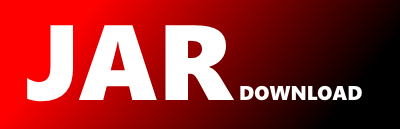
openEHR.v1.template.impl.ENTRYImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of oet-parser Show documentation
Show all versions of oet-parser Show documentation
Java implementation of openEHR OET Template Parser and Flattener
The newest version!
/*
* XML Type: ENTRY
* Namespace: openEHR/v1/Template
* Java type: openEHR.v1.template.ENTRY
*
* Automatically generated - do not modify.
*/
package openEHR.v1.template.impl;
/**
* An XML ENTRY(@openEHR/v1/Template).
*
* This is a complex type.
*/
public class ENTRYImpl extends openEHR.v1.template.impl.ContentItemImpl implements openEHR.v1.template.ENTRY
{
private static final long serialVersionUID = 1L;
public ENTRYImpl(org.apache.xmlbeans.SchemaType sType)
{
super(sType);
}
private static final javax.xml.namespace.QName SUBJECTOFCARE$0 =
new javax.xml.namespace.QName("openEHR/v1/Template", "SubjectOfCare");
private static final javax.xml.namespace.QName OTHERPARTICIPATION$2 =
new javax.xml.namespace.QName("openEHR/v1/Template", "other_participation");
private static final javax.xml.namespace.QName ITEMS$4 =
new javax.xml.namespace.QName("openEHR/v1/Template", "Items");
/**
* Gets the "SubjectOfCare" element
*/
public openEHR.v1.template.TextConstraint getSubjectOfCare()
{
synchronized (monitor())
{
check_orphaned();
openEHR.v1.template.TextConstraint target = null;
target = (openEHR.v1.template.TextConstraint)get_store().find_element_user(SUBJECTOFCARE$0, 0);
if (target == null)
{
return null;
}
return target;
}
}
/**
* True if has "SubjectOfCare" element
*/
public boolean isSetSubjectOfCare()
{
synchronized (monitor())
{
check_orphaned();
return get_store().count_elements(SUBJECTOFCARE$0) != 0;
}
}
/**
* Sets the "SubjectOfCare" element
*/
public void setSubjectOfCare(openEHR.v1.template.TextConstraint subjectOfCare)
{
synchronized (monitor())
{
check_orphaned();
openEHR.v1.template.TextConstraint target = null;
target = (openEHR.v1.template.TextConstraint)get_store().find_element_user(SUBJECTOFCARE$0, 0);
if (target == null)
{
target = (openEHR.v1.template.TextConstraint)get_store().add_element_user(SUBJECTOFCARE$0);
}
target.set(subjectOfCare);
}
}
/**
* Appends and returns a new empty "SubjectOfCare" element
*/
public openEHR.v1.template.TextConstraint addNewSubjectOfCare()
{
synchronized (monitor())
{
check_orphaned();
openEHR.v1.template.TextConstraint target = null;
target = (openEHR.v1.template.TextConstraint)get_store().add_element_user(SUBJECTOFCARE$0);
return target;
}
}
/**
* Unsets the "SubjectOfCare" element
*/
public void unsetSubjectOfCare()
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_element(SUBJECTOFCARE$0, 0);
}
}
/**
* Gets array of all "other_participation" elements
*/
public openEHR.v1.template.PARTICIPATION[] getOtherParticipationArray()
{
synchronized (monitor())
{
check_orphaned();
java.util.List targetList = new java.util.ArrayList();
get_store().find_all_element_users(OTHERPARTICIPATION$2, targetList);
openEHR.v1.template.PARTICIPATION[] result = new openEHR.v1.template.PARTICIPATION[targetList.size()];
targetList.toArray(result);
return result;
}
}
/**
* Gets ith "other_participation" element
*/
public openEHR.v1.template.PARTICIPATION getOtherParticipationArray(int i)
{
synchronized (monitor())
{
check_orphaned();
openEHR.v1.template.PARTICIPATION target = null;
target = (openEHR.v1.template.PARTICIPATION)get_store().find_element_user(OTHERPARTICIPATION$2, i);
if (target == null)
{
throw new IndexOutOfBoundsException();
}
return target;
}
}
/**
* Returns number of "other_participation" element
*/
public int sizeOfOtherParticipationArray()
{
synchronized (monitor())
{
check_orphaned();
return get_store().count_elements(OTHERPARTICIPATION$2);
}
}
/**
* Sets array of all "other_participation" element
*/
public void setOtherParticipationArray(openEHR.v1.template.PARTICIPATION[] otherParticipationArray)
{
synchronized (monitor())
{
check_orphaned();
arraySetterHelper(otherParticipationArray, OTHERPARTICIPATION$2);
}
}
/**
* Sets ith "other_participation" element
*/
public void setOtherParticipationArray(int i, openEHR.v1.template.PARTICIPATION otherParticipation)
{
synchronized (monitor())
{
check_orphaned();
openEHR.v1.template.PARTICIPATION target = null;
target = (openEHR.v1.template.PARTICIPATION)get_store().find_element_user(OTHERPARTICIPATION$2, i);
if (target == null)
{
throw new IndexOutOfBoundsException();
}
target.set(otherParticipation);
}
}
/**
* Inserts and returns a new empty value (as xml) as the ith "other_participation" element
*/
public openEHR.v1.template.PARTICIPATION insertNewOtherParticipation(int i)
{
synchronized (monitor())
{
check_orphaned();
openEHR.v1.template.PARTICIPATION target = null;
target = (openEHR.v1.template.PARTICIPATION)get_store().insert_element_user(OTHERPARTICIPATION$2, i);
return target;
}
}
/**
* Appends and returns a new empty value (as xml) as the last "other_participation" element
*/
public openEHR.v1.template.PARTICIPATION addNewOtherParticipation()
{
synchronized (monitor())
{
check_orphaned();
openEHR.v1.template.PARTICIPATION target = null;
target = (openEHR.v1.template.PARTICIPATION)get_store().add_element_user(OTHERPARTICIPATION$2);
return target;
}
}
/**
* Removes the ith "other_participation" element
*/
public void removeOtherParticipation(int i)
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_element(OTHERPARTICIPATION$2, i);
}
}
/**
* Gets array of all "Items" elements
*/
public openEHR.v1.template.ITEM[] getItemsArray()
{
synchronized (monitor())
{
check_orphaned();
java.util.List targetList = new java.util.ArrayList();
get_store().find_all_element_users(ITEMS$4, targetList);
openEHR.v1.template.ITEM[] result = new openEHR.v1.template.ITEM[targetList.size()];
targetList.toArray(result);
return result;
}
}
/**
* Gets ith "Items" element
*/
public openEHR.v1.template.ITEM getItemsArray(int i)
{
synchronized (monitor())
{
check_orphaned();
openEHR.v1.template.ITEM target = null;
target = (openEHR.v1.template.ITEM)get_store().find_element_user(ITEMS$4, i);
if (target == null)
{
throw new IndexOutOfBoundsException();
}
return target;
}
}
/**
* Returns number of "Items" element
*/
public int sizeOfItemsArray()
{
synchronized (monitor())
{
check_orphaned();
return get_store().count_elements(ITEMS$4);
}
}
/**
* Sets array of all "Items" element
*/
public void setItemsArray(openEHR.v1.template.ITEM[] itemsArray)
{
synchronized (monitor())
{
check_orphaned();
arraySetterHelper(itemsArray, ITEMS$4);
}
}
/**
* Sets ith "Items" element
*/
public void setItemsArray(int i, openEHR.v1.template.ITEM items)
{
synchronized (monitor())
{
check_orphaned();
openEHR.v1.template.ITEM target = null;
target = (openEHR.v1.template.ITEM)get_store().find_element_user(ITEMS$4, i);
if (target == null)
{
throw new IndexOutOfBoundsException();
}
target.set(items);
}
}
/**
* Inserts and returns a new empty value (as xml) as the ith "Items" element
*/
public openEHR.v1.template.ITEM insertNewItems(int i)
{
synchronized (monitor())
{
check_orphaned();
openEHR.v1.template.ITEM target = null;
target = (openEHR.v1.template.ITEM)get_store().insert_element_user(ITEMS$4, i);
return target;
}
}
/**
* Appends and returns a new empty value (as xml) as the last "Items" element
*/
public openEHR.v1.template.ITEM addNewItems()
{
synchronized (monitor())
{
check_orphaned();
openEHR.v1.template.ITEM target = null;
target = (openEHR.v1.template.ITEM)get_store().add_element_user(ITEMS$4);
return target;
}
}
/**
* Removes the ith "Items" element
*/
public void removeItems(int i)
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_element(ITEMS$4, i);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy