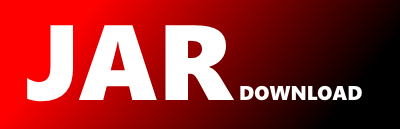
org.opendaylight.aaa.web.WebContextBuilder Maven / Gradle / Ivy
package org.opendaylight.aaa.web;
import com.google.common.base.MoreObjects;
import com.google.common.collect.ImmutableList;
import com.google.common.collect.ImmutableMap;
import com.google.common.primitives.Booleans;
import com.google.errorprone.annotations.CanIgnoreReturnValue;
import com.google.errorprone.annotations.Var;
import edu.umd.cs.findbugs.annotations.SuppressFBWarnings;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import java.util.Objects;
import javax.servlet.ServletContextListener;
import org.immutables.value.Generated;
/**
* Builds instances of type {@link WebContext WebContext}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code WebContextBuilder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@Generated(from = "WebContext", generator = "Immutables")
@SuppressWarnings({"all"})
@SuppressFBWarnings
@javax.annotation.processing.Generated("org.immutables.processor.ProxyProcessor")
public final class WebContextBuilder {
private static final long INIT_BIT_CONTEXT_PATH = 0x1L;
private static final long OPT_BIT_SUPPORTS_SESSIONS = 0x1L;
private long initBits = 0x1L;
private long optBits;
private String contextPath;
private boolean supportsSessions;
private ImmutableList.Builder servlets = ImmutableList.builder();
private ImmutableList.Builder filters = ImmutableList.builder();
private ImmutableList.Builder listeners = ImmutableList.builder();
private ImmutableList.Builder resources = ImmutableList.builder();
private ImmutableMap.Builder contextParams = ImmutableMap.builder();
/**
* Creates a builder for {@link WebContext WebContext} instances.
*
* new WebContextBuilder()
* .contextPath(String) // required {@link WebContext#contextPath() contextPath}
* .supportsSessions(boolean) // optional {@link WebContext#supportsSessions() supportsSessions}
* .addServlet|addAllServlets(org.opendaylight.aaa.web.ServletDetails) // {@link WebContext#servlets() servlets} elements
* .addFilter|addAllFilters(org.opendaylight.aaa.web.FilterDetails) // {@link WebContext#filters() filters} elements
* .addListener|addAllListeners(javax.servlet.ServletContextListener) // {@link WebContext#listeners() listeners} elements
* .addResource|addAllResources(org.opendaylight.aaa.web.ResourceDetails) // {@link WebContext#resources() resources} elements
* .putContextParam|putAllContextParams(String => String) // {@link WebContext#contextParams() contextParams} mappings
* .build();
*
*/
public WebContextBuilder() {
}
/**
* Fill a builder with attribute values from the provided {@code WebContext} instance.
* Regular attribute values will be replaced with those from the given instance.
* Absent optional values will not replace present values.
* Collection elements and entries will be added, not replaced.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final WebContextBuilder from(WebContext instance) {
Objects.requireNonNull(instance, "instance");
contextPath(instance.contextPath());
supportsSessions(instance.supportsSessions());
addAllServlets(instance.servlets());
addAllFilters(instance.filters());
addAllListeners(instance.listeners());
addAllResources(instance.resources());
putAllContextParams(instance.contextParams());
return this;
}
/**
* Initializes the value for the {@link WebContext#contextPath() contextPath} attribute.
* @param contextPath The value for contextPath
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final WebContextBuilder contextPath(String contextPath) {
this.contextPath = Objects.requireNonNull(contextPath, "contextPath");
initBits &= ~INIT_BIT_CONTEXT_PATH;
return this;
}
/**
* Initializes the value for the {@link WebContext#supportsSessions() supportsSessions} attribute.
* If not set, this attribute will have a default value as returned by the initializer of {@link WebContext#supportsSessions() supportsSessions}.
* @param supportsSessions The value for supportsSessions
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final WebContextBuilder supportsSessions(boolean supportsSessions) {
this.supportsSessions = supportsSessions;
optBits |= OPT_BIT_SUPPORTS_SESSIONS;
return this;
}
/**
* Adds one element to {@link WebContext#servlets() servlets} list.
* @param element A servlets element
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final WebContextBuilder addServlet(ServletDetails element) {
this.servlets.add(element);
return this;
}
/**
* Adds elements to {@link WebContext#servlets() servlets} list.
* @param elements An array of servlets elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final WebContextBuilder addServlets(ServletDetails... elements) {
this.servlets.add(elements);
return this;
}
/**
* Sets or replaces all elements for {@link WebContext#servlets() servlets} list.
* @param elements An iterable of servlets elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final WebContextBuilder servlets(Iterable extends ServletDetails> elements) {
this.servlets = ImmutableList.builder();
return addAllServlets(elements);
}
/**
* Adds elements to {@link WebContext#servlets() servlets} list.
* @param elements An iterable of servlets elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final WebContextBuilder addAllServlets(Iterable extends ServletDetails> elements) {
this.servlets.addAll(elements);
return this;
}
/**
* Adds one element to {@link WebContext#filters() filters} list.
* @param element A filters element
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final WebContextBuilder addFilter(FilterDetails element) {
this.filters.add(element);
return this;
}
/**
* Adds elements to {@link WebContext#filters() filters} list.
* @param elements An array of filters elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final WebContextBuilder addFilters(FilterDetails... elements) {
this.filters.add(elements);
return this;
}
/**
* Sets or replaces all elements for {@link WebContext#filters() filters} list.
* @param elements An iterable of filters elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final WebContextBuilder filters(Iterable extends FilterDetails> elements) {
this.filters = ImmutableList.builder();
return addAllFilters(elements);
}
/**
* Adds elements to {@link WebContext#filters() filters} list.
* @param elements An iterable of filters elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final WebContextBuilder addAllFilters(Iterable extends FilterDetails> elements) {
this.filters.addAll(elements);
return this;
}
/**
* Adds one element to {@link WebContext#listeners() listeners} list.
* @param element A listeners element
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final WebContextBuilder addListener(ServletContextListener element) {
this.listeners.add(element);
return this;
}
/**
* Adds elements to {@link WebContext#listeners() listeners} list.
* @param elements An array of listeners elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final WebContextBuilder addListeners(ServletContextListener... elements) {
this.listeners.add(elements);
return this;
}
/**
* Sets or replaces all elements for {@link WebContext#listeners() listeners} list.
* @param elements An iterable of listeners elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final WebContextBuilder listeners(Iterable extends ServletContextListener> elements) {
this.listeners = ImmutableList.builder();
return addAllListeners(elements);
}
/**
* Adds elements to {@link WebContext#listeners() listeners} list.
* @param elements An iterable of listeners elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final WebContextBuilder addAllListeners(Iterable extends ServletContextListener> elements) {
this.listeners.addAll(elements);
return this;
}
/**
* Adds one element to {@link WebContext#resources() resources} list.
* @param element A resources element
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final WebContextBuilder addResource(ResourceDetails element) {
this.resources.add(element);
return this;
}
/**
* Adds elements to {@link WebContext#resources() resources} list.
* @param elements An array of resources elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final WebContextBuilder addResources(ResourceDetails... elements) {
this.resources.add(elements);
return this;
}
/**
* Sets or replaces all elements for {@link WebContext#resources() resources} list.
* @param elements An iterable of resources elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final WebContextBuilder resources(Iterable extends ResourceDetails> elements) {
this.resources = ImmutableList.builder();
return addAllResources(elements);
}
/**
* Adds elements to {@link WebContext#resources() resources} list.
* @param elements An iterable of resources elements
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final WebContextBuilder addAllResources(Iterable extends ResourceDetails> elements) {
this.resources.addAll(elements);
return this;
}
/**
* Put one entry to the {@link WebContext#contextParams() contextParams} map.
* @param key The key in the contextParams map
* @param value The associated value in the contextParams map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final WebContextBuilder putContextParam(String key, String value) {
this.contextParams.put(key, value);
return this;
}
/**
* Put one entry to the {@link WebContext#contextParams() contextParams} map. Nulls are not permitted
* @param entry The key and value entry
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final WebContextBuilder putContextParam(Map.Entry entry) {
this.contextParams.put(entry);
return this;
}
/**
* Sets or replaces all mappings from the specified map as entries for the {@link WebContext#contextParams() contextParams} map. Nulls are not permitted
* @param entries The entries that will be added to the contextParams map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final WebContextBuilder contextParams(Map entries) {
this.contextParams = ImmutableMap.builder();
return putAllContextParams(entries);
}
/**
* Put all mappings from the specified map as entries to {@link WebContext#contextParams() contextParams} map. Nulls are not permitted
* @param entries The entries that will be added to the contextParams map
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final WebContextBuilder putAllContextParams(Map entries) {
this.contextParams.putAll(entries);
return this;
}
/**
* Builds a new {@link WebContext WebContext}.
* @return An immutable instance of WebContext
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public WebContext build() {
if (initBits != 0) {
throw new IllegalStateException(formatRequiredAttributesMessage());
}
return WebContextBuilder.ImmutableWebContext.validate(new WebContextBuilder.ImmutableWebContext(this));
}
private boolean supportsSessionsIsSet() {
return (optBits & OPT_BIT_SUPPORTS_SESSIONS) != 0;
}
private String formatRequiredAttributesMessage() {
List attributes = new ArrayList<>();
if ((initBits & INIT_BIT_CONTEXT_PATH) != 0) attributes.add("contextPath");
return "Cannot build WebContext, some of required attributes are not set " + attributes;
}
/**
* Immutable implementation of {@link WebContext}.
*
* Use the builder to create immutable instances:
* {@code new WebContextBuilder()}.
*/
@Generated(from = "WebContext", generator = "Immutables")
private static final class ImmutableWebContext extends WebContext {
private final String contextPath;
private final boolean supportsSessions;
private final ImmutableList servlets;
private final ImmutableList filters;
private final ImmutableList listeners;
private final ImmutableList resources;
private final ImmutableMap contextParams;
private ImmutableWebContext(WebContextBuilder builder) {
this.contextPath = builder.contextPath;
this.servlets = builder.servlets.build();
this.filters = builder.filters.build();
this.listeners = builder.listeners.build();
this.resources = builder.resources.build();
this.contextParams = builder.contextParams.build();
this.supportsSessions = builder.supportsSessionsIsSet()
? builder.supportsSessions
: super.supportsSessions();
}
/**
* @return The value of the {@code contextPath} attribute
*/
@Override
public String contextPath() {
return contextPath;
}
/**
* @return The value of the {@code supportsSessions} attribute
*/
@Override
public boolean supportsSessions() {
return supportsSessions;
}
/**
* @return The value of the {@code servlets} attribute
*/
@Override
public ImmutableList servlets() {
return servlets;
}
/**
* @return The value of the {@code filters} attribute
*/
@Override
public ImmutableList filters() {
return filters;
}
/**
* @return The value of the {@code listeners} attribute
*/
@Override
public ImmutableList listeners() {
return listeners;
}
/**
* @return The value of the {@code resources} attribute
*/
@Override
public ImmutableList resources() {
return resources;
}
/**
* @return The value of the {@code contextParams} attribute
*/
@Override
public ImmutableMap contextParams() {
return contextParams;
}
/**
* This instance is equal to all instances of {@code ImmutableWebContext} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(Object another) {
if (this == another) return true;
return another instanceof WebContextBuilder.ImmutableWebContext
&& equalTo(0, (WebContextBuilder.ImmutableWebContext) another);
}
private boolean equalTo(int synthetic, WebContextBuilder.ImmutableWebContext another) {
return contextPath.equals(another.contextPath)
&& supportsSessions == another.supportsSessions
&& servlets.equals(another.servlets)
&& filters.equals(another.filters)
&& listeners.equals(another.listeners)
&& resources.equals(another.resources)
&& contextParams.equals(another.contextParams);
}
/**
* Computes a hash code from attributes: {@code contextPath}, {@code supportsSessions}, {@code servlets}, {@code filters}, {@code listeners}, {@code resources}, {@code contextParams}.
* @return hashCode value
*/
@Override
public int hashCode() {
@Var int h = 5381;
h += (h << 5) + contextPath.hashCode();
h += (h << 5) + Booleans.hashCode(supportsSessions);
h += (h << 5) + servlets.hashCode();
h += (h << 5) + filters.hashCode();
h += (h << 5) + listeners.hashCode();
h += (h << 5) + resources.hashCode();
h += (h << 5) + contextParams.hashCode();
return h;
}
/**
* Prints the immutable value {@code WebContext} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return MoreObjects.toStringHelper("WebContext")
.omitNullValues()
.add("contextPath", contextPath)
.add("supportsSessions", supportsSessions)
.add("servlets", servlets)
.add("filters", filters)
.add("listeners", listeners)
.add("resources", resources)
.add("contextParams", contextParams)
.toString();
}
private static WebContextBuilder.ImmutableWebContext validate(WebContextBuilder.ImmutableWebContext instance) {
instance.check();
return instance;
}
}
}