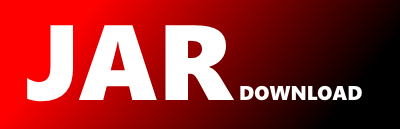
org.openestate.io.immoxml.xml.Objektart Maven / Gradle / Ivy
Show all versions of OpenEstate-IO-ImmoXML Show documentation
package org.openestate.io.immoxml.xml;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.List;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlElement;
import javax.xml.bind.annotation.XmlRootElement;
import javax.xml.bind.annotation.XmlType;
import org.jvnet.jaxb2_commons.lang.CopyStrategy2;
import org.jvnet.jaxb2_commons.lang.CopyTo2;
import org.jvnet.jaxb2_commons.lang.Equals2;
import org.jvnet.jaxb2_commons.lang.EqualsStrategy2;
import org.jvnet.jaxb2_commons.lang.JAXBCopyStrategy;
import org.jvnet.jaxb2_commons.lang.JAXBEqualsStrategy;
import org.jvnet.jaxb2_commons.lang.JAXBToStringStrategy;
import org.jvnet.jaxb2_commons.lang.ToString2;
import org.jvnet.jaxb2_commons.lang.ToStringStrategy2;
import org.jvnet.jaxb2_commons.locator.ObjectLocator;
import org.jvnet.jaxb2_commons.locator.util.LocatorUtils;
/**
* Java class for <objektart> element.
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "", propOrder = {
"zimmer",
"wohnung",
"haus",
"grundstueck",
"bueroPraxen",
"einzelhandel",
"gastgewerbe",
"hallenLagerProd",
"landUndForstwirtschaft",
"sonstige",
"freizeitimmobilieGewerblich",
"zinshausRenditeobjekt"
})
@XmlRootElement(name = "objektart")
public class Objektart implements Serializable, Cloneable, CopyTo2, Equals2, ToString2
{
protected List zimmer;
protected List wohnung;
protected List haus;
protected List grundstueck;
@XmlElement(name = "buero_praxen")
protected List bueroPraxen;
protected List einzelhandel;
protected List gastgewerbe;
@XmlElement(name = "hallen_lager_prod")
protected List hallenLagerProd;
@XmlElement(name = "land_und_forstwirtschaft")
protected List landUndForstwirtschaft;
protected List sonstige;
@XmlElement(name = "freizeitimmobilie_gewerblich")
protected List freizeitimmobilieGewerblich;
@XmlElement(name = "zinshaus_renditeobjekt")
protected List zinshausRenditeobjekt;
/**
* Gets the value of the zimmer property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the zimmer property.
*
*
* For example, to add a new item, do as follows:
*
* getZimmer().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Zimmer }
*
*
*/
public List getZimmer() {
if (zimmer == null) {
zimmer = new ArrayList();
}
return this.zimmer;
}
/**
* Gets the value of the wohnung property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the wohnung property.
*
*
* For example, to add a new item, do as follows:
*
* getWohnung().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Wohnung }
*
*
*/
public List getWohnung() {
if (wohnung == null) {
wohnung = new ArrayList();
}
return this.wohnung;
}
/**
* Gets the value of the haus property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the haus property.
*
*
* For example, to add a new item, do as follows:
*
* getHaus().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Haus }
*
*
*/
public List getHaus() {
if (haus == null) {
haus = new ArrayList();
}
return this.haus;
}
/**
* Gets the value of the grundstueck property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the grundstueck property.
*
*
* For example, to add a new item, do as follows:
*
* getGrundstueck().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Grundstueck }
*
*
*/
public List getGrundstueck() {
if (grundstueck == null) {
grundstueck = new ArrayList();
}
return this.grundstueck;
}
/**
* Gets the value of the bueroPraxen property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the bueroPraxen property.
*
*
* For example, to add a new item, do as follows:
*
* getBueroPraxen().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link BueroPraxen }
*
*
*/
public List getBueroPraxen() {
if (bueroPraxen == null) {
bueroPraxen = new ArrayList();
}
return this.bueroPraxen;
}
/**
* Gets the value of the einzelhandel property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the einzelhandel property.
*
*
* For example, to add a new item, do as follows:
*
* getEinzelhandel().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Einzelhandel }
*
*
*/
public List getEinzelhandel() {
if (einzelhandel == null) {
einzelhandel = new ArrayList();
}
return this.einzelhandel;
}
/**
* Gets the value of the gastgewerbe property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the gastgewerbe property.
*
*
* For example, to add a new item, do as follows:
*
* getGastgewerbe().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Gastgewerbe }
*
*
*/
public List getGastgewerbe() {
if (gastgewerbe == null) {
gastgewerbe = new ArrayList();
}
return this.gastgewerbe;
}
/**
* Gets the value of the hallenLagerProd property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the hallenLagerProd property.
*
*
* For example, to add a new item, do as follows:
*
* getHallenLagerProd().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link HallenLagerProd }
*
*
*/
public List getHallenLagerProd() {
if (hallenLagerProd == null) {
hallenLagerProd = new ArrayList();
}
return this.hallenLagerProd;
}
/**
* Gets the value of the landUndForstwirtschaft property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the landUndForstwirtschaft property.
*
*
* For example, to add a new item, do as follows:
*
* getLandUndForstwirtschaft().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link LandUndForstwirtschaft }
*
*
*/
public List getLandUndForstwirtschaft() {
if (landUndForstwirtschaft == null) {
landUndForstwirtschaft = new ArrayList();
}
return this.landUndForstwirtschaft;
}
/**
* Gets the value of the sonstige property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the sonstige property.
*
*
* For example, to add a new item, do as follows:
*
* getSonstige().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link Sonstige }
*
*
*/
public List getSonstige() {
if (sonstige == null) {
sonstige = new ArrayList();
}
return this.sonstige;
}
/**
* Gets the value of the freizeitimmobilieGewerblich property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the freizeitimmobilieGewerblich property.
*
*
* For example, to add a new item, do as follows:
*
* getFreizeitimmobilieGewerblich().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link FreizeitimmobilieGewerblich }
*
*
*/
public List getFreizeitimmobilieGewerblich() {
if (freizeitimmobilieGewerblich == null) {
freizeitimmobilieGewerblich = new ArrayList();
}
return this.freizeitimmobilieGewerblich;
}
/**
* Gets the value of the zinshausRenditeobjekt property.
*
*
* This accessor method returns a reference to the live list,
* not a snapshot. Therefore any modification you make to the
* returned list will be present inside the JAXB object.
* This is why there is not a set
method for the zinshausRenditeobjekt property.
*
*
* For example, to add a new item, do as follows:
*
* getZinshausRenditeobjekt().add(newItem);
*
*
*
*
* Objects of the following type(s) are allowed in the list
* {@link ZinshausRenditeobjekt }
*
*
*/
public List getZinshausRenditeobjekt() {
if (zinshausRenditeobjekt == null) {
zinshausRenditeobjekt = new ArrayList();
}
return this.zinshausRenditeobjekt;
}
public String toString() {
final ToStringStrategy2 strategy = JAXBToStringStrategy.INSTANCE;
final StringBuilder buffer = new StringBuilder();
append(null, buffer, strategy);
return buffer.toString();
}
public StringBuilder append(ObjectLocator locator, StringBuilder buffer, ToStringStrategy2 strategy) {
strategy.appendStart(locator, this, buffer);
appendFields(locator, buffer, strategy);
strategy.appendEnd(locator, this, buffer);
return buffer;
}
public StringBuilder appendFields(ObjectLocator locator, StringBuilder buffer, ToStringStrategy2 strategy) {
{
List theZimmer;
theZimmer = (((this.zimmer!= null)&&(!this.zimmer.isEmpty()))?this.getZimmer():null);
strategy.appendField(locator, this, "zimmer", buffer, theZimmer, ((this.zimmer!= null)&&(!this.zimmer.isEmpty())));
}
{
List theWohnung;
theWohnung = (((this.wohnung!= null)&&(!this.wohnung.isEmpty()))?this.getWohnung():null);
strategy.appendField(locator, this, "wohnung", buffer, theWohnung, ((this.wohnung!= null)&&(!this.wohnung.isEmpty())));
}
{
List theHaus;
theHaus = (((this.haus!= null)&&(!this.haus.isEmpty()))?this.getHaus():null);
strategy.appendField(locator, this, "haus", buffer, theHaus, ((this.haus!= null)&&(!this.haus.isEmpty())));
}
{
List theGrundstueck;
theGrundstueck = (((this.grundstueck!= null)&&(!this.grundstueck.isEmpty()))?this.getGrundstueck():null);
strategy.appendField(locator, this, "grundstueck", buffer, theGrundstueck, ((this.grundstueck!= null)&&(!this.grundstueck.isEmpty())));
}
{
List theBueroPraxen;
theBueroPraxen = (((this.bueroPraxen!= null)&&(!this.bueroPraxen.isEmpty()))?this.getBueroPraxen():null);
strategy.appendField(locator, this, "bueroPraxen", buffer, theBueroPraxen, ((this.bueroPraxen!= null)&&(!this.bueroPraxen.isEmpty())));
}
{
List theEinzelhandel;
theEinzelhandel = (((this.einzelhandel!= null)&&(!this.einzelhandel.isEmpty()))?this.getEinzelhandel():null);
strategy.appendField(locator, this, "einzelhandel", buffer, theEinzelhandel, ((this.einzelhandel!= null)&&(!this.einzelhandel.isEmpty())));
}
{
List theGastgewerbe;
theGastgewerbe = (((this.gastgewerbe!= null)&&(!this.gastgewerbe.isEmpty()))?this.getGastgewerbe():null);
strategy.appendField(locator, this, "gastgewerbe", buffer, theGastgewerbe, ((this.gastgewerbe!= null)&&(!this.gastgewerbe.isEmpty())));
}
{
List theHallenLagerProd;
theHallenLagerProd = (((this.hallenLagerProd!= null)&&(!this.hallenLagerProd.isEmpty()))?this.getHallenLagerProd():null);
strategy.appendField(locator, this, "hallenLagerProd", buffer, theHallenLagerProd, ((this.hallenLagerProd!= null)&&(!this.hallenLagerProd.isEmpty())));
}
{
List theLandUndForstwirtschaft;
theLandUndForstwirtschaft = (((this.landUndForstwirtschaft!= null)&&(!this.landUndForstwirtschaft.isEmpty()))?this.getLandUndForstwirtschaft():null);
strategy.appendField(locator, this, "landUndForstwirtschaft", buffer, theLandUndForstwirtschaft, ((this.landUndForstwirtschaft!= null)&&(!this.landUndForstwirtschaft.isEmpty())));
}
{
List theSonstige;
theSonstige = (((this.sonstige!= null)&&(!this.sonstige.isEmpty()))?this.getSonstige():null);
strategy.appendField(locator, this, "sonstige", buffer, theSonstige, ((this.sonstige!= null)&&(!this.sonstige.isEmpty())));
}
{
List theFreizeitimmobilieGewerblich;
theFreizeitimmobilieGewerblich = (((this.freizeitimmobilieGewerblich!= null)&&(!this.freizeitimmobilieGewerblich.isEmpty()))?this.getFreizeitimmobilieGewerblich():null);
strategy.appendField(locator, this, "freizeitimmobilieGewerblich", buffer, theFreizeitimmobilieGewerblich, ((this.freizeitimmobilieGewerblich!= null)&&(!this.freizeitimmobilieGewerblich.isEmpty())));
}
{
List theZinshausRenditeobjekt;
theZinshausRenditeobjekt = (((this.zinshausRenditeobjekt!= null)&&(!this.zinshausRenditeobjekt.isEmpty()))?this.getZinshausRenditeobjekt():null);
strategy.appendField(locator, this, "zinshausRenditeobjekt", buffer, theZinshausRenditeobjekt, ((this.zinshausRenditeobjekt!= null)&&(!this.zinshausRenditeobjekt.isEmpty())));
}
return buffer;
}
public Object clone() {
return copyTo(createNewInstance());
}
public Object copyTo(Object target) {
final CopyStrategy2 strategy = JAXBCopyStrategy.INSTANCE;
return copyTo(null, target, strategy);
}
public Object copyTo(ObjectLocator locator, Object target, CopyStrategy2 strategy) {
final Object draftCopy = ((target == null)?createNewInstance():target);
if (draftCopy instanceof Objektart) {
final Objektart copy = ((Objektart) draftCopy);
{
Boolean zimmerShouldBeCopiedAndSet = strategy.shouldBeCopiedAndSet(locator, ((this.zimmer!= null)&&(!this.zimmer.isEmpty())));
if (zimmerShouldBeCopiedAndSet == Boolean.TRUE) {
List sourceZimmer;
sourceZimmer = (((this.zimmer!= null)&&(!this.zimmer.isEmpty()))?this.getZimmer():null);
@SuppressWarnings("unchecked")
List copyZimmer = ((List ) strategy.copy(LocatorUtils.property(locator, "zimmer", sourceZimmer), sourceZimmer, ((this.zimmer!= null)&&(!this.zimmer.isEmpty()))));
copy.zimmer = null;
if (copyZimmer!= null) {
List uniqueZimmerl = copy.getZimmer();
uniqueZimmerl.addAll(copyZimmer);
}
} else {
if (zimmerShouldBeCopiedAndSet == Boolean.FALSE) {
copy.zimmer = null;
}
}
}
{
Boolean wohnungShouldBeCopiedAndSet = strategy.shouldBeCopiedAndSet(locator, ((this.wohnung!= null)&&(!this.wohnung.isEmpty())));
if (wohnungShouldBeCopiedAndSet == Boolean.TRUE) {
List sourceWohnung;
sourceWohnung = (((this.wohnung!= null)&&(!this.wohnung.isEmpty()))?this.getWohnung():null);
@SuppressWarnings("unchecked")
List copyWohnung = ((List ) strategy.copy(LocatorUtils.property(locator, "wohnung", sourceWohnung), sourceWohnung, ((this.wohnung!= null)&&(!this.wohnung.isEmpty()))));
copy.wohnung = null;
if (copyWohnung!= null) {
List uniqueWohnungl = copy.getWohnung();
uniqueWohnungl.addAll(copyWohnung);
}
} else {
if (wohnungShouldBeCopiedAndSet == Boolean.FALSE) {
copy.wohnung = null;
}
}
}
{
Boolean hausShouldBeCopiedAndSet = strategy.shouldBeCopiedAndSet(locator, ((this.haus!= null)&&(!this.haus.isEmpty())));
if (hausShouldBeCopiedAndSet == Boolean.TRUE) {
List sourceHaus;
sourceHaus = (((this.haus!= null)&&(!this.haus.isEmpty()))?this.getHaus():null);
@SuppressWarnings("unchecked")
List copyHaus = ((List ) strategy.copy(LocatorUtils.property(locator, "haus", sourceHaus), sourceHaus, ((this.haus!= null)&&(!this.haus.isEmpty()))));
copy.haus = null;
if (copyHaus!= null) {
List uniqueHausl = copy.getHaus();
uniqueHausl.addAll(copyHaus);
}
} else {
if (hausShouldBeCopiedAndSet == Boolean.FALSE) {
copy.haus = null;
}
}
}
{
Boolean grundstueckShouldBeCopiedAndSet = strategy.shouldBeCopiedAndSet(locator, ((this.grundstueck!= null)&&(!this.grundstueck.isEmpty())));
if (grundstueckShouldBeCopiedAndSet == Boolean.TRUE) {
List sourceGrundstueck;
sourceGrundstueck = (((this.grundstueck!= null)&&(!this.grundstueck.isEmpty()))?this.getGrundstueck():null);
@SuppressWarnings("unchecked")
List copyGrundstueck = ((List ) strategy.copy(LocatorUtils.property(locator, "grundstueck", sourceGrundstueck), sourceGrundstueck, ((this.grundstueck!= null)&&(!this.grundstueck.isEmpty()))));
copy.grundstueck = null;
if (copyGrundstueck!= null) {
List uniqueGrundstueckl = copy.getGrundstueck();
uniqueGrundstueckl.addAll(copyGrundstueck);
}
} else {
if (grundstueckShouldBeCopiedAndSet == Boolean.FALSE) {
copy.grundstueck = null;
}
}
}
{
Boolean bueroPraxenShouldBeCopiedAndSet = strategy.shouldBeCopiedAndSet(locator, ((this.bueroPraxen!= null)&&(!this.bueroPraxen.isEmpty())));
if (bueroPraxenShouldBeCopiedAndSet == Boolean.TRUE) {
List sourceBueroPraxen;
sourceBueroPraxen = (((this.bueroPraxen!= null)&&(!this.bueroPraxen.isEmpty()))?this.getBueroPraxen():null);
@SuppressWarnings("unchecked")
List copyBueroPraxen = ((List ) strategy.copy(LocatorUtils.property(locator, "bueroPraxen", sourceBueroPraxen), sourceBueroPraxen, ((this.bueroPraxen!= null)&&(!this.bueroPraxen.isEmpty()))));
copy.bueroPraxen = null;
if (copyBueroPraxen!= null) {
List uniqueBueroPraxenl = copy.getBueroPraxen();
uniqueBueroPraxenl.addAll(copyBueroPraxen);
}
} else {
if (bueroPraxenShouldBeCopiedAndSet == Boolean.FALSE) {
copy.bueroPraxen = null;
}
}
}
{
Boolean einzelhandelShouldBeCopiedAndSet = strategy.shouldBeCopiedAndSet(locator, ((this.einzelhandel!= null)&&(!this.einzelhandel.isEmpty())));
if (einzelhandelShouldBeCopiedAndSet == Boolean.TRUE) {
List sourceEinzelhandel;
sourceEinzelhandel = (((this.einzelhandel!= null)&&(!this.einzelhandel.isEmpty()))?this.getEinzelhandel():null);
@SuppressWarnings("unchecked")
List copyEinzelhandel = ((List ) strategy.copy(LocatorUtils.property(locator, "einzelhandel", sourceEinzelhandel), sourceEinzelhandel, ((this.einzelhandel!= null)&&(!this.einzelhandel.isEmpty()))));
copy.einzelhandel = null;
if (copyEinzelhandel!= null) {
List uniqueEinzelhandell = copy.getEinzelhandel();
uniqueEinzelhandell.addAll(copyEinzelhandel);
}
} else {
if (einzelhandelShouldBeCopiedAndSet == Boolean.FALSE) {
copy.einzelhandel = null;
}
}
}
{
Boolean gastgewerbeShouldBeCopiedAndSet = strategy.shouldBeCopiedAndSet(locator, ((this.gastgewerbe!= null)&&(!this.gastgewerbe.isEmpty())));
if (gastgewerbeShouldBeCopiedAndSet == Boolean.TRUE) {
List sourceGastgewerbe;
sourceGastgewerbe = (((this.gastgewerbe!= null)&&(!this.gastgewerbe.isEmpty()))?this.getGastgewerbe():null);
@SuppressWarnings("unchecked")
List copyGastgewerbe = ((List ) strategy.copy(LocatorUtils.property(locator, "gastgewerbe", sourceGastgewerbe), sourceGastgewerbe, ((this.gastgewerbe!= null)&&(!this.gastgewerbe.isEmpty()))));
copy.gastgewerbe = null;
if (copyGastgewerbe!= null) {
List uniqueGastgewerbel = copy.getGastgewerbe();
uniqueGastgewerbel.addAll(copyGastgewerbe);
}
} else {
if (gastgewerbeShouldBeCopiedAndSet == Boolean.FALSE) {
copy.gastgewerbe = null;
}
}
}
{
Boolean hallenLagerProdShouldBeCopiedAndSet = strategy.shouldBeCopiedAndSet(locator, ((this.hallenLagerProd!= null)&&(!this.hallenLagerProd.isEmpty())));
if (hallenLagerProdShouldBeCopiedAndSet == Boolean.TRUE) {
List sourceHallenLagerProd;
sourceHallenLagerProd = (((this.hallenLagerProd!= null)&&(!this.hallenLagerProd.isEmpty()))?this.getHallenLagerProd():null);
@SuppressWarnings("unchecked")
List copyHallenLagerProd = ((List ) strategy.copy(LocatorUtils.property(locator, "hallenLagerProd", sourceHallenLagerProd), sourceHallenLagerProd, ((this.hallenLagerProd!= null)&&(!this.hallenLagerProd.isEmpty()))));
copy.hallenLagerProd = null;
if (copyHallenLagerProd!= null) {
List uniqueHallenLagerProdl = copy.getHallenLagerProd();
uniqueHallenLagerProdl.addAll(copyHallenLagerProd);
}
} else {
if (hallenLagerProdShouldBeCopiedAndSet == Boolean.FALSE) {
copy.hallenLagerProd = null;
}
}
}
{
Boolean landUndForstwirtschaftShouldBeCopiedAndSet = strategy.shouldBeCopiedAndSet(locator, ((this.landUndForstwirtschaft!= null)&&(!this.landUndForstwirtschaft.isEmpty())));
if (landUndForstwirtschaftShouldBeCopiedAndSet == Boolean.TRUE) {
List sourceLandUndForstwirtschaft;
sourceLandUndForstwirtschaft = (((this.landUndForstwirtschaft!= null)&&(!this.landUndForstwirtschaft.isEmpty()))?this.getLandUndForstwirtschaft():null);
@SuppressWarnings("unchecked")
List copyLandUndForstwirtschaft = ((List ) strategy.copy(LocatorUtils.property(locator, "landUndForstwirtschaft", sourceLandUndForstwirtschaft), sourceLandUndForstwirtschaft, ((this.landUndForstwirtschaft!= null)&&(!this.landUndForstwirtschaft.isEmpty()))));
copy.landUndForstwirtschaft = null;
if (copyLandUndForstwirtschaft!= null) {
List uniqueLandUndForstwirtschaftl = copy.getLandUndForstwirtschaft();
uniqueLandUndForstwirtschaftl.addAll(copyLandUndForstwirtschaft);
}
} else {
if (landUndForstwirtschaftShouldBeCopiedAndSet == Boolean.FALSE) {
copy.landUndForstwirtschaft = null;
}
}
}
{
Boolean sonstigeShouldBeCopiedAndSet = strategy.shouldBeCopiedAndSet(locator, ((this.sonstige!= null)&&(!this.sonstige.isEmpty())));
if (sonstigeShouldBeCopiedAndSet == Boolean.TRUE) {
List sourceSonstige;
sourceSonstige = (((this.sonstige!= null)&&(!this.sonstige.isEmpty()))?this.getSonstige():null);
@SuppressWarnings("unchecked")
List copySonstige = ((List ) strategy.copy(LocatorUtils.property(locator, "sonstige", sourceSonstige), sourceSonstige, ((this.sonstige!= null)&&(!this.sonstige.isEmpty()))));
copy.sonstige = null;
if (copySonstige!= null) {
List uniqueSonstigel = copy.getSonstige();
uniqueSonstigel.addAll(copySonstige);
}
} else {
if (sonstigeShouldBeCopiedAndSet == Boolean.FALSE) {
copy.sonstige = null;
}
}
}
{
Boolean freizeitimmobilieGewerblichShouldBeCopiedAndSet = strategy.shouldBeCopiedAndSet(locator, ((this.freizeitimmobilieGewerblich!= null)&&(!this.freizeitimmobilieGewerblich.isEmpty())));
if (freizeitimmobilieGewerblichShouldBeCopiedAndSet == Boolean.TRUE) {
List sourceFreizeitimmobilieGewerblich;
sourceFreizeitimmobilieGewerblich = (((this.freizeitimmobilieGewerblich!= null)&&(!this.freizeitimmobilieGewerblich.isEmpty()))?this.getFreizeitimmobilieGewerblich():null);
@SuppressWarnings("unchecked")
List copyFreizeitimmobilieGewerblich = ((List ) strategy.copy(LocatorUtils.property(locator, "freizeitimmobilieGewerblich", sourceFreizeitimmobilieGewerblich), sourceFreizeitimmobilieGewerblich, ((this.freizeitimmobilieGewerblich!= null)&&(!this.freizeitimmobilieGewerblich.isEmpty()))));
copy.freizeitimmobilieGewerblich = null;
if (copyFreizeitimmobilieGewerblich!= null) {
List uniqueFreizeitimmobilieGewerblichl = copy.getFreizeitimmobilieGewerblich();
uniqueFreizeitimmobilieGewerblichl.addAll(copyFreizeitimmobilieGewerblich);
}
} else {
if (freizeitimmobilieGewerblichShouldBeCopiedAndSet == Boolean.FALSE) {
copy.freizeitimmobilieGewerblich = null;
}
}
}
{
Boolean zinshausRenditeobjektShouldBeCopiedAndSet = strategy.shouldBeCopiedAndSet(locator, ((this.zinshausRenditeobjekt!= null)&&(!this.zinshausRenditeobjekt.isEmpty())));
if (zinshausRenditeobjektShouldBeCopiedAndSet == Boolean.TRUE) {
List sourceZinshausRenditeobjekt;
sourceZinshausRenditeobjekt = (((this.zinshausRenditeobjekt!= null)&&(!this.zinshausRenditeobjekt.isEmpty()))?this.getZinshausRenditeobjekt():null);
@SuppressWarnings("unchecked")
List copyZinshausRenditeobjekt = ((List ) strategy.copy(LocatorUtils.property(locator, "zinshausRenditeobjekt", sourceZinshausRenditeobjekt), sourceZinshausRenditeobjekt, ((this.zinshausRenditeobjekt!= null)&&(!this.zinshausRenditeobjekt.isEmpty()))));
copy.zinshausRenditeobjekt = null;
if (copyZinshausRenditeobjekt!= null) {
List uniqueZinshausRenditeobjektl = copy.getZinshausRenditeobjekt();
uniqueZinshausRenditeobjektl.addAll(copyZinshausRenditeobjekt);
}
} else {
if (zinshausRenditeobjektShouldBeCopiedAndSet == Boolean.FALSE) {
copy.zinshausRenditeobjekt = null;
}
}
}
}
return draftCopy;
}
public Object createNewInstance() {
return new Objektart();
}
public boolean equals(ObjectLocator thisLocator, ObjectLocator thatLocator, Object object, EqualsStrategy2 strategy) {
if ((object == null)||(this.getClass()!= object.getClass())) {
return false;
}
if (this == object) {
return true;
}
final Objektart that = ((Objektart) object);
{
List lhsZimmer;
lhsZimmer = (((this.zimmer!= null)&&(!this.zimmer.isEmpty()))?this.getZimmer():null);
List rhsZimmer;
rhsZimmer = (((that.zimmer!= null)&&(!that.zimmer.isEmpty()))?that.getZimmer():null);
if (!strategy.equals(LocatorUtils.property(thisLocator, "zimmer", lhsZimmer), LocatorUtils.property(thatLocator, "zimmer", rhsZimmer), lhsZimmer, rhsZimmer, ((this.zimmer!= null)&&(!this.zimmer.isEmpty())), ((that.zimmer!= null)&&(!that.zimmer.isEmpty())))) {
return false;
}
}
{
List lhsWohnung;
lhsWohnung = (((this.wohnung!= null)&&(!this.wohnung.isEmpty()))?this.getWohnung():null);
List rhsWohnung;
rhsWohnung = (((that.wohnung!= null)&&(!that.wohnung.isEmpty()))?that.getWohnung():null);
if (!strategy.equals(LocatorUtils.property(thisLocator, "wohnung", lhsWohnung), LocatorUtils.property(thatLocator, "wohnung", rhsWohnung), lhsWohnung, rhsWohnung, ((this.wohnung!= null)&&(!this.wohnung.isEmpty())), ((that.wohnung!= null)&&(!that.wohnung.isEmpty())))) {
return false;
}
}
{
List lhsHaus;
lhsHaus = (((this.haus!= null)&&(!this.haus.isEmpty()))?this.getHaus():null);
List rhsHaus;
rhsHaus = (((that.haus!= null)&&(!that.haus.isEmpty()))?that.getHaus():null);
if (!strategy.equals(LocatorUtils.property(thisLocator, "haus", lhsHaus), LocatorUtils.property(thatLocator, "haus", rhsHaus), lhsHaus, rhsHaus, ((this.haus!= null)&&(!this.haus.isEmpty())), ((that.haus!= null)&&(!that.haus.isEmpty())))) {
return false;
}
}
{
List lhsGrundstueck;
lhsGrundstueck = (((this.grundstueck!= null)&&(!this.grundstueck.isEmpty()))?this.getGrundstueck():null);
List rhsGrundstueck;
rhsGrundstueck = (((that.grundstueck!= null)&&(!that.grundstueck.isEmpty()))?that.getGrundstueck():null);
if (!strategy.equals(LocatorUtils.property(thisLocator, "grundstueck", lhsGrundstueck), LocatorUtils.property(thatLocator, "grundstueck", rhsGrundstueck), lhsGrundstueck, rhsGrundstueck, ((this.grundstueck!= null)&&(!this.grundstueck.isEmpty())), ((that.grundstueck!= null)&&(!that.grundstueck.isEmpty())))) {
return false;
}
}
{
List lhsBueroPraxen;
lhsBueroPraxen = (((this.bueroPraxen!= null)&&(!this.bueroPraxen.isEmpty()))?this.getBueroPraxen():null);
List rhsBueroPraxen;
rhsBueroPraxen = (((that.bueroPraxen!= null)&&(!that.bueroPraxen.isEmpty()))?that.getBueroPraxen():null);
if (!strategy.equals(LocatorUtils.property(thisLocator, "bueroPraxen", lhsBueroPraxen), LocatorUtils.property(thatLocator, "bueroPraxen", rhsBueroPraxen), lhsBueroPraxen, rhsBueroPraxen, ((this.bueroPraxen!= null)&&(!this.bueroPraxen.isEmpty())), ((that.bueroPraxen!= null)&&(!that.bueroPraxen.isEmpty())))) {
return false;
}
}
{
List lhsEinzelhandel;
lhsEinzelhandel = (((this.einzelhandel!= null)&&(!this.einzelhandel.isEmpty()))?this.getEinzelhandel():null);
List rhsEinzelhandel;
rhsEinzelhandel = (((that.einzelhandel!= null)&&(!that.einzelhandel.isEmpty()))?that.getEinzelhandel():null);
if (!strategy.equals(LocatorUtils.property(thisLocator, "einzelhandel", lhsEinzelhandel), LocatorUtils.property(thatLocator, "einzelhandel", rhsEinzelhandel), lhsEinzelhandel, rhsEinzelhandel, ((this.einzelhandel!= null)&&(!this.einzelhandel.isEmpty())), ((that.einzelhandel!= null)&&(!that.einzelhandel.isEmpty())))) {
return false;
}
}
{
List lhsGastgewerbe;
lhsGastgewerbe = (((this.gastgewerbe!= null)&&(!this.gastgewerbe.isEmpty()))?this.getGastgewerbe():null);
List rhsGastgewerbe;
rhsGastgewerbe = (((that.gastgewerbe!= null)&&(!that.gastgewerbe.isEmpty()))?that.getGastgewerbe():null);
if (!strategy.equals(LocatorUtils.property(thisLocator, "gastgewerbe", lhsGastgewerbe), LocatorUtils.property(thatLocator, "gastgewerbe", rhsGastgewerbe), lhsGastgewerbe, rhsGastgewerbe, ((this.gastgewerbe!= null)&&(!this.gastgewerbe.isEmpty())), ((that.gastgewerbe!= null)&&(!that.gastgewerbe.isEmpty())))) {
return false;
}
}
{
List lhsHallenLagerProd;
lhsHallenLagerProd = (((this.hallenLagerProd!= null)&&(!this.hallenLagerProd.isEmpty()))?this.getHallenLagerProd():null);
List rhsHallenLagerProd;
rhsHallenLagerProd = (((that.hallenLagerProd!= null)&&(!that.hallenLagerProd.isEmpty()))?that.getHallenLagerProd():null);
if (!strategy.equals(LocatorUtils.property(thisLocator, "hallenLagerProd", lhsHallenLagerProd), LocatorUtils.property(thatLocator, "hallenLagerProd", rhsHallenLagerProd), lhsHallenLagerProd, rhsHallenLagerProd, ((this.hallenLagerProd!= null)&&(!this.hallenLagerProd.isEmpty())), ((that.hallenLagerProd!= null)&&(!that.hallenLagerProd.isEmpty())))) {
return false;
}
}
{
List lhsLandUndForstwirtschaft;
lhsLandUndForstwirtschaft = (((this.landUndForstwirtschaft!= null)&&(!this.landUndForstwirtschaft.isEmpty()))?this.getLandUndForstwirtschaft():null);
List rhsLandUndForstwirtschaft;
rhsLandUndForstwirtschaft = (((that.landUndForstwirtschaft!= null)&&(!that.landUndForstwirtschaft.isEmpty()))?that.getLandUndForstwirtschaft():null);
if (!strategy.equals(LocatorUtils.property(thisLocator, "landUndForstwirtschaft", lhsLandUndForstwirtschaft), LocatorUtils.property(thatLocator, "landUndForstwirtschaft", rhsLandUndForstwirtschaft), lhsLandUndForstwirtschaft, rhsLandUndForstwirtschaft, ((this.landUndForstwirtschaft!= null)&&(!this.landUndForstwirtschaft.isEmpty())), ((that.landUndForstwirtschaft!= null)&&(!that.landUndForstwirtschaft.isEmpty())))) {
return false;
}
}
{
List lhsSonstige;
lhsSonstige = (((this.sonstige!= null)&&(!this.sonstige.isEmpty()))?this.getSonstige():null);
List rhsSonstige;
rhsSonstige = (((that.sonstige!= null)&&(!that.sonstige.isEmpty()))?that.getSonstige():null);
if (!strategy.equals(LocatorUtils.property(thisLocator, "sonstige", lhsSonstige), LocatorUtils.property(thatLocator, "sonstige", rhsSonstige), lhsSonstige, rhsSonstige, ((this.sonstige!= null)&&(!this.sonstige.isEmpty())), ((that.sonstige!= null)&&(!that.sonstige.isEmpty())))) {
return false;
}
}
{
List lhsFreizeitimmobilieGewerblich;
lhsFreizeitimmobilieGewerblich = (((this.freizeitimmobilieGewerblich!= null)&&(!this.freizeitimmobilieGewerblich.isEmpty()))?this.getFreizeitimmobilieGewerblich():null);
List rhsFreizeitimmobilieGewerblich;
rhsFreizeitimmobilieGewerblich = (((that.freizeitimmobilieGewerblich!= null)&&(!that.freizeitimmobilieGewerblich.isEmpty()))?that.getFreizeitimmobilieGewerblich():null);
if (!strategy.equals(LocatorUtils.property(thisLocator, "freizeitimmobilieGewerblich", lhsFreizeitimmobilieGewerblich), LocatorUtils.property(thatLocator, "freizeitimmobilieGewerblich", rhsFreizeitimmobilieGewerblich), lhsFreizeitimmobilieGewerblich, rhsFreizeitimmobilieGewerblich, ((this.freizeitimmobilieGewerblich!= null)&&(!this.freizeitimmobilieGewerblich.isEmpty())), ((that.freizeitimmobilieGewerblich!= null)&&(!that.freizeitimmobilieGewerblich.isEmpty())))) {
return false;
}
}
{
List lhsZinshausRenditeobjekt;
lhsZinshausRenditeobjekt = (((this.zinshausRenditeobjekt!= null)&&(!this.zinshausRenditeobjekt.isEmpty()))?this.getZinshausRenditeobjekt():null);
List rhsZinshausRenditeobjekt;
rhsZinshausRenditeobjekt = (((that.zinshausRenditeobjekt!= null)&&(!that.zinshausRenditeobjekt.isEmpty()))?that.getZinshausRenditeobjekt():null);
if (!strategy.equals(LocatorUtils.property(thisLocator, "zinshausRenditeobjekt", lhsZinshausRenditeobjekt), LocatorUtils.property(thatLocator, "zinshausRenditeobjekt", rhsZinshausRenditeobjekt), lhsZinshausRenditeobjekt, rhsZinshausRenditeobjekt, ((this.zinshausRenditeobjekt!= null)&&(!this.zinshausRenditeobjekt.isEmpty())), ((that.zinshausRenditeobjekt!= null)&&(!that.zinshausRenditeobjekt.isEmpty())))) {
return false;
}
}
return true;
}
public boolean equals(Object object) {
final EqualsStrategy2 strategy = JAXBEqualsStrategy.INSTANCE;
return equals(null, null, object, strategy);
}
}