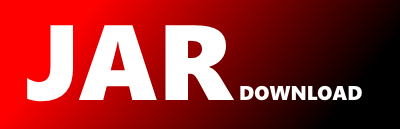
org.openfeed.MarketSnapshotOrBuilder Maven / Gradle / Ivy
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// NO CHECKED-IN PROTOBUF GENCODE
// source: openfeed.proto
// Protobuf Java Version: 4.28.3
package org.openfeed;
public interface MarketSnapshotOrBuilder extends
// @@protoc_insertion_point(interface_extends:org.openfeed.MarketSnapshot)
com.google.protobuf.MessageOrBuilder {
/**
*
* / Unique id identifying the market
*
*
* sint64 marketId = 1;
* @return The marketId.
*/
long getMarketId();
/**
*
* UTC Timestamp of transaction, nano seconds since Unix epoch
*
*
* sint64 transactionTime = 2;
* @return The transactionTime.
*/
long getTransactionTime();
/**
*
* Instrument level sequence number
*
*
* int64 marketSequence = 3;
* @return The marketSequence.
*/
long getMarketSequence();
/**
*
* / Date only, format 2012-07-04 -> 20120704
*
*
* sint32 tradeDate = 4;
* @return The tradeDate.
*/
int getTradeDate();
/**
*
* / A snapshot with market depth may exceed the maximum message size.
* In that case, the snapshot will be broken up across multiple
* snapshot messages.
*
*
* sint32 totalChunks = 5;
* @return The totalChunks.
*/
int getTotalChunks();
/**
* sint32 currentChunk = 6;
* @return The currentChunk.
*/
int getCurrentChunk();
/**
*
* Optional symbol identifier
*
*
* string symbol = 7;
* @return The symbol.
*/
java.lang.String getSymbol();
/**
*
* Optional symbol identifier
*
*
* string symbol = 7;
* @return The bytes for symbol.
*/
com.google.protobuf.ByteString
getSymbolBytes();
/**
*
* / Divide prices by this value to get real price values. Optional, use value
* / from InstrumentDefinition if not set.
*
*
* sint32 priceDenominator = 8;
* @return The priceDenominator.
*/
int getPriceDenominator();
/**
*
*
*
*
* .org.openfeed.Service service = 9;
* @return The enum numeric value on the wire for service.
*/
int getServiceValue();
/**
*
*
*
*
* .org.openfeed.Service service = 9;
* @return The service.
*/
org.openfeed.Service getService();
/**
*
*
*
*
* .org.openfeed.InstrumentStatus instrumentStatus = 10;
* @return Whether the instrumentStatus field is set.
*/
boolean hasInstrumentStatus();
/**
*
*
*
*
* .org.openfeed.InstrumentStatus instrumentStatus = 10;
* @return The instrumentStatus.
*/
org.openfeed.InstrumentStatus getInstrumentStatus();
/**
*
*
*
*
* .org.openfeed.InstrumentStatus instrumentStatus = 10;
*/
org.openfeed.InstrumentStatusOrBuilder getInstrumentStatusOrBuilder();
/**
*
* Best Bid Offer
*
*
* .org.openfeed.BestBidOffer bbo = 11;
* @return Whether the bbo field is set.
*/
boolean hasBbo();
/**
*
* Best Bid Offer
*
*
* .org.openfeed.BestBidOffer bbo = 11;
* @return The bbo.
*/
org.openfeed.BestBidOffer getBbo();
/**
*
* Best Bid Offer
*
*
* .org.openfeed.BestBidOffer bbo = 11;
*/
org.openfeed.BestBidOfferOrBuilder getBboOrBuilder();
/**
*
* Index Value
*
*
* .org.openfeed.IndexValue index = 12;
* @return Whether the index field is set.
*/
boolean hasIndex();
/**
*
* Index Value
*
*
* .org.openfeed.IndexValue index = 12;
* @return The index.
*/
org.openfeed.IndexValue getIndex();
/**
*
* Index Value
*
*
* .org.openfeed.IndexValue index = 12;
*/
org.openfeed.IndexValueOrBuilder getIndexOrBuilder();
/**
*
* Price Level Book
*
*
* repeated .org.openfeed.AddPriceLevel priceLevels = 13;
*/
java.util.List
getPriceLevelsList();
/**
*
* Price Level Book
*
*
* repeated .org.openfeed.AddPriceLevel priceLevels = 13;
*/
org.openfeed.AddPriceLevel getPriceLevels(int index);
/**
*
* Price Level Book
*
*
* repeated .org.openfeed.AddPriceLevel priceLevels = 13;
*/
int getPriceLevelsCount();
/**
*
* Price Level Book
*
*
* repeated .org.openfeed.AddPriceLevel priceLevels = 13;
*/
java.util.List extends org.openfeed.AddPriceLevelOrBuilder>
getPriceLevelsOrBuilderList();
/**
*
* Price Level Book
*
*
* repeated .org.openfeed.AddPriceLevel priceLevels = 13;
*/
org.openfeed.AddPriceLevelOrBuilder getPriceLevelsOrBuilder(
int index);
/**
*
* Order Book
*
*
* repeated .org.openfeed.AddOrder orders = 14;
*/
java.util.List
getOrdersList();
/**
*
* Order Book
*
*
* repeated .org.openfeed.AddOrder orders = 14;
*/
org.openfeed.AddOrder getOrders(int index);
/**
*
* Order Book
*
*
* repeated .org.openfeed.AddOrder orders = 14;
*/
int getOrdersCount();
/**
*
* Order Book
*
*
* repeated .org.openfeed.AddOrder orders = 14;
*/
java.util.List extends org.openfeed.AddOrderOrBuilder>
getOrdersOrBuilderList();
/**
*
* Order Book
*
*
* repeated .org.openfeed.AddOrder orders = 14;
*/
org.openfeed.AddOrderOrBuilder getOrdersOrBuilder(
int index);
/**
* .org.openfeed.News news = 15;
* @return Whether the news field is set.
*/
boolean hasNews();
/**
* .org.openfeed.News news = 15;
* @return The news.
*/
org.openfeed.News getNews();
/**
* .org.openfeed.News news = 15;
*/
org.openfeed.NewsOrBuilder getNewsOrBuilder();
/**
*
* / Most recent opening price
*
*
* .org.openfeed.Open open = 30;
* @return Whether the open field is set.
*/
boolean hasOpen();
/**
*
* / Most recent opening price
*
*
* .org.openfeed.Open open = 30;
* @return The open.
*/
org.openfeed.Open getOpen();
/**
*
* / Most recent opening price
*
*
* .org.openfeed.Open open = 30;
*/
org.openfeed.OpenOrBuilder getOpenOrBuilder();
/**
*
* / High price for the trading session
*
*
* .org.openfeed.High high = 31;
* @return Whether the high field is set.
*/
boolean hasHigh();
/**
*
* / High price for the trading session
*
*
* .org.openfeed.High high = 31;
* @return The high.
*/
org.openfeed.High getHigh();
/**
*
* / High price for the trading session
*
*
* .org.openfeed.High high = 31;
*/
org.openfeed.HighOrBuilder getHighOrBuilder();
/**
*
* / Low price for the trading session
*
*
* .org.openfeed.Low low = 32;
* @return Whether the low field is set.
*/
boolean hasLow();
/**
*
* / Low price for the trading session
*
*
* .org.openfeed.Low low = 32;
* @return The low.
*/
org.openfeed.Low getLow();
/**
*
* / Low price for the trading session
*
*
* .org.openfeed.Low low = 32;
*/
org.openfeed.LowOrBuilder getLowOrBuilder();
/**
*
* / Most recent closing price
*
*
* .org.openfeed.Close close = 33;
* @return Whether the close field is set.
*/
boolean hasClose();
/**
*
* / Most recent closing price
*
*
* .org.openfeed.Close close = 33;
* @return The close.
*/
org.openfeed.Close getClose();
/**
*
* / Most recent closing price
*
*
* .org.openfeed.Close close = 33;
*/
org.openfeed.CloseOrBuilder getCloseOrBuilder();
/**
*
* / Previous closing price
*
*
* .org.openfeed.PrevClose prevClose = 34;
* @return Whether the prevClose field is set.
*/
boolean hasPrevClose();
/**
*
* / Previous closing price
*
*
* .org.openfeed.PrevClose prevClose = 34;
* @return The prevClose.
*/
org.openfeed.PrevClose getPrevClose();
/**
*
* / Previous closing price
*
*
* .org.openfeed.PrevClose prevClose = 34;
*/
org.openfeed.PrevCloseOrBuilder getPrevCloseOrBuilder();
/**
*
* / Most recent traded price and quantity
*
*
* .org.openfeed.Last last = 35;
* @return Whether the last field is set.
*/
boolean hasLast();
/**
*
* / Most recent traded price and quantity
*
*
* .org.openfeed.Last last = 35;
* @return The last.
*/
org.openfeed.Last getLast();
/**
*
* / Most recent traded price and quantity
*
*
* .org.openfeed.Last last = 35;
*/
org.openfeed.LastOrBuilder getLastOrBuilder();
/**
*
* / Year high price
*
*
* .org.openfeed.YearHigh yearHigh = 36;
* @return Whether the yearHigh field is set.
*/
boolean hasYearHigh();
/**
*
* / Year high price
*
*
* .org.openfeed.YearHigh yearHigh = 36;
* @return The yearHigh.
*/
org.openfeed.YearHigh getYearHigh();
/**
*
* / Year high price
*
*
* .org.openfeed.YearHigh yearHigh = 36;
*/
org.openfeed.YearHighOrBuilder getYearHighOrBuilder();
/**
*
* / Year low price
*
*
* .org.openfeed.YearLow yearLow = 37;
* @return Whether the yearLow field is set.
*/
boolean hasYearLow();
/**
*
* / Year low price
*
*
* .org.openfeed.YearLow yearLow = 37;
* @return The yearLow.
*/
org.openfeed.YearLow getYearLow();
/**
*
* / Year low price
*
*
* .org.openfeed.YearLow yearLow = 37;
*/
org.openfeed.YearLowOrBuilder getYearLowOrBuilder();
/**
*
* / Total traded volume
*
*
* .org.openfeed.Volume volume = 38;
* @return Whether the volume field is set.
*/
boolean hasVolume();
/**
*
* / Total traded volume
*
*
* .org.openfeed.Volume volume = 38;
* @return The volume.
*/
org.openfeed.Volume getVolume();
/**
*
* / Total traded volume
*
*
* .org.openfeed.Volume volume = 38;
*/
org.openfeed.VolumeOrBuilder getVolumeOrBuilder();
/**
*
* / Most recent settlement price
*
*
* .org.openfeed.Settlement settlement = 39;
* @return Whether the settlement field is set.
*/
boolean hasSettlement();
/**
*
* / Most recent settlement price
*
*
* .org.openfeed.Settlement settlement = 39;
* @return The settlement.
*/
org.openfeed.Settlement getSettlement();
/**
*
* / Most recent settlement price
*
*
* .org.openfeed.Settlement settlement = 39;
*/
org.openfeed.SettlementOrBuilder getSettlementOrBuilder();
/**
*
* / Most recent open interest
*
*
* .org.openfeed.OpenInterest openInterest = 40;
* @return Whether the openInterest field is set.
*/
boolean hasOpenInterest();
/**
*
* / Most recent open interest
*
*
* .org.openfeed.OpenInterest openInterest = 40;
* @return The openInterest.
*/
org.openfeed.OpenInterest getOpenInterest();
/**
*
* / Most recent open interest
*
*
* .org.openfeed.OpenInterest openInterest = 40;
*/
org.openfeed.OpenInterestOrBuilder getOpenInterestOrBuilder();
/**
*
* / Most recent volume weighted average price
*
*
* .org.openfeed.Vwap vwap = 41;
* @return Whether the vwap field is set.
*/
boolean hasVwap();
/**
*
* / Most recent volume weighted average price
*
*
* .org.openfeed.Vwap vwap = 41;
* @return The vwap.
*/
org.openfeed.Vwap getVwap();
/**
*
* / Most recent volume weighted average price
*
*
* .org.openfeed.Vwap vwap = 41;
*/
org.openfeed.VwapOrBuilder getVwapOrBuilder();
/**
* .org.openfeed.DividendsIncomeDistributions dividendsIncomeDistributions = 42;
* @return Whether the dividendsIncomeDistributions field is set.
*/
boolean hasDividendsIncomeDistributions();
/**
* .org.openfeed.DividendsIncomeDistributions dividendsIncomeDistributions = 42;
* @return The dividendsIncomeDistributions.
*/
org.openfeed.DividendsIncomeDistributions getDividendsIncomeDistributions();
/**
* .org.openfeed.DividendsIncomeDistributions dividendsIncomeDistributions = 42;
*/
org.openfeed.DividendsIncomeDistributionsOrBuilder getDividendsIncomeDistributionsOrBuilder();
/**
* .org.openfeed.NumberOfTrades numberOfTrades = 43;
* @return Whether the numberOfTrades field is set.
*/
boolean hasNumberOfTrades();
/**
* .org.openfeed.NumberOfTrades numberOfTrades = 43;
* @return The numberOfTrades.
*/
org.openfeed.NumberOfTrades getNumberOfTrades();
/**
* .org.openfeed.NumberOfTrades numberOfTrades = 43;
*/
org.openfeed.NumberOfTradesOrBuilder getNumberOfTradesOrBuilder();
/**
* .org.openfeed.MonetaryValue monetaryValue = 44;
* @return Whether the monetaryValue field is set.
*/
boolean hasMonetaryValue();
/**
* .org.openfeed.MonetaryValue monetaryValue = 44;
* @return The monetaryValue.
*/
org.openfeed.MonetaryValue getMonetaryValue();
/**
* .org.openfeed.MonetaryValue monetaryValue = 44;
*/
org.openfeed.MonetaryValueOrBuilder getMonetaryValueOrBuilder();
/**
* .org.openfeed.CapitalDistributions capitalDistributions = 45;
* @return Whether the capitalDistributions field is set.
*/
boolean hasCapitalDistributions();
/**
* .org.openfeed.CapitalDistributions capitalDistributions = 45;
* @return The capitalDistributions.
*/
org.openfeed.CapitalDistributions getCapitalDistributions();
/**
* .org.openfeed.CapitalDistributions capitalDistributions = 45;
*/
org.openfeed.CapitalDistributionsOrBuilder getCapitalDistributionsOrBuilder();
/**
* .org.openfeed.SharesOutstanding sharesOutstanding = 46;
* @return Whether the sharesOutstanding field is set.
*/
boolean hasSharesOutstanding();
/**
* .org.openfeed.SharesOutstanding sharesOutstanding = 46;
* @return The sharesOutstanding.
*/
org.openfeed.SharesOutstanding getSharesOutstanding();
/**
* .org.openfeed.SharesOutstanding sharesOutstanding = 46;
*/
org.openfeed.SharesOutstandingOrBuilder getSharesOutstandingOrBuilder();
/**
* .org.openfeed.NetAssetValue netAssetValue = 47;
* @return Whether the netAssetValue field is set.
*/
boolean hasNetAssetValue();
/**
* .org.openfeed.NetAssetValue netAssetValue = 47;
* @return The netAssetValue.
*/
org.openfeed.NetAssetValue getNetAssetValue();
/**
* .org.openfeed.NetAssetValue netAssetValue = 47;
*/
org.openfeed.NetAssetValueOrBuilder getNetAssetValueOrBuilder();
/**
*
* / Previous session.
*
*
* .org.openfeed.MarketSession previousSession = 48;
* @return Whether the previousSession field is set.
*/
boolean hasPreviousSession();
/**
*
* / Previous session.
*
*
* .org.openfeed.MarketSession previousSession = 48;
* @return The previousSession.
*/
org.openfeed.MarketSession getPreviousSession();
/**
*
* / Previous session.
*
*
* .org.openfeed.MarketSession previousSession = 48;
*/
org.openfeed.MarketSessionOrBuilder getPreviousSessionOrBuilder();
/**
*
* / 'T' session.
*
*
* .org.openfeed.MarketSession tSession = 49;
* @return Whether the tSession field is set.
*/
boolean hasTSession();
/**
*
* / 'T' session.
*
*
* .org.openfeed.MarketSession tSession = 49;
* @return The tSession.
*/
org.openfeed.MarketSession getTSession();
/**
*
* / 'T' session.
*
*
* .org.openfeed.MarketSession tSession = 49;
*/
org.openfeed.MarketSessionOrBuilder getTSessionOrBuilder();
/**
*
* / Volume at price. Used by the market state/ JERQ.
*
*
* .org.openfeed.VolumeAtPrice volumeAtPrice = 50;
* @return Whether the volumeAtPrice field is set.
*/
boolean hasVolumeAtPrice();
/**
*
* / Volume at price. Used by the market state/ JERQ.
*
*
* .org.openfeed.VolumeAtPrice volumeAtPrice = 50;
* @return The volumeAtPrice.
*/
org.openfeed.VolumeAtPrice getVolumeAtPrice();
/**
*
* / Volume at price. Used by the market state/ JERQ.
*
*
* .org.openfeed.VolumeAtPrice volumeAtPrice = 50;
*/
org.openfeed.VolumeAtPriceOrBuilder getVolumeAtPriceOrBuilder();
/**
* .org.openfeed.HighRolling highRolling = 51;
* @return Whether the highRolling field is set.
*/
boolean hasHighRolling();
/**
* .org.openfeed.HighRolling highRolling = 51;
* @return The highRolling.
*/
org.openfeed.HighRolling getHighRolling();
/**
* .org.openfeed.HighRolling highRolling = 51;
*/
org.openfeed.HighRollingOrBuilder getHighRollingOrBuilder();
/**
* .org.openfeed.LowRolling lowRolling = 52;
* @return Whether the lowRolling field is set.
*/
boolean hasLowRolling();
/**
* .org.openfeed.LowRolling lowRolling = 52;
* @return The lowRolling.
*/
org.openfeed.LowRolling getLowRolling();
/**
* .org.openfeed.LowRolling lowRolling = 52;
*/
org.openfeed.LowRollingOrBuilder getLowRollingOrBuilder();
/**
*
* / 'Z' session. Includes all trades, even the ones that do not update Last.
*
*
* .org.openfeed.MarketSession zSession = 53;
* @return Whether the zSession field is set.
*/
boolean hasZSession();
/**
*
* / 'Z' session. Includes all trades, even the ones that do not update Last.
*
*
* .org.openfeed.MarketSession zSession = 53;
* @return The zSession.
*/
org.openfeed.MarketSession getZSession();
/**
*
* / 'Z' session. Includes all trades, even the ones that do not update Last.
*
*
* .org.openfeed.MarketSession zSession = 53;
*/
org.openfeed.MarketSessionOrBuilder getZSessionOrBuilder();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy