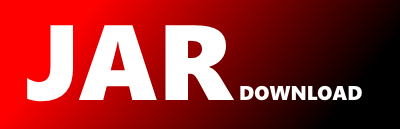
org.openfeed.InstrumentDefinition Maven / Gradle / Ivy
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// NO CHECKED-IN PROTOBUF GENCODE
// source: openfeed_instrument.proto
// Protobuf Java Version: 4.28.3
package org.openfeed;
/**
* Protobuf type {@code org.openfeed.InstrumentDefinition}
*/
public final class InstrumentDefinition extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:org.openfeed.InstrumentDefinition)
InstrumentDefinitionOrBuilder {
private static final long serialVersionUID = 0L;
static {
com.google.protobuf.RuntimeVersion.validateProtobufGencodeVersion(
com.google.protobuf.RuntimeVersion.RuntimeDomain.PUBLIC,
/* major= */ 4,
/* minor= */ 28,
/* patch= */ 3,
/* suffix= */ "",
InstrumentDefinition.class.getName());
}
// Use InstrumentDefinition.newBuilder() to construct.
private InstrumentDefinition(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private InstrumentDefinition() {
instrumentType_ = 0;
supportBookTypes_ = emptyIntList();
vendorId_ = "";
symbol_ = "";
description_ = "";
cfiCode_ = "";
currencyCode_ = "";
exchangeCode_ = "";
timeZoneName_ = "";
instrumentGroup_ = "";
state_ = 0;
auxiliaryData_ = com.google.protobuf.ByteString.EMPTY;
symbols_ = java.util.Collections.emptyList();
optionType_ = 0;
optionStyle_ = 0;
spreadCode_ = "";
spreadLeg_ = java.util.Collections.emptyList();
marketTier_ = "";
financialStatusIndicator_ = "";
isin_ = "";
barchartExchangeCode_ = "";
barchartBaseCode_ = "";
primaryListingMarketParticipantId_ = "";
subscriptionSymbol_ = "";
underlying_ = "";
commodity_ = "";
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.openfeed.OpenfeedInstrument.internal_static_org_openfeed_InstrumentDefinition_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.openfeed.OpenfeedInstrument.internal_static_org_openfeed_InstrumentDefinition_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.openfeed.InstrumentDefinition.class, org.openfeed.InstrumentDefinition.Builder.class);
}
/**
*
* #############################################
*
*
* Protobuf enum {@code org.openfeed.InstrumentDefinition.InstrumentType}
*/
public enum InstrumentType
implements com.google.protobuf.ProtocolMessageEnum {
/**
* UNKNOWN_INSTRUMENT_TYPE = 0;
*/
UNKNOWN_INSTRUMENT_TYPE(0),
/**
* FOREX = 1;
*/
FOREX(1),
/**
* INDEX = 2;
*/
INDEX(2),
/**
* EQUITY = 3;
*/
EQUITY(3),
/**
* FUTURE = 4;
*/
FUTURE(4),
/**
* OPTION = 5;
*/
OPTION(5),
/**
* SPREAD = 6;
*/
SPREAD(6),
/**
* MUTUAL_FUND = 7;
*/
MUTUAL_FUND(7),
/**
* MONEY_MARKET_FUND = 8;
*/
MONEY_MARKET_FUND(8),
/**
* USER_DEFINED_SPREAD = 9;
*/
USER_DEFINED_SPREAD(9),
/**
* EQUITY_OPTION = 10;
*/
EQUITY_OPTION(10),
/**
* OTHER = 99;
*/
OTHER(99),
UNRECOGNIZED(-1),
;
static {
com.google.protobuf.RuntimeVersion.validateProtobufGencodeVersion(
com.google.protobuf.RuntimeVersion.RuntimeDomain.PUBLIC,
/* major= */ 4,
/* minor= */ 28,
/* patch= */ 3,
/* suffix= */ "",
InstrumentType.class.getName());
}
/**
* UNKNOWN_INSTRUMENT_TYPE = 0;
*/
public static final int UNKNOWN_INSTRUMENT_TYPE_VALUE = 0;
/**
* FOREX = 1;
*/
public static final int FOREX_VALUE = 1;
/**
* INDEX = 2;
*/
public static final int INDEX_VALUE = 2;
/**
* EQUITY = 3;
*/
public static final int EQUITY_VALUE = 3;
/**
* FUTURE = 4;
*/
public static final int FUTURE_VALUE = 4;
/**
* OPTION = 5;
*/
public static final int OPTION_VALUE = 5;
/**
* SPREAD = 6;
*/
public static final int SPREAD_VALUE = 6;
/**
* MUTUAL_FUND = 7;
*/
public static final int MUTUAL_FUND_VALUE = 7;
/**
* MONEY_MARKET_FUND = 8;
*/
public static final int MONEY_MARKET_FUND_VALUE = 8;
/**
* USER_DEFINED_SPREAD = 9;
*/
public static final int USER_DEFINED_SPREAD_VALUE = 9;
/**
* EQUITY_OPTION = 10;
*/
public static final int EQUITY_OPTION_VALUE = 10;
/**
* OTHER = 99;
*/
public static final int OTHER_VALUE = 99;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static InstrumentType valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static InstrumentType forNumber(int value) {
switch (value) {
case 0: return UNKNOWN_INSTRUMENT_TYPE;
case 1: return FOREX;
case 2: return INDEX;
case 3: return EQUITY;
case 4: return FUTURE;
case 5: return OPTION;
case 6: return SPREAD;
case 7: return MUTUAL_FUND;
case 8: return MONEY_MARKET_FUND;
case 9: return USER_DEFINED_SPREAD;
case 10: return EQUITY_OPTION;
case 99: return OTHER;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
InstrumentType> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public InstrumentType findValueByNumber(int number) {
return InstrumentType.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return org.openfeed.InstrumentDefinition.getDescriptor().getEnumTypes().get(0);
}
private static final InstrumentType[] VALUES = values();
public static InstrumentType valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private InstrumentType(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:org.openfeed.InstrumentDefinition.InstrumentType)
}
/**
*
* / Market depth implementation type
*
*
* Protobuf enum {@code org.openfeed.InstrumentDefinition.BookType}
*/
public enum BookType
implements com.google.protobuf.ProtocolMessageEnum {
/**
* UNKNOWN_BOOK_TYPE = 0;
*/
UNKNOWN_BOOK_TYPE(0),
/**
*
* BBO
*
*
* TOP_OF_BOOK = 1;
*/
TOP_OF_BOOK(1),
/**
*
* Book uses price level
*
*
* PRICE_LEVEL_DEPTH = 2;
*/
PRICE_LEVEL_DEPTH(2),
/**
*
* Book uses order-id
*
*
* ORDER_DEPTH = 3;
*/
ORDER_DEPTH(3),
UNRECOGNIZED(-1),
;
static {
com.google.protobuf.RuntimeVersion.validateProtobufGencodeVersion(
com.google.protobuf.RuntimeVersion.RuntimeDomain.PUBLIC,
/* major= */ 4,
/* minor= */ 28,
/* patch= */ 3,
/* suffix= */ "",
BookType.class.getName());
}
/**
* UNKNOWN_BOOK_TYPE = 0;
*/
public static final int UNKNOWN_BOOK_TYPE_VALUE = 0;
/**
*
* BBO
*
*
* TOP_OF_BOOK = 1;
*/
public static final int TOP_OF_BOOK_VALUE = 1;
/**
*
* Book uses price level
*
*
* PRICE_LEVEL_DEPTH = 2;
*/
public static final int PRICE_LEVEL_DEPTH_VALUE = 2;
/**
*
* Book uses order-id
*
*
* ORDER_DEPTH = 3;
*/
public static final int ORDER_DEPTH_VALUE = 3;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static BookType valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static BookType forNumber(int value) {
switch (value) {
case 0: return UNKNOWN_BOOK_TYPE;
case 1: return TOP_OF_BOOK;
case 2: return PRICE_LEVEL_DEPTH;
case 3: return ORDER_DEPTH;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
BookType> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public BookType findValueByNumber(int number) {
return BookType.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return org.openfeed.InstrumentDefinition.getDescriptor().getEnumTypes().get(1);
}
private static final BookType[] VALUES = values();
public static BookType valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private BookType(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:org.openfeed.InstrumentDefinition.BookType)
}
/**
*
* / Option type.
*
*
* Protobuf enum {@code org.openfeed.InstrumentDefinition.OptionType}
*/
public enum OptionType
implements com.google.protobuf.ProtocolMessageEnum {
/**
* UNKNOWN_OPTION_TYPE = 0;
*/
UNKNOWN_OPTION_TYPE(0),
/**
* CALL = 1;
*/
CALL(1),
/**
* PUT = 2;
*/
PUT(2),
UNRECOGNIZED(-1),
;
static {
com.google.protobuf.RuntimeVersion.validateProtobufGencodeVersion(
com.google.protobuf.RuntimeVersion.RuntimeDomain.PUBLIC,
/* major= */ 4,
/* minor= */ 28,
/* patch= */ 3,
/* suffix= */ "",
OptionType.class.getName());
}
/**
* UNKNOWN_OPTION_TYPE = 0;
*/
public static final int UNKNOWN_OPTION_TYPE_VALUE = 0;
/**
* CALL = 1;
*/
public static final int CALL_VALUE = 1;
/**
* PUT = 2;
*/
public static final int PUT_VALUE = 2;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static OptionType valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static OptionType forNumber(int value) {
switch (value) {
case 0: return UNKNOWN_OPTION_TYPE;
case 1: return CALL;
case 2: return PUT;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
OptionType> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public OptionType findValueByNumber(int number) {
return OptionType.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return org.openfeed.InstrumentDefinition.getDescriptor().getEnumTypes().get(2);
}
private static final OptionType[] VALUES = values();
public static OptionType valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private OptionType(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:org.openfeed.InstrumentDefinition.OptionType)
}
/**
*
* / Option style.
*
*
* Protobuf enum {@code org.openfeed.InstrumentDefinition.OptionStyle}
*/
public enum OptionStyle
implements com.google.protobuf.ProtocolMessageEnum {
/**
* UNKNOWN_OPTIONS_STYLE = 0;
*/
UNKNOWN_OPTIONS_STYLE(0),
/**
* DEFAULT = 1;
*/
DEFAULT(1),
/**
* AMERICAN = 2;
*/
AMERICAN(2),
/**
* EUROPEAN = 3;
*/
EUROPEAN(3),
UNRECOGNIZED(-1),
;
static {
com.google.protobuf.RuntimeVersion.validateProtobufGencodeVersion(
com.google.protobuf.RuntimeVersion.RuntimeDomain.PUBLIC,
/* major= */ 4,
/* minor= */ 28,
/* patch= */ 3,
/* suffix= */ "",
OptionStyle.class.getName());
}
/**
* UNKNOWN_OPTIONS_STYLE = 0;
*/
public static final int UNKNOWN_OPTIONS_STYLE_VALUE = 0;
/**
* DEFAULT = 1;
*/
public static final int DEFAULT_VALUE = 1;
/**
* AMERICAN = 2;
*/
public static final int AMERICAN_VALUE = 2;
/**
* EUROPEAN = 3;
*/
public static final int EUROPEAN_VALUE = 3;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static OptionStyle valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static OptionStyle forNumber(int value) {
switch (value) {
case 0: return UNKNOWN_OPTIONS_STYLE;
case 1: return DEFAULT;
case 2: return AMERICAN;
case 3: return EUROPEAN;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
OptionStyle> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public OptionStyle findValueByNumber(int number) {
return OptionStyle.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return org.openfeed.InstrumentDefinition.getDescriptor().getEnumTypes().get(3);
}
private static final OptionStyle[] VALUES = values();
public static OptionStyle valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private OptionStyle(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:org.openfeed.InstrumentDefinition.OptionStyle)
}
/**
* Protobuf enum {@code org.openfeed.InstrumentDefinition.State}
*/
public enum State
implements com.google.protobuf.ProtocolMessageEnum {
/**
* UNKNOWN_STATE = 0;
*/
UNKNOWN_STATE(0),
/**
* ACTIVE = 1;
*/
ACTIVE(1),
/**
* PASSIVE = 2;
*/
PASSIVE(2),
UNRECOGNIZED(-1),
;
static {
com.google.protobuf.RuntimeVersion.validateProtobufGencodeVersion(
com.google.protobuf.RuntimeVersion.RuntimeDomain.PUBLIC,
/* major= */ 4,
/* minor= */ 28,
/* patch= */ 3,
/* suffix= */ "",
State.class.getName());
}
/**
* UNKNOWN_STATE = 0;
*/
public static final int UNKNOWN_STATE_VALUE = 0;
/**
* ACTIVE = 1;
*/
public static final int ACTIVE_VALUE = 1;
/**
* PASSIVE = 2;
*/
public static final int PASSIVE_VALUE = 2;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static State valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static State forNumber(int value) {
switch (value) {
case 0: return UNKNOWN_STATE;
case 1: return ACTIVE;
case 2: return PASSIVE;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
State> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public State findValueByNumber(int number) {
return State.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return org.openfeed.InstrumentDefinition.getDescriptor().getEnumTypes().get(4);
}
private static final State[] VALUES = values();
public static State valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private State(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:org.openfeed.InstrumentDefinition.State)
}
/**
* Protobuf enum {@code org.openfeed.InstrumentDefinition.EventType}
*/
public enum EventType
implements com.google.protobuf.ProtocolMessageEnum {
/**
* UNKNOWN_EVENT_TYPE = 0;
*/
UNKNOWN_EVENT_TYPE(0),
/**
*
* All instruments
*
*
* FIRST_TRADE_DATE = 1;
*/
FIRST_TRADE_DATE(1),
/**
* LAST_TRADE_DATE = 2;
*/
LAST_TRADE_DATE(2),
/**
*
* Futures only
*
*
* MATURITY_DATE = 10;
*/
MATURITY_DATE(10),
/**
* FIRST_DELIVERY_DATE = 11;
*/
FIRST_DELIVERY_DATE(11),
/**
* LAST_DELIVERY_DATE = 12;
*/
LAST_DELIVERY_DATE(12),
/**
* FIRST_NOTICE_DATE = 13;
*/
FIRST_NOTICE_DATE(13),
/**
* LAST_NOTICE_DATE = 14;
*/
LAST_NOTICE_DATE(14),
/**
* FIRST_HOLDING_DATE = 15;
*/
FIRST_HOLDING_DATE(15),
/**
* LAST_HOLDING_DATE = 16;
*/
LAST_HOLDING_DATE(16),
/**
* FIRST_POSITION_DATE = 17;
*/
FIRST_POSITION_DATE(17),
/**
* LAST_POSITION_DATE = 18;
*/
LAST_POSITION_DATE(18),
/**
*
* Grain Bids
*
*
* DELIVERY_START_DATE = 30;
*/
DELIVERY_START_DATE(30),
/**
* DELIVERY_END_DATE = 31;
*/
DELIVERY_END_DATE(31),
UNRECOGNIZED(-1),
;
static {
com.google.protobuf.RuntimeVersion.validateProtobufGencodeVersion(
com.google.protobuf.RuntimeVersion.RuntimeDomain.PUBLIC,
/* major= */ 4,
/* minor= */ 28,
/* patch= */ 3,
/* suffix= */ "",
EventType.class.getName());
}
/**
* UNKNOWN_EVENT_TYPE = 0;
*/
public static final int UNKNOWN_EVENT_TYPE_VALUE = 0;
/**
*
* All instruments
*
*
* FIRST_TRADE_DATE = 1;
*/
public static final int FIRST_TRADE_DATE_VALUE = 1;
/**
* LAST_TRADE_DATE = 2;
*/
public static final int LAST_TRADE_DATE_VALUE = 2;
/**
*
* Futures only
*
*
* MATURITY_DATE = 10;
*/
public static final int MATURITY_DATE_VALUE = 10;
/**
* FIRST_DELIVERY_DATE = 11;
*/
public static final int FIRST_DELIVERY_DATE_VALUE = 11;
/**
* LAST_DELIVERY_DATE = 12;
*/
public static final int LAST_DELIVERY_DATE_VALUE = 12;
/**
* FIRST_NOTICE_DATE = 13;
*/
public static final int FIRST_NOTICE_DATE_VALUE = 13;
/**
* LAST_NOTICE_DATE = 14;
*/
public static final int LAST_NOTICE_DATE_VALUE = 14;
/**
* FIRST_HOLDING_DATE = 15;
*/
public static final int FIRST_HOLDING_DATE_VALUE = 15;
/**
* LAST_HOLDING_DATE = 16;
*/
public static final int LAST_HOLDING_DATE_VALUE = 16;
/**
* FIRST_POSITION_DATE = 17;
*/
public static final int FIRST_POSITION_DATE_VALUE = 17;
/**
* LAST_POSITION_DATE = 18;
*/
public static final int LAST_POSITION_DATE_VALUE = 18;
/**
*
* Grain Bids
*
*
* DELIVERY_START_DATE = 30;
*/
public static final int DELIVERY_START_DATE_VALUE = 30;
/**
* DELIVERY_END_DATE = 31;
*/
public static final int DELIVERY_END_DATE_VALUE = 31;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static EventType valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static EventType forNumber(int value) {
switch (value) {
case 0: return UNKNOWN_EVENT_TYPE;
case 1: return FIRST_TRADE_DATE;
case 2: return LAST_TRADE_DATE;
case 10: return MATURITY_DATE;
case 11: return FIRST_DELIVERY_DATE;
case 12: return LAST_DELIVERY_DATE;
case 13: return FIRST_NOTICE_DATE;
case 14: return LAST_NOTICE_DATE;
case 15: return FIRST_HOLDING_DATE;
case 16: return LAST_HOLDING_DATE;
case 17: return FIRST_POSITION_DATE;
case 18: return LAST_POSITION_DATE;
case 30: return DELIVERY_START_DATE;
case 31: return DELIVERY_END_DATE;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
EventType> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public EventType findValueByNumber(int number) {
return EventType.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return org.openfeed.InstrumentDefinition.getDescriptor().getEnumTypes().get(5);
}
private static final EventType[] VALUES = values();
public static EventType valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private EventType(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:org.openfeed.InstrumentDefinition.EventType)
}
public interface ScheduleOrBuilder extends
// @@protoc_insertion_point(interface_extends:org.openfeed.InstrumentDefinition.Schedule)
com.google.protobuf.MessageOrBuilder {
/**
* repeated .org.openfeed.InstrumentDefinition.TimeSpan sessions = 1;
*/
java.util.List
getSessionsList();
/**
* repeated .org.openfeed.InstrumentDefinition.TimeSpan sessions = 1;
*/
org.openfeed.InstrumentDefinition.TimeSpan getSessions(int index);
/**
* repeated .org.openfeed.InstrumentDefinition.TimeSpan sessions = 1;
*/
int getSessionsCount();
/**
* repeated .org.openfeed.InstrumentDefinition.TimeSpan sessions = 1;
*/
java.util.List extends org.openfeed.InstrumentDefinition.TimeSpanOrBuilder>
getSessionsOrBuilderList();
/**
* repeated .org.openfeed.InstrumentDefinition.TimeSpan sessions = 1;
*/
org.openfeed.InstrumentDefinition.TimeSpanOrBuilder getSessionsOrBuilder(
int index);
}
/**
*
* / Typical trading week schedule
*
*
* Protobuf type {@code org.openfeed.InstrumentDefinition.Schedule}
*/
public static final class Schedule extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:org.openfeed.InstrumentDefinition.Schedule)
ScheduleOrBuilder {
private static final long serialVersionUID = 0L;
static {
com.google.protobuf.RuntimeVersion.validateProtobufGencodeVersion(
com.google.protobuf.RuntimeVersion.RuntimeDomain.PUBLIC,
/* major= */ 4,
/* minor= */ 28,
/* patch= */ 3,
/* suffix= */ "",
Schedule.class.getName());
}
// Use Schedule.newBuilder() to construct.
private Schedule(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private Schedule() {
sessions_ = java.util.Collections.emptyList();
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.openfeed.OpenfeedInstrument.internal_static_org_openfeed_InstrumentDefinition_Schedule_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.openfeed.OpenfeedInstrument.internal_static_org_openfeed_InstrumentDefinition_Schedule_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.openfeed.InstrumentDefinition.Schedule.class, org.openfeed.InstrumentDefinition.Schedule.Builder.class);
}
public static final int SESSIONS_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private java.util.List sessions_;
/**
* repeated .org.openfeed.InstrumentDefinition.TimeSpan sessions = 1;
*/
@java.lang.Override
public java.util.List getSessionsList() {
return sessions_;
}
/**
* repeated .org.openfeed.InstrumentDefinition.TimeSpan sessions = 1;
*/
@java.lang.Override
public java.util.List extends org.openfeed.InstrumentDefinition.TimeSpanOrBuilder>
getSessionsOrBuilderList() {
return sessions_;
}
/**
* repeated .org.openfeed.InstrumentDefinition.TimeSpan sessions = 1;
*/
@java.lang.Override
public int getSessionsCount() {
return sessions_.size();
}
/**
* repeated .org.openfeed.InstrumentDefinition.TimeSpan sessions = 1;
*/
@java.lang.Override
public org.openfeed.InstrumentDefinition.TimeSpan getSessions(int index) {
return sessions_.get(index);
}
/**
* repeated .org.openfeed.InstrumentDefinition.TimeSpan sessions = 1;
*/
@java.lang.Override
public org.openfeed.InstrumentDefinition.TimeSpanOrBuilder getSessionsOrBuilder(
int index) {
return sessions_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < sessions_.size(); i++) {
output.writeMessage(1, sessions_.get(i));
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < sessions_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, sessions_.get(i));
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.openfeed.InstrumentDefinition.Schedule)) {
return super.equals(obj);
}
org.openfeed.InstrumentDefinition.Schedule other = (org.openfeed.InstrumentDefinition.Schedule) obj;
if (!getSessionsList()
.equals(other.getSessionsList())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getSessionsCount() > 0) {
hash = (37 * hash) + SESSIONS_FIELD_NUMBER;
hash = (53 * hash) + getSessionsList().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.openfeed.InstrumentDefinition.Schedule parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.openfeed.InstrumentDefinition.Schedule parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.openfeed.InstrumentDefinition.Schedule parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.openfeed.InstrumentDefinition.Schedule parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.openfeed.InstrumentDefinition.Schedule parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.openfeed.InstrumentDefinition.Schedule parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.openfeed.InstrumentDefinition.Schedule parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static org.openfeed.InstrumentDefinition.Schedule parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.openfeed.InstrumentDefinition.Schedule parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input);
}
public static org.openfeed.InstrumentDefinition.Schedule parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.openfeed.InstrumentDefinition.Schedule parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static org.openfeed.InstrumentDefinition.Schedule parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.openfeed.InstrumentDefinition.Schedule prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* / Typical trading week schedule
*
*
* Protobuf type {@code org.openfeed.InstrumentDefinition.Schedule}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:org.openfeed.InstrumentDefinition.Schedule)
org.openfeed.InstrumentDefinition.ScheduleOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.openfeed.OpenfeedInstrument.internal_static_org_openfeed_InstrumentDefinition_Schedule_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.openfeed.OpenfeedInstrument.internal_static_org_openfeed_InstrumentDefinition_Schedule_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.openfeed.InstrumentDefinition.Schedule.class, org.openfeed.InstrumentDefinition.Schedule.Builder.class);
}
// Construct using org.openfeed.InstrumentDefinition.Schedule.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
if (sessionsBuilder_ == null) {
sessions_ = java.util.Collections.emptyList();
} else {
sessions_ = null;
sessionsBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.openfeed.OpenfeedInstrument.internal_static_org_openfeed_InstrumentDefinition_Schedule_descriptor;
}
@java.lang.Override
public org.openfeed.InstrumentDefinition.Schedule getDefaultInstanceForType() {
return org.openfeed.InstrumentDefinition.Schedule.getDefaultInstance();
}
@java.lang.Override
public org.openfeed.InstrumentDefinition.Schedule build() {
org.openfeed.InstrumentDefinition.Schedule result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.openfeed.InstrumentDefinition.Schedule buildPartial() {
org.openfeed.InstrumentDefinition.Schedule result = new org.openfeed.InstrumentDefinition.Schedule(this);
buildPartialRepeatedFields(result);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartialRepeatedFields(org.openfeed.InstrumentDefinition.Schedule result) {
if (sessionsBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)) {
sessions_ = java.util.Collections.unmodifiableList(sessions_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.sessions_ = sessions_;
} else {
result.sessions_ = sessionsBuilder_.build();
}
}
private void buildPartial0(org.openfeed.InstrumentDefinition.Schedule result) {
int from_bitField0_ = bitField0_;
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.openfeed.InstrumentDefinition.Schedule) {
return mergeFrom((org.openfeed.InstrumentDefinition.Schedule)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.openfeed.InstrumentDefinition.Schedule other) {
if (other == org.openfeed.InstrumentDefinition.Schedule.getDefaultInstance()) return this;
if (sessionsBuilder_ == null) {
if (!other.sessions_.isEmpty()) {
if (sessions_.isEmpty()) {
sessions_ = other.sessions_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureSessionsIsMutable();
sessions_.addAll(other.sessions_);
}
onChanged();
}
} else {
if (!other.sessions_.isEmpty()) {
if (sessionsBuilder_.isEmpty()) {
sessionsBuilder_.dispose();
sessionsBuilder_ = null;
sessions_ = other.sessions_;
bitField0_ = (bitField0_ & ~0x00000001);
sessionsBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getSessionsFieldBuilder() : null;
} else {
sessionsBuilder_.addAllMessages(other.sessions_);
}
}
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
org.openfeed.InstrumentDefinition.TimeSpan m =
input.readMessage(
org.openfeed.InstrumentDefinition.TimeSpan.parser(),
extensionRegistry);
if (sessionsBuilder_ == null) {
ensureSessionsIsMutable();
sessions_.add(m);
} else {
sessionsBuilder_.addMessage(m);
}
break;
} // case 10
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.util.List sessions_ =
java.util.Collections.emptyList();
private void ensureSessionsIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
sessions_ = new java.util.ArrayList(sessions_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
org.openfeed.InstrumentDefinition.TimeSpan, org.openfeed.InstrumentDefinition.TimeSpan.Builder, org.openfeed.InstrumentDefinition.TimeSpanOrBuilder> sessionsBuilder_;
/**
* repeated .org.openfeed.InstrumentDefinition.TimeSpan sessions = 1;
*/
public java.util.List getSessionsList() {
if (sessionsBuilder_ == null) {
return java.util.Collections.unmodifiableList(sessions_);
} else {
return sessionsBuilder_.getMessageList();
}
}
/**
* repeated .org.openfeed.InstrumentDefinition.TimeSpan sessions = 1;
*/
public int getSessionsCount() {
if (sessionsBuilder_ == null) {
return sessions_.size();
} else {
return sessionsBuilder_.getCount();
}
}
/**
* repeated .org.openfeed.InstrumentDefinition.TimeSpan sessions = 1;
*/
public org.openfeed.InstrumentDefinition.TimeSpan getSessions(int index) {
if (sessionsBuilder_ == null) {
return sessions_.get(index);
} else {
return sessionsBuilder_.getMessage(index);
}
}
/**
* repeated .org.openfeed.InstrumentDefinition.TimeSpan sessions = 1;
*/
public Builder setSessions(
int index, org.openfeed.InstrumentDefinition.TimeSpan value) {
if (sessionsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureSessionsIsMutable();
sessions_.set(index, value);
onChanged();
} else {
sessionsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .org.openfeed.InstrumentDefinition.TimeSpan sessions = 1;
*/
public Builder setSessions(
int index, org.openfeed.InstrumentDefinition.TimeSpan.Builder builderForValue) {
if (sessionsBuilder_ == null) {
ensureSessionsIsMutable();
sessions_.set(index, builderForValue.build());
onChanged();
} else {
sessionsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .org.openfeed.InstrumentDefinition.TimeSpan sessions = 1;
*/
public Builder addSessions(org.openfeed.InstrumentDefinition.TimeSpan value) {
if (sessionsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureSessionsIsMutable();
sessions_.add(value);
onChanged();
} else {
sessionsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .org.openfeed.InstrumentDefinition.TimeSpan sessions = 1;
*/
public Builder addSessions(
int index, org.openfeed.InstrumentDefinition.TimeSpan value) {
if (sessionsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureSessionsIsMutable();
sessions_.add(index, value);
onChanged();
} else {
sessionsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .org.openfeed.InstrumentDefinition.TimeSpan sessions = 1;
*/
public Builder addSessions(
org.openfeed.InstrumentDefinition.TimeSpan.Builder builderForValue) {
if (sessionsBuilder_ == null) {
ensureSessionsIsMutable();
sessions_.add(builderForValue.build());
onChanged();
} else {
sessionsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .org.openfeed.InstrumentDefinition.TimeSpan sessions = 1;
*/
public Builder addSessions(
int index, org.openfeed.InstrumentDefinition.TimeSpan.Builder builderForValue) {
if (sessionsBuilder_ == null) {
ensureSessionsIsMutable();
sessions_.add(index, builderForValue.build());
onChanged();
} else {
sessionsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .org.openfeed.InstrumentDefinition.TimeSpan sessions = 1;
*/
public Builder addAllSessions(
java.lang.Iterable extends org.openfeed.InstrumentDefinition.TimeSpan> values) {
if (sessionsBuilder_ == null) {
ensureSessionsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, sessions_);
onChanged();
} else {
sessionsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .org.openfeed.InstrumentDefinition.TimeSpan sessions = 1;
*/
public Builder clearSessions() {
if (sessionsBuilder_ == null) {
sessions_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
sessionsBuilder_.clear();
}
return this;
}
/**
* repeated .org.openfeed.InstrumentDefinition.TimeSpan sessions = 1;
*/
public Builder removeSessions(int index) {
if (sessionsBuilder_ == null) {
ensureSessionsIsMutable();
sessions_.remove(index);
onChanged();
} else {
sessionsBuilder_.remove(index);
}
return this;
}
/**
* repeated .org.openfeed.InstrumentDefinition.TimeSpan sessions = 1;
*/
public org.openfeed.InstrumentDefinition.TimeSpan.Builder getSessionsBuilder(
int index) {
return getSessionsFieldBuilder().getBuilder(index);
}
/**
* repeated .org.openfeed.InstrumentDefinition.TimeSpan sessions = 1;
*/
public org.openfeed.InstrumentDefinition.TimeSpanOrBuilder getSessionsOrBuilder(
int index) {
if (sessionsBuilder_ == null) {
return sessions_.get(index); } else {
return sessionsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .org.openfeed.InstrumentDefinition.TimeSpan sessions = 1;
*/
public java.util.List extends org.openfeed.InstrumentDefinition.TimeSpanOrBuilder>
getSessionsOrBuilderList() {
if (sessionsBuilder_ != null) {
return sessionsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(sessions_);
}
}
/**
* repeated .org.openfeed.InstrumentDefinition.TimeSpan sessions = 1;
*/
public org.openfeed.InstrumentDefinition.TimeSpan.Builder addSessionsBuilder() {
return getSessionsFieldBuilder().addBuilder(
org.openfeed.InstrumentDefinition.TimeSpan.getDefaultInstance());
}
/**
* repeated .org.openfeed.InstrumentDefinition.TimeSpan sessions = 1;
*/
public org.openfeed.InstrumentDefinition.TimeSpan.Builder addSessionsBuilder(
int index) {
return getSessionsFieldBuilder().addBuilder(
index, org.openfeed.InstrumentDefinition.TimeSpan.getDefaultInstance());
}
/**
* repeated .org.openfeed.InstrumentDefinition.TimeSpan sessions = 1;
*/
public java.util.List
getSessionsBuilderList() {
return getSessionsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
org.openfeed.InstrumentDefinition.TimeSpan, org.openfeed.InstrumentDefinition.TimeSpan.Builder, org.openfeed.InstrumentDefinition.TimeSpanOrBuilder>
getSessionsFieldBuilder() {
if (sessionsBuilder_ == null) {
sessionsBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
org.openfeed.InstrumentDefinition.TimeSpan, org.openfeed.InstrumentDefinition.TimeSpan.Builder, org.openfeed.InstrumentDefinition.TimeSpanOrBuilder>(
sessions_,
((bitField0_ & 0x00000001) != 0),
getParentForChildren(),
isClean());
sessions_ = null;
}
return sessionsBuilder_;
}
// @@protoc_insertion_point(builder_scope:org.openfeed.InstrumentDefinition.Schedule)
}
// @@protoc_insertion_point(class_scope:org.openfeed.InstrumentDefinition.Schedule)
private static final org.openfeed.InstrumentDefinition.Schedule DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.openfeed.InstrumentDefinition.Schedule();
}
public static org.openfeed.InstrumentDefinition.Schedule getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Schedule parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.openfeed.InstrumentDefinition.Schedule getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface TimeSpanOrBuilder extends
// @@protoc_insertion_point(interface_extends:org.openfeed.InstrumentDefinition.TimeSpan)
com.google.protobuf.MessageOrBuilder {
/**
* sint64 timeStart = 1;
* @return The timeStart.
*/
long getTimeStart();
/**
* sint64 timeFinish = 2;
* @return The timeFinish.
*/
long getTimeFinish();
}
/**
*
* / Trading session
* Times are in nanos since midnight UTC on the Sunday starting a given trading week
*
*
* Protobuf type {@code org.openfeed.InstrumentDefinition.TimeSpan}
*/
public static final class TimeSpan extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:org.openfeed.InstrumentDefinition.TimeSpan)
TimeSpanOrBuilder {
private static final long serialVersionUID = 0L;
static {
com.google.protobuf.RuntimeVersion.validateProtobufGencodeVersion(
com.google.protobuf.RuntimeVersion.RuntimeDomain.PUBLIC,
/* major= */ 4,
/* minor= */ 28,
/* patch= */ 3,
/* suffix= */ "",
TimeSpan.class.getName());
}
// Use TimeSpan.newBuilder() to construct.
private TimeSpan(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private TimeSpan() {
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.openfeed.OpenfeedInstrument.internal_static_org_openfeed_InstrumentDefinition_TimeSpan_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.openfeed.OpenfeedInstrument.internal_static_org_openfeed_InstrumentDefinition_TimeSpan_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.openfeed.InstrumentDefinition.TimeSpan.class, org.openfeed.InstrumentDefinition.TimeSpan.Builder.class);
}
public static final int TIMESTART_FIELD_NUMBER = 1;
private long timeStart_ = 0L;
/**
* sint64 timeStart = 1;
* @return The timeStart.
*/
@java.lang.Override
public long getTimeStart() {
return timeStart_;
}
public static final int TIMEFINISH_FIELD_NUMBER = 2;
private long timeFinish_ = 0L;
/**
* sint64 timeFinish = 2;
* @return The timeFinish.
*/
@java.lang.Override
public long getTimeFinish() {
return timeFinish_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (timeStart_ != 0L) {
output.writeSInt64(1, timeStart_);
}
if (timeFinish_ != 0L) {
output.writeSInt64(2, timeFinish_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (timeStart_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeSInt64Size(1, timeStart_);
}
if (timeFinish_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeSInt64Size(2, timeFinish_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.openfeed.InstrumentDefinition.TimeSpan)) {
return super.equals(obj);
}
org.openfeed.InstrumentDefinition.TimeSpan other = (org.openfeed.InstrumentDefinition.TimeSpan) obj;
if (getTimeStart()
!= other.getTimeStart()) return false;
if (getTimeFinish()
!= other.getTimeFinish()) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + TIMESTART_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getTimeStart());
hash = (37 * hash) + TIMEFINISH_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getTimeFinish());
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.openfeed.InstrumentDefinition.TimeSpan parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.openfeed.InstrumentDefinition.TimeSpan parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.openfeed.InstrumentDefinition.TimeSpan parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.openfeed.InstrumentDefinition.TimeSpan parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.openfeed.InstrumentDefinition.TimeSpan parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.openfeed.InstrumentDefinition.TimeSpan parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.openfeed.InstrumentDefinition.TimeSpan parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static org.openfeed.InstrumentDefinition.TimeSpan parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.openfeed.InstrumentDefinition.TimeSpan parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input);
}
public static org.openfeed.InstrumentDefinition.TimeSpan parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.openfeed.InstrumentDefinition.TimeSpan parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static org.openfeed.InstrumentDefinition.TimeSpan parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.openfeed.InstrumentDefinition.TimeSpan prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* / Trading session
* Times are in nanos since midnight UTC on the Sunday starting a given trading week
*
*
* Protobuf type {@code org.openfeed.InstrumentDefinition.TimeSpan}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:org.openfeed.InstrumentDefinition.TimeSpan)
org.openfeed.InstrumentDefinition.TimeSpanOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.openfeed.OpenfeedInstrument.internal_static_org_openfeed_InstrumentDefinition_TimeSpan_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.openfeed.OpenfeedInstrument.internal_static_org_openfeed_InstrumentDefinition_TimeSpan_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.openfeed.InstrumentDefinition.TimeSpan.class, org.openfeed.InstrumentDefinition.TimeSpan.Builder.class);
}
// Construct using org.openfeed.InstrumentDefinition.TimeSpan.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
timeStart_ = 0L;
timeFinish_ = 0L;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.openfeed.OpenfeedInstrument.internal_static_org_openfeed_InstrumentDefinition_TimeSpan_descriptor;
}
@java.lang.Override
public org.openfeed.InstrumentDefinition.TimeSpan getDefaultInstanceForType() {
return org.openfeed.InstrumentDefinition.TimeSpan.getDefaultInstance();
}
@java.lang.Override
public org.openfeed.InstrumentDefinition.TimeSpan build() {
org.openfeed.InstrumentDefinition.TimeSpan result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.openfeed.InstrumentDefinition.TimeSpan buildPartial() {
org.openfeed.InstrumentDefinition.TimeSpan result = new org.openfeed.InstrumentDefinition.TimeSpan(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(org.openfeed.InstrumentDefinition.TimeSpan result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.timeStart_ = timeStart_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.timeFinish_ = timeFinish_;
}
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.openfeed.InstrumentDefinition.TimeSpan) {
return mergeFrom((org.openfeed.InstrumentDefinition.TimeSpan)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.openfeed.InstrumentDefinition.TimeSpan other) {
if (other == org.openfeed.InstrumentDefinition.TimeSpan.getDefaultInstance()) return this;
if (other.getTimeStart() != 0L) {
setTimeStart(other.getTimeStart());
}
if (other.getTimeFinish() != 0L) {
setTimeFinish(other.getTimeFinish());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
timeStart_ = input.readSInt64();
bitField0_ |= 0x00000001;
break;
} // case 8
case 16: {
timeFinish_ = input.readSInt64();
bitField0_ |= 0x00000002;
break;
} // case 16
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private long timeStart_ ;
/**
* sint64 timeStart = 1;
* @return The timeStart.
*/
@java.lang.Override
public long getTimeStart() {
return timeStart_;
}
/**
* sint64 timeStart = 1;
* @param value The timeStart to set.
* @return This builder for chaining.
*/
public Builder setTimeStart(long value) {
timeStart_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* sint64 timeStart = 1;
* @return This builder for chaining.
*/
public Builder clearTimeStart() {
bitField0_ = (bitField0_ & ~0x00000001);
timeStart_ = 0L;
onChanged();
return this;
}
private long timeFinish_ ;
/**
* sint64 timeFinish = 2;
* @return The timeFinish.
*/
@java.lang.Override
public long getTimeFinish() {
return timeFinish_;
}
/**
* sint64 timeFinish = 2;
* @param value The timeFinish to set.
* @return This builder for chaining.
*/
public Builder setTimeFinish(long value) {
timeFinish_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* sint64 timeFinish = 2;
* @return This builder for chaining.
*/
public Builder clearTimeFinish() {
bitField0_ = (bitField0_ & ~0x00000002);
timeFinish_ = 0L;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:org.openfeed.InstrumentDefinition.TimeSpan)
}
// @@protoc_insertion_point(class_scope:org.openfeed.InstrumentDefinition.TimeSpan)
private static final org.openfeed.InstrumentDefinition.TimeSpan DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.openfeed.InstrumentDefinition.TimeSpan();
}
public static org.openfeed.InstrumentDefinition.TimeSpan getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public TimeSpan parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.openfeed.InstrumentDefinition.TimeSpan getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface CalendarOrBuilder extends
// @@protoc_insertion_point(interface_extends:org.openfeed.InstrumentDefinition.Calendar)
com.google.protobuf.MessageOrBuilder {
/**
* repeated .org.openfeed.InstrumentDefinition.Event events = 1;
*/
java.util.List
getEventsList();
/**
* repeated .org.openfeed.InstrumentDefinition.Event events = 1;
*/
org.openfeed.InstrumentDefinition.Event getEvents(int index);
/**
* repeated .org.openfeed.InstrumentDefinition.Event events = 1;
*/
int getEventsCount();
/**
* repeated .org.openfeed.InstrumentDefinition.Event events = 1;
*/
java.util.List extends org.openfeed.InstrumentDefinition.EventOrBuilder>
getEventsOrBuilderList();
/**
* repeated .org.openfeed.InstrumentDefinition.Event events = 1;
*/
org.openfeed.InstrumentDefinition.EventOrBuilder getEventsOrBuilder(
int index);
}
/**
* Protobuf type {@code org.openfeed.InstrumentDefinition.Calendar}
*/
public static final class Calendar extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:org.openfeed.InstrumentDefinition.Calendar)
CalendarOrBuilder {
private static final long serialVersionUID = 0L;
static {
com.google.protobuf.RuntimeVersion.validateProtobufGencodeVersion(
com.google.protobuf.RuntimeVersion.RuntimeDomain.PUBLIC,
/* major= */ 4,
/* minor= */ 28,
/* patch= */ 3,
/* suffix= */ "",
Calendar.class.getName());
}
// Use Calendar.newBuilder() to construct.
private Calendar(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private Calendar() {
events_ = java.util.Collections.emptyList();
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.openfeed.OpenfeedInstrument.internal_static_org_openfeed_InstrumentDefinition_Calendar_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.openfeed.OpenfeedInstrument.internal_static_org_openfeed_InstrumentDefinition_Calendar_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.openfeed.InstrumentDefinition.Calendar.class, org.openfeed.InstrumentDefinition.Calendar.Builder.class);
}
public static final int EVENTS_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private java.util.List events_;
/**
* repeated .org.openfeed.InstrumentDefinition.Event events = 1;
*/
@java.lang.Override
public java.util.List getEventsList() {
return events_;
}
/**
* repeated .org.openfeed.InstrumentDefinition.Event events = 1;
*/
@java.lang.Override
public java.util.List extends org.openfeed.InstrumentDefinition.EventOrBuilder>
getEventsOrBuilderList() {
return events_;
}
/**
* repeated .org.openfeed.InstrumentDefinition.Event events = 1;
*/
@java.lang.Override
public int getEventsCount() {
return events_.size();
}
/**
* repeated .org.openfeed.InstrumentDefinition.Event events = 1;
*/
@java.lang.Override
public org.openfeed.InstrumentDefinition.Event getEvents(int index) {
return events_.get(index);
}
/**
* repeated .org.openfeed.InstrumentDefinition.Event events = 1;
*/
@java.lang.Override
public org.openfeed.InstrumentDefinition.EventOrBuilder getEventsOrBuilder(
int index) {
return events_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
for (int i = 0; i < events_.size(); i++) {
output.writeMessage(1, events_.get(i));
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
for (int i = 0; i < events_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(1, events_.get(i));
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.openfeed.InstrumentDefinition.Calendar)) {
return super.equals(obj);
}
org.openfeed.InstrumentDefinition.Calendar other = (org.openfeed.InstrumentDefinition.Calendar) obj;
if (!getEventsList()
.equals(other.getEventsList())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getEventsCount() > 0) {
hash = (37 * hash) + EVENTS_FIELD_NUMBER;
hash = (53 * hash) + getEventsList().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.openfeed.InstrumentDefinition.Calendar parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.openfeed.InstrumentDefinition.Calendar parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.openfeed.InstrumentDefinition.Calendar parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.openfeed.InstrumentDefinition.Calendar parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.openfeed.InstrumentDefinition.Calendar parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.openfeed.InstrumentDefinition.Calendar parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.openfeed.InstrumentDefinition.Calendar parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static org.openfeed.InstrumentDefinition.Calendar parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.openfeed.InstrumentDefinition.Calendar parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input);
}
public static org.openfeed.InstrumentDefinition.Calendar parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.openfeed.InstrumentDefinition.Calendar parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static org.openfeed.InstrumentDefinition.Calendar parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.openfeed.InstrumentDefinition.Calendar prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code org.openfeed.InstrumentDefinition.Calendar}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:org.openfeed.InstrumentDefinition.Calendar)
org.openfeed.InstrumentDefinition.CalendarOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.openfeed.OpenfeedInstrument.internal_static_org_openfeed_InstrumentDefinition_Calendar_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.openfeed.OpenfeedInstrument.internal_static_org_openfeed_InstrumentDefinition_Calendar_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.openfeed.InstrumentDefinition.Calendar.class, org.openfeed.InstrumentDefinition.Calendar.Builder.class);
}
// Construct using org.openfeed.InstrumentDefinition.Calendar.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
if (eventsBuilder_ == null) {
events_ = java.util.Collections.emptyList();
} else {
events_ = null;
eventsBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000001);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.openfeed.OpenfeedInstrument.internal_static_org_openfeed_InstrumentDefinition_Calendar_descriptor;
}
@java.lang.Override
public org.openfeed.InstrumentDefinition.Calendar getDefaultInstanceForType() {
return org.openfeed.InstrumentDefinition.Calendar.getDefaultInstance();
}
@java.lang.Override
public org.openfeed.InstrumentDefinition.Calendar build() {
org.openfeed.InstrumentDefinition.Calendar result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.openfeed.InstrumentDefinition.Calendar buildPartial() {
org.openfeed.InstrumentDefinition.Calendar result = new org.openfeed.InstrumentDefinition.Calendar(this);
buildPartialRepeatedFields(result);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartialRepeatedFields(org.openfeed.InstrumentDefinition.Calendar result) {
if (eventsBuilder_ == null) {
if (((bitField0_ & 0x00000001) != 0)) {
events_ = java.util.Collections.unmodifiableList(events_);
bitField0_ = (bitField0_ & ~0x00000001);
}
result.events_ = events_;
} else {
result.events_ = eventsBuilder_.build();
}
}
private void buildPartial0(org.openfeed.InstrumentDefinition.Calendar result) {
int from_bitField0_ = bitField0_;
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.openfeed.InstrumentDefinition.Calendar) {
return mergeFrom((org.openfeed.InstrumentDefinition.Calendar)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.openfeed.InstrumentDefinition.Calendar other) {
if (other == org.openfeed.InstrumentDefinition.Calendar.getDefaultInstance()) return this;
if (eventsBuilder_ == null) {
if (!other.events_.isEmpty()) {
if (events_.isEmpty()) {
events_ = other.events_;
bitField0_ = (bitField0_ & ~0x00000001);
} else {
ensureEventsIsMutable();
events_.addAll(other.events_);
}
onChanged();
}
} else {
if (!other.events_.isEmpty()) {
if (eventsBuilder_.isEmpty()) {
eventsBuilder_.dispose();
eventsBuilder_ = null;
events_ = other.events_;
bitField0_ = (bitField0_ & ~0x00000001);
eventsBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getEventsFieldBuilder() : null;
} else {
eventsBuilder_.addAllMessages(other.events_);
}
}
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
org.openfeed.InstrumentDefinition.Event m =
input.readMessage(
org.openfeed.InstrumentDefinition.Event.parser(),
extensionRegistry);
if (eventsBuilder_ == null) {
ensureEventsIsMutable();
events_.add(m);
} else {
eventsBuilder_.addMessage(m);
}
break;
} // case 10
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.util.List events_ =
java.util.Collections.emptyList();
private void ensureEventsIsMutable() {
if (!((bitField0_ & 0x00000001) != 0)) {
events_ = new java.util.ArrayList(events_);
bitField0_ |= 0x00000001;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
org.openfeed.InstrumentDefinition.Event, org.openfeed.InstrumentDefinition.Event.Builder, org.openfeed.InstrumentDefinition.EventOrBuilder> eventsBuilder_;
/**
* repeated .org.openfeed.InstrumentDefinition.Event events = 1;
*/
public java.util.List getEventsList() {
if (eventsBuilder_ == null) {
return java.util.Collections.unmodifiableList(events_);
} else {
return eventsBuilder_.getMessageList();
}
}
/**
* repeated .org.openfeed.InstrumentDefinition.Event events = 1;
*/
public int getEventsCount() {
if (eventsBuilder_ == null) {
return events_.size();
} else {
return eventsBuilder_.getCount();
}
}
/**
* repeated .org.openfeed.InstrumentDefinition.Event events = 1;
*/
public org.openfeed.InstrumentDefinition.Event getEvents(int index) {
if (eventsBuilder_ == null) {
return events_.get(index);
} else {
return eventsBuilder_.getMessage(index);
}
}
/**
* repeated .org.openfeed.InstrumentDefinition.Event events = 1;
*/
public Builder setEvents(
int index, org.openfeed.InstrumentDefinition.Event value) {
if (eventsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureEventsIsMutable();
events_.set(index, value);
onChanged();
} else {
eventsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .org.openfeed.InstrumentDefinition.Event events = 1;
*/
public Builder setEvents(
int index, org.openfeed.InstrumentDefinition.Event.Builder builderForValue) {
if (eventsBuilder_ == null) {
ensureEventsIsMutable();
events_.set(index, builderForValue.build());
onChanged();
} else {
eventsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .org.openfeed.InstrumentDefinition.Event events = 1;
*/
public Builder addEvents(org.openfeed.InstrumentDefinition.Event value) {
if (eventsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureEventsIsMutable();
events_.add(value);
onChanged();
} else {
eventsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .org.openfeed.InstrumentDefinition.Event events = 1;
*/
public Builder addEvents(
int index, org.openfeed.InstrumentDefinition.Event value) {
if (eventsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureEventsIsMutable();
events_.add(index, value);
onChanged();
} else {
eventsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .org.openfeed.InstrumentDefinition.Event events = 1;
*/
public Builder addEvents(
org.openfeed.InstrumentDefinition.Event.Builder builderForValue) {
if (eventsBuilder_ == null) {
ensureEventsIsMutable();
events_.add(builderForValue.build());
onChanged();
} else {
eventsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .org.openfeed.InstrumentDefinition.Event events = 1;
*/
public Builder addEvents(
int index, org.openfeed.InstrumentDefinition.Event.Builder builderForValue) {
if (eventsBuilder_ == null) {
ensureEventsIsMutable();
events_.add(index, builderForValue.build());
onChanged();
} else {
eventsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .org.openfeed.InstrumentDefinition.Event events = 1;
*/
public Builder addAllEvents(
java.lang.Iterable extends org.openfeed.InstrumentDefinition.Event> values) {
if (eventsBuilder_ == null) {
ensureEventsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, events_);
onChanged();
} else {
eventsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .org.openfeed.InstrumentDefinition.Event events = 1;
*/
public Builder clearEvents() {
if (eventsBuilder_ == null) {
events_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
} else {
eventsBuilder_.clear();
}
return this;
}
/**
* repeated .org.openfeed.InstrumentDefinition.Event events = 1;
*/
public Builder removeEvents(int index) {
if (eventsBuilder_ == null) {
ensureEventsIsMutable();
events_.remove(index);
onChanged();
} else {
eventsBuilder_.remove(index);
}
return this;
}
/**
* repeated .org.openfeed.InstrumentDefinition.Event events = 1;
*/
public org.openfeed.InstrumentDefinition.Event.Builder getEventsBuilder(
int index) {
return getEventsFieldBuilder().getBuilder(index);
}
/**
* repeated .org.openfeed.InstrumentDefinition.Event events = 1;
*/
public org.openfeed.InstrumentDefinition.EventOrBuilder getEventsOrBuilder(
int index) {
if (eventsBuilder_ == null) {
return events_.get(index); } else {
return eventsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .org.openfeed.InstrumentDefinition.Event events = 1;
*/
public java.util.List extends org.openfeed.InstrumentDefinition.EventOrBuilder>
getEventsOrBuilderList() {
if (eventsBuilder_ != null) {
return eventsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(events_);
}
}
/**
* repeated .org.openfeed.InstrumentDefinition.Event events = 1;
*/
public org.openfeed.InstrumentDefinition.Event.Builder addEventsBuilder() {
return getEventsFieldBuilder().addBuilder(
org.openfeed.InstrumentDefinition.Event.getDefaultInstance());
}
/**
* repeated .org.openfeed.InstrumentDefinition.Event events = 1;
*/
public org.openfeed.InstrumentDefinition.Event.Builder addEventsBuilder(
int index) {
return getEventsFieldBuilder().addBuilder(
index, org.openfeed.InstrumentDefinition.Event.getDefaultInstance());
}
/**
* repeated .org.openfeed.InstrumentDefinition.Event events = 1;
*/
public java.util.List
getEventsBuilderList() {
return getEventsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
org.openfeed.InstrumentDefinition.Event, org.openfeed.InstrumentDefinition.Event.Builder, org.openfeed.InstrumentDefinition.EventOrBuilder>
getEventsFieldBuilder() {
if (eventsBuilder_ == null) {
eventsBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
org.openfeed.InstrumentDefinition.Event, org.openfeed.InstrumentDefinition.Event.Builder, org.openfeed.InstrumentDefinition.EventOrBuilder>(
events_,
((bitField0_ & 0x00000001) != 0),
getParentForChildren(),
isClean());
events_ = null;
}
return eventsBuilder_;
}
// @@protoc_insertion_point(builder_scope:org.openfeed.InstrumentDefinition.Calendar)
}
// @@protoc_insertion_point(class_scope:org.openfeed.InstrumentDefinition.Calendar)
private static final org.openfeed.InstrumentDefinition.Calendar DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.openfeed.InstrumentDefinition.Calendar();
}
public static org.openfeed.InstrumentDefinition.Calendar getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Calendar parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.openfeed.InstrumentDefinition.Calendar getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface EventOrBuilder extends
// @@protoc_insertion_point(interface_extends:org.openfeed.InstrumentDefinition.Event)
com.google.protobuf.MessageOrBuilder {
/**
* .org.openfeed.InstrumentDefinition.EventType type = 1;
* @return The enum numeric value on the wire for type.
*/
int getTypeValue();
/**
* .org.openfeed.InstrumentDefinition.EventType type = 1;
* @return The type.
*/
org.openfeed.InstrumentDefinition.EventType getType();
/**
*
* / Epoch time in ms
*
*
* sint64 date = 2;
* @return The date.
*/
long getDate();
}
/**
* Protobuf type {@code org.openfeed.InstrumentDefinition.Event}
*/
public static final class Event extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:org.openfeed.InstrumentDefinition.Event)
EventOrBuilder {
private static final long serialVersionUID = 0L;
static {
com.google.protobuf.RuntimeVersion.validateProtobufGencodeVersion(
com.google.protobuf.RuntimeVersion.RuntimeDomain.PUBLIC,
/* major= */ 4,
/* minor= */ 28,
/* patch= */ 3,
/* suffix= */ "",
Event.class.getName());
}
// Use Event.newBuilder() to construct.
private Event(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private Event() {
type_ = 0;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.openfeed.OpenfeedInstrument.internal_static_org_openfeed_InstrumentDefinition_Event_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.openfeed.OpenfeedInstrument.internal_static_org_openfeed_InstrumentDefinition_Event_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.openfeed.InstrumentDefinition.Event.class, org.openfeed.InstrumentDefinition.Event.Builder.class);
}
public static final int TYPE_FIELD_NUMBER = 1;
private int type_ = 0;
/**
* .org.openfeed.InstrumentDefinition.EventType type = 1;
* @return The enum numeric value on the wire for type.
*/
@java.lang.Override public int getTypeValue() {
return type_;
}
/**
* .org.openfeed.InstrumentDefinition.EventType type = 1;
* @return The type.
*/
@java.lang.Override public org.openfeed.InstrumentDefinition.EventType getType() {
org.openfeed.InstrumentDefinition.EventType result = org.openfeed.InstrumentDefinition.EventType.forNumber(type_);
return result == null ? org.openfeed.InstrumentDefinition.EventType.UNRECOGNIZED : result;
}
public static final int DATE_FIELD_NUMBER = 2;
private long date_ = 0L;
/**
*
* / Epoch time in ms
*
*
* sint64 date = 2;
* @return The date.
*/
@java.lang.Override
public long getDate() {
return date_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (type_ != org.openfeed.InstrumentDefinition.EventType.UNKNOWN_EVENT_TYPE.getNumber()) {
output.writeEnum(1, type_);
}
if (date_ != 0L) {
output.writeSInt64(2, date_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (type_ != org.openfeed.InstrumentDefinition.EventType.UNKNOWN_EVENT_TYPE.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(1, type_);
}
if (date_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeSInt64Size(2, date_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.openfeed.InstrumentDefinition.Event)) {
return super.equals(obj);
}
org.openfeed.InstrumentDefinition.Event other = (org.openfeed.InstrumentDefinition.Event) obj;
if (type_ != other.type_) return false;
if (getDate()
!= other.getDate()) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + TYPE_FIELD_NUMBER;
hash = (53 * hash) + type_;
hash = (37 * hash) + DATE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getDate());
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.openfeed.InstrumentDefinition.Event parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.openfeed.InstrumentDefinition.Event parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.openfeed.InstrumentDefinition.Event parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.openfeed.InstrumentDefinition.Event parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.openfeed.InstrumentDefinition.Event parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.openfeed.InstrumentDefinition.Event parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.openfeed.InstrumentDefinition.Event parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static org.openfeed.InstrumentDefinition.Event parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.openfeed.InstrumentDefinition.Event parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input);
}
public static org.openfeed.InstrumentDefinition.Event parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.openfeed.InstrumentDefinition.Event parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static org.openfeed.InstrumentDefinition.Event parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.openfeed.InstrumentDefinition.Event prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code org.openfeed.InstrumentDefinition.Event}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:org.openfeed.InstrumentDefinition.Event)
org.openfeed.InstrumentDefinition.EventOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.openfeed.OpenfeedInstrument.internal_static_org_openfeed_InstrumentDefinition_Event_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.openfeed.OpenfeedInstrument.internal_static_org_openfeed_InstrumentDefinition_Event_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.openfeed.InstrumentDefinition.Event.class, org.openfeed.InstrumentDefinition.Event.Builder.class);
}
// Construct using org.openfeed.InstrumentDefinition.Event.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
type_ = 0;
date_ = 0L;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.openfeed.OpenfeedInstrument.internal_static_org_openfeed_InstrumentDefinition_Event_descriptor;
}
@java.lang.Override
public org.openfeed.InstrumentDefinition.Event getDefaultInstanceForType() {
return org.openfeed.InstrumentDefinition.Event.getDefaultInstance();
}
@java.lang.Override
public org.openfeed.InstrumentDefinition.Event build() {
org.openfeed.InstrumentDefinition.Event result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.openfeed.InstrumentDefinition.Event buildPartial() {
org.openfeed.InstrumentDefinition.Event result = new org.openfeed.InstrumentDefinition.Event(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(org.openfeed.InstrumentDefinition.Event result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.type_ = type_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.date_ = date_;
}
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.openfeed.InstrumentDefinition.Event) {
return mergeFrom((org.openfeed.InstrumentDefinition.Event)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.openfeed.InstrumentDefinition.Event other) {
if (other == org.openfeed.InstrumentDefinition.Event.getDefaultInstance()) return this;
if (other.type_ != 0) {
setTypeValue(other.getTypeValue());
}
if (other.getDate() != 0L) {
setDate(other.getDate());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
type_ = input.readEnum();
bitField0_ |= 0x00000001;
break;
} // case 8
case 16: {
date_ = input.readSInt64();
bitField0_ |= 0x00000002;
break;
} // case 16
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private int type_ = 0;
/**
* .org.openfeed.InstrumentDefinition.EventType type = 1;
* @return The enum numeric value on the wire for type.
*/
@java.lang.Override public int getTypeValue() {
return type_;
}
/**
* .org.openfeed.InstrumentDefinition.EventType type = 1;
* @param value The enum numeric value on the wire for type to set.
* @return This builder for chaining.
*/
public Builder setTypeValue(int value) {
type_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* .org.openfeed.InstrumentDefinition.EventType type = 1;
* @return The type.
*/
@java.lang.Override
public org.openfeed.InstrumentDefinition.EventType getType() {
org.openfeed.InstrumentDefinition.EventType result = org.openfeed.InstrumentDefinition.EventType.forNumber(type_);
return result == null ? org.openfeed.InstrumentDefinition.EventType.UNRECOGNIZED : result;
}
/**
* .org.openfeed.InstrumentDefinition.EventType type = 1;
* @param value The type to set.
* @return This builder for chaining.
*/
public Builder setType(org.openfeed.InstrumentDefinition.EventType value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000001;
type_ = value.getNumber();
onChanged();
return this;
}
/**
* .org.openfeed.InstrumentDefinition.EventType type = 1;
* @return This builder for chaining.
*/
public Builder clearType() {
bitField0_ = (bitField0_ & ~0x00000001);
type_ = 0;
onChanged();
return this;
}
private long date_ ;
/**
*
* / Epoch time in ms
*
*
* sint64 date = 2;
* @return The date.
*/
@java.lang.Override
public long getDate() {
return date_;
}
/**
*
* / Epoch time in ms
*
*
* sint64 date = 2;
* @param value The date to set.
* @return This builder for chaining.
*/
public Builder setDate(long value) {
date_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
* / Epoch time in ms
*
*
* sint64 date = 2;
* @return This builder for chaining.
*/
public Builder clearDate() {
bitField0_ = (bitField0_ & ~0x00000002);
date_ = 0L;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:org.openfeed.InstrumentDefinition.Event)
}
// @@protoc_insertion_point(class_scope:org.openfeed.InstrumentDefinition.Event)
private static final org.openfeed.InstrumentDefinition.Event DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.openfeed.InstrumentDefinition.Event();
}
public static org.openfeed.InstrumentDefinition.Event getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Event parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.openfeed.InstrumentDefinition.Event getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface SpreadLegOrBuilder extends
// @@protoc_insertion_point(interface_extends:org.openfeed.InstrumentDefinition.SpreadLeg)
com.google.protobuf.MessageOrBuilder {
/**
*
* The marketId of the leg
*
*
* sint64 marketId = 1;
* @return The marketId.
*/
long getMarketId();
/**
*
* The ratio of the this leg with respect to the spread.
* Negative means short the absolute value, positive means long the absolute value.
* FIXME? Alternatively, we could have separate LONG/SHORT indicator in the message
* and use this field as only the absolute value
*
*
* sint32 ratio = 2;
* @return The ratio.
*/
int getRatio();
/**
* string symbol = 3;
* @return The symbol.
*/
java.lang.String getSymbol();
/**
* string symbol = 3;
* @return The bytes for symbol.
*/
com.google.protobuf.ByteString
getSymbolBytes();
/**
* string longSymbol = 4;
* @return The longSymbol.
*/
java.lang.String getLongSymbol();
/**
* string longSymbol = 4;
* @return The bytes for longSymbol.
*/
com.google.protobuf.ByteString
getLongSymbolBytes();
/**
* float legOptionDelta = 5;
* @return The legOptionDelta.
*/
float getLegOptionDelta();
/**
*
* Additional information about the leg will be found in the instrument definition
* for the leg. It is not included here to reduce duplication.
*
*
* float legPrice = 6;
* @return The legPrice.
*/
float getLegPrice();
}
/**
* Protobuf type {@code org.openfeed.InstrumentDefinition.SpreadLeg}
*/
public static final class SpreadLeg extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:org.openfeed.InstrumentDefinition.SpreadLeg)
SpreadLegOrBuilder {
private static final long serialVersionUID = 0L;
static {
com.google.protobuf.RuntimeVersion.validateProtobufGencodeVersion(
com.google.protobuf.RuntimeVersion.RuntimeDomain.PUBLIC,
/* major= */ 4,
/* minor= */ 28,
/* patch= */ 3,
/* suffix= */ "",
SpreadLeg.class.getName());
}
// Use SpreadLeg.newBuilder() to construct.
private SpreadLeg(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private SpreadLeg() {
symbol_ = "";
longSymbol_ = "";
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.openfeed.OpenfeedInstrument.internal_static_org_openfeed_InstrumentDefinition_SpreadLeg_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.openfeed.OpenfeedInstrument.internal_static_org_openfeed_InstrumentDefinition_SpreadLeg_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.openfeed.InstrumentDefinition.SpreadLeg.class, org.openfeed.InstrumentDefinition.SpreadLeg.Builder.class);
}
public static final int MARKETID_FIELD_NUMBER = 1;
private long marketId_ = 0L;
/**
*
* The marketId of the leg
*
*
* sint64 marketId = 1;
* @return The marketId.
*/
@java.lang.Override
public long getMarketId() {
return marketId_;
}
public static final int RATIO_FIELD_NUMBER = 2;
private int ratio_ = 0;
/**
*
* The ratio of the this leg with respect to the spread.
* Negative means short the absolute value, positive means long the absolute value.
* FIXME? Alternatively, we could have separate LONG/SHORT indicator in the message
* and use this field as only the absolute value
*
*
* sint32 ratio = 2;
* @return The ratio.
*/
@java.lang.Override
public int getRatio() {
return ratio_;
}
public static final int SYMBOL_FIELD_NUMBER = 3;
@SuppressWarnings("serial")
private volatile java.lang.Object symbol_ = "";
/**
* string symbol = 3;
* @return The symbol.
*/
@java.lang.Override
public java.lang.String getSymbol() {
java.lang.Object ref = symbol_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
symbol_ = s;
return s;
}
}
/**
* string symbol = 3;
* @return The bytes for symbol.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getSymbolBytes() {
java.lang.Object ref = symbol_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
symbol_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int LONGSYMBOL_FIELD_NUMBER = 4;
@SuppressWarnings("serial")
private volatile java.lang.Object longSymbol_ = "";
/**
* string longSymbol = 4;
* @return The longSymbol.
*/
@java.lang.Override
public java.lang.String getLongSymbol() {
java.lang.Object ref = longSymbol_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
longSymbol_ = s;
return s;
}
}
/**
* string longSymbol = 4;
* @return The bytes for longSymbol.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getLongSymbolBytes() {
java.lang.Object ref = longSymbol_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
longSymbol_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int LEGOPTIONDELTA_FIELD_NUMBER = 5;
private float legOptionDelta_ = 0F;
/**
* float legOptionDelta = 5;
* @return The legOptionDelta.
*/
@java.lang.Override
public float getLegOptionDelta() {
return legOptionDelta_;
}
public static final int LEGPRICE_FIELD_NUMBER = 6;
private float legPrice_ = 0F;
/**
*
* Additional information about the leg will be found in the instrument definition
* for the leg. It is not included here to reduce duplication.
*
*
* float legPrice = 6;
* @return The legPrice.
*/
@java.lang.Override
public float getLegPrice() {
return legPrice_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (marketId_ != 0L) {
output.writeSInt64(1, marketId_);
}
if (ratio_ != 0) {
output.writeSInt32(2, ratio_);
}
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(symbol_)) {
com.google.protobuf.GeneratedMessage.writeString(output, 3, symbol_);
}
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(longSymbol_)) {
com.google.protobuf.GeneratedMessage.writeString(output, 4, longSymbol_);
}
if (java.lang.Float.floatToRawIntBits(legOptionDelta_) != 0) {
output.writeFloat(5, legOptionDelta_);
}
if (java.lang.Float.floatToRawIntBits(legPrice_) != 0) {
output.writeFloat(6, legPrice_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (marketId_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeSInt64Size(1, marketId_);
}
if (ratio_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeSInt32Size(2, ratio_);
}
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(symbol_)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(3, symbol_);
}
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(longSymbol_)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(4, longSymbol_);
}
if (java.lang.Float.floatToRawIntBits(legOptionDelta_) != 0) {
size += com.google.protobuf.CodedOutputStream
.computeFloatSize(5, legOptionDelta_);
}
if (java.lang.Float.floatToRawIntBits(legPrice_) != 0) {
size += com.google.protobuf.CodedOutputStream
.computeFloatSize(6, legPrice_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.openfeed.InstrumentDefinition.SpreadLeg)) {
return super.equals(obj);
}
org.openfeed.InstrumentDefinition.SpreadLeg other = (org.openfeed.InstrumentDefinition.SpreadLeg) obj;
if (getMarketId()
!= other.getMarketId()) return false;
if (getRatio()
!= other.getRatio()) return false;
if (!getSymbol()
.equals(other.getSymbol())) return false;
if (!getLongSymbol()
.equals(other.getLongSymbol())) return false;
if (java.lang.Float.floatToIntBits(getLegOptionDelta())
!= java.lang.Float.floatToIntBits(
other.getLegOptionDelta())) return false;
if (java.lang.Float.floatToIntBits(getLegPrice())
!= java.lang.Float.floatToIntBits(
other.getLegPrice())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + MARKETID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getMarketId());
hash = (37 * hash) + RATIO_FIELD_NUMBER;
hash = (53 * hash) + getRatio();
hash = (37 * hash) + SYMBOL_FIELD_NUMBER;
hash = (53 * hash) + getSymbol().hashCode();
hash = (37 * hash) + LONGSYMBOL_FIELD_NUMBER;
hash = (53 * hash) + getLongSymbol().hashCode();
hash = (37 * hash) + LEGOPTIONDELTA_FIELD_NUMBER;
hash = (53 * hash) + java.lang.Float.floatToIntBits(
getLegOptionDelta());
hash = (37 * hash) + LEGPRICE_FIELD_NUMBER;
hash = (53 * hash) + java.lang.Float.floatToIntBits(
getLegPrice());
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.openfeed.InstrumentDefinition.SpreadLeg parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.openfeed.InstrumentDefinition.SpreadLeg parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.openfeed.InstrumentDefinition.SpreadLeg parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.openfeed.InstrumentDefinition.SpreadLeg parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.openfeed.InstrumentDefinition.SpreadLeg parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.openfeed.InstrumentDefinition.SpreadLeg parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.openfeed.InstrumentDefinition.SpreadLeg parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static org.openfeed.InstrumentDefinition.SpreadLeg parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.openfeed.InstrumentDefinition.SpreadLeg parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input);
}
public static org.openfeed.InstrumentDefinition.SpreadLeg parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.openfeed.InstrumentDefinition.SpreadLeg parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static org.openfeed.InstrumentDefinition.SpreadLeg parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.openfeed.InstrumentDefinition.SpreadLeg prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code org.openfeed.InstrumentDefinition.SpreadLeg}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:org.openfeed.InstrumentDefinition.SpreadLeg)
org.openfeed.InstrumentDefinition.SpreadLegOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.openfeed.OpenfeedInstrument.internal_static_org_openfeed_InstrumentDefinition_SpreadLeg_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.openfeed.OpenfeedInstrument.internal_static_org_openfeed_InstrumentDefinition_SpreadLeg_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.openfeed.InstrumentDefinition.SpreadLeg.class, org.openfeed.InstrumentDefinition.SpreadLeg.Builder.class);
}
// Construct using org.openfeed.InstrumentDefinition.SpreadLeg.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
marketId_ = 0L;
ratio_ = 0;
symbol_ = "";
longSymbol_ = "";
legOptionDelta_ = 0F;
legPrice_ = 0F;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.openfeed.OpenfeedInstrument.internal_static_org_openfeed_InstrumentDefinition_SpreadLeg_descriptor;
}
@java.lang.Override
public org.openfeed.InstrumentDefinition.SpreadLeg getDefaultInstanceForType() {
return org.openfeed.InstrumentDefinition.SpreadLeg.getDefaultInstance();
}
@java.lang.Override
public org.openfeed.InstrumentDefinition.SpreadLeg build() {
org.openfeed.InstrumentDefinition.SpreadLeg result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.openfeed.InstrumentDefinition.SpreadLeg buildPartial() {
org.openfeed.InstrumentDefinition.SpreadLeg result = new org.openfeed.InstrumentDefinition.SpreadLeg(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(org.openfeed.InstrumentDefinition.SpreadLeg result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.marketId_ = marketId_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.ratio_ = ratio_;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.symbol_ = symbol_;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.longSymbol_ = longSymbol_;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.legOptionDelta_ = legOptionDelta_;
}
if (((from_bitField0_ & 0x00000020) != 0)) {
result.legPrice_ = legPrice_;
}
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.openfeed.InstrumentDefinition.SpreadLeg) {
return mergeFrom((org.openfeed.InstrumentDefinition.SpreadLeg)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.openfeed.InstrumentDefinition.SpreadLeg other) {
if (other == org.openfeed.InstrumentDefinition.SpreadLeg.getDefaultInstance()) return this;
if (other.getMarketId() != 0L) {
setMarketId(other.getMarketId());
}
if (other.getRatio() != 0) {
setRatio(other.getRatio());
}
if (!other.getSymbol().isEmpty()) {
symbol_ = other.symbol_;
bitField0_ |= 0x00000004;
onChanged();
}
if (!other.getLongSymbol().isEmpty()) {
longSymbol_ = other.longSymbol_;
bitField0_ |= 0x00000008;
onChanged();
}
if (other.getLegOptionDelta() != 0F) {
setLegOptionDelta(other.getLegOptionDelta());
}
if (other.getLegPrice() != 0F) {
setLegPrice(other.getLegPrice());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
marketId_ = input.readSInt64();
bitField0_ |= 0x00000001;
break;
} // case 8
case 16: {
ratio_ = input.readSInt32();
bitField0_ |= 0x00000002;
break;
} // case 16
case 26: {
symbol_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000004;
break;
} // case 26
case 34: {
longSymbol_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000008;
break;
} // case 34
case 45: {
legOptionDelta_ = input.readFloat();
bitField0_ |= 0x00000010;
break;
} // case 45
case 53: {
legPrice_ = input.readFloat();
bitField0_ |= 0x00000020;
break;
} // case 53
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private long marketId_ ;
/**
*
* The marketId of the leg
*
*
* sint64 marketId = 1;
* @return The marketId.
*/
@java.lang.Override
public long getMarketId() {
return marketId_;
}
/**
*
* The marketId of the leg
*
*
* sint64 marketId = 1;
* @param value The marketId to set.
* @return This builder for chaining.
*/
public Builder setMarketId(long value) {
marketId_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* The marketId of the leg
*
*
* sint64 marketId = 1;
* @return This builder for chaining.
*/
public Builder clearMarketId() {
bitField0_ = (bitField0_ & ~0x00000001);
marketId_ = 0L;
onChanged();
return this;
}
private int ratio_ ;
/**
*
* The ratio of the this leg with respect to the spread.
* Negative means short the absolute value, positive means long the absolute value.
* FIXME? Alternatively, we could have separate LONG/SHORT indicator in the message
* and use this field as only the absolute value
*
*
* sint32 ratio = 2;
* @return The ratio.
*/
@java.lang.Override
public int getRatio() {
return ratio_;
}
/**
*
* The ratio of the this leg with respect to the spread.
* Negative means short the absolute value, positive means long the absolute value.
* FIXME? Alternatively, we could have separate LONG/SHORT indicator in the message
* and use this field as only the absolute value
*
*
* sint32 ratio = 2;
* @param value The ratio to set.
* @return This builder for chaining.
*/
public Builder setRatio(int value) {
ratio_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
* The ratio of the this leg with respect to the spread.
* Negative means short the absolute value, positive means long the absolute value.
* FIXME? Alternatively, we could have separate LONG/SHORT indicator in the message
* and use this field as only the absolute value
*
*
* sint32 ratio = 2;
* @return This builder for chaining.
*/
public Builder clearRatio() {
bitField0_ = (bitField0_ & ~0x00000002);
ratio_ = 0;
onChanged();
return this;
}
private java.lang.Object symbol_ = "";
/**
* string symbol = 3;
* @return The symbol.
*/
public java.lang.String getSymbol() {
java.lang.Object ref = symbol_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
symbol_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string symbol = 3;
* @return The bytes for symbol.
*/
public com.google.protobuf.ByteString
getSymbolBytes() {
java.lang.Object ref = symbol_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
symbol_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string symbol = 3;
* @param value The symbol to set.
* @return This builder for chaining.
*/
public Builder setSymbol(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
symbol_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
* string symbol = 3;
* @return This builder for chaining.
*/
public Builder clearSymbol() {
symbol_ = getDefaultInstance().getSymbol();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
return this;
}
/**
* string symbol = 3;
* @param value The bytes for symbol to set.
* @return This builder for chaining.
*/
public Builder setSymbolBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
symbol_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
private java.lang.Object longSymbol_ = "";
/**
* string longSymbol = 4;
* @return The longSymbol.
*/
public java.lang.String getLongSymbol() {
java.lang.Object ref = longSymbol_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
longSymbol_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string longSymbol = 4;
* @return The bytes for longSymbol.
*/
public com.google.protobuf.ByteString
getLongSymbolBytes() {
java.lang.Object ref = longSymbol_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
longSymbol_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string longSymbol = 4;
* @param value The longSymbol to set.
* @return This builder for chaining.
*/
public Builder setLongSymbol(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
longSymbol_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
* string longSymbol = 4;
* @return This builder for chaining.
*/
public Builder clearLongSymbol() {
longSymbol_ = getDefaultInstance().getLongSymbol();
bitField0_ = (bitField0_ & ~0x00000008);
onChanged();
return this;
}
/**
* string longSymbol = 4;
* @param value The bytes for longSymbol to set.
* @return This builder for chaining.
*/
public Builder setLongSymbolBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
longSymbol_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
private float legOptionDelta_ ;
/**
* float legOptionDelta = 5;
* @return The legOptionDelta.
*/
@java.lang.Override
public float getLegOptionDelta() {
return legOptionDelta_;
}
/**
* float legOptionDelta = 5;
* @param value The legOptionDelta to set.
* @return This builder for chaining.
*/
public Builder setLegOptionDelta(float value) {
legOptionDelta_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
* float legOptionDelta = 5;
* @return This builder for chaining.
*/
public Builder clearLegOptionDelta() {
bitField0_ = (bitField0_ & ~0x00000010);
legOptionDelta_ = 0F;
onChanged();
return this;
}
private float legPrice_ ;
/**
*
* Additional information about the leg will be found in the instrument definition
* for the leg. It is not included here to reduce duplication.
*
*
* float legPrice = 6;
* @return The legPrice.
*/
@java.lang.Override
public float getLegPrice() {
return legPrice_;
}
/**
*
* Additional information about the leg will be found in the instrument definition
* for the leg. It is not included here to reduce duplication.
*
*
* float legPrice = 6;
* @param value The legPrice to set.
* @return This builder for chaining.
*/
public Builder setLegPrice(float value) {
legPrice_ = value;
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
*
* Additional information about the leg will be found in the instrument definition
* for the leg. It is not included here to reduce duplication.
*
*
* float legPrice = 6;
* @return This builder for chaining.
*/
public Builder clearLegPrice() {
bitField0_ = (bitField0_ & ~0x00000020);
legPrice_ = 0F;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:org.openfeed.InstrumentDefinition.SpreadLeg)
}
// @@protoc_insertion_point(class_scope:org.openfeed.InstrumentDefinition.SpreadLeg)
private static final org.openfeed.InstrumentDefinition.SpreadLeg DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.openfeed.InstrumentDefinition.SpreadLeg();
}
public static org.openfeed.InstrumentDefinition.SpreadLeg getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public SpreadLeg parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.openfeed.InstrumentDefinition.SpreadLeg getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface MaturityDateOrBuilder extends
// @@protoc_insertion_point(interface_extends:org.openfeed.InstrumentDefinition.MaturityDate)
com.google.protobuf.MessageOrBuilder {
/**
*
* Year of century.
*
*
* sint32 year = 1;
* @return The year.
*/
int getYear();
/**
*
* Month of year.
*
*
* sint32 month = 2;
* @return The month.
*/
int getMonth();
/**
*
* Day of month.
*
*
* sint32 day = 3;
* @return The day.
*/
int getDay();
}
/**
*
* / Date and time with time zone.
*
*
* Protobuf type {@code org.openfeed.InstrumentDefinition.MaturityDate}
*/
public static final class MaturityDate extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:org.openfeed.InstrumentDefinition.MaturityDate)
MaturityDateOrBuilder {
private static final long serialVersionUID = 0L;
static {
com.google.protobuf.RuntimeVersion.validateProtobufGencodeVersion(
com.google.protobuf.RuntimeVersion.RuntimeDomain.PUBLIC,
/* major= */ 4,
/* minor= */ 28,
/* patch= */ 3,
/* suffix= */ "",
MaturityDate.class.getName());
}
// Use MaturityDate.newBuilder() to construct.
private MaturityDate(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private MaturityDate() {
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.openfeed.OpenfeedInstrument.internal_static_org_openfeed_InstrumentDefinition_MaturityDate_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.openfeed.OpenfeedInstrument.internal_static_org_openfeed_InstrumentDefinition_MaturityDate_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.openfeed.InstrumentDefinition.MaturityDate.class, org.openfeed.InstrumentDefinition.MaturityDate.Builder.class);
}
public static final int YEAR_FIELD_NUMBER = 1;
private int year_ = 0;
/**
*
* Year of century.
*
*
* sint32 year = 1;
* @return The year.
*/
@java.lang.Override
public int getYear() {
return year_;
}
public static final int MONTH_FIELD_NUMBER = 2;
private int month_ = 0;
/**
*
* Month of year.
*
*
* sint32 month = 2;
* @return The month.
*/
@java.lang.Override
public int getMonth() {
return month_;
}
public static final int DAY_FIELD_NUMBER = 3;
private int day_ = 0;
/**
*
* Day of month.
*
*
* sint32 day = 3;
* @return The day.
*/
@java.lang.Override
public int getDay() {
return day_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (year_ != 0) {
output.writeSInt32(1, year_);
}
if (month_ != 0) {
output.writeSInt32(2, month_);
}
if (day_ != 0) {
output.writeSInt32(3, day_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (year_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeSInt32Size(1, year_);
}
if (month_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeSInt32Size(2, month_);
}
if (day_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeSInt32Size(3, day_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.openfeed.InstrumentDefinition.MaturityDate)) {
return super.equals(obj);
}
org.openfeed.InstrumentDefinition.MaturityDate other = (org.openfeed.InstrumentDefinition.MaturityDate) obj;
if (getYear()
!= other.getYear()) return false;
if (getMonth()
!= other.getMonth()) return false;
if (getDay()
!= other.getDay()) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + YEAR_FIELD_NUMBER;
hash = (53 * hash) + getYear();
hash = (37 * hash) + MONTH_FIELD_NUMBER;
hash = (53 * hash) + getMonth();
hash = (37 * hash) + DAY_FIELD_NUMBER;
hash = (53 * hash) + getDay();
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.openfeed.InstrumentDefinition.MaturityDate parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.openfeed.InstrumentDefinition.MaturityDate parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.openfeed.InstrumentDefinition.MaturityDate parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.openfeed.InstrumentDefinition.MaturityDate parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.openfeed.InstrumentDefinition.MaturityDate parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.openfeed.InstrumentDefinition.MaturityDate parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.openfeed.InstrumentDefinition.MaturityDate parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static org.openfeed.InstrumentDefinition.MaturityDate parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.openfeed.InstrumentDefinition.MaturityDate parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input);
}
public static org.openfeed.InstrumentDefinition.MaturityDate parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.openfeed.InstrumentDefinition.MaturityDate parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static org.openfeed.InstrumentDefinition.MaturityDate parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.openfeed.InstrumentDefinition.MaturityDate prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* / Date and time with time zone.
*
*
* Protobuf type {@code org.openfeed.InstrumentDefinition.MaturityDate}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:org.openfeed.InstrumentDefinition.MaturityDate)
org.openfeed.InstrumentDefinition.MaturityDateOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.openfeed.OpenfeedInstrument.internal_static_org_openfeed_InstrumentDefinition_MaturityDate_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.openfeed.OpenfeedInstrument.internal_static_org_openfeed_InstrumentDefinition_MaturityDate_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.openfeed.InstrumentDefinition.MaturityDate.class, org.openfeed.InstrumentDefinition.MaturityDate.Builder.class);
}
// Construct using org.openfeed.InstrumentDefinition.MaturityDate.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
year_ = 0;
month_ = 0;
day_ = 0;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.openfeed.OpenfeedInstrument.internal_static_org_openfeed_InstrumentDefinition_MaturityDate_descriptor;
}
@java.lang.Override
public org.openfeed.InstrumentDefinition.MaturityDate getDefaultInstanceForType() {
return org.openfeed.InstrumentDefinition.MaturityDate.getDefaultInstance();
}
@java.lang.Override
public org.openfeed.InstrumentDefinition.MaturityDate build() {
org.openfeed.InstrumentDefinition.MaturityDate result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.openfeed.InstrumentDefinition.MaturityDate buildPartial() {
org.openfeed.InstrumentDefinition.MaturityDate result = new org.openfeed.InstrumentDefinition.MaturityDate(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(org.openfeed.InstrumentDefinition.MaturityDate result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.year_ = year_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.month_ = month_;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.day_ = day_;
}
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.openfeed.InstrumentDefinition.MaturityDate) {
return mergeFrom((org.openfeed.InstrumentDefinition.MaturityDate)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.openfeed.InstrumentDefinition.MaturityDate other) {
if (other == org.openfeed.InstrumentDefinition.MaturityDate.getDefaultInstance()) return this;
if (other.getYear() != 0) {
setYear(other.getYear());
}
if (other.getMonth() != 0) {
setMonth(other.getMonth());
}
if (other.getDay() != 0) {
setDay(other.getDay());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
year_ = input.readSInt32();
bitField0_ |= 0x00000001;
break;
} // case 8
case 16: {
month_ = input.readSInt32();
bitField0_ |= 0x00000002;
break;
} // case 16
case 24: {
day_ = input.readSInt32();
bitField0_ |= 0x00000004;
break;
} // case 24
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private int year_ ;
/**
*
* Year of century.
*
*
* sint32 year = 1;
* @return The year.
*/
@java.lang.Override
public int getYear() {
return year_;
}
/**
*
* Year of century.
*
*
* sint32 year = 1;
* @param value The year to set.
* @return This builder for chaining.
*/
public Builder setYear(int value) {
year_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* Year of century.
*
*
* sint32 year = 1;
* @return This builder for chaining.
*/
public Builder clearYear() {
bitField0_ = (bitField0_ & ~0x00000001);
year_ = 0;
onChanged();
return this;
}
private int month_ ;
/**
*
* Month of year.
*
*
* sint32 month = 2;
* @return The month.
*/
@java.lang.Override
public int getMonth() {
return month_;
}
/**
*
* Month of year.
*
*
* sint32 month = 2;
* @param value The month to set.
* @return This builder for chaining.
*/
public Builder setMonth(int value) {
month_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
* Month of year.
*
*
* sint32 month = 2;
* @return This builder for chaining.
*/
public Builder clearMonth() {
bitField0_ = (bitField0_ & ~0x00000002);
month_ = 0;
onChanged();
return this;
}
private int day_ ;
/**
*
* Day of month.
*
*
* sint32 day = 3;
* @return The day.
*/
@java.lang.Override
public int getDay() {
return day_;
}
/**
*
* Day of month.
*
*
* sint32 day = 3;
* @param value The day to set.
* @return This builder for chaining.
*/
public Builder setDay(int value) {
day_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
* Day of month.
*
*
* sint32 day = 3;
* @return This builder for chaining.
*/
public Builder clearDay() {
bitField0_ = (bitField0_ & ~0x00000004);
day_ = 0;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:org.openfeed.InstrumentDefinition.MaturityDate)
}
// @@protoc_insertion_point(class_scope:org.openfeed.InstrumentDefinition.MaturityDate)
private static final org.openfeed.InstrumentDefinition.MaturityDate DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.openfeed.InstrumentDefinition.MaturityDate();
}
public static org.openfeed.InstrumentDefinition.MaturityDate getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public MaturityDate parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.openfeed.InstrumentDefinition.MaturityDate getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface SymbolOrBuilder extends
// @@protoc_insertion_point(interface_extends:org.openfeed.InstrumentDefinition.Symbol)
com.google.protobuf.MessageOrBuilder {
/**
*
* The vendor that provides this symbol.
*
*
* string vendor = 1;
* @return The vendor.
*/
java.lang.String getVendor();
/**
*
* The vendor that provides this symbol.
*
*
* string vendor = 1;
* @return The bytes for vendor.
*/
com.google.protobuf.ByteString
getVendorBytes();
/**
*
* The symbol assigned by the vendor.
*
*
* string symbol = 2;
* @return The symbol.
*/
java.lang.String getSymbol();
/**
*
* The symbol assigned by the vendor.
*
*
* string symbol = 2;
* @return The bytes for symbol.
*/
com.google.protobuf.ByteString
getSymbolBytes();
/**
*
* The long symbol assigned by the vendor. Includes 2 char year for futures.
*
*
* string longSymbol = 3;
* @return The longSymbol.
*/
java.lang.String getLongSymbol();
/**
*
* The long symbol assigned by the vendor. Includes 2 char year for futures.
*
*
* string longSymbol = 3;
* @return The bytes for longSymbol.
*/
com.google.protobuf.ByteString
getLongSymbolBytes();
}
/**
*
* / A vendor's symbol for an instrument
*
*
* Protobuf type {@code org.openfeed.InstrumentDefinition.Symbol}
*/
public static final class Symbol extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:org.openfeed.InstrumentDefinition.Symbol)
SymbolOrBuilder {
private static final long serialVersionUID = 0L;
static {
com.google.protobuf.RuntimeVersion.validateProtobufGencodeVersion(
com.google.protobuf.RuntimeVersion.RuntimeDomain.PUBLIC,
/* major= */ 4,
/* minor= */ 28,
/* patch= */ 3,
/* suffix= */ "",
Symbol.class.getName());
}
// Use Symbol.newBuilder() to construct.
private Symbol(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private Symbol() {
vendor_ = "";
symbol_ = "";
longSymbol_ = "";
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.openfeed.OpenfeedInstrument.internal_static_org_openfeed_InstrumentDefinition_Symbol_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.openfeed.OpenfeedInstrument.internal_static_org_openfeed_InstrumentDefinition_Symbol_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.openfeed.InstrumentDefinition.Symbol.class, org.openfeed.InstrumentDefinition.Symbol.Builder.class);
}
public static final int VENDOR_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private volatile java.lang.Object vendor_ = "";
/**
*
* The vendor that provides this symbol.
*
*
* string vendor = 1;
* @return The vendor.
*/
@java.lang.Override
public java.lang.String getVendor() {
java.lang.Object ref = vendor_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
vendor_ = s;
return s;
}
}
/**
*
* The vendor that provides this symbol.
*
*
* string vendor = 1;
* @return The bytes for vendor.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getVendorBytes() {
java.lang.Object ref = vendor_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
vendor_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SYMBOL_FIELD_NUMBER = 2;
@SuppressWarnings("serial")
private volatile java.lang.Object symbol_ = "";
/**
*
* The symbol assigned by the vendor.
*
*
* string symbol = 2;
* @return The symbol.
*/
@java.lang.Override
public java.lang.String getSymbol() {
java.lang.Object ref = symbol_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
symbol_ = s;
return s;
}
}
/**
*
* The symbol assigned by the vendor.
*
*
* string symbol = 2;
* @return The bytes for symbol.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getSymbolBytes() {
java.lang.Object ref = symbol_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
symbol_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int LONGSYMBOL_FIELD_NUMBER = 3;
@SuppressWarnings("serial")
private volatile java.lang.Object longSymbol_ = "";
/**
*
* The long symbol assigned by the vendor. Includes 2 char year for futures.
*
*
* string longSymbol = 3;
* @return The longSymbol.
*/
@java.lang.Override
public java.lang.String getLongSymbol() {
java.lang.Object ref = longSymbol_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
longSymbol_ = s;
return s;
}
}
/**
*
* The long symbol assigned by the vendor. Includes 2 char year for futures.
*
*
* string longSymbol = 3;
* @return The bytes for longSymbol.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getLongSymbolBytes() {
java.lang.Object ref = longSymbol_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
longSymbol_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(vendor_)) {
com.google.protobuf.GeneratedMessage.writeString(output, 1, vendor_);
}
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(symbol_)) {
com.google.protobuf.GeneratedMessage.writeString(output, 2, symbol_);
}
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(longSymbol_)) {
com.google.protobuf.GeneratedMessage.writeString(output, 3, longSymbol_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(vendor_)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(1, vendor_);
}
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(symbol_)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(2, symbol_);
}
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(longSymbol_)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(3, longSymbol_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.openfeed.InstrumentDefinition.Symbol)) {
return super.equals(obj);
}
org.openfeed.InstrumentDefinition.Symbol other = (org.openfeed.InstrumentDefinition.Symbol) obj;
if (!getVendor()
.equals(other.getVendor())) return false;
if (!getSymbol()
.equals(other.getSymbol())) return false;
if (!getLongSymbol()
.equals(other.getLongSymbol())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + VENDOR_FIELD_NUMBER;
hash = (53 * hash) + getVendor().hashCode();
hash = (37 * hash) + SYMBOL_FIELD_NUMBER;
hash = (53 * hash) + getSymbol().hashCode();
hash = (37 * hash) + LONGSYMBOL_FIELD_NUMBER;
hash = (53 * hash) + getLongSymbol().hashCode();
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.openfeed.InstrumentDefinition.Symbol parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.openfeed.InstrumentDefinition.Symbol parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.openfeed.InstrumentDefinition.Symbol parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.openfeed.InstrumentDefinition.Symbol parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.openfeed.InstrumentDefinition.Symbol parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.openfeed.InstrumentDefinition.Symbol parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.openfeed.InstrumentDefinition.Symbol parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static org.openfeed.InstrumentDefinition.Symbol parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.openfeed.InstrumentDefinition.Symbol parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input);
}
public static org.openfeed.InstrumentDefinition.Symbol parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.openfeed.InstrumentDefinition.Symbol parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static org.openfeed.InstrumentDefinition.Symbol parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.openfeed.InstrumentDefinition.Symbol prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* / A vendor's symbol for an instrument
*
*
* Protobuf type {@code org.openfeed.InstrumentDefinition.Symbol}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:org.openfeed.InstrumentDefinition.Symbol)
org.openfeed.InstrumentDefinition.SymbolOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.openfeed.OpenfeedInstrument.internal_static_org_openfeed_InstrumentDefinition_Symbol_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.openfeed.OpenfeedInstrument.internal_static_org_openfeed_InstrumentDefinition_Symbol_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.openfeed.InstrumentDefinition.Symbol.class, org.openfeed.InstrumentDefinition.Symbol.Builder.class);
}
// Construct using org.openfeed.InstrumentDefinition.Symbol.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
vendor_ = "";
symbol_ = "";
longSymbol_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.openfeed.OpenfeedInstrument.internal_static_org_openfeed_InstrumentDefinition_Symbol_descriptor;
}
@java.lang.Override
public org.openfeed.InstrumentDefinition.Symbol getDefaultInstanceForType() {
return org.openfeed.InstrumentDefinition.Symbol.getDefaultInstance();
}
@java.lang.Override
public org.openfeed.InstrumentDefinition.Symbol build() {
org.openfeed.InstrumentDefinition.Symbol result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.openfeed.InstrumentDefinition.Symbol buildPartial() {
org.openfeed.InstrumentDefinition.Symbol result = new org.openfeed.InstrumentDefinition.Symbol(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(org.openfeed.InstrumentDefinition.Symbol result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.vendor_ = vendor_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.symbol_ = symbol_;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.longSymbol_ = longSymbol_;
}
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.openfeed.InstrumentDefinition.Symbol) {
return mergeFrom((org.openfeed.InstrumentDefinition.Symbol)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.openfeed.InstrumentDefinition.Symbol other) {
if (other == org.openfeed.InstrumentDefinition.Symbol.getDefaultInstance()) return this;
if (!other.getVendor().isEmpty()) {
vendor_ = other.vendor_;
bitField0_ |= 0x00000001;
onChanged();
}
if (!other.getSymbol().isEmpty()) {
symbol_ = other.symbol_;
bitField0_ |= 0x00000002;
onChanged();
}
if (!other.getLongSymbol().isEmpty()) {
longSymbol_ = other.longSymbol_;
bitField0_ |= 0x00000004;
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
vendor_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000001;
break;
} // case 10
case 18: {
symbol_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000002;
break;
} // case 18
case 26: {
longSymbol_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000004;
break;
} // case 26
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.lang.Object vendor_ = "";
/**
*
* The vendor that provides this symbol.
*
*
* string vendor = 1;
* @return The vendor.
*/
public java.lang.String getVendor() {
java.lang.Object ref = vendor_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
vendor_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* The vendor that provides this symbol.
*
*
* string vendor = 1;
* @return The bytes for vendor.
*/
public com.google.protobuf.ByteString
getVendorBytes() {
java.lang.Object ref = vendor_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
vendor_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* The vendor that provides this symbol.
*
*
* string vendor = 1;
* @param value The vendor to set.
* @return This builder for chaining.
*/
public Builder setVendor(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
vendor_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* The vendor that provides this symbol.
*
*
* string vendor = 1;
* @return This builder for chaining.
*/
public Builder clearVendor() {
vendor_ = getDefaultInstance().getVendor();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
*
* The vendor that provides this symbol.
*
*
* string vendor = 1;
* @param value The bytes for vendor to set.
* @return This builder for chaining.
*/
public Builder setVendorBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
vendor_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
private java.lang.Object symbol_ = "";
/**
*
* The symbol assigned by the vendor.
*
*
* string symbol = 2;
* @return The symbol.
*/
public java.lang.String getSymbol() {
java.lang.Object ref = symbol_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
symbol_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* The symbol assigned by the vendor.
*
*
* string symbol = 2;
* @return The bytes for symbol.
*/
public com.google.protobuf.ByteString
getSymbolBytes() {
java.lang.Object ref = symbol_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
symbol_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* The symbol assigned by the vendor.
*
*
* string symbol = 2;
* @param value The symbol to set.
* @return This builder for chaining.
*/
public Builder setSymbol(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
symbol_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
* The symbol assigned by the vendor.
*
*
* string symbol = 2;
* @return This builder for chaining.
*/
public Builder clearSymbol() {
symbol_ = getDefaultInstance().getSymbol();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
*
* The symbol assigned by the vendor.
*
*
* string symbol = 2;
* @param value The bytes for symbol to set.
* @return This builder for chaining.
*/
public Builder setSymbolBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
symbol_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
private java.lang.Object longSymbol_ = "";
/**
*
* The long symbol assigned by the vendor. Includes 2 char year for futures.
*
*
* string longSymbol = 3;
* @return The longSymbol.
*/
public java.lang.String getLongSymbol() {
java.lang.Object ref = longSymbol_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
longSymbol_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* The long symbol assigned by the vendor. Includes 2 char year for futures.
*
*
* string longSymbol = 3;
* @return The bytes for longSymbol.
*/
public com.google.protobuf.ByteString
getLongSymbolBytes() {
java.lang.Object ref = longSymbol_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
longSymbol_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* The long symbol assigned by the vendor. Includes 2 char year for futures.
*
*
* string longSymbol = 3;
* @param value The longSymbol to set.
* @return This builder for chaining.
*/
public Builder setLongSymbol(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
longSymbol_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
* The long symbol assigned by the vendor. Includes 2 char year for futures.
*
*
* string longSymbol = 3;
* @return This builder for chaining.
*/
public Builder clearLongSymbol() {
longSymbol_ = getDefaultInstance().getLongSymbol();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
return this;
}
/**
*
* The long symbol assigned by the vendor. Includes 2 char year for futures.
*
*
* string longSymbol = 3;
* @param value The bytes for longSymbol to set.
* @return This builder for chaining.
*/
public Builder setLongSymbolBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
longSymbol_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:org.openfeed.InstrumentDefinition.Symbol)
}
// @@protoc_insertion_point(class_scope:org.openfeed.InstrumentDefinition.Symbol)
private static final org.openfeed.InstrumentDefinition.Symbol DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.openfeed.InstrumentDefinition.Symbol();
}
public static org.openfeed.InstrumentDefinition.Symbol getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Symbol parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.openfeed.InstrumentDefinition.Symbol getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface PriceFormatOrBuilder extends
// @@protoc_insertion_point(interface_extends:org.openfeed.InstrumentDefinition.PriceFormat)
com.google.protobuf.MessageOrBuilder {
/**
* bool isFractional = 1;
* @return The isFractional.
*/
boolean getIsFractional();
/**
* sint32 denominator = 2;
* @return The denominator.
*/
int getDenominator();
/**
* sint32 subDenominator = 4;
* @return The subDenominator.
*/
int getSubDenominator();
/**
* .org.openfeed.InstrumentDefinition.PriceFormat.SubFormat subFormat = 6;
* @return The enum numeric value on the wire for subFormat.
*/
int getSubFormatValue();
/**
* .org.openfeed.InstrumentDefinition.PriceFormat.SubFormat subFormat = 6;
* @return The subFormat.
*/
org.openfeed.InstrumentDefinition.PriceFormat.SubFormat getSubFormat();
}
/**
*
* / Recommended display format for prices.
*
*
* Protobuf type {@code org.openfeed.InstrumentDefinition.PriceFormat}
*/
public static final class PriceFormat extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:org.openfeed.InstrumentDefinition.PriceFormat)
PriceFormatOrBuilder {
private static final long serialVersionUID = 0L;
static {
com.google.protobuf.RuntimeVersion.validateProtobufGencodeVersion(
com.google.protobuf.RuntimeVersion.RuntimeDomain.PUBLIC,
/* major= */ 4,
/* minor= */ 28,
/* patch= */ 3,
/* suffix= */ "",
PriceFormat.class.getName());
}
// Use PriceFormat.newBuilder() to construct.
private PriceFormat(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private PriceFormat() {
subFormat_ = 0;
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.openfeed.OpenfeedInstrument.internal_static_org_openfeed_InstrumentDefinition_PriceFormat_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.openfeed.OpenfeedInstrument.internal_static_org_openfeed_InstrumentDefinition_PriceFormat_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.openfeed.InstrumentDefinition.PriceFormat.class, org.openfeed.InstrumentDefinition.PriceFormat.Builder.class);
}
/**
* Protobuf enum {@code org.openfeed.InstrumentDefinition.PriceFormat.SubFormat}
*/
public enum SubFormat
implements com.google.protobuf.ProtocolMessageEnum {
/**
* FLAT = 0;
*/
FLAT(0),
/**
* FRACTIONAL = 1;
*/
FRACTIONAL(1),
/**
* DECIMAL = 2;
*/
DECIMAL(2),
UNRECOGNIZED(-1),
;
static {
com.google.protobuf.RuntimeVersion.validateProtobufGencodeVersion(
com.google.protobuf.RuntimeVersion.RuntimeDomain.PUBLIC,
/* major= */ 4,
/* minor= */ 28,
/* patch= */ 3,
/* suffix= */ "",
SubFormat.class.getName());
}
/**
* FLAT = 0;
*/
public static final int FLAT_VALUE = 0;
/**
* FRACTIONAL = 1;
*/
public static final int FRACTIONAL_VALUE = 1;
/**
* DECIMAL = 2;
*/
public static final int DECIMAL_VALUE = 2;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static SubFormat valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static SubFormat forNumber(int value) {
switch (value) {
case 0: return FLAT;
case 1: return FRACTIONAL;
case 2: return DECIMAL;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
SubFormat> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public SubFormat findValueByNumber(int number) {
return SubFormat.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return org.openfeed.InstrumentDefinition.PriceFormat.getDescriptor().getEnumTypes().get(0);
}
private static final SubFormat[] VALUES = values();
public static SubFormat valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private SubFormat(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:org.openfeed.InstrumentDefinition.PriceFormat.SubFormat)
}
public static final int ISFRACTIONAL_FIELD_NUMBER = 1;
private boolean isFractional_ = false;
/**
* bool isFractional = 1;
* @return The isFractional.
*/
@java.lang.Override
public boolean getIsFractional() {
return isFractional_;
}
public static final int DENOMINATOR_FIELD_NUMBER = 2;
private int denominator_ = 0;
/**
* sint32 denominator = 2;
* @return The denominator.
*/
@java.lang.Override
public int getDenominator() {
return denominator_;
}
public static final int SUBDENOMINATOR_FIELD_NUMBER = 4;
private int subDenominator_ = 0;
/**
* sint32 subDenominator = 4;
* @return The subDenominator.
*/
@java.lang.Override
public int getSubDenominator() {
return subDenominator_;
}
public static final int SUBFORMAT_FIELD_NUMBER = 6;
private int subFormat_ = 0;
/**
* .org.openfeed.InstrumentDefinition.PriceFormat.SubFormat subFormat = 6;
* @return The enum numeric value on the wire for subFormat.
*/
@java.lang.Override public int getSubFormatValue() {
return subFormat_;
}
/**
* .org.openfeed.InstrumentDefinition.PriceFormat.SubFormat subFormat = 6;
* @return The subFormat.
*/
@java.lang.Override public org.openfeed.InstrumentDefinition.PriceFormat.SubFormat getSubFormat() {
org.openfeed.InstrumentDefinition.PriceFormat.SubFormat result = org.openfeed.InstrumentDefinition.PriceFormat.SubFormat.forNumber(subFormat_);
return result == null ? org.openfeed.InstrumentDefinition.PriceFormat.SubFormat.UNRECOGNIZED : result;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (isFractional_ != false) {
output.writeBool(1, isFractional_);
}
if (denominator_ != 0) {
output.writeSInt32(2, denominator_);
}
if (subDenominator_ != 0) {
output.writeSInt32(4, subDenominator_);
}
if (subFormat_ != org.openfeed.InstrumentDefinition.PriceFormat.SubFormat.FLAT.getNumber()) {
output.writeEnum(6, subFormat_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (isFractional_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(1, isFractional_);
}
if (denominator_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeSInt32Size(2, denominator_);
}
if (subDenominator_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeSInt32Size(4, subDenominator_);
}
if (subFormat_ != org.openfeed.InstrumentDefinition.PriceFormat.SubFormat.FLAT.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(6, subFormat_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.openfeed.InstrumentDefinition.PriceFormat)) {
return super.equals(obj);
}
org.openfeed.InstrumentDefinition.PriceFormat other = (org.openfeed.InstrumentDefinition.PriceFormat) obj;
if (getIsFractional()
!= other.getIsFractional()) return false;
if (getDenominator()
!= other.getDenominator()) return false;
if (getSubDenominator()
!= other.getSubDenominator()) return false;
if (subFormat_ != other.subFormat_) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + ISFRACTIONAL_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getIsFractional());
hash = (37 * hash) + DENOMINATOR_FIELD_NUMBER;
hash = (53 * hash) + getDenominator();
hash = (37 * hash) + SUBDENOMINATOR_FIELD_NUMBER;
hash = (53 * hash) + getSubDenominator();
hash = (37 * hash) + SUBFORMAT_FIELD_NUMBER;
hash = (53 * hash) + subFormat_;
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.openfeed.InstrumentDefinition.PriceFormat parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.openfeed.InstrumentDefinition.PriceFormat parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.openfeed.InstrumentDefinition.PriceFormat parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.openfeed.InstrumentDefinition.PriceFormat parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.openfeed.InstrumentDefinition.PriceFormat parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.openfeed.InstrumentDefinition.PriceFormat parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.openfeed.InstrumentDefinition.PriceFormat parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static org.openfeed.InstrumentDefinition.PriceFormat parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.openfeed.InstrumentDefinition.PriceFormat parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input);
}
public static org.openfeed.InstrumentDefinition.PriceFormat parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.openfeed.InstrumentDefinition.PriceFormat parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static org.openfeed.InstrumentDefinition.PriceFormat parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.openfeed.InstrumentDefinition.PriceFormat prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* / Recommended display format for prices.
*
*
* Protobuf type {@code org.openfeed.InstrumentDefinition.PriceFormat}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:org.openfeed.InstrumentDefinition.PriceFormat)
org.openfeed.InstrumentDefinition.PriceFormatOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.openfeed.OpenfeedInstrument.internal_static_org_openfeed_InstrumentDefinition_PriceFormat_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.openfeed.OpenfeedInstrument.internal_static_org_openfeed_InstrumentDefinition_PriceFormat_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.openfeed.InstrumentDefinition.PriceFormat.class, org.openfeed.InstrumentDefinition.PriceFormat.Builder.class);
}
// Construct using org.openfeed.InstrumentDefinition.PriceFormat.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
isFractional_ = false;
denominator_ = 0;
subDenominator_ = 0;
subFormat_ = 0;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.openfeed.OpenfeedInstrument.internal_static_org_openfeed_InstrumentDefinition_PriceFormat_descriptor;
}
@java.lang.Override
public org.openfeed.InstrumentDefinition.PriceFormat getDefaultInstanceForType() {
return org.openfeed.InstrumentDefinition.PriceFormat.getDefaultInstance();
}
@java.lang.Override
public org.openfeed.InstrumentDefinition.PriceFormat build() {
org.openfeed.InstrumentDefinition.PriceFormat result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.openfeed.InstrumentDefinition.PriceFormat buildPartial() {
org.openfeed.InstrumentDefinition.PriceFormat result = new org.openfeed.InstrumentDefinition.PriceFormat(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(org.openfeed.InstrumentDefinition.PriceFormat result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.isFractional_ = isFractional_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.denominator_ = denominator_;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.subDenominator_ = subDenominator_;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.subFormat_ = subFormat_;
}
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.openfeed.InstrumentDefinition.PriceFormat) {
return mergeFrom((org.openfeed.InstrumentDefinition.PriceFormat)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.openfeed.InstrumentDefinition.PriceFormat other) {
if (other == org.openfeed.InstrumentDefinition.PriceFormat.getDefaultInstance()) return this;
if (other.getIsFractional() != false) {
setIsFractional(other.getIsFractional());
}
if (other.getDenominator() != 0) {
setDenominator(other.getDenominator());
}
if (other.getSubDenominator() != 0) {
setSubDenominator(other.getSubDenominator());
}
if (other.subFormat_ != 0) {
setSubFormatValue(other.getSubFormatValue());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
isFractional_ = input.readBool();
bitField0_ |= 0x00000001;
break;
} // case 8
case 16: {
denominator_ = input.readSInt32();
bitField0_ |= 0x00000002;
break;
} // case 16
case 32: {
subDenominator_ = input.readSInt32();
bitField0_ |= 0x00000004;
break;
} // case 32
case 48: {
subFormat_ = input.readEnum();
bitField0_ |= 0x00000008;
break;
} // case 48
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private boolean isFractional_ ;
/**
* bool isFractional = 1;
* @return The isFractional.
*/
@java.lang.Override
public boolean getIsFractional() {
return isFractional_;
}
/**
* bool isFractional = 1;
* @param value The isFractional to set.
* @return This builder for chaining.
*/
public Builder setIsFractional(boolean value) {
isFractional_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* bool isFractional = 1;
* @return This builder for chaining.
*/
public Builder clearIsFractional() {
bitField0_ = (bitField0_ & ~0x00000001);
isFractional_ = false;
onChanged();
return this;
}
private int denominator_ ;
/**
* sint32 denominator = 2;
* @return The denominator.
*/
@java.lang.Override
public int getDenominator() {
return denominator_;
}
/**
* sint32 denominator = 2;
* @param value The denominator to set.
* @return This builder for chaining.
*/
public Builder setDenominator(int value) {
denominator_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* sint32 denominator = 2;
* @return This builder for chaining.
*/
public Builder clearDenominator() {
bitField0_ = (bitField0_ & ~0x00000002);
denominator_ = 0;
onChanged();
return this;
}
private int subDenominator_ ;
/**
* sint32 subDenominator = 4;
* @return The subDenominator.
*/
@java.lang.Override
public int getSubDenominator() {
return subDenominator_;
}
/**
* sint32 subDenominator = 4;
* @param value The subDenominator to set.
* @return This builder for chaining.
*/
public Builder setSubDenominator(int value) {
subDenominator_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
* sint32 subDenominator = 4;
* @return This builder for chaining.
*/
public Builder clearSubDenominator() {
bitField0_ = (bitField0_ & ~0x00000004);
subDenominator_ = 0;
onChanged();
return this;
}
private int subFormat_ = 0;
/**
* .org.openfeed.InstrumentDefinition.PriceFormat.SubFormat subFormat = 6;
* @return The enum numeric value on the wire for subFormat.
*/
@java.lang.Override public int getSubFormatValue() {
return subFormat_;
}
/**
* .org.openfeed.InstrumentDefinition.PriceFormat.SubFormat subFormat = 6;
* @param value The enum numeric value on the wire for subFormat to set.
* @return This builder for chaining.
*/
public Builder setSubFormatValue(int value) {
subFormat_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
* .org.openfeed.InstrumentDefinition.PriceFormat.SubFormat subFormat = 6;
* @return The subFormat.
*/
@java.lang.Override
public org.openfeed.InstrumentDefinition.PriceFormat.SubFormat getSubFormat() {
org.openfeed.InstrumentDefinition.PriceFormat.SubFormat result = org.openfeed.InstrumentDefinition.PriceFormat.SubFormat.forNumber(subFormat_);
return result == null ? org.openfeed.InstrumentDefinition.PriceFormat.SubFormat.UNRECOGNIZED : result;
}
/**
* .org.openfeed.InstrumentDefinition.PriceFormat.SubFormat subFormat = 6;
* @param value The subFormat to set.
* @return This builder for chaining.
*/
public Builder setSubFormat(org.openfeed.InstrumentDefinition.PriceFormat.SubFormat value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000008;
subFormat_ = value.getNumber();
onChanged();
return this;
}
/**
* .org.openfeed.InstrumentDefinition.PriceFormat.SubFormat subFormat = 6;
* @return This builder for chaining.
*/
public Builder clearSubFormat() {
bitField0_ = (bitField0_ & ~0x00000008);
subFormat_ = 0;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:org.openfeed.InstrumentDefinition.PriceFormat)
}
// @@protoc_insertion_point(class_scope:org.openfeed.InstrumentDefinition.PriceFormat)
private static final org.openfeed.InstrumentDefinition.PriceFormat DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.openfeed.InstrumentDefinition.PriceFormat();
}
public static org.openfeed.InstrumentDefinition.PriceFormat getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public PriceFormat parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.openfeed.InstrumentDefinition.PriceFormat getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public interface CurrencyPairOrBuilder extends
// @@protoc_insertion_point(interface_extends:org.openfeed.InstrumentDefinition.CurrencyPair)
com.google.protobuf.MessageOrBuilder {
/**
* string currency1 = 1;
* @return The currency1.
*/
java.lang.String getCurrency1();
/**
* string currency1 = 1;
* @return The bytes for currency1.
*/
com.google.protobuf.ByteString
getCurrency1Bytes();
/**
* string currency2 = 2;
* @return The currency2.
*/
java.lang.String getCurrency2();
/**
* string currency2 = 2;
* @return The bytes for currency2.
*/
com.google.protobuf.ByteString
getCurrency2Bytes();
}
/**
*
* / Currency Pair
*
*
* Protobuf type {@code org.openfeed.InstrumentDefinition.CurrencyPair}
*/
public static final class CurrencyPair extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:org.openfeed.InstrumentDefinition.CurrencyPair)
CurrencyPairOrBuilder {
private static final long serialVersionUID = 0L;
static {
com.google.protobuf.RuntimeVersion.validateProtobufGencodeVersion(
com.google.protobuf.RuntimeVersion.RuntimeDomain.PUBLIC,
/* major= */ 4,
/* minor= */ 28,
/* patch= */ 3,
/* suffix= */ "",
CurrencyPair.class.getName());
}
// Use CurrencyPair.newBuilder() to construct.
private CurrencyPair(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private CurrencyPair() {
currency1_ = "";
currency2_ = "";
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.openfeed.OpenfeedInstrument.internal_static_org_openfeed_InstrumentDefinition_CurrencyPair_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.openfeed.OpenfeedInstrument.internal_static_org_openfeed_InstrumentDefinition_CurrencyPair_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.openfeed.InstrumentDefinition.CurrencyPair.class, org.openfeed.InstrumentDefinition.CurrencyPair.Builder.class);
}
public static final int CURRENCY1_FIELD_NUMBER = 1;
@SuppressWarnings("serial")
private volatile java.lang.Object currency1_ = "";
/**
* string currency1 = 1;
* @return The currency1.
*/
@java.lang.Override
public java.lang.String getCurrency1() {
java.lang.Object ref = currency1_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
currency1_ = s;
return s;
}
}
/**
* string currency1 = 1;
* @return The bytes for currency1.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getCurrency1Bytes() {
java.lang.Object ref = currency1_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
currency1_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CURRENCY2_FIELD_NUMBER = 2;
@SuppressWarnings("serial")
private volatile java.lang.Object currency2_ = "";
/**
* string currency2 = 2;
* @return The currency2.
*/
@java.lang.Override
public java.lang.String getCurrency2() {
java.lang.Object ref = currency2_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
currency2_ = s;
return s;
}
}
/**
* string currency2 = 2;
* @return The bytes for currency2.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getCurrency2Bytes() {
java.lang.Object ref = currency2_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
currency2_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(currency1_)) {
com.google.protobuf.GeneratedMessage.writeString(output, 1, currency1_);
}
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(currency2_)) {
com.google.protobuf.GeneratedMessage.writeString(output, 2, currency2_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(currency1_)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(1, currency1_);
}
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(currency2_)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(2, currency2_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.openfeed.InstrumentDefinition.CurrencyPair)) {
return super.equals(obj);
}
org.openfeed.InstrumentDefinition.CurrencyPair other = (org.openfeed.InstrumentDefinition.CurrencyPair) obj;
if (!getCurrency1()
.equals(other.getCurrency1())) return false;
if (!getCurrency2()
.equals(other.getCurrency2())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + CURRENCY1_FIELD_NUMBER;
hash = (53 * hash) + getCurrency1().hashCode();
hash = (37 * hash) + CURRENCY2_FIELD_NUMBER;
hash = (53 * hash) + getCurrency2().hashCode();
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.openfeed.InstrumentDefinition.CurrencyPair parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.openfeed.InstrumentDefinition.CurrencyPair parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.openfeed.InstrumentDefinition.CurrencyPair parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.openfeed.InstrumentDefinition.CurrencyPair parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.openfeed.InstrumentDefinition.CurrencyPair parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.openfeed.InstrumentDefinition.CurrencyPair parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.openfeed.InstrumentDefinition.CurrencyPair parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static org.openfeed.InstrumentDefinition.CurrencyPair parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.openfeed.InstrumentDefinition.CurrencyPair parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input);
}
public static org.openfeed.InstrumentDefinition.CurrencyPair parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.openfeed.InstrumentDefinition.CurrencyPair parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static org.openfeed.InstrumentDefinition.CurrencyPair parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.openfeed.InstrumentDefinition.CurrencyPair prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* / Currency Pair
*
*
* Protobuf type {@code org.openfeed.InstrumentDefinition.CurrencyPair}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:org.openfeed.InstrumentDefinition.CurrencyPair)
org.openfeed.InstrumentDefinition.CurrencyPairOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.openfeed.OpenfeedInstrument.internal_static_org_openfeed_InstrumentDefinition_CurrencyPair_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.openfeed.OpenfeedInstrument.internal_static_org_openfeed_InstrumentDefinition_CurrencyPair_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.openfeed.InstrumentDefinition.CurrencyPair.class, org.openfeed.InstrumentDefinition.CurrencyPair.Builder.class);
}
// Construct using org.openfeed.InstrumentDefinition.CurrencyPair.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
currency1_ = "";
currency2_ = "";
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.openfeed.OpenfeedInstrument.internal_static_org_openfeed_InstrumentDefinition_CurrencyPair_descriptor;
}
@java.lang.Override
public org.openfeed.InstrumentDefinition.CurrencyPair getDefaultInstanceForType() {
return org.openfeed.InstrumentDefinition.CurrencyPair.getDefaultInstance();
}
@java.lang.Override
public org.openfeed.InstrumentDefinition.CurrencyPair build() {
org.openfeed.InstrumentDefinition.CurrencyPair result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.openfeed.InstrumentDefinition.CurrencyPair buildPartial() {
org.openfeed.InstrumentDefinition.CurrencyPair result = new org.openfeed.InstrumentDefinition.CurrencyPair(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(org.openfeed.InstrumentDefinition.CurrencyPair result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.currency1_ = currency1_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.currency2_ = currency2_;
}
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.openfeed.InstrumentDefinition.CurrencyPair) {
return mergeFrom((org.openfeed.InstrumentDefinition.CurrencyPair)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.openfeed.InstrumentDefinition.CurrencyPair other) {
if (other == org.openfeed.InstrumentDefinition.CurrencyPair.getDefaultInstance()) return this;
if (!other.getCurrency1().isEmpty()) {
currency1_ = other.currency1_;
bitField0_ |= 0x00000001;
onChanged();
}
if (!other.getCurrency2().isEmpty()) {
currency2_ = other.currency2_;
bitField0_ |= 0x00000002;
onChanged();
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
currency1_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000001;
break;
} // case 10
case 18: {
currency2_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000002;
break;
} // case 18
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private java.lang.Object currency1_ = "";
/**
* string currency1 = 1;
* @return The currency1.
*/
public java.lang.String getCurrency1() {
java.lang.Object ref = currency1_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
currency1_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string currency1 = 1;
* @return The bytes for currency1.
*/
public com.google.protobuf.ByteString
getCurrency1Bytes() {
java.lang.Object ref = currency1_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
currency1_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string currency1 = 1;
* @param value The currency1 to set.
* @return This builder for chaining.
*/
public Builder setCurrency1(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
currency1_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* string currency1 = 1;
* @return This builder for chaining.
*/
public Builder clearCurrency1() {
currency1_ = getDefaultInstance().getCurrency1();
bitField0_ = (bitField0_ & ~0x00000001);
onChanged();
return this;
}
/**
* string currency1 = 1;
* @param value The bytes for currency1 to set.
* @return This builder for chaining.
*/
public Builder setCurrency1Bytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
currency1_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
private java.lang.Object currency2_ = "";
/**
* string currency2 = 2;
* @return The currency2.
*/
public java.lang.String getCurrency2() {
java.lang.Object ref = currency2_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
currency2_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string currency2 = 2;
* @return The bytes for currency2.
*/
public com.google.protobuf.ByteString
getCurrency2Bytes() {
java.lang.Object ref = currency2_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
currency2_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string currency2 = 2;
* @param value The currency2 to set.
* @return This builder for chaining.
*/
public Builder setCurrency2(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
currency2_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* string currency2 = 2;
* @return This builder for chaining.
*/
public Builder clearCurrency2() {
currency2_ = getDefaultInstance().getCurrency2();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
* string currency2 = 2;
* @param value The bytes for currency2 to set.
* @return This builder for chaining.
*/
public Builder setCurrency2Bytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
currency2_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:org.openfeed.InstrumentDefinition.CurrencyPair)
}
// @@protoc_insertion_point(class_scope:org.openfeed.InstrumentDefinition.CurrencyPair)
private static final org.openfeed.InstrumentDefinition.CurrencyPair DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.openfeed.InstrumentDefinition.CurrencyPair();
}
public static org.openfeed.InstrumentDefinition.CurrencyPair getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public CurrencyPair parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.openfeed.InstrumentDefinition.CurrencyPair getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
private int bitField0_;
public static final int MARKETID_FIELD_NUMBER = 1;
private long marketId_ = 0L;
/**
*
* / Unique ID used in the data feed.
*
*
* sint64 marketId = 1;
* @return The marketId.
*/
@java.lang.Override
public long getMarketId() {
return marketId_;
}
public static final int INSTRUMENTTYPE_FIELD_NUMBER = 2;
private int instrumentType_ = 0;
/**
*
* / Instrument type as enum...
*
*
* .org.openfeed.InstrumentDefinition.InstrumentType instrumentType = 2;
* @return The enum numeric value on the wire for instrumentType.
*/
@java.lang.Override public int getInstrumentTypeValue() {
return instrumentType_;
}
/**
*
* / Instrument type as enum...
*
*
* .org.openfeed.InstrumentDefinition.InstrumentType instrumentType = 2;
* @return The instrumentType.
*/
@java.lang.Override public org.openfeed.InstrumentDefinition.InstrumentType getInstrumentType() {
org.openfeed.InstrumentDefinition.InstrumentType result = org.openfeed.InstrumentDefinition.InstrumentType.forNumber(instrumentType_);
return result == null ? org.openfeed.InstrumentDefinition.InstrumentType.UNRECOGNIZED : result;
}
public static final int SUPPORTBOOKTYPES_FIELD_NUMBER = 3;
@SuppressWarnings("serial")
private com.google.protobuf.Internal.IntList supportBookTypes_;
private static final com.google.protobuf.Internal.IntListAdapter.IntConverter<
org.openfeed.InstrumentDefinition.BookType> supportBookTypes_converter_ =
new com.google.protobuf.Internal.IntListAdapter.IntConverter<
org.openfeed.InstrumentDefinition.BookType>() {
public org.openfeed.InstrumentDefinition.BookType convert(int from) {
org.openfeed.InstrumentDefinition.BookType result = org.openfeed.InstrumentDefinition.BookType.forNumber(from);
return result == null ? org.openfeed.InstrumentDefinition.BookType.UNRECOGNIZED : result;
}
};
/**
*
* / Supported Book Types
*
*
* repeated .org.openfeed.InstrumentDefinition.BookType supportBookTypes = 3;
* @return A list containing the supportBookTypes.
*/
@java.lang.Override
public java.util.List getSupportBookTypesList() {
return new com.google.protobuf.Internal.IntListAdapter<
org.openfeed.InstrumentDefinition.BookType>(supportBookTypes_, supportBookTypes_converter_);
}
/**
*
* / Supported Book Types
*
*
* repeated .org.openfeed.InstrumentDefinition.BookType supportBookTypes = 3;
* @return The count of supportBookTypes.
*/
@java.lang.Override
public int getSupportBookTypesCount() {
return supportBookTypes_.size();
}
/**
*
* / Supported Book Types
*
*
* repeated .org.openfeed.InstrumentDefinition.BookType supportBookTypes = 3;
* @param index The index of the element to return.
* @return The supportBookTypes at the given index.
*/
@java.lang.Override
public org.openfeed.InstrumentDefinition.BookType getSupportBookTypes(int index) {
return supportBookTypes_converter_.convert(supportBookTypes_.getInt(index));
}
/**
*
* / Supported Book Types
*
*
* repeated .org.openfeed.InstrumentDefinition.BookType supportBookTypes = 3;
* @return A list containing the enum numeric values on the wire for supportBookTypes.
*/
@java.lang.Override
public java.util.List
getSupportBookTypesValueList() {
return supportBookTypes_;
}
/**
*
* / Supported Book Types
*
*
* repeated .org.openfeed.InstrumentDefinition.BookType supportBookTypes = 3;
* @param index The index of the value to return.
* @return The enum numeric value on the wire of supportBookTypes at the given index.
*/
@java.lang.Override
public int getSupportBookTypesValue(int index) {
return supportBookTypes_.getInt(index);
}
private int supportBookTypesMemoizedSerializedSize;
public static final int BOOKDEPTH_FIELD_NUMBER = 4;
private int bookDepth_ = 0;
/**
*
* / Maximum depth of market-by-price order book
*
*
* sint32 bookDepth = 4;
* @return The bookDepth.
*/
@java.lang.Override
public int getBookDepth() {
return bookDepth_;
}
public static final int VENDORID_FIELD_NUMBER = 5;
@SuppressWarnings("serial")
private volatile java.lang.Object vendorId_ = "";
/**
*
* / The name of the market data vendor
*
*
* string vendorId = 5;
* @return The vendorId.
*/
@java.lang.Override
public java.lang.String getVendorId() {
java.lang.Object ref = vendorId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
vendorId_ = s;
return s;
}
}
/**
*
* / The name of the market data vendor
*
*
* string vendorId = 5;
* @return The bytes for vendorId.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getVendorIdBytes() {
java.lang.Object ref = vendorId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
vendorId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SYMBOL_FIELD_NUMBER = 6;
@SuppressWarnings("serial")
private volatile java.lang.Object symbol_ = "";
/**
*
* / Human readable market symbol, assigned by the exchange or venue.
* Not necessarily unique as the exchange or vendor could assign the same symbol to different
* instruments, for example if the instruments trade on different exchanges.
*
*
* string symbol = 6;
* @return The symbol.
*/
@java.lang.Override
public java.lang.String getSymbol() {
java.lang.Object ref = symbol_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
symbol_ = s;
return s;
}
}
/**
*
* / Human readable market symbol, assigned by the exchange or venue.
* Not necessarily unique as the exchange or vendor could assign the same symbol to different
* instruments, for example if the instruments trade on different exchanges.
*
*
* string symbol = 6;
* @return The bytes for symbol.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getSymbolBytes() {
java.lang.Object ref = symbol_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
symbol_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int DESCRIPTION_FIELD_NUMBER = 7;
@SuppressWarnings("serial")
private volatile java.lang.Object description_ = "";
/**
*
* / Human readable market description.
*
*
* string description = 7;
* @return The description.
