsonProperty(JSON_PROPERTY_VERSION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setVersion(String version) {
this.version = version;
}
public DataEntity description(String description) {
this.description = description;
return this;
}
/**
* Get description
* @return description
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_DESCRIPTION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getDescription() {
return description;
}
@JsonProperty(JSON_PROPERTY_DESCRIPTION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setDescription(String description) {
this.description = description;
}
public DataEntity owner(String owner) {
this.owner = owner;
return this;
}
/**
* Get owner
* @return owner
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_OWNER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getOwner() {
return owner;
}
@JsonProperty(JSON_PROPERTY_OWNER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setOwner(String owner) {
this.owner = owner;
}
public DataEntity metadata(List metadata) {
this.metadata = metadata;
return this;
}
public DataEntity addMetadataItem(MetadataExtension metadataItem) {
if (this.metadata == null) {
this.metadata = new ArrayList<>();
}
this.metadata.add(metadataItem);
return this;
}
/**
* Get metadata
* @return metadata
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_METADATA)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getMetadata() {
return metadata;
}
@JsonProperty(JSON_PROPERTY_METADATA)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setMetadata(List metadata) {
this.metadata = metadata;
}
public DataEntity tags(List tags) {
this.tags = tags;
return this;
}
public DataEntity addTagsItem(Tag tagsItem) {
if (this.tags == null) {
this.tags = new ArrayList<>();
}
this.tags.add(tagsItem);
return this;
}
/**
* Get tags
* @return tags
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_TAGS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getTags() {
return tags;
}
@JsonProperty(JSON_PROPERTY_TAGS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setTags(List tags) {
this.tags = tags;
}
public DataEntity updatedAt(OffsetDateTime updatedAt) {
this.updatedAt = updatedAt;
return this;
}
/**
* Get updatedAt
* @return updatedAt
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_UPDATED_AT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public OffsetDateTime getUpdatedAt() {
return updatedAt;
}
@JsonProperty(JSON_PROPERTY_UPDATED_AT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setUpdatedAt(OffsetDateTime updatedAt) {
this.updatedAt = updatedAt;
}
public DataEntity createdAt(OffsetDateTime createdAt) {
this.createdAt = createdAt;
return this;
}
/**
* Get createdAt
* @return createdAt
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_CREATED_AT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public OffsetDateTime getCreatedAt() {
return createdAt;
}
@JsonProperty(JSON_PROPERTY_CREATED_AT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setCreatedAt(OffsetDateTime createdAt) {
this.createdAt = createdAt;
}
public DataEntity type(DataEntityType type) {
this.type = type;
return this;
}
/**
* Get type
* @return type
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_TYPE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public DataEntityType getType() {
return type;
}
@JsonProperty(JSON_PROPERTY_TYPE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setType(DataEntityType type) {
this.type = type;
}
public DataEntity dataset(DataSet dataset) {
this.dataset = dataset;
return this;
}
/**
* Get dataset
* @return dataset
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_DATASET)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public DataSet getDataset() {
return dataset;
}
@JsonProperty(JSON_PROPERTY_DATASET)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setDataset(DataSet dataset) {
this.dataset = dataset;
}
public DataEntity dataTransformer(DataTransformer dataTransformer) {
this.dataTransformer = dataTransformer;
return this;
}
/**
* Get dataTransformer
* @return dataTransformer
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_DATA_TRANSFORMER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public DataTransformer getDataTransformer() {
return dataTransformer;
}
@JsonProperty(JSON_PROPERTY_DATA_TRANSFORMER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setDataTransformer(DataTransformer dataTransformer) {
this.dataTransformer = dataTransformer;
}
public DataEntity dataTransformerRun(DataTransformerRun dataTransformerRun) {
this.dataTransformerRun = dataTransformerRun;
return this;
}
/**
* Get dataTransformerRun
* @return dataTransformerRun
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_DATA_TRANSFORMER_RUN)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public DataTransformerRun getDataTransformerRun() {
return dataTransformerRun;
}
@JsonProperty(JSON_PROPERTY_DATA_TRANSFORMER_RUN)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setDataTransformerRun(DataTransformerRun dataTransformerRun) {
this.dataTransformerRun = dataTransformerRun;
}
public DataEntity dataQualityTest(DataQualityTest dataQualityTest) {
this.dataQualityTest = dataQualityTest;
return this;
}
/**
* Get dataQualityTest
* @return dataQualityTest
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_DATA_QUALITY_TEST)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public DataQualityTest getDataQualityTest() {
return dataQualityTest;
}
@JsonProperty(JSON_PROPERTY_DATA_QUALITY_TEST)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setDataQualityTest(DataQualityTest dataQualityTest) {
this.dataQualityTest = dataQualityTest;
}
public DataEntity dataQualityTestRun(DataQualityTestRun dataQualityTestRun) {
