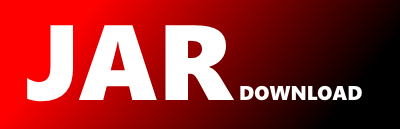
org.opendatadiscovery.client.model.MetricPoint Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of ingestion-contract-client Show documentation
Show all versions of ingestion-contract-client Show documentation
Ingestion Contract WebFlux Client defines OpenDataDiscovery APIs and models for Spring WebClient
/*
* OpenDataDiscovery API Contract
* OpenDataDiscovery API Contract
*
* The version of the OpenAPI document: 0.0.1
*
*
* NOTE: This class is auto generated by OpenAPI Generator (https://openapi-generator.tech).
* https://openapi-generator.tech
* Do not edit the class manually.
*/
package org.opendatadiscovery.client.model;
import java.util.Objects;
import java.util.Arrays;
import com.fasterxml.jackson.annotation.JsonInclude;
import com.fasterxml.jackson.annotation.JsonProperty;
import com.fasterxml.jackson.annotation.JsonCreator;
import com.fasterxml.jackson.annotation.JsonTypeName;
import com.fasterxml.jackson.annotation.JsonValue;
import org.opendatadiscovery.client.model.CounterValue;
import org.opendatadiscovery.client.model.GaugeValue;
import org.opendatadiscovery.client.model.HistogramValue;
import org.opendatadiscovery.client.model.InfoValue;
import org.opendatadiscovery.client.model.StateSetValue;
import org.opendatadiscovery.client.model.SummaryValue;
import org.opendatadiscovery.client.model.UnknownValue;
import com.fasterxml.jackson.annotation.JsonPropertyOrder;
import com.fasterxml.jackson.annotation.JsonTypeName;
/**
* MetricPoint
*/
@JsonPropertyOrder({
MetricPoint.JSON_PROPERTY_TIMESTAMP,
MetricPoint.JSON_PROPERTY_UNKNOWN_VALUE,
MetricPoint.JSON_PROPERTY_GAUGE_VALUE,
MetricPoint.JSON_PROPERTY_COUNTER_VALUE,
MetricPoint.JSON_PROPERTY_HISTOGRAM_VALUE,
MetricPoint.JSON_PROPERTY_STATE_SET_VALUE,
MetricPoint.JSON_PROPERTY_INFO_VALUE,
MetricPoint.JSON_PROPERTY_SUMMARY_VALUE
})
@javax.annotation.Generated(value = "org.openapitools.codegen.languages.JavaClientCodegen", date = "2024-04-17T12:42:16.563637609Z[Etc/UTC]")
public class MetricPoint {
public static final String JSON_PROPERTY_TIMESTAMP = "timestamp";
private Integer timestamp;
public static final String JSON_PROPERTY_UNKNOWN_VALUE = "unknown_value";
private UnknownValue unknownValue;
public static final String JSON_PROPERTY_GAUGE_VALUE = "gauge_value";
private GaugeValue gaugeValue;
public static final String JSON_PROPERTY_COUNTER_VALUE = "counter_value";
private CounterValue counterValue;
public static final String JSON_PROPERTY_HISTOGRAM_VALUE = "histogram_value";
private HistogramValue histogramValue;
public static final String JSON_PROPERTY_STATE_SET_VALUE = "state_set_value";
private StateSetValue stateSetValue;
public static final String JSON_PROPERTY_INFO_VALUE = "info_value";
private InfoValue infoValue;
public static final String JSON_PROPERTY_SUMMARY_VALUE = "summary_value";
private SummaryValue summaryValue;
public MetricPoint() {
}
public MetricPoint timestamp(Integer timestamp) {
this.timestamp = timestamp;
return this;
}
/**
* Get timestamp
* @return timestamp
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_TIMESTAMP)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public Integer getTimestamp() {
return timestamp;
}
@JsonProperty(JSON_PROPERTY_TIMESTAMP)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setTimestamp(Integer timestamp) {
this.timestamp = timestamp;
}
public MetricPoint unknownValue(UnknownValue unknownValue) {
this.unknownValue = unknownValue;
return this;
}
/**
* Get unknownValue
* @return unknownValue
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_UNKNOWN_VALUE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public UnknownValue getUnknownValue() {
return unknownValue;
}
@JsonProperty(JSON_PROPERTY_UNKNOWN_VALUE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setUnknownValue(UnknownValue unknownValue) {
this.unknownValue = unknownValue;
}
public MetricPoint gaugeValue(GaugeValue gaugeValue) {
this.gaugeValue = gaugeValue;
return this;
}
/**
* Get gaugeValue
* @return gaugeValue
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_GAUGE_VALUE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public GaugeValue getGaugeValue() {
return gaugeValue;
}
@JsonProperty(JSON_PROPERTY_GAUGE_VALUE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setGaugeValue(GaugeValue gaugeValue) {
this.gaugeValue = gaugeValue;
}
public MetricPoint counterValue(CounterValue counterValue) {
this.counterValue = counterValue;
return this;
}
/**
* Get counterValue
* @return counterValue
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_COUNTER_VALUE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public CounterValue getCounterValue() {
return counterValue;
}
@JsonProperty(JSON_PROPERTY_COUNTER_VALUE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setCounterValue(CounterValue counterValue) {
this.counterValue = counterValue;
}
public MetricPoint histogramValue(HistogramValue histogramValue) {
this.histogramValue = histogramValue;
return this;
}
/**
* Get histogramValue
* @return histogramValue
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_HISTOGRAM_VALUE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public HistogramValue getHistogramValue() {
return histogramValue;
}
@JsonProperty(JSON_PROPERTY_HISTOGRAM_VALUE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setHistogramValue(HistogramValue histogramValue) {
this.histogramValue = histogramValue;
}
public MetricPoint stateSetValue(StateSetValue stateSetValue) {
this.stateSetValue = stateSetValue;
return this;
}
/**
* Get stateSetValue
* @return stateSetValue
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_STATE_SET_VALUE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public StateSetValue getStateSetValue() {
return stateSetValue;
}
@JsonProperty(JSON_PROPERTY_STATE_SET_VALUE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setStateSetValue(StateSetValue stateSetValue) {
this.stateSetValue = stateSetValue;
}
public MetricPoint infoValue(InfoValue infoValue) {
this.infoValue = infoValue;
return this;
}
/**
* Get infoValue
* @return infoValue
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_INFO_VALUE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public InfoValue getInfoValue() {
return infoValue;
}
@JsonProperty(JSON_PROPERTY_INFO_VALUE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setInfoValue(InfoValue infoValue) {
this.infoValue = infoValue;
}
public MetricPoint summaryValue(SummaryValue summaryValue) {
this.summaryValue = summaryValue;
return this;
}
/**
* Get summaryValue
* @return summaryValue
**/
@javax.annotation.Nullable
@JsonProperty(JSON_PROPERTY_SUMMARY_VALUE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public SummaryValue getSummaryValue() {
return summaryValue;
}
@JsonProperty(JSON_PROPERTY_SUMMARY_VALUE)
@JsonInclude(value = JsonInclude.Include.USE_DEFAULTS)
public void setSummaryValue(SummaryValue summaryValue) {
this.summaryValue = summaryValue;
}
@Override
public boolean equals(Object o) {
if (this == o) {
return true;
}
if (o == null || getClass() != o.getClass()) {
return false;
}
MetricPoint metricPoint = (MetricPoint) o;
return Objects.equals(this.timestamp, metricPoint.timestamp) &&
Objects.equals(this.unknownValue, metricPoint.unknownValue) &&
Objects.equals(this.gaugeValue, metricPoint.gaugeValue) &&
Objects.equals(this.counterValue, metricPoint.counterValue) &&
Objects.equals(this.histogramValue, metricPoint.histogramValue) &&
Objects.equals(this.stateSetValue, metricPoint.stateSetValue) &&
Objects.equals(this.infoValue, metricPoint.infoValue) &&
Objects.equals(this.summaryValue, metricPoint.summaryValue);
}
@Override
public int hashCode() {
return Objects.hash(timestamp, unknownValue, gaugeValue, counterValue, histogramValue, stateSetValue, infoValue, summaryValue);
}
@Override
public String toString() {
StringBuilder sb = new StringBuilder();
sb.append("class MetricPoint {\n");
sb.append(" timestamp: ").append(toIndentedString(timestamp)).append("\n");
sb.append(" unknownValue: ").append(toIndentedString(unknownValue)).append("\n");
sb.append(" gaugeValue: ").append(toIndentedString(gaugeValue)).append("\n");
sb.append(" counterValue: ").append(toIndentedString(counterValue)).append("\n");
sb.append(" histogramValue: ").append(toIndentedString(histogramValue)).append("\n");
sb.append(" stateSetValue: ").append(toIndentedString(stateSetValue)).append("\n");
sb.append(" infoValue: ").append(toIndentedString(infoValue)).append("\n");
sb.append(" summaryValue: ").append(toIndentedString(summaryValue)).append("\n");
sb.append("}");
return sb.toString();
}
/**
* Convert the given object to string with each line indented by 4 spaces
* (except the first line).
*/
private String toIndentedString(Object o) {
if (o == null) {
return "null";
}
return o.toString().replace("\n", "\n ");
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy