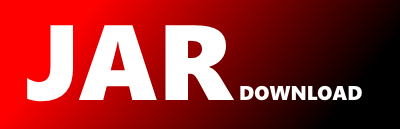
org.openehr.schemas.v1.impl.DVMULTIMEDIAImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of oet-parser Show documentation
Show all versions of oet-parser Show documentation
Java implementation of openEHR OET Template Parser and Flattener
The newest version!
/*
* XML Type: DV_MULTIMEDIA
* Namespace: http://schemas.openehr.org/v1
* Java type: org.openehr.schemas.v1.DVMULTIMEDIA
*
* Automatically generated - do not modify.
*/
package org.openehr.schemas.v1.impl;
/**
* An XML DV_MULTIMEDIA(@http://schemas.openehr.org/v1).
*
* This is a complex type.
*/
public class DVMULTIMEDIAImpl extends org.openehr.schemas.v1.impl.DVENCAPSULATEDImpl implements org.openehr.schemas.v1.DVMULTIMEDIA
{
private static final long serialVersionUID = 1L;
public DVMULTIMEDIAImpl(org.apache.xmlbeans.SchemaType sType)
{
super(sType);
}
private static final javax.xml.namespace.QName ALTERNATETEXT$0 =
new javax.xml.namespace.QName("http://schemas.openehr.org/v1", "alternate_text");
private static final javax.xml.namespace.QName URI$2 =
new javax.xml.namespace.QName("http://schemas.openehr.org/v1", "uri");
private static final javax.xml.namespace.QName DATA$4 =
new javax.xml.namespace.QName("http://schemas.openehr.org/v1", "data");
private static final javax.xml.namespace.QName MEDIATYPE$6 =
new javax.xml.namespace.QName("http://schemas.openehr.org/v1", "media_type");
private static final javax.xml.namespace.QName COMPRESSIONALGORITHM$8 =
new javax.xml.namespace.QName("http://schemas.openehr.org/v1", "compression_algorithm");
private static final javax.xml.namespace.QName INTEGRITYCHECK$10 =
new javax.xml.namespace.QName("http://schemas.openehr.org/v1", "integrity_check");
private static final javax.xml.namespace.QName INTEGRITYCHECKALGORITHM$12 =
new javax.xml.namespace.QName("http://schemas.openehr.org/v1", "integrity_check_algorithm");
private static final javax.xml.namespace.QName SIZE$14 =
new javax.xml.namespace.QName("http://schemas.openehr.org/v1", "size");
private static final javax.xml.namespace.QName THUMBNAIL$16 =
new javax.xml.namespace.QName("http://schemas.openehr.org/v1", "thumbnail");
/**
* Gets the "alternate_text" element
*/
public java.lang.String getAlternateText()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_element_user(ALTERNATETEXT$0, 0);
if (target == null)
{
return null;
}
return target.getStringValue();
}
}
/**
* Gets (as xml) the "alternate_text" element
*/
public org.apache.xmlbeans.XmlString xgetAlternateText()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlString target = null;
target = (org.apache.xmlbeans.XmlString)get_store().find_element_user(ALTERNATETEXT$0, 0);
return target;
}
}
/**
* True if has "alternate_text" element
*/
public boolean isSetAlternateText()
{
synchronized (monitor())
{
check_orphaned();
return get_store().count_elements(ALTERNATETEXT$0) != 0;
}
}
/**
* Sets the "alternate_text" element
*/
public void setAlternateText(java.lang.String alternateText)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_element_user(ALTERNATETEXT$0, 0);
if (target == null)
{
target = (org.apache.xmlbeans.SimpleValue)get_store().add_element_user(ALTERNATETEXT$0);
}
target.setStringValue(alternateText);
}
}
/**
* Sets (as xml) the "alternate_text" element
*/
public void xsetAlternateText(org.apache.xmlbeans.XmlString alternateText)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlString target = null;
target = (org.apache.xmlbeans.XmlString)get_store().find_element_user(ALTERNATETEXT$0, 0);
if (target == null)
{
target = (org.apache.xmlbeans.XmlString)get_store().add_element_user(ALTERNATETEXT$0);
}
target.set(alternateText);
}
}
/**
* Unsets the "alternate_text" element
*/
public void unsetAlternateText()
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_element(ALTERNATETEXT$0, 0);
}
}
/**
* Gets the "uri" element
*/
public org.openehr.schemas.v1.DVURI getUri()
{
synchronized (monitor())
{
check_orphaned();
org.openehr.schemas.v1.DVURI target = null;
target = (org.openehr.schemas.v1.DVURI)get_store().find_element_user(URI$2, 0);
if (target == null)
{
return null;
}
return target;
}
}
/**
* True if has "uri" element
*/
public boolean isSetUri()
{
synchronized (monitor())
{
check_orphaned();
return get_store().count_elements(URI$2) != 0;
}
}
/**
* Sets the "uri" element
*/
public void setUri(org.openehr.schemas.v1.DVURI uri)
{
synchronized (monitor())
{
check_orphaned();
org.openehr.schemas.v1.DVURI target = null;
target = (org.openehr.schemas.v1.DVURI)get_store().find_element_user(URI$2, 0);
if (target == null)
{
target = (org.openehr.schemas.v1.DVURI)get_store().add_element_user(URI$2);
}
target.set(uri);
}
}
/**
* Appends and returns a new empty "uri" element
*/
public org.openehr.schemas.v1.DVURI addNewUri()
{
synchronized (monitor())
{
check_orphaned();
org.openehr.schemas.v1.DVURI target = null;
target = (org.openehr.schemas.v1.DVURI)get_store().add_element_user(URI$2);
return target;
}
}
/**
* Unsets the "uri" element
*/
public void unsetUri()
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_element(URI$2, 0);
}
}
/**
* Gets the "data" element
*/
public byte[] getData()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_element_user(DATA$4, 0);
if (target == null)
{
return null;
}
return target.getByteArrayValue();
}
}
/**
* Gets (as xml) the "data" element
*/
public org.apache.xmlbeans.XmlBase64Binary xgetData()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlBase64Binary target = null;
target = (org.apache.xmlbeans.XmlBase64Binary)get_store().find_element_user(DATA$4, 0);
return target;
}
}
/**
* True if has "data" element
*/
public boolean isSetData()
{
synchronized (monitor())
{
check_orphaned();
return get_store().count_elements(DATA$4) != 0;
}
}
/**
* Sets the "data" element
*/
public void setData(byte[] data)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_element_user(DATA$4, 0);
if (target == null)
{
target = (org.apache.xmlbeans.SimpleValue)get_store().add_element_user(DATA$4);
}
target.setByteArrayValue(data);
}
}
/**
* Sets (as xml) the "data" element
*/
public void xsetData(org.apache.xmlbeans.XmlBase64Binary data)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlBase64Binary target = null;
target = (org.apache.xmlbeans.XmlBase64Binary)get_store().find_element_user(DATA$4, 0);
if (target == null)
{
target = (org.apache.xmlbeans.XmlBase64Binary)get_store().add_element_user(DATA$4);
}
target.set(data);
}
}
/**
* Unsets the "data" element
*/
public void unsetData()
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_element(DATA$4, 0);
}
}
/**
* Gets the "media_type" element
*/
public org.openehr.schemas.v1.CODEPHRASE getMediaType()
{
synchronized (monitor())
{
check_orphaned();
org.openehr.schemas.v1.CODEPHRASE target = null;
target = (org.openehr.schemas.v1.CODEPHRASE)get_store().find_element_user(MEDIATYPE$6, 0);
if (target == null)
{
return null;
}
return target;
}
}
/**
* Sets the "media_type" element
*/
public void setMediaType(org.openehr.schemas.v1.CODEPHRASE mediaType)
{
synchronized (monitor())
{
check_orphaned();
org.openehr.schemas.v1.CODEPHRASE target = null;
target = (org.openehr.schemas.v1.CODEPHRASE)get_store().find_element_user(MEDIATYPE$6, 0);
if (target == null)
{
target = (org.openehr.schemas.v1.CODEPHRASE)get_store().add_element_user(MEDIATYPE$6);
}
target.set(mediaType);
}
}
/**
* Appends and returns a new empty "media_type" element
*/
public org.openehr.schemas.v1.CODEPHRASE addNewMediaType()
{
synchronized (monitor())
{
check_orphaned();
org.openehr.schemas.v1.CODEPHRASE target = null;
target = (org.openehr.schemas.v1.CODEPHRASE)get_store().add_element_user(MEDIATYPE$6);
return target;
}
}
/**
* Gets the "compression_algorithm" element
*/
public org.openehr.schemas.v1.CODEPHRASE getCompressionAlgorithm()
{
synchronized (monitor())
{
check_orphaned();
org.openehr.schemas.v1.CODEPHRASE target = null;
target = (org.openehr.schemas.v1.CODEPHRASE)get_store().find_element_user(COMPRESSIONALGORITHM$8, 0);
if (target == null)
{
return null;
}
return target;
}
}
/**
* True if has "compression_algorithm" element
*/
public boolean isSetCompressionAlgorithm()
{
synchronized (monitor())
{
check_orphaned();
return get_store().count_elements(COMPRESSIONALGORITHM$8) != 0;
}
}
/**
* Sets the "compression_algorithm" element
*/
public void setCompressionAlgorithm(org.openehr.schemas.v1.CODEPHRASE compressionAlgorithm)
{
synchronized (monitor())
{
check_orphaned();
org.openehr.schemas.v1.CODEPHRASE target = null;
target = (org.openehr.schemas.v1.CODEPHRASE)get_store().find_element_user(COMPRESSIONALGORITHM$8, 0);
if (target == null)
{
target = (org.openehr.schemas.v1.CODEPHRASE)get_store().add_element_user(COMPRESSIONALGORITHM$8);
}
target.set(compressionAlgorithm);
}
}
/**
* Appends and returns a new empty "compression_algorithm" element
*/
public org.openehr.schemas.v1.CODEPHRASE addNewCompressionAlgorithm()
{
synchronized (monitor())
{
check_orphaned();
org.openehr.schemas.v1.CODEPHRASE target = null;
target = (org.openehr.schemas.v1.CODEPHRASE)get_store().add_element_user(COMPRESSIONALGORITHM$8);
return target;
}
}
/**
* Unsets the "compression_algorithm" element
*/
public void unsetCompressionAlgorithm()
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_element(COMPRESSIONALGORITHM$8, 0);
}
}
/**
* Gets the "integrity_check" element
*/
public byte[] getIntegrityCheck()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_element_user(INTEGRITYCHECK$10, 0);
if (target == null)
{
return null;
}
return target.getByteArrayValue();
}
}
/**
* Gets (as xml) the "integrity_check" element
*/
public org.apache.xmlbeans.XmlBase64Binary xgetIntegrityCheck()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlBase64Binary target = null;
target = (org.apache.xmlbeans.XmlBase64Binary)get_store().find_element_user(INTEGRITYCHECK$10, 0);
return target;
}
}
/**
* True if has "integrity_check" element
*/
public boolean isSetIntegrityCheck()
{
synchronized (monitor())
{
check_orphaned();
return get_store().count_elements(INTEGRITYCHECK$10) != 0;
}
}
/**
* Sets the "integrity_check" element
*/
public void setIntegrityCheck(byte[] integrityCheck)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_element_user(INTEGRITYCHECK$10, 0);
if (target == null)
{
target = (org.apache.xmlbeans.SimpleValue)get_store().add_element_user(INTEGRITYCHECK$10);
}
target.setByteArrayValue(integrityCheck);
}
}
/**
* Sets (as xml) the "integrity_check" element
*/
public void xsetIntegrityCheck(org.apache.xmlbeans.XmlBase64Binary integrityCheck)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlBase64Binary target = null;
target = (org.apache.xmlbeans.XmlBase64Binary)get_store().find_element_user(INTEGRITYCHECK$10, 0);
if (target == null)
{
target = (org.apache.xmlbeans.XmlBase64Binary)get_store().add_element_user(INTEGRITYCHECK$10);
}
target.set(integrityCheck);
}
}
/**
* Unsets the "integrity_check" element
*/
public void unsetIntegrityCheck()
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_element(INTEGRITYCHECK$10, 0);
}
}
/**
* Gets the "integrity_check_algorithm" element
*/
public org.openehr.schemas.v1.CODEPHRASE getIntegrityCheckAlgorithm()
{
synchronized (monitor())
{
check_orphaned();
org.openehr.schemas.v1.CODEPHRASE target = null;
target = (org.openehr.schemas.v1.CODEPHRASE)get_store().find_element_user(INTEGRITYCHECKALGORITHM$12, 0);
if (target == null)
{
return null;
}
return target;
}
}
/**
* True if has "integrity_check_algorithm" element
*/
public boolean isSetIntegrityCheckAlgorithm()
{
synchronized (monitor())
{
check_orphaned();
return get_store().count_elements(INTEGRITYCHECKALGORITHM$12) != 0;
}
}
/**
* Sets the "integrity_check_algorithm" element
*/
public void setIntegrityCheckAlgorithm(org.openehr.schemas.v1.CODEPHRASE integrityCheckAlgorithm)
{
synchronized (monitor())
{
check_orphaned();
org.openehr.schemas.v1.CODEPHRASE target = null;
target = (org.openehr.schemas.v1.CODEPHRASE)get_store().find_element_user(INTEGRITYCHECKALGORITHM$12, 0);
if (target == null)
{
target = (org.openehr.schemas.v1.CODEPHRASE)get_store().add_element_user(INTEGRITYCHECKALGORITHM$12);
}
target.set(integrityCheckAlgorithm);
}
}
/**
* Appends and returns a new empty "integrity_check_algorithm" element
*/
public org.openehr.schemas.v1.CODEPHRASE addNewIntegrityCheckAlgorithm()
{
synchronized (monitor())
{
check_orphaned();
org.openehr.schemas.v1.CODEPHRASE target = null;
target = (org.openehr.schemas.v1.CODEPHRASE)get_store().add_element_user(INTEGRITYCHECKALGORITHM$12);
return target;
}
}
/**
* Unsets the "integrity_check_algorithm" element
*/
public void unsetIntegrityCheckAlgorithm()
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_element(INTEGRITYCHECKALGORITHM$12, 0);
}
}
/**
* Gets the "size" element
*/
public int getSize()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_element_user(SIZE$14, 0);
if (target == null)
{
return 0;
}
return target.getIntValue();
}
}
/**
* Gets (as xml) the "size" element
*/
public org.apache.xmlbeans.XmlInt xgetSize()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlInt target = null;
target = (org.apache.xmlbeans.XmlInt)get_store().find_element_user(SIZE$14, 0);
return target;
}
}
/**
* Sets the "size" element
*/
public void setSize(int size)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_element_user(SIZE$14, 0);
if (target == null)
{
target = (org.apache.xmlbeans.SimpleValue)get_store().add_element_user(SIZE$14);
}
target.setIntValue(size);
}
}
/**
* Sets (as xml) the "size" element
*/
public void xsetSize(org.apache.xmlbeans.XmlInt size)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlInt target = null;
target = (org.apache.xmlbeans.XmlInt)get_store().find_element_user(SIZE$14, 0);
if (target == null)
{
target = (org.apache.xmlbeans.XmlInt)get_store().add_element_user(SIZE$14);
}
target.set(size);
}
}
/**
* Gets the "thumbnail" element
*/
public org.openehr.schemas.v1.DVMULTIMEDIA getThumbnail()
{
synchronized (monitor())
{
check_orphaned();
org.openehr.schemas.v1.DVMULTIMEDIA target = null;
target = (org.openehr.schemas.v1.DVMULTIMEDIA)get_store().find_element_user(THUMBNAIL$16, 0);
if (target == null)
{
return null;
}
return target;
}
}
/**
* True if has "thumbnail" element
*/
public boolean isSetThumbnail()
{
synchronized (monitor())
{
check_orphaned();
return get_store().count_elements(THUMBNAIL$16) != 0;
}
}
/**
* Sets the "thumbnail" element
*/
public void setThumbnail(org.openehr.schemas.v1.DVMULTIMEDIA thumbnail)
{
synchronized (monitor())
{
check_orphaned();
org.openehr.schemas.v1.DVMULTIMEDIA target = null;
target = (org.openehr.schemas.v1.DVMULTIMEDIA)get_store().find_element_user(THUMBNAIL$16, 0);
if (target == null)
{
target = (org.openehr.schemas.v1.DVMULTIMEDIA)get_store().add_element_user(THUMBNAIL$16);
}
target.set(thumbnail);
}
}
/**
* Appends and returns a new empty "thumbnail" element
*/
public org.openehr.schemas.v1.DVMULTIMEDIA addNewThumbnail()
{
synchronized (monitor())
{
check_orphaned();
org.openehr.schemas.v1.DVMULTIMEDIA target = null;
target = (org.openehr.schemas.v1.DVMULTIMEDIA)get_store().add_element_user(THUMBNAIL$16);
return target;
}
}
/**
* Unsets the "thumbnail" element
*/
public void unsetThumbnail()
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_element(THUMBNAIL$16, 0);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy