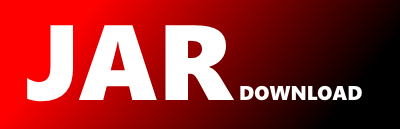
org.openfeed.IndexValue Maven / Gradle / Ivy
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// NO CHECKED-IN PROTOBUF GENCODE
// source: openfeed.proto
// Protobuf Java Version: 4.28.3
package org.openfeed;
/**
*
* / For non-tradable index products
*
*
* Protobuf type {@code org.openfeed.IndexValue}
*/
public final class IndexValue extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:org.openfeed.IndexValue)
IndexValueOrBuilder {
private static final long serialVersionUID = 0L;
static {
com.google.protobuf.RuntimeVersion.validateProtobufGencodeVersion(
com.google.protobuf.RuntimeVersion.RuntimeDomain.PUBLIC,
/* major= */ 4,
/* minor= */ 28,
/* patch= */ 3,
/* suffix= */ "",
IndexValue.class.getName());
}
// Use IndexValue.newBuilder() to construct.
private IndexValue(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private IndexValue() {
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.openfeed.Openfeed.internal_static_org_openfeed_IndexValue_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.openfeed.Openfeed.internal_static_org_openfeed_IndexValue_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.openfeed.IndexValue.class, org.openfeed.IndexValue.Builder.class);
}
public static final int TRANSACTIONTIME_FIELD_NUMBER = 9;
private long transactionTime_ = 0L;
/**
*
* UTC Timestamp, nano seconds since Unix epoch
*
*
* sint64 transactionTime = 9;
* @return The transactionTime.
*/
@java.lang.Override
public long getTransactionTime() {
return transactionTime_;
}
public static final int TRADEDATE_FIELD_NUMBER = 10;
private int tradeDate_ = 0;
/**
*
* Date only, format 2012-07-04 -> 20120704
*
*
* sint32 tradeDate = 10;
* @return The tradeDate.
*/
@java.lang.Override
public int getTradeDate() {
return tradeDate_;
}
public static final int LAST_FIELD_NUMBER = 11;
private long last_ = 0L;
/**
* sint64 last = 11;
* @return The last.
*/
@java.lang.Override
public long getLast() {
return last_;
}
public static final int VOLUME_FIELD_NUMBER = 12;
private long volume_ = 0L;
/**
* sint64 volume = 12;
* @return The volume.
*/
@java.lang.Override
public long getVolume() {
return volume_;
}
public static final int OPEN_FIELD_NUMBER = 13;
private long open_ = 0L;
/**
* sint64 open = 13;
* @return The open.
*/
@java.lang.Override
public long getOpen() {
return open_;
}
public static final int SETTLEMENTOPEN_FIELD_NUMBER = 14;
private long settlementOpen_ = 0L;
/**
* sint64 settlementOpen = 14;
* @return The settlementOpen.
*/
@java.lang.Override
public long getSettlementOpen() {
return settlementOpen_;
}
public static final int SPECIALOPEN_FIELD_NUMBER = 15;
private long specialOpen_ = 0L;
/**
* sint64 specialOpen = 15;
* @return The specialOpen.
*/
@java.lang.Override
public long getSpecialOpen() {
return specialOpen_;
}
public static final int HIGH_FIELD_NUMBER = 16;
private long high_ = 0L;
/**
* sint64 high = 16;
* @return The high.
*/
@java.lang.Override
public long getHigh() {
return high_;
}
public static final int LOW_FIELD_NUMBER = 17;
private long low_ = 0L;
/**
* sint64 low = 17;
* @return The low.
*/
@java.lang.Override
public long getLow() {
return low_;
}
public static final int CLOSE_FIELD_NUMBER = 18;
private long close_ = 0L;
/**
* sint64 close = 18;
* @return The close.
*/
@java.lang.Override
public long getClose() {
return close_;
}
public static final int BID_FIELD_NUMBER = 19;
private long bid_ = 0L;
/**
* sint64 bid = 19;
* @return The bid.
*/
@java.lang.Override
public long getBid() {
return bid_;
}
public static final int OFFER_FIELD_NUMBER = 20;
private long offer_ = 0L;
/**
* sint64 offer = 20;
* @return The offer.
*/
@java.lang.Override
public long getOffer() {
return offer_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (transactionTime_ != 0L) {
output.writeSInt64(9, transactionTime_);
}
if (tradeDate_ != 0) {
output.writeSInt32(10, tradeDate_);
}
if (last_ != 0L) {
output.writeSInt64(11, last_);
}
if (volume_ != 0L) {
output.writeSInt64(12, volume_);
}
if (open_ != 0L) {
output.writeSInt64(13, open_);
}
if (settlementOpen_ != 0L) {
output.writeSInt64(14, settlementOpen_);
}
if (specialOpen_ != 0L) {
output.writeSInt64(15, specialOpen_);
}
if (high_ != 0L) {
output.writeSInt64(16, high_);
}
if (low_ != 0L) {
output.writeSInt64(17, low_);
}
if (close_ != 0L) {
output.writeSInt64(18, close_);
}
if (bid_ != 0L) {
output.writeSInt64(19, bid_);
}
if (offer_ != 0L) {
output.writeSInt64(20, offer_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (transactionTime_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeSInt64Size(9, transactionTime_);
}
if (tradeDate_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeSInt32Size(10, tradeDate_);
}
if (last_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeSInt64Size(11, last_);
}
if (volume_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeSInt64Size(12, volume_);
}
if (open_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeSInt64Size(13, open_);
}
if (settlementOpen_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeSInt64Size(14, settlementOpen_);
}
if (specialOpen_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeSInt64Size(15, specialOpen_);
}
if (high_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeSInt64Size(16, high_);
}
if (low_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeSInt64Size(17, low_);
}
if (close_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeSInt64Size(18, close_);
}
if (bid_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeSInt64Size(19, bid_);
}
if (offer_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeSInt64Size(20, offer_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.openfeed.IndexValue)) {
return super.equals(obj);
}
org.openfeed.IndexValue other = (org.openfeed.IndexValue) obj;
if (getTransactionTime()
!= other.getTransactionTime()) return false;
if (getTradeDate()
!= other.getTradeDate()) return false;
if (getLast()
!= other.getLast()) return false;
if (getVolume()
!= other.getVolume()) return false;
if (getOpen()
!= other.getOpen()) return false;
if (getSettlementOpen()
!= other.getSettlementOpen()) return false;
if (getSpecialOpen()
!= other.getSpecialOpen()) return false;
if (getHigh()
!= other.getHigh()) return false;
if (getLow()
!= other.getLow()) return false;
if (getClose()
!= other.getClose()) return false;
if (getBid()
!= other.getBid()) return false;
if (getOffer()
!= other.getOffer()) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + TRANSACTIONTIME_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getTransactionTime());
hash = (37 * hash) + TRADEDATE_FIELD_NUMBER;
hash = (53 * hash) + getTradeDate();
hash = (37 * hash) + LAST_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getLast());
hash = (37 * hash) + VOLUME_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getVolume());
hash = (37 * hash) + OPEN_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getOpen());
hash = (37 * hash) + SETTLEMENTOPEN_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getSettlementOpen());
hash = (37 * hash) + SPECIALOPEN_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getSpecialOpen());
hash = (37 * hash) + HIGH_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getHigh());
hash = (37 * hash) + LOW_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getLow());
hash = (37 * hash) + CLOSE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getClose());
hash = (37 * hash) + BID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getBid());
hash = (37 * hash) + OFFER_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getOffer());
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.openfeed.IndexValue parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.openfeed.IndexValue parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.openfeed.IndexValue parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.openfeed.IndexValue parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.openfeed.IndexValue parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.openfeed.IndexValue parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.openfeed.IndexValue parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static org.openfeed.IndexValue parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.openfeed.IndexValue parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input);
}
public static org.openfeed.IndexValue parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.openfeed.IndexValue parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static org.openfeed.IndexValue parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.openfeed.IndexValue prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* / For non-tradable index products
*
*
* Protobuf type {@code org.openfeed.IndexValue}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:org.openfeed.IndexValue)
org.openfeed.IndexValueOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.openfeed.Openfeed.internal_static_org_openfeed_IndexValue_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.openfeed.Openfeed.internal_static_org_openfeed_IndexValue_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.openfeed.IndexValue.class, org.openfeed.IndexValue.Builder.class);
}
// Construct using org.openfeed.IndexValue.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
transactionTime_ = 0L;
tradeDate_ = 0;
last_ = 0L;
volume_ = 0L;
open_ = 0L;
settlementOpen_ = 0L;
specialOpen_ = 0L;
high_ = 0L;
low_ = 0L;
close_ = 0L;
bid_ = 0L;
offer_ = 0L;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.openfeed.Openfeed.internal_static_org_openfeed_IndexValue_descriptor;
}
@java.lang.Override
public org.openfeed.IndexValue getDefaultInstanceForType() {
return org.openfeed.IndexValue.getDefaultInstance();
}
@java.lang.Override
public org.openfeed.IndexValue build() {
org.openfeed.IndexValue result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.openfeed.IndexValue buildPartial() {
org.openfeed.IndexValue result = new org.openfeed.IndexValue(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(org.openfeed.IndexValue result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.transactionTime_ = transactionTime_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.tradeDate_ = tradeDate_;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.last_ = last_;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.volume_ = volume_;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.open_ = open_;
}
if (((from_bitField0_ & 0x00000020) != 0)) {
result.settlementOpen_ = settlementOpen_;
}
if (((from_bitField0_ & 0x00000040) != 0)) {
result.specialOpen_ = specialOpen_;
}
if (((from_bitField0_ & 0x00000080) != 0)) {
result.high_ = high_;
}
if (((from_bitField0_ & 0x00000100) != 0)) {
result.low_ = low_;
}
if (((from_bitField0_ & 0x00000200) != 0)) {
result.close_ = close_;
}
if (((from_bitField0_ & 0x00000400) != 0)) {
result.bid_ = bid_;
}
if (((from_bitField0_ & 0x00000800) != 0)) {
result.offer_ = offer_;
}
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.openfeed.IndexValue) {
return mergeFrom((org.openfeed.IndexValue)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.openfeed.IndexValue other) {
if (other == org.openfeed.IndexValue.getDefaultInstance()) return this;
if (other.getTransactionTime() != 0L) {
setTransactionTime(other.getTransactionTime());
}
if (other.getTradeDate() != 0) {
setTradeDate(other.getTradeDate());
}
if (other.getLast() != 0L) {
setLast(other.getLast());
}
if (other.getVolume() != 0L) {
setVolume(other.getVolume());
}
if (other.getOpen() != 0L) {
setOpen(other.getOpen());
}
if (other.getSettlementOpen() != 0L) {
setSettlementOpen(other.getSettlementOpen());
}
if (other.getSpecialOpen() != 0L) {
setSpecialOpen(other.getSpecialOpen());
}
if (other.getHigh() != 0L) {
setHigh(other.getHigh());
}
if (other.getLow() != 0L) {
setLow(other.getLow());
}
if (other.getClose() != 0L) {
setClose(other.getClose());
}
if (other.getBid() != 0L) {
setBid(other.getBid());
}
if (other.getOffer() != 0L) {
setOffer(other.getOffer());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 72: {
transactionTime_ = input.readSInt64();
bitField0_ |= 0x00000001;
break;
} // case 72
case 80: {
tradeDate_ = input.readSInt32();
bitField0_ |= 0x00000002;
break;
} // case 80
case 88: {
last_ = input.readSInt64();
bitField0_ |= 0x00000004;
break;
} // case 88
case 96: {
volume_ = input.readSInt64();
bitField0_ |= 0x00000008;
break;
} // case 96
case 104: {
open_ = input.readSInt64();
bitField0_ |= 0x00000010;
break;
} // case 104
case 112: {
settlementOpen_ = input.readSInt64();
bitField0_ |= 0x00000020;
break;
} // case 112
case 120: {
specialOpen_ = input.readSInt64();
bitField0_ |= 0x00000040;
break;
} // case 120
case 128: {
high_ = input.readSInt64();
bitField0_ |= 0x00000080;
break;
} // case 128
case 136: {
low_ = input.readSInt64();
bitField0_ |= 0x00000100;
break;
} // case 136
case 144: {
close_ = input.readSInt64();
bitField0_ |= 0x00000200;
break;
} // case 144
case 152: {
bid_ = input.readSInt64();
bitField0_ |= 0x00000400;
break;
} // case 152
case 160: {
offer_ = input.readSInt64();
bitField0_ |= 0x00000800;
break;
} // case 160
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private long transactionTime_ ;
/**
*
* UTC Timestamp, nano seconds since Unix epoch
*
*
* sint64 transactionTime = 9;
* @return The transactionTime.
*/
@java.lang.Override
public long getTransactionTime() {
return transactionTime_;
}
/**
*
* UTC Timestamp, nano seconds since Unix epoch
*
*
* sint64 transactionTime = 9;
* @param value The transactionTime to set.
* @return This builder for chaining.
*/
public Builder setTransactionTime(long value) {
transactionTime_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* UTC Timestamp, nano seconds since Unix epoch
*
*
* sint64 transactionTime = 9;
* @return This builder for chaining.
*/
public Builder clearTransactionTime() {
bitField0_ = (bitField0_ & ~0x00000001);
transactionTime_ = 0L;
onChanged();
return this;
}
private int tradeDate_ ;
/**
*
* Date only, format 2012-07-04 -> 20120704
*
*
* sint32 tradeDate = 10;
* @return The tradeDate.
*/
@java.lang.Override
public int getTradeDate() {
return tradeDate_;
}
/**
*
* Date only, format 2012-07-04 -> 20120704
*
*
* sint32 tradeDate = 10;
* @param value The tradeDate to set.
* @return This builder for chaining.
*/
public Builder setTradeDate(int value) {
tradeDate_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
* Date only, format 2012-07-04 -> 20120704
*
*
* sint32 tradeDate = 10;
* @return This builder for chaining.
*/
public Builder clearTradeDate() {
bitField0_ = (bitField0_ & ~0x00000002);
tradeDate_ = 0;
onChanged();
return this;
}
private long last_ ;
/**
* sint64 last = 11;
* @return The last.
*/
@java.lang.Override
public long getLast() {
return last_;
}
/**
* sint64 last = 11;
* @param value The last to set.
* @return This builder for chaining.
*/
public Builder setLast(long value) {
last_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
* sint64 last = 11;
* @return This builder for chaining.
*/
public Builder clearLast() {
bitField0_ = (bitField0_ & ~0x00000004);
last_ = 0L;
onChanged();
return this;
}
private long volume_ ;
/**
* sint64 volume = 12;
* @return The volume.
*/
@java.lang.Override
public long getVolume() {
return volume_;
}
/**
* sint64 volume = 12;
* @param value The volume to set.
* @return This builder for chaining.
*/
public Builder setVolume(long value) {
volume_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
* sint64 volume = 12;
* @return This builder for chaining.
*/
public Builder clearVolume() {
bitField0_ = (bitField0_ & ~0x00000008);
volume_ = 0L;
onChanged();
return this;
}
private long open_ ;
/**
* sint64 open = 13;
* @return The open.
*/
@java.lang.Override
public long getOpen() {
return open_;
}
/**
* sint64 open = 13;
* @param value The open to set.
* @return This builder for chaining.
*/
public Builder setOpen(long value) {
open_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
* sint64 open = 13;
* @return This builder for chaining.
*/
public Builder clearOpen() {
bitField0_ = (bitField0_ & ~0x00000010);
open_ = 0L;
onChanged();
return this;
}
private long settlementOpen_ ;
/**
* sint64 settlementOpen = 14;
* @return The settlementOpen.
*/
@java.lang.Override
public long getSettlementOpen() {
return settlementOpen_;
}
/**
* sint64 settlementOpen = 14;
* @param value The settlementOpen to set.
* @return This builder for chaining.
*/
public Builder setSettlementOpen(long value) {
settlementOpen_ = value;
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
* sint64 settlementOpen = 14;
* @return This builder for chaining.
*/
public Builder clearSettlementOpen() {
bitField0_ = (bitField0_ & ~0x00000020);
settlementOpen_ = 0L;
onChanged();
return this;
}
private long specialOpen_ ;
/**
* sint64 specialOpen = 15;
* @return The specialOpen.
*/
@java.lang.Override
public long getSpecialOpen() {
return specialOpen_;
}
/**
* sint64 specialOpen = 15;
* @param value The specialOpen to set.
* @return This builder for chaining.
*/
public Builder setSpecialOpen(long value) {
specialOpen_ = value;
bitField0_ |= 0x00000040;
onChanged();
return this;
}
/**
* sint64 specialOpen = 15;
* @return This builder for chaining.
*/
public Builder clearSpecialOpen() {
bitField0_ = (bitField0_ & ~0x00000040);
specialOpen_ = 0L;
onChanged();
return this;
}
private long high_ ;
/**
* sint64 high = 16;
* @return The high.
*/
@java.lang.Override
public long getHigh() {
return high_;
}
/**
* sint64 high = 16;
* @param value The high to set.
* @return This builder for chaining.
*/
public Builder setHigh(long value) {
high_ = value;
bitField0_ |= 0x00000080;
onChanged();
return this;
}
/**
* sint64 high = 16;
* @return This builder for chaining.
*/
public Builder clearHigh() {
bitField0_ = (bitField0_ & ~0x00000080);
high_ = 0L;
onChanged();
return this;
}
private long low_ ;
/**
* sint64 low = 17;
* @return The low.
*/
@java.lang.Override
public long getLow() {
return low_;
}
/**
* sint64 low = 17;
* @param value The low to set.
* @return This builder for chaining.
*/
public Builder setLow(long value) {
low_ = value;
bitField0_ |= 0x00000100;
onChanged();
return this;
}
/**
* sint64 low = 17;
* @return This builder for chaining.
*/
public Builder clearLow() {
bitField0_ = (bitField0_ & ~0x00000100);
low_ = 0L;
onChanged();
return this;
}
private long close_ ;
/**
* sint64 close = 18;
* @return The close.
*/
@java.lang.Override
public long getClose() {
return close_;
}
/**
* sint64 close = 18;
* @param value The close to set.
* @return This builder for chaining.
*/
public Builder setClose(long value) {
close_ = value;
bitField0_ |= 0x00000200;
onChanged();
return this;
}
/**
* sint64 close = 18;
* @return This builder for chaining.
*/
public Builder clearClose() {
bitField0_ = (bitField0_ & ~0x00000200);
close_ = 0L;
onChanged();
return this;
}
private long bid_ ;
/**
* sint64 bid = 19;
* @return The bid.
*/
@java.lang.Override
public long getBid() {
return bid_;
}
/**
* sint64 bid = 19;
* @param value The bid to set.
* @return This builder for chaining.
*/
public Builder setBid(long value) {
bid_ = value;
bitField0_ |= 0x00000400;
onChanged();
return this;
}
/**
* sint64 bid = 19;
* @return This builder for chaining.
*/
public Builder clearBid() {
bitField0_ = (bitField0_ & ~0x00000400);
bid_ = 0L;
onChanged();
return this;
}
private long offer_ ;
/**
* sint64 offer = 20;
* @return The offer.
*/
@java.lang.Override
public long getOffer() {
return offer_;
}
/**
* sint64 offer = 20;
* @param value The offer to set.
* @return This builder for chaining.
*/
public Builder setOffer(long value) {
offer_ = value;
bitField0_ |= 0x00000800;
onChanged();
return this;
}
/**
* sint64 offer = 20;
* @return This builder for chaining.
*/
public Builder clearOffer() {
bitField0_ = (bitField0_ & ~0x00000800);
offer_ = 0L;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:org.openfeed.IndexValue)
}
// @@protoc_insertion_point(class_scope:org.openfeed.IndexValue)
private static final org.openfeed.IndexValue DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.openfeed.IndexValue();
}
public static org.openfeed.IndexValue getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public IndexValue parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.openfeed.IndexValue getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy