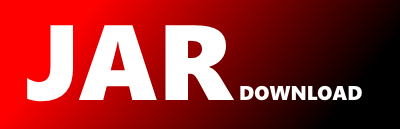
org.opendaylight.mdsal.binding.api.query.MatchBuilderPath Maven / Gradle / Ivy
/*
* Copyright (c) 2020 PANTHEON.tech, s.r.o. and others. All rights reserved.
*
* This program and the accompanying materials are made available under the
* terms of the Eclipse Public License v1.0 which accompanies this distribution,
* and is available at http://www.eclipse.org/legal/epl-v10.html
*/
package org.opendaylight.mdsal.binding.api.query;
import com.google.common.annotations.Beta;
import java.io.Serializable;
import org.eclipse.jdt.annotation.NonNull;
import org.opendaylight.yangtools.binding.BaseIdentity;
import org.opendaylight.yangtools.binding.ChildOf;
import org.opendaylight.yangtools.binding.ChoiceIn;
import org.opendaylight.yangtools.binding.DataObject;
import org.opendaylight.yangtools.binding.EntryObject;
import org.opendaylight.yangtools.binding.Key;
import org.opendaylight.yangtools.binding.TypeObject;
import org.opendaylight.yangtools.concepts.Mutable;
import org.opendaylight.yangtools.yang.common.Decimal64;
import org.opendaylight.yangtools.yang.common.Empty;
import org.opendaylight.yangtools.yang.common.Uint16;
import org.opendaylight.yangtools.yang.common.Uint32;
import org.opendaylight.yangtools.yang.common.Uint64;
import org.opendaylight.yangtools.yang.common.Uint8;
@Beta
public interface MatchBuilderPath extends Mutable {
/**
* Descend match into a child container.
*
* @param Child container type
* @param childClass Child container type class
* @return This builder
* @throws NullPointerException if childClass is null
*/
> @NonNull MatchBuilderPath childObject(Class childClass);
/**
* Descend match into a child container in a particular case.
*
* @param Case type
* @param Child container type
* @param childClass Child container type class
* @return This builder
* @throws NullPointerException if any argument is null
*/
& DataObject, N extends ChildOf super C>>
@NonNull MatchBuilderPath extractChild(Class caseClass, Class childClass);
/**
* Add a child path component to the specification of what needs to be extracted, specifying an exact match in
* a keyed list. This method, along with its alternatives, can be used to specify which object type to select from
* the root path.
*
* @param List type
* @param Key type
* @param listKey List key
* @return This builder
* @throws NullPointerException if childClass is null
*/
& ChildOf super T>, K extends Key> @NonNull MatchBuilderPath extractChild(
Class<@NonNull N> listItem, K listKey);
/**
* Match an {@code boolean} leaf's value.
*
* @param methodRef method reference to the getter method
* @return A {@link ValueMatchBuilder}
* @throws NullPointerException if methodRef is null
*/
@NonNull ValueMatchBuilder leaf(BooleanLeafReference methodRef);
/**
* Match an {@code decimal64} leaf's value.
*
* @param methodRef method reference to the getter method
* @return A {@link ValueMatchBuilder}
* @throws NullPointerException if methodRef is null
*/
@NonNull ComparableMatchBuilder leaf(Decimal64LeafReference methodRef);
/**
* Match an {@code empty} leaf's value.
*
* @param methodRef method reference to the getter method
* @return A {@link ValueMatchBuilder}
* @throws NullPointerException if methodRef is null
*/
@NonNull ValueMatchBuilder leaf(EmptyLeafReference methodRef);
/**
* Match an {@code string} leaf's value.
*
* @param methodRef method reference to the getter method
* @return A {@link StringMatchBuilder}
* @throws NullPointerException if methodRef is null
*/
@NonNull StringMatchBuilder leaf(StringLeafReference methodRef);
/**
* Match an {@code int8} leaf's value.
*
* @param methodRef method reference to the getter method
* @return A {@link ComparableMatchBuilder}
* @throws NullPointerException if methodRef is null
*/
@NonNull ComparableMatchBuilder leaf(Int8LeafReference methodRef);
/**
* Match an {@code int16} leaf's value.
*
* @param methodRef method reference to the getter method
* @return A {@link ComparableMatchBuilder}
* @throws NullPointerException if methodRef is null
*/
@NonNull ComparableMatchBuilder leaf(Int16LeafReference methodRef);
/**
* Match an {@code int32} leaf's value.
*
* @param methodRef method reference to the getter method
* @return A {@link ComparableMatchBuilder}
* @throws NullPointerException if methodRef is null
*/
@NonNull ComparableMatchBuilder leaf(Int32LeafReference methodRef);
/**
* Match an {@code int64} leaf's value.
*
* @param methodRef method reference to the getter method
* @return A {@link ComparableMatchBuilder}
* @throws NullPointerException if methodRef is null
*/
@NonNull ComparableMatchBuilder leaf(Int64LeafReference methodRef);
/**
* Match an {@code uint8} leaf's value.
*
* @param methodRef method reference to the getter method
* @return A {@link ComparableMatchBuilder}
* @throws NullPointerException if methodRef is null
*/
@NonNull ComparableMatchBuilder leaf(Uint8LeafReference methodRef);
/**
* Match an {@code uint16} leaf's value.
*
* @param methodRef method reference to the getter method
* @return A {@link ComparableMatchBuilder}
* @throws NullPointerException if methodRef is null
*/
@NonNull ComparableMatchBuilder leaf(Uint16LeafReference methodRef);
/**
* Match an {@code uint32} leaf's value.
*
* @param methodRef method reference to the getter method
* @return A {@link ComparableMatchBuilder}
* @throws NullPointerException if methodRef is null
*/
@NonNull ComparableMatchBuilder leaf(Uint32LeafReference methodRef);
/**
* Match an {@code uint64} leaf's value.
*
* @param methodRef method reference to the getter method
* @return A {@link ComparableMatchBuilder}
* @throws NullPointerException if methodRef is null
*/
@NonNull ComparableMatchBuilder leaf(Uint64LeafReference methodRef);
/**
* Match an {@code identityref} leaf's value.
*
* @param methodRef method reference to the getter method
* @return A {@link ValueMatchBuilder}
* @throws NullPointerException if methodRef is null
*/
@NonNull ValueMatchBuilder leaf(IdentityLeafReference methodRef);
/**
* Match a generic leaf value.
*
* @param methodRef method reference to the getter method
* @return A {@link ValueMatchBuilder}
* @throws NullPointerException if methodRef is null
*/
@NonNull ValueMatchBuilder leaf(TypeObjectLeafReference methodRef);
/**
* Base interface for capturing binding getter method references through lambda expressions. This interface should
* never be used directly, but rather through one of its specializations.
*
* This interface uncharacteristically extends {@link Serializable} for the purposes of making the resulting
* lambda also Serializable. This part is critical for the process of introspection into the lambda and identifying
* the method being invoked.
*
* @param
Parent type
* @param Child type
*/
sealed interface LeafReference extends Serializable {
/**
* Dummy method to express the method signature of a typical getter. Due to this match we can match any Java
* method reference which takes in {@code parent} and results in {@code child} -- expose the feature of using
* {@code Parent::getChild} method shorthand.
*
* @param parent Parent object
* @return Leaf value
* @deprecated This method is present only for technical realization of taking the method reference and should
* never be involved directly. See {@code LambdaDecoder} for gory details.
*/
@Deprecated(forRemoval = true)
C dummyMethod(P parent);
}
@FunctionalInterface
non-sealed interface BooleanLeafReference
extends LeafReference
{
// Nothing here
}
@FunctionalInterface
non-sealed interface Decimal64LeafReference
extends LeafReference
{
// Nothing here
}
@FunctionalInterface
non-sealed interface EmptyLeafReference
extends LeafReference
{
// Nothing here
}
@FunctionalInterface
non-sealed interface StringLeafReference
extends LeafReference
{
// Nothing here
}
@FunctionalInterface
non-sealed interface Int8LeafReference
extends LeafReference
{
// Nothing here
}
@FunctionalInterface
non-sealed interface Int16LeafReference
extends LeafReference
{
// Nothing here
}
@FunctionalInterface
non-sealed interface Int32LeafReference
extends LeafReference
{
// Nothing here
}
@FunctionalInterface
non-sealed interface Int64LeafReference
extends LeafReference
{
// Nothing here
}
@FunctionalInterface
non-sealed interface Uint8LeafReference
extends LeafReference
{
// Nothing here
}
@FunctionalInterface
non-sealed interface Uint16LeafReference
extends LeafReference
{
// Nothing here
}
@FunctionalInterface
non-sealed interface Uint32LeafReference
extends LeafReference
{
// Nothing here
}
@FunctionalInterface
non-sealed interface Uint64LeafReference
extends LeafReference
{
// Nothing here
}
@FunctionalInterface
non-sealed interface IdentityLeafReference
extends LeafReference
{
// Nothing here
}
@FunctionalInterface
non-sealed interface TypeObjectLeafReference
extends LeafReference
{
// Nothing here
}
}