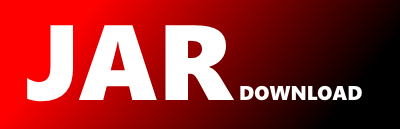
org.openehr.rm.binding.DADLBinding Maven / Gradle / Ivy
/*
* component: "openEHR Java Reference Implementation"
* description: "Class DADLBinding"
* keywords: "binding"
*
* author: "Rong Chen "
* copyright: "Copyright (c) 2008 Cambio Healthcare Systems, Sweden"
* license: "See notice at bottom of class"
*
* file: "$URL$"
* revision: "$LastChangedRevision$"
* last_change: "$LastChangedDate$"
*/
package org.openehr.rm.binding;
import java.lang.annotation.Annotation;
import java.lang.reflect.Constructor;
import java.lang.reflect.Method;
import java.lang.reflect.Type;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.SortedMap;
import java.util.TreeMap;
import org.apache.commons.lang.StringUtils;
import org.apache.log4j.Logger;
import org.openehr.am.parser.AttributeValue;
import org.openehr.am.parser.ComplexObjectBlock;
import org.openehr.am.parser.ContentObject;
import org.openehr.am.parser.KeyedObject;
import org.openehr.am.parser.MultipleAttributeObjectBlock;
import org.openehr.am.parser.ObjectBlock;
import org.openehr.am.parser.PrimitiveObjectBlock;
import org.openehr.am.parser.SimpleValue;
import org.openehr.am.parser.SingleAttributeObjectBlock;
import org.openehr.build.RMObjectBuilder;
import org.openehr.build.RMObjectBuildingException;
import org.openehr.build.SystemValue;
import org.openehr.rm.Attribute;
import org.openehr.rm.FullConstructor;
import org.openehr.rm.RMObject;
import org.openehr.rm.datatypes.quantity.ProportionKind;
import org.openehr.rm.datatypes.text.CodePhrase;
import org.openehr.rm.support.basic.Interval;
import org.openehr.rm.support.measurement.MeasurementService;
import org.openehr.rm.support.measurement.SimpleMeasurementService;
import org.openehr.rm.support.terminology.TerminologyService;
import org.openehr.terminology.SimpleTerminologyService;
/**
* Utility class that binds data in DADL format to openEHR RM
*
* @author rong.chen
*/
public class DADLBinding {
public DADLBinding() {
try {
TerminologyService termServ;
MeasurementService measureServ;
termServ = SimpleTerminologyService.getInstance();
measureServ = SimpleMeasurementService.getInstance();
CodePhrase lang = new CodePhrase("ISO_639-1", "en");
CodePhrase charset = new CodePhrase("IANA_character-sets", "UTF-8");
Map values = new HashMap();
values.put(SystemValue.LANGUAGE, lang);
values.put(SystemValue.CHARSET, charset);
values.put(SystemValue.ENCODING, charset);
values.put(SystemValue.TERMINOLOGY_SERVICE, termServ);
values.put(SystemValue.MEASUREMENT_SERVICE, measureServ);
builder = new RMObjectBuilder(values);
} catch (Exception e) {
e.printStackTrace();
throw new RuntimeException("failed to start DADLBinding..");
}
}
public Object bind(ContentObject co) throws DADLBindingException,
RMObjectBuildingException {
if (co.getAttributeValues() != null) {
return bindAttributes(null, co.getAttributeValues());
} else {
ComplexObjectBlock complexObj = co.getComplexObjectBlock();
return bindComplexBlock(complexObj);
}
}
RMObject bindAttributes(String type, List attributes)
throws DADLBindingException, RMObjectBuildingException {
Map values = new HashMap();
for (AttributeValue attr : attributes) {
String id = attr.getId();
Object value = bindObjectBlock(attr.getValue());
values.put(id, value);
}
return invokeRMObjectBuilder(type, values);
}
RMObject invokeRMObjectBuilder(String type, Map valueMap)
throws DADLBindingException, RMObjectBuildingException {
if(type == null) {
type = builder.findMatchingRMClass(valueMap);
if(type == null) {
throw new DADLBindingException("failed untyped binding with - "
+ valueMap);
}
}
RMObject rmObj = builder.construct(type, valueMap);
return rmObj;
}
Object bindObjectBlock(ObjectBlock block) throws DADLBindingException,
RMObjectBuildingException {
if (block instanceof PrimitiveObjectBlock) {
return bindPrimitiveBlock((PrimitiveObjectBlock) block);
} else {
return bindComplexBlock((ComplexObjectBlock) block);
}
}
Object bindPrimitiveBlock(PrimitiveObjectBlock block)
throws DADLBindingException {
if (block.getSimpleValue() != null) {
return block.getSimpleValue().getValue();
} else if (block.getSimpleListValue() != null) {
List values = block.getSimpleListValue();
List list = new ArrayList(values.size());
for (SimpleValue sv : values) {
list.add(sv.getValue());
}
return list;
} else if (block.getSimpleIntervalValue() != null) {
return null;
} else if (block.getTermCode() != null) {
return block.getTermCode();
} else if (block.getTermCodeListValue() != null) {
return block.getTermCodeListValue();
} else {
throw new DADLBindingException("empty block");
}
}
Object bindComplexBlock(ComplexObjectBlock block)
throws DADLBindingException, RMObjectBuildingException {
if (block instanceof SingleAttributeObjectBlock) {
SingleAttributeObjectBlock singleBlock =
(SingleAttributeObjectBlock) block;
// a special case to deal with empty attribute list
if("LIST".equalsIgnoreCase(singleBlock.getTypeIdentifier())
&& singleBlock.getAttributeValues().isEmpty()) {
return new ArrayList();
}
return bindAttributes(singleBlock.getTypeIdentifier(), singleBlock
.getAttributeValues());
} else {
MultipleAttributeObjectBlock multiBlock =
(MultipleAttributeObjectBlock) block;
List list = multiBlock.getKeyObjects();
// TODO assume list?
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy