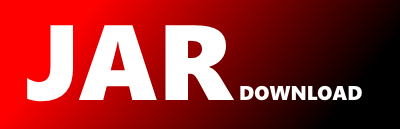
org.openfeed.SubscriptionRequest Maven / Gradle / Ivy
// Generated by the protocol buffer compiler. DO NOT EDIT!
// NO CHECKED-IN PROTOBUF GENCODE
// source: openfeed_api.proto
// Protobuf Java Version: 4.28.3
package org.openfeed;
/**
*
* / Subscription Request
*
*
* Protobuf type {@code org.openfeed.SubscriptionRequest}
*/
public final class SubscriptionRequest extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:org.openfeed.SubscriptionRequest)
SubscriptionRequestOrBuilder {
private static final long serialVersionUID = 0L;
static {
com.google.protobuf.RuntimeVersion.validateProtobufGencodeVersion(
com.google.protobuf.RuntimeVersion.RuntimeDomain.PUBLIC,
/* major= */ 4,
/* minor= */ 28,
/* patch= */ 3,
/* suffix= */ "",
SubscriptionRequest.class.getName());
}
// Use SubscriptionRequest.newBuilder() to construct.
private SubscriptionRequest(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private SubscriptionRequest() {
token_ = "";
service_ = 0;
requests_ = java.util.Collections.emptyList();
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.openfeed.OpenfeedApi.internal_static_org_openfeed_SubscriptionRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.openfeed.OpenfeedApi.internal_static_org_openfeed_SubscriptionRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.openfeed.SubscriptionRequest.class, org.openfeed.SubscriptionRequest.Builder.class);
}
public interface RequestOrBuilder extends
// @@protoc_insertion_point(interface_extends:org.openfeed.SubscriptionRequest.Request)
com.google.protobuf.MessageOrBuilder {
/**
* string symbol = 1;
* @return Whether the symbol field is set.
*/
boolean hasSymbol();
/**
* string symbol = 1;
* @return The symbol.
*/
java.lang.String getSymbol();
/**
* string symbol = 1;
* @return The bytes for symbol.
*/
com.google.protobuf.ByteString
getSymbolBytes();
/**
* sint64 marketId = 2;
* @return Whether the marketId field is set.
*/
boolean hasMarketId();
/**
* sint64 marketId = 2;
* @return The marketId.
*/
long getMarketId();
/**
* string exchange = 3;
* @return Whether the exchange field is set.
*/
boolean hasExchange();
/**
* string exchange = 3;
* @return The exchange.
*/
java.lang.String getExchange();
/**
* string exchange = 3;
* @return The bytes for exchange.
*/
com.google.protobuf.ByteString
getExchangeBytes();
/**
* sint32 channelId = 4;
* @return Whether the channelId field is set.
*/
boolean hasChannelId();
/**
* sint32 channelId = 4;
* @return The channelId.
*/
int getChannelId();
/**
* repeated .org.openfeed.SubscriptionType subscriptionType = 10;
* @return A list containing the subscriptionType.
*/
java.util.List getSubscriptionTypeList();
/**
* repeated .org.openfeed.SubscriptionType subscriptionType = 10;
* @return The count of subscriptionType.
*/
int getSubscriptionTypeCount();
/**
* repeated .org.openfeed.SubscriptionType subscriptionType = 10;
* @param index The index of the element to return.
* @return The subscriptionType at the given index.
*/
org.openfeed.SubscriptionType getSubscriptionType(int index);
/**
* repeated .org.openfeed.SubscriptionType subscriptionType = 10;
* @return A list containing the enum numeric values on the wire for subscriptionType.
*/
java.util.List
getSubscriptionTypeValueList();
/**
* repeated .org.openfeed.SubscriptionType subscriptionType = 10;
* @param index The index of the value to return.
* @return The enum numeric value on the wire of subscriptionType at the given index.
*/
int getSubscriptionTypeValue(int index);
/**
*
* / 0 = send only current snapshot once, else send at interval seconds
*
*
* sint32 snapshotIntervalSeconds = 11;
* @return The snapshotIntervalSeconds.
*/
int getSnapshotIntervalSeconds();
/**
*
* / Spreads and Options must be explicitly requested.
*
*
* repeated .org.openfeed.InstrumentDefinition.InstrumentType instrumentType = 12;
* @return A list containing the instrumentType.
*/
java.util.List getInstrumentTypeList();
/**
*
* / Spreads and Options must be explicitly requested.
*
*
* repeated .org.openfeed.InstrumentDefinition.InstrumentType instrumentType = 12;
* @return The count of instrumentType.
*/
int getInstrumentTypeCount();
/**
*
* / Spreads and Options must be explicitly requested.
*
*
* repeated .org.openfeed.InstrumentDefinition.InstrumentType instrumentType = 12;
* @param index The index of the element to return.
* @return The instrumentType at the given index.
*/
org.openfeed.InstrumentDefinition.InstrumentType getInstrumentType(int index);
/**
*
* / Spreads and Options must be explicitly requested.
*
*
* repeated .org.openfeed.InstrumentDefinition.InstrumentType instrumentType = 12;
* @return A list containing the enum numeric values on the wire for instrumentType.
*/
java.util.List
getInstrumentTypeValueList();
/**
*
* / Spreads and Options must be explicitly requested.
*
*
* repeated .org.openfeed.InstrumentDefinition.InstrumentType instrumentType = 12;
* @param index The index of the value to return.
* @return The enum numeric value on the wire of instrumentType at the given index.
*/
int getInstrumentTypeValue(int index);
/**
*
* / Filter for the exchange and channel subscriptions.
*
*
* repeated .org.openfeed.BulkSubscriptionFilter bulkSubscriptionFilter = 13;
*/
java.util.List
getBulkSubscriptionFilterList();
/**
*
* / Filter for the exchange and channel subscriptions.
*
*
* repeated .org.openfeed.BulkSubscriptionFilter bulkSubscriptionFilter = 13;
*/
org.openfeed.BulkSubscriptionFilter getBulkSubscriptionFilter(int index);
/**
*
* / Filter for the exchange and channel subscriptions.
*
*
* repeated .org.openfeed.BulkSubscriptionFilter bulkSubscriptionFilter = 13;
*/
int getBulkSubscriptionFilterCount();
/**
*
* / Filter for the exchange and channel subscriptions.
*
*
* repeated .org.openfeed.BulkSubscriptionFilter bulkSubscriptionFilter = 13;
*/
java.util.List extends org.openfeed.BulkSubscriptionFilterOrBuilder>
getBulkSubscriptionFilterOrBuilderList();
/**
*
* / Filter for the exchange and channel subscriptions.
*
*
* repeated .org.openfeed.BulkSubscriptionFilter bulkSubscriptionFilter = 13;
*/
org.openfeed.BulkSubscriptionFilterOrBuilder getBulkSubscriptionFilterOrBuilder(
int index);
/**
*
* / Filter for Spread Types
*
*
* repeated string spreadTypeFilter = 14;
* @return A list containing the spreadTypeFilter.
*/
java.util.List
getSpreadTypeFilterList();
/**
*
* / Filter for Spread Types
*
*
* repeated string spreadTypeFilter = 14;
* @return The count of spreadTypeFilter.
*/
int getSpreadTypeFilterCount();
/**
*
* / Filter for Spread Types
*
*
* repeated string spreadTypeFilter = 14;
* @param index The index of the element to return.
* @return The spreadTypeFilter at the given index.
*/
java.lang.String getSpreadTypeFilter(int index);
/**
*
* / Filter for Spread Types
*
*
* repeated string spreadTypeFilter = 14;
* @param index The index of the value to return.
* @return The bytes of the spreadTypeFilter at the given index.
*/
com.google.protobuf.ByteString
getSpreadTypeFilterBytes(int index);
/**
*
* / Do not send instrument(s) on successful subscription
*
*
* bool subscriptionDoNotSendInstruments = 15;
* @return The subscriptionDoNotSendInstruments.
*/
boolean getSubscriptionDoNotSendInstruments();
/**
*
* / Do not send market snapshot(s) on successful subscription
*
*
* bool subscriptionDoNotSendSnapshots = 16;
* @return The subscriptionDoNotSendSnapshots.
*/
boolean getSubscriptionDoNotSendSnapshots();
org.openfeed.SubscriptionRequest.Request.DataCase getDataCase();
}
/**
* Protobuf type {@code org.openfeed.SubscriptionRequest.Request}
*/
public static final class Request extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:org.openfeed.SubscriptionRequest.Request)
RequestOrBuilder {
private static final long serialVersionUID = 0L;
static {
com.google.protobuf.RuntimeVersion.validateProtobufGencodeVersion(
com.google.protobuf.RuntimeVersion.RuntimeDomain.PUBLIC,
/* major= */ 4,
/* minor= */ 28,
/* patch= */ 3,
/* suffix= */ "",
Request.class.getName());
}
// Use Request.newBuilder() to construct.
private Request(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private Request() {
subscriptionType_ = emptyIntList();
instrumentType_ = emptyIntList();
bulkSubscriptionFilter_ = java.util.Collections.emptyList();
spreadTypeFilter_ =
com.google.protobuf.LazyStringArrayList.emptyList();
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.openfeed.OpenfeedApi.internal_static_org_openfeed_SubscriptionRequest_Request_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.openfeed.OpenfeedApi.internal_static_org_openfeed_SubscriptionRequest_Request_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.openfeed.SubscriptionRequest.Request.class, org.openfeed.SubscriptionRequest.Request.Builder.class);
}
private int dataCase_ = 0;
@SuppressWarnings("serial")
private java.lang.Object data_;
public enum DataCase
implements com.google.protobuf.Internal.EnumLite,
com.google.protobuf.AbstractMessage.InternalOneOfEnum {
SYMBOL(1),
MARKETID(2),
EXCHANGE(3),
CHANNELID(4),
DATA_NOT_SET(0);
private final int value;
private DataCase(int value) {
this.value = value;
}
/**
* @param value The number of the enum to look for.
* @return The enum associated with the given number.
* @deprecated Use {@link #forNumber(int)} instead.
*/
@java.lang.Deprecated
public static DataCase valueOf(int value) {
return forNumber(value);
}
public static DataCase forNumber(int value) {
switch (value) {
case 1: return SYMBOL;
case 2: return MARKETID;
case 3: return EXCHANGE;
case 4: return CHANNELID;
case 0: return DATA_NOT_SET;
default: return null;
}
}
public int getNumber() {
return this.value;
}
};
public DataCase
getDataCase() {
return DataCase.forNumber(
dataCase_);
}
public static final int SYMBOL_FIELD_NUMBER = 1;
/**
* string symbol = 1;
* @return Whether the symbol field is set.
*/
public boolean hasSymbol() {
return dataCase_ == 1;
}
/**
* string symbol = 1;
* @return The symbol.
*/
public java.lang.String getSymbol() {
java.lang.Object ref = "";
if (dataCase_ == 1) {
ref = data_;
}
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (dataCase_ == 1) {
data_ = s;
}
return s;
}
}
/**
* string symbol = 1;
* @return The bytes for symbol.
*/
public com.google.protobuf.ByteString
getSymbolBytes() {
java.lang.Object ref = "";
if (dataCase_ == 1) {
ref = data_;
}
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
if (dataCase_ == 1) {
data_ = b;
}
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int MARKETID_FIELD_NUMBER = 2;
/**
* sint64 marketId = 2;
* @return Whether the marketId field is set.
*/
@java.lang.Override
public boolean hasMarketId() {
return dataCase_ == 2;
}
/**
* sint64 marketId = 2;
* @return The marketId.
*/
@java.lang.Override
public long getMarketId() {
if (dataCase_ == 2) {
return (java.lang.Long) data_;
}
return 0L;
}
public static final int EXCHANGE_FIELD_NUMBER = 3;
/**
* string exchange = 3;
* @return Whether the exchange field is set.
*/
public boolean hasExchange() {
return dataCase_ == 3;
}
/**
* string exchange = 3;
* @return The exchange.
*/
public java.lang.String getExchange() {
java.lang.Object ref = "";
if (dataCase_ == 3) {
ref = data_;
}
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (dataCase_ == 3) {
data_ = s;
}
return s;
}
}
/**
* string exchange = 3;
* @return The bytes for exchange.
*/
public com.google.protobuf.ByteString
getExchangeBytes() {
java.lang.Object ref = "";
if (dataCase_ == 3) {
ref = data_;
}
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
if (dataCase_ == 3) {
data_ = b;
}
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int CHANNELID_FIELD_NUMBER = 4;
/**
* sint32 channelId = 4;
* @return Whether the channelId field is set.
*/
@java.lang.Override
public boolean hasChannelId() {
return dataCase_ == 4;
}
/**
* sint32 channelId = 4;
* @return The channelId.
*/
@java.lang.Override
public int getChannelId() {
if (dataCase_ == 4) {
return (java.lang.Integer) data_;
}
return 0;
}
public static final int SUBSCRIPTIONTYPE_FIELD_NUMBER = 10;
@SuppressWarnings("serial")
private com.google.protobuf.Internal.IntList subscriptionType_;
private static final com.google.protobuf.Internal.IntListAdapter.IntConverter<
org.openfeed.SubscriptionType> subscriptionType_converter_ =
new com.google.protobuf.Internal.IntListAdapter.IntConverter<
org.openfeed.SubscriptionType>() {
public org.openfeed.SubscriptionType convert(int from) {
org.openfeed.SubscriptionType result = org.openfeed.SubscriptionType.forNumber(from);
return result == null ? org.openfeed.SubscriptionType.UNRECOGNIZED : result;
}
};
/**
* repeated .org.openfeed.SubscriptionType subscriptionType = 10;
* @return A list containing the subscriptionType.
*/
@java.lang.Override
public java.util.List getSubscriptionTypeList() {
return new com.google.protobuf.Internal.IntListAdapter<
org.openfeed.SubscriptionType>(subscriptionType_, subscriptionType_converter_);
}
/**
* repeated .org.openfeed.SubscriptionType subscriptionType = 10;
* @return The count of subscriptionType.
*/
@java.lang.Override
public int getSubscriptionTypeCount() {
return subscriptionType_.size();
}
/**
* repeated .org.openfeed.SubscriptionType subscriptionType = 10;
* @param index The index of the element to return.
* @return The subscriptionType at the given index.
*/
@java.lang.Override
public org.openfeed.SubscriptionType getSubscriptionType(int index) {
return subscriptionType_converter_.convert(subscriptionType_.getInt(index));
}
/**
* repeated .org.openfeed.SubscriptionType subscriptionType = 10;
* @return A list containing the enum numeric values on the wire for subscriptionType.
*/
@java.lang.Override
public java.util.List
getSubscriptionTypeValueList() {
return subscriptionType_;
}
/**
* repeated .org.openfeed.SubscriptionType subscriptionType = 10;
* @param index The index of the value to return.
* @return The enum numeric value on the wire of subscriptionType at the given index.
*/
@java.lang.Override
public int getSubscriptionTypeValue(int index) {
return subscriptionType_.getInt(index);
}
private int subscriptionTypeMemoizedSerializedSize;
public static final int SNAPSHOTINTERVALSECONDS_FIELD_NUMBER = 11;
private int snapshotIntervalSeconds_ = 0;
/**
*
* / 0 = send only current snapshot once, else send at interval seconds
*
*
* sint32 snapshotIntervalSeconds = 11;
* @return The snapshotIntervalSeconds.
*/
@java.lang.Override
public int getSnapshotIntervalSeconds() {
return snapshotIntervalSeconds_;
}
public static final int INSTRUMENTTYPE_FIELD_NUMBER = 12;
@SuppressWarnings("serial")
private com.google.protobuf.Internal.IntList instrumentType_;
private static final com.google.protobuf.Internal.IntListAdapter.IntConverter<
org.openfeed.InstrumentDefinition.InstrumentType> instrumentType_converter_ =
new com.google.protobuf.Internal.IntListAdapter.IntConverter<
org.openfeed.InstrumentDefinition.InstrumentType>() {
public org.openfeed.InstrumentDefinition.InstrumentType convert(int from) {
org.openfeed.InstrumentDefinition.InstrumentType result = org.openfeed.InstrumentDefinition.InstrumentType.forNumber(from);
return result == null ? org.openfeed.InstrumentDefinition.InstrumentType.UNRECOGNIZED : result;
}
};
/**
*
* / Spreads and Options must be explicitly requested.
*
*
* repeated .org.openfeed.InstrumentDefinition.InstrumentType instrumentType = 12;
* @return A list containing the instrumentType.
*/
@java.lang.Override
public java.util.List getInstrumentTypeList() {
return new com.google.protobuf.Internal.IntListAdapter<
org.openfeed.InstrumentDefinition.InstrumentType>(instrumentType_, instrumentType_converter_);
}
/**
*
* / Spreads and Options must be explicitly requested.
*
*
* repeated .org.openfeed.InstrumentDefinition.InstrumentType instrumentType = 12;
* @return The count of instrumentType.
*/
@java.lang.Override
public int getInstrumentTypeCount() {
return instrumentType_.size();
}
/**
*
* / Spreads and Options must be explicitly requested.
*
*
* repeated .org.openfeed.InstrumentDefinition.InstrumentType instrumentType = 12;
* @param index The index of the element to return.
* @return The instrumentType at the given index.
*/
@java.lang.Override
public org.openfeed.InstrumentDefinition.InstrumentType getInstrumentType(int index) {
return instrumentType_converter_.convert(instrumentType_.getInt(index));
}
/**
*
* / Spreads and Options must be explicitly requested.
*
*
* repeated .org.openfeed.InstrumentDefinition.InstrumentType instrumentType = 12;
* @return A list containing the enum numeric values on the wire for instrumentType.
*/
@java.lang.Override
public java.util.List
getInstrumentTypeValueList() {
return instrumentType_;
}
/**
*
* / Spreads and Options must be explicitly requested.
*
*
* repeated .org.openfeed.InstrumentDefinition.InstrumentType instrumentType = 12;
* @param index The index of the value to return.
* @return The enum numeric value on the wire of instrumentType at the given index.
*/
@java.lang.Override
public int getInstrumentTypeValue(int index) {
return instrumentType_.getInt(index);
}
private int instrumentTypeMemoizedSerializedSize;
public static final int BULKSUBSCRIPTIONFILTER_FIELD_NUMBER = 13;
@SuppressWarnings("serial")
private java.util.List bulkSubscriptionFilter_;
/**
*
* / Filter for the exchange and channel subscriptions.
*
*
* repeated .org.openfeed.BulkSubscriptionFilter bulkSubscriptionFilter = 13;
*/
@java.lang.Override
public java.util.List getBulkSubscriptionFilterList() {
return bulkSubscriptionFilter_;
}
/**
*
* / Filter for the exchange and channel subscriptions.
*
*
* repeated .org.openfeed.BulkSubscriptionFilter bulkSubscriptionFilter = 13;
*/
@java.lang.Override
public java.util.List extends org.openfeed.BulkSubscriptionFilterOrBuilder>
getBulkSubscriptionFilterOrBuilderList() {
return bulkSubscriptionFilter_;
}
/**
*
* / Filter for the exchange and channel subscriptions.
*
*
* repeated .org.openfeed.BulkSubscriptionFilter bulkSubscriptionFilter = 13;
*/
@java.lang.Override
public int getBulkSubscriptionFilterCount() {
return bulkSubscriptionFilter_.size();
}
/**
*
* / Filter for the exchange and channel subscriptions.
*
*
* repeated .org.openfeed.BulkSubscriptionFilter bulkSubscriptionFilter = 13;
*/
@java.lang.Override
public org.openfeed.BulkSubscriptionFilter getBulkSubscriptionFilter(int index) {
return bulkSubscriptionFilter_.get(index);
}
/**
*
* / Filter for the exchange and channel subscriptions.
*
*
* repeated .org.openfeed.BulkSubscriptionFilter bulkSubscriptionFilter = 13;
*/
@java.lang.Override
public org.openfeed.BulkSubscriptionFilterOrBuilder getBulkSubscriptionFilterOrBuilder(
int index) {
return bulkSubscriptionFilter_.get(index);
}
public static final int SPREADTYPEFILTER_FIELD_NUMBER = 14;
@SuppressWarnings("serial")
private com.google.protobuf.LazyStringArrayList spreadTypeFilter_ =
com.google.protobuf.LazyStringArrayList.emptyList();
/**
*
* / Filter for Spread Types
*
*
* repeated string spreadTypeFilter = 14;
* @return A list containing the spreadTypeFilter.
*/
public com.google.protobuf.ProtocolStringList
getSpreadTypeFilterList() {
return spreadTypeFilter_;
}
/**
*
* / Filter for Spread Types
*
*
* repeated string spreadTypeFilter = 14;
* @return The count of spreadTypeFilter.
*/
public int getSpreadTypeFilterCount() {
return spreadTypeFilter_.size();
}
/**
*
* / Filter for Spread Types
*
*
* repeated string spreadTypeFilter = 14;
* @param index The index of the element to return.
* @return The spreadTypeFilter at the given index.
*/
public java.lang.String getSpreadTypeFilter(int index) {
return spreadTypeFilter_.get(index);
}
/**
*
* / Filter for Spread Types
*
*
* repeated string spreadTypeFilter = 14;
* @param index The index of the value to return.
* @return The bytes of the spreadTypeFilter at the given index.
*/
public com.google.protobuf.ByteString
getSpreadTypeFilterBytes(int index) {
return spreadTypeFilter_.getByteString(index);
}
public static final int SUBSCRIPTIONDONOTSENDINSTRUMENTS_FIELD_NUMBER = 15;
private boolean subscriptionDoNotSendInstruments_ = false;
/**
*
* / Do not send instrument(s) on successful subscription
*
*
* bool subscriptionDoNotSendInstruments = 15;
* @return The subscriptionDoNotSendInstruments.
*/
@java.lang.Override
public boolean getSubscriptionDoNotSendInstruments() {
return subscriptionDoNotSendInstruments_;
}
public static final int SUBSCRIPTIONDONOTSENDSNAPSHOTS_FIELD_NUMBER = 16;
private boolean subscriptionDoNotSendSnapshots_ = false;
/**
*
* / Do not send market snapshot(s) on successful subscription
*
*
* bool subscriptionDoNotSendSnapshots = 16;
* @return The subscriptionDoNotSendSnapshots.
*/
@java.lang.Override
public boolean getSubscriptionDoNotSendSnapshots() {
return subscriptionDoNotSendSnapshots_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
getSerializedSize();
if (dataCase_ == 1) {
com.google.protobuf.GeneratedMessage.writeString(output, 1, data_);
}
if (dataCase_ == 2) {
output.writeSInt64(
2, (long)((java.lang.Long) data_));
}
if (dataCase_ == 3) {
com.google.protobuf.GeneratedMessage.writeString(output, 3, data_);
}
if (dataCase_ == 4) {
output.writeSInt32(
4, (int)((java.lang.Integer) data_));
}
if (getSubscriptionTypeList().size() > 0) {
output.writeUInt32NoTag(82);
output.writeUInt32NoTag(subscriptionTypeMemoizedSerializedSize);
}
for (int i = 0; i < subscriptionType_.size(); i++) {
output.writeEnumNoTag(subscriptionType_.getInt(i));
}
if (snapshotIntervalSeconds_ != 0) {
output.writeSInt32(11, snapshotIntervalSeconds_);
}
if (getInstrumentTypeList().size() > 0) {
output.writeUInt32NoTag(98);
output.writeUInt32NoTag(instrumentTypeMemoizedSerializedSize);
}
for (int i = 0; i < instrumentType_.size(); i++) {
output.writeEnumNoTag(instrumentType_.getInt(i));
}
for (int i = 0; i < bulkSubscriptionFilter_.size(); i++) {
output.writeMessage(13, bulkSubscriptionFilter_.get(i));
}
for (int i = 0; i < spreadTypeFilter_.size(); i++) {
com.google.protobuf.GeneratedMessage.writeString(output, 14, spreadTypeFilter_.getRaw(i));
}
if (subscriptionDoNotSendInstruments_ != false) {
output.writeBool(15, subscriptionDoNotSendInstruments_);
}
if (subscriptionDoNotSendSnapshots_ != false) {
output.writeBool(16, subscriptionDoNotSendSnapshots_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (dataCase_ == 1) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(1, data_);
}
if (dataCase_ == 2) {
size += com.google.protobuf.CodedOutputStream
.computeSInt64Size(
2, (long)((java.lang.Long) data_));
}
if (dataCase_ == 3) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(3, data_);
}
if (dataCase_ == 4) {
size += com.google.protobuf.CodedOutputStream
.computeSInt32Size(
4, (int)((java.lang.Integer) data_));
}
{
int dataSize = 0;
for (int i = 0; i < subscriptionType_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeEnumSizeNoTag(subscriptionType_.getInt(i));
}
size += dataSize;
if (!getSubscriptionTypeList().isEmpty()) { size += 1;
size += com.google.protobuf.CodedOutputStream
.computeUInt32SizeNoTag(dataSize);
}subscriptionTypeMemoizedSerializedSize = dataSize;
}
if (snapshotIntervalSeconds_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeSInt32Size(11, snapshotIntervalSeconds_);
}
{
int dataSize = 0;
for (int i = 0; i < instrumentType_.size(); i++) {
dataSize += com.google.protobuf.CodedOutputStream
.computeEnumSizeNoTag(instrumentType_.getInt(i));
}
size += dataSize;
if (!getInstrumentTypeList().isEmpty()) { size += 1;
size += com.google.protobuf.CodedOutputStream
.computeUInt32SizeNoTag(dataSize);
}instrumentTypeMemoizedSerializedSize = dataSize;
}
for (int i = 0; i < bulkSubscriptionFilter_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(13, bulkSubscriptionFilter_.get(i));
}
{
int dataSize = 0;
for (int i = 0; i < spreadTypeFilter_.size(); i++) {
dataSize += computeStringSizeNoTag(spreadTypeFilter_.getRaw(i));
}
size += dataSize;
size += 1 * getSpreadTypeFilterList().size();
}
if (subscriptionDoNotSendInstruments_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(15, subscriptionDoNotSendInstruments_);
}
if (subscriptionDoNotSendSnapshots_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(16, subscriptionDoNotSendSnapshots_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.openfeed.SubscriptionRequest.Request)) {
return super.equals(obj);
}
org.openfeed.SubscriptionRequest.Request other = (org.openfeed.SubscriptionRequest.Request) obj;
if (!subscriptionType_.equals(other.subscriptionType_)) return false;
if (getSnapshotIntervalSeconds()
!= other.getSnapshotIntervalSeconds()) return false;
if (!instrumentType_.equals(other.instrumentType_)) return false;
if (!getBulkSubscriptionFilterList()
.equals(other.getBulkSubscriptionFilterList())) return false;
if (!getSpreadTypeFilterList()
.equals(other.getSpreadTypeFilterList())) return false;
if (getSubscriptionDoNotSendInstruments()
!= other.getSubscriptionDoNotSendInstruments()) return false;
if (getSubscriptionDoNotSendSnapshots()
!= other.getSubscriptionDoNotSendSnapshots()) return false;
if (!getDataCase().equals(other.getDataCase())) return false;
switch (dataCase_) {
case 1:
if (!getSymbol()
.equals(other.getSymbol())) return false;
break;
case 2:
if (getMarketId()
!= other.getMarketId()) return false;
break;
case 3:
if (!getExchange()
.equals(other.getExchange())) return false;
break;
case 4:
if (getChannelId()
!= other.getChannelId()) return false;
break;
case 0:
default:
}
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
if (getSubscriptionTypeCount() > 0) {
hash = (37 * hash) + SUBSCRIPTIONTYPE_FIELD_NUMBER;
hash = (53 * hash) + subscriptionType_.hashCode();
}
hash = (37 * hash) + SNAPSHOTINTERVALSECONDS_FIELD_NUMBER;
hash = (53 * hash) + getSnapshotIntervalSeconds();
if (getInstrumentTypeCount() > 0) {
hash = (37 * hash) + INSTRUMENTTYPE_FIELD_NUMBER;
hash = (53 * hash) + instrumentType_.hashCode();
}
if (getBulkSubscriptionFilterCount() > 0) {
hash = (37 * hash) + BULKSUBSCRIPTIONFILTER_FIELD_NUMBER;
hash = (53 * hash) + getBulkSubscriptionFilterList().hashCode();
}
if (getSpreadTypeFilterCount() > 0) {
hash = (37 * hash) + SPREADTYPEFILTER_FIELD_NUMBER;
hash = (53 * hash) + getSpreadTypeFilterList().hashCode();
}
hash = (37 * hash) + SUBSCRIPTIONDONOTSENDINSTRUMENTS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getSubscriptionDoNotSendInstruments());
hash = (37 * hash) + SUBSCRIPTIONDONOTSENDSNAPSHOTS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getSubscriptionDoNotSendSnapshots());
switch (dataCase_) {
case 1:
hash = (37 * hash) + SYMBOL_FIELD_NUMBER;
hash = (53 * hash) + getSymbol().hashCode();
break;
case 2:
hash = (37 * hash) + MARKETID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getMarketId());
break;
case 3:
hash = (37 * hash) + EXCHANGE_FIELD_NUMBER;
hash = (53 * hash) + getExchange().hashCode();
break;
case 4:
hash = (37 * hash) + CHANNELID_FIELD_NUMBER;
hash = (53 * hash) + getChannelId();
break;
case 0:
default:
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.openfeed.SubscriptionRequest.Request parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.openfeed.SubscriptionRequest.Request parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.openfeed.SubscriptionRequest.Request parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.openfeed.SubscriptionRequest.Request parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.openfeed.SubscriptionRequest.Request parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.openfeed.SubscriptionRequest.Request parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.openfeed.SubscriptionRequest.Request parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static org.openfeed.SubscriptionRequest.Request parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.openfeed.SubscriptionRequest.Request parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input);
}
public static org.openfeed.SubscriptionRequest.Request parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.openfeed.SubscriptionRequest.Request parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static org.openfeed.SubscriptionRequest.Request parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.openfeed.SubscriptionRequest.Request prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code org.openfeed.SubscriptionRequest.Request}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:org.openfeed.SubscriptionRequest.Request)
org.openfeed.SubscriptionRequest.RequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.openfeed.OpenfeedApi.internal_static_org_openfeed_SubscriptionRequest_Request_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.openfeed.OpenfeedApi.internal_static_org_openfeed_SubscriptionRequest_Request_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.openfeed.SubscriptionRequest.Request.class, org.openfeed.SubscriptionRequest.Request.Builder.class);
}
// Construct using org.openfeed.SubscriptionRequest.Request.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
subscriptionType_ = emptyIntList();
bitField0_ = (bitField0_ & ~0x00000010);
snapshotIntervalSeconds_ = 0;
instrumentType_ = emptyIntList();
bitField0_ = (bitField0_ & ~0x00000040);
if (bulkSubscriptionFilterBuilder_ == null) {
bulkSubscriptionFilter_ = java.util.Collections.emptyList();
} else {
bulkSubscriptionFilter_ = null;
bulkSubscriptionFilterBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000080);
spreadTypeFilter_ =
com.google.protobuf.LazyStringArrayList.emptyList();
subscriptionDoNotSendInstruments_ = false;
subscriptionDoNotSendSnapshots_ = false;
dataCase_ = 0;
data_ = null;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.openfeed.OpenfeedApi.internal_static_org_openfeed_SubscriptionRequest_Request_descriptor;
}
@java.lang.Override
public org.openfeed.SubscriptionRequest.Request getDefaultInstanceForType() {
return org.openfeed.SubscriptionRequest.Request.getDefaultInstance();
}
@java.lang.Override
public org.openfeed.SubscriptionRequest.Request build() {
org.openfeed.SubscriptionRequest.Request result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.openfeed.SubscriptionRequest.Request buildPartial() {
org.openfeed.SubscriptionRequest.Request result = new org.openfeed.SubscriptionRequest.Request(this);
buildPartialRepeatedFields(result);
if (bitField0_ != 0) { buildPartial0(result); }
buildPartialOneofs(result);
onBuilt();
return result;
}
private void buildPartialRepeatedFields(org.openfeed.SubscriptionRequest.Request result) {
if (((bitField0_ & 0x00000010) != 0)) {
subscriptionType_.makeImmutable();
bitField0_ = (bitField0_ & ~0x00000010);
}
result.subscriptionType_ = subscriptionType_;
if (((bitField0_ & 0x00000040) != 0)) {
instrumentType_.makeImmutable();
bitField0_ = (bitField0_ & ~0x00000040);
}
result.instrumentType_ = instrumentType_;
if (bulkSubscriptionFilterBuilder_ == null) {
if (((bitField0_ & 0x00000080) != 0)) {
bulkSubscriptionFilter_ = java.util.Collections.unmodifiableList(bulkSubscriptionFilter_);
bitField0_ = (bitField0_ & ~0x00000080);
}
result.bulkSubscriptionFilter_ = bulkSubscriptionFilter_;
} else {
result.bulkSubscriptionFilter_ = bulkSubscriptionFilterBuilder_.build();
}
}
private void buildPartial0(org.openfeed.SubscriptionRequest.Request result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000020) != 0)) {
result.snapshotIntervalSeconds_ = snapshotIntervalSeconds_;
}
if (((from_bitField0_ & 0x00000100) != 0)) {
spreadTypeFilter_.makeImmutable();
result.spreadTypeFilter_ = spreadTypeFilter_;
}
if (((from_bitField0_ & 0x00000200) != 0)) {
result.subscriptionDoNotSendInstruments_ = subscriptionDoNotSendInstruments_;
}
if (((from_bitField0_ & 0x00000400) != 0)) {
result.subscriptionDoNotSendSnapshots_ = subscriptionDoNotSendSnapshots_;
}
}
private void buildPartialOneofs(org.openfeed.SubscriptionRequest.Request result) {
result.dataCase_ = dataCase_;
result.data_ = this.data_;
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.openfeed.SubscriptionRequest.Request) {
return mergeFrom((org.openfeed.SubscriptionRequest.Request)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.openfeed.SubscriptionRequest.Request other) {
if (other == org.openfeed.SubscriptionRequest.Request.getDefaultInstance()) return this;
if (!other.subscriptionType_.isEmpty()) {
if (subscriptionType_.isEmpty()) {
subscriptionType_ = other.subscriptionType_;
bitField0_ = (bitField0_ & ~0x00000010);
} else {
ensureSubscriptionTypeIsMutable();
subscriptionType_.addAll(other.subscriptionType_);
}
onChanged();
}
if (other.getSnapshotIntervalSeconds() != 0) {
setSnapshotIntervalSeconds(other.getSnapshotIntervalSeconds());
}
if (!other.instrumentType_.isEmpty()) {
if (instrumentType_.isEmpty()) {
instrumentType_ = other.instrumentType_;
bitField0_ = (bitField0_ & ~0x00000040);
} else {
ensureInstrumentTypeIsMutable();
instrumentType_.addAll(other.instrumentType_);
}
onChanged();
}
if (bulkSubscriptionFilterBuilder_ == null) {
if (!other.bulkSubscriptionFilter_.isEmpty()) {
if (bulkSubscriptionFilter_.isEmpty()) {
bulkSubscriptionFilter_ = other.bulkSubscriptionFilter_;
bitField0_ = (bitField0_ & ~0x00000080);
} else {
ensureBulkSubscriptionFilterIsMutable();
bulkSubscriptionFilter_.addAll(other.bulkSubscriptionFilter_);
}
onChanged();
}
} else {
if (!other.bulkSubscriptionFilter_.isEmpty()) {
if (bulkSubscriptionFilterBuilder_.isEmpty()) {
bulkSubscriptionFilterBuilder_.dispose();
bulkSubscriptionFilterBuilder_ = null;
bulkSubscriptionFilter_ = other.bulkSubscriptionFilter_;
bitField0_ = (bitField0_ & ~0x00000080);
bulkSubscriptionFilterBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getBulkSubscriptionFilterFieldBuilder() : null;
} else {
bulkSubscriptionFilterBuilder_.addAllMessages(other.bulkSubscriptionFilter_);
}
}
}
if (!other.spreadTypeFilter_.isEmpty()) {
if (spreadTypeFilter_.isEmpty()) {
spreadTypeFilter_ = other.spreadTypeFilter_;
bitField0_ |= 0x00000100;
} else {
ensureSpreadTypeFilterIsMutable();
spreadTypeFilter_.addAll(other.spreadTypeFilter_);
}
onChanged();
}
if (other.getSubscriptionDoNotSendInstruments() != false) {
setSubscriptionDoNotSendInstruments(other.getSubscriptionDoNotSendInstruments());
}
if (other.getSubscriptionDoNotSendSnapshots() != false) {
setSubscriptionDoNotSendSnapshots(other.getSubscriptionDoNotSendSnapshots());
}
switch (other.getDataCase()) {
case SYMBOL: {
dataCase_ = 1;
data_ = other.data_;
onChanged();
break;
}
case MARKETID: {
setMarketId(other.getMarketId());
break;
}
case EXCHANGE: {
dataCase_ = 3;
data_ = other.data_;
onChanged();
break;
}
case CHANNELID: {
setChannelId(other.getChannelId());
break;
}
case DATA_NOT_SET: {
break;
}
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 10: {
java.lang.String s = input.readStringRequireUtf8();
dataCase_ = 1;
data_ = s;
break;
} // case 10
case 16: {
data_ = input.readSInt64();
dataCase_ = 2;
break;
} // case 16
case 26: {
java.lang.String s = input.readStringRequireUtf8();
dataCase_ = 3;
data_ = s;
break;
} // case 26
case 32: {
data_ = input.readSInt32();
dataCase_ = 4;
break;
} // case 32
case 80: {
int tmpRaw = input.readEnum();
ensureSubscriptionTypeIsMutable();
subscriptionType_.addInt(tmpRaw);
break;
} // case 80
case 82: {
int length = input.readRawVarint32();
int oldLimit = input.pushLimit(length);
while(input.getBytesUntilLimit() > 0) {
int tmpRaw = input.readEnum();
ensureSubscriptionTypeIsMutable();
subscriptionType_.addInt(tmpRaw);
}
input.popLimit(oldLimit);
break;
} // case 82
case 88: {
snapshotIntervalSeconds_ = input.readSInt32();
bitField0_ |= 0x00000020;
break;
} // case 88
case 96: {
int tmpRaw = input.readEnum();
ensureInstrumentTypeIsMutable();
instrumentType_.addInt(tmpRaw);
break;
} // case 96
case 98: {
int length = input.readRawVarint32();
int oldLimit = input.pushLimit(length);
while(input.getBytesUntilLimit() > 0) {
int tmpRaw = input.readEnum();
ensureInstrumentTypeIsMutable();
instrumentType_.addInt(tmpRaw);
}
input.popLimit(oldLimit);
break;
} // case 98
case 106: {
org.openfeed.BulkSubscriptionFilter m =
input.readMessage(
org.openfeed.BulkSubscriptionFilter.parser(),
extensionRegistry);
if (bulkSubscriptionFilterBuilder_ == null) {
ensureBulkSubscriptionFilterIsMutable();
bulkSubscriptionFilter_.add(m);
} else {
bulkSubscriptionFilterBuilder_.addMessage(m);
}
break;
} // case 106
case 114: {
java.lang.String s = input.readStringRequireUtf8();
ensureSpreadTypeFilterIsMutable();
spreadTypeFilter_.add(s);
break;
} // case 114
case 120: {
subscriptionDoNotSendInstruments_ = input.readBool();
bitField0_ |= 0x00000200;
break;
} // case 120
case 128: {
subscriptionDoNotSendSnapshots_ = input.readBool();
bitField0_ |= 0x00000400;
break;
} // case 128
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int dataCase_ = 0;
private java.lang.Object data_;
public DataCase
getDataCase() {
return DataCase.forNumber(
dataCase_);
}
public Builder clearData() {
dataCase_ = 0;
data_ = null;
onChanged();
return this;
}
private int bitField0_;
/**
* string symbol = 1;
* @return Whether the symbol field is set.
*/
@java.lang.Override
public boolean hasSymbol() {
return dataCase_ == 1;
}
/**
* string symbol = 1;
* @return The symbol.
*/
@java.lang.Override
public java.lang.String getSymbol() {
java.lang.Object ref = "";
if (dataCase_ == 1) {
ref = data_;
}
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (dataCase_ == 1) {
data_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string symbol = 1;
* @return The bytes for symbol.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getSymbolBytes() {
java.lang.Object ref = "";
if (dataCase_ == 1) {
ref = data_;
}
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
if (dataCase_ == 1) {
data_ = b;
}
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string symbol = 1;
* @param value The symbol to set.
* @return This builder for chaining.
*/
public Builder setSymbol(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
dataCase_ = 1;
data_ = value;
onChanged();
return this;
}
/**
* string symbol = 1;
* @return This builder for chaining.
*/
public Builder clearSymbol() {
if (dataCase_ == 1) {
dataCase_ = 0;
data_ = null;
onChanged();
}
return this;
}
/**
* string symbol = 1;
* @param value The bytes for symbol to set.
* @return This builder for chaining.
*/
public Builder setSymbolBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
dataCase_ = 1;
data_ = value;
onChanged();
return this;
}
/**
* sint64 marketId = 2;
* @return Whether the marketId field is set.
*/
public boolean hasMarketId() {
return dataCase_ == 2;
}
/**
* sint64 marketId = 2;
* @return The marketId.
*/
public long getMarketId() {
if (dataCase_ == 2) {
return (java.lang.Long) data_;
}
return 0L;
}
/**
* sint64 marketId = 2;
* @param value The marketId to set.
* @return This builder for chaining.
*/
public Builder setMarketId(long value) {
dataCase_ = 2;
data_ = value;
onChanged();
return this;
}
/**
* sint64 marketId = 2;
* @return This builder for chaining.
*/
public Builder clearMarketId() {
if (dataCase_ == 2) {
dataCase_ = 0;
data_ = null;
onChanged();
}
return this;
}
/**
* string exchange = 3;
* @return Whether the exchange field is set.
*/
@java.lang.Override
public boolean hasExchange() {
return dataCase_ == 3;
}
/**
* string exchange = 3;
* @return The exchange.
*/
@java.lang.Override
public java.lang.String getExchange() {
java.lang.Object ref = "";
if (dataCase_ == 3) {
ref = data_;
}
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
if (dataCase_ == 3) {
data_ = s;
}
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string exchange = 3;
* @return The bytes for exchange.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getExchangeBytes() {
java.lang.Object ref = "";
if (dataCase_ == 3) {
ref = data_;
}
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
if (dataCase_ == 3) {
data_ = b;
}
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string exchange = 3;
* @param value The exchange to set.
* @return This builder for chaining.
*/
public Builder setExchange(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
dataCase_ = 3;
data_ = value;
onChanged();
return this;
}
/**
* string exchange = 3;
* @return This builder for chaining.
*/
public Builder clearExchange() {
if (dataCase_ == 3) {
dataCase_ = 0;
data_ = null;
onChanged();
}
return this;
}
/**
* string exchange = 3;
* @param value The bytes for exchange to set.
* @return This builder for chaining.
*/
public Builder setExchangeBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
dataCase_ = 3;
data_ = value;
onChanged();
return this;
}
/**
* sint32 channelId = 4;
* @return Whether the channelId field is set.
*/
public boolean hasChannelId() {
return dataCase_ == 4;
}
/**
* sint32 channelId = 4;
* @return The channelId.
*/
public int getChannelId() {
if (dataCase_ == 4) {
return (java.lang.Integer) data_;
}
return 0;
}
/**
* sint32 channelId = 4;
* @param value The channelId to set.
* @return This builder for chaining.
*/
public Builder setChannelId(int value) {
dataCase_ = 4;
data_ = value;
onChanged();
return this;
}
/**
* sint32 channelId = 4;
* @return This builder for chaining.
*/
public Builder clearChannelId() {
if (dataCase_ == 4) {
dataCase_ = 0;
data_ = null;
onChanged();
}
return this;
}
private com.google.protobuf.Internal.IntList subscriptionType_ =
emptyIntList();
private void ensureSubscriptionTypeIsMutable() {
if (!((bitField0_ & 0x00000010) != 0)) {
subscriptionType_ = makeMutableCopy(subscriptionType_);
bitField0_ |= 0x00000010;
}
}
/**
* repeated .org.openfeed.SubscriptionType subscriptionType = 10;
* @return A list containing the subscriptionType.
*/
public java.util.List getSubscriptionTypeList() {
return new com.google.protobuf.Internal.IntListAdapter<
org.openfeed.SubscriptionType>(subscriptionType_, subscriptionType_converter_);
}
/**
* repeated .org.openfeed.SubscriptionType subscriptionType = 10;
* @return The count of subscriptionType.
*/
public int getSubscriptionTypeCount() {
return subscriptionType_.size();
}
/**
* repeated .org.openfeed.SubscriptionType subscriptionType = 10;
* @param index The index of the element to return.
* @return The subscriptionType at the given index.
*/
public org.openfeed.SubscriptionType getSubscriptionType(int index) {
return subscriptionType_converter_.convert(subscriptionType_.getInt(index));
}
/**
* repeated .org.openfeed.SubscriptionType subscriptionType = 10;
* @param index The index to set the value at.
* @param value The subscriptionType to set.
* @return This builder for chaining.
*/
public Builder setSubscriptionType(
int index, org.openfeed.SubscriptionType value) {
if (value == null) {
throw new NullPointerException();
}
ensureSubscriptionTypeIsMutable();
subscriptionType_.setInt(index, value.getNumber());
onChanged();
return this;
}
/**
* repeated .org.openfeed.SubscriptionType subscriptionType = 10;
* @param value The subscriptionType to add.
* @return This builder for chaining.
*/
public Builder addSubscriptionType(org.openfeed.SubscriptionType value) {
if (value == null) {
throw new NullPointerException();
}
ensureSubscriptionTypeIsMutable();
subscriptionType_.addInt(value.getNumber());
onChanged();
return this;
}
/**
* repeated .org.openfeed.SubscriptionType subscriptionType = 10;
* @param values The subscriptionType to add.
* @return This builder for chaining.
*/
public Builder addAllSubscriptionType(
java.lang.Iterable extends org.openfeed.SubscriptionType> values) {
ensureSubscriptionTypeIsMutable();
for (org.openfeed.SubscriptionType value : values) {
subscriptionType_.addInt(value.getNumber());
}
onChanged();
return this;
}
/**
* repeated .org.openfeed.SubscriptionType subscriptionType = 10;
* @return This builder for chaining.
*/
public Builder clearSubscriptionType() {
subscriptionType_ = emptyIntList();
bitField0_ = (bitField0_ & ~0x00000010);
onChanged();
return this;
}
/**
* repeated .org.openfeed.SubscriptionType subscriptionType = 10;
* @return A list containing the enum numeric values on the wire for subscriptionType.
*/
public java.util.List
getSubscriptionTypeValueList() {
return java.util.Collections.unmodifiableList(subscriptionType_);
}
/**
* repeated .org.openfeed.SubscriptionType subscriptionType = 10;
* @param index The index of the value to return.
* @return The enum numeric value on the wire of subscriptionType at the given index.
*/
public int getSubscriptionTypeValue(int index) {
return subscriptionType_.getInt(index);
}
/**
* repeated .org.openfeed.SubscriptionType subscriptionType = 10;
* @param index The index to set the value at.
* @param value The enum numeric value on the wire for subscriptionType to set.
* @return This builder for chaining.
*/
public Builder setSubscriptionTypeValue(
int index, int value) {
ensureSubscriptionTypeIsMutable();
subscriptionType_.setInt(index, value);
onChanged();
return this;
}
/**
* repeated .org.openfeed.SubscriptionType subscriptionType = 10;
* @param value The enum numeric value on the wire for subscriptionType to add.
* @return This builder for chaining.
*/
public Builder addSubscriptionTypeValue(int value) {
ensureSubscriptionTypeIsMutable();
subscriptionType_.addInt(value);
onChanged();
return this;
}
/**
* repeated .org.openfeed.SubscriptionType subscriptionType = 10;
* @param values The enum numeric values on the wire for subscriptionType to add.
* @return This builder for chaining.
*/
public Builder addAllSubscriptionTypeValue(
java.lang.Iterable values) {
ensureSubscriptionTypeIsMutable();
for (int value : values) {
subscriptionType_.addInt(value);
}
onChanged();
return this;
}
private int snapshotIntervalSeconds_ ;
/**
*
* / 0 = send only current snapshot once, else send at interval seconds
*
*
* sint32 snapshotIntervalSeconds = 11;
* @return The snapshotIntervalSeconds.
*/
@java.lang.Override
public int getSnapshotIntervalSeconds() {
return snapshotIntervalSeconds_;
}
/**
*
* / 0 = send only current snapshot once, else send at interval seconds
*
*
* sint32 snapshotIntervalSeconds = 11;
* @param value The snapshotIntervalSeconds to set.
* @return This builder for chaining.
*/
public Builder setSnapshotIntervalSeconds(int value) {
snapshotIntervalSeconds_ = value;
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
*
* / 0 = send only current snapshot once, else send at interval seconds
*
*
* sint32 snapshotIntervalSeconds = 11;
* @return This builder for chaining.
*/
public Builder clearSnapshotIntervalSeconds() {
bitField0_ = (bitField0_ & ~0x00000020);
snapshotIntervalSeconds_ = 0;
onChanged();
return this;
}
private com.google.protobuf.Internal.IntList instrumentType_ =
emptyIntList();
private void ensureInstrumentTypeIsMutable() {
if (!((bitField0_ & 0x00000040) != 0)) {
instrumentType_ = makeMutableCopy(instrumentType_);
bitField0_ |= 0x00000040;
}
}
/**
*
* / Spreads and Options must be explicitly requested.
*
*
* repeated .org.openfeed.InstrumentDefinition.InstrumentType instrumentType = 12;
* @return A list containing the instrumentType.
*/
public java.util.List getInstrumentTypeList() {
return new com.google.protobuf.Internal.IntListAdapter<
org.openfeed.InstrumentDefinition.InstrumentType>(instrumentType_, instrumentType_converter_);
}
/**
*
* / Spreads and Options must be explicitly requested.
*
*
* repeated .org.openfeed.InstrumentDefinition.InstrumentType instrumentType = 12;
* @return The count of instrumentType.
*/
public int getInstrumentTypeCount() {
return instrumentType_.size();
}
/**
*
* / Spreads and Options must be explicitly requested.
*
*
* repeated .org.openfeed.InstrumentDefinition.InstrumentType instrumentType = 12;
* @param index The index of the element to return.
* @return The instrumentType at the given index.
*/
public org.openfeed.InstrumentDefinition.InstrumentType getInstrumentType(int index) {
return instrumentType_converter_.convert(instrumentType_.getInt(index));
}
/**
*
* / Spreads and Options must be explicitly requested.
*
*
* repeated .org.openfeed.InstrumentDefinition.InstrumentType instrumentType = 12;
* @param index The index to set the value at.
* @param value The instrumentType to set.
* @return This builder for chaining.
*/
public Builder setInstrumentType(
int index, org.openfeed.InstrumentDefinition.InstrumentType value) {
if (value == null) {
throw new NullPointerException();
}
ensureInstrumentTypeIsMutable();
instrumentType_.setInt(index, value.getNumber());
onChanged();
return this;
}
/**
*
* / Spreads and Options must be explicitly requested.
*
*
* repeated .org.openfeed.InstrumentDefinition.InstrumentType instrumentType = 12;
* @param value The instrumentType to add.
* @return This builder for chaining.
*/
public Builder addInstrumentType(org.openfeed.InstrumentDefinition.InstrumentType value) {
if (value == null) {
throw new NullPointerException();
}
ensureInstrumentTypeIsMutable();
instrumentType_.addInt(value.getNumber());
onChanged();
return this;
}
/**
*
* / Spreads and Options must be explicitly requested.
*
*
* repeated .org.openfeed.InstrumentDefinition.InstrumentType instrumentType = 12;
* @param values The instrumentType to add.
* @return This builder for chaining.
*/
public Builder addAllInstrumentType(
java.lang.Iterable extends org.openfeed.InstrumentDefinition.InstrumentType> values) {
ensureInstrumentTypeIsMutable();
for (org.openfeed.InstrumentDefinition.InstrumentType value : values) {
instrumentType_.addInt(value.getNumber());
}
onChanged();
return this;
}
/**
*
* / Spreads and Options must be explicitly requested.
*
*
* repeated .org.openfeed.InstrumentDefinition.InstrumentType instrumentType = 12;
* @return This builder for chaining.
*/
public Builder clearInstrumentType() {
instrumentType_ = emptyIntList();
bitField0_ = (bitField0_ & ~0x00000040);
onChanged();
return this;
}
/**
*
* / Spreads and Options must be explicitly requested.
*
*
* repeated .org.openfeed.InstrumentDefinition.InstrumentType instrumentType = 12;
* @return A list containing the enum numeric values on the wire for instrumentType.
*/
public java.util.List
getInstrumentTypeValueList() {
return java.util.Collections.unmodifiableList(instrumentType_);
}
/**
*
* / Spreads and Options must be explicitly requested.
*
*
* repeated .org.openfeed.InstrumentDefinition.InstrumentType instrumentType = 12;
* @param index The index of the value to return.
* @return The enum numeric value on the wire of instrumentType at the given index.
*/
public int getInstrumentTypeValue(int index) {
return instrumentType_.getInt(index);
}
/**
*
* / Spreads and Options must be explicitly requested.
*
*
* repeated .org.openfeed.InstrumentDefinition.InstrumentType instrumentType = 12;
* @param index The index to set the value at.
* @param value The enum numeric value on the wire for instrumentType to set.
* @return This builder for chaining.
*/
public Builder setInstrumentTypeValue(
int index, int value) {
ensureInstrumentTypeIsMutable();
instrumentType_.setInt(index, value);
onChanged();
return this;
}
/**
*
* / Spreads and Options must be explicitly requested.
*
*
* repeated .org.openfeed.InstrumentDefinition.InstrumentType instrumentType = 12;
* @param value The enum numeric value on the wire for instrumentType to add.
* @return This builder for chaining.
*/
public Builder addInstrumentTypeValue(int value) {
ensureInstrumentTypeIsMutable();
instrumentType_.addInt(value);
onChanged();
return this;
}
/**
*
* / Spreads and Options must be explicitly requested.
*
*
* repeated .org.openfeed.InstrumentDefinition.InstrumentType instrumentType = 12;
* @param values The enum numeric values on the wire for instrumentType to add.
* @return This builder for chaining.
*/
public Builder addAllInstrumentTypeValue(
java.lang.Iterable values) {
ensureInstrumentTypeIsMutable();
for (int value : values) {
instrumentType_.addInt(value);
}
onChanged();
return this;
}
private java.util.List bulkSubscriptionFilter_ =
java.util.Collections.emptyList();
private void ensureBulkSubscriptionFilterIsMutable() {
if (!((bitField0_ & 0x00000080) != 0)) {
bulkSubscriptionFilter_ = new java.util.ArrayList(bulkSubscriptionFilter_);
bitField0_ |= 0x00000080;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
org.openfeed.BulkSubscriptionFilter, org.openfeed.BulkSubscriptionFilter.Builder, org.openfeed.BulkSubscriptionFilterOrBuilder> bulkSubscriptionFilterBuilder_;
/**
*
* / Filter for the exchange and channel subscriptions.
*
*
* repeated .org.openfeed.BulkSubscriptionFilter bulkSubscriptionFilter = 13;
*/
public java.util.List getBulkSubscriptionFilterList() {
if (bulkSubscriptionFilterBuilder_ == null) {
return java.util.Collections.unmodifiableList(bulkSubscriptionFilter_);
} else {
return bulkSubscriptionFilterBuilder_.getMessageList();
}
}
/**
*
* / Filter for the exchange and channel subscriptions.
*
*
* repeated .org.openfeed.BulkSubscriptionFilter bulkSubscriptionFilter = 13;
*/
public int getBulkSubscriptionFilterCount() {
if (bulkSubscriptionFilterBuilder_ == null) {
return bulkSubscriptionFilter_.size();
} else {
return bulkSubscriptionFilterBuilder_.getCount();
}
}
/**
*
* / Filter for the exchange and channel subscriptions.
*
*
* repeated .org.openfeed.BulkSubscriptionFilter bulkSubscriptionFilter = 13;
*/
public org.openfeed.BulkSubscriptionFilter getBulkSubscriptionFilter(int index) {
if (bulkSubscriptionFilterBuilder_ == null) {
return bulkSubscriptionFilter_.get(index);
} else {
return bulkSubscriptionFilterBuilder_.getMessage(index);
}
}
/**
*
* / Filter for the exchange and channel subscriptions.
*
*
* repeated .org.openfeed.BulkSubscriptionFilter bulkSubscriptionFilter = 13;
*/
public Builder setBulkSubscriptionFilter(
int index, org.openfeed.BulkSubscriptionFilter value) {
if (bulkSubscriptionFilterBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureBulkSubscriptionFilterIsMutable();
bulkSubscriptionFilter_.set(index, value);
onChanged();
} else {
bulkSubscriptionFilterBuilder_.setMessage(index, value);
}
return this;
}
/**
*
* / Filter for the exchange and channel subscriptions.
*
*
* repeated .org.openfeed.BulkSubscriptionFilter bulkSubscriptionFilter = 13;
*/
public Builder setBulkSubscriptionFilter(
int index, org.openfeed.BulkSubscriptionFilter.Builder builderForValue) {
if (bulkSubscriptionFilterBuilder_ == null) {
ensureBulkSubscriptionFilterIsMutable();
bulkSubscriptionFilter_.set(index, builderForValue.build());
onChanged();
} else {
bulkSubscriptionFilterBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
*
* / Filter for the exchange and channel subscriptions.
*
*
* repeated .org.openfeed.BulkSubscriptionFilter bulkSubscriptionFilter = 13;
*/
public Builder addBulkSubscriptionFilter(org.openfeed.BulkSubscriptionFilter value) {
if (bulkSubscriptionFilterBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureBulkSubscriptionFilterIsMutable();
bulkSubscriptionFilter_.add(value);
onChanged();
} else {
bulkSubscriptionFilterBuilder_.addMessage(value);
}
return this;
}
/**
*
* / Filter for the exchange and channel subscriptions.
*
*
* repeated .org.openfeed.BulkSubscriptionFilter bulkSubscriptionFilter = 13;
*/
public Builder addBulkSubscriptionFilter(
int index, org.openfeed.BulkSubscriptionFilter value) {
if (bulkSubscriptionFilterBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureBulkSubscriptionFilterIsMutable();
bulkSubscriptionFilter_.add(index, value);
onChanged();
} else {
bulkSubscriptionFilterBuilder_.addMessage(index, value);
}
return this;
}
/**
*
* / Filter for the exchange and channel subscriptions.
*
*
* repeated .org.openfeed.BulkSubscriptionFilter bulkSubscriptionFilter = 13;
*/
public Builder addBulkSubscriptionFilter(
org.openfeed.BulkSubscriptionFilter.Builder builderForValue) {
if (bulkSubscriptionFilterBuilder_ == null) {
ensureBulkSubscriptionFilterIsMutable();
bulkSubscriptionFilter_.add(builderForValue.build());
onChanged();
} else {
bulkSubscriptionFilterBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
*
* / Filter for the exchange and channel subscriptions.
*
*
* repeated .org.openfeed.BulkSubscriptionFilter bulkSubscriptionFilter = 13;
*/
public Builder addBulkSubscriptionFilter(
int index, org.openfeed.BulkSubscriptionFilter.Builder builderForValue) {
if (bulkSubscriptionFilterBuilder_ == null) {
ensureBulkSubscriptionFilterIsMutable();
bulkSubscriptionFilter_.add(index, builderForValue.build());
onChanged();
} else {
bulkSubscriptionFilterBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
*
* / Filter for the exchange and channel subscriptions.
*
*
* repeated .org.openfeed.BulkSubscriptionFilter bulkSubscriptionFilter = 13;
*/
public Builder addAllBulkSubscriptionFilter(
java.lang.Iterable extends org.openfeed.BulkSubscriptionFilter> values) {
if (bulkSubscriptionFilterBuilder_ == null) {
ensureBulkSubscriptionFilterIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, bulkSubscriptionFilter_);
onChanged();
} else {
bulkSubscriptionFilterBuilder_.addAllMessages(values);
}
return this;
}
/**
*
* / Filter for the exchange and channel subscriptions.
*
*
* repeated .org.openfeed.BulkSubscriptionFilter bulkSubscriptionFilter = 13;
*/
public Builder clearBulkSubscriptionFilter() {
if (bulkSubscriptionFilterBuilder_ == null) {
bulkSubscriptionFilter_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000080);
onChanged();
} else {
bulkSubscriptionFilterBuilder_.clear();
}
return this;
}
/**
*
* / Filter for the exchange and channel subscriptions.
*
*
* repeated .org.openfeed.BulkSubscriptionFilter bulkSubscriptionFilter = 13;
*/
public Builder removeBulkSubscriptionFilter(int index) {
if (bulkSubscriptionFilterBuilder_ == null) {
ensureBulkSubscriptionFilterIsMutable();
bulkSubscriptionFilter_.remove(index);
onChanged();
} else {
bulkSubscriptionFilterBuilder_.remove(index);
}
return this;
}
/**
*
* / Filter for the exchange and channel subscriptions.
*
*
* repeated .org.openfeed.BulkSubscriptionFilter bulkSubscriptionFilter = 13;
*/
public org.openfeed.BulkSubscriptionFilter.Builder getBulkSubscriptionFilterBuilder(
int index) {
return getBulkSubscriptionFilterFieldBuilder().getBuilder(index);
}
/**
*
* / Filter for the exchange and channel subscriptions.
*
*
* repeated .org.openfeed.BulkSubscriptionFilter bulkSubscriptionFilter = 13;
*/
public org.openfeed.BulkSubscriptionFilterOrBuilder getBulkSubscriptionFilterOrBuilder(
int index) {
if (bulkSubscriptionFilterBuilder_ == null) {
return bulkSubscriptionFilter_.get(index); } else {
return bulkSubscriptionFilterBuilder_.getMessageOrBuilder(index);
}
}
/**
*
* / Filter for the exchange and channel subscriptions.
*
*
* repeated .org.openfeed.BulkSubscriptionFilter bulkSubscriptionFilter = 13;
*/
public java.util.List extends org.openfeed.BulkSubscriptionFilterOrBuilder>
getBulkSubscriptionFilterOrBuilderList() {
if (bulkSubscriptionFilterBuilder_ != null) {
return bulkSubscriptionFilterBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(bulkSubscriptionFilter_);
}
}
/**
*
* / Filter for the exchange and channel subscriptions.
*
*
* repeated .org.openfeed.BulkSubscriptionFilter bulkSubscriptionFilter = 13;
*/
public org.openfeed.BulkSubscriptionFilter.Builder addBulkSubscriptionFilterBuilder() {
return getBulkSubscriptionFilterFieldBuilder().addBuilder(
org.openfeed.BulkSubscriptionFilter.getDefaultInstance());
}
/**
*
* / Filter for the exchange and channel subscriptions.
*
*
* repeated .org.openfeed.BulkSubscriptionFilter bulkSubscriptionFilter = 13;
*/
public org.openfeed.BulkSubscriptionFilter.Builder addBulkSubscriptionFilterBuilder(
int index) {
return getBulkSubscriptionFilterFieldBuilder().addBuilder(
index, org.openfeed.BulkSubscriptionFilter.getDefaultInstance());
}
/**
*
* / Filter for the exchange and channel subscriptions.
*
*
* repeated .org.openfeed.BulkSubscriptionFilter bulkSubscriptionFilter = 13;
*/
public java.util.List
getBulkSubscriptionFilterBuilderList() {
return getBulkSubscriptionFilterFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
org.openfeed.BulkSubscriptionFilter, org.openfeed.BulkSubscriptionFilter.Builder, org.openfeed.BulkSubscriptionFilterOrBuilder>
getBulkSubscriptionFilterFieldBuilder() {
if (bulkSubscriptionFilterBuilder_ == null) {
bulkSubscriptionFilterBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
org.openfeed.BulkSubscriptionFilter, org.openfeed.BulkSubscriptionFilter.Builder, org.openfeed.BulkSubscriptionFilterOrBuilder>(
bulkSubscriptionFilter_,
((bitField0_ & 0x00000080) != 0),
getParentForChildren(),
isClean());
bulkSubscriptionFilter_ = null;
}
return bulkSubscriptionFilterBuilder_;
}
private com.google.protobuf.LazyStringArrayList spreadTypeFilter_ =
com.google.protobuf.LazyStringArrayList.emptyList();
private void ensureSpreadTypeFilterIsMutable() {
if (!spreadTypeFilter_.isModifiable()) {
spreadTypeFilter_ = new com.google.protobuf.LazyStringArrayList(spreadTypeFilter_);
}
bitField0_ |= 0x00000100;
}
/**
*
* / Filter for Spread Types
*
*
* repeated string spreadTypeFilter = 14;
* @return A list containing the spreadTypeFilter.
*/
public com.google.protobuf.ProtocolStringList
getSpreadTypeFilterList() {
spreadTypeFilter_.makeImmutable();
return spreadTypeFilter_;
}
/**
*
* / Filter for Spread Types
*
*
* repeated string spreadTypeFilter = 14;
* @return The count of spreadTypeFilter.
*/
public int getSpreadTypeFilterCount() {
return spreadTypeFilter_.size();
}
/**
*
* / Filter for Spread Types
*
*
* repeated string spreadTypeFilter = 14;
* @param index The index of the element to return.
* @return The spreadTypeFilter at the given index.
*/
public java.lang.String getSpreadTypeFilter(int index) {
return spreadTypeFilter_.get(index);
}
/**
*
* / Filter for Spread Types
*
*
* repeated string spreadTypeFilter = 14;
* @param index The index of the value to return.
* @return The bytes of the spreadTypeFilter at the given index.
*/
public com.google.protobuf.ByteString
getSpreadTypeFilterBytes(int index) {
return spreadTypeFilter_.getByteString(index);
}
/**
*
* / Filter for Spread Types
*
*
* repeated string spreadTypeFilter = 14;
* @param index The index to set the value at.
* @param value The spreadTypeFilter to set.
* @return This builder for chaining.
*/
public Builder setSpreadTypeFilter(
int index, java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
ensureSpreadTypeFilterIsMutable();
spreadTypeFilter_.set(index, value);
bitField0_ |= 0x00000100;
onChanged();
return this;
}
/**
*
* / Filter for Spread Types
*
*
* repeated string spreadTypeFilter = 14;
* @param value The spreadTypeFilter to add.
* @return This builder for chaining.
*/
public Builder addSpreadTypeFilter(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
ensureSpreadTypeFilterIsMutable();
spreadTypeFilter_.add(value);
bitField0_ |= 0x00000100;
onChanged();
return this;
}
/**
*
* / Filter for Spread Types
*
*
* repeated string spreadTypeFilter = 14;
* @param values The spreadTypeFilter to add.
* @return This builder for chaining.
*/
public Builder addAllSpreadTypeFilter(
java.lang.Iterable values) {
ensureSpreadTypeFilterIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, spreadTypeFilter_);
bitField0_ |= 0x00000100;
onChanged();
return this;
}
/**
*
* / Filter for Spread Types
*
*
* repeated string spreadTypeFilter = 14;
* @return This builder for chaining.
*/
public Builder clearSpreadTypeFilter() {
spreadTypeFilter_ =
com.google.protobuf.LazyStringArrayList.emptyList();
bitField0_ = (bitField0_ & ~0x00000100);;
onChanged();
return this;
}
/**
*
* / Filter for Spread Types
*
*
* repeated string spreadTypeFilter = 14;
* @param value The bytes of the spreadTypeFilter to add.
* @return This builder for chaining.
*/
public Builder addSpreadTypeFilterBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
ensureSpreadTypeFilterIsMutable();
spreadTypeFilter_.add(value);
bitField0_ |= 0x00000100;
onChanged();
return this;
}
private boolean subscriptionDoNotSendInstruments_ ;
/**
*
* / Do not send instrument(s) on successful subscription
*
*
* bool subscriptionDoNotSendInstruments = 15;
* @return The subscriptionDoNotSendInstruments.
*/
@java.lang.Override
public boolean getSubscriptionDoNotSendInstruments() {
return subscriptionDoNotSendInstruments_;
}
/**
*
* / Do not send instrument(s) on successful subscription
*
*
* bool subscriptionDoNotSendInstruments = 15;
* @param value The subscriptionDoNotSendInstruments to set.
* @return This builder for chaining.
*/
public Builder setSubscriptionDoNotSendInstruments(boolean value) {
subscriptionDoNotSendInstruments_ = value;
bitField0_ |= 0x00000200;
onChanged();
return this;
}
/**
*
* / Do not send instrument(s) on successful subscription
*
*
* bool subscriptionDoNotSendInstruments = 15;
* @return This builder for chaining.
*/
public Builder clearSubscriptionDoNotSendInstruments() {
bitField0_ = (bitField0_ & ~0x00000200);
subscriptionDoNotSendInstruments_ = false;
onChanged();
return this;
}
private boolean subscriptionDoNotSendSnapshots_ ;
/**
*
* / Do not send market snapshot(s) on successful subscription
*
*
* bool subscriptionDoNotSendSnapshots = 16;
* @return The subscriptionDoNotSendSnapshots.
*/
@java.lang.Override
public boolean getSubscriptionDoNotSendSnapshots() {
return subscriptionDoNotSendSnapshots_;
}
/**
*
* / Do not send market snapshot(s) on successful subscription
*
*
* bool subscriptionDoNotSendSnapshots = 16;
* @param value The subscriptionDoNotSendSnapshots to set.
* @return This builder for chaining.
*/
public Builder setSubscriptionDoNotSendSnapshots(boolean value) {
subscriptionDoNotSendSnapshots_ = value;
bitField0_ |= 0x00000400;
onChanged();
return this;
}
/**
*
* / Do not send market snapshot(s) on successful subscription
*
*
* bool subscriptionDoNotSendSnapshots = 16;
* @return This builder for chaining.
*/
public Builder clearSubscriptionDoNotSendSnapshots() {
bitField0_ = (bitField0_ & ~0x00000400);
subscriptionDoNotSendSnapshots_ = false;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:org.openfeed.SubscriptionRequest.Request)
}
// @@protoc_insertion_point(class_scope:org.openfeed.SubscriptionRequest.Request)
private static final org.openfeed.SubscriptionRequest.Request DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.openfeed.SubscriptionRequest.Request();
}
public static org.openfeed.SubscriptionRequest.Request getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public Request parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.openfeed.SubscriptionRequest.Request getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public static final int CORRELATIONID_FIELD_NUMBER = 1;
private long correlationId_ = 0L;
/**
*
* / Client-assigned id for this request. Response will include same id
*
*
* sint64 correlationId = 1;
* @return The correlationId.
*/
@java.lang.Override
public long getCorrelationId() {
return correlationId_;
}
public static final int TOKEN_FIELD_NUMBER = 2;
@SuppressWarnings("serial")
private volatile java.lang.Object token_ = "";
/**
* string token = 2;
* @return The token.
*/
@java.lang.Override
public java.lang.String getToken() {
java.lang.Object ref = token_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
token_ = s;
return s;
}
}
/**
* string token = 2;
* @return The bytes for token.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getTokenBytes() {
java.lang.Object ref = token_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
token_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int SERVICE_FIELD_NUMBER = 3;
private int service_ = 0;
/**
*
* / Preferred service (realtime or delayed). REAL_TIME is the default.
*
*
* .org.openfeed.Service service = 3;
* @return The enum numeric value on the wire for service.
*/
@java.lang.Override public int getServiceValue() {
return service_;
}
/**
*
* / Preferred service (realtime or delayed). REAL_TIME is the default.
*
*
* .org.openfeed.Service service = 3;
* @return The service.
*/
@java.lang.Override public org.openfeed.Service getService() {
org.openfeed.Service result = org.openfeed.Service.forNumber(service_);
return result == null ? org.openfeed.Service.UNRECOGNIZED : result;
}
public static final int UNSUBSCRIBE_FIELD_NUMBER = 4;
private boolean unsubscribe_ = false;
/**
* bool unsubscribe = 4;
* @return The unsubscribe.
*/
@java.lang.Override
public boolean getUnsubscribe() {
return unsubscribe_;
}
public static final int REQUESTS_FIELD_NUMBER = 5;
@SuppressWarnings("serial")
private java.util.List requests_;
/**
* repeated .org.openfeed.SubscriptionRequest.Request requests = 5;
*/
@java.lang.Override
public java.util.List getRequestsList() {
return requests_;
}
/**
* repeated .org.openfeed.SubscriptionRequest.Request requests = 5;
*/
@java.lang.Override
public java.util.List extends org.openfeed.SubscriptionRequest.RequestOrBuilder>
getRequestsOrBuilderList() {
return requests_;
}
/**
* repeated .org.openfeed.SubscriptionRequest.Request requests = 5;
*/
@java.lang.Override
public int getRequestsCount() {
return requests_.size();
}
/**
* repeated .org.openfeed.SubscriptionRequest.Request requests = 5;
*/
@java.lang.Override
public org.openfeed.SubscriptionRequest.Request getRequests(int index) {
return requests_.get(index);
}
/**
* repeated .org.openfeed.SubscriptionRequest.Request requests = 5;
*/
@java.lang.Override
public org.openfeed.SubscriptionRequest.RequestOrBuilder getRequestsOrBuilder(
int index) {
return requests_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (correlationId_ != 0L) {
output.writeSInt64(1, correlationId_);
}
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(token_)) {
com.google.protobuf.GeneratedMessage.writeString(output, 2, token_);
}
if (service_ != org.openfeed.Service.UNKNOWN_SERVICE.getNumber()) {
output.writeEnum(3, service_);
}
if (unsubscribe_ != false) {
output.writeBool(4, unsubscribe_);
}
for (int i = 0; i < requests_.size(); i++) {
output.writeMessage(5, requests_.get(i));
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (correlationId_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeSInt64Size(1, correlationId_);
}
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(token_)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(2, token_);
}
if (service_ != org.openfeed.Service.UNKNOWN_SERVICE.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(3, service_);
}
if (unsubscribe_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(4, unsubscribe_);
}
for (int i = 0; i < requests_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(5, requests_.get(i));
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.openfeed.SubscriptionRequest)) {
return super.equals(obj);
}
org.openfeed.SubscriptionRequest other = (org.openfeed.SubscriptionRequest) obj;
if (getCorrelationId()
!= other.getCorrelationId()) return false;
if (!getToken()
.equals(other.getToken())) return false;
if (service_ != other.service_) return false;
if (getUnsubscribe()
!= other.getUnsubscribe()) return false;
if (!getRequestsList()
.equals(other.getRequestsList())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + CORRELATIONID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getCorrelationId());
hash = (37 * hash) + TOKEN_FIELD_NUMBER;
hash = (53 * hash) + getToken().hashCode();
hash = (37 * hash) + SERVICE_FIELD_NUMBER;
hash = (53 * hash) + service_;
hash = (37 * hash) + UNSUBSCRIBE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getUnsubscribe());
if (getRequestsCount() > 0) {
hash = (37 * hash) + REQUESTS_FIELD_NUMBER;
hash = (53 * hash) + getRequestsList().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.openfeed.SubscriptionRequest parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.openfeed.SubscriptionRequest parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.openfeed.SubscriptionRequest parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.openfeed.SubscriptionRequest parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.openfeed.SubscriptionRequest parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.openfeed.SubscriptionRequest parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.openfeed.SubscriptionRequest parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static org.openfeed.SubscriptionRequest parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.openfeed.SubscriptionRequest parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input);
}
public static org.openfeed.SubscriptionRequest parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.openfeed.SubscriptionRequest parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static org.openfeed.SubscriptionRequest parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.openfeed.SubscriptionRequest prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* / Subscription Request
*
*
* Protobuf type {@code org.openfeed.SubscriptionRequest}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:org.openfeed.SubscriptionRequest)
org.openfeed.SubscriptionRequestOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.openfeed.OpenfeedApi.internal_static_org_openfeed_SubscriptionRequest_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.openfeed.OpenfeedApi.internal_static_org_openfeed_SubscriptionRequest_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.openfeed.SubscriptionRequest.class, org.openfeed.SubscriptionRequest.Builder.class);
}
// Construct using org.openfeed.SubscriptionRequest.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
correlationId_ = 0L;
token_ = "";
service_ = 0;
unsubscribe_ = false;
if (requestsBuilder_ == null) {
requests_ = java.util.Collections.emptyList();
} else {
requests_ = null;
requestsBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000010);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.openfeed.OpenfeedApi.internal_static_org_openfeed_SubscriptionRequest_descriptor;
}
@java.lang.Override
public org.openfeed.SubscriptionRequest getDefaultInstanceForType() {
return org.openfeed.SubscriptionRequest.getDefaultInstance();
}
@java.lang.Override
public org.openfeed.SubscriptionRequest build() {
org.openfeed.SubscriptionRequest result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.openfeed.SubscriptionRequest buildPartial() {
org.openfeed.SubscriptionRequest result = new org.openfeed.SubscriptionRequest(this);
buildPartialRepeatedFields(result);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartialRepeatedFields(org.openfeed.SubscriptionRequest result) {
if (requestsBuilder_ == null) {
if (((bitField0_ & 0x00000010) != 0)) {
requests_ = java.util.Collections.unmodifiableList(requests_);
bitField0_ = (bitField0_ & ~0x00000010);
}
result.requests_ = requests_;
} else {
result.requests_ = requestsBuilder_.build();
}
}
private void buildPartial0(org.openfeed.SubscriptionRequest result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.correlationId_ = correlationId_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.token_ = token_;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.service_ = service_;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.unsubscribe_ = unsubscribe_;
}
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.openfeed.SubscriptionRequest) {
return mergeFrom((org.openfeed.SubscriptionRequest)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.openfeed.SubscriptionRequest other) {
if (other == org.openfeed.SubscriptionRequest.getDefaultInstance()) return this;
if (other.getCorrelationId() != 0L) {
setCorrelationId(other.getCorrelationId());
}
if (!other.getToken().isEmpty()) {
token_ = other.token_;
bitField0_ |= 0x00000002;
onChanged();
}
if (other.service_ != 0) {
setServiceValue(other.getServiceValue());
}
if (other.getUnsubscribe() != false) {
setUnsubscribe(other.getUnsubscribe());
}
if (requestsBuilder_ == null) {
if (!other.requests_.isEmpty()) {
if (requests_.isEmpty()) {
requests_ = other.requests_;
bitField0_ = (bitField0_ & ~0x00000010);
} else {
ensureRequestsIsMutable();
requests_.addAll(other.requests_);
}
onChanged();
}
} else {
if (!other.requests_.isEmpty()) {
if (requestsBuilder_.isEmpty()) {
requestsBuilder_.dispose();
requestsBuilder_ = null;
requests_ = other.requests_;
bitField0_ = (bitField0_ & ~0x00000010);
requestsBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getRequestsFieldBuilder() : null;
} else {
requestsBuilder_.addAllMessages(other.requests_);
}
}
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
correlationId_ = input.readSInt64();
bitField0_ |= 0x00000001;
break;
} // case 8
case 18: {
token_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000002;
break;
} // case 18
case 24: {
service_ = input.readEnum();
bitField0_ |= 0x00000004;
break;
} // case 24
case 32: {
unsubscribe_ = input.readBool();
bitField0_ |= 0x00000008;
break;
} // case 32
case 42: {
org.openfeed.SubscriptionRequest.Request m =
input.readMessage(
org.openfeed.SubscriptionRequest.Request.parser(),
extensionRegistry);
if (requestsBuilder_ == null) {
ensureRequestsIsMutable();
requests_.add(m);
} else {
requestsBuilder_.addMessage(m);
}
break;
} // case 42
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private long correlationId_ ;
/**
*
* / Client-assigned id for this request. Response will include same id
*
*
* sint64 correlationId = 1;
* @return The correlationId.
*/
@java.lang.Override
public long getCorrelationId() {
return correlationId_;
}
/**
*
* / Client-assigned id for this request. Response will include same id
*
*
* sint64 correlationId = 1;
* @param value The correlationId to set.
* @return This builder for chaining.
*/
public Builder setCorrelationId(long value) {
correlationId_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* / Client-assigned id for this request. Response will include same id
*
*
* sint64 correlationId = 1;
* @return This builder for chaining.
*/
public Builder clearCorrelationId() {
bitField0_ = (bitField0_ & ~0x00000001);
correlationId_ = 0L;
onChanged();
return this;
}
private java.lang.Object token_ = "";
/**
* string token = 2;
* @return The token.
*/
public java.lang.String getToken() {
java.lang.Object ref = token_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
token_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string token = 2;
* @return The bytes for token.
*/
public com.google.protobuf.ByteString
getTokenBytes() {
java.lang.Object ref = token_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
token_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string token = 2;
* @param value The token to set.
* @return This builder for chaining.
*/
public Builder setToken(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
token_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* string token = 2;
* @return This builder for chaining.
*/
public Builder clearToken() {
token_ = getDefaultInstance().getToken();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
* string token = 2;
* @param value The bytes for token to set.
* @return This builder for chaining.
*/
public Builder setTokenBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
token_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
private int service_ = 0;
/**
*
* / Preferred service (realtime or delayed). REAL_TIME is the default.
*
*
* .org.openfeed.Service service = 3;
* @return The enum numeric value on the wire for service.
*/
@java.lang.Override public int getServiceValue() {
return service_;
}
/**
*
* / Preferred service (realtime or delayed). REAL_TIME is the default.
*
*
* .org.openfeed.Service service = 3;
* @param value The enum numeric value on the wire for service to set.
* @return This builder for chaining.
*/
public Builder setServiceValue(int value) {
service_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
* / Preferred service (realtime or delayed). REAL_TIME is the default.
*
*
* .org.openfeed.Service service = 3;
* @return The service.
*/
@java.lang.Override
public org.openfeed.Service getService() {
org.openfeed.Service result = org.openfeed.Service.forNumber(service_);
return result == null ? org.openfeed.Service.UNRECOGNIZED : result;
}
/**
*
* / Preferred service (realtime or delayed). REAL_TIME is the default.
*
*
* .org.openfeed.Service service = 3;
* @param value The service to set.
* @return This builder for chaining.
*/
public Builder setService(org.openfeed.Service value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000004;
service_ = value.getNumber();
onChanged();
return this;
}
/**
*
* / Preferred service (realtime or delayed). REAL_TIME is the default.
*
*
* .org.openfeed.Service service = 3;
* @return This builder for chaining.
*/
public Builder clearService() {
bitField0_ = (bitField0_ & ~0x00000004);
service_ = 0;
onChanged();
return this;
}
private boolean unsubscribe_ ;
/**
* bool unsubscribe = 4;
* @return The unsubscribe.
*/
@java.lang.Override
public boolean getUnsubscribe() {
return unsubscribe_;
}
/**
* bool unsubscribe = 4;
* @param value The unsubscribe to set.
* @return This builder for chaining.
*/
public Builder setUnsubscribe(boolean value) {
unsubscribe_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
* bool unsubscribe = 4;
* @return This builder for chaining.
*/
public Builder clearUnsubscribe() {
bitField0_ = (bitField0_ & ~0x00000008);
unsubscribe_ = false;
onChanged();
return this;
}
private java.util.List requests_ =
java.util.Collections.emptyList();
private void ensureRequestsIsMutable() {
if (!((bitField0_ & 0x00000010) != 0)) {
requests_ = new java.util.ArrayList(requests_);
bitField0_ |= 0x00000010;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
org.openfeed.SubscriptionRequest.Request, org.openfeed.SubscriptionRequest.Request.Builder, org.openfeed.SubscriptionRequest.RequestOrBuilder> requestsBuilder_;
/**
* repeated .org.openfeed.SubscriptionRequest.Request requests = 5;
*/
public java.util.List getRequestsList() {
if (requestsBuilder_ == null) {
return java.util.Collections.unmodifiableList(requests_);
} else {
return requestsBuilder_.getMessageList();
}
}
/**
* repeated .org.openfeed.SubscriptionRequest.Request requests = 5;
*/
public int getRequestsCount() {
if (requestsBuilder_ == null) {
return requests_.size();
} else {
return requestsBuilder_.getCount();
}
}
/**
* repeated .org.openfeed.SubscriptionRequest.Request requests = 5;
*/
public org.openfeed.SubscriptionRequest.Request getRequests(int index) {
if (requestsBuilder_ == null) {
return requests_.get(index);
} else {
return requestsBuilder_.getMessage(index);
}
}
/**
* repeated .org.openfeed.SubscriptionRequest.Request requests = 5;
*/
public Builder setRequests(
int index, org.openfeed.SubscriptionRequest.Request value) {
if (requestsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureRequestsIsMutable();
requests_.set(index, value);
onChanged();
} else {
requestsBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .org.openfeed.SubscriptionRequest.Request requests = 5;
*/
public Builder setRequests(
int index, org.openfeed.SubscriptionRequest.Request.Builder builderForValue) {
if (requestsBuilder_ == null) {
ensureRequestsIsMutable();
requests_.set(index, builderForValue.build());
onChanged();
} else {
requestsBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .org.openfeed.SubscriptionRequest.Request requests = 5;
*/
public Builder addRequests(org.openfeed.SubscriptionRequest.Request value) {
if (requestsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureRequestsIsMutable();
requests_.add(value);
onChanged();
} else {
requestsBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .org.openfeed.SubscriptionRequest.Request requests = 5;
*/
public Builder addRequests(
int index, org.openfeed.SubscriptionRequest.Request value) {
if (requestsBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensureRequestsIsMutable();
requests_.add(index, value);
onChanged();
} else {
requestsBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .org.openfeed.SubscriptionRequest.Request requests = 5;
*/
public Builder addRequests(
org.openfeed.SubscriptionRequest.Request.Builder builderForValue) {
if (requestsBuilder_ == null) {
ensureRequestsIsMutable();
requests_.add(builderForValue.build());
onChanged();
} else {
requestsBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .org.openfeed.SubscriptionRequest.Request requests = 5;
*/
public Builder addRequests(
int index, org.openfeed.SubscriptionRequest.Request.Builder builderForValue) {
if (requestsBuilder_ == null) {
ensureRequestsIsMutable();
requests_.add(index, builderForValue.build());
onChanged();
} else {
requestsBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .org.openfeed.SubscriptionRequest.Request requests = 5;
*/
public Builder addAllRequests(
java.lang.Iterable extends org.openfeed.SubscriptionRequest.Request> values) {
if (requestsBuilder_ == null) {
ensureRequestsIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, requests_);
onChanged();
} else {
requestsBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .org.openfeed.SubscriptionRequest.Request requests = 5;
*/
public Builder clearRequests() {
if (requestsBuilder_ == null) {
requests_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000010);
onChanged();
} else {
requestsBuilder_.clear();
}
return this;
}
/**
* repeated .org.openfeed.SubscriptionRequest.Request requests = 5;
*/
public Builder removeRequests(int index) {
if (requestsBuilder_ == null) {
ensureRequestsIsMutable();
requests_.remove(index);
onChanged();
} else {
requestsBuilder_.remove(index);
}
return this;
}
/**
* repeated .org.openfeed.SubscriptionRequest.Request requests = 5;
*/
public org.openfeed.SubscriptionRequest.Request.Builder getRequestsBuilder(
int index) {
return getRequestsFieldBuilder().getBuilder(index);
}
/**
* repeated .org.openfeed.SubscriptionRequest.Request requests = 5;
*/
public org.openfeed.SubscriptionRequest.RequestOrBuilder getRequestsOrBuilder(
int index) {
if (requestsBuilder_ == null) {
return requests_.get(index); } else {
return requestsBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .org.openfeed.SubscriptionRequest.Request requests = 5;
*/
public java.util.List extends org.openfeed.SubscriptionRequest.RequestOrBuilder>
getRequestsOrBuilderList() {
if (requestsBuilder_ != null) {
return requestsBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(requests_);
}
}
/**
* repeated .org.openfeed.SubscriptionRequest.Request requests = 5;
*/
public org.openfeed.SubscriptionRequest.Request.Builder addRequestsBuilder() {
return getRequestsFieldBuilder().addBuilder(
org.openfeed.SubscriptionRequest.Request.getDefaultInstance());
}
/**
* repeated .org.openfeed.SubscriptionRequest.Request requests = 5;
*/
public org.openfeed.SubscriptionRequest.Request.Builder addRequestsBuilder(
int index) {
return getRequestsFieldBuilder().addBuilder(
index, org.openfeed.SubscriptionRequest.Request.getDefaultInstance());
}
/**
* repeated .org.openfeed.SubscriptionRequest.Request requests = 5;
*/
public java.util.List
getRequestsBuilderList() {
return getRequestsFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
org.openfeed.SubscriptionRequest.Request, org.openfeed.SubscriptionRequest.Request.Builder, org.openfeed.SubscriptionRequest.RequestOrBuilder>
getRequestsFieldBuilder() {
if (requestsBuilder_ == null) {
requestsBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
org.openfeed.SubscriptionRequest.Request, org.openfeed.SubscriptionRequest.Request.Builder, org.openfeed.SubscriptionRequest.RequestOrBuilder>(
requests_,
((bitField0_ & 0x00000010) != 0),
getParentForChildren(),
isClean());
requests_ = null;
}
return requestsBuilder_;
}
// @@protoc_insertion_point(builder_scope:org.openfeed.SubscriptionRequest)
}
// @@protoc_insertion_point(class_scope:org.openfeed.SubscriptionRequest)
private static final org.openfeed.SubscriptionRequest DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.openfeed.SubscriptionRequest();
}
public static org.openfeed.SubscriptionRequest getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public SubscriptionRequest parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.openfeed.SubscriptionRequest getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy