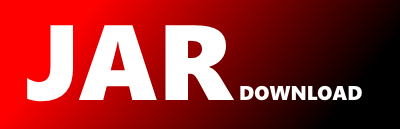
org.openfeed.Result Maven / Gradle / Ivy
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// NO CHECKED-IN PROTOBUF GENCODE
// source: openfeed_api.proto
// Protobuf Java Version: 4.28.3
package org.openfeed;
/**
* Protobuf enum {@code org.openfeed.Result}
*/
public enum Result
implements com.google.protobuf.ProtocolMessageEnum {
/**
* UNKNOWN_RESULT = 0;
*/
UNKNOWN_RESULT(0),
/**
* SUCCESS = 1;
*/
SUCCESS(1),
/**
* INSTRUMENTS_NOT_FOUND = 112;
*/
INSTRUMENTS_NOT_FOUND(112),
/**
* JWT_EXPIRED = 113;
*/
JWT_EXPIRED(113),
/**
* JWT_INVALID = 114;
*/
JWT_INVALID(114),
/**
* DUPLICATE_LOGIN = 115;
*/
DUPLICATE_LOGIN(115),
/**
* INVALID_SYMBOL = 116;
*/
INVALID_SYMBOL(116),
/**
* INVALID_MARKET_ID = 117;
*/
INVALID_MARKET_ID(117),
/**
* INVALID_EXCHANGE = 118;
*/
INVALID_EXCHANGE(118),
/**
* INVALID_CHANNEL_ID = 119;
*/
INVALID_CHANNEL_ID(119),
/**
* MALFORMED_MESSAGE = 120;
*/
MALFORMED_MESSAGE(120),
/**
* UNEXPECTED_MESSAGE = 121;
*/
UNEXPECTED_MESSAGE(121),
/**
* NOT_SUBSCRIBED = 122;
*/
NOT_SUBSCRIBED(122),
/**
* DUPLICATE_SUBSCRIPTION = 123;
*/
DUPLICATE_SUBSCRIPTION(123),
/**
* INVALID_CREDENTIALS = 124;
*/
INVALID_CREDENTIALS(124),
/**
* INSUFFICIENT_PRIVILEGES = 125;
*/
INSUFFICIENT_PRIVILEGES(125),
/**
* AUTHENTICATION_REQUIRED = 126;
*/
AUTHENTICATION_REQUIRED(126),
/**
* GENERIC_FAILURE = 127;
*/
GENERIC_FAILURE(127),
/**
* INVALID_USERNAME = 128;
*/
INVALID_USERNAME(128),
UNRECOGNIZED(-1),
;
static {
com.google.protobuf.RuntimeVersion.validateProtobufGencodeVersion(
com.google.protobuf.RuntimeVersion.RuntimeDomain.PUBLIC,
/* major= */ 4,
/* minor= */ 28,
/* patch= */ 3,
/* suffix= */ "",
Result.class.getName());
}
/**
* UNKNOWN_RESULT = 0;
*/
public static final int UNKNOWN_RESULT_VALUE = 0;
/**
* SUCCESS = 1;
*/
public static final int SUCCESS_VALUE = 1;
/**
* INSTRUMENTS_NOT_FOUND = 112;
*/
public static final int INSTRUMENTS_NOT_FOUND_VALUE = 112;
/**
* JWT_EXPIRED = 113;
*/
public static final int JWT_EXPIRED_VALUE = 113;
/**
* JWT_INVALID = 114;
*/
public static final int JWT_INVALID_VALUE = 114;
/**
* DUPLICATE_LOGIN = 115;
*/
public static final int DUPLICATE_LOGIN_VALUE = 115;
/**
* INVALID_SYMBOL = 116;
*/
public static final int INVALID_SYMBOL_VALUE = 116;
/**
* INVALID_MARKET_ID = 117;
*/
public static final int INVALID_MARKET_ID_VALUE = 117;
/**
* INVALID_EXCHANGE = 118;
*/
public static final int INVALID_EXCHANGE_VALUE = 118;
/**
* INVALID_CHANNEL_ID = 119;
*/
public static final int INVALID_CHANNEL_ID_VALUE = 119;
/**
* MALFORMED_MESSAGE = 120;
*/
public static final int MALFORMED_MESSAGE_VALUE = 120;
/**
* UNEXPECTED_MESSAGE = 121;
*/
public static final int UNEXPECTED_MESSAGE_VALUE = 121;
/**
* NOT_SUBSCRIBED = 122;
*/
public static final int NOT_SUBSCRIBED_VALUE = 122;
/**
* DUPLICATE_SUBSCRIPTION = 123;
*/
public static final int DUPLICATE_SUBSCRIPTION_VALUE = 123;
/**
* INVALID_CREDENTIALS = 124;
*/
public static final int INVALID_CREDENTIALS_VALUE = 124;
/**
* INSUFFICIENT_PRIVILEGES = 125;
*/
public static final int INSUFFICIENT_PRIVILEGES_VALUE = 125;
/**
* AUTHENTICATION_REQUIRED = 126;
*/
public static final int AUTHENTICATION_REQUIRED_VALUE = 126;
/**
* GENERIC_FAILURE = 127;
*/
public static final int GENERIC_FAILURE_VALUE = 127;
/**
* INVALID_USERNAME = 128;
*/
public static final int INVALID_USERNAME_VALUE = 128;
public final int getNumber() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalArgumentException(
"Can't get the number of an unknown enum value.");
}
return value;
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static Result valueOf(int value) {
return forNumber(value);
}
/**
* @param value The numeric wire value of the corresponding enum entry.
* @return The enum associated with the given numeric wire value.
*/
public static Result forNumber(int value) {
switch (value) {
case 0: return UNKNOWN_RESULT;
case 1: return SUCCESS;
case 112: return INSTRUMENTS_NOT_FOUND;
case 113: return JWT_EXPIRED;
case 114: return JWT_INVALID;
case 115: return DUPLICATE_LOGIN;
case 116: return INVALID_SYMBOL;
case 117: return INVALID_MARKET_ID;
case 118: return INVALID_EXCHANGE;
case 119: return INVALID_CHANNEL_ID;
case 120: return MALFORMED_MESSAGE;
case 121: return UNEXPECTED_MESSAGE;
case 122: return NOT_SUBSCRIBED;
case 123: return DUPLICATE_SUBSCRIPTION;
case 124: return INVALID_CREDENTIALS;
case 125: return INSUFFICIENT_PRIVILEGES;
case 126: return AUTHENTICATION_REQUIRED;
case 127: return GENERIC_FAILURE;
case 128: return INVALID_USERNAME;
default: return null;
}
}
public static com.google.protobuf.Internal.EnumLiteMap
internalGetValueMap() {
return internalValueMap;
}
private static final com.google.protobuf.Internal.EnumLiteMap<
Result> internalValueMap =
new com.google.protobuf.Internal.EnumLiteMap() {
public Result findValueByNumber(int number) {
return Result.forNumber(number);
}
};
public final com.google.protobuf.Descriptors.EnumValueDescriptor
getValueDescriptor() {
if (this == UNRECOGNIZED) {
throw new java.lang.IllegalStateException(
"Can't get the descriptor of an unrecognized enum value.");
}
return getDescriptor().getValues().get(ordinal());
}
public final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptorForType() {
return getDescriptor();
}
public static final com.google.protobuf.Descriptors.EnumDescriptor
getDescriptor() {
return org.openfeed.OpenfeedApi.getDescriptor().getEnumTypes().get(0);
}
private static final Result[] VALUES = values();
public static Result valueOf(
com.google.protobuf.Descriptors.EnumValueDescriptor desc) {
if (desc.getType() != getDescriptor()) {
throw new java.lang.IllegalArgumentException(
"EnumValueDescriptor is not for this type.");
}
if (desc.getIndex() == -1) {
return UNRECOGNIZED;
}
return VALUES[desc.getIndex()];
}
private final int value;
private Result(int value) {
this.value = value;
}
// @@protoc_insertion_point(enum_scope:org.openfeed.Result)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy