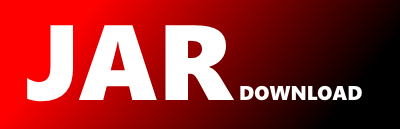
org.openfeed.InstrumentTradingStatus Maven / Gradle / Ivy
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// NO CHECKED-IN PROTOBUF GENCODE
// source: openfeed.proto
// Protobuf Java Version: 4.28.3
package org.openfeed;
/**
* Protobuf enum {@code org.openfeed.InstrumentTradingStatus}
*/
public enum InstrumentTradingStatus
implements com.google.protobuf.ProtocolMessageEnum {
/**
* UNKNOWN_TRADING_STATUS = 0;
*/
UNKNOWN_TRADING_STATUS(0),
/**
* TRADING_RESUME = 1;
*/
TRADING_RESUME(1),
/**
* PRE_OPEN = 2;
*/
PRE_OPEN(2),
/**
* OPEN = 3;
*/
OPEN(3),
/**
* PRE_CLOSE = 4;
*/
PRE_CLOSE(4),
/**
* CLOSE = 5;
*/
CLOSE(5),
/**
* TRADING_HALT = 6;
*/
TRADING_HALT(6),
/**
* QUOTATION_RESUME = 7;
*/
QUOTATION_RESUME(7),
/**
* OPEN_DELAY = 8;
*/
OPEN_DELAY(8),
/**
* NO_OPEN_NO_RESUME = 9;
*/
NO_OPEN_NO_RESUME(9),
/**
* FAST_MARKET = 10;
*/
FAST_MARKET(10),
/**
* FAST_MARKET_END = 11;
*/
FAST_MARKET_END(11),
/**
* LATE_MARKET = 12;
*/
LATE_MARKET(12),
/**
* LATE_MARKET_END = 13;
*/
LATE_MARKET_END(13),
/**
* POST_SESSION = 14;
*/
POST_SESSION(14),
/**
* POST_SESSION_END = 15;
*/
POST_SESSION_END(15),
/**
* NEW_PRICE_INDICATION = 16;
*/
NEW_PRICE_INDICATION(16),
/**
* NOT_AVAILABLE_FOR_TRADING = 17;
*/
NOT_AVAILABLE_FOR_TRADING(17),
/**
* PRE_CROSS = 18;
*/
PRE_CROSS(18),
/**
* CROSS = 19;
*/
CROSS(19),
/**
* POST_CLOSE = 20;
*/
POST_CLOSE(20),
/**
* NO_CHANGE = 21;
*/
NO_CHANGE(21),
/**
*
* Not available for trading.
*
*
* NAFT = 22;
*/
NAFT(22),
/**
* TRADING_RANGE_INDICATION = 23;
*/
TRADING_RANGE_INDICATION(23),
/**
* MARKET_IMBALANCE_BUY = 24;
*/
MARKET_IMBALANCE_BUY(24),
/**
* MARKET_IMBALANCE_SELL = 25;
*/
MARKET_IMBALANCE_SELL(25),
/**
*
* Market On Close Imbalance Buy
*
*
* MOC_IMBALANCE_BUY = 26;
*/
MOC_IMBALANCE_BUY(26),
/**
* MOC_IMBALANCE_SELL = 27;
*/
MOC_IMBALANCE_SELL(27),
/**
* NO_MARKET_IMBALANCE = 28;
*/
NO_MARKET_IMBALANCE(28),
/**
* NO_MOC_IMBALANCE = 29;
*/
NO_MOC_IMBALANCE(29),
/**
* SHORT_SELL_RESTRICTION = 30;
*/
SHORT_SELL_RESTRICTION(30),
/**
* LIMIT_UP_LIMIT_DOWN = 31;
*/
LIMIT_UP_LIMIT_DOWN(31),
UNRECOGNIZED(-1),
;
static {
com.google.protobuf.RuntimeVersion.validateProtobufGencodeVersion(
com.google.protobuf.RuntimeVersion.RuntimeDomain.PUBLIC,
/* major= */ 4,
/* minor= */ 28,
/* patch= */ 3,
/* suffix= */ "",
InstrumentTradingStatus.class.getName());
}
/**
* UNKNOWN_TRADING_STATUS = 0;
*/
public static final int UNKNOWN_TRADING_STATUS_VALUE = 0;
/**
* TRADING_RESUME = 1;
*/
public static final int TRADING_RESUME_VALUE = 1;
/**
* PRE_OPEN = 2;
*/
public static final int PRE_OPEN_VALUE = 2;
/**
* OPEN = 3;
*/
public static final int OPEN_VALUE = 3;
/**
* PRE_CLOSE = 4;
*/
public static final int PRE_CLOSE_VALUE = 4;
/**
* CLOSE = 5;
*/
public static final int CLOSE_VALUE = 5;
/**
* TRADING_HALT = 6;
*/
public static final int TRADING_HALT_VALUE = 6;
/**
* QUOTATION_RESUME = 7;
*/
public static final int QUOTATION_RESUME_VALUE = 7;
/**
* OPEN_DELAY = 8;
*/
public static final int OPEN_DELAY_VALUE = 8;
/**
* NO_OPEN_NO_RESUME = 9;
*/
public static final int NO_OPEN_NO_RESUME_VALUE = 9;
/**
* FAST_MARKET = 10;
*/
public static final int FAST_MARKET_VALUE = 10;
/**
* FAST_MARKET_END = 11;
*/
public static final int FAST_MARKET_END_VALUE = 11;
/**
* LATE_MARKET = 12;
*/
public static final int LATE_MARKET_VALUE = 12;
/**
* LATE_MARKET_END = 13;
*/
public static final int LATE_MARKET_END_VALUE = 13;
/**
* POST_SESSION = 14;
*/
public static final int POST_SESSION_VALUE = 14;
/**
* POST_SESSION_END = 15;
*/
public static final int POST_SESSION_END_VALUE = 15;
/**
* NEW_PRICE_INDICATION = 16;
*/
public static final int NEW_PRICE_INDICATION_VALUE = 16;
/**
* NOT_AVAILABLE_FOR_TRADING = 17;
*/
public static final int NOT_AVAILABLE_FOR_TRADING_VALUE = 17;
/**
* PRE_CROSS = 18;
*/
public static final int PRE_CROSS_VALUE = 18;
/**
* CROSS = 19;
*/
public static final int CROSS_VALUE = 19;
/**
* POST_CLOSE = 20;
*/
public static final int POST_CLOSE_VALUE = 20;
/**
* NO_CHANGE = 21;
*/
public static final int NO_CHANGE_VALUE = 21;
/**
*
* Not available for trading.
*
*
* NAFT = 22;
*/
public static final int NAFT_VALUE = 22;
/**
* TRADING_RANGE_INDICATION = 23;
*/
public static final int TRADING_RANGE_INDICATION_VALUE = 23;
/**
* MARKET_IMBALANCE_BUY = 24;
*/
public static final int MARKET_IMBALANCE_BUY_VALUE = 24;
/**
* MARKET_IMBALANCE_SELL = 25;
*/
public static final int MARKET_IMBALANCE_SELL_VALUE = 25;
/**
*
* Market On Close Imbalance Buy
*
*
* MOC_IMBALANCE_BUY = 26;
*/
public static final int MOC_IMBALANCE_BUY_VALUE = 26;
/**
* MOC_IMBALANCE_SELL = 27;
*/
public static final int MOC_IMBALANCE_SELL_VALUE = 27;
/**
* NO_MARKET_IMBALANCE = 28;
*/
public static final int NO_MARKET_IMBALANCE_VALUE = 28;
/**
* NO_MOC_IMBALANCE = 29;
*/
public static final int NO_MOC_IMBALANCE_VALUE = 29;
/**
* SHORT_SELL_RESTRICTION = 30;
*/
public static final int SHORT_SELL_RESTRICTION_VALUE = 30;
/**
* LIMIT_UP_LIMIT_DOWN = 31;
*/
public static final int LIMIT_UP_LIMIT_DOWN_VALUE = 31;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static InstrumentTradingStatus valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static InstrumentTradingStatus forNumber(int value) {
switch (value) {
case 0: return UNKNOWN_TRADING_STATUS;
case 1: return TRADING_RESUME;
case 2: return PRE_OPEN;
case 3: return OPEN;
case 4: return PRE_CLOSE;
case 5: return CLOSE;
case 6: return TRADING_HALT;
case 7: return QUOTATION_RESUME;
case 8: return OPEN_DELAY;
case 9: return NO_OPEN_NO_RESUME;
case 10: return FAST_MARKET;
case 11: return FAST_MARKET_END;
case 12: return LATE_MARKET;
case 13: return LATE_MARKET_END;
case 14: return POST_SESSION;
case 15: return POST_SESSION_END;
case 16: return NEW_PRICE_INDICATION;
case 17: return NOT_AVAILABLE_FOR_TRADING;
case 18: return PRE_CROSS;
case 19: return CROSS;
case 20: return POST_CLOSE;
case 21: return NO_CHANGE;
case 22: return NAFT;
case 23: return TRADING_RANGE_INDICATION;
case 24: return MARKET_IMBALANCE_BUY;
case 25: return MARKET_IMBALANCE_SELL;
case 26: return MOC_IMBALANCE_BUY;
case 27: return MOC_IMBALANCE_SELL;
case 28: return NO_MARKET_IMBALANCE;
case 29: return NO_MOC_IMBALANCE;
case 30: return SHORT_SELL_RESTRICTION;
case 31: return LIMIT_UP_LIMIT_DOWN;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
InstrumentTradingStatus> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public InstrumentTradingStatus findValueByNumber(int number) {
return InstrumentTradingStatus.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return org.openfeed.Openfeed.getDescriptor().getEnumTypes().get(1);
}
private static final InstrumentTradingStatus[] VALUES = values();
public static InstrumentTradingStatus valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private InstrumentTradingStatus(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:org.openfeed.InstrumentTradingStatus)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy