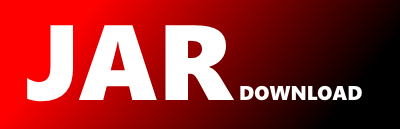
org.openfeed.MarketUpdateOrBuilder Maven / Gradle / Ivy
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// NO CHECKED-IN PROTOBUF GENCODE
// source: openfeed.proto
// Protobuf Java Version: 4.28.3
package org.openfeed;
public interface MarketUpdateOrBuilder extends
// @@protoc_insertion_point(interface_extends:org.openfeed.MarketUpdate)
com.google.protobuf.MessageOrBuilder {
/**
*
* / Unique id identifying the market
*
*
* sint64 marketId = 1;
* @return The marketId.
*/
long getMarketId();
/**
*
* Optional symbol identifier
*
*
* string symbol = 2;
* @return The symbol.
*/
java.lang.String getSymbol();
/**
*
* Optional symbol identifier
*
*
* string symbol = 2;
* @return The bytes for symbol.
*/
com.google.protobuf.ByteString
getSymbolBytes();
/**
*
* / UTC Timestamp of transaction, nano seconds since Unix epoch
* / This is usually the execution venue timestamp.
*
*
* sint64 transactionTime = 3;
* @return The transactionTime.
*/
long getTransactionTime();
/**
*
* / Distribution time in nano seconds since epoch.
*
*
* sint64 distributionTime = 4;
* @return The distributionTime.
*/
long getDistributionTime();
/**
*
* / Market level sequencing number
*
*
* sint64 marketSequence = 5;
* @return The marketSequence.
*/
long getMarketSequence();
/**
*
* / Data source sequence number
*
*
* sint64 sourceSequence = 6;
* @return The sourceSequence.
*/
long getSourceSequence();
/**
*
* Market participant/originator
*
*
* bytes originatorId = 7;
* @return The originatorId.
*/
com.google.protobuf.ByteString getOriginatorId();
/**
*
* / Divide prices by this value to get real price values. Optional, use value
* / from InstrumentDefinition if not set.
*
*
* sint32 priceDenominator = 9;
* @return The priceDenominator.
*/
int getPriceDenominator();
/**
*
* Feed specific context data set as required.
*
*
* .org.openfeed.Context context = 10;
* @return Whether the context field is set.
*/
boolean hasContext();
/**
*
* Feed specific context data set as required.
*
*
* .org.openfeed.Context context = 10;
* @return The context.
*/
org.openfeed.Context getContext();
/**
*
* Feed specific context data set as required.
*
*
* .org.openfeed.Context context = 10;
*/
org.openfeed.ContextOrBuilder getContextOrBuilder();
/**
*
* / Current session. This is used to 'enhance' updates from the translator in the Market State
*
*
* .org.openfeed.MarketSession session = 11;
* @return Whether the session field is set.
*/
boolean hasSession();
/**
*
* / Current session. This is used to 'enhance' updates from the translator in the Market State
*
*
* .org.openfeed.MarketSession session = 11;
* @return The session.
*/
org.openfeed.MarketSession getSession();
/**
*
* / Current session. This is used to 'enhance' updates from the translator in the Market State
*
*
* .org.openfeed.MarketSession session = 11;
*/
org.openfeed.MarketSessionOrBuilder getSessionOrBuilder();
/**
*
* / 'T' session. This is used to 'enhance' updates from the translator in the Market State
*
*
* .org.openfeed.MarketSession tSession = 12;
* @return Whether the tSession field is set.
*/
boolean hasTSession();
/**
*
* / 'T' session. This is used to 'enhance' updates from the translator in the Market State
*
*
* .org.openfeed.MarketSession tSession = 12;
* @return The tSession.
*/
org.openfeed.MarketSession getTSession();
/**
*
* / 'T' session. This is used to 'enhance' updates from the translator in the Market State
*
*
* .org.openfeed.MarketSession tSession = 12;
*/
org.openfeed.MarketSessionOrBuilder getTSessionOrBuilder();
/**
*
* / Previous session. This is used to 'enhance' updates from the translator in the Market State
*
*
* .org.openfeed.MarketSession previousSession = 13;
* @return Whether the previousSession field is set.
*/
boolean hasPreviousSession();
/**
*
* / Previous session. This is used to 'enhance' updates from the translator in the Market State
*
*
* .org.openfeed.MarketSession previousSession = 13;
* @return The previousSession.
*/
org.openfeed.MarketSession getPreviousSession();
/**
*
* / Previous session. This is used to 'enhance' updates from the translator in the Market State
*
*
* .org.openfeed.MarketSession previousSession = 13;
*/
org.openfeed.MarketSessionOrBuilder getPreviousSessionOrBuilder();
/**
*
* / True if message applies to regional/participant member
*
*
* bool regional = 14;
* @return The regional.
*/
boolean getRegional();
/**
*
* / 'Z' session. Includes all trades, even the ones that do not update Last.
*
*
* .org.openfeed.MarketSession zSession = 15;
* @return Whether the zSession field is set.
*/
boolean hasZSession();
/**
*
* / 'Z' session. Includes all trades, even the ones that do not update Last.
*
*
* .org.openfeed.MarketSession zSession = 15;
* @return The zSession.
*/
org.openfeed.MarketSession getZSession();
/**
*
* / 'Z' session. Includes all trades, even the ones that do not update Last.
*
*
* .org.openfeed.MarketSession zSession = 15;
*/
org.openfeed.MarketSessionOrBuilder getZSessionOrBuilder();
/**
* .org.openfeed.News news = 20;
* @return Whether the news field is set.
*/
boolean hasNews();
/**
* .org.openfeed.News news = 20;
* @return The news.
*/
org.openfeed.News getNews();
/**
* .org.openfeed.News news = 20;
*/
org.openfeed.NewsOrBuilder getNewsOrBuilder();
/**
* .org.openfeed.ClearBook clearBook = 21;
* @return Whether the clearBook field is set.
*/
boolean hasClearBook();
/**
* .org.openfeed.ClearBook clearBook = 21;
* @return The clearBook.
*/
org.openfeed.ClearBook getClearBook();
/**
* .org.openfeed.ClearBook clearBook = 21;
*/
org.openfeed.ClearBookOrBuilder getClearBookOrBuilder();
/**
* .org.openfeed.InstrumentStatus instrumentStatus = 22;
* @return Whether the instrumentStatus field is set.
*/
boolean hasInstrumentStatus();
/**
* .org.openfeed.InstrumentStatus instrumentStatus = 22;
* @return The instrumentStatus.
*/
org.openfeed.InstrumentStatus getInstrumentStatus();
/**
* .org.openfeed.InstrumentStatus instrumentStatus = 22;
*/
org.openfeed.InstrumentStatusOrBuilder getInstrumentStatusOrBuilder();
/**
* .org.openfeed.BestBidOffer bbo = 23;
* @return Whether the bbo field is set.
*/
boolean hasBbo();
/**
* .org.openfeed.BestBidOffer bbo = 23;
* @return The bbo.
*/
org.openfeed.BestBidOffer getBbo();
/**
* .org.openfeed.BestBidOffer bbo = 23;
*/
org.openfeed.BestBidOfferOrBuilder getBboOrBuilder();
/**
* .org.openfeed.DepthPriceLevel depthPriceLevel = 24;
* @return Whether the depthPriceLevel field is set.
*/
boolean hasDepthPriceLevel();
/**
* .org.openfeed.DepthPriceLevel depthPriceLevel = 24;
* @return The depthPriceLevel.
*/
org.openfeed.DepthPriceLevel getDepthPriceLevel();
/**
* .org.openfeed.DepthPriceLevel depthPriceLevel = 24;
*/
org.openfeed.DepthPriceLevelOrBuilder getDepthPriceLevelOrBuilder();
/**
* .org.openfeed.DepthOrder depthOrder = 25;
* @return Whether the depthOrder field is set.
*/
boolean hasDepthOrder();
/**
* .org.openfeed.DepthOrder depthOrder = 25;
* @return The depthOrder.
*/
org.openfeed.DepthOrder getDepthOrder();
/**
* .org.openfeed.DepthOrder depthOrder = 25;
*/
org.openfeed.DepthOrderOrBuilder getDepthOrderOrBuilder();
/**
* .org.openfeed.IndexValue index = 26;
* @return Whether the index field is set.
*/
boolean hasIndex();
/**
* .org.openfeed.IndexValue index = 26;
* @return The index.
*/
org.openfeed.IndexValue getIndex();
/**
* .org.openfeed.IndexValue index = 26;
*/
org.openfeed.IndexValueOrBuilder getIndexOrBuilder();
/**
* .org.openfeed.Trades trades = 27;
* @return Whether the trades field is set.
*/
boolean hasTrades();
/**
* .org.openfeed.Trades trades = 27;
* @return The trades.
*/
org.openfeed.Trades getTrades();
/**
* .org.openfeed.Trades trades = 27;
*/
org.openfeed.TradesOrBuilder getTradesOrBuilder();
/**
* .org.openfeed.Open open = 28;
* @return Whether the open field is set.
*/
boolean hasOpen();
/**
* .org.openfeed.Open open = 28;
* @return The open.
*/
org.openfeed.Open getOpen();
/**
* .org.openfeed.Open open = 28;
*/
org.openfeed.OpenOrBuilder getOpenOrBuilder();
/**
* .org.openfeed.High high = 29;
* @return Whether the high field is set.
*/
boolean hasHigh();
/**
* .org.openfeed.High high = 29;
* @return The high.
*/
org.openfeed.High getHigh();
/**
* .org.openfeed.High high = 29;
*/
org.openfeed.HighOrBuilder getHighOrBuilder();
/**
* .org.openfeed.Low low = 30;
* @return Whether the low field is set.
*/
boolean hasLow();
/**
* .org.openfeed.Low low = 30;
* @return The low.
*/
org.openfeed.Low getLow();
/**
* .org.openfeed.Low low = 30;
*/
org.openfeed.LowOrBuilder getLowOrBuilder();
/**
* .org.openfeed.Close close = 31;
* @return Whether the close field is set.
*/
boolean hasClose();
/**
* .org.openfeed.Close close = 31;
* @return The close.
*/
org.openfeed.Close getClose();
/**
* .org.openfeed.Close close = 31;
*/
org.openfeed.CloseOrBuilder getCloseOrBuilder();
/**
* .org.openfeed.PrevClose prevClose = 32;
* @return Whether the prevClose field is set.
*/
boolean hasPrevClose();
/**
* .org.openfeed.PrevClose prevClose = 32;
* @return The prevClose.
*/
org.openfeed.PrevClose getPrevClose();
/**
* .org.openfeed.PrevClose prevClose = 32;
*/
org.openfeed.PrevCloseOrBuilder getPrevCloseOrBuilder();
/**
* .org.openfeed.Last last = 33;
* @return Whether the last field is set.
*/
boolean hasLast();
/**
* .org.openfeed.Last last = 33;
* @return The last.
*/
org.openfeed.Last getLast();
/**
* .org.openfeed.Last last = 33;
*/
org.openfeed.LastOrBuilder getLastOrBuilder();
/**
* .org.openfeed.YearHigh yearHigh = 34;
* @return Whether the yearHigh field is set.
*/
boolean hasYearHigh();
/**
* .org.openfeed.YearHigh yearHigh = 34;
* @return The yearHigh.
*/
org.openfeed.YearHigh getYearHigh();
/**
* .org.openfeed.YearHigh yearHigh = 34;
*/
org.openfeed.YearHighOrBuilder getYearHighOrBuilder();
/**
* .org.openfeed.YearLow yearLow = 35;
* @return Whether the yearLow field is set.
*/
boolean hasYearLow();
/**
* .org.openfeed.YearLow yearLow = 35;
* @return The yearLow.
*/
org.openfeed.YearLow getYearLow();
/**
* .org.openfeed.YearLow yearLow = 35;
*/
org.openfeed.YearLowOrBuilder getYearLowOrBuilder();
/**
* .org.openfeed.Volume volume = 36;
* @return Whether the volume field is set.
*/
boolean hasVolume();
/**
* .org.openfeed.Volume volume = 36;
* @return The volume.
*/
org.openfeed.Volume getVolume();
/**
* .org.openfeed.Volume volume = 36;
*/
org.openfeed.VolumeOrBuilder getVolumeOrBuilder();
/**
* .org.openfeed.Settlement settlement = 37;
* @return Whether the settlement field is set.
*/
boolean hasSettlement();
/**
* .org.openfeed.Settlement settlement = 37;
* @return The settlement.
*/
org.openfeed.Settlement getSettlement();
/**
* .org.openfeed.Settlement settlement = 37;
*/
org.openfeed.SettlementOrBuilder getSettlementOrBuilder();
/**
* .org.openfeed.OpenInterest openInterest = 38;
* @return Whether the openInterest field is set.
*/
boolean hasOpenInterest();
/**
* .org.openfeed.OpenInterest openInterest = 38;
* @return The openInterest.
*/
org.openfeed.OpenInterest getOpenInterest();
/**
* .org.openfeed.OpenInterest openInterest = 38;
*/
org.openfeed.OpenInterestOrBuilder getOpenInterestOrBuilder();
/**
* .org.openfeed.Vwap vwap = 39;
* @return Whether the vwap field is set.
*/
boolean hasVwap();
/**
* .org.openfeed.Vwap vwap = 39;
* @return The vwap.
*/
org.openfeed.Vwap getVwap();
/**
* .org.openfeed.Vwap vwap = 39;
*/
org.openfeed.VwapOrBuilder getVwapOrBuilder();
/**
* .org.openfeed.DividendsIncomeDistributions dividendsIncomeDistributions = 40;
* @return Whether the dividendsIncomeDistributions field is set.
*/
boolean hasDividendsIncomeDistributions();
/**
* .org.openfeed.DividendsIncomeDistributions dividendsIncomeDistributions = 40;
* @return The dividendsIncomeDistributions.
*/
org.openfeed.DividendsIncomeDistributions getDividendsIncomeDistributions();
/**
* .org.openfeed.DividendsIncomeDistributions dividendsIncomeDistributions = 40;
*/
org.openfeed.DividendsIncomeDistributionsOrBuilder getDividendsIncomeDistributionsOrBuilder();
/**
* .org.openfeed.NumberOfTrades numberOfTrades = 41;
* @return Whether the numberOfTrades field is set.
*/
boolean hasNumberOfTrades();
/**
* .org.openfeed.NumberOfTrades numberOfTrades = 41;
* @return The numberOfTrades.
*/
org.openfeed.NumberOfTrades getNumberOfTrades();
/**
* .org.openfeed.NumberOfTrades numberOfTrades = 41;
*/
org.openfeed.NumberOfTradesOrBuilder getNumberOfTradesOrBuilder();
/**
* .org.openfeed.MonetaryValue monetaryValue = 42;
* @return Whether the monetaryValue field is set.
*/
boolean hasMonetaryValue();
/**
* .org.openfeed.MonetaryValue monetaryValue = 42;
* @return The monetaryValue.
*/
org.openfeed.MonetaryValue getMonetaryValue();
/**
* .org.openfeed.MonetaryValue monetaryValue = 42;
*/
org.openfeed.MonetaryValueOrBuilder getMonetaryValueOrBuilder();
/**
* .org.openfeed.CapitalDistributions capitalDistributions = 43;
* @return Whether the capitalDistributions field is set.
*/
boolean hasCapitalDistributions();
/**
* .org.openfeed.CapitalDistributions capitalDistributions = 43;
* @return The capitalDistributions.
*/
org.openfeed.CapitalDistributions getCapitalDistributions();
/**
* .org.openfeed.CapitalDistributions capitalDistributions = 43;
*/
org.openfeed.CapitalDistributionsOrBuilder getCapitalDistributionsOrBuilder();
/**
* .org.openfeed.SharesOutstanding sharesOutstanding = 44;
* @return Whether the sharesOutstanding field is set.
*/
boolean hasSharesOutstanding();
/**
* .org.openfeed.SharesOutstanding sharesOutstanding = 44;
* @return The sharesOutstanding.
*/
org.openfeed.SharesOutstanding getSharesOutstanding();
/**
* .org.openfeed.SharesOutstanding sharesOutstanding = 44;
*/
org.openfeed.SharesOutstandingOrBuilder getSharesOutstandingOrBuilder();
/**
* .org.openfeed.NetAssetValue netAssetValue = 45;
* @return Whether the netAssetValue field is set.
*/
boolean hasNetAssetValue();
/**
* .org.openfeed.NetAssetValue netAssetValue = 45;
* @return The netAssetValue.
*/
org.openfeed.NetAssetValue getNetAssetValue();
/**
* .org.openfeed.NetAssetValue netAssetValue = 45;
*/
org.openfeed.NetAssetValueOrBuilder getNetAssetValueOrBuilder();
/**
* .org.openfeed.MarketSummary marketSummary = 46;
* @return Whether the marketSummary field is set.
*/
boolean hasMarketSummary();
/**
* .org.openfeed.MarketSummary marketSummary = 46;
* @return The marketSummary.
*/
org.openfeed.MarketSummary getMarketSummary();
/**
* .org.openfeed.MarketSummary marketSummary = 46;
*/
org.openfeed.MarketSummaryOrBuilder getMarketSummaryOrBuilder();
/**
* .org.openfeed.HighRolling highRolling = 47;
* @return Whether the highRolling field is set.
*/
boolean hasHighRolling();
/**
* .org.openfeed.HighRolling highRolling = 47;
* @return The highRolling.
*/
org.openfeed.HighRolling getHighRolling();
/**
* .org.openfeed.HighRolling highRolling = 47;
*/
org.openfeed.HighRollingOrBuilder getHighRollingOrBuilder();
/**
* .org.openfeed.LowRolling lowRolling = 48;
* @return Whether the lowRolling field is set.
*/
boolean hasLowRolling();
/**
* .org.openfeed.LowRolling lowRolling = 48;
* @return The lowRolling.
*/
org.openfeed.LowRolling getLowRolling();
/**
* .org.openfeed.LowRolling lowRolling = 48;
*/
org.openfeed.LowRollingOrBuilder getLowRollingOrBuilder();
/**
* .org.openfeed.RequestForQuote requestForQuote = 49;
* @return Whether the requestForQuote field is set.
*/
boolean hasRequestForQuote();
/**
* .org.openfeed.RequestForQuote requestForQuote = 49;
* @return The requestForQuote.
*/
org.openfeed.RequestForQuote getRequestForQuote();
/**
* .org.openfeed.RequestForQuote requestForQuote = 49;
*/
org.openfeed.RequestForQuoteOrBuilder getRequestForQuoteOrBuilder();
org.openfeed.MarketUpdate.DataCase getDataCase();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy