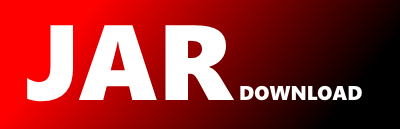
org.opendaylight.restconf.server.spi.AbstractRestconfStreamRegistry Maven / Gradle / Ivy
/*
* Copyright (c) 2023 PANTHEON.tech, s.r.o. and others. All rights reserved.
*
* This program and the accompanying materials are made available under the
* terms of the Eclipse Public License v1.0 which accompanies this distribution,
* and is available at http://www.eclipse.org/legal/epl-v10.html
*/
package org.opendaylight.restconf.server.spi;
import static java.util.Objects.requireNonNull;
import com.google.common.annotations.VisibleForTesting;
import com.google.common.util.concurrent.FutureCallback;
import com.google.common.util.concurrent.Futures;
import com.google.common.util.concurrent.ListenableFuture;
import com.google.common.util.concurrent.MoreExecutors;
import java.net.URI;
import java.util.Set;
import java.util.UUID;
import java.util.concurrent.ConcurrentHashMap;
import java.util.concurrent.ConcurrentMap;
import org.eclipse.jdt.annotation.NonNull;
import org.eclipse.jdt.annotation.Nullable;
import org.opendaylight.restconf.server.api.ServerException;
import org.opendaylight.restconf.server.api.ServerRequest;
import org.opendaylight.restconf.server.spi.RestconfStream.EncodingName;
import org.opendaylight.restconf.server.spi.RestconfStream.Source;
import org.opendaylight.yang.gen.v1.urn.ietf.params.xml.ns.yang.ietf.restconf.monitoring.rev170126.restconf.state.streams.Stream;
import org.opendaylight.yang.gen.v1.urn.ietf.params.xml.ns.yang.ietf.restconf.monitoring.rev170126.restconf.state.streams.stream.Access;
import org.opendaylight.yangtools.yang.common.QName;
import org.opendaylight.yangtools.yang.data.api.YangInstanceIdentifier.NodeIdentifier;
import org.opendaylight.yangtools.yang.data.api.YangInstanceIdentifier.NodeIdentifierWithPredicates;
import org.opendaylight.yangtools.yang.data.api.schema.MapEntryNode;
import org.opendaylight.yangtools.yang.data.spi.node.ImmutableNodes;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
/**
* Reference base class for {@link RestconfStream.Registry} implementations.
*/
public abstract class AbstractRestconfStreamRegistry implements RestconfStream.Registry {
private static final Logger LOG = LoggerFactory.getLogger(AbstractRestconfStreamRegistry.class);
@VisibleForTesting
public static final QName NAME_QNAME = QName.create(Stream.QNAME, "name").intern();
@VisibleForTesting
public static final QName DESCRIPTION_QNAME = QName.create(Stream.QNAME, "description").intern();
@VisibleForTesting
public static final QName ENCODING_QNAME = QName.create(Stream.QNAME, "encoding").intern();
@VisibleForTesting
public static final QName LOCATION_QNAME = QName.create(Stream.QNAME, "location").intern();
private final ConcurrentMap> streams = new ConcurrentHashMap<>();
private final RestconfStream.LocationProvider locationProvider;
protected AbstractRestconfStreamRegistry(final RestconfStream.LocationProvider locationProvider) {
this.locationProvider = requireNonNull(locationProvider);
}
@Override
public final @Nullable RestconfStream> lookupStream(final String name) {
return streams.get(requireNonNull(name));
}
@Override
public final void createStream(final ServerRequest> request, final URI restconfURI,
final Source source, final String description) {
final var baseStreamLocation = locationProvider.baseStreamLocation(restconfURI);
final var stream = allocateStream(source);
final var name = stream.name();
if (description.isBlank()) {
throw new IllegalArgumentException("Description must be descriptive");
}
Futures.addCallback(putStream(streamEntry(name, description, baseStreamLocation.toString(),
stream.encodings())), new FutureCallback
© 2015 - 2024 Weber Informatics LLC | Privacy Policy