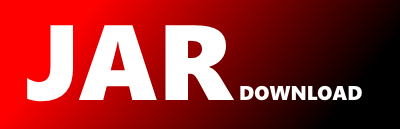
org.openestate.is24.restapi.xml.common.Attachment Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of OpenEstate-IS24-REST Show documentation
Show all versions of OpenEstate-IS24-REST Show documentation
OpenEstate-IS24-REST is a client library for the REST-Webservice of ImmobilienScout24.de written in Java.
package org.openestate.is24.restapi.xml.common;
import java.io.Serializable;
import java.net.URL;
import java.util.Calendar;
import javax.xml.bind.annotation.XmlAccessType;
import javax.xml.bind.annotation.XmlAccessorType;
import javax.xml.bind.annotation.XmlAttribute;
import javax.xml.bind.annotation.XmlSchemaType;
import javax.xml.bind.annotation.XmlSeeAlso;
import javax.xml.bind.annotation.XmlType;
import javax.xml.bind.annotation.adapters.XmlJavaTypeAdapter;
import org.jvnet.jaxb2_commons.lang.CopyStrategy2;
import org.jvnet.jaxb2_commons.lang.CopyTo2;
import org.jvnet.jaxb2_commons.lang.Equals2;
import org.jvnet.jaxb2_commons.lang.EqualsStrategy2;
import org.jvnet.jaxb2_commons.lang.JAXBCopyStrategy;
import org.jvnet.jaxb2_commons.lang.JAXBEqualsStrategy;
import org.jvnet.jaxb2_commons.lang.JAXBToStringStrategy;
import org.jvnet.jaxb2_commons.lang.ToString2;
import org.jvnet.jaxb2_commons.lang.ToStringStrategy2;
import org.jvnet.jaxb2_commons.locator.ObjectLocator;
import org.jvnet.jaxb2_commons.locator.util.LocatorUtils;
import org.openestate.is24.restapi.xml.Adapter2;
import org.openestate.is24.restapi.xml.Adapter4;
/**
* Ein Anhang
*
* Java class for Attachment complex type.
*
*
The following schema fragment specifies the expected content contained within this class.
*
*
* <complexType name="Attachment">
* <complexContent>
* <restriction base="{http://www.w3.org/2001/XMLSchema}anyType">
* <sequence>
* <element name="title" minOccurs="0">
* <simpleType>
* <restriction base="{http://rest.immobilienscout24.de/schema/common/1.0}TextField">
* <maxLength value="30"/>
* </restriction>
* </simpleType>
* </element>
* <element name="checkSum" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* <element name="externalId" minOccurs="0">
* <simpleType>
* <restriction base="{http://rest.immobilienscout24.de/schema/common/1.0}TextField">
* <maxLength value="50"/>
* </restriction>
* </simpleType>
* </element>
* <element name="externalCheckSum" type="{http://www.w3.org/2001/XMLSchema}string" minOccurs="0"/>
* </sequence>
* <attGroup ref="{http://rest.immobilienscout24.de/schema/common/1.0}Reference"/>
* </restriction>
* </complexContent>
* </complexType>
*
*
*
*/
@XmlAccessorType(XmlAccessType.FIELD)
@XmlType(name = "Attachment", propOrder = {
"title",
"checkSum",
"externalId",
"externalCheckSum"
})
@XmlSeeAlso({
Picture.class,
StreamingVideo.class,
ExtendedAttachment.class,
Link.class
})
public abstract class Attachment implements Serializable, Cloneable, CopyTo2, Equals2, ToString2
{
@XmlJavaTypeAdapter(Adapter34 .class)
protected String title;
protected String checkSum;
@XmlJavaTypeAdapter(Adapter35 .class)
protected String externalId;
protected String externalCheckSum;
@XmlAttribute(name = "href", namespace = "http://www.w3.org/1999/xlink")
@XmlJavaTypeAdapter(Adapter4 .class)
@XmlSchemaType(name = "anyURI")
protected URL href;
@XmlAttribute(name = "id")
protected Long id;
@XmlAttribute(name = "label")
protected String label;
@XmlAttribute(name = "modification")
@XmlJavaTypeAdapter(Adapter2 .class)
@XmlSchemaType(name = "dateTime")
protected Calendar modification;
@XmlAttribute(name = "creation")
@XmlJavaTypeAdapter(Adapter2 .class)
@XmlSchemaType(name = "dateTime")
protected Calendar creation;
@XmlAttribute(name = "publishDate")
@XmlJavaTypeAdapter(Adapter2 .class)
@XmlSchemaType(name = "dateTime")
protected Calendar publishDate;
/**
* Gets the value of the title property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getTitle() {
return title;
}
/**
* Sets the value of the title property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setTitle(String value) {
this.title = value;
}
/**
* Gets the value of the checkSum property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getCheckSum() {
return checkSum;
}
/**
* Sets the value of the checkSum property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setCheckSum(String value) {
this.checkSum = value;
}
/**
* Gets the value of the externalId property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getExternalId() {
return externalId;
}
/**
* Sets the value of the externalId property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setExternalId(String value) {
this.externalId = value;
}
/**
* Gets the value of the externalCheckSum property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getExternalCheckSum() {
return externalCheckSum;
}
/**
* Sets the value of the externalCheckSum property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setExternalCheckSum(String value) {
this.externalCheckSum = value;
}
/**
* Link zum Element.
*
* @return
* possible object is
* {@link String }
*
*/
public URL getHref() {
return href;
}
/**
* Sets the value of the href property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setHref(URL value) {
this.href = value;
}
/**
* Gets the value of the id property.
*
* @return
* possible object is
* {@link Long }
*
*/
public Long getId() {
return id;
}
/**
* Sets the value of the id property.
*
* @param value
* allowed object is
* {@link Long }
*
*/
public void setId(Long value) {
this.id = value;
}
/**
* Gets the value of the label property.
*
* @return
* possible object is
* {@link String }
*
*/
public String getLabel() {
return label;
}
/**
* Sets the value of the label property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setLabel(String value) {
this.label = value;
}
/**
* Gets the value of the modification property.
*
* @return
* possible object is
* {@link String }
*
*/
public Calendar getModification() {
return modification;
}
/**
* Sets the value of the modification property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setModification(Calendar value) {
this.modification = value;
}
/**
* Gets the value of the creation property.
*
* @return
* possible object is
* {@link String }
*
*/
public Calendar getCreation() {
return creation;
}
/**
* Sets the value of the creation property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setCreation(Calendar value) {
this.creation = value;
}
/**
* Gets the value of the publishDate property.
*
* @return
* possible object is
* {@link String }
*
*/
public Calendar getPublishDate() {
return publishDate;
}
/**
* Sets the value of the publishDate property.
*
* @param value
* allowed object is
* {@link String }
*
*/
public void setPublishDate(Calendar value) {
this.publishDate = value;
}
public String toString() {
final ToStringStrategy2 strategy = JAXBToStringStrategy.INSTANCE;
final StringBuilder buffer = new StringBuilder();
append(null, buffer, strategy);
return buffer.toString();
}
public StringBuilder append(ObjectLocator locator, StringBuilder buffer, ToStringStrategy2 strategy) {
strategy.appendStart(locator, this, buffer);
appendFields(locator, buffer, strategy);
strategy.appendEnd(locator, this, buffer);
return buffer;
}
public StringBuilder appendFields(ObjectLocator locator, StringBuilder buffer, ToStringStrategy2 strategy) {
{
String theTitle;
theTitle = this.getTitle();
strategy.appendField(locator, this, "title", buffer, theTitle, (this.title!= null));
}
{
String theCheckSum;
theCheckSum = this.getCheckSum();
strategy.appendField(locator, this, "checkSum", buffer, theCheckSum, (this.checkSum!= null));
}
{
String theExternalId;
theExternalId = this.getExternalId();
strategy.appendField(locator, this, "externalId", buffer, theExternalId, (this.externalId!= null));
}
{
String theExternalCheckSum;
theExternalCheckSum = this.getExternalCheckSum();
strategy.appendField(locator, this, "externalCheckSum", buffer, theExternalCheckSum, (this.externalCheckSum!= null));
}
{
URL theHref;
theHref = this.getHref();
strategy.appendField(locator, this, "href", buffer, theHref, (this.href!= null));
}
{
Long theId;
theId = this.getId();
strategy.appendField(locator, this, "id", buffer, theId, (this.id!= null));
}
{
String theLabel;
theLabel = this.getLabel();
strategy.appendField(locator, this, "label", buffer, theLabel, (this.label!= null));
}
{
Calendar theModification;
theModification = this.getModification();
strategy.appendField(locator, this, "modification", buffer, theModification, (this.modification!= null));
}
{
Calendar theCreation;
theCreation = this.getCreation();
strategy.appendField(locator, this, "creation", buffer, theCreation, (this.creation!= null));
}
{
Calendar thePublishDate;
thePublishDate = this.getPublishDate();
strategy.appendField(locator, this, "publishDate", buffer, thePublishDate, (this.publishDate!= null));
}
return buffer;
}
public Object clone() {
return copyTo(createNewInstance());
}
public Object copyTo(Object target) {
final CopyStrategy2 strategy = JAXBCopyStrategy.INSTANCE;
return copyTo(null, target, strategy);
}
public Object copyTo(ObjectLocator locator, Object target, CopyStrategy2 strategy) {
if (null == target) {
throw new IllegalArgumentException("Target argument must not be null for abstract copyable classes.");
}
if (target instanceof Attachment) {
final Attachment copy = ((Attachment) target);
{
Boolean titleShouldBeCopiedAndSet = strategy.shouldBeCopiedAndSet(locator, (this.title!= null));
if (titleShouldBeCopiedAndSet == Boolean.TRUE) {
String sourceTitle;
sourceTitle = this.getTitle();
String copyTitle = ((String) strategy.copy(LocatorUtils.property(locator, "title", sourceTitle), sourceTitle, (this.title!= null)));
copy.setTitle(copyTitle);
} else {
if (titleShouldBeCopiedAndSet == Boolean.FALSE) {
copy.title = null;
}
}
}
{
Boolean checkSumShouldBeCopiedAndSet = strategy.shouldBeCopiedAndSet(locator, (this.checkSum!= null));
if (checkSumShouldBeCopiedAndSet == Boolean.TRUE) {
String sourceCheckSum;
sourceCheckSum = this.getCheckSum();
String copyCheckSum = ((String) strategy.copy(LocatorUtils.property(locator, "checkSum", sourceCheckSum), sourceCheckSum, (this.checkSum!= null)));
copy.setCheckSum(copyCheckSum);
} else {
if (checkSumShouldBeCopiedAndSet == Boolean.FALSE) {
copy.checkSum = null;
}
}
}
{
Boolean externalIdShouldBeCopiedAndSet = strategy.shouldBeCopiedAndSet(locator, (this.externalId!= null));
if (externalIdShouldBeCopiedAndSet == Boolean.TRUE) {
String sourceExternalId;
sourceExternalId = this.getExternalId();
String copyExternalId = ((String) strategy.copy(LocatorUtils.property(locator, "externalId", sourceExternalId), sourceExternalId, (this.externalId!= null)));
copy.setExternalId(copyExternalId);
} else {
if (externalIdShouldBeCopiedAndSet == Boolean.FALSE) {
copy.externalId = null;
}
}
}
{
Boolean externalCheckSumShouldBeCopiedAndSet = strategy.shouldBeCopiedAndSet(locator, (this.externalCheckSum!= null));
if (externalCheckSumShouldBeCopiedAndSet == Boolean.TRUE) {
String sourceExternalCheckSum;
sourceExternalCheckSum = this.getExternalCheckSum();
String copyExternalCheckSum = ((String) strategy.copy(LocatorUtils.property(locator, "externalCheckSum", sourceExternalCheckSum), sourceExternalCheckSum, (this.externalCheckSum!= null)));
copy.setExternalCheckSum(copyExternalCheckSum);
} else {
if (externalCheckSumShouldBeCopiedAndSet == Boolean.FALSE) {
copy.externalCheckSum = null;
}
}
}
{
Boolean hrefShouldBeCopiedAndSet = strategy.shouldBeCopiedAndSet(locator, (this.href!= null));
if (hrefShouldBeCopiedAndSet == Boolean.TRUE) {
URL sourceHref;
sourceHref = this.getHref();
URL copyHref = ((URL) strategy.copy(LocatorUtils.property(locator, "href", sourceHref), sourceHref, (this.href!= null)));
copy.setHref(copyHref);
} else {
if (hrefShouldBeCopiedAndSet == Boolean.FALSE) {
copy.href = null;
}
}
}
{
Boolean idShouldBeCopiedAndSet = strategy.shouldBeCopiedAndSet(locator, (this.id!= null));
if (idShouldBeCopiedAndSet == Boolean.TRUE) {
Long sourceId;
sourceId = this.getId();
Long copyId = ((Long) strategy.copy(LocatorUtils.property(locator, "id", sourceId), sourceId, (this.id!= null)));
copy.setId(copyId);
} else {
if (idShouldBeCopiedAndSet == Boolean.FALSE) {
copy.id = null;
}
}
}
{
Boolean labelShouldBeCopiedAndSet = strategy.shouldBeCopiedAndSet(locator, (this.label!= null));
if (labelShouldBeCopiedAndSet == Boolean.TRUE) {
String sourceLabel;
sourceLabel = this.getLabel();
String copyLabel = ((String) strategy.copy(LocatorUtils.property(locator, "label", sourceLabel), sourceLabel, (this.label!= null)));
copy.setLabel(copyLabel);
} else {
if (labelShouldBeCopiedAndSet == Boolean.FALSE) {
copy.label = null;
}
}
}
{
Boolean modificationShouldBeCopiedAndSet = strategy.shouldBeCopiedAndSet(locator, (this.modification!= null));
if (modificationShouldBeCopiedAndSet == Boolean.TRUE) {
Calendar sourceModification;
sourceModification = this.getModification();
Calendar copyModification = ((Calendar) strategy.copy(LocatorUtils.property(locator, "modification", sourceModification), sourceModification, (this.modification!= null)));
copy.setModification(copyModification);
} else {
if (modificationShouldBeCopiedAndSet == Boolean.FALSE) {
copy.modification = null;
}
}
}
{
Boolean creationShouldBeCopiedAndSet = strategy.shouldBeCopiedAndSet(locator, (this.creation!= null));
if (creationShouldBeCopiedAndSet == Boolean.TRUE) {
Calendar sourceCreation;
sourceCreation = this.getCreation();
Calendar copyCreation = ((Calendar) strategy.copy(LocatorUtils.property(locator, "creation", sourceCreation), sourceCreation, (this.creation!= null)));
copy.setCreation(copyCreation);
} else {
if (creationShouldBeCopiedAndSet == Boolean.FALSE) {
copy.creation = null;
}
}
}
{
Boolean publishDateShouldBeCopiedAndSet = strategy.shouldBeCopiedAndSet(locator, (this.publishDate!= null));
if (publishDateShouldBeCopiedAndSet == Boolean.TRUE) {
Calendar sourcePublishDate;
sourcePublishDate = this.getPublishDate();
Calendar copyPublishDate = ((Calendar) strategy.copy(LocatorUtils.property(locator, "publishDate", sourcePublishDate), sourcePublishDate, (this.publishDate!= null)));
copy.setPublishDate(copyPublishDate);
} else {
if (publishDateShouldBeCopiedAndSet == Boolean.FALSE) {
copy.publishDate = null;
}
}
}
}
return target;
}
public boolean equals(ObjectLocator thisLocator, ObjectLocator thatLocator, Object object, EqualsStrategy2 strategy) {
if ((object == null)||(this.getClass()!= object.getClass())) {
return false;
}
if (this == object) {
return true;
}
final Attachment that = ((Attachment) object);
{
String lhsTitle;
lhsTitle = this.getTitle();
String rhsTitle;
rhsTitle = that.getTitle();
if (!strategy.equals(LocatorUtils.property(thisLocator, "title", lhsTitle), LocatorUtils.property(thatLocator, "title", rhsTitle), lhsTitle, rhsTitle, (this.title!= null), (that.title!= null))) {
return false;
}
}
{
String lhsCheckSum;
lhsCheckSum = this.getCheckSum();
String rhsCheckSum;
rhsCheckSum = that.getCheckSum();
if (!strategy.equals(LocatorUtils.property(thisLocator, "checkSum", lhsCheckSum), LocatorUtils.property(thatLocator, "checkSum", rhsCheckSum), lhsCheckSum, rhsCheckSum, (this.checkSum!= null), (that.checkSum!= null))) {
return false;
}
}
{
String lhsExternalId;
lhsExternalId = this.getExternalId();
String rhsExternalId;
rhsExternalId = that.getExternalId();
if (!strategy.equals(LocatorUtils.property(thisLocator, "externalId", lhsExternalId), LocatorUtils.property(thatLocator, "externalId", rhsExternalId), lhsExternalId, rhsExternalId, (this.externalId!= null), (that.externalId!= null))) {
return false;
}
}
{
String lhsExternalCheckSum;
lhsExternalCheckSum = this.getExternalCheckSum();
String rhsExternalCheckSum;
rhsExternalCheckSum = that.getExternalCheckSum();
if (!strategy.equals(LocatorUtils.property(thisLocator, "externalCheckSum", lhsExternalCheckSum), LocatorUtils.property(thatLocator, "externalCheckSum", rhsExternalCheckSum), lhsExternalCheckSum, rhsExternalCheckSum, (this.externalCheckSum!= null), (that.externalCheckSum!= null))) {
return false;
}
}
{
URL lhsHref;
lhsHref = this.getHref();
URL rhsHref;
rhsHref = that.getHref();
if (!strategy.equals(LocatorUtils.property(thisLocator, "href", lhsHref), LocatorUtils.property(thatLocator, "href", rhsHref), lhsHref, rhsHref, (this.href!= null), (that.href!= null))) {
return false;
}
}
{
Long lhsId;
lhsId = this.getId();
Long rhsId;
rhsId = that.getId();
if (!strategy.equals(LocatorUtils.property(thisLocator, "id", lhsId), LocatorUtils.property(thatLocator, "id", rhsId), lhsId, rhsId, (this.id!= null), (that.id!= null))) {
return false;
}
}
{
String lhsLabel;
lhsLabel = this.getLabel();
String rhsLabel;
rhsLabel = that.getLabel();
if (!strategy.equals(LocatorUtils.property(thisLocator, "label", lhsLabel), LocatorUtils.property(thatLocator, "label", rhsLabel), lhsLabel, rhsLabel, (this.label!= null), (that.label!= null))) {
return false;
}
}
{
Calendar lhsModification;
lhsModification = this.getModification();
Calendar rhsModification;
rhsModification = that.getModification();
if (!strategy.equals(LocatorUtils.property(thisLocator, "modification", lhsModification), LocatorUtils.property(thatLocator, "modification", rhsModification), lhsModification, rhsModification, (this.modification!= null), (that.modification!= null))) {
return false;
}
}
{
Calendar lhsCreation;
lhsCreation = this.getCreation();
Calendar rhsCreation;
rhsCreation = that.getCreation();
if (!strategy.equals(LocatorUtils.property(thisLocator, "creation", lhsCreation), LocatorUtils.property(thatLocator, "creation", rhsCreation), lhsCreation, rhsCreation, (this.creation!= null), (that.creation!= null))) {
return false;
}
}
{
Calendar lhsPublishDate;
lhsPublishDate = this.getPublishDate();
Calendar rhsPublishDate;
rhsPublishDate = that.getPublishDate();
if (!strategy.equals(LocatorUtils.property(thisLocator, "publishDate", lhsPublishDate), LocatorUtils.property(thatLocator, "publishDate", rhsPublishDate), lhsPublishDate, rhsPublishDate, (this.publishDate!= null), (that.publishDate!= null))) {
return false;
}
}
return true;
}
public boolean equals(Object object) {
final EqualsStrategy2 strategy = JAXBEqualsStrategy.INSTANCE;
return equals(null, null, object, strategy);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy