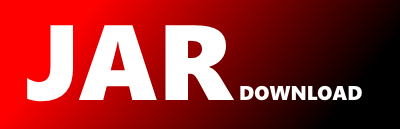
org.opendaylight.infrautils.caches.CachePolicyBuilder Maven / Gradle / Ivy
package org.opendaylight.infrautils.caches;
import com.google.common.base.MoreObjects;
import com.google.common.primitives.Booleans;
import com.google.common.primitives.Longs;
import com.google.errorprone.annotations.CanIgnoreReturnValue;
import com.google.errorprone.annotations.Var;
import edu.umd.cs.findbugs.annotations.SuppressFBWarnings;
import java.util.ArrayList;
import java.util.List;
import java.util.Objects;
import org.immutables.value.Generated;
/**
* Builds instances of type {@link CachePolicy CachePolicy}.
* Initialize attributes and then invoke the {@link #build()} method to create an
* immutable instance.
* {@code CachePolicyBuilder} is not thread-safe and generally should not be stored in a field or collection,
* but instead used immediately to create instances.
*/
@Generated(from = "CachePolicy", generator = "Immutables")
@SuppressWarnings({"all"})
@SuppressFBWarnings
@javax.annotation.processing.Generated("org.immutables.processor.ProxyProcessor")
public final class CachePolicyBuilder {
private static final long OPT_BIT_STATS_ENABLED = 0x1L;
private static final long OPT_BIT_MAX_ENTRIES = 0x2L;
private long optBits;
private boolean statsEnabled;
private long maxEntries;
/**
* Creates a builder for {@link CachePolicy CachePolicy} instances.
*
* new CachePolicyBuilder()
* .statsEnabled(boolean) // optional {@link CachePolicy#statsEnabled() statsEnabled}
* .maxEntries(long) // optional {@link CachePolicy#maxEntries() maxEntries}
* .build();
*
*/
public CachePolicyBuilder() {
}
/**
* Fill a builder with attribute values from the provided {@code CachePolicy} instance.
* Regular attribute values will be replaced with those from the given instance.
* Absent optional values will not replace present values.
* @param instance The instance from which to copy values
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final CachePolicyBuilder from(CachePolicy instance) {
Objects.requireNonNull(instance, "instance");
statsEnabled(instance.statsEnabled());
maxEntries(instance.maxEntries());
return this;
}
/**
* Initializes the value for the {@link CachePolicy#statsEnabled() statsEnabled} attribute.
* If not set, this attribute will have a default value as returned by the initializer of {@link CachePolicy#statsEnabled() statsEnabled}.
* @param statsEnabled The value for statsEnabled
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final CachePolicyBuilder statsEnabled(boolean statsEnabled) {
this.statsEnabled = statsEnabled;
optBits |= OPT_BIT_STATS_ENABLED;
return this;
}
/**
* Initializes the value for the {@link CachePolicy#maxEntries() maxEntries} attribute.
*
If not set, this attribute will have a default value as returned by the initializer of {@link CachePolicy#maxEntries() maxEntries}.
* @param maxEntries The value for maxEntries
* @return {@code this} builder for use in a chained invocation
*/
@CanIgnoreReturnValue
public final CachePolicyBuilder maxEntries(long maxEntries) {
this.maxEntries = maxEntries;
optBits |= OPT_BIT_MAX_ENTRIES;
return this;
}
/**
* Builds a new {@link CachePolicy CachePolicy}.
* @return An immutable instance of CachePolicy
* @throws java.lang.IllegalStateException if any required attributes are missing
*/
public CachePolicy build() {
return new CachePolicyBuilder.ImmutableCachePolicy(this);
}
private boolean statsEnabledIsSet() {
return (optBits & OPT_BIT_STATS_ENABLED) != 0;
}
private boolean maxEntriesIsSet() {
return (optBits & OPT_BIT_MAX_ENTRIES) != 0;
}
/**
* Immutable implementation of {@link CachePolicy}.
*
* Use the builder to create immutable instances:
* {@code new CachePolicyBuilder()}.
*/
@Generated(from = "CachePolicy", generator = "Immutables")
private static final class ImmutableCachePolicy implements CachePolicy {
private final boolean statsEnabled;
private final long maxEntries;
private ImmutableCachePolicy(CachePolicyBuilder builder) {
if (builder.statsEnabledIsSet()) {
initShim.statsEnabled(builder.statsEnabled);
}
if (builder.maxEntriesIsSet()) {
initShim.maxEntries(builder.maxEntries);
}
this.statsEnabled = initShim.statsEnabled();
this.maxEntries = initShim.maxEntries();
this.initShim = null;
}
private static final byte STAGE_INITIALIZING = -1;
private static final byte STAGE_UNINITIALIZED = 0;
private static final byte STAGE_INITIALIZED = 1;
@SuppressWarnings("Immutable")
private transient volatile InitShim initShim = new InitShim();
@Generated(from = "CachePolicy", generator = "Immutables")
private final class InitShim {
private byte statsEnabledBuildStage = STAGE_UNINITIALIZED;
private boolean statsEnabled;
boolean statsEnabled() {
if (statsEnabledBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (statsEnabledBuildStage == STAGE_UNINITIALIZED) {
statsEnabledBuildStage = STAGE_INITIALIZING;
this.statsEnabled = statsEnabledInitialize();
statsEnabledBuildStage = STAGE_INITIALIZED;
}
return this.statsEnabled;
}
void statsEnabled(boolean statsEnabled) {
this.statsEnabled = statsEnabled;
statsEnabledBuildStage = STAGE_INITIALIZED;
}
private byte maxEntriesBuildStage = STAGE_UNINITIALIZED;
private long maxEntries;
long maxEntries() {
if (maxEntriesBuildStage == STAGE_INITIALIZING) throw new IllegalStateException(formatInitCycleMessage());
if (maxEntriesBuildStage == STAGE_UNINITIALIZED) {
maxEntriesBuildStage = STAGE_INITIALIZING;
this.maxEntries = maxEntriesInitialize();
maxEntriesBuildStage = STAGE_INITIALIZED;
}
return this.maxEntries;
}
void maxEntries(long maxEntries) {
this.maxEntries = maxEntries;
maxEntriesBuildStage = STAGE_INITIALIZED;
}
private String formatInitCycleMessage() {
List attributes = new ArrayList<>();
if (statsEnabledBuildStage == STAGE_INITIALIZING) attributes.add("statsEnabled");
if (maxEntriesBuildStage == STAGE_INITIALIZING) attributes.add("maxEntries");
return "Cannot build CachePolicy, attribute initializers form cycle " + attributes;
}
}
private boolean statsEnabledInitialize() {
return CachePolicy.super.statsEnabled();
}
private long maxEntriesInitialize() {
return CachePolicy.super.maxEntries();
}
/**
* @return The value of the {@code statsEnabled} attribute
*/
@Override
public boolean statsEnabled() {
InitShim shim = this.initShim;
return shim != null
? shim.statsEnabled()
: this.statsEnabled;
}
/**
* @return The value of the {@code maxEntries} attribute
*/
@Override
public long maxEntries() {
InitShim shim = this.initShim;
return shim != null
? shim.maxEntries()
: this.maxEntries;
}
/**
* This instance is equal to all instances of {@code ImmutableCachePolicy} that have equal attribute values.
* @return {@code true} if {@code this} is equal to {@code another} instance
*/
@Override
public boolean equals(Object another) {
if (this == another) return true;
return another instanceof CachePolicyBuilder.ImmutableCachePolicy
&& equalTo((CachePolicyBuilder.ImmutableCachePolicy) another);
}
private boolean equalTo(CachePolicyBuilder.ImmutableCachePolicy another) {
return statsEnabled == another.statsEnabled
&& maxEntries == another.maxEntries;
}
/**
* Computes a hash code from attributes: {@code statsEnabled}, {@code maxEntries}.
* @return hashCode value
*/
@Override
public int hashCode() {
@Var int h = 5381;
h += (h << 5) + Booleans.hashCode(statsEnabled);
h += (h << 5) + Longs.hashCode(maxEntries);
return h;
}
/**
* Prints the immutable value {@code CachePolicy} with attribute values.
* @return A string representation of the value
*/
@Override
public String toString() {
return MoreObjects.toStringHelper("CachePolicy")
.omitNullValues()
.add("statsEnabled", statsEnabled)
.add("maxEntries", maxEntries)
.toString();
}
}
}