*/
@java.lang.Override
public java.lang.String getDescription() {
java.lang.Object ref = description_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
description_ = s;
return s;
}
}
/**
*
* / Human readable market description.
*
*
* string description = 7;
* @return The bytes for description.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getDescriptionBytes() {
java.lang.Object ref = description_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
description_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CFICODE_FIELD_NUMBER = 8;
@SuppressWarnings("serial")
private volatile java.lang.Object cfiCode_ = "";
/**
*
* / Market CFI code: http://en.wikipedia.org/wiki/ISO_10962
*
*
* string cfiCode = 8;
* @return The cfiCode.
*/
@java.lang.Override
public java.lang.String getCfiCode() {
java.lang.Object ref = cfiCode_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
cfiCode_ = s;
return s;
}
}
/**
*
* / Market CFI code: http://en.wikipedia.org/wiki/ISO_10962
*
*
* string cfiCode = 8;
* @return The bytes for cfiCode.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getCfiCodeBytes() {
java.lang.Object ref = cfiCode_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
cfiCode_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CURRENCYCODE_FIELD_NUMBER = 9;
@SuppressWarnings("serial")
private volatile java.lang.Object currencyCode_ = "";
/**
*
* / Market currency code: http://en.wikipedia.org/wiki/ISO_4217
*
*
* string currencyCode = 9;
* @return The currencyCode.
*/
@java.lang.Override
public java.lang.String getCurrencyCode() {
java.lang.Object ref = currencyCode_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
currencyCode_ = s;
return s;
}
}
/**
*
* / Market currency code: http://en.wikipedia.org/wiki/ISO_4217
*
*
* string currencyCode = 9;
* @return The bytes for currencyCode.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getCurrencyCodeBytes() {
java.lang.Object ref = currencyCode_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
currencyCode_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int EXCHANGECODE_FIELD_NUMBER = 10;
@SuppressWarnings("serial")
private volatile java.lang.Object exchangeCode_ = "";
/**
*
* Market exchange code: http://en.wikipedia.org/wiki/ISO_10383
* For inter-exchange spreads, use the leg MICs separated by a hyphen
*
*
* string exchangeCode = 10;
* @return The exchangeCode.
*/
@java.lang.Override
public java.lang.String getExchangeCode() {
java.lang.Object ref = exchangeCode_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
exchangeCode_ = s;
return s;
}
}
/**
*
* Market exchange code: http://en.wikipedia.org/wiki/ISO_10383
* For inter-exchange spreads, use the leg MICs separated by a hyphen
*
*
* string exchangeCode = 10;
* @return The bytes for exchangeCode.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getExchangeCodeBytes() {
java.lang.Object ref = exchangeCode_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
exchangeCode_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int MINIMUMPRICEINCREMENT_FIELD_NUMBER = 11;
private float minimumPriceIncrement_ = 0F;
/**
*
* / Minimum price increment in market currency.
*
*
* float minimumPriceIncrement = 11;
* @return The minimumPriceIncrement.
*/
@java.lang.Override
public float getMinimumPriceIncrement() {
return minimumPriceIncrement_;
}
public static final int CONTRACTPOINTVALUE_FIELD_NUMBER = 12;
private float contractPointValue_ = 0F;
/**
*
* / Contract point value in market currency.
*
*
* float contractPointValue = 12;
* @return The contractPointValue.
*/
@java.lang.Override
public float getContractPointValue() {
return contractPointValue_;
}
public static final int SCHEDULE_FIELD_NUMBER = 13;
private org.openfeed.InstrumentDefinition.Schedule schedule_;
/**
*
* / Trading schedule for a typical week
*
*
* .org.openfeed.InstrumentDefinition.Schedule schedule = 13;
* @return Whether the schedule field is set.
*/
@java.lang.Override
public boolean hasSchedule() {
return ((bitField0_ & 0x00000001) != 0);
}
/**
*
* / Trading schedule for a typical week
*
*
* .org.openfeed.InstrumentDefinition.Schedule schedule = 13;
* @return The schedule.
*/
@java.lang.Override
public org.openfeed.InstrumentDefinition.Schedule getSchedule() {
return schedule_ == null ? org.openfeed.InstrumentDefinition.Schedule.getDefaultInstance() : schedule_;
}
/**
*
* / Trading schedule for a typical week
*
*
* .org.openfeed.InstrumentDefinition.Schedule schedule = 13;
*/
@java.lang.Override
public org.openfeed.InstrumentDefinition.ScheduleOrBuilder getScheduleOrBuilder() {
return schedule_ == null ? org.openfeed.InstrumentDefinition.Schedule.getDefaultInstance() : schedule_;
}
public static final int CALENDAR_FIELD_NUMBER = 14;
private org.openfeed.InstrumentDefinition.Calendar calendar_;
/**
*
* / Trading calendar (expiration, notice days, holidays?, etc)
*
*
* .org.openfeed.InstrumentDefinition.Calendar calendar = 14;
* @return Whether the calendar field is set.
*/
@java.lang.Override
public boolean hasCalendar() {
return ((bitField0_ & 0x00000002) != 0);
}
/**
*
* / Trading calendar (expiration, notice days, holidays?, etc)
*
*
* .org.openfeed.InstrumentDefinition.Calendar calendar = 14;
* @return The calendar.
*/
@java.lang.Override
public org.openfeed.InstrumentDefinition.Calendar getCalendar() {
return calendar_ == null ? org.openfeed.InstrumentDefinition.Calendar.getDefaultInstance() : calendar_;
}
/**
*
* / Trading calendar (expiration, notice days, holidays?, etc)
*
*
* .org.openfeed.InstrumentDefinition.Calendar calendar = 14;
*/
@java.lang.Override
public org.openfeed.InstrumentDefinition.CalendarOrBuilder getCalendarOrBuilder() {
return calendar_ == null ? org.openfeed.InstrumentDefinition.Calendar.getDefaultInstance() : calendar_;
}
public static final int RECORDCREATETIME_FIELD_NUMBER = 15;
private long recordCreateTime_ = 0L;
/**
*
* / UTC Timestamp of creation, nano seconds since Unix epoch
*
*
* sint64 recordCreateTime = 15;
* @return The recordCreateTime.
*/
@java.lang.Override
public long getRecordCreateTime() {
return recordCreateTime_;
}
public static final int RECORDUPDATETIME_FIELD_NUMBER = 16;
private long recordUpdateTime_ = 0L;
/**
*
* / UTC Timestamp of update, nano seconds since Unix epoch
*
*
* sint64 recordUpdateTime = 16;
* @return The recordUpdateTime.
*/
@java.lang.Override
public long getRecordUpdateTime() {
return recordUpdateTime_;
}
public static final int TIMEZONENAME_FIELD_NUMBER = 17;
@SuppressWarnings("serial")
private volatile java.lang.Object timeZoneName_ = "";
/**
*
* / Market time zone TZ database name.
* Permanent. Can be resolved into timeZoneOffset for given date/time.
* See http://joda-time.sourceforge.net/timezones.html
* See http://en.wikipedia.org/wiki/List_of_tz_database_time_zones
*
*
* string timeZoneName = 17;
* @return The timeZoneName.
*/
@java.lang.Override
public java.lang.String getTimeZoneName() {
java.lang.Object ref = timeZoneName_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
timeZoneName_ = s;
return s;
}
}
/**
*
* / Market time zone TZ database name.
* Permanent. Can be resolved into timeZoneOffset for given date/time.
* See http://joda-time.sourceforge.net/timezones.html
* See http://en.wikipedia.org/wiki/List_of_tz_database_time_zones
*
*
* string timeZoneName = 17;
* @return The bytes for timeZoneName.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getTimeZoneNameBytes() {
java.lang.Object ref = timeZoneName_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
timeZoneName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int INSTRUMENTGROUP_FIELD_NUMBER = 18;
@SuppressWarnings("serial")
private volatile java.lang.Object instrumentGroup_ = "";
/**
*
* / Identifies a logical grouping of instruments. By product, for example.
*
*
* string instrumentGroup = 18;
* @return The instrumentGroup.
*/
@java.lang.Override
public java.lang.String getInstrumentGroup() {
java.lang.Object ref = instrumentGroup_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
instrumentGroup_ = s;
return s;
}
}
/**
*
* / Identifies a logical grouping of instruments. By product, for example.
*
*
* string instrumentGroup = 18;
* @return The bytes for instrumentGroup.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getInstrumentGroupBytes() {
java.lang.Object ref = instrumentGroup_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
instrumentGroup_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SYMBOLEXPIRATION_FIELD_NUMBER = 19;
private org.openfeed.InstrumentDefinition.MaturityDate symbolExpiration_;
/**
*
* / The Date of expiration for futures and options.
*
*
* .org.openfeed.InstrumentDefinition.MaturityDate symbolExpiration = 19;
* @return Whether the symbolExpiration field is set.
*/
@java.lang.Override
public boolean hasSymbolExpiration() {
return ((bitField0_ & 0x00000004) != 0);
}
/**
*
* / The Date of expiration for futures and options.
*
*
* .org.openfeed.InstrumentDefinition.MaturityDate symbolExpiration = 19;
* @return The symbolExpiration.
*/
@java.lang.Override
public org.openfeed.InstrumentDefinition.MaturityDate getSymbolExpiration() {
return symbolExpiration_ == null ? org.openfeed.InstrumentDefinition.MaturityDate.getDefaultInstance() : symbolExpiration_;
}
/**
*
* / The Date of expiration for futures and options.
*
*
* .org.openfeed.InstrumentDefinition.MaturityDate symbolExpiration = 19;
*/
@java.lang.Override
public org.openfeed.InstrumentDefinition.MaturityDateOrBuilder getSymbolExpirationOrBuilder() {
return symbolExpiration_ == null ? org.openfeed.InstrumentDefinition.MaturityDate.getDefaultInstance() : symbolExpiration_;
}
public static final int STATE_FIELD_NUMBER = 20;
private int state_ = 0;
/**
*
* / active: can have market state updates, can have historical data
* passive: can NOT have market state updates, but can have historical data
* normally "active" means newly listed or currently non expired markets
* normally "passive" means expired options, de-listed equities, etc.
*
*
* .org.openfeed.InstrumentDefinition.State state = 20;
* @return The enum numeric value on the wire for state.
*/
@java.lang.Override public int getStateValue() {
return state_;
}
/**
*
* / active: can have market state updates, can have historical data
* passive: can NOT have market state updates, but can have historical data
* normally "active" means newly listed or currently non expired markets
* normally "passive" means expired options, de-listed equities, etc.
*
*
* .org.openfeed.InstrumentDefinition.State state = 20;
* @return The state.
*/
@java.lang.Override public org.openfeed.InstrumentDefinition.State getState() {
org.openfeed.InstrumentDefinition.State result = org.openfeed.InstrumentDefinition.State.forNumber(state_);
return result == null ? org.openfeed.InstrumentDefinition.State.UNRECOGNIZED : result;
}
public static final int CHANNEL_FIELD_NUMBER = 21;
private int channel_ = 0;
/**
*
* / The channel that updates for this instrument will appear on.
*
*
* sint32 channel = 21;
* @return The channel.
*/
@java.lang.Override
public int getChannel() {
return channel_;
}
public static final int UNDERLYINGMARKETID_FIELD_NUMBER = 22;
private long underlyingMarketId_ = 0L;
/**
*
* / The marketId of the underlying asset.
* Used by Futures and Options when the underlying instrument is defined by the vendor
*
*
* sint64 underlyingMarketId = 22;
* @return The underlyingMarketId.
*/
@java.lang.Override
public long getUnderlyingMarketId() {
return underlyingMarketId_;
}
public static final int PRICEFORMAT_FIELD_NUMBER = 23;
private org.openfeed.InstrumentDefinition.PriceFormat priceFormat_;
/**
*
* / Display format
*
*
* .org.openfeed.InstrumentDefinition.PriceFormat priceFormat = 23;
* @return Whether the priceFormat field is set.
*/
@java.lang.Override
public boolean hasPriceFormat() {
return ((bitField0_ & 0x00000008) != 0);
}
/**
*
* / Display format
*
*
* .org.openfeed.InstrumentDefinition.PriceFormat priceFormat = 23;
* @return The priceFormat.
*/
@java.lang.Override
public org.openfeed.InstrumentDefinition.PriceFormat getPriceFormat() {
return priceFormat_ == null ? org.openfeed.InstrumentDefinition.PriceFormat.getDefaultInstance() : priceFormat_;
}
/**
*
* / Display format
*
*
* .org.openfeed.InstrumentDefinition.PriceFormat priceFormat = 23;
*/
@java.lang.Override
public org.openfeed.InstrumentDefinition.PriceFormatOrBuilder getPriceFormatOrBuilder() {
return priceFormat_ == null ? org.openfeed.InstrumentDefinition.PriceFormat.getDefaultInstance() : priceFormat_;
}
public static final int OPTIONSTRIKEPRICEFORMAT_FIELD_NUMBER = 24;
private org.openfeed.InstrumentDefinition.PriceFormat optionStrikePriceFormat_;
/**
*
* / Strike price display format
*
*
* .org.openfeed.InstrumentDefinition.PriceFormat optionStrikePriceFormat = 24;
* @return Whether the optionStrikePriceFormat field is set.
*/
@java.lang.Override
public boolean hasOptionStrikePriceFormat() {
return ((bitField0_ & 0x00000010) != 0);
}
/**
*
* / Strike price display format
*
*
* .org.openfeed.InstrumentDefinition.PriceFormat optionStrikePriceFormat = 24;
* @return The optionStrikePriceFormat.
*/
@java.lang.Override
public org.openfeed.InstrumentDefinition.PriceFormat getOptionStrikePriceFormat() {
return optionStrikePriceFormat_ == null ? org.openfeed.InstrumentDefinition.PriceFormat.getDefaultInstance() : optionStrikePriceFormat_;
}
/**
*
* / Strike price display format
*
*
* .org.openfeed.InstrumentDefinition.PriceFormat optionStrikePriceFormat = 24;
*/
@java.lang.Override
public org.openfeed.InstrumentDefinition.PriceFormatOrBuilder getOptionStrikePriceFormatOrBuilder() {
return optionStrikePriceFormat_ == null ? org.openfeed.InstrumentDefinition.PriceFormat.getDefaultInstance() : optionStrikePriceFormat_;
}
public static final int PRICEDENOMINATOR_FIELD_NUMBER = 28;
private int priceDenominator_ = 0;
/**
*
* / Divide prices by this value to get real price values
*
*
* sint32 priceDenominator = 28;
* @return The priceDenominator.
*/
@java.lang.Override
public int getPriceDenominator() {
return priceDenominator_;
}
public static final int QUANTITYDENOMINATOR_FIELD_NUMBER = 29;
private int quantityDenominator_ = 0;
/**
*
* / Divide trade quantities by this value to get real quantities
*
*
* sint32 quantityDenominator = 29;
* @return The quantityDenominator.
*/
@java.lang.Override
public int getQuantityDenominator() {
return quantityDenominator_;
}
public static final int ISTRADABLE_FIELD_NUMBER = 30;
private boolean isTradable_ = false;
/**
*
* / true if this is a tradable instrument
*
*
* bool isTradable = 30;
* @return The isTradable.
*/
@java.lang.Override
public boolean getIsTradable() {
return isTradable_;
}
public static final int TRANSACTIONTIME_FIELD_NUMBER = 50;
private long transactionTime_ = 0L;
/**
*
* / UTC timestamp of transaction, nano seconds since Unix epoch
*
*
* sint64 transactionTime = 50;
* @return The transactionTime.
*/
@java.lang.Override
public long getTransactionTime() {
return transactionTime_;
}
public static final int AUXILIARYDATA_FIELD_NUMBER = 99;
private com.google.protobuf.ByteString auxiliaryData_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* / For internal use only. Ignore
*
*
* bytes auxiliaryData = 99;
* @return The auxiliaryData.
*/
@java.lang.Override
public com.google.protobuf.ByteString getAuxiliaryData() {
return auxiliaryData_;
}
public static final int SYMBOLS_FIELD_NUMBER = 100;
@SuppressWarnings("serial")
private java.util.List symbols_;
/**
*
* / List of alternate symbols for this instrument. A single instrument
* may be provided by many different market data vendors, each with
* their own unique symbology. Allows this instrument to be tagged
* with as many vendor symbols as necessary.
*
*
* repeated .org.openfeed.InstrumentDefinition.Symbol symbols = 100;
*/
@java.lang.Override
public java.util.List getSymbolsList() {
return symbols_;
}
/**
*
* / List of alternate symbols for this instrument. A single instrument
* may be provided by many different market data vendors, each with
* their own unique symbology. Allows this instrument to be tagged
* with as many vendor symbols as necessary.
*
*
* repeated .org.openfeed.InstrumentDefinition.Symbol symbols = 100;
*/
@java.lang.Override
public java.util.List extends org.openfeed.InstrumentDefinition.SymbolOrBuilder>
getSymbolsOrBuilderList() {
return symbols_;
}
/**
*
* / List of alternate symbols for this instrument. A single instrument
* may be provided by many different market data vendors, each with
* their own unique symbology. Allows this instrument to be tagged
* with as many vendor symbols as necessary.
*
*
* repeated .org.openfeed.InstrumentDefinition.Symbol symbols = 100;
*/
@java.lang.Override
public int getSymbolsCount() {
return symbols_.size();
}
/**
*
* / List of alternate symbols for this instrument. A single instrument
* may be provided by many different market data vendors, each with
* their own unique symbology. Allows this instrument to be tagged
* with as many vendor symbols as necessary.
*
*
* repeated .org.openfeed.InstrumentDefinition.Symbol symbols = 100;
*/
@java.lang.Override
public org.openfeed.InstrumentDefinition.Symbol getSymbols(int index) {
return symbols_.get(index);
}
/**
*
* / List of alternate symbols for this instrument. A single instrument
* may be provided by many different market data vendors, each with
* their own unique symbology. Allows this instrument to be tagged
* with as many vendor symbols as necessary.
*
*
* repeated .org.openfeed.InstrumentDefinition.Symbol symbols = 100;
*/
@java.lang.Override
public org.openfeed.InstrumentDefinition.SymbolOrBuilder getSymbolsOrBuilder(
int index) {
return symbols_.get(index);
}
public static final int OPTIONSTRIKE_FIELD_NUMBER = 200;
private long optionStrike_ = 0L;
/**
*
* / Option strike price in market currency. Multiply by
* / factorOptionsStrike to get actual strike
*
*
* sint64 optionStrike = 200;
* @return The optionStrike.
*/
@java.lang.Override
public long getOptionStrike() {
return optionStrike_;
}
public static final int OPTIONTYPE_FIELD_NUMBER = 202;
private int optionType_ = 0;
/**
*
* / Option type: call vs put.
*
*
* .org.openfeed.InstrumentDefinition.OptionType optionType = 202;
* @return The enum numeric value on the wire for optionType.
*/
@java.lang.Override public int getOptionTypeValue() {
return optionType_;
}
/**
*
* / Option type: call vs put.
*
*
* .org.openfeed.InstrumentDefinition.OptionType optionType = 202;
* @return The optionType.
*/
@java.lang.Override public org.openfeed.InstrumentDefinition.OptionType getOptionType() {
org.openfeed.InstrumentDefinition.OptionType result = org.openfeed.InstrumentDefinition.OptionType.forNumber(optionType_);
return result == null ? org.openfeed.InstrumentDefinition.OptionType.UNRECOGNIZED : result;
}
public static final int OPTIONSTYLE_FIELD_NUMBER = 203;
private int optionStyle_ = 0;
/**
*
* / Option style : American vs European.
*
*
* .org.openfeed.InstrumentDefinition.OptionStyle optionStyle = 203;
* @return The enum numeric value on the wire for optionStyle.
*/
@java.lang.Override public int getOptionStyleValue() {
return optionStyle_;
}
/**
*
* / Option style : American vs European.
*
*
* .org.openfeed.InstrumentDefinition.OptionStyle optionStyle = 203;
* @return The optionStyle.
*/
@java.lang.Override public org.openfeed.InstrumentDefinition.OptionStyle getOptionStyle() {
org.openfeed.InstrumentDefinition.OptionStyle result = org.openfeed.InstrumentDefinition.OptionStyle.forNumber(optionStyle_);
return result == null ? org.openfeed.InstrumentDefinition.OptionStyle.UNRECOGNIZED : result;
}
public static final int OPTIONSTRIKEDENOMINATOR_FIELD_NUMBER = 204;
private int optionStrikeDenominator_ = 0;
/**
*
* / Divide optionStrike by this value to get real strike price
*
*
* sint32 optionStrikeDenominator = 204;
* @return The optionStrikeDenominator.
*/
@java.lang.Override
public int getOptionStrikeDenominator() {
return optionStrikeDenominator_;
}
public static final int SPREADCODE_FIELD_NUMBER = 210;
@SuppressWarnings("serial")
private volatile java.lang.Object spreadCode_ = "";
/**
*
* / Spread type, can be vendor specific
*
*
* string spreadCode = 210;
* @return The spreadCode.
*/
@java.lang.Override
public java.lang.String getSpreadCode() {
java.lang.Object ref = spreadCode_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
spreadCode_ = s;
return s;
}
}
/**
*
* / Spread type, can be vendor specific
*
*
* string spreadCode = 210;
* @return The bytes for spreadCode.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getSpreadCodeBytes() {
java.lang.Object ref = spreadCode_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
spreadCode_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SPREADLEG_FIELD_NUMBER = 211;
@SuppressWarnings("serial")
private java.util.List spreadLeg_;
/**
*
* / Ordered list of underlying legs in a spread.
*
*
* repeated .org.openfeed.InstrumentDefinition.SpreadLeg spreadLeg = 211;
*/
@java.lang.Override
public java.util.List getSpreadLegList() {
return spreadLeg_;
}
/**
*
* / Ordered list of underlying legs in a spread.
*
*
* repeated .org.openfeed.InstrumentDefinition.SpreadLeg spreadLeg = 211;
*/
@java.lang.Override
public java.util.List extends org.openfeed.InstrumentDefinition.SpreadLegOrBuilder>
getSpreadLegOrBuilderList() {
return spreadLeg_;
}
/**
*
* / Ordered list of underlying legs in a spread.
*
*
* repeated .org.openfeed.InstrumentDefinition.SpreadLeg spreadLeg = 211;
*/
@java.lang.Override
public int getSpreadLegCount() {
return spreadLeg_.size();
}
/**
*
* / Ordered list of underlying legs in a spread.
*
*
* repeated .org.openfeed.InstrumentDefinition.SpreadLeg spreadLeg = 211;
*/
@java.lang.Override
public org.openfeed.InstrumentDefinition.SpreadLeg getSpreadLeg(int index) {
return spreadLeg_.get(index);
}
/**
*
* / Ordered list of underlying legs in a spread.
*
*
* repeated .org.openfeed.InstrumentDefinition.SpreadLeg spreadLeg = 211;
*/
@java.lang.Override
public org.openfeed.InstrumentDefinition.SpreadLegOrBuilder getSpreadLegOrBuilder(
int index) {
return spreadLeg_.get(index);
}
public static final int USERDEFINEDSPREAD_FIELD_NUMBER = 212;
private boolean userDefinedSpread_ = false;
/**
*
* / true if user defined spread
*
*
* bool userDefinedSpread = 212;
* @return The userDefinedSpread.
*/
@java.lang.Override
public boolean getUserDefinedSpread() {
return userDefinedSpread_;
}
public static final int MARKETTIER_FIELD_NUMBER = 213;
@SuppressWarnings("serial")
private volatile java.lang.Object marketTier_ = "";
/**
*
* / Listing market classification
*
*
* string marketTier = 213;
* @return The marketTier.
*/
@java.lang.Override
public java.lang.String getMarketTier() {
java.lang.Object ref = marketTier_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
marketTier_ = s;
return s;
}
}
/**
*
* / Listing market classification
*
*
* string marketTier = 213;
* @return The bytes for marketTier.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getMarketTierBytes() {
java.lang.Object ref = marketTier_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
marketTier_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int FINANCIALSTATUSINDICATOR_FIELD_NUMBER = 214;
@SuppressWarnings("serial")
private volatile java.lang.Object financialStatusIndicator_ = "";
/**
*
* / Current financial status of the issuer
*
*
* string financialStatusIndicator = 214;
* @return The financialStatusIndicator.
*/
@java.lang.Override
public java.lang.String getFinancialStatusIndicator() {
java.lang.Object ref = financialStatusIndicator_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
financialStatusIndicator_ = s;
return s;
}
}
/**
*
* / Current financial status of the issuer
*
*
* string financialStatusIndicator = 214;
* @return The bytes for financialStatusIndicator.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getFinancialStatusIndicatorBytes() {
java.lang.Object ref = financialStatusIndicator_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
financialStatusIndicator_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int ISIN_FIELD_NUMBER = 215;
@SuppressWarnings("serial")
private volatile java.lang.Object isin_ = "";
/**
*
* / ISIN: https://en.wikipedia.org/wiki/International_Securities_Identification_Number
*
*
* string isin = 215;
* @return The isin.
*/
@java.lang.Override
public java.lang.String getIsin() {
java.lang.Object ref = isin_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
isin_ = s;
return s;
}
}
/**
*
* / ISIN: https://en.wikipedia.org/wiki/International_Securities_Identification_Number
*
*
* string isin = 215;
* @return The bytes for isin.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getIsinBytes() {
java.lang.Object ref = isin_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
isin_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CURRENCYPAIR_FIELD_NUMBER = 216;
private org.openfeed.InstrumentDefinition.CurrencyPair currencyPair_;
/**
*
* / Break out of currency pair
*
*
* .org.openfeed.InstrumentDefinition.CurrencyPair currencyPair = 216;
* @return Whether the currencyPair field is set.
*/
@java.lang.Override
public boolean hasCurrencyPair() {
return ((bitField0_ & 0x00000020) != 0);
}
/**
*
* / Break out of currency pair
*
*
* .org.openfeed.InstrumentDefinition.CurrencyPair currencyPair = 216;
* @return The currencyPair.
*/
@java.lang.Override
public org.openfeed.InstrumentDefinition.CurrencyPair getCurrencyPair() {
return currencyPair_ == null ? org.openfeed.InstrumentDefinition.CurrencyPair.getDefaultInstance() : currencyPair_;
}
/**
*
* / Break out of currency pair
*
*
* .org.openfeed.InstrumentDefinition.CurrencyPair currencyPair = 216;
*/
@java.lang.Override
public org.openfeed.InstrumentDefinition.CurrencyPairOrBuilder getCurrencyPairOrBuilder() {
return currencyPair_ == null ? org.openfeed.InstrumentDefinition.CurrencyPair.getDefaultInstance() : currencyPair_;
}
public static final int EXCHANGESENDSVOLUME_FIELD_NUMBER = 217;
private boolean exchangeSendsVolume_ = false;
/**
*
* / true if exchange sends volume.
*
*
* bool exchangeSendsVolume = 217;
* @return The exchangeSendsVolume.
*/
@java.lang.Override
public boolean getExchangeSendsVolume() {
return exchangeSendsVolume_;
}
public static final int EXCHANGESENDSHIGH_FIELD_NUMBER = 218;
private boolean exchangeSendsHigh_ = false;
/**
*
* / true if exchange sends high.
*
*
* bool exchangeSendsHigh = 218;
* @return The exchangeSendsHigh.
*/
@java.lang.Override
public boolean getExchangeSendsHigh() {
return exchangeSendsHigh_;
}
public static final int EXCHANGESENDSLOW_FIELD_NUMBER = 219;
private boolean exchangeSendsLow_ = false;
/**
*
* / true if exchange sends low.
*
*
* bool exchangeSendsLow = 219;
* @return The exchangeSendsLow.
*/
@java.lang.Override
public boolean getExchangeSendsLow() {
return exchangeSendsLow_;
}
public static final int EXCHANGESENDSOPEN_FIELD_NUMBER = 220;
private boolean exchangeSendsOpen_ = false;
/**
*
* / true if exchange sends open.
*
*
* bool exchangeSendsOpen = 220;
* @return The exchangeSendsOpen.
*/
@java.lang.Override
public boolean getExchangeSendsOpen() {
return exchangeSendsOpen_;
}
public static final int CONSOLIDATEDFEEDINSTRUMENT_FIELD_NUMBER = 221;
private boolean consolidatedFeedInstrument_ = false;
/**
*
* / true if this instrument represents consolidated NBBO.
*
*
* bool consolidatedFeedInstrument = 221;
* @return The consolidatedFeedInstrument.
*/
@java.lang.Override
public boolean getConsolidatedFeedInstrument() {
return consolidatedFeedInstrument_;
}
public static final int OPENOUTCRYINSTRUMENT_FIELD_NUMBER = 222;
private boolean openOutcryInstrument_ = false;
/**
*
* / true if this instrument represents Pit symbol.
*
*
* bool openOutcryInstrument = 222;
* @return The openOutcryInstrument.
*/
@java.lang.Override
public boolean getOpenOutcryInstrument() {
return openOutcryInstrument_;
}
public static final int SYNTHETICAMERICANOPTIONINSTRUMENT_FIELD_NUMBER = 223;
private boolean syntheticAmericanOptionInstrument_ = false;
/**
*
* / true if this instrument generated FX option.
*
*
* bool syntheticAmericanOptionInstrument = 223;
* @return The syntheticAmericanOptionInstrument.
*/
@java.lang.Override
public boolean getSyntheticAmericanOptionInstrument() {
return syntheticAmericanOptionInstrument_;
}
public static final int BARCHARTEXCHANGECODE_FIELD_NUMBER = 224;
@SuppressWarnings("serial")
private volatile java.lang.Object barchartExchangeCode_ = "";
/**
*
* /
*
*
* string barchartExchangeCode = 224;
* @return The barchartExchangeCode.
*/
@java.lang.Override
public java.lang.String getBarchartExchangeCode() {
java.lang.Object ref = barchartExchangeCode_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
barchartExchangeCode_ = s;
return s;
}
}
/**
*
* /
*
*
* string barchartExchangeCode = 224;
* @return The bytes for barchartExchangeCode.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getBarchartExchangeCodeBytes() {
java.lang.Object ref = barchartExchangeCode_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
barchartExchangeCode_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int BARCHARTBASECODE_FIELD_NUMBER = 225;
@SuppressWarnings("serial")
private volatile java.lang.Object barchartBaseCode_ = "";
/**
*
* /
*
*
* string barchartBaseCode = 225;
* @return The barchartBaseCode.
*/
@java.lang.Override
public java.lang.String getBarchartBaseCode() {
java.lang.Object ref = barchartBaseCode_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
barchartBaseCode_ = s;
return s;
}
}
/**
*
* /
*
*
* string barchartBaseCode = 225;
* @return The bytes for barchartBaseCode.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getBarchartBaseCodeBytes() {
java.lang.Object ref = barchartBaseCode_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
barchartBaseCode_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int VOLUMEDENOMINATOR_FIELD_NUMBER = 226;
private int volumeDenominator_ = 0;
/**
*
* /
*
*
* sint32 volumeDenominator = 226;
* @return The volumeDenominator.
*/
@java.lang.Override
public int getVolumeDenominator() {
return volumeDenominator_;
}
public static final int BIDOFFERQUANTITYDENOMINATOR_FIELD_NUMBER = 227;
private int bidOfferQuantityDenominator_ = 0;
/**
*
* /
*
*
* sint32 bidOfferQuantityDenominator = 227;
* @return The bidOfferQuantityDenominator.
*/
@java.lang.Override
public int getBidOfferQuantityDenominator() {
return bidOfferQuantityDenominator_;
}
public static final int PRIMARYLISTINGMARKETPARTICIPANTID_FIELD_NUMBER = 228;
@SuppressWarnings("serial")
private volatile java.lang.Object primaryListingMarketParticipantId_ = "";
/**
*
* /
*
*
* string primaryListingMarketParticipantId = 228;
* @return The primaryListingMarketParticipantId.
*/
@java.lang.Override
public java.lang.String getPrimaryListingMarketParticipantId() {
java.lang.Object ref = primaryListingMarketParticipantId_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
primaryListingMarketParticipantId_ = s;
return s;
}
}
/**
*
* /
*
*
* string primaryListingMarketParticipantId = 228;
* @return The bytes for primaryListingMarketParticipantId.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getPrimaryListingMarketParticipantIdBytes() {
java.lang.Object ref = primaryListingMarketParticipantId_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
primaryListingMarketParticipantId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SUBSCRIPTIONSYMBOL_FIELD_NUMBER = 229;
@SuppressWarnings("serial")
private volatile java.lang.Object subscriptionSymbol_ = "";
/**
*
* /
*
*
* string subscriptionSymbol = 229;
* @return The subscriptionSymbol.
*/
@java.lang.Override
public java.lang.String getSubscriptionSymbol() {
java.lang.Object ref = subscriptionSymbol_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
subscriptionSymbol_ = s;
return s;
}
}
/**
*
* /
*
*
* string subscriptionSymbol = 229;
* @return The bytes for subscriptionSymbol.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getSubscriptionSymbolBytes() {
java.lang.Object ref = subscriptionSymbol_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
subscriptionSymbol_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CONTRACTMATURITY_FIELD_NUMBER = 230;
private org.openfeed.InstrumentDefinition.MaturityDate contractMaturity_;
/**
*
* / The Month/ Day of expiration for futures and options. Corresponds to the expiration month.
*
*
* .org.openfeed.InstrumentDefinition.MaturityDate contractMaturity = 230;
* @return Whether the contractMaturity field is set.
*/
@java.lang.Override
public boolean hasContractMaturity() {
return ((bitField0_ & 0x00000040) != 0);
}
/**
*
* / The Month/ Day of expiration for futures and options. Corresponds to the expiration month.
*
*
* .org.openfeed.InstrumentDefinition.MaturityDate contractMaturity = 230;
* @return The contractMaturity.
*/
@java.lang.Override
public org.openfeed.InstrumentDefinition.MaturityDate getContractMaturity() {
return contractMaturity_ == null ? org.openfeed.InstrumentDefinition.MaturityDate.getDefaultInstance() : contractMaturity_;
}
/**
*
* / The Month/ Day of expiration for futures and options. Corresponds to the expiration month.
*
*
* .org.openfeed.InstrumentDefinition.MaturityDate contractMaturity = 230;
*/
@java.lang.Override
public org.openfeed.InstrumentDefinition.MaturityDateOrBuilder getContractMaturityOrBuilder() {
return contractMaturity_ == null ? org.openfeed.InstrumentDefinition.MaturityDate.getDefaultInstance() : contractMaturity_;
}
public static final int UNDERLYING_FIELD_NUMBER = 231;
@SuppressWarnings("serial")
private volatile java.lang.Object underlying_ = "";
/**
*
* / Barchart Underlying Symbol
*
*
* string underlying = 231;
* @return The underlying.
*/
@java.lang.Override
public java.lang.String getUnderlying() {
java.lang.Object ref = underlying_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
underlying_ = s;
return s;
}
}
/**
*
* / Barchart Underlying Symbol
*
*
* string underlying = 231;
* @return The bytes for underlying.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getUnderlyingBytes() {
java.lang.Object ref = underlying_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
underlying_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int COMMODITY_FIELD_NUMBER = 232;
@SuppressWarnings("serial")
private volatile java.lang.Object commodity_ = "";
/**
* string commodity = 232;
* @return The commodity.
*/
@java.lang.Override
public java.lang.String getCommodity() {
java.lang.Object ref = commodity_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
commodity_ = s;
return s;
}
}
/**
* string commodity = 232;
* @return The bytes for commodity.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getCommodityBytes() {
java.lang.Object ref = commodity_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
commodity_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int EXCHANGEID_FIELD_NUMBER = 233;
private int exchangeId_ = 0;
/**
*
* / Barchart Exchange Id
*
*
* sint32 exchangeId = 233;
* @return The exchangeId.
*/
@java.lang.Override
public int getExchangeId() {
return exchangeId_;
}
public static final int PRICESCALINGEXPONENT_FIELD_NUMBER = 234;
private int priceScalingExponent_ = 0;
/**
*
* / Barchart Price Scaling Exponent
*
*
* sint32 priceScalingExponent = 234;
* @return The priceScalingExponent.
*/
@java.lang.Override
public int getPriceScalingExponent() {
return priceScalingExponent_;
}
public static final int UNDERLYINGOPENFEEDMARKETID_FIELD_NUMBER = 235;
private long underlyingOpenfeedMarketId_ = 0L;
/**
*
* / The Openfeed marketId of the underlying asset.
*
*
* sint64 underlyingOpenfeedMarketId = 235;
* @return The underlyingOpenfeedMarketId.
*/
@java.lang.Override
public long getUnderlyingOpenfeedMarketId() {
return underlyingOpenfeedMarketId_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (marketId_ != 0L) {
output.writeSInt64(1, marketId_);
}
if (instrumentType_ != org.openfeed.InstrumentDefinition.InstrumentType.UNKNOWN_INSTRUMENT_TYPE.getNumber()) {
output.writeEnum(2, instrumentType_);
}
if (getSupportBookTypesList().size() > 0) {
output.writeUInt32NoTag(26);
output.writeUInt32NoTag(supportBookTypesMemoizedSerializedSize);
}
for (int i = 0; i < supportBookTypes_.size(); i++) {
output.writeEnumNoTag(supportBookTypes_.getInt(i));
}
if (bookDepth_ != 0) {
output.writeSInt32(4, bookDepth_);
}
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(vendorId_)) {
com.google.protobuf.GeneratedMessage.writeString(output, 5, vendorId_);
}
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(symbol_)) {
com.google.protobuf.GeneratedMessage.writeString(output, 6, symbol_);
}
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(description_)) {
com.google.protobuf.GeneratedMessage.writeString(output, 7, description_);
}
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(cfiCode_)) {
com.google.protobuf.GeneratedMessage.writeString(output, 8, cfiCode_);
}
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(currencyCode_)) {
com.google.protobuf.GeneratedMessage.writeString(output, 9, currencyCode_);
}
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(exchangeCode_)) {
com.google.protobuf.GeneratedMessage.writeString(output, 10, exchangeCode_);
}
if (java.lang.Float.floatToRawIntBits(minimumPriceIncrement_) != 0) {
output.writeFloat(11, minimumPriceIncrement_);
}
if (java.lang.Float.floatToRawIntBits(contractPointValue_) != 0) {
output.writeFloat(12, contractPointValue_);
}
if (((bitField0_ & 0x00000001) != 0)) {
output.writeMessage(13, getSchedule());
}
if (((bitField0_ & 0x00000002) != 0)) {
output.writeMessage(14, getCalendar());
}
if (recordCreateTime_ != 0L) {
output.writeSInt64(15, recordCreateTime_);
}
if (recordUpdateTime_ != 0L) {
output.writeSInt64(16, recordUpdateTime_);
}
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(timeZoneName_)) {
com.google.protobuf.GeneratedMessage.writeString(output, 17, timeZoneName_);
}
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(instrumentGroup_)) {
com.google.protobuf.GeneratedMessage.writeString(output, 18, instrumentGroup_);
}
if (((bitField0_ & 0x00000004) != 0)) {
output.writeMessage(19, getSymbolExpiration());
}
if (state_ != org.openfeed.InstrumentDefinition.State.UNKNOWN_STATE.getNumber()) {
output.writeEnum(20, state_);
}
if (channel_ != 0) {
output.writeSInt32(21, channel_);
}
if (underlyingMarketId_ != 0L) {
output.writeSInt64(22, underlyingMarketId_);
}
if (((bitField0_ & 0x00000008) != 0)) {
output.writeMessage(23, getPriceFormat());
}
if (((bitField0_ & 0x00000010) != 0)) {
output.writeMessage(24, getOptionStrikePriceFormat());
}
if (priceDenominator_ != 0) {
output.writeSInt32(28, priceDenominator_);
}
if (quantityDenominator_ != 0) {
output.writeSInt32(29, quantityDenominator_);
}
if (isTradable_ != false) {
output.writeBool(30, isTradable_);
}
if (transactionTime_ != 0L) {
output.writeSInt64(50, transactionTime_);
}
if (!auxiliaryData_.isEmpty()) {
output.writeBytes(99, auxiliaryData_);
}
for (int i = 0; i < symbols_.size(); i++) {
output.writeMessage(100, symbols_.get(i));
}
if (optionStrike_ != 0L) {
output.writeSInt64(200, optionStrike_);
}
if (optionType_ != org.openfeed.InstrumentDefinition.OptionType.UNKNOWN_OPTION_TYPE.getNumber()) {
output.writeEnum(202, optionType_);
}
if (optionStyle_ != org.openfeed.InstrumentDefinition.OptionStyle.UNKNOWN_OPTIONS_STYLE.getNumber()) {
output.writeEnum(203, optionStyle_);
}
if (optionStrikeDenominator_ != 0) {
output.writeSInt32(204, optionStrikeDenominator_);
}
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(spreadCode_)) {
com.google.protobuf.GeneratedMessage.writeString(output, 210, spreadCode_);
}
for (int i = 0; i < spreadLeg_.size(); i++) {
output.writeMessage(211, spreadLeg_.get(i));
}
if (userDefinedSpread_ != false) {
output.writeBool(212, userDefinedSpread_);
}
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(marketTier_)) {
com.google.protobuf.GeneratedMessage.writeString(output, 213, marketTier_);
}
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(financialStatusIndicator_)) {
com.google.protobuf.GeneratedMessage.writeString(output, 214, financialStatusIndicator_);
}
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(isin_)) {
com.google.protobuf.GeneratedMessage.writeString(output, 215, isin_);
}
if (((bitField0_ & 0x00000020) != 0)) {
output.writeMessage(216, getCurrencyPair());
}
if (exchangeSendsVolume_ != false) {
output.writeBool(217, exchangeSendsVolume_);
}
if (exchangeSendsHigh_ != false) {
output.writeBool(218, exchangeSendsHigh_);
}
if (exchangeSendsLow_ != false) {
output.writeBool(219, exchangeSendsLow_);
}
if (exchangeSendsOpen_ != false) {
output.writeBool(220, exchangeSendsOpen_);
}
if (consolidatedFeedInstrument_ != false) {
output.writeBool(221, consolidatedFeedInstrument_);
}
if (openOutcryInstrument_ != false) {
output.writeBool(222, openOutcryInstrument_);
}
if (syntheticAmericanOptionInstrument_ != false) {
output.writeBool(223, syntheticAmericanOptionInstrument_);
}
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(barchartExchangeCode_)) {
com.google.protobuf.GeneratedMessage.writeString(output, 224, barchartExchangeCode_);
}
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(barchartBaseCode_)) {
com.google.protobuf.GeneratedMessage.writeString(output, 225, barchartBaseCode_);
}
if (volumeDenominator_ != 0) {
output.writeSInt32(226, volumeDenominator_);
}
if (bidOfferQuantityDenominator_ != 0) {
output.writeSInt32(227, bidOfferQuantityDenominator_);
}
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(primaryListingMarketParticipantId_)) {
com.google.protobuf.GeneratedMessage.writeString(output, 228, primaryListingMarketParticipantId_);
}
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(subscriptionSymbol_)) {
com.google.protobuf.GeneratedMessage.writeString(output, 229, subscriptionSymbol_);
}
if (((bitField0_ & 0x00000040) != 0)) {
output.writeMessage(230, getContractMaturity());
}
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(underlying_)) {
com.google.protobuf.GeneratedMessage.writeString(output, 231, underlying_);
}
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(commodity_)) {
com.google.protobuf.GeneratedMessage.writeString(output, 232, commodity_);
}
if (exchangeId_ != 0) {
output.writeSInt32(233, exchangeId_);
}
if (priceScalingExponent_ != 0) {
output.writeSInt32(234, priceScalingExponent_);
}
if (underlyingOpenfeedMarketId_ != 0L) {
output.writeSInt64(235, underlyingOpenfeedMarketId_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (marketId_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeSInt64Size(1, marketId_);
}
if (instrumentType_ != org.openfeed.InstrumentDefinition.InstrumentType.UNKNOWN_INSTRUMENT_TYPE.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(2, instrumentType_);
}
{
int dataSize = 0;
for (int i = 0; i < supportBookTypes_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeEnumSizeNoTag(supportBookTypes_.getInt(i));
}
size += dataSize;
if (!getSupportBookTypesList().isEmpty()) { size += 1;
size += com.google.protobuf.CodedOutputStream
.computeUInt32SizeNoTag(dataSize);
}supportBookTypesMemoizedSerializedSize = dataSize;
}
if (bookDepth_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeSInt32Size(4, bookDepth_);
}
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(vendorId_)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(5, vendorId_);
}
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(symbol_)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(6, symbol_);
}
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(description_)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(7, description_);
}
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(cfiCode_)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(8, cfiCode_);
}
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(currencyCode_)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(9, currencyCode_);
}
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(exchangeCode_)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(10, exchangeCode_);
}
if (java.lang.Float.floatToRawIntBits(minimumPriceIncrement_) != 0) {
size += com.google.protobuf.CodedOutputStream
.computeFloatSize(11, minimumPriceIncrement_);
}
if (java.lang.Float.floatToRawIntBits(contractPointValue_) != 0) {
size += com.google.protobuf.CodedOutputStream
.computeFloatSize(12, contractPointValue_);
}
if (((bitField0_ & 0x00000001) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(13, getSchedule());
}
if (((bitField0_ & 0x00000002) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(14, getCalendar());
}
if (recordCreateTime_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeSInt64Size(15, recordCreateTime_);
}
if (recordUpdateTime_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeSInt64Size(16, recordUpdateTime_);
}
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(timeZoneName_)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(17, timeZoneName_);
}
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(instrumentGroup_)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(18, instrumentGroup_);
}
if (((bitField0_ & 0x00000004) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(19, getSymbolExpiration());
}
if (state_ != org.openfeed.InstrumentDefinition.State.UNKNOWN_STATE.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(20, state_);
}
if (channel_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeSInt32Size(21, channel_);
}
if (underlyingMarketId_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeSInt64Size(22, underlyingMarketId_);
}
if (((bitField0_ & 0x00000008) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(23, getPriceFormat());
}
if (((bitField0_ & 0x00000010) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(24, getOptionStrikePriceFormat());
}
if (priceDenominator_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeSInt32Size(28, priceDenominator_);
}
if (quantityDenominator_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeSInt32Size(29, quantityDenominator_);
}
if (isTradable_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(30, isTradable_);
}
if (transactionTime_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeSInt64Size(50, transactionTime_);
}
if (!auxiliaryData_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(99, auxiliaryData_);
}
for (int i = 0; i < symbols_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(100, symbols_.get(i));
}
if (optionStrike_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeSInt64Size(200, optionStrike_);
}
if (optionType_ != org.openfeed.InstrumentDefinition.OptionType.UNKNOWN_OPTION_TYPE.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(202, optionType_);
}
if (optionStyle_ != org.openfeed.InstrumentDefinition.OptionStyle.UNKNOWN_OPTIONS_STYLE.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(203, optionStyle_);
}
if (optionStrikeDenominator_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeSInt32Size(204, optionStrikeDenominator_);
}
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(spreadCode_)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(210, spreadCode_);
}
for (int i = 0; i < spreadLeg_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(211, spreadLeg_.get(i));
}
if (userDefinedSpread_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(212, userDefinedSpread_);
}
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(marketTier_)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(213, marketTier_);
}
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(financialStatusIndicator_)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(214, financialStatusIndicator_);
}
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(isin_)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(215, isin_);
}
if (((bitField0_ & 0x00000020) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(216, getCurrencyPair());
}
if (exchangeSendsVolume_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(217, exchangeSendsVolume_);
}
if (exchangeSendsHigh_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(218, exchangeSendsHigh_);
}
if (exchangeSendsLow_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(219, exchangeSendsLow_);
}
if (exchangeSendsOpen_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(220, exchangeSendsOpen_);
}
if (consolidatedFeedInstrument_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(221, consolidatedFeedInstrument_);
}
if (openOutcryInstrument_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(222, openOutcryInstrument_);
}
if (syntheticAmericanOptionInstrument_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(223, syntheticAmericanOptionInstrument_);
}
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(barchartExchangeCode_)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(224, barchartExchangeCode_);
}
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(barchartBaseCode_)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(225, barchartBaseCode_);
}
if (volumeDenominator_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeSInt32Size(226, volumeDenominator_);
}
if (bidOfferQuantityDenominator_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeSInt32Size(227, bidOfferQuantityDenominator_);
}
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(primaryListingMarketParticipantId_)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(228, primaryListingMarketParticipantId_);
}
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(subscriptionSymbol_)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(229, subscriptionSymbol_);
}
if (((bitField0_ & 0x00000040) != 0)) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(230, getContractMaturity());
}
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(underlying_)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(231, underlying_);
}
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(commodity_)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(232, commodity_);
}
if (exchangeId_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeSInt32Size(233, exchangeId_);
}
if (priceScalingExponent_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeSInt32Size(234, priceScalingExponent_);
}
if (underlyingOpenfeedMarketId_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeSInt64Size(235, underlyingOpenfeedMarketId_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.openfeed.InstrumentDefinition)) {
return super.equals(obj);
}
org.openfeed.InstrumentDefinition other = (org.openfeed.InstrumentDefinition) obj;
if (getMarketId()
!= other.getMarketId()) return false;
if (instrumentType_ != other.instrumentType_) return false;
if (!supportBookTypes_.equals(other.supportBookTypes_)) return false;
if (getBookDepth()
!= other.getBookDepth()) return false;
if (!getVendorId()
.equals(other.getVendorId())) return false;
if (!getSymbol()
.equals(other.getSymbol())) return false;
if (!getDescription()
.equals(other.getDescription())) return false;
if (!getCfiCode()
.equals(other.getCfiCode())) return false;
if (!getCurrencyCode()
.equals(other.getCurrencyCode())) return false;
if (!getExchangeCode()
.equals(other.getExchangeCode())) return false;
if (java.lang.Float.floatToIntBits(getMinimumPriceIncrement())
!= java.lang.Float.floatToIntBits(
other.getMinimumPriceIncrement())) return false;
if (java.lang.Float.floatToIntBits(getContractPointValue())
!= java.lang.Float.floatToIntBits(
other.getContractPointValue())) return false;
if (hasSchedule() != other.hasSchedule()) return false;
if (hasSchedule()) {
if (!getSchedule()
.equals(other.getSchedule())) return false;
}
if (hasCalendar() != other.hasCalendar()) return false;
if (hasCalendar()) {
if (!getCalendar()
.equals(other.getCalendar())) return false;
}
if (getRecordCreateTime()
!= other.getRecordCreateTime()) return false;
if (getRecordUpdateTime()
!= other.getRecordUpdateTime()) return false;
if (!getTimeZoneName()
.equals(other.getTimeZoneName())) return false;
if (!getInstrumentGroup()
.equals(other.getInstrumentGroup())) return false;
if (hasSymbolExpiration() != other.hasSymbolExpiration()) return false;
if (hasSymbolExpiration()) {
if (!getSymbolExpiration()
.equals(other.getSymbolExpiration())) return false;
}
if (state_ != other.state_) return false;
if (getChannel()
!= other.getChannel()) return false;
if (getUnderlyingMarketId()
!= other.getUnderlyingMarketId()) return false;
if (hasPriceFormat() != other.hasPriceFormat()) return false;
if (hasPriceFormat()) {
if (!getPriceFormat()
.equals(other.getPriceFormat())) return false;
}
if (hasOptionStrikePriceFormat() != other.hasOptionStrikePriceFormat()) return false;
if (hasOptionStrikePriceFormat()) {
if (!getOptionStrikePriceFormat()
.equals(other.getOptionStrikePriceFormat())) return false;
}
if (getPriceDenominator()
!= other.getPriceDenominator()) return false;
if (getQuantityDenominator()
!= other.getQuantityDenominator()) return false;
if (getIsTradable()
!= other.getIsTradable()) return false;
if (getTransactionTime()
!= other.getTransactionTime()) return false;
if (!getAuxiliaryData()
.equals(other.getAuxiliaryData())) return false;
if (!getSymbolsList()
.equals(other.getSymbolsList())) return false;
if (getOptionStrike()
!= other.getOptionStrike()) return false;
if (optionType_ != other.optionType_) return false;
if (optionStyle_ != other.optionStyle_) return false;
if (getOptionStrikeDenominator()
!= other.getOptionStrikeDenominator()) return false;
if (!getSpreadCode()
.equals(other.getSpreadCode())) return false;
if (!getSpreadLegList()
.equals(other.getSpreadLegList())) return false;
if (getUserDefinedSpread()
!= other.getUserDefinedSpread()) return false;
if (!getMarketTier()
.equals(other.getMarketTier())) return false;
if (!getFinancialStatusIndicator()
.equals(other.getFinancialStatusIndicator())) return false;
if (!getIsin()
.equals(other.getIsin())) return false;
if (hasCurrencyPair() != other.hasCurrencyPair()) return false;
if (hasCurrencyPair()) {
if (!getCurrencyPair()
.equals(other.getCurrencyPair())) return false;
}
if (getExchangeSendsVolume()
!= other.getExchangeSendsVolume()) return false;
if (getExchangeSendsHigh()
!= other.getExchangeSendsHigh()) return false;
if (getExchangeSendsLow()
!= other.getExchangeSendsLow()) return false;
if (getExchangeSendsOpen()
!= other.getExchangeSendsOpen()) return false;
if (getConsolidatedFeedInstrument()
!= other.getConsolidatedFeedInstrument()) return false;
if (getOpenOutcryInstrument()
!= other.getOpenOutcryInstrument()) return false;
if (getSyntheticAmericanOptionInstrument()
!= other.getSyntheticAmericanOptionInstrument()) return false;
if (!getBarchartExchangeCode()
.equals(other.getBarchartExchangeCode())) return false;
if (!getBarchartBaseCode()
.equals(other.getBarchartBaseCode())) return false;
if (getVolumeDenominator()
!= other.getVolumeDenominator()) return false;
if (getBidOfferQuantityDenominator()
!= other.getBidOfferQuantityDenominator()) return false;
if (!getPrimaryListingMarketParticipantId()
.equals(other.getPrimaryListingMarketParticipantId())) return false;
if (!getSubscriptionSymbol()
.equals(other.getSubscriptionSymbol())) return false;
if (hasContractMaturity() != other.hasContractMaturity()) return false;
if (hasContractMaturity()) {
if (!getContractMaturity()
.equals(other.getContractMaturity())) return false;
}
if (!getUnderlying()
.equals(other.getUnderlying())) return false;
if (!getCommodity()
.equals(other.getCommodity())) return false;
if (getExchangeId()
!= other.getExchangeId()) return false;
if (getPriceScalingExponent()
!= other.getPriceScalingExponent()) return false;
if (getUnderlyingOpenfeedMarketId()
!= other.getUnderlyingOpenfeedMarketId()) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + MARKETID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getMarketId());
hash = (37 * hash) + INSTRUMENTTYPE_FIELD_NUMBER;
hash = (53 * hash) + instrumentType_;
if (getSupportBookTypesCount() > 0) {
hash = (37 * hash) + SUPPORTBOOKTYPES_FIELD_NUMBER;
hash = (53 * hash) + supportBookTypes_.hashCode();
}
hash = (37 * hash) + BOOKDEPTH_FIELD_NUMBER;
hash = (53 * hash) + getBookDepth();
hash = (37 * hash) + VENDORID_FIELD_NUMBER;
hash = (53 * hash) + getVendorId().hashCode();
hash = (37 * hash) + SYMBOL_FIELD_NUMBER;
hash = (53 * hash) + getSymbol().hashCode();
hash = (37 * hash) + DESCRIPTION_FIELD_NUMBER;
hash = (53 * hash) + getDescription().hashCode();
hash = (37 * hash) + CFICODE_FIELD_NUMBER;
hash = (53 * hash) + getCfiCode().hashCode();
hash = (37 * hash) + CURRENCYCODE_FIELD_NUMBER;
hash = (53 * hash) + getCurrencyCode().hashCode();
hash = (37 * hash) + EXCHANGECODE_FIELD_NUMBER;
hash = (53 * hash) + getExchangeCode().hashCode();
hash = (37 * hash) + MINIMUMPRICEINCREMENT_FIELD_NUMBER;
hash = (53 * hash) + java.lang.Float.floatToIntBits(
getMinimumPriceIncrement());
hash = (37 * hash) + CONTRACTPOINTVALUE_FIELD_NUMBER;
hash = (53 * hash) + java.lang.Float.floatToIntBits(
getContractPointValue());
if (hasSchedule()) {
hash = (37 * hash) + SCHEDULE_FIELD_NUMBER;
hash = (53 * hash) + getSchedule().hashCode();
}
if (hasCalendar()) {
hash = (37 * hash) + CALENDAR_FIELD_NUMBER;
hash = (53 * hash) + getCalendar().hashCode();
}
hash = (37 * hash) + RECORDCREATETIME_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getRecordCreateTime());
hash = (37 * hash) + RECORDUPDATETIME_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getRecordUpdateTime());
hash = (37 * hash) + TIMEZONENAME_FIELD_NUMBER;
hash = (53 * hash) + getTimeZoneName().hashCode();
hash = (37 * hash) + INSTRUMENTGROUP_FIELD_NUMBER;
hash = (53 * hash) + getInstrumentGroup().hashCode();
if (hasSymbolExpiration()) {
hash = (37 * hash) + SYMBOLEXPIRATION_FIELD_NUMBER;
hash = (53 * hash) + getSymbolExpiration().hashCode();
}
hash = (37 * hash) + STATE_FIELD_NUMBER;
hash = (53 * hash) + state_;
hash = (37 * hash) + CHANNEL_FIELD_NUMBER;
hash = (53 * hash) + getChannel();
hash = (37 * hash) + UNDERLYINGMARKETID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getUnderlyingMarketId());
if (hasPriceFormat()) {
hash = (37 * hash) + PRICEFORMAT_FIELD_NUMBER;
hash = (53 * hash) + getPriceFormat().hashCode();
}
if (hasOptionStrikePriceFormat()) {
hash = (37 * hash) + OPTIONSTRIKEPRICEFORMAT_FIELD_NUMBER;
hash = (53 * hash) + getOptionStrikePriceFormat().hashCode();
}
hash = (37 * hash) + PRICEDENOMINATOR_FIELD_NUMBER;
hash = (53 * hash) + getPriceDenominator();
hash = (37 * hash) + QUANTITYDENOMINATOR_FIELD_NUMBER;
hash = (53 * hash) + getQuantityDenominator();
hash = (37 * hash) + ISTRADABLE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getIsTradable());
hash = (37 * hash) + TRANSACTIONTIME_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getTransactionTime());
hash = (37 * hash) + AUXILIARYDATA_FIELD_NUMBER;
hash = (53 * hash) + getAuxiliaryData().hashCode();
if (getSymbolsCount() > 0) {
hash = (37 * hash) + SYMBOLS_FIELD_NUMBER;
hash = (53 * hash) + getSymbolsList().hashCode();
}
hash = (37 * hash) + OPTIONSTRIKE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getOptionStrike());
hash = (37 * hash) + OPTIONTYPE_FIELD_NUMBER;
hash = (53 * hash) + optionType_;
hash = (37 * hash) + OPTIONSTYLE_FIELD_NUMBER;
hash = (53 * hash) + optionStyle_;
hash = (37 * hash) + OPTIONSTRIKEDENOMINATOR_FIELD_NUMBER;
hash = (53 * hash) + getOptionStrikeDenominator();
hash = (37 * hash) + SPREADCODE_FIELD_NUMBER;
hash = (53 * hash) + getSpreadCode().hashCode();
if (getSpreadLegCount() > 0) {
hash = (37 * hash) + SPREADLEG_FIELD_NUMBER;
hash = (53 * hash) + getSpreadLegList().hashCode();
}
hash = (37 * hash) + USERDEFINEDSPREAD_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getUserDefinedSpread());
hash = (37 * hash) + MARKETTIER_FIELD_NUMBER;
hash = (53 * hash) + getMarketTier().hashCode();
hash = (37 * hash) + FINANCIALSTATUSINDICATOR_FIELD_NUMBER;
hash = (53 * hash) + getFinancialStatusIndicator().hashCode();
hash = (37 * hash) + ISIN_FIELD_NUMBER;
hash = (53 * hash) + getIsin().hashCode();
if (hasCurrencyPair()) {
hash = (37 * hash) + CURRENCYPAIR_FIELD_NUMBER;
hash = (53 * hash) + getCurrencyPair().hashCode();
}
hash = (37 * hash) + EXCHANGESENDSVOLUME_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getExchangeSendsVolume());
hash = (37 * hash) + EXCHANGESENDSHIGH_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getExchangeSendsHigh());
hash = (37 * hash) + EXCHANGESENDSLOW_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getExchangeSendsLow());
hash = (37 * hash) + EXCHANGESENDSOPEN_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getExchangeSendsOpen());
hash = (37 * hash) + CONSOLIDATEDFEEDINSTRUMENT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getConsolidatedFeedInstrument());
hash = (37 * hash) + OPENOUTCRYINSTRUMENT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getOpenOutcryInstrument());
hash = (37 * hash) + SYNTHETICAMERICANOPTIONINSTRUMENT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getSyntheticAmericanOptionInstrument());
hash = (37 * hash) + BARCHARTEXCHANGECODE_FIELD_NUMBER;
hash = (53 * hash) + getBarchartExchangeCode().hashCode();
hash = (37 * hash) + BARCHARTBASECODE_FIELD_NUMBER;
hash = (53 * hash) + getBarchartBaseCode().hashCode();
hash = (37 * hash) + VOLUMEDENOMINATOR_FIELD_NUMBER;
hash = (53 * hash) + getVolumeDenominator();
hash = (37 * hash) + BIDOFFERQUANTITYDENOMINATOR_FIELD_NUMBER;
hash = (53 * hash) + getBidOfferQuantityDenominator();
hash = (37 * hash) + PRIMARYLISTINGMARKETPARTICIPANTID_FIELD_NUMBER;
hash = (53 * hash) + getPrimaryListingMarketParticipantId().hashCode();
hash = (37 * hash) + SUBSCRIPTIONSYMBOL_FIELD_NUMBER;
hash = (53 * hash) + getSubscriptionSymbol().hashCode();
if (hasContractMaturity()) {
hash = (37 * hash) + CONTRACTMATURITY_FIELD_NUMBER;
hash = (53 * hash) + getContractMaturity().hashCode();
}
hash = (37 * hash) + UNDERLYING_FIELD_NUMBER;
hash = (53 * hash) + getUnderlying().hashCode();
hash = (37 * hash) + COMMODITY_FIELD_NUMBER;
hash = (53 * hash) + getCommodity().hashCode();
hash = (37 * hash) + EXCHANGEID_FIELD_NUMBER;
hash = (53 * hash) + getExchangeId();
hash = (37 * hash) + PRICESCALINGEXPONENT_FIELD_NUMBER;
hash = (53 * hash) + getPriceScalingExponent();
hash = (37 * hash) + UNDERLYINGOPENFEEDMARKETID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getUnderlyingOpenfeedMarketId());
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.openfeed.InstrumentDefinition parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.openfeed.InstrumentDefinition parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.openfeed.InstrumentDefinition parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.openfeed.InstrumentDefinition parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.openfeed.InstrumentDefinition parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.openfeed.InstrumentDefinition parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.openfeed.InstrumentDefinition parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static org.openfeed.InstrumentDefinition parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.openfeed.InstrumentDefinition parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input);
}
public static org.openfeed.InstrumentDefinition parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.openfeed.InstrumentDefinition parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static org.openfeed.InstrumentDefinition parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.openfeed.InstrumentDefinition prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code org.openfeed.InstrumentDefinition}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:org.openfeed.InstrumentDefinition)
org.openfeed.InstrumentDefinitionOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.openfeed.OpenfeedInstrument.internal_static_org_openfeed_InstrumentDefinition_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.openfeed.OpenfeedInstrument.internal_static_org_openfeed_InstrumentDefinition_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.openfeed.InstrumentDefinition.class, org.openfeed.InstrumentDefinition.Builder.class);
}
// Construct using org.openfeed.InstrumentDefinition.newBuilder()
private Builder() {
maybeForceBuilderInitialization();
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
maybeForceBuilderInitialization();
}
private void maybeForceBuilderInitialization() {
if (com.google.protobuf.GeneratedMessage
.alwaysUseFieldBuilders) {
getScheduleFieldBuilder();
getCalendarFieldBuilder();
getSymbolExpirationFieldBuilder();
getPriceFormatFieldBuilder();
getOptionStrikePriceFormatFieldBuilder();
getSymbolsFieldBuilder();
getSpreadLegFieldBuilder();
getCurrencyPairFieldBuilder();
getContractMaturityFieldBuilder();
}
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
bitField1_ = 0;
marketId_ = 0L;
instrumentType_ = 0;
supportBookTypes_ = emptyIntList();
bitField0_ = (bitField0_ & ~0x00000004);
bookDepth_ = 0;
vendorId_ = "";
symbol_ = "";
description_ = "";
cfiCode_ = "";
currencyCode_ = "";
exchangeCode_ = "";
minimumPriceIncrement_ = 0F;
contractPointValue_ = 0F;
schedule_ = null;
if (scheduleBuilder_ != null) {
scheduleBuilder_.dispose();
scheduleBuilder_ = null;
}
calendar_ = null;
if (calendarBuilder_ != null) {
calendarBuilder_.dispose();
calendarBuilder_ = null;
}
recordCreateTime_ = 0L;
recordUpdateTime_ = 0L;
timeZoneName_ = "";
instrumentGroup_ = "";
symbolExpiration_ = null;
if (symbolExpirationBuilder_ != null) {
symbolExpirationBuilder_.dispose();
symbolExpirationBuilder_ = null;
}
state_ = 0;
channel_ = 0;
underlyingMarketId_ = 0L;
priceFormat_ = null;
if (priceFormatBuilder_ != null) {
priceFormatBuilder_.dispose();
priceFormatBuilder_ = null;
}
optionStrikePriceFormat_ = null;
if (optionStrikePriceFormatBuilder_ != null) {
optionStrikePriceFormatBuilder_.dispose();
optionStrikePriceFormatBuilder_ = null;
}
priceDenominator_ = 0;
quantityDenominator_ = 0;
isTradable_ = false;
transactionTime_ = 0L;
auxiliaryData_ = com.google.protobuf.ByteString.EMPTY;
if (symbolsBuilder_ == null) {
symbols_ = java.util.Collections.emptyList();
} else {
symbols_ = null;
symbolsBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x20000000);
optionStrike_ = 0L;
optionType_ = 0;
optionStyle_ = 0;
optionStrikeDenominator_ = 0;
spreadCode_ = "";
if (spreadLegBuilder_ == null) {
spreadLeg_ = java.util.Collections.emptyList();
} else {
spreadLeg_ = null;
spreadLegBuilder_.clear();
}
bitField1_ = (bitField1_ & ~0x00000008);
userDefinedSpread_ = false;
marketTier_ = "";
financialStatusIndicator_ = "";
isin_ = "";
currencyPair_ = null;
if (currencyPairBuilder_ != null) {
currencyPairBuilder_.dispose();
currencyPairBuilder_ = null;
}
exchangeSendsVolume_ = false;
exchangeSendsHigh_ = false;
exchangeSendsLow_ = false;
exchangeSendsOpen_ = false;
consolidatedFeedInstrument_ = false;
openOutcryInstrument_ = false;
syntheticAmericanOptionInstrument_ = false;
barchartExchangeCode_ = "";
barchartBaseCode_ = "";
volumeDenominator_ = 0;
bidOfferQuantityDenominator_ = 0;
primaryListingMarketParticipantId_ = "";
subscriptionSymbol_ = "";
contractMaturity_ = null;
if (contractMaturityBuilder_ != null) {
contractMaturityBuilder_.dispose();
contractMaturityBuilder_ = null;
}
underlying_ = "";
commodity_ = "";
exchangeId_ = 0;
priceScalingExponent_ = 0;
underlyingOpenfeedMarketId_ = 0L;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.openfeed.OpenfeedInstrument.internal_static_org_openfeed_InstrumentDefinition_descriptor;
}
@java.lang.Override
public org.openfeed.InstrumentDefinition getDefaultInstanceForType() {
return org.openfeed.InstrumentDefinition.getDefaultInstance();
}
@java.lang.Override
public org.openfeed.InstrumentDefinition build() {
org.openfeed.InstrumentDefinition result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.openfeed.InstrumentDefinition buildPartial() {
org.openfeed.InstrumentDefinition result = new org.openfeed.InstrumentDefinition(this);
buildPartialRepeatedFields(result);
if (bitField0_ != 0) { buildPartial0(result); }
if (bitField1_ != 0) { buildPartial1(result); }
onBuilt();
return result;
}
private void buildPartialRepeatedFields(org.openfeed.InstrumentDefinition result) {
if (((bitField0_ & 0x00000004) != 0)) {
supportBookTypes_.makeImmutable();
bitField0_ = (bitField0_ & ~0x00000004);
}
result.supportBookTypes_ = supportBookTypes_;
if (symbolsBuilder_ == null) {
if (((bitField0_ & 0x20000000) != 0)) {
symbols_ = java.util.Collections.unmodifiableList(symbols_);
bitField0_ = (bitField0_ & ~0x20000000);
}
result.symbols_ = symbols_;
} else {
result.symbols_ = symbolsBuilder_.build();
}
if (spreadLegBuilder_ == null) {
if (((bitField1_ & 0x00000008) != 0)) {
spreadLeg_ = java.util.Collections.unmodifiableList(spreadLeg_);
bitField1_ = (bitField1_ & ~0x00000008);
}
result.spreadLeg_ = spreadLeg_;
} else {
result.spreadLeg_ = spreadLegBuilder_.build();
}
}
private void buildPartial0(org.openfeed.InstrumentDefinition result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.marketId_ = marketId_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.instrumentType_ = instrumentType_;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.bookDepth_ = bookDepth_;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.vendorId_ = vendorId_;
}
if (((from_bitField0_ & 0x00000020) != 0)) {
result.symbol_ = symbol_;
}
if (((from_bitField0_ & 0x00000040) != 0)) {
result.description_ = description_;
}
if (((from_bitField0_ & 0x00000080) != 0)) {
result.cfiCode_ = cfiCode_;
}
if (((from_bitField0_ & 0x00000100) != 0)) {
result.currencyCode_ = currencyCode_;
}
if (((from_bitField0_ & 0x00000200) != 0)) {
result.exchangeCode_ = exchangeCode_;
}
if (((from_bitField0_ & 0x00000400) != 0)) {
result.minimumPriceIncrement_ = minimumPriceIncrement_;
}
if (((from_bitField0_ & 0x00000800) != 0)) {
result.contractPointValue_ = contractPointValue_;
}
int to_bitField0_ = 0;
if (((from_bitField0_ & 0x00001000) != 0)) {
result.schedule_ = scheduleBuilder_ == null
? schedule_
: scheduleBuilder_.build();
to_bitField0_ |= 0x00000001;
}
if (((from_bitField0_ & 0x00002000) != 0)) {
result.calendar_ = calendarBuilder_ == null
? calendar_
: calendarBuilder_.build();
to_bitField0_ |= 0x00000002;
}
if (((from_bitField0_ & 0x00004000) != 0)) {
result.recordCreateTime_ = recordCreateTime_;
}
if (((from_bitField0_ & 0x00008000) != 0)) {
result.recordUpdateTime_ = recordUpdateTime_;
}
if (((from_bitField0_ & 0x00010000) != 0)) {
result.timeZoneName_ = timeZoneName_;
}
if (((from_bitField0_ & 0x00020000) != 0)) {
result.instrumentGroup_ = instrumentGroup_;
}
if (((from_bitField0_ & 0x00040000) != 0)) {
result.symbolExpiration_ = symbolExpirationBuilder_ == null
? symbolExpiration_
: symbolExpirationBuilder_.build();
to_bitField0_ |= 0x00000004;
}
if (((from_bitField0_ & 0x00080000) != 0)) {
result.state_ = state_;
}
if (((from_bitField0_ & 0x00100000) != 0)) {
result.channel_ = channel_;
}
if (((from_bitField0_ & 0x00200000) != 0)) {
result.underlyingMarketId_ = underlyingMarketId_;
}
if (((from_bitField0_ & 0x00400000) != 0)) {
result.priceFormat_ = priceFormatBuilder_ == null
? priceFormat_
: priceFormatBuilder_.build();
to_bitField0_ |= 0x00000008;
}
if (((from_bitField0_ & 0x00800000) != 0)) {
result.optionStrikePriceFormat_ = optionStrikePriceFormatBuilder_ == null
? optionStrikePriceFormat_
: optionStrikePriceFormatBuilder_.build();
to_bitField0_ |= 0x00000010;
}
if (((from_bitField0_ & 0x01000000) != 0)) {
result.priceDenominator_ = priceDenominator_;
}
if (((from_bitField0_ & 0x02000000) != 0)) {
result.quantityDenominator_ = quantityDenominator_;
}
if (((from_bitField0_ & 0x04000000) != 0)) {
result.isTradable_ = isTradable_;
}
if (((from_bitField0_ & 0x08000000) != 0)) {
result.transactionTime_ = transactionTime_;
}
if (((from_bitField0_ & 0x10000000) != 0)) {
result.auxiliaryData_ = auxiliaryData_;
}
if (((from_bitField0_ & 0x40000000) != 0)) {
result.optionStrike_ = optionStrike_;
}
if (((from_bitField0_ & 0x80000000) != 0)) {
result.optionType_ = optionType_;
}
result.bitField0_ |= to_bitField0_;
}
private void buildPartial1(org.openfeed.InstrumentDefinition result) {
int from_bitField1_ = bitField1_;
if (((from_bitField1_ & 0x00000001) != 0)) {
result.optionStyle_ = optionStyle_;
}
if (((from_bitField1_ & 0x00000002) != 0)) {
result.optionStrikeDenominator_ = optionStrikeDenominator_;
}
if (((from_bitField1_ & 0x00000004) != 0)) {
result.spreadCode_ = spreadCode_;
}
if (((from_bitField1_ & 0x00000010) != 0)) {
result.userDefinedSpread_ = userDefinedSpread_;
}
if (((from_bitField1_ & 0x00000020) != 0)) {
result.marketTier_ = marketTier_;
}
if (((from_bitField1_ & 0x00000040) != 0)) {
result.financialStatusIndicator_ = financialStatusIndicator_;
}
if (((from_bitField1_ & 0x00000080) != 0)) {
result.isin_ = isin_;
}
int to_bitField0_ = 0;
if (((from_bitField1_ & 0x00000100) != 0)) {
result.currencyPair_ = currencyPairBuilder_ == null
? currencyPair_
: currencyPairBuilder_.build();
to_bitField0_ |= 0x00000020;
}
if (((from_bitField1_ & 0x00000200) != 0)) {
result.exchangeSendsVolume_ = exchangeSendsVolume_;
}
if (((from_bitField1_ & 0x00000400) != 0)) {
result.exchangeSendsHigh_ = exchangeSendsHigh_;
}
if (((from_bitField1_ & 0x00000800) != 0)) {
result.exchangeSendsLow_ = exchangeSendsLow_;
}
if (((from_bitField1_ & 0x00001000) != 0)) {
result.exchangeSendsOpen_ = exchangeSendsOpen_;
}
if (((from_bitField1_ & 0x00002000) != 0)) {
result.consolidatedFeedInstrument_ = consolidatedFeedInstrument_;
}
if (((from_bitField1_ & 0x00004000) != 0)) {
result.openOutcryInstrument_ = openOutcryInstrument_;
}
if (((from_bitField1_ & 0x00008000) != 0)) {
result.syntheticAmericanOptionInstrument_ = syntheticAmericanOptionInstrument_;
}
if (((from_bitField1_ & 0x00010000) != 0)) {
result.barchartExchangeCode_ = barchartExchangeCode_;
}
if (((from_bitField1_ & 0x00020000) != 0)) {
result.barchartBaseCode_ = barchartBaseCode_;
}
if (((from_bitField1_ & 0x00040000) != 0)) {
result.volumeDenominator_ = volumeDenominator_;
}
if (((from_bitField1_ & 0x00080000) != 0)) {
result.bidOfferQuantityDenominator_ = bidOfferQuantityDenominator_;
}
if (((from_bitField1_ & 0x00100000) != 0)) {
result.primaryListingMarketParticipantId_ = primaryListingMarketParticipantId_;
}
if (((from_bitField1_ & 0x00200000) != 0)) {
result.subscriptionSymbol_ = subscriptionSymbol_;
}
if (((from_bitField1_ & 0x00400000) != 0)) {
result.contractMaturity_ = contractMaturityBuilder_ == null
? contractMaturity_
: contractMaturityBuilder_.build();
to_bitField0_ |= 0x00000040;
}
if (((from_bitField1_ & 0x00800000) != 0)) {
result.underlying_ = underlying_;
}
if (((from_bitField1_ & 0x01000000) != 0)) {
result.commodity_ = commodity_;
}
if (((from_bitField1_ & 0x02000000) != 0)) {
result.exchangeId_ = exchangeId_;
}
if (((from_bitField1_ & 0x04000000) != 0)) {
result.priceScalingExponent_ = priceScalingExponent_;
}
if (((from_bitField1_ & 0x08000000) != 0)) {
result.underlyingOpenfeedMarketId_ = underlyingOpenfeedMarketId_;
}
result.bitField0_ |= to_bitField0_;
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.openfeed.InstrumentDefinition) {
return mergeFrom((org.openfeed.InstrumentDefinition)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.openfeed.InstrumentDefinition other) {
if (other == org.openfeed.InstrumentDefinition.getDefaultInstance()) return this;
if (other.getMarketId() != 0L) {
setMarketId(other.getMarketId());
}
if (other.instrumentType_ != 0) {
setInstrumentTypeValue(other.getInstrumentTypeValue());
}
if (!other.supportBookTypes_.isEmpty()) {
if (supportBookTypes_.isEmpty()) {
supportBookTypes_ = other.supportBookTypes_;
bitField0_ = (bitField0_ & ~0x00000004);
} else {
ensureSupportBookTypesIsMutable();
supportBookTypes_.addAll(other.supportBookTypes_);
}
onChanged();
}
if (other.getBookDepth() != 0) {
setBookDepth(other.getBookDepth());
}
if (!other.getVendorId().isEmpty()) {
vendorId_ = other.vendorId_;
bitField0_ |= 0x00000010;
onChanged();
}
if (!other.getSymbol().isEmpty()) {
symbol_ = other.symbol_;
bitField0_ |= 0x00000020;
onChanged();
}
if (!other.getDescription().isEmpty()) {
description_ = other.description_;
bitField0_ |= 0x00000040;
onChanged();
}
if (!other.getCfiCode().isEmpty()) {
cfiCode_ = other.cfiCode_;
bitField0_ |= 0x00000080;
onChanged();
}
if (!other.getCurrencyCode().isEmpty()) {
currencyCode_ = other.currencyCode_;
bitField0_ |= 0x00000100;
onChanged();
}
if (!other.getExchangeCode().isEmpty()) {
exchangeCode_ = other.exchangeCode_;
bitField0_ |= 0x00000200;
onChanged();
}
if (other.getMinimumPriceIncrement() != 0F) {
setMinimumPriceIncrement(other.getMinimumPriceIncrement());
}
if (other.getContractPointValue() != 0F) {
setContractPointValue(other.getContractPointValue());
}
if (other.hasSchedule()) {
mergeSchedule(other.getSchedule());
}
if (other.hasCalendar()) {
mergeCalendar(other.getCalendar());
}
if (other.getRecordCreateTime() != 0L) {
setRecordCreateTime(other.getRecordCreateTime());
}
if (other.getRecordUpdateTime() != 0L) {
setRecordUpdateTime(other.getRecordUpdateTime());
}
if (!other.getTimeZoneName().isEmpty()) {
timeZoneName_ = other.timeZoneName_;
bitField0_ |= 0x00010000;
onChanged();
}
if (!other.getInstrumentGroup().isEmpty()) {
instrumentGroup_ = other.instrumentGroup_;
bitField0_ |= 0x00020000;
onChanged();
}
if (other.hasSymbolExpiration()) {
mergeSymbolExpiration(other.getSymbolExpiration());
}
if (other.state_ != 0) {
setStateValue(other.getStateValue());
}
if (other.getChannel() != 0) {
setChannel(other.getChannel());
}
if (other.getUnderlyingMarketId() != 0L) {
setUnderlyingMarketId(other.getUnderlyingMarketId());
}
if (other.hasPriceFormat()) {
mergePriceFormat(other.getPriceFormat());
}
if (other.hasOptionStrikePriceFormat()) {
mergeOptionStrikePriceFormat(other.getOptionStrikePriceFormat());
}
if (other.getPriceDenominator() != 0) {
setPriceDenominator(other.getPriceDenominator());
}
if (other.getQuantityDenominator() != 0) {
setQuantityDenominator(other.getQuantityDenominator());
}
if (other.getIsTradable() != false) {
setIsTradable(other.getIsTradable());
}
if (other.getTransactionTime() != 0L) {
setTransactionTime(other.getTransactionTime());
}
if (other.getAuxiliaryData() != com.google.protobuf.ByteString.EMPTY) {
setAuxiliaryData(other.getAuxiliaryData());
}
if (symbolsBuilder_ == null) {
if (!other.symbols_.isEmpty()) {
if (symbols_.isEmpty()) {
symbols_ = other.symbols_;
bitField0_ = (bitField0_ & ~0x20000000);
} else {
ensureSymbolsIsMutable();
symbols_.addAll(other.symbols_);
}
onChanged();
}
} else {
if (!other.symbols_.isEmpty()) {
if (symbolsBuilder_.isEmpty()) {
symbolsBuilder_.dispose();
symbolsBuilder_ = null;
symbols_ = other.symbols_;
bitField0_ = (bitField0_ & ~0x20000000);
symbolsBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getSymbolsFieldBuilder() : null;
} else {
symbolsBuilder_.addAllMessages(other.symbols_);
}
}
}
if (other.getOptionStrike() != 0L) {
setOptionStrike(other.getOptionStrike());
}
if (other.optionType_ != 0) {
setOptionTypeValue(other.getOptionTypeValue());
}
if (other.optionStyle_ != 0) {
setOptionStyleValue(other.getOptionStyleValue());
}
if (other.getOptionStrikeDenominator() != 0) {
setOptionStrikeDenominator(other.getOptionStrikeDenominator());
}
if (!other.getSpreadCode().isEmpty()) {
spreadCode_ = other.spreadCode_;
bitField1_ |= 0x00000004;
onChanged();
}
if (spreadLegBuilder_ == null) {
if (!other.spreadLeg_.isEmpty()) {
if (spreadLeg_.isEmpty()) {
spreadLeg_ = other.spreadLeg_;
bitField1_ = (bitField1_ & ~0x00000008);
} else {
ensureSpreadLegIsMutable();
spreadLeg_.addAll(other.spreadLeg_);
}
onChanged();
}
} else {
if (!other.spreadLeg_.isEmpty()) {
if (spreadLegBuilder_.isEmpty()) {
spreadLegBuilder_.dispose();
spreadLegBuilder_ = null;
spreadLeg_ = other.spreadLeg_;
bitField1_ = (bitField1_ & ~0x00000008);
spreadLegBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getSpreadLegFieldBuilder() : null;
} else {
spreadLegBuilder_.addAllMessages(other.spreadLeg_);
}
}
}
if (other.getUserDefinedSpread() != false) {
setUserDefinedSpread(other.getUserDefinedSpread());
}
if (!other.getMarketTier().isEmpty()) {
marketTier_ = other.marketTier_;
bitField1_ |= 0x00000020;
onChanged();
}
if (!other.getFinancialStatusIndicator().isEmpty()) {
financialStatusIndicator_ = other.financialStatusIndicator_;
bitField1_ |= 0x00000040;
onChanged();
}
if (!other.getIsin().isEmpty()) {
isin_ = other.isin_;
bitField1_ |= 0x00000080;
onChanged();
}
if (other.hasCurrencyPair()) {
mergeCurrencyPair(other.getCurrencyPair());
}
if (other.getExchangeSendsVolume() != false) {
setExchangeSendsVolume(other.getExchangeSendsVolume());
}
if (other.getExchangeSendsHigh() != false) {
setExchangeSendsHigh(other.getExchangeSendsHigh());
}
if (other.getExchangeSendsLow() != false) {
setExchangeSendsLow(other.getExchangeSendsLow());
}
if (other.getExchangeSendsOpen() != false) {
setExchangeSendsOpen(other.getExchangeSendsOpen());
}
if (other.getConsolidatedFeedInstrument() != false) {
setConsolidatedFeedInstrument(other.getConsolidatedFeedInstrument());
}
if (other.getOpenOutcryInstrument() != false) {
setOpenOutcryInstrument(other.getOpenOutcryInstrument());
}
if (other.getSyntheticAmericanOptionInstrument() != false) {
setSyntheticAmericanOptionInstrument(other.getSyntheticAmericanOptionInstrument());
}
if (!other.getBarchartExchangeCode().isEmpty()) {
barchartExchangeCode_ = other.barchartExchangeCode_;
bitField1_ |= 0x00010000;
onChanged();
}
if (!other.getBarchartBaseCode().isEmpty()) {
barchartBaseCode_ = other.barchartBaseCode_;
bitField1_ |= 0x00020000;
onChanged();
}
if (other.getVolumeDenominator() != 0) {
setVolumeDenominator(other.getVolumeDenominator());
}
if (other.getBidOfferQuantityDenominator() != 0) {
setBidOfferQuantityDenominator(other.getBidOfferQuantityDenominator());
}
if (!other.getPrimaryListingMarketParticipantId().isEmpty()) {
primaryListingMarketParticipantId_ = other.primaryListingMarketParticipantId_;
bitField1_ |= 0x00100000;
onChanged();
}
if (!other.getSubscriptionSymbol().isEmpty()) {
subscriptionSymbol_ = other.subscriptionSymbol_;
bitField1_ |= 0x00200000;
onChanged();
}
if (other.hasContractMaturity()) {
mergeContractMaturity(other.getContractMaturity());
}
if (!other.getUnderlying().isEmpty()) {
underlying_ = other.underlying_;
bitField1_ |= 0x00800000;
onChanged();
}
if (!other.getCommodity().isEmpty()) {
commodity_ = other.commodity_;
bitField1_ |= 0x01000000;
onChanged();
}
if (other.getExchangeId() != 0) {
setExchangeId(other.getExchangeId());
}
if (other.getPriceScalingExponent() != 0) {
setPriceScalingExponent(other.getPriceScalingExponent());
}
if (other.getUnderlyingOpenfeedMarketId() != 0L) {
setUnderlyingOpenfeedMarketId(other.getUnderlyingOpenfeedMarketId());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
marketId_ = input.readSInt64();
bitField0_ |= 0x00000001;
break;
} // case 8
case 16: {
instrumentType_ = input.readEnum();
bitField0_ |= 0x00000002;
break;
} // case 16
case 24: {
int tmpRaw = input.readEnum();
ensureSupportBookTypesIsMutable();
supportBookTypes_.addInt(tmpRaw);
break;
} // case 24
case 26: {
int length = input.readRawVarint32();
int oldLimit = input.pushLimit(length);
while(input.getBytesUntilLimit() > 0) {
int tmpRaw = input.readEnum();
ensureSupportBookTypesIsMutable();
supportBookTypes_.addInt(tmpRaw);
}
input.popLimit(oldLimit);
break;
} // case 26
case 32: {
bookDepth_ = input.readSInt32();
bitField0_ |= 0x00000008;
break;
} // case 32
case 42: {
vendorId_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000010;
break;
} // case 42
case 50: {
symbol_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000020;
break;
} // case 50
case 58: {
description_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000040;
break;
} // case 58
case 66: {
cfiCode_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000080;
break;
} // case 66
case 74: {
currencyCode_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000100;
break;
} // case 74
case 82: {
exchangeCode_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000200;
break;
} // case 82
case 93: {
minimumPriceIncrement_ = input.readFloat();
bitField0_ |= 0x00000400;
break;
} // case 93
case 101: {
contractPointValue_ = input.readFloat();
bitField0_ |= 0x00000800;
break;
} // case 101
case 106: {
input.readMessage(
getScheduleFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00001000;
break;
} // case 106
case 114: {
input.readMessage(
getCalendarFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00002000;
break;
} // case 114
case 120: {
recordCreateTime_ = input.readSInt64();
bitField0_ |= 0x00004000;
break;
} // case 120
case 128: {
recordUpdateTime_ = input.readSInt64();
bitField0_ |= 0x00008000;
break;
} // case 128
case 138: {
timeZoneName_ = input.readStringRequireUtf8();
bitField0_ |= 0x00010000;
break;
} // case 138
case 146: {
instrumentGroup_ = input.readStringRequireUtf8();
bitField0_ |= 0x00020000;
break;
} // case 146
case 154: {
input.readMessage(
getSymbolExpirationFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00040000;
break;
} // case 154
case 160: {
state_ = input.readEnum();
bitField0_ |= 0x00080000;
break;
} // case 160
case 168: {
channel_ = input.readSInt32();
bitField0_ |= 0x00100000;
break;
} // case 168
case 176: {
underlyingMarketId_ = input.readSInt64();
bitField0_ |= 0x00200000;
break;
} // case 176
case 186: {
input.readMessage(
getPriceFormatFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00400000;
break;
} // case 186
case 194: {
input.readMessage(
getOptionStrikePriceFormatFieldBuilder().getBuilder(),
extensionRegistry);
bitField0_ |= 0x00800000;
break;
} // case 194
case 224: {
priceDenominator_ = input.readSInt32();
bitField0_ |= 0x01000000;
break;
} // case 224
case 232: {
quantityDenominator_ = input.readSInt32();
bitField0_ |= 0x02000000;
break;
} // case 232
case 240: {
isTradable_ = input.readBool();
bitField0_ |= 0x04000000;
break;
} // case 240
case 400: {
transactionTime_ = input.readSInt64();
bitField0_ |= 0x08000000;
break;
} // case 400
case 794: {
auxiliaryData_ = input.readBytes();
bitField0_ |= 0x10000000;
break;
} // case 794
case 802: {
org.openfeed.InstrumentDefinition.Symbol m =
input.readMessage(
org.openfeed.InstrumentDefinition.Symbol.parser(),
extensionRegistry);
if (symbolsBuilder_ == null) {
ensureSymbolsIsMutable();
symbols_.add(m);
} else {
symbolsBuilder_.addMessage(m);
}
break;
} // case 802
case 1600: {
optionStrike_ = input.readSInt64();
bitField0_ |= 0x40000000;
break;
} // case 1600
case 1616: {
optionType_ = input.readEnum();
bitField0_ |= 0x80000000;
break;
} // case 1616
case 1624: {
optionStyle_ = input.readEnum();
bitField1_ |= 0x00000001;
break;
} // case 1624
case 1632: {
optionStrikeDenominator_ = input.readSInt32();
bitField1_ |= 0x00000002;
break;
} // case 1632
case 1682: {
spreadCode_ = input.readStringRequireUtf8();
bitField1_ |= 0x00000004;
break;
} // case 1682
case 1690: {
org.openfeed.InstrumentDefinition.SpreadLeg m =
input.readMessage(
org.openfeed.InstrumentDefinition.SpreadLeg.parser(),
extensionRegistry);
if (spreadLegBuilder_ == null) {
ensureSpreadLegIsMutable();
spreadLeg_.add(m);
} else {
spreadLegBuilder_.addMessage(m);
}
break;
} // case 1690
case 1696: {
userDefinedSpread_ = input.readBool();
bitField1_ |= 0x00000010;
break;
} // case 1696
case 1706: {
marketTier_ = input.readStringRequireUtf8();
bitField1_ |= 0x00000020;
break;
} // case 1706
case 1714: {
financialStatusIndicator_ = input.readStringRequireUtf8();
bitField1_ |= 0x00000040;
break;
} // case 1714
case 1722: {
isin_ = input.readStringRequireUtf8();
bitField1_ |= 0x00000080;
break;
} // case 1722
case 1730: {
input.readMessage(
getCurrencyPairFieldBuilder().getBuilder(),
extensionRegistry);
bitField1_ |= 0x00000100;
break;
} // case 1730
case 1736: {
exchangeSendsVolume_ = input.readBool();
bitField1_ |= 0x00000200;
break;
} // case 1736
case 1744: {
exchangeSendsHigh_ = input.readBool();
bitField1_ |= 0x00000400;
break;
} // case 1744
case 1752: {
exchangeSendsLow_ = input.readBool();
bitField1_ |= 0x00000800;
break;
} // case 1752
case 1760: {
exchangeSendsOpen_ = input.readBool();
bitField1_ |= 0x00001000;
break;
} // case 1760
case 1768: {
consolidatedFeedInstrument_ = input.readBool();
bitField1_ |= 0x00002000;
break;
} // case 1768
case 1776: {
openOutcryInstrument_ = input.readBool();
bitField1_ |= 0x00004000;
break;
} // case 1776
case 1784: {
syntheticAmericanOptionInstrument_ = input.readBool();
bitField1_ |= 0x00008000;
break;
} // case 1784
case 1794: {
barchartExchangeCode_ = input.readStringRequireUtf8();
bitField1_ |= 0x00010000;
break;
} // case 1794
case 1802: {
barchartBaseCode_ = input.readStringRequireUtf8();
bitField1_ |= 0x00020000;
break;
} // case 1802
case 1808: {
volumeDenominator_ = input.readSInt32();
bitField1_ |= 0x00040000;
break;
} // case 1808
case 1816: {
bidOfferQuantityDenominator_ = input.readSInt32();
bitField1_ |= 0x00080000;
break;
} // case 1816
case 1826: {
primaryListingMarketParticipantId_ = input.readStringRequireUtf8();
bitField1_ |= 0x00100000;
break;
} // case 1826
case 1834: {
subscriptionSymbol_ = input.readStringRequireUtf8();
bitField1_ |= 0x00200000;
break;
} // case 1834
case 1842: {
input.readMessage(
getContractMaturityFieldBuilder().getBuilder(),
extensionRegistry);
bitField1_ |= 0x00400000;
break;
} // case 1842
case 1850: {
underlying_ = input.readStringRequireUtf8();
bitField1_ |= 0x00800000;
break;
} // case 1850
case 1858: {
commodity_ = input.readStringRequireUtf8();
bitField1_ |= 0x01000000;
break;
} // case 1858
case 1864: {
exchangeId_ = input.readSInt32();
bitField1_ |= 0x02000000;
break;
} // case 1864
case 1872: {
priceScalingExponent_ = input.readSInt32();
bitField1_ |= 0x04000000;
break;
} // case 1872
case 1880: {
underlyingOpenfeedMarketId_ = input.readSInt64();
bitField1_ |= 0x08000000;
break;
} // case 1880
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private int bitField1_;
private long marketId_ ;
/**
*
* / Unique ID used in the data feed.
*
*
* sint64 marketId = 1;
* @return The marketId.
*/
@java.lang.Override
public long getMarketId() {
return marketId_;
}
/**
*
* / Unique ID used in the data feed.
*
*
* sint64 marketId = 1;
* @param value The marketId to set.
* @return This builder for chaining.
*/
public Builder setMarketId(long value) {
marketId_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* / Unique ID used in the data feed.
*
*
* sint64 marketId = 1;
* @return This builder for chaining.
*/
public Builder clearMarketId() {
bitField0_ = (bitField0_ & ~0x00000001);
marketId_ = 0L;
onChanged();
return this;
}
private int instrumentType_ = 0;
/**
*
* / Instrument type as enum...
*
*
* .org.openfeed.InstrumentDefinition.InstrumentType instrumentType = 2;
* @return The enum numeric value on the wire for instrumentType.
*/
@java.lang.Override public int getInstrumentTypeValue() {
return instrumentType_;
}
/**
*
* / Instrument type as enum...
*
*
* .org.openfeed.InstrumentDefinition.InstrumentType instrumentType = 2;
* @param value The enum numeric value on the wire for instrumentType to set.
* @return This builder for chaining.
*/
public Builder setInstrumentTypeValue(int value) {
instrumentType_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
*
* / Instrument type as enum...
*
*
* .org.openfeed.InstrumentDefinition.InstrumentType instrumentType = 2;
* @return The instrumentType.
*/
@java.lang.Override
public org.openfeed.InstrumentDefinition.InstrumentType getInstrumentType() {
org.openfeed.InstrumentDefinition.InstrumentType result = org.openfeed.InstrumentDefinition.InstrumentType.forNumber(instrumentType_);
return result == null ? org.openfeed.InstrumentDefinition.InstrumentType.UNRECOGNIZED : result;
}
/**
*
* / Instrument type as enum...
*
*
* .org.openfeed.InstrumentDefinition.InstrumentType instrumentType = 2;
* @param value The instrumentType to set.
* @return This builder for chaining.
*/
public Builder setInstrumentType(org.openfeed.InstrumentDefinition.InstrumentType value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000002;
instrumentType_ = value.getNumber();
onChanged();
return this;
}
/**
*
* / Instrument type as enum...
*
*
* .org.openfeed.InstrumentDefinition.InstrumentType instrumentType = 2;
* @return This builder for chaining.
*/
public Builder clearInstrumentType() {
bitField0_ = (bitField0_ & ~0x00000002);
instrumentType_ = 0;
onChanged();
return this;
}
private com.google.protobuf.Internal.IntList supportBookTypes_ =
emptyIntList();
private void ensureSupportBookTypesIsMutable() {
if (!((bitField0_ & 0x00000004) != 0)) {
supportBookTypes_ = makeMutableCopy(supportBookTypes_);
bitField0_ |= 0x00000004;
}
}
/**
*
* / Supported Book Types
*
*
* repeated .org.openfeed.InstrumentDefinition.BookType supportBookTypes = 3;
* @return A list containing the supportBookTypes.
*/
public java.util.List getSupportBookTypesList() {
return new com.google.protobuf.Internal.IntListAdapter<
org.openfeed.InstrumentDefinition.BookType>(supportBookTypes_, supportBookTypes_converter_);
}
/**
*
* / Supported Book Types
*
*
* repeated .org.openfeed.InstrumentDefinition.BookType supportBookTypes = 3;
* @return The count of supportBookTypes.
*/
public int getSupportBookTypesCount() {
return supportBookTypes_.size();
}
/**
*
* / Supported Book Types
*
*
* repeated .org.openfeed.InstrumentDefinition.BookType supportBookTypes = 3;
* @param index The index of the element to return.
* @return The supportBookTypes at the given index.
*/
public org.openfeed.InstrumentDefinition.BookType getSupportBookTypes(int index) {
return supportBookTypes_converter_.convert(supportBookTypes_.getInt(index));
}
/**
*
* / Supported Book Types
*
*
* repeated .org.openfeed.InstrumentDefinition.BookType supportBookTypes = 3;
* @param index The index to set the value at.
* @param value The supportBookTypes to set.
* @return This builder for chaining.
*/
public Builder setSupportBookTypes(
int index, org.openfeed.InstrumentDefinition.BookType value) {
if (value == null) {
throw new NullPointerException();
}
ensureSupportBookTypesIsMutable();
supportBookTypes_.setInt(index, value.getNumber());
onChanged();
return this;
}
/**
*
* / Supported Book Types
*
*
* repeated .org.openfeed.InstrumentDefinition.BookType supportBookTypes = 3;
* @param value The supportBookTypes to add.
* @return This builder for chaining.
*/
public Builder addSupportBookTypes(org.openfeed.InstrumentDefinition.BookType value) {
if (value == null) {
throw new NullPointerException();
}
ensureSupportBookTypesIsMutable();
supportBookTypes_.addInt(value.getNumber());
onChanged();
return this;
}
/**
*
* / Supported Book Types
*
*
* repeated .org.openfeed.InstrumentDefinition.BookType supportBookTypes = 3;
* @param values The supportBookTypes to add.
* @return This builder for chaining.
*/
public Builder addAllSupportBookTypes(
java.lang.Iterable extends org.openfeed.InstrumentDefinition.BookType> values) {
ensureSupportBookTypesIsMutable();
for (org.openfeed.InstrumentDefinition.BookType value : values) {
supportBookTypes_.addInt(value.getNumber());
}
onChanged();
return this;
}
/**
*
* / Supported Book Types
*
*
* repeated .org.openfeed.InstrumentDefinition.BookType supportBookTypes = 3;
* @return This builder for chaining.
*/
public Builder clearSupportBookTypes() {
supportBookTypes_ = emptyIntList();
bitField0_ = (bitField0_ & ~0x00000004);
onChanged();
return this;
}
/**
*
* / Supported Book Types
*
*
* repeated .org.openfeed.InstrumentDefinition.BookType supportBookTypes = 3;
* @return A list containing the enum numeric values on the wire for supportBookTypes.
*/
public java.util.List
getSupportBookTypesValueList() {
return java.util.Collections.unmodifiableList(supportBookTypes_);
}
/**
*
* / Supported Book Types
*
*
* repeated .org.openfeed.InstrumentDefinition.BookType supportBookTypes = 3;
* @param index The index of the value to return.
* @return The enum numeric value on the wire of supportBookTypes at the given index.
*/
public int getSupportBookTypesValue(int index) {
return supportBookTypes_.getInt(index);
}
/**
*
* / Supported Book Types
*
*
* repeated .org.openfeed.InstrumentDefinition.BookType supportBookTypes = 3;
* @param index The index to set the value at.
* @param value The enum numeric value on the wire for supportBookTypes to set.
* @return This builder for chaining.
*/
public Builder setSupportBookTypesValue(
int index, int value) {
ensureSupportBookTypesIsMutable();
supportBookTypes_.setInt(index, value);
onChanged();
return this;
}
/**
*
* / Supported Book Types
*
*
* repeated .org.openfeed.InstrumentDefinition.BookType supportBookTypes = 3;
* @param value The enum numeric value on the wire for supportBookTypes to add.
* @return This builder for chaining.
*/
public Builder addSupportBookTypesValue(int value) {
ensureSupportBookTypesIsMutable();
supportBookTypes_.addInt(value);
onChanged();
return this;
}
/**
*
* / Supported Book Types
*
*
* repeated .org.openfeed.InstrumentDefinition.BookType supportBookTypes = 3;
* @param values The enum numeric values on the wire for supportBookTypes to add.
* @return This builder for chaining.
*/
public Builder addAllSupportBookTypesValue(
java.lang.Iterable values) {
ensureSupportBookTypesIsMutable();
for (int value : values) {
supportBookTypes_.addInt(value);
}
onChanged();
return this;
}
private int bookDepth_ ;
/**
*
* / Maximum depth of market-by-price order book
*
*
* sint32 bookDepth = 4;
* @return The bookDepth.
*/
@java.lang.Override
public int getBookDepth() {
return bookDepth_;
}
/**
*
* / Maximum depth of market-by-price order book
*
*
* sint32 bookDepth = 4;
* @param value The bookDepth to set.
* @return This builder for chaining.
*/
public Builder setBookDepth(int value) {
bookDepth_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
* / Maximum depth of market-by-price order book
*
*
* sint32 bookDepth = 4;
* @return This builder for chaining.
*/
public Builder clearBookDepth() {
bitField0_ = (bitField0_ & ~0x00000008);
bookDepth_ = 0;
onChanged();
return this;
}
private java.lang.Object vendorId_ = "";
/**
*
* / The name of the market data vendor
*
*
* string vendorId = 5;
* @return The vendorId.
*/
public java.lang.String getVendorId() {
java.lang.Object ref = vendorId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
vendorId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* / The name of the market data vendor
*
*
* string vendorId = 5;
* @return The bytes for vendorId.
*/
public com.google.protobuf.ByteString
getVendorIdBytes() {
java.lang.Object ref = vendorId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
vendorId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* / The name of the market data vendor
*
*
* string vendorId = 5;
* @param value The vendorId to set.
* @return This builder for chaining.
*/
public Builder setVendorId(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
vendorId_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
*
* / The name of the market data vendor
*
*
* string vendorId = 5;
* @return This builder for chaining.
*/
public Builder clearVendorId() {
vendorId_ = getDefaultInstance().getVendorId();
bitField0_ = (bitField0_ & ~0x00000010);
onChanged();
return this;
}
/**
*
* / The name of the market data vendor
*
*
* string vendorId = 5;
* @param value The bytes for vendorId to set.
* @return This builder for chaining.
*/
public Builder setVendorIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
vendorId_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
private java.lang.Object symbol_ = "";
/**
*
* / Human readable market symbol, assigned by the exchange or venue.
* Not necessarily unique as the exchange or vendor could assign the same symbol to different
* instruments, for example if the instruments trade on different exchanges.
*
*
* string symbol = 6;
* @return The symbol.
*/
public java.lang.String getSymbol() {
java.lang.Object ref = symbol_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
symbol_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* / Human readable market symbol, assigned by the exchange or venue.
* Not necessarily unique as the exchange or vendor could assign the same symbol to different
* instruments, for example if the instruments trade on different exchanges.
*
*
* string symbol = 6;
* @return The bytes for symbol.
*/
public com.google.protobuf.ByteString
getSymbolBytes() {
java.lang.Object ref = symbol_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
symbol_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* / Human readable market symbol, assigned by the exchange or venue.
* Not necessarily unique as the exchange or vendor could assign the same symbol to different
* instruments, for example if the instruments trade on different exchanges.
*
*
* string symbol = 6;
* @param value The symbol to set.
* @return This builder for chaining.
*/
public Builder setSymbol(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
symbol_ = value;
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
*
* / Human readable market symbol, assigned by the exchange or venue.
* Not necessarily unique as the exchange or vendor could assign the same symbol to different
* instruments, for example if the instruments trade on different exchanges.
*
*
* string symbol = 6;
* @return This builder for chaining.
*/
public Builder clearSymbol() {
symbol_ = getDefaultInstance().getSymbol();
bitField0_ = (bitField0_ & ~0x00000020);
onChanged();
return this;
}
/**
*
* / Human readable market symbol, assigned by the exchange or venue.
* Not necessarily unique as the exchange or vendor could assign the same symbol to different
* instruments, for example if the instruments trade on different exchanges.
*
*
* string symbol = 6;
* @param value The bytes for symbol to set.
* @return This builder for chaining.
*/
public Builder setSymbolBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
symbol_ = value;
bitField0_ |= 0x00000020;
onChanged();
return this;
}
private java.lang.Object description_ = "";
/**
*
* / Human readable market description.
*
*
* string description = 7;
* @return The description.
*/
public java.lang.String getDescription() {
java.lang.Object ref = description_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
description_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* / Human readable market description.
*
*
* string description = 7;
* @return The bytes for description.
*/
public com.google.protobuf.ByteString
getDescriptionBytes() {
java.lang.Object ref = description_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
description_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* / Human readable market description.
*
*
* string description = 7;
* @param value The description to set.
* @return This builder for chaining.
*/
public Builder setDescription(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
description_ = value;
bitField0_ |= 0x00000040;
onChanged();
return this;
}
/**
*
* / Human readable market description.
*
*
* string description = 7;
* @return This builder for chaining.
*/
public Builder clearDescription() {
description_ = getDefaultInstance().getDescription();
bitField0_ = (bitField0_ & ~0x00000040);
onChanged();
return this;
}
/**
*
* / Human readable market description.
*
*
* string description = 7;
* @param value The bytes for description to set.
* @return This builder for chaining.
*/
public Builder setDescriptionBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
description_ = value;
bitField0_ |= 0x00000040;
onChanged();
return this;
}
private java.lang.Object cfiCode_ = "";
/**
*
* / Market CFI code: http://en.wikipedia.org/wiki/ISO_10962
*
*
* string cfiCode = 8;
* @return The cfiCode.
*/
public java.lang.String getCfiCode() {
java.lang.Object ref = cfiCode_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
cfiCode_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* / Market CFI code: http://en.wikipedia.org/wiki/ISO_10962
*
*
* string cfiCode = 8;
* @return The bytes for cfiCode.
*/
public com.google.protobuf.ByteString
getCfiCodeBytes() {
java.lang.Object ref = cfiCode_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
cfiCode_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* / Market CFI code: http://en.wikipedia.org/wiki/ISO_10962
*
*
* string cfiCode = 8;
* @param value The cfiCode to set.
* @return This builder for chaining.
*/
public Builder setCfiCode(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
cfiCode_ = value;
bitField0_ |= 0x00000080;
onChanged();
return this;
}
/**
*
* / Market CFI code: http://en.wikipedia.org/wiki/ISO_10962
*
*
* string cfiCode = 8;
* @return This builder for chaining.
*/
public Builder clearCfiCode() {
cfiCode_ = getDefaultInstance().getCfiCode();
bitField0_ = (bitField0_ & ~0x00000080);
onChanged();
return this;
}
/**
*
* / Market CFI code: http://en.wikipedia.org/wiki/ISO_10962
*
*
* string cfiCode = 8;
* @param value The bytes for cfiCode to set.
* @return This builder for chaining.
*/
public Builder setCfiCodeBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
cfiCode_ = value;
bitField0_ |= 0x00000080;
onChanged();
return this;
}
private java.lang.Object currencyCode_ = "";
/**
*
* / Market currency code: http://en.wikipedia.org/wiki/ISO_4217
*
*
* string currencyCode = 9;
* @return The currencyCode.
*/
public java.lang.String getCurrencyCode() {
java.lang.Object ref = currencyCode_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
currencyCode_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* / Market currency code: http://en.wikipedia.org/wiki/ISO_4217
*
*
* string currencyCode = 9;
* @return The bytes for currencyCode.
*/
public com.google.protobuf.ByteString
getCurrencyCodeBytes() {
java.lang.Object ref = currencyCode_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
currencyCode_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* / Market currency code: http://en.wikipedia.org/wiki/ISO_4217
*
*
* string currencyCode = 9;
* @param value The currencyCode to set.
* @return This builder for chaining.
*/
public Builder setCurrencyCode(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
currencyCode_ = value;
bitField0_ |= 0x00000100;
onChanged();
return this;
}
/**
*
* / Market currency code: http://en.wikipedia.org/wiki/ISO_4217
*
*
* string currencyCode = 9;
* @return This builder for chaining.
*/
public Builder clearCurrencyCode() {
currencyCode_ = getDefaultInstance().getCurrencyCode();
bitField0_ = (bitField0_ & ~0x00000100);
onChanged();
return this;
}
/**
*
* / Market currency code: http://en.wikipedia.org/wiki/ISO_4217
*
*
* string currencyCode = 9;
* @param value The bytes for currencyCode to set.
* @return This builder for chaining.
*/
public Builder setCurrencyCodeBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
currencyCode_ = value;
bitField0_ |= 0x00000100;
onChanged();
return this;
}
private java.lang.Object exchangeCode_ = "";
/**
*
* Market exchange code: http://en.wikipedia.org/wiki/ISO_10383
* For inter-exchange spreads, use the leg MICs separated by a hyphen
*
*
* string exchangeCode = 10;
* @return The exchangeCode.
*/
public java.lang.String getExchangeCode() {
java.lang.Object ref = exchangeCode_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
exchangeCode_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* Market exchange code: http://en.wikipedia.org/wiki/ISO_10383
* For inter-exchange spreads, use the leg MICs separated by a hyphen
*
*
* string exchangeCode = 10;
* @return The bytes for exchangeCode.
*/
public com.google.protobuf.ByteString
getExchangeCodeBytes() {
java.lang.Object ref = exchangeCode_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
exchangeCode_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* Market exchange code: http://en.wikipedia.org/wiki/ISO_10383
* For inter-exchange spreads, use the leg MICs separated by a hyphen
*
*
* string exchangeCode = 10;
* @param value The exchangeCode to set.
* @return This builder for chaining.
*/
public Builder setExchangeCode(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
exchangeCode_ = value;
bitField0_ |= 0x00000200;
onChanged();
return this;
}
/**
*
* Market exchange code: http://en.wikipedia.org/wiki/ISO_10383
* For inter-exchange spreads, use the leg MICs separated by a hyphen
*
*
* string exchangeCode = 10;
* @return This builder for chaining.
*/
public Builder clearExchangeCode() {
exchangeCode_ = getDefaultInstance().getExchangeCode();
bitField0_ = (bitField0_ & ~0x00000200);
onChanged();
return this;
}
/**
*
* Market exchange code: http://en.wikipedia.org/wiki/ISO_10383
* For inter-exchange spreads, use the leg MICs separated by a hyphen
*
*
* string exchangeCode = 10;
* @param value The bytes for exchangeCode to set.
* @return This builder for chaining.
*/
public Builder setExchangeCodeBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
exchangeCode_ = value;
bitField0_ |= 0x00000200;
onChanged();
return this;
}
private float minimumPriceIncrement_ ;
/**
*
* / Minimum price increment in market currency.
*
*
* float minimumPriceIncrement = 11;
* @return The minimumPriceIncrement.
*/
@java.lang.Override
public float getMinimumPriceIncrement() {
return minimumPriceIncrement_;
}
/**
*
* / Minimum price increment in market currency.
*
*
* float minimumPriceIncrement = 11;
* @param value The minimumPriceIncrement to set.
* @return This builder for chaining.
*/
public Builder setMinimumPriceIncrement(float value) {
minimumPriceIncrement_ = value;
bitField0_ |= 0x00000400;
onChanged();
return this;
}
/**
*
* / Minimum price increment in market currency.
*
*
* float minimumPriceIncrement = 11;
* @return This builder for chaining.
*/
public Builder clearMinimumPriceIncrement() {
bitField0_ = (bitField0_ & ~0x00000400);
minimumPriceIncrement_ = 0F;
onChanged();
return this;
}
private float contractPointValue_ ;
/**
*
* / Contract point value in market currency.
*
*
* float contractPointValue = 12;
* @return The contractPointValue.
*/
@java.lang.Override
public float getContractPointValue() {
return contractPointValue_;
}
/**
*
* / Contract point value in market currency.
*
*
* float contractPointValue = 12;
* @param value The contractPointValue to set.
* @return This builder for chaining.
*/
public Builder setContractPointValue(float value) {
contractPointValue_ = value;
bitField0_ |= 0x00000800;
onChanged();
return this;
}
/**
*
* / Contract point value in market currency.
*
*
* float contractPointValue = 12;
* @return This builder for chaining.
*/
public Builder clearContractPointValue() {
bitField0_ = (bitField0_ & ~0x00000800);
contractPointValue_ = 0F;
onChanged();
return this;
}
private org.openfeed.InstrumentDefinition.Schedule schedule_;
private com.google.protobuf.SingleFieldBuilder<
org.openfeed.InstrumentDefinition.Schedule, org.openfeed.InstrumentDefinition.Schedule.Builder, org.openfeed.InstrumentDefinition.ScheduleOrBuilder> scheduleBuilder_;
/**
*
* / Trading schedule for a typical week
*
*
* .org.openfeed.InstrumentDefinition.Schedule schedule = 13;
* @return Whether the schedule field is set.
*/
public boolean hasSchedule() {
return ((bitField0_ & 0x00001000) != 0);
}
/**
*
* / Trading schedule for a typical week
*
*
* .org.openfeed.InstrumentDefinition.Schedule schedule = 13;
* @return The schedule.
*/
public org.openfeed.InstrumentDefinition.Schedule getSchedule() {
if (scheduleBuilder_ == null) {
return schedule_ == null ? org.openfeed.InstrumentDefinition.Schedule.getDefaultInstance() : schedule_;
} else {
return scheduleBuilder_.getMessage();
}
}
/**
*
* / Trading schedule for a typical week
*
*
* .org.openfeed.InstrumentDefinition.Schedule schedule = 13;
*/
public Builder setSchedule(org.openfeed.InstrumentDefinition.Schedule value) {
if (scheduleBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
schedule_ = value;
} else {
scheduleBuilder_.setMessage(value);
}
bitField0_ |= 0x00001000;
onChanged();
return this;
}
/**
*
* / Trading schedule for a typical week
*
*
* .org.openfeed.InstrumentDefinition.Schedule schedule = 13;
*/
public Builder setSchedule(
org.openfeed.InstrumentDefinition.Schedule.Builder builderForValue) {
if (scheduleBuilder_ == null) {
schedule_ = builderForValue.build();
} else {
scheduleBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00001000;
onChanged();
return this;
}
/**
*
* / Trading schedule for a typical week
*
*
* .org.openfeed.InstrumentDefinition.Schedule schedule = 13;
*/
public Builder mergeSchedule(org.openfeed.InstrumentDefinition.Schedule value) {
if (scheduleBuilder_ == null) {
if (((bitField0_ & 0x00001000) != 0) &&
schedule_ != null &&
schedule_ != org.openfeed.InstrumentDefinition.Schedule.getDefaultInstance()) {
getScheduleBuilder().mergeFrom(value);
} else {
schedule_ = value;
}
} else {
scheduleBuilder_.mergeFrom(value);
}
if (schedule_ != null) {
bitField0_ |= 0x00001000;
onChanged();
}
return this;
}
/**
*
* / Trading schedule for a typical week
*
*
* .org.openfeed.InstrumentDefinition.Schedule schedule = 13;
*/
public Builder clearSchedule() {
bitField0_ = (bitField0_ & ~0x00001000);
schedule_ = null;
if (scheduleBuilder_ != null) {
scheduleBuilder_.dispose();
scheduleBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* / Trading schedule for a typical week
*
*
* .org.openfeed.InstrumentDefinition.Schedule schedule = 13;
*/
public org.openfeed.InstrumentDefinition.Schedule.Builder getScheduleBuilder() {
bitField0_ |= 0x00001000;
onChanged();
return getScheduleFieldBuilder().getBuilder();
}
/**
*
* / Trading schedule for a typical week
*
*
* .org.openfeed.InstrumentDefinition.Schedule schedule = 13;
*/
public org.openfeed.InstrumentDefinition.ScheduleOrBuilder getScheduleOrBuilder() {
if (scheduleBuilder_ != null) {
return scheduleBuilder_.getMessageOrBuilder();
} else {
return schedule_ == null ?
org.openfeed.InstrumentDefinition.Schedule.getDefaultInstance() : schedule_;
}
}
/**
*
* / Trading schedule for a typical week
*
*
* .org.openfeed.InstrumentDefinition.Schedule schedule = 13;
*/
private com.google.protobuf.SingleFieldBuilder<
org.openfeed.InstrumentDefinition.Schedule, org.openfeed.InstrumentDefinition.Schedule.Builder, org.openfeed.InstrumentDefinition.ScheduleOrBuilder>
getScheduleFieldBuilder() {
if (scheduleBuilder_ == null) {
scheduleBuilder_ = new com.google.protobuf.SingleFieldBuilder<
org.openfeed.InstrumentDefinition.Schedule, org.openfeed.InstrumentDefinition.Schedule.Builder, org.openfeed.InstrumentDefinition.ScheduleOrBuilder>(
getSchedule(),
getParentForChildren(),
isClean());
schedule_ = null;
}
return scheduleBuilder_;
}
private org.openfeed.InstrumentDefinition.Calendar calendar_;
private com.google.protobuf.SingleFieldBuilder<
org.openfeed.InstrumentDefinition.Calendar, org.openfeed.InstrumentDefinition.Calendar.Builder, org.openfeed.InstrumentDefinition.CalendarOrBuilder> calendarBuilder_;
/**
*
* / Trading calendar (expiration, notice days, holidays?, etc)
*
*
* .org.openfeed.InstrumentDefinition.Calendar calendar = 14;
* @return Whether the calendar field is set.
*/
public boolean hasCalendar() {
return ((bitField0_ & 0x00002000) != 0);
}
/**
*
* / Trading calendar (expiration, notice days, holidays?, etc)
*
*
* .org.openfeed.InstrumentDefinition.Calendar calendar = 14;
* @return The calendar.
*/
public org.openfeed.InstrumentDefinition.Calendar getCalendar() {
if (calendarBuilder_ == null) {
return calendar_ == null ? org.openfeed.InstrumentDefinition.Calendar.getDefaultInstance() : calendar_;
} else {
return calendarBuilder_.getMessage();
}
}
/**
*
* / Trading calendar (expiration, notice days, holidays?, etc)
*
*
* .org.openfeed.InstrumentDefinition.Calendar calendar = 14;
*/
public Builder setCalendar(org.openfeed.InstrumentDefinition.Calendar value) {
if (calendarBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
calendar_ = value;
} else {
calendarBuilder_.setMessage(value);
}
bitField0_ |= 0x00002000;
onChanged();
return this;
}
/**
*
* / Trading calendar (expiration, notice days, holidays?, etc)
*
*
* .org.openfeed.InstrumentDefinition.Calendar calendar = 14;
*/
public Builder setCalendar(
org.openfeed.InstrumentDefinition.Calendar.Builder builderForValue) {
if (calendarBuilder_ == null) {
calendar_ = builderForValue.build();
} else {
calendarBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00002000;
onChanged();
return this;
}
/**
*
* / Trading calendar (expiration, notice days, holidays?, etc)
*
*
* .org.openfeed.InstrumentDefinition.Calendar calendar = 14;
*/
public Builder mergeCalendar(org.openfeed.InstrumentDefinition.Calendar value) {
if (calendarBuilder_ == null) {
if (((bitField0_ & 0x00002000) != 0) &&
calendar_ != null &&
calendar_ != org.openfeed.InstrumentDefinition.Calendar.getDefaultInstance()) {
getCalendarBuilder().mergeFrom(value);
} else {
calendar_ = value;
}
} else {
calendarBuilder_.mergeFrom(value);
}
if (calendar_ != null) {
bitField0_ |= 0x00002000;
onChanged();
}
return this;
}
/**
*
* / Trading calendar (expiration, notice days, holidays?, etc)
*
*
* .org.openfeed.InstrumentDefinition.Calendar calendar = 14;
*/
public Builder clearCalendar() {
bitField0_ = (bitField0_ & ~0x00002000);
calendar_ = null;
if (calendarBuilder_ != null) {
calendarBuilder_.dispose();
calendarBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* / Trading calendar (expiration, notice days, holidays?, etc)
*
*
* .org.openfeed.InstrumentDefinition.Calendar calendar = 14;
*/
public org.openfeed.InstrumentDefinition.Calendar.Builder getCalendarBuilder() {
bitField0_ |= 0x00002000;
onChanged();
return getCalendarFieldBuilder().getBuilder();
}
/**
*
* / Trading calendar (expiration, notice days, holidays?, etc)
*
*
* .org.openfeed.InstrumentDefinition.Calendar calendar = 14;
*/
public org.openfeed.InstrumentDefinition.CalendarOrBuilder getCalendarOrBuilder() {
if (calendarBuilder_ != null) {
return calendarBuilder_.getMessageOrBuilder();
} else {
return calendar_ == null ?
org.openfeed.InstrumentDefinition.Calendar.getDefaultInstance() : calendar_;
}
}
/**
*
* / Trading calendar (expiration, notice days, holidays?, etc)
*
*
* .org.openfeed.InstrumentDefinition.Calendar calendar = 14;
*/
private com.google.protobuf.SingleFieldBuilder<
org.openfeed.InstrumentDefinition.Calendar, org.openfeed.InstrumentDefinition.Calendar.Builder, org.openfeed.InstrumentDefinition.CalendarOrBuilder>
getCalendarFieldBuilder() {
if (calendarBuilder_ == null) {
calendarBuilder_ = new com.google.protobuf.SingleFieldBuilder<
org.openfeed.InstrumentDefinition.Calendar, org.openfeed.InstrumentDefinition.Calendar.Builder, org.openfeed.InstrumentDefinition.CalendarOrBuilder>(
getCalendar(),
getParentForChildren(),
isClean());
calendar_ = null;
}
return calendarBuilder_;
}
private long recordCreateTime_ ;
/**
*
* / UTC Timestamp of creation, nano seconds since Unix epoch
*
*
* sint64 recordCreateTime = 15;
* @return The recordCreateTime.
*/
@java.lang.Override
public long getRecordCreateTime() {
return recordCreateTime_;
}
/**
*
* / UTC Timestamp of creation, nano seconds since Unix epoch
*
*
* sint64 recordCreateTime = 15;
* @param value The recordCreateTime to set.
* @return This builder for chaining.
*/
public Builder setRecordCreateTime(long value) {
recordCreateTime_ = value;
bitField0_ |= 0x00004000;
onChanged();
return this;
}
/**
*
* / UTC Timestamp of creation, nano seconds since Unix epoch
*
*
* sint64 recordCreateTime = 15;
* @return This builder for chaining.
*/
public Builder clearRecordCreateTime() {
bitField0_ = (bitField0_ & ~0x00004000);
recordCreateTime_ = 0L;
onChanged();
return this;
}
private long recordUpdateTime_ ;
/**
*
* / UTC Timestamp of update, nano seconds since Unix epoch
*
*
* sint64 recordUpdateTime = 16;
* @return The recordUpdateTime.
*/
@java.lang.Override
public long getRecordUpdateTime() {
return recordUpdateTime_;
}
/**
*
* / UTC Timestamp of update, nano seconds since Unix epoch
*
*
* sint64 recordUpdateTime = 16;
* @param value The recordUpdateTime to set.
* @return This builder for chaining.
*/
public Builder setRecordUpdateTime(long value) {
recordUpdateTime_ = value;
bitField0_ |= 0x00008000;
onChanged();
return this;
}
/**
*
* / UTC Timestamp of update, nano seconds since Unix epoch
*
*
* sint64 recordUpdateTime = 16;
* @return This builder for chaining.
*/
public Builder clearRecordUpdateTime() {
bitField0_ = (bitField0_ & ~0x00008000);
recordUpdateTime_ = 0L;
onChanged();
return this;
}
private java.lang.Object timeZoneName_ = "";
/**
*
* / Market time zone TZ database name.
* Permanent. Can be resolved into timeZoneOffset for given date/time.
* See http://joda-time.sourceforge.net/timezones.html
* See http://en.wikipedia.org/wiki/List_of_tz_database_time_zones
*
*
* string timeZoneName = 17;
* @return The timeZoneName.
*/
public java.lang.String getTimeZoneName() {
java.lang.Object ref = timeZoneName_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
timeZoneName_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* / Market time zone TZ database name.
* Permanent. Can be resolved into timeZoneOffset for given date/time.
* See http://joda-time.sourceforge.net/timezones.html
* See http://en.wikipedia.org/wiki/List_of_tz_database_time_zones
*
*
* string timeZoneName = 17;
* @return The bytes for timeZoneName.
*/
public com.google.protobuf.ByteString
getTimeZoneNameBytes() {
java.lang.Object ref = timeZoneName_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
timeZoneName_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* / Market time zone TZ database name.
* Permanent. Can be resolved into timeZoneOffset for given date/time.
* See http://joda-time.sourceforge.net/timezones.html
* See http://en.wikipedia.org/wiki/List_of_tz_database_time_zones
*
*
* string timeZoneName = 17;
* @param value The timeZoneName to set.
* @return This builder for chaining.
*/
public Builder setTimeZoneName(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
timeZoneName_ = value;
bitField0_ |= 0x00010000;
onChanged();
return this;
}
/**
*
* / Market time zone TZ database name.
* Permanent. Can be resolved into timeZoneOffset for given date/time.
* See http://joda-time.sourceforge.net/timezones.html
* See http://en.wikipedia.org/wiki/List_of_tz_database_time_zones
*
*
* string timeZoneName = 17;
* @return This builder for chaining.
*/
public Builder clearTimeZoneName() {
timeZoneName_ = getDefaultInstance().getTimeZoneName();
bitField0_ = (bitField0_ & ~0x00010000);
onChanged();
return this;
}
/**
*
* / Market time zone TZ database name.
* Permanent. Can be resolved into timeZoneOffset for given date/time.
* See http://joda-time.sourceforge.net/timezones.html
* See http://en.wikipedia.org/wiki/List_of_tz_database_time_zones
*
*
* string timeZoneName = 17;
* @param value The bytes for timeZoneName to set.
* @return This builder for chaining.
*/
public Builder setTimeZoneNameBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
timeZoneName_ = value;
bitField0_ |= 0x00010000;
onChanged();
return this;
}
private java.lang.Object instrumentGroup_ = "";
/**
*
* / Identifies a logical grouping of instruments. By product, for example.
*
*
* string instrumentGroup = 18;
* @return The instrumentGroup.
*/
public java.lang.String getInstrumentGroup() {
java.lang.Object ref = instrumentGroup_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
instrumentGroup_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* / Identifies a logical grouping of instruments. By product, for example.
*
*
* string instrumentGroup = 18;
* @return The bytes for instrumentGroup.
*/
public com.google.protobuf.ByteString
getInstrumentGroupBytes() {
java.lang.Object ref = instrumentGroup_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
instrumentGroup_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* / Identifies a logical grouping of instruments. By product, for example.
*
*
* string instrumentGroup = 18;
* @param value The instrumentGroup to set.
* @return This builder for chaining.
*/
public Builder setInstrumentGroup(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
instrumentGroup_ = value;
bitField0_ |= 0x00020000;
onChanged();
return this;
}
/**
*
* / Identifies a logical grouping of instruments. By product, for example.
*
*
* string instrumentGroup = 18;
* @return This builder for chaining.
*/
public Builder clearInstrumentGroup() {
instrumentGroup_ = getDefaultInstance().getInstrumentGroup();
bitField0_ = (bitField0_ & ~0x00020000);
onChanged();
return this;
}
/**
*
* / Identifies a logical grouping of instruments. By product, for example.
*
*
* string instrumentGroup = 18;
* @param value The bytes for instrumentGroup to set.
* @return This builder for chaining.
*/
public Builder setInstrumentGroupBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
instrumentGroup_ = value;
bitField0_ |= 0x00020000;
onChanged();
return this;
}
private org.openfeed.InstrumentDefinition.MaturityDate symbolExpiration_;
private com.google.protobuf.SingleFieldBuilder<
org.openfeed.InstrumentDefinition.MaturityDate, org.openfeed.InstrumentDefinition.MaturityDate.Builder, org.openfeed.InstrumentDefinition.MaturityDateOrBuilder> symbolExpirationBuilder_;
/**
*
* / The Date of expiration for futures and options.
*
*
* .org.openfeed.InstrumentDefinition.MaturityDate symbolExpiration = 19;
* @return Whether the symbolExpiration field is set.
*/
public boolean hasSymbolExpiration() {
return ((bitField0_ & 0x00040000) != 0);
}
/**
*
* / The Date of expiration for futures and options.
*
*
* .org.openfeed.InstrumentDefinition.MaturityDate symbolExpiration = 19;
* @return The symbolExpiration.
*/
public org.openfeed.InstrumentDefinition.MaturityDate getSymbolExpiration() {
if (symbolExpirationBuilder_ == null) {
return symbolExpiration_ == null ? org.openfeed.InstrumentDefinition.MaturityDate.getDefaultInstance() : symbolExpiration_;
} else {
return symbolExpirationBuilder_.getMessage();
}
}
/**
*
* / The Date of expiration for futures and options.
*
*
* .org.openfeed.InstrumentDefinition.MaturityDate symbolExpiration = 19;
*/
public Builder setSymbolExpiration(org.openfeed.InstrumentDefinition.MaturityDate value) {
if (symbolExpirationBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
symbolExpiration_ = value;
} else {
symbolExpirationBuilder_.setMessage(value);
}
bitField0_ |= 0x00040000;
onChanged();
return this;
}
/**
*
* / The Date of expiration for futures and options.
*
*
* .org.openfeed.InstrumentDefinition.MaturityDate symbolExpiration = 19;
*/
public Builder setSymbolExpiration(
org.openfeed.InstrumentDefinition.MaturityDate.Builder builderForValue) {
if (symbolExpirationBuilder_ == null) {
symbolExpiration_ = builderForValue.build();
} else {
symbolExpirationBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00040000;
onChanged();
return this;
}
/**
*
* / The Date of expiration for futures and options.
*
*
* .org.openfeed.InstrumentDefinition.MaturityDate symbolExpiration = 19;
*/
public Builder mergeSymbolExpiration(org.openfeed.InstrumentDefinition.MaturityDate value) {
if (symbolExpirationBuilder_ == null) {
if (((bitField0_ & 0x00040000) != 0) &&
symbolExpiration_ != null &&
symbolExpiration_ != org.openfeed.InstrumentDefinition.MaturityDate.getDefaultInstance()) {
getSymbolExpirationBuilder().mergeFrom(value);
} else {
symbolExpiration_ = value;
}
} else {
symbolExpirationBuilder_.mergeFrom(value);
}
if (symbolExpiration_ != null) {
bitField0_ |= 0x00040000;
onChanged();
}
return this;
}
/**
*
* / The Date of expiration for futures and options.
*
*
* .org.openfeed.InstrumentDefinition.MaturityDate symbolExpiration = 19;
*/
public Builder clearSymbolExpiration() {
bitField0_ = (bitField0_ & ~0x00040000);
symbolExpiration_ = null;
if (symbolExpirationBuilder_ != null) {
symbolExpirationBuilder_.dispose();
symbolExpirationBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* / The Date of expiration for futures and options.
*
*
* .org.openfeed.InstrumentDefinition.MaturityDate symbolExpiration = 19;
*/
public org.openfeed.InstrumentDefinition.MaturityDate.Builder getSymbolExpirationBuilder() {
bitField0_ |= 0x00040000;
onChanged();
return getSymbolExpirationFieldBuilder().getBuilder();
}
/**
*
* / The Date of expiration for futures and options.
*
*
* .org.openfeed.InstrumentDefinition.MaturityDate symbolExpiration = 19;
*/
public org.openfeed.InstrumentDefinition.MaturityDateOrBuilder getSymbolExpirationOrBuilder() {
if (symbolExpirationBuilder_ != null) {
return symbolExpirationBuilder_.getMessageOrBuilder();
} else {
return symbolExpiration_ == null ?
org.openfeed.InstrumentDefinition.MaturityDate.getDefaultInstance() : symbolExpiration_;
}
}
/**
*
* / The Date of expiration for futures and options.
*
*
* .org.openfeed.InstrumentDefinition.MaturityDate symbolExpiration = 19;
*/
private com.google.protobuf.SingleFieldBuilder<
org.openfeed.InstrumentDefinition.MaturityDate, org.openfeed.InstrumentDefinition.MaturityDate.Builder, org.openfeed.InstrumentDefinition.MaturityDateOrBuilder>
getSymbolExpirationFieldBuilder() {
if (symbolExpirationBuilder_ == null) {
symbolExpirationBuilder_ = new com.google.protobuf.SingleFieldBuilder<
org.openfeed.InstrumentDefinition.MaturityDate, org.openfeed.InstrumentDefinition.MaturityDate.Builder, org.openfeed.InstrumentDefinition.MaturityDateOrBuilder>(
getSymbolExpiration(),
getParentForChildren(),
isClean());
symbolExpiration_ = null;
}
return symbolExpirationBuilder_;
}
private int state_ = 0;
/**
*
* / active: can have market state updates, can have historical data
* passive: can NOT have market state updates, but can have historical data
* normally "active" means newly listed or currently non expired markets
* normally "passive" means expired options, de-listed equities, etc.
*
*
* .org.openfeed.InstrumentDefinition.State state = 20;
* @return The enum numeric value on the wire for state.
*/
@java.lang.Override public int getStateValue() {
return state_;
}
/**
*
* / active: can have market state updates, can have historical data
* passive: can NOT have market state updates, but can have historical data
* normally "active" means newly listed or currently non expired markets
* normally "passive" means expired options, de-listed equities, etc.
*
*
* .org.openfeed.InstrumentDefinition.State state = 20;
* @param value The enum numeric value on the wire for state to set.
* @return This builder for chaining.
*/
public Builder setStateValue(int value) {
state_ = value;
bitField0_ |= 0x00080000;
onChanged();
return this;
}
/**
*
* / active: can have market state updates, can have historical data
* passive: can NOT have market state updates, but can have historical data
* normally "active" means newly listed or currently non expired markets
* normally "passive" means expired options, de-listed equities, etc.
*
*
* .org.openfeed.InstrumentDefinition.State state = 20;
* @return The state.
*/
@java.lang.Override
public org.openfeed.InstrumentDefinition.State getState() {
org.openfeed.InstrumentDefinition.State result = org.openfeed.InstrumentDefinition.State.forNumber(state_);
return result == null ? org.openfeed.InstrumentDefinition.State.UNRECOGNIZED : result;
}
/**
*
* / active: can have market state updates, can have historical data
* passive: can NOT have market state updates, but can have historical data
* normally "active" means newly listed or currently non expired markets
* normally "passive" means expired options, de-listed equities, etc.
*
*
* .org.openfeed.InstrumentDefinition.State state = 20;
* @param value The state to set.
* @return This builder for chaining.
*/
public Builder setState(org.openfeed.InstrumentDefinition.State value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00080000;
state_ = value.getNumber();
onChanged();
return this;
}
/**
*
* / active: can have market state updates, can have historical data
* passive: can NOT have market state updates, but can have historical data
* normally "active" means newly listed or currently non expired markets
* normally "passive" means expired options, de-listed equities, etc.
*
*
* .org.openfeed.InstrumentDefinition.State state = 20;
* @return This builder for chaining.
*/
public Builder clearState() {
bitField0_ = (bitField0_ & ~0x00080000);
state_ = 0;
onChanged();
return this;
}
private int channel_ ;
/**
*
* / The channel that updates for this instrument will appear on.
*
*
* sint32 channel = 21;
* @return The channel.
*/
@java.lang.Override
public int getChannel() {
return channel_;
}
/**
*
* / The channel that updates for this instrument will appear on.
*
*
* sint32 channel = 21;
* @param value The channel to set.
* @return This builder for chaining.
*/
public Builder setChannel(int value) {
channel_ = value;
bitField0_ |= 0x00100000;
onChanged();
return this;
}
/**
*
* / The channel that updates for this instrument will appear on.
*
*
* sint32 channel = 21;
* @return This builder for chaining.
*/
public Builder clearChannel() {
bitField0_ = (bitField0_ & ~0x00100000);
channel_ = 0;
onChanged();
return this;
}
private long underlyingMarketId_ ;
/**
*
* / The marketId of the underlying asset.
* Used by Futures and Options when the underlying instrument is defined by the vendor
*
*
* sint64 underlyingMarketId = 22;
* @return The underlyingMarketId.
*/
@java.lang.Override
public long getUnderlyingMarketId() {
return underlyingMarketId_;
}
/**
*
* / The marketId of the underlying asset.
* Used by Futures and Options when the underlying instrument is defined by the vendor
*
*
* sint64 underlyingMarketId = 22;
* @param value The underlyingMarketId to set.
* @return This builder for chaining.
*/
public Builder setUnderlyingMarketId(long value) {
underlyingMarketId_ = value;
bitField0_ |= 0x00200000;
onChanged();
return this;
}
/**
*
* / The marketId of the underlying asset.
* Used by Futures and Options when the underlying instrument is defined by the vendor
*
*
* sint64 underlyingMarketId = 22;
* @return This builder for chaining.
*/
public Builder clearUnderlyingMarketId() {
bitField0_ = (bitField0_ & ~0x00200000);
underlyingMarketId_ = 0L;
onChanged();
return this;
}
private org.openfeed.InstrumentDefinition.PriceFormat priceFormat_;
private com.google.protobuf.SingleFieldBuilder<
org.openfeed.InstrumentDefinition.PriceFormat, org.openfeed.InstrumentDefinition.PriceFormat.Builder, org.openfeed.InstrumentDefinition.PriceFormatOrBuilder> priceFormatBuilder_;
/**
*
* / Display format
*
*
* .org.openfeed.InstrumentDefinition.PriceFormat priceFormat = 23;
* @return Whether the priceFormat field is set.
*/
public boolean hasPriceFormat() {
return ((bitField0_ & 0x00400000) != 0);
}
/**
*
* / Display format
*
*
* .org.openfeed.InstrumentDefinition.PriceFormat priceFormat = 23;
* @return The priceFormat.
*/
public org.openfeed.InstrumentDefinition.PriceFormat getPriceFormat() {
if (priceFormatBuilder_ == null) {
return priceFormat_ == null ? org.openfeed.InstrumentDefinition.PriceFormat.getDefaultInstance() : priceFormat_;
} else {
return priceFormatBuilder_.getMessage();
}
}
/**
*
* / Display format
*
*
* .org.openfeed.InstrumentDefinition.PriceFormat priceFormat = 23;
*/
public Builder setPriceFormat(org.openfeed.InstrumentDefinition.PriceFormat value) {
if (priceFormatBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
priceFormat_ = value;
} else {
priceFormatBuilder_.setMessage(value);
}
bitField0_ |= 0x00400000;
onChanged();
return this;
}
/**
*
* / Display format
*
*
* .org.openfeed.InstrumentDefinition.PriceFormat priceFormat = 23;
*/
public Builder setPriceFormat(
org.openfeed.InstrumentDefinition.PriceFormat.Builder builderForValue) {
if (priceFormatBuilder_ == null) {
priceFormat_ = builderForValue.build();
} else {
priceFormatBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00400000;
onChanged();
return this;
}
/**
*
* / Display format
*
*
* .org.openfeed.InstrumentDefinition.PriceFormat priceFormat = 23;
*/
public Builder mergePriceFormat(org.openfeed.InstrumentDefinition.PriceFormat value) {
if (priceFormatBuilder_ == null) {
if (((bitField0_ & 0x00400000) != 0) &&
priceFormat_ != null &&
priceFormat_ != org.openfeed.InstrumentDefinition.PriceFormat.getDefaultInstance()) {
getPriceFormatBuilder().mergeFrom(value);
} else {
priceFormat_ = value;
}
} else {
priceFormatBuilder_.mergeFrom(value);
}
if (priceFormat_ != null) {
bitField0_ |= 0x00400000;
onChanged();
}
return this;
}
/**
*
* / Display format
*
*
* .org.openfeed.InstrumentDefinition.PriceFormat priceFormat = 23;
*/
public Builder clearPriceFormat() {
bitField0_ = (bitField0_ & ~0x00400000);
priceFormat_ = null;
if (priceFormatBuilder_ != null) {
priceFormatBuilder_.dispose();
priceFormatBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* / Display format
*
*
* .org.openfeed.InstrumentDefinition.PriceFormat priceFormat = 23;
*/
public org.openfeed.InstrumentDefinition.PriceFormat.Builder getPriceFormatBuilder() {
bitField0_ |= 0x00400000;
onChanged();
return getPriceFormatFieldBuilder().getBuilder();
}
/**
*
* / Display format
*
*
* .org.openfeed.InstrumentDefinition.PriceFormat priceFormat = 23;
*/
public org.openfeed.InstrumentDefinition.PriceFormatOrBuilder getPriceFormatOrBuilder() {
if (priceFormatBuilder_ != null) {
return priceFormatBuilder_.getMessageOrBuilder();
} else {
return priceFormat_ == null ?
org.openfeed.InstrumentDefinition.PriceFormat.getDefaultInstance() : priceFormat_;
}
}
/**
*
* / Display format
*
*
* .org.openfeed.InstrumentDefinition.PriceFormat priceFormat = 23;
*/
private com.google.protobuf.SingleFieldBuilder<
org.openfeed.InstrumentDefinition.PriceFormat, org.openfeed.InstrumentDefinition.PriceFormat.Builder, org.openfeed.InstrumentDefinition.PriceFormatOrBuilder>
getPriceFormatFieldBuilder() {
if (priceFormatBuilder_ == null) {
priceFormatBuilder_ = new com.google.protobuf.SingleFieldBuilder<
org.openfeed.InstrumentDefinition.PriceFormat, org.openfeed.InstrumentDefinition.PriceFormat.Builder, org.openfeed.InstrumentDefinition.PriceFormatOrBuilder>(
getPriceFormat(),
getParentForChildren(),
isClean());
priceFormat_ = null;
}
return priceFormatBuilder_;
}
private org.openfeed.InstrumentDefinition.PriceFormat optionStrikePriceFormat_;
private com.google.protobuf.SingleFieldBuilder<
org.openfeed.InstrumentDefinition.PriceFormat, org.openfeed.InstrumentDefinition.PriceFormat.Builder, org.openfeed.InstrumentDefinition.PriceFormatOrBuilder> optionStrikePriceFormatBuilder_;
/**
*
* / Strike price display format
*
*
* .org.openfeed.InstrumentDefinition.PriceFormat optionStrikePriceFormat = 24;
* @return Whether the optionStrikePriceFormat field is set.
*/
public boolean hasOptionStrikePriceFormat() {
return ((bitField0_ & 0x00800000) != 0);
}
/**
*
* / Strike price display format
*
*
* .org.openfeed.InstrumentDefinition.PriceFormat optionStrikePriceFormat = 24;
* @return The optionStrikePriceFormat.
*/
public org.openfeed.InstrumentDefinition.PriceFormat getOptionStrikePriceFormat() {
if (optionStrikePriceFormatBuilder_ == null) {
return optionStrikePriceFormat_ == null ? org.openfeed.InstrumentDefinition.PriceFormat.getDefaultInstance() : optionStrikePriceFormat_;
} else {
return optionStrikePriceFormatBuilder_.getMessage();
}
}
/**
*
* / Strike price display format
*
*
* .org.openfeed.InstrumentDefinition.PriceFormat optionStrikePriceFormat = 24;
*/
public Builder setOptionStrikePriceFormat(org.openfeed.InstrumentDefinition.PriceFormat value) {
if (optionStrikePriceFormatBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
optionStrikePriceFormat_ = value;
} else {
optionStrikePriceFormatBuilder_.setMessage(value);
}
bitField0_ |= 0x00800000;
onChanged();
return this;
}
/**
*
* / Strike price display format
*
*
* .org.openfeed.InstrumentDefinition.PriceFormat optionStrikePriceFormat = 24;
*/
public Builder setOptionStrikePriceFormat(
org.openfeed.InstrumentDefinition.PriceFormat.Builder builderForValue) {
if (optionStrikePriceFormatBuilder_ == null) {
optionStrikePriceFormat_ = builderForValue.build();
} else {
optionStrikePriceFormatBuilder_.setMessage(builderForValue.build());
}
bitField0_ |= 0x00800000;
onChanged();
return this;
}
/**
*
* / Strike price display format
*
*
* .org.openfeed.InstrumentDefinition.PriceFormat optionStrikePriceFormat = 24;
*/
public Builder mergeOptionStrikePriceFormat(org.openfeed.InstrumentDefinition.PriceFormat value) {
if (optionStrikePriceFormatBuilder_ == null) {
if (((bitField0_ & 0x00800000) != 0) &&
optionStrikePriceFormat_ != null &&
optionStrikePriceFormat_ != org.openfeed.InstrumentDefinition.PriceFormat.getDefaultInstance()) {
getOptionStrikePriceFormatBuilder().mergeFrom(value);
} else {
optionStrikePriceFormat_ = value;
}
} else {
optionStrikePriceFormatBuilder_.mergeFrom(value);
}
if (optionStrikePriceFormat_ != null) {
bitField0_ |= 0x00800000;
onChanged();
}
return this;
}
/**
*
* / Strike price display format
*
*
* .org.openfeed.InstrumentDefinition.PriceFormat optionStrikePriceFormat = 24;
*/
public Builder clearOptionStrikePriceFormat() {
bitField0_ = (bitField0_ & ~0x00800000);
optionStrikePriceFormat_ = null;
if (optionStrikePriceFormatBuilder_ != null) {
optionStrikePriceFormatBuilder_.dispose();
optionStrikePriceFormatBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* / Strike price display format
*
*
* .org.openfeed.InstrumentDefinition.PriceFormat optionStrikePriceFormat = 24;
*/
public org.openfeed.InstrumentDefinition.PriceFormat.Builder getOptionStrikePriceFormatBuilder() {
bitField0_ |= 0x00800000;
onChanged();
return getOptionStrikePriceFormatFieldBuilder().getBuilder();
}
/**
*
* / Strike price display format
*
*
* .org.openfeed.InstrumentDefinition.PriceFormat optionStrikePriceFormat = 24;
*/
public org.openfeed.InstrumentDefinition.PriceFormatOrBuilder getOptionStrikePriceFormatOrBuilder() {
if (optionStrikePriceFormatBuilder_ != null) {
return optionStrikePriceFormatBuilder_.getMessageOrBuilder();
} else {
return optionStrikePriceFormat_ == null ?
org.openfeed.InstrumentDefinition.PriceFormat.getDefaultInstance() : optionStrikePriceFormat_;
}
}
/**
*
* / Strike price display format
*
*
* .org.openfeed.InstrumentDefinition.PriceFormat optionStrikePriceFormat = 24;
*/
private com.google.protobuf.SingleFieldBuilder<
org.openfeed.InstrumentDefinition.PriceFormat, org.openfeed.InstrumentDefinition.PriceFormat.Builder, org.openfeed.InstrumentDefinition.PriceFormatOrBuilder>
getOptionStrikePriceFormatFieldBuilder() {
if (optionStrikePriceFormatBuilder_ == null) {
optionStrikePriceFormatBuilder_ = new com.google.protobuf.SingleFieldBuilder<
org.openfeed.InstrumentDefinition.PriceFormat, org.openfeed.InstrumentDefinition.PriceFormat.Builder, org.openfeed.InstrumentDefinition.PriceFormatOrBuilder>(
getOptionStrikePriceFormat(),
getParentForChildren(),
isClean());
optionStrikePriceFormat_ = null;
}
return optionStrikePriceFormatBuilder_;
}
private int priceDenominator_ ;
/**
*
* / Divide prices by this value to get real price values
*
*
* sint32 priceDenominator = 28;
* @return The priceDenominator.
*/
@java.lang.Override
public int getPriceDenominator() {
return priceDenominator_;
}
/**
*
* / Divide prices by this value to get real price values
*
*
* sint32 priceDenominator = 28;
* @param value The priceDenominator to set.
* @return This builder for chaining.
*/
public Builder setPriceDenominator(int value) {
priceDenominator_ = value;
bitField0_ |= 0x01000000;
onChanged();
return this;
}
/**
*
* / Divide prices by this value to get real price values
*
*
* sint32 priceDenominator = 28;
* @return This builder for chaining.
*/
public Builder clearPriceDenominator() {
bitField0_ = (bitField0_ & ~0x01000000);
priceDenominator_ = 0;
onChanged();
return this;
}
private int quantityDenominator_ ;
/**
*
* / Divide trade quantities by this value to get real quantities
*
*
* sint32 quantityDenominator = 29;
* @return The quantityDenominator.
*/
@java.lang.Override
public int getQuantityDenominator() {
return quantityDenominator_;
}
/**
*
* / Divide trade quantities by this value to get real quantities
*
*
* sint32 quantityDenominator = 29;
* @param value The quantityDenominator to set.
* @return This builder for chaining.
*/
public Builder setQuantityDenominator(int value) {
quantityDenominator_ = value;
bitField0_ |= 0x02000000;
onChanged();
return this;
}
/**
*
* / Divide trade quantities by this value to get real quantities
*
*
* sint32 quantityDenominator = 29;
* @return This builder for chaining.
*/
public Builder clearQuantityDenominator() {
bitField0_ = (bitField0_ & ~0x02000000);
quantityDenominator_ = 0;
onChanged();
return this;
}
private boolean isTradable_ ;
/**
*
* / true if this is a tradable instrument
*
*
* bool isTradable = 30;
* @return The isTradable.
*/
@java.lang.Override
public boolean getIsTradable() {
return isTradable_;
}
/**
*
* / true if this is a tradable instrument
*
*
* bool isTradable = 30;
* @param value The isTradable to set.
* @return This builder for chaining.
*/
public Builder setIsTradable(boolean value) {
isTradable_ = value;
bitField0_ |= 0x04000000;
onChanged();
return this;
}
/**
*
* / true if this is a tradable instrument
*
*
* bool isTradable = 30;
* @return This builder for chaining.
*/
public Builder clearIsTradable() {
bitField0_ = (bitField0_ & ~0x04000000);
isTradable_ = false;
onChanged();
return this;
}
private long transactionTime_ ;
/**
*
* / UTC timestamp of transaction, nano seconds since Unix epoch
*
*
* sint64 transactionTime = 50;
* @return The transactionTime.
*/
@java.lang.Override
public long getTransactionTime() {
return transactionTime_;
}
/**
*
* / UTC timestamp of transaction, nano seconds since Unix epoch
*
*
* sint64 transactionTime = 50;
* @param value The transactionTime to set.
* @return This builder for chaining.
*/
public Builder setTransactionTime(long value) {
transactionTime_ = value;
bitField0_ |= 0x08000000;
onChanged();
return this;
}
/**
*
* / UTC timestamp of transaction, nano seconds since Unix epoch
*
*
* sint64 transactionTime = 50;
* @return This builder for chaining.
*/
public Builder clearTransactionTime() {
bitField0_ = (bitField0_ & ~0x08000000);
transactionTime_ = 0L;
onChanged();
return this;
}
private com.google.protobuf.ByteString auxiliaryData_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* / For internal use only. Ignore
*
*
* bytes auxiliaryData = 99;
* @return The auxiliaryData.
*/
@java.lang.Override
public com.google.protobuf.ByteString getAuxiliaryData() {
return auxiliaryData_;
}
/**
*
* / For internal use only. Ignore
*
*
* bytes auxiliaryData = 99;
* @param value The auxiliaryData to set.
* @return This builder for chaining.
*/
public Builder setAuxiliaryData(com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
auxiliaryData_ = value;
bitField0_ |= 0x10000000;
onChanged();
return this;
}
/**
*
* / For internal use only. Ignore
*
*
* bytes auxiliaryData = 99;
* @return This builder for chaining.
*/
public Builder clearAuxiliaryData() {
bitField0_ = (bitField0_ & ~0x10000000);
auxiliaryData_ = getDefaultInstance().getAuxiliaryData();
onChanged();
return this;
}
private java.util.List symbols_ =
java.util.Collections.emptyList();
private void ensureSymbolsIsMutable() {
if (!((bitField0_ & 0x20000000) != 0)) {
symbols_ = new java.util.ArrayList(symbols_);
bitField0_ |= 0x20000000;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
org.openfeed.InstrumentDefinition.Symbol, org.openfeed.InstrumentDefinition.Symbol.Builder, org.openfeed.InstrumentDefinition.SymbolOrBuilder> symbolsBuilder_;
/**
*
* / List of alternate symbols for this instrument. A single instrument
* may be provided by many different market data vendors, each with
* their own unique symbology. Allows this instrument to be tagged
* with as many vendor symbols as necessary.
*
*
* repeated .org.openfeed.InstrumentDefinition.Symbol symbols = 100;
*/
public java.util.List getSymbolsList() {
if (symbolsBuilder_ == null) {
return java.util.Collections.unmodifiableList(symbols_);
} else {
return symbolsBuilder_.getMessageList();
}
}
/**
*
* / List of alternate symbols for this instrument. A single instrument
* may be provided by many different market data vendors, each with
* their own unique symbology. Allows this instrument to be tagged
* with as many vendor symbols as necessary.
*
*
* repeated .org.openfeed.InstrumentDefinition.Symbol symbols = 100;
*/
public int getSymbolsCount() {
if (symbolsBuilder_ == null) {
return symbols_.size();
} else {
return symbolsBuilder_.getCount();
}
}
/**
*
* / List of alternate symbols for this instrument. A single instrument
* may be provided by many different market data vendors, each with
* their own unique symbology. Allows this instrument to be tagged
* with as many vendor symbols as necessary.
*
*
* repeated .org.openfeed.InstrumentDefinition.Symbol symbols = 100;
*/
public org.openfeed.InstrumentDefinition.Symbol getSymbols(int index) {
if (symbolsBuilder_ == null) {
return symbols_.get(index);
} else {
return symbolsBuilder_.getMessage(index);
}
}
/**
*
* / List of alternate symbols for this instrument. A single instrument
* may be provided by many different market data vendors, each with
* their own unique symbology. Allows this instrument to be tagged
* with as many vendor symbols as necessary.
*
*
* repeated .org.openfeed.InstrumentDefinition.Symbol symbols = 100;
*/
public Builder setSymbols(
int index, org.openfeed.InstrumentDefinition.Symbol value) {
if (symbolsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureSymbolsIsMutable();
symbols_.set(index, value);
onChanged();
} else {
symbolsBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* / List of alternate symbols for this instrument. A single instrument
* may be provided by many different market data vendors, each with
* their own unique symbology. Allows this instrument to be tagged
* with as many vendor symbols as necessary.
*
*
* repeated .org.openfeed.InstrumentDefinition.Symbol symbols = 100;
*/
public Builder setSymbols(
int index, org.openfeed.InstrumentDefinition.Symbol.Builder builderForValue) {
if (symbolsBuilder_ == null) {
ensureSymbolsIsMutable();
symbols_.set(index, builderForValue.build());
onChanged();
} else {
symbolsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* / List of alternate symbols for this instrument. A single instrument
* may be provided by many different market data vendors, each with
* their own unique symbology. Allows this instrument to be tagged
* with as many vendor symbols as necessary.
*
*
* repeated .org.openfeed.InstrumentDefinition.Symbol symbols = 100;
*/
public Builder addSymbols(org.openfeed.InstrumentDefinition.Symbol value) {
if (symbolsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureSymbolsIsMutable();
symbols_.add(value);
onChanged();
} else {
symbolsBuilder_.addMessage(value);
}
return this;
}
/**
*
* / List of alternate symbols for this instrument. A single instrument
* may be provided by many different market data vendors, each with
* their own unique symbology. Allows this instrument to be tagged
* with as many vendor symbols as necessary.
*
*
* repeated .org.openfeed.InstrumentDefinition.Symbol symbols = 100;
*/
public Builder addSymbols(
int index, org.openfeed.InstrumentDefinition.Symbol value) {
if (symbolsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureSymbolsIsMutable();
symbols_.add(index, value);
onChanged();
} else {
symbolsBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* / List of alternate symbols for this instrument. A single instrument
* may be provided by many different market data vendors, each with
* their own unique symbology. Allows this instrument to be tagged
* with as many vendor symbols as necessary.
*
*
* repeated .org.openfeed.InstrumentDefinition.Symbol symbols = 100;
*/
public Builder addSymbols(
org.openfeed.InstrumentDefinition.Symbol.Builder builderForValue) {
if (symbolsBuilder_ == null) {
ensureSymbolsIsMutable();
symbols_.add(builderForValue.build());
onChanged();
} else {
symbolsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* / List of alternate symbols for this instrument. A single instrument
* may be provided by many different market data vendors, each with
* their own unique symbology. Allows this instrument to be tagged
* with as many vendor symbols as necessary.
*
*
* repeated .org.openfeed.InstrumentDefinition.Symbol symbols = 100;
*/
public Builder addSymbols(
int index, org.openfeed.InstrumentDefinition.Symbol.Builder builderForValue) {
if (symbolsBuilder_ == null) {
ensureSymbolsIsMutable();
symbols_.add(index, builderForValue.build());
onChanged();
} else {
symbolsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* / List of alternate symbols for this instrument. A single instrument
* may be provided by many different market data vendors, each with
* their own unique symbology. Allows this instrument to be tagged
* with as many vendor symbols as necessary.
*
*
* repeated .org.openfeed.InstrumentDefinition.Symbol symbols = 100;
*/
public Builder addAllSymbols(
java.lang.Iterable extends org.openfeed.InstrumentDefinition.Symbol> values) {
if (symbolsBuilder_ == null) {
ensureSymbolsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, symbols_);
onChanged();
} else {
symbolsBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* / List of alternate symbols for this instrument. A single instrument
* may be provided by many different market data vendors, each with
* their own unique symbology. Allows this instrument to be tagged
* with as many vendor symbols as necessary.
*
*
* repeated .org.openfeed.InstrumentDefinition.Symbol symbols = 100;
*/
public Builder clearSymbols() {
if (symbolsBuilder_ == null) {
symbols_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x20000000);
onChanged();
} else {
symbolsBuilder_.clear();
}
return this;
}
/**
*
* / List of alternate symbols for this instrument. A single instrument
* may be provided by many different market data vendors, each with
* their own unique symbology. Allows this instrument to be tagged
* with as many vendor symbols as necessary.
*
*
* repeated .org.openfeed.InstrumentDefinition.Symbol symbols = 100;
*/
public Builder removeSymbols(int index) {
if (symbolsBuilder_ == null) {
ensureSymbolsIsMutable();
symbols_.remove(index);
onChanged();
} else {
symbolsBuilder_.remove(index);
}
return this;
}
/**
*
* / List of alternate symbols for this instrument. A single instrument
* may be provided by many different market data vendors, each with
* their own unique symbology. Allows this instrument to be tagged
* with as many vendor symbols as necessary.
*
*
* repeated .org.openfeed.InstrumentDefinition.Symbol symbols = 100;
*/
public org.openfeed.InstrumentDefinition.Symbol.Builder getSymbolsBuilder(
int index) {
return getSymbolsFieldBuilder().getBuilder(index);
}
/**
*
* / List of alternate symbols for this instrument. A single instrument
* may be provided by many different market data vendors, each with
* their own unique symbology. Allows this instrument to be tagged
* with as many vendor symbols as necessary.
*
*
* repeated .org.openfeed.InstrumentDefinition.Symbol symbols = 100;
*/
public org.openfeed.InstrumentDefinition.SymbolOrBuilder getSymbolsOrBuilder(
int index) {
if (symbolsBuilder_ == null) {
return symbols_.get(index); } else {
return symbolsBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* / List of alternate symbols for this instrument. A single instrument
* may be provided by many different market data vendors, each with
* their own unique symbology. Allows this instrument to be tagged
* with as many vendor symbols as necessary.
*
*
* repeated .org.openfeed.InstrumentDefinition.Symbol symbols = 100;
*/
public java.util.List extends org.openfeed.InstrumentDefinition.SymbolOrBuilder>
getSymbolsOrBuilderList() {
if (symbolsBuilder_ != null) {
return symbolsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(symbols_);
}
}
/**
*
* / List of alternate symbols for this instrument. A single instrument
* may be provided by many different market data vendors, each with
* their own unique symbology. Allows this instrument to be tagged
* with as many vendor symbols as necessary.
*
*
* repeated .org.openfeed.InstrumentDefinition.Symbol symbols = 100;
*/
public org.openfeed.InstrumentDefinition.Symbol.Builder addSymbolsBuilder() {
return getSymbolsFieldBuilder().addBuilder(
org.openfeed.InstrumentDefinition.Symbol.getDefaultInstance());
}
/**
*
* / List of alternate symbols for this instrument. A single instrument
* may be provided by many different market data vendors, each with
* their own unique symbology. Allows this instrument to be tagged
* with as many vendor symbols as necessary.
*
*
* repeated .org.openfeed.InstrumentDefinition.Symbol symbols = 100;
*/
public org.openfeed.InstrumentDefinition.Symbol.Builder addSymbolsBuilder(
int index) {
return getSymbolsFieldBuilder().addBuilder(
index, org.openfeed.InstrumentDefinition.Symbol.getDefaultInstance());
}
/**
*
* / List of alternate symbols for this instrument. A single instrument
* may be provided by many different market data vendors, each with
* their own unique symbology. Allows this instrument to be tagged
* with as many vendor symbols as necessary.
*
*
* repeated .org.openfeed.InstrumentDefinition.Symbol symbols = 100;
*/
public java.util.List
getSymbolsBuilderList() {
return getSymbolsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
org.openfeed.InstrumentDefinition.Symbol, org.openfeed.InstrumentDefinition.Symbol.Builder, org.openfeed.InstrumentDefinition.SymbolOrBuilder>
getSymbolsFieldBuilder() {
if (symbolsBuilder_ == null) {
symbolsBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
org.openfeed.InstrumentDefinition.Symbol, org.openfeed.InstrumentDefinition.Symbol.Builder, org.openfeed.InstrumentDefinition.SymbolOrBuilder>(
symbols_,
((bitField0_ & 0x20000000) != 0),
getParentForChildren(),
isClean());
symbols_ = null;
}
return symbolsBuilder_;
}
private long optionStrike_ ;
/**
*
* / Option strike price in market currency. Multiply by
* / factorOptionsStrike to get actual strike
*
*
* sint64 optionStrike = 200;
* @return The optionStrike.
*/
@java.lang.Override
public long getOptionStrike() {
return optionStrike_;
}
/**
*
* / Option strike price in market currency. Multiply by
* / factorOptionsStrike to get actual strike
*
*
* sint64 optionStrike = 200;
* @param value The optionStrike to set.
* @return This builder for chaining.
*/
public Builder setOptionStrike(long value) {
optionStrike_ = value;
bitField0_ |= 0x40000000;
onChanged();
return this;
}
/**
*
* / Option strike price in market currency. Multiply by
* / factorOptionsStrike to get actual strike
*
*
* sint64 optionStrike = 200;
* @return This builder for chaining.
*/
public Builder clearOptionStrike() {
bitField0_ = (bitField0_ & ~0x40000000);
optionStrike_ = 0L;
onChanged();
return this;
}
private int optionType_ = 0;
/**
*
* / Option type: call vs put.
*
*
* .org.openfeed.InstrumentDefinition.OptionType optionType = 202;
* @return The enum numeric value on the wire for optionType.
*/
@java.lang.Override public int getOptionTypeValue() {
return optionType_;
}
/**
*
* / Option type: call vs put.
*
*
* .org.openfeed.InstrumentDefinition.OptionType optionType = 202;
* @param value The enum numeric value on the wire for optionType to set.
* @return This builder for chaining.
*/
public Builder setOptionTypeValue(int value) {
optionType_ = value;
bitField0_ |= 0x80000000;
onChanged();
return this;
}
/**
*
* / Option type: call vs put.
*
*
* .org.openfeed.InstrumentDefinition.OptionType optionType = 202;
* @return The optionType.
*/
@java.lang.Override
public org.openfeed.InstrumentDefinition.OptionType getOptionType() {
org.openfeed.InstrumentDefinition.OptionType result = org.openfeed.InstrumentDefinition.OptionType.forNumber(optionType_);
return result == null ? org.openfeed.InstrumentDefinition.OptionType.UNRECOGNIZED : result;
}
/**
*
* / Option type: call vs put.
*
*
* .org.openfeed.InstrumentDefinition.OptionType optionType = 202;
* @param value The optionType to set.
* @return This builder for chaining.
*/
public Builder setOptionType(org.openfeed.InstrumentDefinition.OptionType value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x80000000;
optionType_ = value.getNumber();
onChanged();
return this;
}
/**
*
* / Option type: call vs put.
*
*
* .org.openfeed.InstrumentDefinition.OptionType optionType = 202;
* @return This builder for chaining.
*/
public Builder clearOptionType() {
bitField0_ = (bitField0_ & ~0x80000000);
optionType_ = 0;
onChanged();
return this;
}
private int optionStyle_ = 0;
/**
*
* / Option style : American vs European.
*
*
* .org.openfeed.InstrumentDefinition.OptionStyle optionStyle = 203;
* @return The enum numeric value on the wire for optionStyle.
*/
@java.lang.Override public int getOptionStyleValue() {
return optionStyle_;
}
/**
*
* / Option style : American vs European.
*
*
* .org.openfeed.InstrumentDefinition.OptionStyle optionStyle = 203;
* @param value The enum numeric value on the wire for optionStyle to set.
* @return This builder for chaining.
*/
public Builder setOptionStyleValue(int value) {
optionStyle_ = value;
bitField1_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* / Option style : American vs European.
*
*
* .org.openfeed.InstrumentDefinition.OptionStyle optionStyle = 203;
* @return The optionStyle.
*/
@java.lang.Override
public org.openfeed.InstrumentDefinition.OptionStyle getOptionStyle() {
org.openfeed.InstrumentDefinition.OptionStyle result = org.openfeed.InstrumentDefinition.OptionStyle.forNumber(optionStyle_);
return result == null ? org.openfeed.InstrumentDefinition.OptionStyle.UNRECOGNIZED : result;
}
/**
*
* / Option style : American vs European.
*
*
* .org.openfeed.InstrumentDefinition.OptionStyle optionStyle = 203;
* @param value The optionStyle to set.
* @return This builder for chaining.
*/
public Builder setOptionStyle(org.openfeed.InstrumentDefinition.OptionStyle value) {
if (value == null) {
throw new NullPointerException();
}
bitField1_ |= 0x00000001;
optionStyle_ = value.getNumber();
onChanged();
return this;
}
/**
*
* / Option style : American vs European.
*
*
* .org.openfeed.InstrumentDefinition.OptionStyle optionStyle = 203;
* @return This builder for chaining.
*/
public Builder clearOptionStyle() {
bitField1_ = (bitField1_ & ~0x00000001);
optionStyle_ = 0;
onChanged();
return this;
}
private int optionStrikeDenominator_ ;
/**
*
* / Divide optionStrike by this value to get real strike price
*
*
* sint32 optionStrikeDenominator = 204;
* @return The optionStrikeDenominator.
*/
@java.lang.Override
public int getOptionStrikeDenominator() {
return optionStrikeDenominator_;
}
/**
*
* / Divide optionStrike by this value to get real strike price
*
*
* sint32 optionStrikeDenominator = 204;
* @param value The optionStrikeDenominator to set.
* @return This builder for chaining.
*/
public Builder setOptionStrikeDenominator(int value) {
optionStrikeDenominator_ = value;
bitField1_ |= 0x00000002;
onChanged();
return this;
}
/**
*
* / Divide optionStrike by this value to get real strike price
*
*
* sint32 optionStrikeDenominator = 204;
* @return This builder for chaining.
*/
public Builder clearOptionStrikeDenominator() {
bitField1_ = (bitField1_ & ~0x00000002);
optionStrikeDenominator_ = 0;
onChanged();
return this;
}
private java.lang.Object spreadCode_ = "";
/**
*
* / Spread type, can be vendor specific
*
*
* string spreadCode = 210;
* @return The spreadCode.
*/
public java.lang.String getSpreadCode() {
java.lang.Object ref = spreadCode_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
spreadCode_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* / Spread type, can be vendor specific
*
*
* string spreadCode = 210;
* @return The bytes for spreadCode.
*/
public com.google.protobuf.ByteString
getSpreadCodeBytes() {
java.lang.Object ref = spreadCode_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
spreadCode_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* / Spread type, can be vendor specific
*
*
* string spreadCode = 210;
* @param value The spreadCode to set.
* @return This builder for chaining.
*/
public Builder setSpreadCode(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
spreadCode_ = value;
bitField1_ |= 0x00000004;
onChanged();
return this;
}
/**
*
* / Spread type, can be vendor specific
*
*
* string spreadCode = 210;
* @return This builder for chaining.
*/
public Builder clearSpreadCode() {
spreadCode_ = getDefaultInstance().getSpreadCode();
bitField1_ = (bitField1_ & ~0x00000004);
onChanged();
return this;
}
/**
*
* / Spread type, can be vendor specific
*
*
* string spreadCode = 210;
* @param value The bytes for spreadCode to set.
* @return This builder for chaining.
*/
public Builder setSpreadCodeBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
spreadCode_ = value;
bitField1_ |= 0x00000004;
onChanged();
return this;
}
private java.util.List spreadLeg_ =
java.util.Collections.emptyList();
private void ensureSpreadLegIsMutable() {
if (!((bitField1_ & 0x00000008) != 0)) {
spreadLeg_ = new java.util.ArrayList(spreadLeg_);
bitField1_ |= 0x00000008;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
org.openfeed.InstrumentDefinition.SpreadLeg, org.openfeed.InstrumentDefinition.SpreadLeg.Builder, org.openfeed.InstrumentDefinition.SpreadLegOrBuilder> spreadLegBuilder_;
/**
*
* / Ordered list of underlying legs in a spread.
*
*
* repeated .org.openfeed.InstrumentDefinition.SpreadLeg spreadLeg = 211;
*/
public java.util.List getSpreadLegList() {
if (spreadLegBuilder_ == null) {
return java.util.Collections.unmodifiableList(spreadLeg_);
} else {
return spreadLegBuilder_.getMessageList();
}
}
/**
*
* / Ordered list of underlying legs in a spread.
*
*
* repeated .org.openfeed.InstrumentDefinition.SpreadLeg spreadLeg = 211;
*/
public int getSpreadLegCount() {
if (spreadLegBuilder_ == null) {
return spreadLeg_.size();
} else {
return spreadLegBuilder_.getCount();
}
}
/**
*
* / Ordered list of underlying legs in a spread.
*
*
* repeated .org.openfeed.InstrumentDefinition.SpreadLeg spreadLeg = 211;
*/
public org.openfeed.InstrumentDefinition.SpreadLeg getSpreadLeg(int index) {
if (spreadLegBuilder_ == null) {
return spreadLeg_.get(index);
} else {
return spreadLegBuilder_.getMessage(index);
}
}
/**
*
* / Ordered list of underlying legs in a spread.
*
*
* repeated .org.openfeed.InstrumentDefinition.SpreadLeg spreadLeg = 211;
*/
public Builder setSpreadLeg(
int index, org.openfeed.InstrumentDefinition.SpreadLeg value) {
if (spreadLegBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureSpreadLegIsMutable();
spreadLeg_.set(index, value);
onChanged();
} else {
spreadLegBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* / Ordered list of underlying legs in a spread.
*
*
* repeated .org.openfeed.InstrumentDefinition.SpreadLeg spreadLeg = 211;
*/
public Builder setSpreadLeg(
int index, org.openfeed.InstrumentDefinition.SpreadLeg.Builder builderForValue) {
if (spreadLegBuilder_ == null) {
ensureSpreadLegIsMutable();
spreadLeg_.set(index, builderForValue.build());
onChanged();
} else {
spreadLegBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* / Ordered list of underlying legs in a spread.
*
*
* repeated .org.openfeed.InstrumentDefinition.SpreadLeg spreadLeg = 211;
*/
public Builder addSpreadLeg(org.openfeed.InstrumentDefinition.SpreadLeg value) {
if (spreadLegBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureSpreadLegIsMutable();
spreadLeg_.add(value);
onChanged();
} else {
spreadLegBuilder_.addMessage(value);
}
return this;
}
/**
*
* / Ordered list of underlying legs in a spread.
*
*
* repeated .org.openfeed.InstrumentDefinition.SpreadLeg spreadLeg = 211;
*/
public Builder addSpreadLeg(
int index, org.openfeed.InstrumentDefinition.SpreadLeg value) {
if (spreadLegBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureSpreadLegIsMutable();
spreadLeg_.add(index, value);
onChanged();
} else {
spreadLegBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* / Ordered list of underlying legs in a spread.
*
*
* repeated .org.openfeed.InstrumentDefinition.SpreadLeg spreadLeg = 211;
*/
public Builder addSpreadLeg(
org.openfeed.InstrumentDefinition.SpreadLeg.Builder builderForValue) {
if (spreadLegBuilder_ == null) {
ensureSpreadLegIsMutable();
spreadLeg_.add(builderForValue.build());
onChanged();
} else {
spreadLegBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* / Ordered list of underlying legs in a spread.
*
*
* repeated .org.openfeed.InstrumentDefinition.SpreadLeg spreadLeg = 211;
*/
public Builder addSpreadLeg(
int index, org.openfeed.InstrumentDefinition.SpreadLeg.Builder builderForValue) {
if (spreadLegBuilder_ == null) {
ensureSpreadLegIsMutable();
spreadLeg_.add(index, builderForValue.build());
onChanged();
} else {
spreadLegBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* / Ordered list of underlying legs in a spread.
*
*
* repeated .org.openfeed.InstrumentDefinition.SpreadLeg spreadLeg = 211;
*/
public Builder addAllSpreadLeg(
java.lang.Iterable extends org.openfeed.InstrumentDefinition.SpreadLeg> values) {
if (spreadLegBuilder_ == null) {
ensureSpreadLegIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, spreadLeg_);
onChanged();
} else {
spreadLegBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* / Ordered list of underlying legs in a spread.
*
*
* repeated .org.openfeed.InstrumentDefinition.SpreadLeg spreadLeg = 211;
*/
public Builder clearSpreadLeg() {
if (spreadLegBuilder_ == null) {
spreadLeg_ = java.util.Collections.emptyList();
bitField1_ = (bitField1_ & ~0x00000008);
onChanged();
} else {
spreadLegBuilder_.clear();
}
return this;
}
/**
*
* / Ordered list of underlying legs in a spread.
*
*
* repeated .org.openfeed.InstrumentDefinition.SpreadLeg spreadLeg = 211;
*/
public Builder removeSpreadLeg(int index) {
if (spreadLegBuilder_ == null) {
ensureSpreadLegIsMutable();
spreadLeg_.remove(index);
onChanged();
} else {
spreadLegBuilder_.remove(index);
}
return this;
}
/**
*
* / Ordered list of underlying legs in a spread.
*
*
* repeated .org.openfeed.InstrumentDefinition.SpreadLeg spreadLeg = 211;
*/
public org.openfeed.InstrumentDefinition.SpreadLeg.Builder getSpreadLegBuilder(
int index) {
return getSpreadLegFieldBuilder().getBuilder(index);
}
/**
*
* / Ordered list of underlying legs in a spread.
*
*
* repeated .org.openfeed.InstrumentDefinition.SpreadLeg spreadLeg = 211;
*/
public org.openfeed.InstrumentDefinition.SpreadLegOrBuilder getSpreadLegOrBuilder(
int index) {
if (spreadLegBuilder_ == null) {
return spreadLeg_.get(index); } else {
return spreadLegBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* / Ordered list of underlying legs in a spread.
*
*
* repeated .org.openfeed.InstrumentDefinition.SpreadLeg spreadLeg = 211;
*/
public java.util.List extends org.openfeed.InstrumentDefinition.SpreadLegOrBuilder>
getSpreadLegOrBuilderList() {
if (spreadLegBuilder_ != null) {
return spreadLegBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(spreadLeg_);
}
}
/**
*
* / Ordered list of underlying legs in a spread.
*
*
* repeated .org.openfeed.InstrumentDefinition.SpreadLeg spreadLeg = 211;
*/
public org.openfeed.InstrumentDefinition.SpreadLeg.Builder addSpreadLegBuilder() {
return getSpreadLegFieldBuilder().addBuilder(
org.openfeed.InstrumentDefinition.SpreadLeg.getDefaultInstance());
}
/**
*
* / Ordered list of underlying legs in a spread.
*
*
* repeated .org.openfeed.InstrumentDefinition.SpreadLeg spreadLeg = 211;
*/
public org.openfeed.InstrumentDefinition.SpreadLeg.Builder addSpreadLegBuilder(
int index) {
return getSpreadLegFieldBuilder().addBuilder(
index, org.openfeed.InstrumentDefinition.SpreadLeg.getDefaultInstance());
}
/**
*
* / Ordered list of underlying legs in a spread.
*
*
* repeated .org.openfeed.InstrumentDefinition.SpreadLeg spreadLeg = 211;
*/
public java.util.List
getSpreadLegBuilderList() {
return getSpreadLegFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
org.openfeed.InstrumentDefinition.SpreadLeg, org.openfeed.InstrumentDefinition.SpreadLeg.Builder, org.openfeed.InstrumentDefinition.SpreadLegOrBuilder>
getSpreadLegFieldBuilder() {
if (spreadLegBuilder_ == null) {
spreadLegBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
org.openfeed.InstrumentDefinition.SpreadLeg, org.openfeed.InstrumentDefinition.SpreadLeg.Builder, org.openfeed.InstrumentDefinition.SpreadLegOrBuilder>(
spreadLeg_,
((bitField1_ & 0x00000008) != 0),
getParentForChildren(),
isClean());
spreadLeg_ = null;
}
return spreadLegBuilder_;
}
private boolean userDefinedSpread_ ;
/**
*
* / true if user defined spread
*
*
* bool userDefinedSpread = 212;
* @return The userDefinedSpread.
*/
@java.lang.Override
public boolean getUserDefinedSpread() {
return userDefinedSpread_;
}
/**
*
* / true if user defined spread
*
*
* bool userDefinedSpread = 212;
* @param value The userDefinedSpread to set.
* @return This builder for chaining.
*/
public Builder setUserDefinedSpread(boolean value) {
userDefinedSpread_ = value;
bitField1_ |= 0x00000010;
onChanged();
return this;
}
/**
*
* / true if user defined spread
*
*
* bool userDefinedSpread = 212;
* @return This builder for chaining.
*/
public Builder clearUserDefinedSpread() {
bitField1_ = (bitField1_ & ~0x00000010);
userDefinedSpread_ = false;
onChanged();
return this;
}
private java.lang.Object marketTier_ = "";
/**
*
* / Listing market classification
*
*
* string marketTier = 213;
* @return The marketTier.
*/
public java.lang.String getMarketTier() {
java.lang.Object ref = marketTier_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
marketTier_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* / Listing market classification
*
*
* string marketTier = 213;
* @return The bytes for marketTier.
*/
public com.google.protobuf.ByteString
getMarketTierBytes() {
java.lang.Object ref = marketTier_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
marketTier_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* / Listing market classification
*
*
* string marketTier = 213;
* @param value The marketTier to set.
* @return This builder for chaining.
*/
public Builder setMarketTier(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
marketTier_ = value;
bitField1_ |= 0x00000020;
onChanged();
return this;
}
/**
*
* / Listing market classification
*
*
* string marketTier = 213;
* @return This builder for chaining.
*/
public Builder clearMarketTier() {
marketTier_ = getDefaultInstance().getMarketTier();
bitField1_ = (bitField1_ & ~0x00000020);
onChanged();
return this;
}
/**
*
* / Listing market classification
*
*
* string marketTier = 213;
* @param value The bytes for marketTier to set.
* @return This builder for chaining.
*/
public Builder setMarketTierBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
marketTier_ = value;
bitField1_ |= 0x00000020;
onChanged();
return this;
}
private java.lang.Object financialStatusIndicator_ = "";
/**
*
* / Current financial status of the issuer
*
*
* string financialStatusIndicator = 214;
* @return The financialStatusIndicator.
*/
public java.lang.String getFinancialStatusIndicator() {
java.lang.Object ref = financialStatusIndicator_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
financialStatusIndicator_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* / Current financial status of the issuer
*
*
* string financialStatusIndicator = 214;
* @return The bytes for financialStatusIndicator.
*/
public com.google.protobuf.ByteString
getFinancialStatusIndicatorBytes() {
java.lang.Object ref = financialStatusIndicator_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
financialStatusIndicator_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* / Current financial status of the issuer
*
*
* string financialStatusIndicator = 214;
* @param value The financialStatusIndicator to set.
* @return This builder for chaining.
*/
public Builder setFinancialStatusIndicator(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
financialStatusIndicator_ = value;
bitField1_ |= 0x00000040;
onChanged();
return this;
}
/**
*
* / Current financial status of the issuer
*
*
* string financialStatusIndicator = 214;
* @return This builder for chaining.
*/
public Builder clearFinancialStatusIndicator() {
financialStatusIndicator_ = getDefaultInstance().getFinancialStatusIndicator();
bitField1_ = (bitField1_ & ~0x00000040);
onChanged();
return this;
}
/**
*
* / Current financial status of the issuer
*
*
* string financialStatusIndicator = 214;
* @param value The bytes for financialStatusIndicator to set.
* @return This builder for chaining.
*/
public Builder setFinancialStatusIndicatorBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
financialStatusIndicator_ = value;
bitField1_ |= 0x00000040;
onChanged();
return this;
}
private java.lang.Object isin_ = "";
/**
*
* / ISIN: https://en.wikipedia.org/wiki/International_Securities_Identification_Number
*
*
* string isin = 215;
* @return The isin.
*/
public java.lang.String getIsin() {
java.lang.Object ref = isin_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
isin_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* / ISIN: https://en.wikipedia.org/wiki/International_Securities_Identification_Number
*
*
* string isin = 215;
* @return The bytes for isin.
*/
public com.google.protobuf.ByteString
getIsinBytes() {
java.lang.Object ref = isin_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
isin_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* / ISIN: https://en.wikipedia.org/wiki/International_Securities_Identification_Number
*
*
* string isin = 215;
* @param value The isin to set.
* @return This builder for chaining.
*/
public Builder setIsin(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
isin_ = value;
bitField1_ |= 0x00000080;
onChanged();
return this;
}
/**
*
* / ISIN: https://en.wikipedia.org/wiki/International_Securities_Identification_Number
*
*
* string isin = 215;
* @return This builder for chaining.
*/
public Builder clearIsin() {
isin_ = getDefaultInstance().getIsin();
bitField1_ = (bitField1_ & ~0x00000080);
onChanged();
return this;
}
/**
*
* / ISIN: https://en.wikipedia.org/wiki/International_Securities_Identification_Number
*
*
* string isin = 215;
* @param value The bytes for isin to set.
* @return This builder for chaining.
*/
public Builder setIsinBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
isin_ = value;
bitField1_ |= 0x00000080;
onChanged();
return this;
}
private org.openfeed.InstrumentDefinition.CurrencyPair currencyPair_;
private com.google.protobuf.SingleFieldBuilder<
org.openfeed.InstrumentDefinition.CurrencyPair, org.openfeed.InstrumentDefinition.CurrencyPair.Builder, org.openfeed.InstrumentDefinition.CurrencyPairOrBuilder> currencyPairBuilder_;
/**
*
* / Break out of currency pair
*
*
* .org.openfeed.InstrumentDefinition.CurrencyPair currencyPair = 216;
* @return Whether the currencyPair field is set.
*/
public boolean hasCurrencyPair() {
return ((bitField1_ & 0x00000100) != 0);
}
/**
*
* / Break out of currency pair
*
*
* .org.openfeed.InstrumentDefinition.CurrencyPair currencyPair = 216;
* @return The currencyPair.
*/
public org.openfeed.InstrumentDefinition.CurrencyPair getCurrencyPair() {
if (currencyPairBuilder_ == null) {
return currencyPair_ == null ? org.openfeed.InstrumentDefinition.CurrencyPair.getDefaultInstance() : currencyPair_;
} else {
return currencyPairBuilder_.getMessage();
}
}
/**
*
* / Break out of currency pair
*
*
* .org.openfeed.InstrumentDefinition.CurrencyPair currencyPair = 216;
*/
public Builder setCurrencyPair(org.openfeed.InstrumentDefinition.CurrencyPair value) {
if (currencyPairBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
currencyPair_ = value;
} else {
currencyPairBuilder_.setMessage(value);
}
bitField1_ |= 0x00000100;
onChanged();
return this;
}
/**
*
* / Break out of currency pair
*
*
* .org.openfeed.InstrumentDefinition.CurrencyPair currencyPair = 216;
*/
public Builder setCurrencyPair(
org.openfeed.InstrumentDefinition.CurrencyPair.Builder builderForValue) {
if (currencyPairBuilder_ == null) {
currencyPair_ = builderForValue.build();
} else {
currencyPairBuilder_.setMessage(builderForValue.build());
}
bitField1_ |= 0x00000100;
onChanged();
return this;
}
/**
*
* / Break out of currency pair
*
*
* .org.openfeed.InstrumentDefinition.CurrencyPair currencyPair = 216;
*/
public Builder mergeCurrencyPair(org.openfeed.InstrumentDefinition.CurrencyPair value) {
if (currencyPairBuilder_ == null) {
if (((bitField1_ & 0x00000100) != 0) &&
currencyPair_ != null &&
currencyPair_ != org.openfeed.InstrumentDefinition.CurrencyPair.getDefaultInstance()) {
getCurrencyPairBuilder().mergeFrom(value);
} else {
currencyPair_ = value;
}
} else {
currencyPairBuilder_.mergeFrom(value);
}
if (currencyPair_ != null) {
bitField1_ |= 0x00000100;
onChanged();
}
return this;
}
/**
*
* / Break out of currency pair
*
*
* .org.openfeed.InstrumentDefinition.CurrencyPair currencyPair = 216;
*/
public Builder clearCurrencyPair() {
bitField1_ = (bitField1_ & ~0x00000100);
currencyPair_ = null;
if (currencyPairBuilder_ != null) {
currencyPairBuilder_.dispose();
currencyPairBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* / Break out of currency pair
*
*
* .org.openfeed.InstrumentDefinition.CurrencyPair currencyPair = 216;
*/
public org.openfeed.InstrumentDefinition.CurrencyPair.Builder getCurrencyPairBuilder() {
bitField1_ |= 0x00000100;
onChanged();
return getCurrencyPairFieldBuilder().getBuilder();
}
/**
*
* / Break out of currency pair
*
*
* .org.openfeed.InstrumentDefinition.CurrencyPair currencyPair = 216;
*/
public org.openfeed.InstrumentDefinition.CurrencyPairOrBuilder getCurrencyPairOrBuilder() {
if (currencyPairBuilder_ != null) {
return currencyPairBuilder_.getMessageOrBuilder();
} else {
return currencyPair_ == null ?
org.openfeed.InstrumentDefinition.CurrencyPair.getDefaultInstance() : currencyPair_;
}
}
/**
*
* / Break out of currency pair
*
*
* .org.openfeed.InstrumentDefinition.CurrencyPair currencyPair = 216;
*/
private com.google.protobuf.SingleFieldBuilder<
org.openfeed.InstrumentDefinition.CurrencyPair, org.openfeed.InstrumentDefinition.CurrencyPair.Builder, org.openfeed.InstrumentDefinition.CurrencyPairOrBuilder>
getCurrencyPairFieldBuilder() {
if (currencyPairBuilder_ == null) {
currencyPairBuilder_ = new com.google.protobuf.SingleFieldBuilder<
org.openfeed.InstrumentDefinition.CurrencyPair, org.openfeed.InstrumentDefinition.CurrencyPair.Builder, org.openfeed.InstrumentDefinition.CurrencyPairOrBuilder>(
getCurrencyPair(),
getParentForChildren(),
isClean());
currencyPair_ = null;
}
return currencyPairBuilder_;
}
private boolean exchangeSendsVolume_ ;
/**
*
* / true if exchange sends volume.
*
*
* bool exchangeSendsVolume = 217;
* @return The exchangeSendsVolume.
*/
@java.lang.Override
public boolean getExchangeSendsVolume() {
return exchangeSendsVolume_;
}
/**
*
* / true if exchange sends volume.
*
*
* bool exchangeSendsVolume = 217;
* @param value The exchangeSendsVolume to set.
* @return This builder for chaining.
*/
public Builder setExchangeSendsVolume(boolean value) {
exchangeSendsVolume_ = value;
bitField1_ |= 0x00000200;
onChanged();
return this;
}
/**
*
* / true if exchange sends volume.
*
*
* bool exchangeSendsVolume = 217;
* @return This builder for chaining.
*/
public Builder clearExchangeSendsVolume() {
bitField1_ = (bitField1_ & ~0x00000200);
exchangeSendsVolume_ = false;
onChanged();
return this;
}
private boolean exchangeSendsHigh_ ;
/**
*
* / true if exchange sends high.
*
*
* bool exchangeSendsHigh = 218;
* @return The exchangeSendsHigh.
*/
@java.lang.Override
public boolean getExchangeSendsHigh() {
return exchangeSendsHigh_;
}
/**
*
* / true if exchange sends high.
*
*
* bool exchangeSendsHigh = 218;
* @param value The exchangeSendsHigh to set.
* @return This builder for chaining.
*/
public Builder setExchangeSendsHigh(boolean value) {
exchangeSendsHigh_ = value;
bitField1_ |= 0x00000400;
onChanged();
return this;
}
/**
*
* / true if exchange sends high.
*
*
* bool exchangeSendsHigh = 218;
* @return This builder for chaining.
*/
public Builder clearExchangeSendsHigh() {
bitField1_ = (bitField1_ & ~0x00000400);
exchangeSendsHigh_ = false;
onChanged();
return this;
}
private boolean exchangeSendsLow_ ;
/**
*
* / true if exchange sends low.
*
*
* bool exchangeSendsLow = 219;
* @return The exchangeSendsLow.
*/
@java.lang.Override
public boolean getExchangeSendsLow() {
return exchangeSendsLow_;
}
/**
*
* / true if exchange sends low.
*
*
* bool exchangeSendsLow = 219;
* @param value The exchangeSendsLow to set.
* @return This builder for chaining.
*/
public Builder setExchangeSendsLow(boolean value) {
exchangeSendsLow_ = value;
bitField1_ |= 0x00000800;
onChanged();
return this;
}
/**
*
* / true if exchange sends low.
*
*
* bool exchangeSendsLow = 219;
* @return This builder for chaining.
*/
public Builder clearExchangeSendsLow() {
bitField1_ = (bitField1_ & ~0x00000800);
exchangeSendsLow_ = false;
onChanged();
return this;
}
private boolean exchangeSendsOpen_ ;
/**
*
* / true if exchange sends open.
*
*
* bool exchangeSendsOpen = 220;
* @return The exchangeSendsOpen.
*/
@java.lang.Override
public boolean getExchangeSendsOpen() {
return exchangeSendsOpen_;
}
/**
*
* / true if exchange sends open.
*
*
* bool exchangeSendsOpen = 220;
* @param value The exchangeSendsOpen to set.
* @return This builder for chaining.
*/
public Builder setExchangeSendsOpen(boolean value) {
exchangeSendsOpen_ = value;
bitField1_ |= 0x00001000;
onChanged();
return this;
}
/**
*
* / true if exchange sends open.
*
*
* bool exchangeSendsOpen = 220;
* @return This builder for chaining.
*/
public Builder clearExchangeSendsOpen() {
bitField1_ = (bitField1_ & ~0x00001000);
exchangeSendsOpen_ = false;
onChanged();
return this;
}
private boolean consolidatedFeedInstrument_ ;
/**
*
* / true if this instrument represents consolidated NBBO.
*
*
* bool consolidatedFeedInstrument = 221;
* @return The consolidatedFeedInstrument.
*/
@java.lang.Override
public boolean getConsolidatedFeedInstrument() {
return consolidatedFeedInstrument_;
}
/**
*
* / true if this instrument represents consolidated NBBO.
*
*
* bool consolidatedFeedInstrument = 221;
* @param value The consolidatedFeedInstrument to set.
* @return This builder for chaining.
*/
public Builder setConsolidatedFeedInstrument(boolean value) {
consolidatedFeedInstrument_ = value;
bitField1_ |= 0x00002000;
onChanged();
return this;
}
/**
*
* / true if this instrument represents consolidated NBBO.
*
*
* bool consolidatedFeedInstrument = 221;
* @return This builder for chaining.
*/
public Builder clearConsolidatedFeedInstrument() {
bitField1_ = (bitField1_ & ~0x00002000);
consolidatedFeedInstrument_ = false;
onChanged();
return this;
}
private boolean openOutcryInstrument_ ;
/**
*
* / true if this instrument represents Pit symbol.
*
*
* bool openOutcryInstrument = 222;
* @return The openOutcryInstrument.
*/
@java.lang.Override
public boolean getOpenOutcryInstrument() {
return openOutcryInstrument_;
}
/**
*
* / true if this instrument represents Pit symbol.
*
*
* bool openOutcryInstrument = 222;
* @param value The openOutcryInstrument to set.
* @return This builder for chaining.
*/
public Builder setOpenOutcryInstrument(boolean value) {
openOutcryInstrument_ = value;
bitField1_ |= 0x00004000;
onChanged();
return this;
}
/**
*
* / true if this instrument represents Pit symbol.
*
*
* bool openOutcryInstrument = 222;
* @return This builder for chaining.
*/
public Builder clearOpenOutcryInstrument() {
bitField1_ = (bitField1_ & ~0x00004000);
openOutcryInstrument_ = false;
onChanged();
return this;
}
private boolean syntheticAmericanOptionInstrument_ ;
/**
*
* / true if this instrument generated FX option.
*
*
* bool syntheticAmericanOptionInstrument = 223;
* @return The syntheticAmericanOptionInstrument.
*/
@java.lang.Override
public boolean getSyntheticAmericanOptionInstrument() {
return syntheticAmericanOptionInstrument_;
}
/**
*
* / true if this instrument generated FX option.
*
*
* bool syntheticAmericanOptionInstrument = 223;
* @param value The syntheticAmericanOptionInstrument to set.
* @return This builder for chaining.
*/
public Builder setSyntheticAmericanOptionInstrument(boolean value) {
syntheticAmericanOptionInstrument_ = value;
bitField1_ |= 0x00008000;
onChanged();
return this;
}
/**
*
* / true if this instrument generated FX option.
*
*
* bool syntheticAmericanOptionInstrument = 223;
* @return This builder for chaining.
*/
public Builder clearSyntheticAmericanOptionInstrument() {
bitField1_ = (bitField1_ & ~0x00008000);
syntheticAmericanOptionInstrument_ = false;
onChanged();
return this;
}
private java.lang.Object barchartExchangeCode_ = "";
/**
*
* /
*
*
* string barchartExchangeCode = 224;
* @return The barchartExchangeCode.
*/
public java.lang.String getBarchartExchangeCode() {
java.lang.Object ref = barchartExchangeCode_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
barchartExchangeCode_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* /
*
*
* string barchartExchangeCode = 224;
* @return The bytes for barchartExchangeCode.
*/
public com.google.protobuf.ByteString
getBarchartExchangeCodeBytes() {
java.lang.Object ref = barchartExchangeCode_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
barchartExchangeCode_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* /
*
*
* string barchartExchangeCode = 224;
* @param value The barchartExchangeCode to set.
* @return This builder for chaining.
*/
public Builder setBarchartExchangeCode(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
barchartExchangeCode_ = value;
bitField1_ |= 0x00010000;
onChanged();
return this;
}
/**
*
* /
*
*
* string barchartExchangeCode = 224;
* @return This builder for chaining.
*/
public Builder clearBarchartExchangeCode() {
barchartExchangeCode_ = getDefaultInstance().getBarchartExchangeCode();
bitField1_ = (bitField1_ & ~0x00010000);
onChanged();
return this;
}
/**
*
* /
*
*
* string barchartExchangeCode = 224;
* @param value The bytes for barchartExchangeCode to set.
* @return This builder for chaining.
*/
public Builder setBarchartExchangeCodeBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
barchartExchangeCode_ = value;
bitField1_ |= 0x00010000;
onChanged();
return this;
}
private java.lang.Object barchartBaseCode_ = "";
/**
*
* /
*
*
* string barchartBaseCode = 225;
* @return The barchartBaseCode.
*/
public java.lang.String getBarchartBaseCode() {
java.lang.Object ref = barchartBaseCode_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
barchartBaseCode_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* /
*
*
* string barchartBaseCode = 225;
* @return The bytes for barchartBaseCode.
*/
public com.google.protobuf.ByteString
getBarchartBaseCodeBytes() {
java.lang.Object ref = barchartBaseCode_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
barchartBaseCode_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* /
*
*
* string barchartBaseCode = 225;
* @param value The barchartBaseCode to set.
* @return This builder for chaining.
*/
public Builder setBarchartBaseCode(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
barchartBaseCode_ = value;
bitField1_ |= 0x00020000;
onChanged();
return this;
}
/**
*
* /
*
*
* string barchartBaseCode = 225;
* @return This builder for chaining.
*/
public Builder clearBarchartBaseCode() {
barchartBaseCode_ = getDefaultInstance().getBarchartBaseCode();
bitField1_ = (bitField1_ & ~0x00020000);
onChanged();
return this;
}
/**
*
* /
*
*
* string barchartBaseCode = 225;
* @param value The bytes for barchartBaseCode to set.
* @return This builder for chaining.
*/
public Builder setBarchartBaseCodeBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
barchartBaseCode_ = value;
bitField1_ |= 0x00020000;
onChanged();
return this;
}
private int volumeDenominator_ ;
/**
*
* /
*
*
* sint32 volumeDenominator = 226;
* @return The volumeDenominator.
*/
@java.lang.Override
public int getVolumeDenominator() {
return volumeDenominator_;
}
/**
*
* /
*
*
* sint32 volumeDenominator = 226;
* @param value The volumeDenominator to set.
* @return This builder for chaining.
*/
public Builder setVolumeDenominator(int value) {
volumeDenominator_ = value;
bitField1_ |= 0x00040000;
onChanged();
return this;
}
/**
*
* /
*
*
* sint32 volumeDenominator = 226;
* @return This builder for chaining.
*/
public Builder clearVolumeDenominator() {
bitField1_ = (bitField1_ & ~0x00040000);
volumeDenominator_ = 0;
onChanged();
return this;
}
private int bidOfferQuantityDenominator_ ;
/**
*
* /
*
*
* sint32 bidOfferQuantityDenominator = 227;
* @return The bidOfferQuantityDenominator.
*/
@java.lang.Override
public int getBidOfferQuantityDenominator() {
return bidOfferQuantityDenominator_;
}
/**
*
* /
*
*
* sint32 bidOfferQuantityDenominator = 227;
* @param value The bidOfferQuantityDenominator to set.
* @return This builder for chaining.
*/
public Builder setBidOfferQuantityDenominator(int value) {
bidOfferQuantityDenominator_ = value;
bitField1_ |= 0x00080000;
onChanged();
return this;
}
/**
*
* /
*
*
* sint32 bidOfferQuantityDenominator = 227;
* @return This builder for chaining.
*/
public Builder clearBidOfferQuantityDenominator() {
bitField1_ = (bitField1_ & ~0x00080000);
bidOfferQuantityDenominator_ = 0;
onChanged();
return this;
}
private java.lang.Object primaryListingMarketParticipantId_ = "";
/**
*
* /
*
*
* string primaryListingMarketParticipantId = 228;
* @return The primaryListingMarketParticipantId.
*/
public java.lang.String getPrimaryListingMarketParticipantId() {
java.lang.Object ref = primaryListingMarketParticipantId_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
primaryListingMarketParticipantId_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* /
*
*
* string primaryListingMarketParticipantId = 228;
* @return The bytes for primaryListingMarketParticipantId.
*/
public com.google.protobuf.ByteString
getPrimaryListingMarketParticipantIdBytes() {
java.lang.Object ref = primaryListingMarketParticipantId_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
primaryListingMarketParticipantId_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* /
*
*
* string primaryListingMarketParticipantId = 228;
* @param value The primaryListingMarketParticipantId to set.
* @return This builder for chaining.
*/
public Builder setPrimaryListingMarketParticipantId(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
primaryListingMarketParticipantId_ = value;
bitField1_ |= 0x00100000;
onChanged();
return this;
}
/**
*
* /
*
*
* string primaryListingMarketParticipantId = 228;
* @return This builder for chaining.
*/
public Builder clearPrimaryListingMarketParticipantId() {
primaryListingMarketParticipantId_ = getDefaultInstance().getPrimaryListingMarketParticipantId();
bitField1_ = (bitField1_ & ~0x00100000);
onChanged();
return this;
}
/**
*
* /
*
*
* string primaryListingMarketParticipantId = 228;
* @param value The bytes for primaryListingMarketParticipantId to set.
* @return This builder for chaining.
*/
public Builder setPrimaryListingMarketParticipantIdBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
primaryListingMarketParticipantId_ = value;
bitField1_ |= 0x00100000;
onChanged();
return this;
}
private java.lang.Object subscriptionSymbol_ = "";
/**
*
* /
*
*
* string subscriptionSymbol = 229;
* @return The subscriptionSymbol.
*/
public java.lang.String getSubscriptionSymbol() {
java.lang.Object ref = subscriptionSymbol_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
subscriptionSymbol_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* /
*
*
* string subscriptionSymbol = 229;
* @return The bytes for subscriptionSymbol.
*/
public com.google.protobuf.ByteString
getSubscriptionSymbolBytes() {
java.lang.Object ref = subscriptionSymbol_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
subscriptionSymbol_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* /
*
*
* string subscriptionSymbol = 229;
* @param value The subscriptionSymbol to set.
* @return This builder for chaining.
*/
public Builder setSubscriptionSymbol(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
subscriptionSymbol_ = value;
bitField1_ |= 0x00200000;
onChanged();
return this;
}
/**
*
* /
*
*
* string subscriptionSymbol = 229;
* @return This builder for chaining.
*/
public Builder clearSubscriptionSymbol() {
subscriptionSymbol_ = getDefaultInstance().getSubscriptionSymbol();
bitField1_ = (bitField1_ & ~0x00200000);
onChanged();
return this;
}
/**
*
* /
*
*
* string subscriptionSymbol = 229;
* @param value The bytes for subscriptionSymbol to set.
* @return This builder for chaining.
*/
public Builder setSubscriptionSymbolBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
subscriptionSymbol_ = value;
bitField1_ |= 0x00200000;
onChanged();
return this;
}
private org.openfeed.InstrumentDefinition.MaturityDate contractMaturity_;
private com.google.protobuf.SingleFieldBuilder<
org.openfeed.InstrumentDefinition.MaturityDate, org.openfeed.InstrumentDefinition.MaturityDate.Builder, org.openfeed.InstrumentDefinition.MaturityDateOrBuilder> contractMaturityBuilder_;
/**
*
* / The Month/ Day of expiration for futures and options. Corresponds to the expiration month.
*
*
* .org.openfeed.InstrumentDefinition.MaturityDate contractMaturity = 230;
* @return Whether the contractMaturity field is set.
*/
public boolean hasContractMaturity() {
return ((bitField1_ & 0x00400000) != 0);
}
/**
*
* / The Month/ Day of expiration for futures and options. Corresponds to the expiration month.
*
*
* .org.openfeed.InstrumentDefinition.MaturityDate contractMaturity = 230;
* @return The contractMaturity.
*/
public org.openfeed.InstrumentDefinition.MaturityDate getContractMaturity() {
if (contractMaturityBuilder_ == null) {
return contractMaturity_ == null ? org.openfeed.InstrumentDefinition.MaturityDate.getDefaultInstance() : contractMaturity_;
} else {
return contractMaturityBuilder_.getMessage();
}
}
/**
*
* / The Month/ Day of expiration for futures and options. Corresponds to the expiration month.
*
*
* .org.openfeed.InstrumentDefinition.MaturityDate contractMaturity = 230;
*/
public Builder setContractMaturity(org.openfeed.InstrumentDefinition.MaturityDate value) {
if (contractMaturityBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
contractMaturity_ = value;
} else {
contractMaturityBuilder_.setMessage(value);
}
bitField1_ |= 0x00400000;
onChanged();
return this;
}
/**
*
* / The Month/ Day of expiration for futures and options. Corresponds to the expiration month.
*
*
* .org.openfeed.InstrumentDefinition.MaturityDate contractMaturity = 230;
*/
public Builder setContractMaturity(
org.openfeed.InstrumentDefinition.MaturityDate.Builder builderForValue) {
if (contractMaturityBuilder_ == null) {
contractMaturity_ = builderForValue.build();
} else {
contractMaturityBuilder_.setMessage(builderForValue.build());
}
bitField1_ |= 0x00400000;
onChanged();
return this;
}
/**
*
* / The Month/ Day of expiration for futures and options. Corresponds to the expiration month.
*
*
* .org.openfeed.InstrumentDefinition.MaturityDate contractMaturity = 230;
*/
public Builder mergeContractMaturity(org.openfeed.InstrumentDefinition.MaturityDate value) {
if (contractMaturityBuilder_ == null) {
if (((bitField1_ & 0x00400000) != 0) &&
contractMaturity_ != null &&
contractMaturity_ != org.openfeed.InstrumentDefinition.MaturityDate.getDefaultInstance()) {
getContractMaturityBuilder().mergeFrom(value);
} else {
contractMaturity_ = value;
}
} else {
contractMaturityBuilder_.mergeFrom(value);
}
if (contractMaturity_ != null) {
bitField1_ |= 0x00400000;
onChanged();
}
return this;
}
/**
*
* / The Month/ Day of expiration for futures and options. Corresponds to the expiration month.
*
*
* .org.openfeed.InstrumentDefinition.MaturityDate contractMaturity = 230;
*/
public Builder clearContractMaturity() {
bitField1_ = (bitField1_ & ~0x00400000);
contractMaturity_ = null;
if (contractMaturityBuilder_ != null) {
contractMaturityBuilder_.dispose();
contractMaturityBuilder_ = null;
}
onChanged();
return this;
}
/**
*
* / The Month/ Day of expiration for futures and options. Corresponds to the expiration month.
*
*
* .org.openfeed.InstrumentDefinition.MaturityDate contractMaturity = 230;
*/
public org.openfeed.InstrumentDefinition.MaturityDate.Builder getContractMaturityBuilder() {
bitField1_ |= 0x00400000;
onChanged();
return getContractMaturityFieldBuilder().getBuilder();
}
/**
*
* / The Month/ Day of expiration for futures and options. Corresponds to the expiration month.
*
*
* .org.openfeed.InstrumentDefinition.MaturityDate contractMaturity = 230;
*/
public org.openfeed.InstrumentDefinition.MaturityDateOrBuilder getContractMaturityOrBuilder() {
if (contractMaturityBuilder_ != null) {
return contractMaturityBuilder_.getMessageOrBuilder();
} else {
return contractMaturity_ == null ?
org.openfeed.InstrumentDefinition.MaturityDate.getDefaultInstance() : contractMaturity_;
}
}
/**
*
* / The Month/ Day of expiration for futures and options. Corresponds to the expiration month.
*
*
* .org.openfeed.InstrumentDefinition.MaturityDate contractMaturity = 230;
*/
private com.google.protobuf.SingleFieldBuilder<
org.openfeed.InstrumentDefinition.MaturityDate, org.openfeed.InstrumentDefinition.MaturityDate.Builder, org.openfeed.InstrumentDefinition.MaturityDateOrBuilder>
getContractMaturityFieldBuilder() {
if (contractMaturityBuilder_ == null) {
contractMaturityBuilder_ = new com.google.protobuf.SingleFieldBuilder<
org.openfeed.InstrumentDefinition.MaturityDate, org.openfeed.InstrumentDefinition.MaturityDate.Builder, org.openfeed.InstrumentDefinition.MaturityDateOrBuilder>(
getContractMaturity(),
getParentForChildren(),
isClean());
contractMaturity_ = null;
}
return contractMaturityBuilder_;
}
private java.lang.Object underlying_ = "";
/**
*
* / Barchart Underlying Symbol
*
*
* string underlying = 231;
* @return The underlying.
*/
public java.lang.String getUnderlying() {
java.lang.Object ref = underlying_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
underlying_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
*
* / Barchart Underlying Symbol
*
*
* string underlying = 231;
* @return The bytes for underlying.
*/
public com.google.protobuf.ByteString
getUnderlyingBytes() {
java.lang.Object ref = underlying_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
underlying_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
*
* / Barchart Underlying Symbol
*
*
* string underlying = 231;
* @param value The underlying to set.
* @return This builder for chaining.
*/
public Builder setUnderlying(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
underlying_ = value;
bitField1_ |= 0x00800000;
onChanged();
return this;
}
/**
*
* / Barchart Underlying Symbol
*
*
* string underlying = 231;
* @return This builder for chaining.
*/
public Builder clearUnderlying() {
underlying_ = getDefaultInstance().getUnderlying();
bitField1_ = (bitField1_ & ~0x00800000);
onChanged();
return this;
}
/**
*
* / Barchart Underlying Symbol
*
*
* string underlying = 231;
* @param value The bytes for underlying to set.
* @return This builder for chaining.
*/
public Builder setUnderlyingBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
underlying_ = value;
bitField1_ |= 0x00800000;
onChanged();
return this;
}
private java.lang.Object commodity_ = "";
/**
* string commodity = 232;
* @return The commodity.
*/
public java.lang.String getCommodity() {
java.lang.Object ref = commodity_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
commodity_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string commodity = 232;
* @return The bytes for commodity.
*/
public com.google.protobuf.ByteString
getCommodityBytes() {
java.lang.Object ref = commodity_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
commodity_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string commodity = 232;
* @param value The commodity to set.
* @return This builder for chaining.
*/
public Builder setCommodity(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
commodity_ = value;
bitField1_ |= 0x01000000;
onChanged();
return this;
}
/**
* string commodity = 232;
* @return This builder for chaining.
*/
public Builder clearCommodity() {
commodity_ = getDefaultInstance().getCommodity();
bitField1_ = (bitField1_ & ~0x01000000);
onChanged();
return this;
}
/**
* string commodity = 232;
* @param value The bytes for commodity to set.
* @return This builder for chaining.
*/
public Builder setCommodityBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
commodity_ = value;
bitField1_ |= 0x01000000;
onChanged();
return this;
}
private int exchangeId_ ;
/**
*
* / Barchart Exchange Id
*
*
* sint32 exchangeId = 233;
* @return The exchangeId.
*/
@java.lang.Override
public int getExchangeId() {
return exchangeId_;
}
/**
*
* / Barchart Exchange Id
*
*
* sint32 exchangeId = 233;
* @param value The exchangeId to set.
* @return This builder for chaining.
*/
public Builder setExchangeId(int value) {
exchangeId_ = value;
bitField1_ |= 0x02000000;
onChanged();
return this;
}
/**
*
* / Barchart Exchange Id
*
*
* sint32 exchangeId = 233;
* @return This builder for chaining.
*/
public Builder clearExchangeId() {
bitField1_ = (bitField1_ & ~0x02000000);
exchangeId_ = 0;
onChanged();
return this;
}
private int priceScalingExponent_ ;
/**
*
* / Barchart Price Scaling Exponent
*
*
* sint32 priceScalingExponent = 234;
* @return The priceScalingExponent.
*/
@java.lang.Override
public int getPriceScalingExponent() {
return priceScalingExponent_;
}
/**
*
* / Barchart Price Scaling Exponent
*
*
* sint32 priceScalingExponent = 234;
* @param value The priceScalingExponent to set.
* @return This builder for chaining.
*/
public Builder setPriceScalingExponent(int value) {
priceScalingExponent_ = value;
bitField1_ |= 0x04000000;
onChanged();
return this;
}
/**
*
* / Barchart Price Scaling Exponent
*
*
* sint32 priceScalingExponent = 234;
* @return This builder for chaining.
*/
public Builder clearPriceScalingExponent() {
bitField1_ = (bitField1_ & ~0x04000000);
priceScalingExponent_ = 0;
onChanged();
return this;
}
private long underlyingOpenfeedMarketId_ ;
/**
*
* / The Openfeed marketId of the underlying asset.
*
*
* sint64 underlyingOpenfeedMarketId = 235;
* @return The underlyingOpenfeedMarketId.
*/
@java.lang.Override
public long getUnderlyingOpenfeedMarketId() {
return underlyingOpenfeedMarketId_;
}
/**
*
* / The Openfeed marketId of the underlying asset.
*
*
* sint64 underlyingOpenfeedMarketId = 235;
* @param value The underlyingOpenfeedMarketId to set.
* @return This builder for chaining.
*/
public Builder setUnderlyingOpenfeedMarketId(long value) {
underlyingOpenfeedMarketId_ = value;
bitField1_ |= 0x08000000;
onChanged();
return this;
}
/**
*
* / The Openfeed marketId of the underlying asset.
*
*
* sint64 underlyingOpenfeedMarketId = 235;
* @return This builder for chaining.
*/
public Builder clearUnderlyingOpenfeedMarketId() {
bitField1_ = (bitField1_ & ~0x08000000);
underlyingOpenfeedMarketId_ = 0L;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:org.openfeed.InstrumentDefinition)
}
// @@protoc_insertion_point(class_scope:org.openfeed.InstrumentDefinition)
private static final org.openfeed.InstrumentDefinition DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.openfeed.InstrumentDefinition();
}
public static org.openfeed.InstrumentDefinition getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public InstrumentDefinition parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.openfeed.InstrumentDefinition getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy