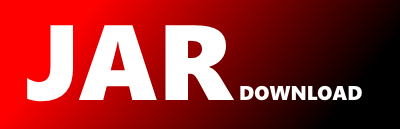
org.openehr.rm.binding.XPathUtil Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of dadl-binding Show documentation
Show all versions of dadl-binding Show documentation
java openEHR RM and dADL Binding Component
The newest version!
package org.openehr.rm.binding;
import org.apache.log4j.Logger;
import org.openehr.rm.Attribute;
import org.openehr.rm.common.archetyped.Locatable;
import org.openehr.rm.composition.content.entry.Activity;
import org.openehr.rm.composition.content.entry.Entry;
import org.openehr.rm.datastructure.itemstructure.representation.Element;
import java.lang.reflect.Method;
import java.util.*;
public class XPathUtil {
public Set extractXPaths(Locatable root) throws Exception {
Set set = new HashSet();
buildPath(root, "", set);
return set;
}
private void buildPath(Object obj, String path, Set paths) throws Exception {
if(obj instanceof List) {
List list = (List) obj;
for(int i = 0, j = list.size(); i attributeMap = inspector.attributeMap(klass);
for(Map.Entry entry : attributeMap.entrySet()) {
String attributeName = entry.getKey();
Attribute attribute = entry.getValue();
if(attribute.system()) {
continue;
}
Method method = klass.getMethod("get" +
attributeName.substring(0, 1).toUpperCase() +
attributeName.substring(1), null);
assert(method != null);
Object value = method.invoke(obj, null);
if(value != null) {
buildPath(value, path + "/" + attributeName, paths);
}
}
}
}
public Map> extractPaths(Locatable root) throws Exception {
Map> map = new HashMap>();
buildPaths(root, "", "", map);
return map;
}
private void buildPaths(Object obj, String apath, String xpath, Map> paths) throws Exception {
//System.out.println("apath: " + apath + ", xpath: " + xpath
// + ", nodeId: " + (obj instanceof Locatable ? ((Locatable) obj).getArchetypeNodeId() : ""));
if(obj instanceof List) {
List list = (List) obj;
for(int i = 0, j = list.size(); i set = paths.get(apath);
if(set == null) {
set = new HashSet();
paths.put(apath, set);
}
set.add(xpath);
} else if(obj instanceof Locatable) {
Locatable locatable = (Locatable) obj;
inspector.retrieveRMAttributes(obj.getClass().getSimpleName());
Class klass = obj.getClass();
Map attributeMap = inspector.attributeMap(klass);
for(Map.Entry entry : attributeMap.entrySet()) {
String attributeName = entry.getKey();
Attribute attribute = entry.getValue();
if(attribute.system()) {
continue;
}
String methodName = "get" +
attributeName.substring(0, 1).toUpperCase() +
attributeName.substring(1);
Method method = klass.getMethod(methodName, null);
assert(method != null);
Object value = method.invoke(obj, null);
if(value != null && !methodName.equals("getParent")) {
String nodeIdStr = "";
if (value instanceof Locatable){
nodeIdStr = "[" + ((Locatable)value).getArchetypeNodeId() + "]";
}
String currentAPath = apath + "/" + attributeName+nodeIdStr;
String currentXPath = xpath + "/" + attributeName;
buildPaths(value, currentAPath, currentXPath, paths);
}
}
}
}
private String getNodeId(Locatable loc, String nodeId) {
StringBuilder sb = new StringBuilder();
sb.append(nodeId);
if (loc instanceof Entry){
sb.append(" and name/value='" + loc.getName().getValue()+"'");
}
return sb.toString();
}
public Set extractRootXPaths(Locatable root) throws Exception {
Set set = new HashSet();
buildRootPath(root, "", set);
return set;
}
private void buildRootPath(Object obj, String path, Set paths) throws Exception {
if(obj instanceof List) {
List list = (List) obj;
for(int i = 0, j = list.size(); i attributeMap = inspector.attributeMap(klass);
for(Map.Entry entry : attributeMap.entrySet()) {
String attributeName = entry.getKey();
Attribute attribute = entry.getValue();
if(attribute.system()) {
continue; // skip system attributes
}
String getterName = "get"+
attributeName.substring(0, 1).toUpperCase() +
attributeName.substring(1);
Method method = klass.getMethod(getterName, null);
assert(method != null);
Object value = method.invoke(obj, null);
if(value != null) {
buildRootPath(value, path + "/" + attributeName, paths);
}
}
}
}
private RMInspector inspector = RMInspector.getInstance();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy