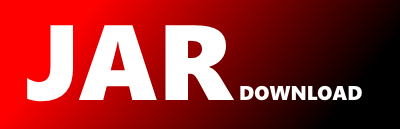
org.opendaylight.ovsdb.utils.yang.YangUtils Maven / Gradle / Ivy
/*
* Copyright © 2016 Red Hat, Inc. and others.
*
* This program and the accompanying materials are made available under the
* terms of the Eclipse Public License v1.0 which accompanies this distribution,
* and is available at http://www.eclipse.org/legal/epl-v10.html
*/
package org.opendaylight.ovsdb.utils.yang;
import java.util.HashMap;
import java.util.Map;
import java.util.function.Function;
import javax.annotation.Nonnull;
import javax.annotation.Nullable;
import com.google.common.base.Preconditions;
/**
* YANG utility functions.
*/
public final class YangUtils {
/**
* Prevent instantiation.
*/
private YangUtils() {
// Nothing to do
}
/**
* Copies a list of YANG key-value items to the given map. Any {@code null} key or value will cause an error.
*
* @param map The map to fill.
* @param yangList The list of YANG key-value items.
* @param keyExtractor The key extractor function to use.
* @param valueExtractor The value extractor function to use.
* @param The YANG item type.
* @param The key type.
* @param The value type.
* @return The map.
*/
@Nonnull
public static Map copyYangKeyValueListToMap(@Nonnull Map map, @Nullable Iterable yangList,
@Nonnull Function keyExtractor,
@Nonnull Function valueExtractor) {
if (yangList != null) {
for (T yangValue : yangList) {
K key = keyExtractor.apply(yangValue);
V value = valueExtractor.apply(yangValue);
Preconditions.checkNotNull(key);
Preconditions.checkNotNull(value);
map.put(key, value);
}
}
return map;
}
/**
* Converts a list of YANG key-value items to a map.
*
* @param yangList The list of YANG key-value items.
* @param keyExtractor The key extractor function to use.
* @param valueExtractor The value extractor function to use.
* @param The YANG item type.
* @param The key type.
* @param The value type.
* @return The map.
*/
@Nonnull
public static Map convertYangKeyValueListToMap(@Nullable Iterable yangList,
@Nonnull Function keyExtractor,
@Nonnull Function valueExtractor) {
return copyYangKeyValueListToMap(new HashMap<>(), yangList, keyExtractor, valueExtractor);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy