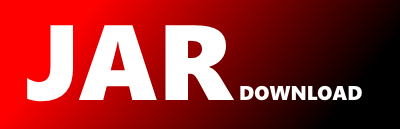
org.openfeed.BestBidOffer Maven / Gradle / Ivy
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// NO CHECKED-IN PROTOBUF GENCODE
// source: openfeed.proto
// Protobuf Java Version: 4.28.3
package org.openfeed;
/**
*
* / Best Bid and Offer.
* / If a side is not present, then that side has been deleted.
* / By default this value is the NBBO, if regional/participant quote then regional = true
*
*
* Protobuf type {@code org.openfeed.BestBidOffer}
*/
public final class BestBidOffer extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:org.openfeed.BestBidOffer)
BestBidOfferOrBuilder {
private static final long serialVersionUID = 0L;
static {
com.google.protobuf.RuntimeVersion.validateProtobufGencodeVersion(
com.google.protobuf.RuntimeVersion.RuntimeDomain.PUBLIC,
/* major= */ 4,
/* minor= */ 28,
/* patch= */ 3,
/* suffix= */ "",
BestBidOffer.class.getName());
}
// Use BestBidOffer.newBuilder() to construct.
private BestBidOffer(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private BestBidOffer() {
bidOriginator_ = com.google.protobuf.ByteString.EMPTY;
bidQuoteCondition_ = com.google.protobuf.ByteString.EMPTY;
offerOriginator_ = com.google.protobuf.ByteString.EMPTY;
offerQuoteCondition_ = com.google.protobuf.ByteString.EMPTY;
quoteCondition_ = com.google.protobuf.ByteString.EMPTY;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.openfeed.Openfeed.internal_static_org_openfeed_BestBidOffer_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.openfeed.Openfeed.internal_static_org_openfeed_BestBidOffer_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.openfeed.BestBidOffer.class, org.openfeed.BestBidOffer.Builder.class);
}
public static final int TRANSACTIONTIME_FIELD_NUMBER = 9;
private long transactionTime_ = 0L;
/**
*
* UTC Timestamp, nano seconds since Unix epoch
*
*
* sint64 transactionTime = 9;
* @return The transactionTime.
*/
@java.lang.Override
public long getTransactionTime() {
return transactionTime_;
}
public static final int BIDPRICE_FIELD_NUMBER = 10;
private long bidPrice_ = 0L;
/**
*
* / Divide by priceDenominator
*
*
* sint64 bidPrice = 10;
* @return The bidPrice.
*/
@java.lang.Override
public long getBidPrice() {
return bidPrice_;
}
public static final int BIDQUANTITY_FIELD_NUMBER = 11;
private long bidQuantity_ = 0L;
/**
*
* / Divide by quantityDenominator
*
*
* sint64 bidQuantity = 11;
* @return The bidQuantity.
*/
@java.lang.Override
public long getBidQuantity() {
return bidQuantity_;
}
public static final int BIDORDERCOUNT_FIELD_NUMBER = 12;
private int bidOrderCount_ = 0;
/**
* sint32 bidOrderCount = 12;
* @return The bidOrderCount.
*/
@java.lang.Override
public int getBidOrderCount() {
return bidOrderCount_;
}
public static final int BIDORIGINATOR_FIELD_NUMBER = 13;
private com.google.protobuf.ByteString bidOriginator_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* / Liquidity provider information
* For Forex: BANK:CITY
* For Equities: EXCHANGE_MIC
*
*
* bytes bidOriginator = 13;
* @return The bidOriginator.
*/
@java.lang.Override
public com.google.protobuf.ByteString getBidOriginator() {
return bidOriginator_;
}
public static final int BIDQUOTECONDITION_FIELD_NUMBER = 14;
private com.google.protobuf.ByteString bidQuoteCondition_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes bidQuoteCondition = 14;
* @return The bidQuoteCondition.
*/
@java.lang.Override
public com.google.protobuf.ByteString getBidQuoteCondition() {
return bidQuoteCondition_;
}
public static final int OFFERPRICE_FIELD_NUMBER = 20;
private long offerPrice_ = 0L;
/**
*
* / Divide by priceDenominator
*
*
* sint64 offerPrice = 20;
* @return The offerPrice.
*/
@java.lang.Override
public long getOfferPrice() {
return offerPrice_;
}
public static final int OFFERQUANTITY_FIELD_NUMBER = 21;
private long offerQuantity_ = 0L;
/**
*
* / Divide by quantityDenominator
*
*
* sint64 offerQuantity = 21;
* @return The offerQuantity.
*/
@java.lang.Override
public long getOfferQuantity() {
return offerQuantity_;
}
public static final int OFFERORDERCOUNT_FIELD_NUMBER = 22;
private int offerOrderCount_ = 0;
/**
* sint32 offerOrderCount = 22;
* @return The offerOrderCount.
*/
@java.lang.Override
public int getOfferOrderCount() {
return offerOrderCount_;
}
public static final int OFFERORIGINATOR_FIELD_NUMBER = 23;
private com.google.protobuf.ByteString offerOriginator_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* / Liquidity provider information
* For Forex: BANK:CITY
* For Equities: EXCHANGE_MIC
*
*
* bytes offerOriginator = 23;
* @return The offerOriginator.
*/
@java.lang.Override
public com.google.protobuf.ByteString getOfferOriginator() {
return offerOriginator_;
}
public static final int OFFERQUOTECONDITION_FIELD_NUMBER = 24;
private com.google.protobuf.ByteString offerQuoteCondition_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes offerQuoteCondition = 24;
* @return The offerQuoteCondition.
*/
@java.lang.Override
public com.google.protobuf.ByteString getOfferQuoteCondition() {
return offerQuoteCondition_;
}
public static final int QUOTECONDITION_FIELD_NUMBER = 30;
private com.google.protobuf.ByteString quoteCondition_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes quoteCondition = 30;
* @return The quoteCondition.
*/
@java.lang.Override
public com.google.protobuf.ByteString getQuoteCondition() {
return quoteCondition_;
}
public static final int REGIONAL_FIELD_NUMBER = 32;
private boolean regional_ = false;
/**
*
* / True if regional/participant member quote
*
*
* bool regional = 32;
* @return The regional.
*/
@java.lang.Override
public boolean getRegional() {
return regional_;
}
public static final int TRANSIENT_FIELD_NUMBER = 33;
private boolean transient_ = false;
/**
*
* / True if not persisted in the EOD database.
*
*
* bool transient = 33;
* @return The transient.
*/
@java.lang.Override
public boolean getTransient() {
return transient_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (transactionTime_ != 0L) {
output.writeSInt64(9, transactionTime_);
}
if (bidPrice_ != 0L) {
output.writeSInt64(10, bidPrice_);
}
if (bidQuantity_ != 0L) {
output.writeSInt64(11, bidQuantity_);
}
if (bidOrderCount_ != 0) {
output.writeSInt32(12, bidOrderCount_);
}
if (!bidOriginator_.isEmpty()) {
output.writeBytes(13, bidOriginator_);
}
if (!bidQuoteCondition_.isEmpty()) {
output.writeBytes(14, bidQuoteCondition_);
}
if (offerPrice_ != 0L) {
output.writeSInt64(20, offerPrice_);
}
if (offerQuantity_ != 0L) {
output.writeSInt64(21, offerQuantity_);
}
if (offerOrderCount_ != 0) {
output.writeSInt32(22, offerOrderCount_);
}
if (!offerOriginator_.isEmpty()) {
output.writeBytes(23, offerOriginator_);
}
if (!offerQuoteCondition_.isEmpty()) {
output.writeBytes(24, offerQuoteCondition_);
}
if (!quoteCondition_.isEmpty()) {
output.writeBytes(30, quoteCondition_);
}
if (regional_ != false) {
output.writeBool(32, regional_);
}
if (transient_ != false) {
output.writeBool(33, transient_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (transactionTime_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeSInt64Size(9, transactionTime_);
}
if (bidPrice_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeSInt64Size(10, bidPrice_);
}
if (bidQuantity_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeSInt64Size(11, bidQuantity_);
}
if (bidOrderCount_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeSInt32Size(12, bidOrderCount_);
}
if (!bidOriginator_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(13, bidOriginator_);
}
if (!bidQuoteCondition_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(14, bidQuoteCondition_);
}
if (offerPrice_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeSInt64Size(20, offerPrice_);
}
if (offerQuantity_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeSInt64Size(21, offerQuantity_);
}
if (offerOrderCount_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeSInt32Size(22, offerOrderCount_);
}
if (!offerOriginator_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(23, offerOriginator_);
}
if (!offerQuoteCondition_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(24, offerQuoteCondition_);
}
if (!quoteCondition_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(30, quoteCondition_);
}
if (regional_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(32, regional_);
}
if (transient_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(33, transient_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.openfeed.BestBidOffer)) {
return super.equals(obj);
}
org.openfeed.BestBidOffer other = (org.openfeed.BestBidOffer) obj;
if (getTransactionTime()
!= other.getTransactionTime()) return false;
if (getBidPrice()
!= other.getBidPrice()) return false;
if (getBidQuantity()
!= other.getBidQuantity()) return false;
if (getBidOrderCount()
!= other.getBidOrderCount()) return false;
if (!getBidOriginator()
.equals(other.getBidOriginator())) return false;
if (!getBidQuoteCondition()
.equals(other.getBidQuoteCondition())) return false;
if (getOfferPrice()
!= other.getOfferPrice()) return false;
if (getOfferQuantity()
!= other.getOfferQuantity()) return false;
if (getOfferOrderCount()
!= other.getOfferOrderCount()) return false;
if (!getOfferOriginator()
.equals(other.getOfferOriginator())) return false;
if (!getOfferQuoteCondition()
.equals(other.getOfferQuoteCondition())) return false;
if (!getQuoteCondition()
.equals(other.getQuoteCondition())) return false;
if (getRegional()
!= other.getRegional()) return false;
if (getTransient()
!= other.getTransient()) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + TRANSACTIONTIME_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getTransactionTime());
hash = (37 * hash) + BIDPRICE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getBidPrice());
hash = (37 * hash) + BIDQUANTITY_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getBidQuantity());
hash = (37 * hash) + BIDORDERCOUNT_FIELD_NUMBER;
hash = (53 * hash) + getBidOrderCount();
hash = (37 * hash) + BIDORIGINATOR_FIELD_NUMBER;
hash = (53 * hash) + getBidOriginator().hashCode();
hash = (37 * hash) + BIDQUOTECONDITION_FIELD_NUMBER;
hash = (53 * hash) + getBidQuoteCondition().hashCode();
hash = (37 * hash) + OFFERPRICE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getOfferPrice());
hash = (37 * hash) + OFFERQUANTITY_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getOfferQuantity());
hash = (37 * hash) + OFFERORDERCOUNT_FIELD_NUMBER;
hash = (53 * hash) + getOfferOrderCount();
hash = (37 * hash) + OFFERORIGINATOR_FIELD_NUMBER;
hash = (53 * hash) + getOfferOriginator().hashCode();
hash = (37 * hash) + OFFERQUOTECONDITION_FIELD_NUMBER;
hash = (53 * hash) + getOfferQuoteCondition().hashCode();
hash = (37 * hash) + QUOTECONDITION_FIELD_NUMBER;
hash = (53 * hash) + getQuoteCondition().hashCode();
hash = (37 * hash) + REGIONAL_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getRegional());
hash = (37 * hash) + TRANSIENT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getTransient());
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.openfeed.BestBidOffer parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.openfeed.BestBidOffer parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.openfeed.BestBidOffer parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.openfeed.BestBidOffer parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.openfeed.BestBidOffer parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.openfeed.BestBidOffer parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.openfeed.BestBidOffer parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static org.openfeed.BestBidOffer parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.openfeed.BestBidOffer parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input);
}
public static org.openfeed.BestBidOffer parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.openfeed.BestBidOffer parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static org.openfeed.BestBidOffer parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.openfeed.BestBidOffer prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* / Best Bid and Offer.
* / If a side is not present, then that side has been deleted.
* / By default this value is the NBBO, if regional/participant quote then regional = true
*
*
* Protobuf type {@code org.openfeed.BestBidOffer}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:org.openfeed.BestBidOffer)
org.openfeed.BestBidOfferOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.openfeed.Openfeed.internal_static_org_openfeed_BestBidOffer_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.openfeed.Openfeed.internal_static_org_openfeed_BestBidOffer_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.openfeed.BestBidOffer.class, org.openfeed.BestBidOffer.Builder.class);
}
// Construct using org.openfeed.BestBidOffer.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
transactionTime_ = 0L;
bidPrice_ = 0L;
bidQuantity_ = 0L;
bidOrderCount_ = 0;
bidOriginator_ = com.google.protobuf.ByteString.EMPTY;
bidQuoteCondition_ = com.google.protobuf.ByteString.EMPTY;
offerPrice_ = 0L;
offerQuantity_ = 0L;
offerOrderCount_ = 0;
offerOriginator_ = com.google.protobuf.ByteString.EMPTY;
offerQuoteCondition_ = com.google.protobuf.ByteString.EMPTY;
quoteCondition_ = com.google.protobuf.ByteString.EMPTY;
regional_ = false;
transient_ = false;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.openfeed.Openfeed.internal_static_org_openfeed_BestBidOffer_descriptor;
}
@java.lang.Override
public org.openfeed.BestBidOffer getDefaultInstanceForType() {
return org.openfeed.BestBidOffer.getDefaultInstance();
}
@java.lang.Override
public org.openfeed.BestBidOffer build() {
org.openfeed.BestBidOffer result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.openfeed.BestBidOffer buildPartial() {
org.openfeed.BestBidOffer result = new org.openfeed.BestBidOffer(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(org.openfeed.BestBidOffer result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.transactionTime_ = transactionTime_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.bidPrice_ = bidPrice_;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.bidQuantity_ = bidQuantity_;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.bidOrderCount_ = bidOrderCount_;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.bidOriginator_ = bidOriginator_;
}
if (((from_bitField0_ & 0x00000020) != 0)) {
result.bidQuoteCondition_ = bidQuoteCondition_;
}
if (((from_bitField0_ & 0x00000040) != 0)) {
result.offerPrice_ = offerPrice_;
}
if (((from_bitField0_ & 0x00000080) != 0)) {
result.offerQuantity_ = offerQuantity_;
}
if (((from_bitField0_ & 0x00000100) != 0)) {
result.offerOrderCount_ = offerOrderCount_;
}
if (((from_bitField0_ & 0x00000200) != 0)) {
result.offerOriginator_ = offerOriginator_;
}
if (((from_bitField0_ & 0x00000400) != 0)) {
result.offerQuoteCondition_ = offerQuoteCondition_;
}
if (((from_bitField0_ & 0x00000800) != 0)) {
result.quoteCondition_ = quoteCondition_;
}
if (((from_bitField0_ & 0x00001000) != 0)) {
result.regional_ = regional_;
}
if (((from_bitField0_ & 0x00002000) != 0)) {
result.transient_ = transient_;
}
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.openfeed.BestBidOffer) {
return mergeFrom((org.openfeed.BestBidOffer)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.openfeed.BestBidOffer other) {
if (other == org.openfeed.BestBidOffer.getDefaultInstance()) return this;
if (other.getTransactionTime() != 0L) {
setTransactionTime(other.getTransactionTime());
}
if (other.getBidPrice() != 0L) {
setBidPrice(other.getBidPrice());
}
if (other.getBidQuantity() != 0L) {
setBidQuantity(other.getBidQuantity());
}
if (other.getBidOrderCount() != 0) {
setBidOrderCount(other.getBidOrderCount());
}
if (other.getBidOriginator() != com.google.protobuf.ByteString.EMPTY) {
setBidOriginator(other.getBidOriginator());
}
if (other.getBidQuoteCondition() != com.google.protobuf.ByteString.EMPTY) {
setBidQuoteCondition(other.getBidQuoteCondition());
}
if (other.getOfferPrice() != 0L) {
setOfferPrice(other.getOfferPrice());
}
if (other.getOfferQuantity() != 0L) {
setOfferQuantity(other.getOfferQuantity());
}
if (other.getOfferOrderCount() != 0) {
setOfferOrderCount(other.getOfferOrderCount());
}
if (other.getOfferOriginator() != com.google.protobuf.ByteString.EMPTY) {
setOfferOriginator(other.getOfferOriginator());
}
if (other.getOfferQuoteCondition() != com.google.protobuf.ByteString.EMPTY) {
setOfferQuoteCondition(other.getOfferQuoteCondition());
}
if (other.getQuoteCondition() != com.google.protobuf.ByteString.EMPTY) {
setQuoteCondition(other.getQuoteCondition());
}
if (other.getRegional() != false) {
setRegional(other.getRegional());
}
if (other.getTransient() != false) {
setTransient(other.getTransient());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 72: {
transactionTime_ = input.readSInt64();
bitField0_ |= 0x00000001;
break;
} // case 72
case 80: {
bidPrice_ = input.readSInt64();
bitField0_ |= 0x00000002;
break;
} // case 80
case 88: {
bidQuantity_ = input.readSInt64();
bitField0_ |= 0x00000004;
break;
} // case 88
case 96: {
bidOrderCount_ = input.readSInt32();
bitField0_ |= 0x00000008;
break;
} // case 96
case 106: {
bidOriginator_ = input.readBytes();
bitField0_ |= 0x00000010;
break;
} // case 106
case 114: {
bidQuoteCondition_ = input.readBytes();
bitField0_ |= 0x00000020;
break;
} // case 114
case 160: {
offerPrice_ = input.readSInt64();
bitField0_ |= 0x00000040;
break;
} // case 160
case 168: {
offerQuantity_ = input.readSInt64();
bitField0_ |= 0x00000080;
break;
} // case 168
case 176: {
offerOrderCount_ = input.readSInt32();
bitField0_ |= 0x00000100;
break;
} // case 176
case 186: {
offerOriginator_ = input.readBytes();
bitField0_ |= 0x00000200;
break;
} // case 186
case 194: {
offerQuoteCondition_ = input.readBytes();
bitField0_ |= 0x00000400;
break;
} // case 194
case 242: {
quoteCondition_ = input.readBytes();
bitField0_ |= 0x00000800;
break;
} // case 242
case 256: {
regional_ = input.readBool();
bitField0_ |= 0x00001000;
break;
} // case 256
case 264: {
transient_ = input.readBool();
bitField0_ |= 0x00002000;
break;
} // case 264
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private long transactionTime_ ;
/**
*
* UTC Timestamp, nano seconds since Unix epoch
*
*
* sint64 transactionTime = 9;
* @return The transactionTime.
*/
@java.lang.Override
public long getTransactionTime() {
return transactionTime_;
}
/**
*
* UTC Timestamp, nano seconds since Unix epoch
*
*
* sint64 transactionTime = 9;
* @param value The transactionTime to set.
* @return This builder for chaining.
*/
public Builder setTransactionTime(long value) {
transactionTime_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* UTC Timestamp, nano seconds since Unix epoch
*
*
* sint64 transactionTime = 9;
* @return This builder for chaining.
*/
public Builder clearTransactionTime() {
bitField0_ = (bitField0_ & ~0x00000001);
transactionTime_ = 0L;
onChanged();
return this;
}
private long bidPrice_ ;
/**
*
* / Divide by priceDenominator
*
*
* sint64 bidPrice = 10;
* @return The bidPrice.
*/
@java.lang.Override
public long getBidPrice() {
return bidPrice_;
}
/**
*
* / Divide by priceDenominator
*
*
* sint64 bidPrice = 10;
* @param value The bidPrice to set.
* @return This builder for chaining.
*/
public Builder setBidPrice(long value) {
bidPrice_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
* / Divide by priceDenominator
*
*
* sint64 bidPrice = 10;
* @return This builder for chaining.
*/
public Builder clearBidPrice() {
bitField0_ = (bitField0_ & ~0x00000002);
bidPrice_ = 0L;
onChanged();
return this;
}
private long bidQuantity_ ;
/**
*
* / Divide by quantityDenominator
*
*
* sint64 bidQuantity = 11;
* @return The bidQuantity.
*/
@java.lang.Override
public long getBidQuantity() {
return bidQuantity_;
}
/**
*
* / Divide by quantityDenominator
*
*
* sint64 bidQuantity = 11;
* @param value The bidQuantity to set.
* @return This builder for chaining.
*/
public Builder setBidQuantity(long value) {
bidQuantity_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
* / Divide by quantityDenominator
*
*
* sint64 bidQuantity = 11;
* @return This builder for chaining.
*/
public Builder clearBidQuantity() {
bitField0_ = (bitField0_ & ~0x00000004);
bidQuantity_ = 0L;
onChanged();
return this;
}
private int bidOrderCount_ ;
/**
* sint32 bidOrderCount = 12;
* @return The bidOrderCount.
*/
@java.lang.Override
public int getBidOrderCount() {
return bidOrderCount_;
}
/**
* sint32 bidOrderCount = 12;
* @param value The bidOrderCount to set.
* @return This builder for chaining.
*/
public Builder setBidOrderCount(int value) {
bidOrderCount_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
* sint32 bidOrderCount = 12;
* @return This builder for chaining.
*/
public Builder clearBidOrderCount() {
bitField0_ = (bitField0_ & ~0x00000008);
bidOrderCount_ = 0;
onChanged();
return this;
}
private com.google.protobuf.ByteString bidOriginator_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* / Liquidity provider information
* For Forex: BANK:CITY
* For Equities: EXCHANGE_MIC
*
*
* bytes bidOriginator = 13;
* @return The bidOriginator.
*/
@java.lang.Override
public com.google.protobuf.ByteString getBidOriginator() {
return bidOriginator_;
}
/**
*
* / Liquidity provider information
* For Forex: BANK:CITY
* For Equities: EXCHANGE_MIC
*
*
* bytes bidOriginator = 13;
* @param value The bidOriginator to set.
* @return This builder for chaining.
*/
public Builder setBidOriginator(com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
bidOriginator_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
*
* / Liquidity provider information
* For Forex: BANK:CITY
* For Equities: EXCHANGE_MIC
*
*
* bytes bidOriginator = 13;
* @return This builder for chaining.
*/
public Builder clearBidOriginator() {
bitField0_ = (bitField0_ & ~0x00000010);
bidOriginator_ = getDefaultInstance().getBidOriginator();
onChanged();
return this;
}
private com.google.protobuf.ByteString bidQuoteCondition_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes bidQuoteCondition = 14;
* @return The bidQuoteCondition.
*/
@java.lang.Override
public com.google.protobuf.ByteString getBidQuoteCondition() {
return bidQuoteCondition_;
}
/**
* bytes bidQuoteCondition = 14;
* @param value The bidQuoteCondition to set.
* @return This builder for chaining.
*/
public Builder setBidQuoteCondition(com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
bidQuoteCondition_ = value;
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
* bytes bidQuoteCondition = 14;
* @return This builder for chaining.
*/
public Builder clearBidQuoteCondition() {
bitField0_ = (bitField0_ & ~0x00000020);
bidQuoteCondition_ = getDefaultInstance().getBidQuoteCondition();
onChanged();
return this;
}
private long offerPrice_ ;
/**
*
* / Divide by priceDenominator
*
*
* sint64 offerPrice = 20;
* @return The offerPrice.
*/
@java.lang.Override
public long getOfferPrice() {
return offerPrice_;
}
/**
*
* / Divide by priceDenominator
*
*
* sint64 offerPrice = 20;
* @param value The offerPrice to set.
* @return This builder for chaining.
*/
public Builder setOfferPrice(long value) {
offerPrice_ = value;
bitField0_ |= 0x00000040;
onChanged();
return this;
}
/**
*
* / Divide by priceDenominator
*
*
* sint64 offerPrice = 20;
* @return This builder for chaining.
*/
public Builder clearOfferPrice() {
bitField0_ = (bitField0_ & ~0x00000040);
offerPrice_ = 0L;
onChanged();
return this;
}
private long offerQuantity_ ;
/**
*
* / Divide by quantityDenominator
*
*
* sint64 offerQuantity = 21;
* @return The offerQuantity.
*/
@java.lang.Override
public long getOfferQuantity() {
return offerQuantity_;
}
/**
*
* / Divide by quantityDenominator
*
*
* sint64 offerQuantity = 21;
* @param value The offerQuantity to set.
* @return This builder for chaining.
*/
public Builder setOfferQuantity(long value) {
offerQuantity_ = value;
bitField0_ |= 0x00000080;
onChanged();
return this;
}
/**
*
* / Divide by quantityDenominator
*
*
* sint64 offerQuantity = 21;
* @return This builder for chaining.
*/
public Builder clearOfferQuantity() {
bitField0_ = (bitField0_ & ~0x00000080);
offerQuantity_ = 0L;
onChanged();
return this;
}
private int offerOrderCount_ ;
/**
* sint32 offerOrderCount = 22;
* @return The offerOrderCount.
*/
@java.lang.Override
public int getOfferOrderCount() {
return offerOrderCount_;
}
/**
* sint32 offerOrderCount = 22;
* @param value The offerOrderCount to set.
* @return This builder for chaining.
*/
public Builder setOfferOrderCount(int value) {
offerOrderCount_ = value;
bitField0_ |= 0x00000100;
onChanged();
return this;
}
/**
* sint32 offerOrderCount = 22;
* @return This builder for chaining.
*/
public Builder clearOfferOrderCount() {
bitField0_ = (bitField0_ & ~0x00000100);
offerOrderCount_ = 0;
onChanged();
return this;
}
private com.google.protobuf.ByteString offerOriginator_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* / Liquidity provider information
* For Forex: BANK:CITY
* For Equities: EXCHANGE_MIC
*
*
* bytes offerOriginator = 23;
* @return The offerOriginator.
*/
@java.lang.Override
public com.google.protobuf.ByteString getOfferOriginator() {
return offerOriginator_;
}
/**
*
* / Liquidity provider information
* For Forex: BANK:CITY
* For Equities: EXCHANGE_MIC
*
*
* bytes offerOriginator = 23;
* @param value The offerOriginator to set.
* @return This builder for chaining.
*/
public Builder setOfferOriginator(com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
offerOriginator_ = value;
bitField0_ |= 0x00000200;
onChanged();
return this;
}
/**
*
* / Liquidity provider information
* For Forex: BANK:CITY
* For Equities: EXCHANGE_MIC
*
*
* bytes offerOriginator = 23;
* @return This builder for chaining.
*/
public Builder clearOfferOriginator() {
bitField0_ = (bitField0_ & ~0x00000200);
offerOriginator_ = getDefaultInstance().getOfferOriginator();
onChanged();
return this;
}
private com.google.protobuf.ByteString offerQuoteCondition_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes offerQuoteCondition = 24;
* @return The offerQuoteCondition.
*/
@java.lang.Override
public com.google.protobuf.ByteString getOfferQuoteCondition() {
return offerQuoteCondition_;
}
/**
* bytes offerQuoteCondition = 24;
* @param value The offerQuoteCondition to set.
* @return This builder for chaining.
*/
public Builder setOfferQuoteCondition(com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
offerQuoteCondition_ = value;
bitField0_ |= 0x00000400;
onChanged();
return this;
}
/**
* bytes offerQuoteCondition = 24;
* @return This builder for chaining.
*/
public Builder clearOfferQuoteCondition() {
bitField0_ = (bitField0_ & ~0x00000400);
offerQuoteCondition_ = getDefaultInstance().getOfferQuoteCondition();
onChanged();
return this;
}
private com.google.protobuf.ByteString quoteCondition_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes quoteCondition = 30;
* @return The quoteCondition.
*/
@java.lang.Override
public com.google.protobuf.ByteString getQuoteCondition() {
return quoteCondition_;
}
/**
* bytes quoteCondition = 30;
* @param value The quoteCondition to set.
* @return This builder for chaining.
*/
public Builder setQuoteCondition(com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
quoteCondition_ = value;
bitField0_ |= 0x00000800;
onChanged();
return this;
}
/**
* bytes quoteCondition = 30;
* @return This builder for chaining.
*/
public Builder clearQuoteCondition() {
bitField0_ = (bitField0_ & ~0x00000800);
quoteCondition_ = getDefaultInstance().getQuoteCondition();
onChanged();
return this;
}
private boolean regional_ ;
/**
*
* / True if regional/participant member quote
*
*
* bool regional = 32;
* @return The regional.
*/
@java.lang.Override
public boolean getRegional() {
return regional_;
}
/**
*
* / True if regional/participant member quote
*
*
* bool regional = 32;
* @param value The regional to set.
* @return This builder for chaining.
*/
public Builder setRegional(boolean value) {
regional_ = value;
bitField0_ |= 0x00001000;
onChanged();
return this;
}
/**
*
* / True if regional/participant member quote
*
*
* bool regional = 32;
* @return This builder for chaining.
*/
public Builder clearRegional() {
bitField0_ = (bitField0_ & ~0x00001000);
regional_ = false;
onChanged();
return this;
}
private boolean transient_ ;
/**
*
* / True if not persisted in the EOD database.
*
*
* bool transient = 33;
* @return The transient.
*/
@java.lang.Override
public boolean getTransient() {
return transient_;
}
/**
*
* / True if not persisted in the EOD database.
*
*
* bool transient = 33;
* @param value The transient to set.
* @return This builder for chaining.
*/
public Builder setTransient(boolean value) {
transient_ = value;
bitField0_ |= 0x00002000;
onChanged();
return this;
}
/**
*
* / True if not persisted in the EOD database.
*
*
* bool transient = 33;
* @return This builder for chaining.
*/
public Builder clearTransient() {
bitField0_ = (bitField0_ & ~0x00002000);
transient_ = false;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:org.openfeed.BestBidOffer)
}
// @@protoc_insertion_point(class_scope:org.openfeed.BestBidOffer)
private static final org.openfeed.BestBidOffer DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.openfeed.BestBidOffer();
}
public static org.openfeed.BestBidOffer getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public BestBidOffer parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.openfeed.BestBidOffer getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy