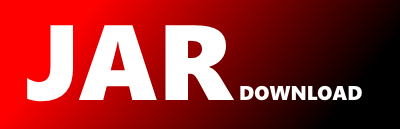
org.openehr.schemas.v1.impl.EXTRACTVERSIONSPECImpl Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of oet-parser Show documentation
Show all versions of oet-parser Show documentation
Java implementation of openEHR OET Template Parser and Flattener
The newest version!
/*
* XML Type: EXTRACT_VERSION_SPEC
* Namespace: http://schemas.openehr.org/v1
* Java type: org.openehr.schemas.v1.EXTRACTVERSIONSPEC
*
* Automatically generated - do not modify.
*/
package org.openehr.schemas.v1.impl;
/**
* An XML EXTRACT_VERSION_SPEC(@http://schemas.openehr.org/v1).
*
* This is a complex type.
*/
public class EXTRACTVERSIONSPECImpl extends org.apache.xmlbeans.impl.values.XmlComplexContentImpl implements org.openehr.schemas.v1.EXTRACTVERSIONSPEC
{
private static final long serialVersionUID = 1L;
public EXTRACTVERSIONSPECImpl(org.apache.xmlbeans.SchemaType sType)
{
super(sType);
}
private static final javax.xml.namespace.QName INCLUDEALLVERSIONS$0 =
new javax.xml.namespace.QName("http://schemas.openehr.org/v1", "include_all_versions");
private static final javax.xml.namespace.QName COMMITTIMEINTERVAL$2 =
new javax.xml.namespace.QName("http://schemas.openehr.org/v1", "commit_time_interval");
private static final javax.xml.namespace.QName INCLUDESREVISIONHISTORY$4 =
new javax.xml.namespace.QName("http://schemas.openehr.org/v1", "includes_revision_history");
private static final javax.xml.namespace.QName INCLUDESDATA$6 =
new javax.xml.namespace.QName("http://schemas.openehr.org/v1", "includes_data");
/**
* Gets the "include_all_versions" element
*/
public boolean getIncludeAllVersions()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_element_user(INCLUDEALLVERSIONS$0, 0);
if (target == null)
{
return false;
}
return target.getBooleanValue();
}
}
/**
* Gets (as xml) the "include_all_versions" element
*/
public org.apache.xmlbeans.XmlBoolean xgetIncludeAllVersions()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlBoolean target = null;
target = (org.apache.xmlbeans.XmlBoolean)get_store().find_element_user(INCLUDEALLVERSIONS$0, 0);
return target;
}
}
/**
* Sets the "include_all_versions" element
*/
public void setIncludeAllVersions(boolean includeAllVersions)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_element_user(INCLUDEALLVERSIONS$0, 0);
if (target == null)
{
target = (org.apache.xmlbeans.SimpleValue)get_store().add_element_user(INCLUDEALLVERSIONS$0);
}
target.setBooleanValue(includeAllVersions);
}
}
/**
* Sets (as xml) the "include_all_versions" element
*/
public void xsetIncludeAllVersions(org.apache.xmlbeans.XmlBoolean includeAllVersions)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlBoolean target = null;
target = (org.apache.xmlbeans.XmlBoolean)get_store().find_element_user(INCLUDEALLVERSIONS$0, 0);
if (target == null)
{
target = (org.apache.xmlbeans.XmlBoolean)get_store().add_element_user(INCLUDEALLVERSIONS$0);
}
target.set(includeAllVersions);
}
}
/**
* Gets the "commit_time_interval" element
*/
public org.openehr.schemas.v1.DVINTERVAL getCommitTimeInterval()
{
synchronized (monitor())
{
check_orphaned();
org.openehr.schemas.v1.DVINTERVAL target = null;
target = (org.openehr.schemas.v1.DVINTERVAL)get_store().find_element_user(COMMITTIMEINTERVAL$2, 0);
if (target == null)
{
return null;
}
return target;
}
}
/**
* True if has "commit_time_interval" element
*/
public boolean isSetCommitTimeInterval()
{
synchronized (monitor())
{
check_orphaned();
return get_store().count_elements(COMMITTIMEINTERVAL$2) != 0;
}
}
/**
* Sets the "commit_time_interval" element
*/
public void setCommitTimeInterval(org.openehr.schemas.v1.DVINTERVAL commitTimeInterval)
{
synchronized (monitor())
{
check_orphaned();
org.openehr.schemas.v1.DVINTERVAL target = null;
target = (org.openehr.schemas.v1.DVINTERVAL)get_store().find_element_user(COMMITTIMEINTERVAL$2, 0);
if (target == null)
{
target = (org.openehr.schemas.v1.DVINTERVAL)get_store().add_element_user(COMMITTIMEINTERVAL$2);
}
target.set(commitTimeInterval);
}
}
/**
* Appends and returns a new empty "commit_time_interval" element
*/
public org.openehr.schemas.v1.DVINTERVAL addNewCommitTimeInterval()
{
synchronized (monitor())
{
check_orphaned();
org.openehr.schemas.v1.DVINTERVAL target = null;
target = (org.openehr.schemas.v1.DVINTERVAL)get_store().add_element_user(COMMITTIMEINTERVAL$2);
return target;
}
}
/**
* Unsets the "commit_time_interval" element
*/
public void unsetCommitTimeInterval()
{
synchronized (monitor())
{
check_orphaned();
get_store().remove_element(COMMITTIMEINTERVAL$2, 0);
}
}
/**
* Gets the "includes_revision_history" element
*/
public boolean getIncludesRevisionHistory()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_element_user(INCLUDESREVISIONHISTORY$4, 0);
if (target == null)
{
return false;
}
return target.getBooleanValue();
}
}
/**
* Gets (as xml) the "includes_revision_history" element
*/
public org.apache.xmlbeans.XmlBoolean xgetIncludesRevisionHistory()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlBoolean target = null;
target = (org.apache.xmlbeans.XmlBoolean)get_store().find_element_user(INCLUDESREVISIONHISTORY$4, 0);
return target;
}
}
/**
* Sets the "includes_revision_history" element
*/
public void setIncludesRevisionHistory(boolean includesRevisionHistory)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_element_user(INCLUDESREVISIONHISTORY$4, 0);
if (target == null)
{
target = (org.apache.xmlbeans.SimpleValue)get_store().add_element_user(INCLUDESREVISIONHISTORY$4);
}
target.setBooleanValue(includesRevisionHistory);
}
}
/**
* Sets (as xml) the "includes_revision_history" element
*/
public void xsetIncludesRevisionHistory(org.apache.xmlbeans.XmlBoolean includesRevisionHistory)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlBoolean target = null;
target = (org.apache.xmlbeans.XmlBoolean)get_store().find_element_user(INCLUDESREVISIONHISTORY$4, 0);
if (target == null)
{
target = (org.apache.xmlbeans.XmlBoolean)get_store().add_element_user(INCLUDESREVISIONHISTORY$4);
}
target.set(includesRevisionHistory);
}
}
/**
* Gets the "includes_data" element
*/
public boolean getIncludesData()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_element_user(INCLUDESDATA$6, 0);
if (target == null)
{
return false;
}
return target.getBooleanValue();
}
}
/**
* Gets (as xml) the "includes_data" element
*/
public org.apache.xmlbeans.XmlBoolean xgetIncludesData()
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlBoolean target = null;
target = (org.apache.xmlbeans.XmlBoolean)get_store().find_element_user(INCLUDESDATA$6, 0);
return target;
}
}
/**
* Sets the "includes_data" element
*/
public void setIncludesData(boolean includesData)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.SimpleValue target = null;
target = (org.apache.xmlbeans.SimpleValue)get_store().find_element_user(INCLUDESDATA$6, 0);
if (target == null)
{
target = (org.apache.xmlbeans.SimpleValue)get_store().add_element_user(INCLUDESDATA$6);
}
target.setBooleanValue(includesData);
}
}
/**
* Sets (as xml) the "includes_data" element
*/
public void xsetIncludesData(org.apache.xmlbeans.XmlBoolean includesData)
{
synchronized (monitor())
{
check_orphaned();
org.apache.xmlbeans.XmlBoolean target = null;
target = (org.apache.xmlbeans.XmlBoolean)get_store().find_element_user(INCLUDESDATA$6, 0);
if (target == null)
{
target = (org.apache.xmlbeans.XmlBoolean)get_store().add_element_user(INCLUDESDATA$6);
}
target.set(includesData);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy