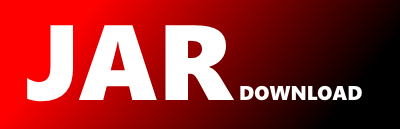
org.opendatadiscovery.client.model.DataSetField Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ingestion-contract-client Show documentation
Show all versions of ingestion-contract-client Show documentation
Ingestion Contract WebFlux Client defines OpenDataDiscovery APIs and models for Spring WebClient
/*
* OpenDataDiscovery API Contract
* OpenDataDiscovery API Contract
*
* The version of the OpenAPI document: 0.0.1
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package org.opendatadiscovery.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import java.util.ArrayList;
import java.util.List;
import org.opendatadiscovery.client.model.DataSetFieldEnumValue;
import org.opendatadiscovery.client.model.DataSetFieldStat;
import org.opendatadiscovery.client.model.DataSetFieldType;
import org.opendatadiscovery.client.model.MetadataExtension;
import org.opendatadiscovery.client.model.Tag;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonTypeName;
/**
* DataSetField
*/
@JsonPropertyOrder({
DataSetField.JSON_PROPERTY_ODDRN,
DataSetField.JSON_PROPERTY_NAME,
DataSetField.JSON_PROPERTY_VERSION,
DataSetField.JSON_PROPERTY_DESCRIPTION,
DataSetField.JSON_PROPERTY_OWNER,
DataSetField.JSON_PROPERTY_METADATA,
DataSetField.JSON_PROPERTY_TAGS,
DataSetField.JSON_PROPERTY_PARENT_FIELD_ODDRN,
DataSetField.JSON_PROPERTY_TYPE,
DataSetField.JSON_PROPERTY_IS_PRIMARY_KEY,
DataSetField.JSON_PROPERTY_IS_SORT_KEY,
DataSetField.JSON_PROPERTY_IS_KEY,
DataSetField.JSON_PROPERTY_IS_VALUE,
DataSetField.JSON_PROPERTY_REFERENCE_ODDRN,
DataSetField.JSON_PROPERTY_DEFAULT_VALUE,
DataSetField.JSON_PROPERTY_STATS,
DataSetField.JSON_PROPERTY_ENUM_VALUES
})
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-04-17T12:42:16.563637609Z[Etc/UTC]")
public class DataSetField {
public static final String JSON_PROPERTY_ODDRN = "oddrn";
private String oddrn;
public static final String JSON_PROPERTY_NAME = "name";
private String name;
public static final String JSON_PROPERTY_VERSION = "version";
private String version;
public static final String JSON_PROPERTY_DESCRIPTION = "description";
private String description;
public static final String JSON_PROPERTY_OWNER = "owner";
private String owner;
public static final String JSON_PROPERTY_METADATA = "metadata";
private List metadata;
public static final String JSON_PROPERTY_TAGS = "tags";
private List tags;
public static final String JSON_PROPERTY_PARENT_FIELD_ODDRN = "parent_field_oddrn";
private String parentFieldOddrn;
public static final String JSON_PROPERTY_TYPE = "type";
private DataSetFieldType type;
public static final String JSON_PROPERTY_IS_PRIMARY_KEY = "is_primary_key";
private Boolean isPrimaryKey;
public static final String JSON_PROPERTY_IS_SORT_KEY = "is_sort_key";
private Boolean isSortKey;
public static final String JSON_PROPERTY_IS_KEY = "is_key";
private Boolean isKey;
public static final String JSON_PROPERTY_IS_VALUE = "is_value";
private Boolean isValue;
public static final String JSON_PROPERTY_REFERENCE_ODDRN = "reference_oddrn";
private String referenceOddrn;
public static final String JSON_PROPERTY_DEFAULT_VALUE = "default_value";
private String defaultValue;
public static final String JSON_PROPERTY_STATS = "stats";
private DataSetFieldStat stats;
public static final String JSON_PROPERTY_ENUM_VALUES = "enum_values";
private List enumValues;
public DataSetField() {
}
public DataSetField oddrn(String oddrn) {
this.oddrn = oddrn;
return this;
}
/**
* Get oddrn
* @return oddrn
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_ODDRN)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getOddrn() {
return oddrn;
}
@JsonProperty(JSON_PROPERTY_ODDRN)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setOddrn(String oddrn) {
this.oddrn = oddrn;
}
public DataSetField name(String name) {
this.name = name;
return this;
}
/**
* Get name
* @return name
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_NAME)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public String getName() {
return name;
}
@JsonProperty(JSON_PROPERTY_NAME)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setName(String name) {
this.name = name;
}
public DataSetField version(String version) {
this.version = version;
return this;
}
/**
* Get version
* @return version
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_VERSION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getVersion() {
return version;
}
@JsonProperty(JSON_PROPERTY_VERSION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setVersion(String version) {
this.version = version;
}
public DataSetField description(String description) {
this.description = description;
return this;
}
/**
* Get description
* @return description
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_DESCRIPTION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getDescription() {
return description;
}
@JsonProperty(JSON_PROPERTY_DESCRIPTION)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setDescription(String description) {
this.description = description;
}
public DataSetField owner(String owner) {
this.owner = owner;
return this;
}
/**
* Get owner
* @return owner
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_OWNER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getOwner() {
return owner;
}
@JsonProperty(JSON_PROPERTY_OWNER)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setOwner(String owner) {
this.owner = owner;
}
public DataSetField metadata(List metadata) {
this.metadata = metadata;
return this;
}
public DataSetField addMetadataItem(MetadataExtension metadataItem) {
if (this.metadata == null) {
this.metadata = new ArrayList<>();
}
this.metadata.add(metadataItem);
return this;
}
/**
* Get metadata
* @return metadata
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_METADATA)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getMetadata() {
return metadata;
}
@JsonProperty(JSON_PROPERTY_METADATA)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setMetadata(List metadata) {
this.metadata = metadata;
}
public DataSetField tags(List tags) {
this.tags = tags;
return this;
}
public DataSetField addTagsItem(Tag tagsItem) {
if (this.tags == null) {
this.tags = new ArrayList<>();
}
this.tags.add(tagsItem);
return this;
}
/**
* Get tags
* @return tags
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_TAGS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getTags() {
return tags;
}
@JsonProperty(JSON_PROPERTY_TAGS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setTags(List tags) {
this.tags = tags;
}
public DataSetField parentFieldOddrn(String parentFieldOddrn) {
this.parentFieldOddrn = parentFieldOddrn;
return this;
}
/**
* Get parentFieldOddrn
* @return parentFieldOddrn
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_PARENT_FIELD_ODDRN)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getParentFieldOddrn() {
return parentFieldOddrn;
}
@JsonProperty(JSON_PROPERTY_PARENT_FIELD_ODDRN)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setParentFieldOddrn(String parentFieldOddrn) {
this.parentFieldOddrn = parentFieldOddrn;
}
public DataSetField type(DataSetFieldType type) {
this.type = type;
return this;
}
/**
* Get type
* @return type
**/
@javax.annotation.Nonnull
@JsonProperty(JSON_PROPERTY_TYPE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public DataSetFieldType getType() {
return type;
}
@JsonProperty(JSON_PROPERTY_TYPE)
@JsonInclude(value = JsonInclude.Include.ALWAYS)
public void setType(DataSetFieldType type) {
this.type = type;
}
public DataSetField isPrimaryKey(Boolean isPrimaryKey) {
this.isPrimaryKey = isPrimaryKey;
return this;
}
/**
* Get isPrimaryKey
* @return isPrimaryKey
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_IS_PRIMARY_KEY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Boolean getIsPrimaryKey() {
return isPrimaryKey;
}
@JsonProperty(JSON_PROPERTY_IS_PRIMARY_KEY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setIsPrimaryKey(Boolean isPrimaryKey) {
this.isPrimaryKey = isPrimaryKey;
}
public DataSetField isSortKey(Boolean isSortKey) {
this.isSortKey = isSortKey;
return this;
}
/**
* Get isSortKey
* @return isSortKey
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_IS_SORT_KEY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Boolean getIsSortKey() {
return isSortKey;
}
@JsonProperty(JSON_PROPERTY_IS_SORT_KEY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setIsSortKey(Boolean isSortKey) {
this.isSortKey = isSortKey;
}
public DataSetField isKey(Boolean isKey) {
this.isKey = isKey;
return this;
}
/**
* Get isKey
* @return isKey
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_IS_KEY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Boolean getIsKey() {
return isKey;
}
@JsonProperty(JSON_PROPERTY_IS_KEY)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setIsKey(Boolean isKey) {
this.isKey = isKey;
}
public DataSetField isValue(Boolean isValue) {
this.isValue = isValue;
return this;
}
/**
* Get isValue
* @return isValue
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_IS_VALUE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Boolean getIsValue() {
return isValue;
}
@JsonProperty(JSON_PROPERTY_IS_VALUE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setIsValue(Boolean isValue) {
this.isValue = isValue;
}
public DataSetField referenceOddrn(String referenceOddrn) {
this.referenceOddrn = referenceOddrn;
return this;
}
/**
* Get referenceOddrn
* @return referenceOddrn
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_REFERENCE_ODDRN)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getReferenceOddrn() {
return referenceOddrn;
}
@JsonProperty(JSON_PROPERTY_REFERENCE_ODDRN)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setReferenceOddrn(String referenceOddrn) {
this.referenceOddrn = referenceOddrn;
}
public DataSetField defaultValue(String defaultValue) {
this.defaultValue = defaultValue;
return this;
}
/**
* Get defaultValue
* @return defaultValue
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_DEFAULT_VALUE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public String getDefaultValue() {
return defaultValue;
}
@JsonProperty(JSON_PROPERTY_DEFAULT_VALUE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setDefaultValue(String defaultValue) {
this.defaultValue = defaultValue;
}
public DataSetField stats(DataSetFieldStat stats) {
this.stats = stats;
return this;
}
/**
* Get stats
* @return stats
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_STATS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public DataSetFieldStat getStats() {
return stats;
}
@JsonProperty(JSON_PROPERTY_STATS)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setStats(DataSetFieldStat stats) {
this.stats = stats;
}
public DataSetField enumValues(List enumValues) {
this.enumValues = enumValues;
return this;
}
public DataSetField addEnumValuesItem(DataSetFieldEnumValue enumValuesItem) {
if (this.enumValues == null) {
this.enumValues = new ArrayList<>();
}
this.enumValues.add(enumValuesItem);
return this;
}
/**
* Get enumValues
* @return enumValues
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_ENUM_VALUES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public List getEnumValues() {
return enumValues;
}
@JsonProperty(JSON_PROPERTY_ENUM_VALUES)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setEnumValues(List enumValues) {
this.enumValues = enumValues;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
DataSetField dataSetField = (DataSetField) o;
return Objects.equals(this.oddrn, dataSetField.oddrn) &&
Objects.equals(this.name, dataSetField.name) &&
Objects.equals(this.version, dataSetField.version) &&
Objects.equals(this.description, dataSetField.description) &&
Objects.equals(this.owner, dataSetField.owner) &&
Objects.equals(this.metadata, dataSetField.metadata) &&
Objects.equals(this.tags, dataSetField.tags) &&
Objects.equals(this.parentFieldOddrn, dataSetField.parentFieldOddrn) &&
Objects.equals(this.type, dataSetField.type) &&
Objects.equals(this.isPrimaryKey, dataSetField.isPrimaryKey) &&
Objects.equals(this.isSortKey, dataSetField.isSortKey) &&
Objects.equals(this.isKey, dataSetField.isKey) &&
Objects.equals(this.isValue, dataSetField.isValue) &&
Objects.equals(this.referenceOddrn, dataSetField.referenceOddrn) &&
Objects.equals(this.defaultValue, dataSetField.defaultValue) &&
Objects.equals(this.stats, dataSetField.stats) &&
Objects.equals(this.enumValues, dataSetField.enumValues);
}
@Override
public int hashCode() {
return Objects.hash(oddrn, name, version, description, owner, metadata, tags, parentFieldOddrn, type, isPrimaryKey, isSortKey, isKey, isValue, referenceOddrn, defaultValue, stats, enumValues);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class DataSetField {\n");
sb.append(" oddrn: ").append(toIndentedString(oddrn)).append("\n");
sb.append(" name: ").append(toIndentedString(name)).append("\n");
sb.append(" version: ").append(toIndentedString(version)).append("\n");
sb.append(" description: ").append(toIndentedString(description)).append("\n");
sb.append(" owner: ").append(toIndentedString(owner)).append("\n");
sb.append(" metadata: ").append(toIndentedString(metadata)).append("\n");
sb.append(" tags: ").append(toIndentedString(tags)).append("\n");
sb.append(" parentFieldOddrn: ").append(toIndentedString(parentFieldOddrn)).append("\n");
sb.append(" type: ").append(toIndentedString(type)).append("\n");
sb.append(" isPrimaryKey: ").append(toIndentedString(isPrimaryKey)).append("\n");
sb.append(" isSortKey: ").append(toIndentedString(isSortKey)).append("\n");
sb.append(" isKey: ").append(toIndentedString(isKey)).append("\n");
sb.append(" isValue: ").append(toIndentedString(isValue)).append("\n");
sb.append(" referenceOddrn: ").append(toIndentedString(referenceOddrn)).append("\n");
sb.append(" defaultValue: ").append(toIndentedString(defaultValue)).append("\n");
sb.append(" stats: ").append(toIndentedString(stats)).append("\n");
sb.append(" enumValues: ").append(toIndentedString(enumValues)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy