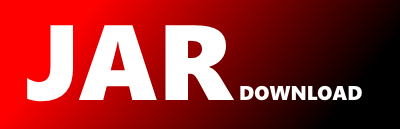
org.opendaylight.jsonrpc.bus.messagelib.package-info Maven / Gradle / Ivy
/*
* Copyright (c) 2018 Lumina Networks, Inc. All rights reserved.
*
* This program and the accompanying materials are made available under the
* terms of the Eclipse Public License v1.0 which accompanies this distribution,
* and is available at http://www.eclipse.org/legal/epl-v10.html
*/
/**
* This package contains public API that can be used to create instances of
* various session types and proxy objects.
*
* Requester proxy - send request to remote responder instance
* and read response back. To create requester proxy, follow these steps:
*
* - create responder (on remote peer) by providing implementation of known
* API
* - connect requester proxy to it (on local peer) using known API and
* URL
* - send request and read response
*
*
*
* interface SomeAPI {
* int method1(int num, String str);
* }
*
* // Instance of this class is exposed on remote peer
* class SomeService implements SomeAPI {
* @Override
* int method1(int num, String str) {
* return 10;
* }
* }
*
*
* step 1 (remote peer)
*
*
* TransportFactory tf = ...
*
* SomeService svc = tf.createResponder("zmq://192.168.1.10:20000", new SomeService());
*
*
*
* step 2 (local peer)
*
*
*
* TransportFactory tf = ...
* SomeService svc = tf.createRequesterProxy(SomeAPI.class, "zmq://192.168.1.10:20000");
*
*
*
* step 3 (local peer)
*
*
* int x = svc.method1(1, "ABC"); // return value assigned to x is 10 (see
* // implementation above)
*
*
* This will result in following communication on bus:
*
*
* > { "id" : 1, "jsonrpc" : "2.0", "method" : "method1", params: [1, "ABC"] }
* < { "id" : 1, "jsonrpc" : "2.0", "result" : 10 }
*
*
* Publisher proxy - send notification to all channels
* subscribed on remote publisher instance. To create publisher proxy instance,
* follow these steps:
*
* - create publisher proxy (provide interface and URL - local peer)
* - connect (multiple) subscribers to it (remote peers)
* - invoke notification method on publisher proxy (provided interface)
*
*
* Example of API:
*
*
* interface SomeAPI {
* void notification_method1(String info); // return type must be void
* }
*
* class SomeSubscriber implements SomeAPI {
* @Override
* void notification_method1(String info) {
* // this method will be called when notification comes in
* }
* }
*
*
* step 1 - local peer
*
*
* TransportFactory tf = ...
*
* SomeAPI proxy = tf.createPublisherProxy(SomeAPI.class, "ws://192.168.1.12:12345");
*
*
* step 2 - (multiple) remote peers
*
*
* TransportFactory tf = ...
* SomeSubscriber session = tf.createSubscriber("ws://192.168.1.12:12345", new SomeSubscriber());
*
*
* step 3 - local peer
*
*
* proxy.notification_method1("ABC")
*
*
* This will send notification to all connected subscribers:
*
* { "jsonrpc" : "2.0", "method" : "notification_method1", params: ["ABC"] }
*
*/
package org.opendaylight.jsonrpc.bus.messagelib;
© 2015 - 2025 Weber Informatics LLC | Privacy Policy