this.dataQualityTestRun = dataQualityTestRun;
return this;
}
/**
* Get dataQualityTestRun
* @return dataQualityTestRun
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_DATA_QUALITY_TEST_RUN)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public DataQualityTestRun getDataQualityTestRun() {
return dataQualityTestRun;
}
@JsonProperty(JSON_PROPERTY_DATA_QUALITY_TEST_RUN)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setDataQualityTestRun(DataQualityTestRun dataQualityTestRun) {
this.dataQualityTestRun = dataQualityTestRun;
}
public DataEntity dataInput(DataInput dataInput) {
this.dataInput = dataInput;
return this;
}
/**
* Get dataInput
* @return dataInput
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_DATA_INPUT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public DataInput getDataInput() {
return dataInput;
}
@JsonProperty(JSON_PROPERTY_DATA_INPUT)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setDataInput(DataInput dataInput) {
this.dataInput = dataInput;
}
public DataEntity dataConsumer(DataConsumer dataConsumer) {
this.dataConsumer = dataConsumer;
return this;
}
/**
* Get dataConsumer
* @return dataConsumer
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_DATA_CONSUMER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public DataConsumer getDataConsumer() {
return dataConsumer;
}
@JsonProperty(JSON_PROPERTY_DATA_CONSUMER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setDataConsumer(DataConsumer dataConsumer) {
this.dataConsumer = dataConsumer;
}
public DataEntity dataEntityGroup(DataEntityGroup dataEntityGroup) {
this.dataEntityGroup = dataEntityGroup;
return this;
}
/**
* Get dataEntityGroup
* @return dataEntityGroup
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_DATA_ENTITY_GROUP)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public DataEntityGroup getDataEntityGroup() {
return dataEntityGroup;
}
@JsonProperty(JSON_PROPERTY_DATA_ENTITY_GROUP)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setDataEntityGroup(DataEntityGroup dataEntityGroup) {
this.dataEntityGroup = dataEntityGroup;
}
public DataEntity dataRelationship(DataRelationship dataRelationship) {
this.dataRelationship = dataRelationship;
return this;
}
/**
* Get dataRelationship
* @return dataRelationship
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_DATA_RELATIONSHIP)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public DataRelationship getDataRelationship() {
return dataRelationship;
}
@JsonProperty(JSON_PROPERTY_DATA_RELATIONSHIP)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setDataRelationship(DataRelationship dataRelationship) {
this.dataRelationship = dataRelationship;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
DataEntity dataEntity = (DataEntity) o;
return Objects.equals(this.oddrn, dataEntity.oddrn) &&
Objects.equals(this.name, dataEntity.name) &&
Objects.equals(this.version, dataEntity.version) &&
Objects.equals(this.description, dataEntity.description) &&
Objects.equals(this.owner, dataEntity.owner) &&
Objects.equals(this.metadata, dataEntity.metadata) &&
Objects.equals(this.tags, dataEntity.tags) &&
Objects.equals(this.updatedAt, dataEntity.updatedAt) &&
Objects.equals(this.createdAt, dataEntity.createdAt) &&
Objects.equals(this.type, dataEntity.type) &&
Objects.equals(this.dataset, dataEntity.dataset) &&
Objects.equals(this.dataTransformer, dataEntity.dataTransformer) &&
Objects.equals(this.dataTransformerRun, dataEntity.dataTransformerRun) &&
Objects.equals(this.dataQualityTest, dataEntity.dataQualityTest) &&
Objects.equals(this.dataQualityTestRun, dataEntity.dataQualityTestRun) &&
Objects.equals(this.dataInput, dataEntity.dataInput) &&
Objects.equals(this.dataConsumer, dataEntity.dataConsumer) &&
Objects.equals(this.dataEntityGroup, dataEntity.dataEntityGroup) &&
Objects.equals(this.dataRelationship, dataEntity.dataRelationship);
}
@Override
public int hashCode() {
return Objects.hash(oddrn, name, version, description, owner, metadata, tags, updatedAt, createdAt, type, dataset, dataTransformer, dataTransformerRun, dataQualityTest, dataQualityTestRun, dataInput, dataConsumer, dataEntityGroup, dataRelationship);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class DataEntity {\n");
sb.append(" oddrn: ").append(toIndentedString(oddrn)).append("\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" version: ").append(toIndentedString(version)).append("\n");
sb.append(" description: ").append(toIndentedString(description)).append("\n");
sb.append(" owner: ").append(toIndentedString(owner)).append("\n");
sb.append(" metadata: ").append(toIndentedString(metadata)).append("\n");
sb.append(" tags: ").append(toIndentedString(tags)).append("\n");
sb.append(" updatedAt: ").append(toIndentedString(updatedAt)).append("\n");
sb.append(" createdAt: ").append(toIndentedString(createdAt)).append("\n");
sb.append(" type: ").append(toIndentedString(type)).append("\n");
sb.append(" dataset: ").append(toIndentedString(dataset)).append("\n");
sb.append(" dataTransformer: ").append(toIndentedString(dataTransformer)).append("\n");
sb.append(" dataTransformerRun: ").append(toIndentedString(dataTransformerRun)).append("\n");
sb.append(" dataQualityTest: ").append(toIndentedString(dataQualityTest)).append("\n");
sb.append(" dataQualityTestRun: ").append(toIndentedString(dataQualityTestRun)).append("\n");
sb.append(" dataInput: ").append(toIndentedString(dataInput)).append("\n");
sb.append(" dataConsumer: ").append(toIndentedString(dataConsumer)).append("\n");
sb.append(" dataEntityGroup: ").append(toIndentedString(dataEntityGroup)).append("\n");
sb.append(" dataRelationship: ").append(toIndentedString(dataRelationship)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy