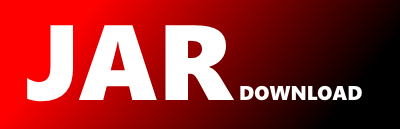
org.openfeed.TradeCorrection Maven / Gradle / Ivy
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// NO CHECKED-IN PROTOBUF GENCODE
// source: openfeed.proto
// Protobuf Java Version: 4.28.3
package org.openfeed;
/**
*
* / Trade Correction
*
*
* Protobuf type {@code org.openfeed.TradeCorrection}
*/
public final class TradeCorrection extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:org.openfeed.TradeCorrection)
TradeCorrectionOrBuilder {
private static final long serialVersionUID = 0L;
static {
com.google.protobuf.RuntimeVersion.validateProtobufGencodeVersion(
com.google.protobuf.RuntimeVersion.RuntimeDomain.PUBLIC,
/* major= */ 4,
/* minor= */ 28,
/* patch= */ 3,
/* suffix= */ "",
TradeCorrection.class.getName());
}
// Use TradeCorrection.newBuilder() to construct.
private TradeCorrection(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private TradeCorrection() {
originatorId_ = com.google.protobuf.ByteString.EMPTY;
tradeId_ = com.google.protobuf.ByteString.EMPTY;
side_ = 0;
buyerId_ = com.google.protobuf.ByteString.EMPTY;
sellerId_ = com.google.protobuf.ByteString.EMPTY;
settlementTerms_ = 0;
crossType_ = 0;
originalTradeId_ = com.google.protobuf.ByteString.EMPTY;
saleCondition_ = com.google.protobuf.ByteString.EMPTY;
currency_ = "";
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.openfeed.Openfeed.internal_static_org_openfeed_TradeCorrection_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.openfeed.Openfeed.internal_static_org_openfeed_TradeCorrection_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.openfeed.TradeCorrection.class, org.openfeed.TradeCorrection.Builder.class);
}
public static final int ORIGINATORID_FIELD_NUMBER = 8;
private com.google.protobuf.ByteString originatorId_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* Market participant/originator
*
*
* bytes originatorId = 8;
* @return The originatorId.
*/
@java.lang.Override
public com.google.protobuf.ByteString getOriginatorId() {
return originatorId_;
}
public static final int TRANSACTIONTIME_FIELD_NUMBER = 9;
private long transactionTime_ = 0L;
/**
* sint64 transactionTime = 9;
* @return The transactionTime.
*/
@java.lang.Override
public long getTransactionTime() {
return transactionTime_;
}
public static final int PRICE_FIELD_NUMBER = 10;
private long price_ = 0L;
/**
*
* Corrected Price
*
*
* sint64 price = 10;
* @return The price.
*/
@java.lang.Override
public long getPrice() {
return price_;
}
public static final int QUANTITY_FIELD_NUMBER = 11;
private long quantity_ = 0L;
/**
*
* Corrected Quantity
*
*
* sint64 quantity = 11;
* @return The quantity.
*/
@java.lang.Override
public long getQuantity() {
return quantity_;
}
public static final int TRADEID_FIELD_NUMBER = 12;
private com.google.protobuf.ByteString tradeId_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes tradeId = 12;
* @return The tradeId.
*/
@java.lang.Override
public com.google.protobuf.ByteString getTradeId() {
return tradeId_;
}
public static final int SIDE_FIELD_NUMBER = 13;
private int side_ = 0;
/**
* .org.openfeed.BookSide side = 13;
* @return The enum numeric value on the wire for side.
*/
@java.lang.Override public int getSideValue() {
return side_;
}
/**
* .org.openfeed.BookSide side = 13;
* @return The side.
*/
@java.lang.Override public org.openfeed.BookSide getSide() {
org.openfeed.BookSide result = org.openfeed.BookSide.forNumber(side_);
return result == null ? org.openfeed.BookSide.UNRECOGNIZED : result;
}
public static final int TRADEDATE_FIELD_NUMBER = 14;
private int tradeDate_ = 0;
/**
*
* Date only, format 2012-07-04 -> 20120704
*
*
* sint32 tradeDate = 14;
* @return The tradeDate.
*/
@java.lang.Override
public int getTradeDate() {
return tradeDate_;
}
public static final int BUYERID_FIELD_NUMBER = 15;
private com.google.protobuf.ByteString buyerId_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes buyerId = 15;
* @return The buyerId.
*/
@java.lang.Override
public com.google.protobuf.ByteString getBuyerId() {
return buyerId_;
}
public static final int SELLERID_FIELD_NUMBER = 16;
private com.google.protobuf.ByteString sellerId_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes sellerId = 16;
* @return The sellerId.
*/
@java.lang.Override
public com.google.protobuf.ByteString getSellerId() {
return sellerId_;
}
public static final int OPENINGTRADE_FIELD_NUMBER = 17;
private boolean openingTrade_ = false;
/**
* bool openingTrade = 17;
* @return The openingTrade.
*/
@java.lang.Override
public boolean getOpeningTrade() {
return openingTrade_;
}
public static final int SYSTEMPRICED_FIELD_NUMBER = 18;
private boolean systemPriced_ = false;
/**
* bool systemPriced = 18;
* @return The systemPriced.
*/
@java.lang.Override
public boolean getSystemPriced() {
return systemPriced_;
}
public static final int MARKETONCLOSE_FIELD_NUMBER = 19;
private boolean marketOnClose_ = false;
/**
* bool marketOnClose = 19;
* @return The marketOnClose.
*/
@java.lang.Override
public boolean getMarketOnClose() {
return marketOnClose_;
}
public static final int ODDLOT_FIELD_NUMBER = 20;
private boolean oddLot_ = false;
/**
* bool oddLot = 20;
* @return The oddLot.
*/
@java.lang.Override
public boolean getOddLot() {
return oddLot_;
}
public static final int SETTLEMENTTERMS_FIELD_NUMBER = 21;
private int settlementTerms_ = 0;
/**
* .org.openfeed.SettlementTerms settlementTerms = 21;
* @return The enum numeric value on the wire for settlementTerms.
*/
@java.lang.Override public int getSettlementTermsValue() {
return settlementTerms_;
}
/**
* .org.openfeed.SettlementTerms settlementTerms = 21;
* @return The settlementTerms.
*/
@java.lang.Override public org.openfeed.SettlementTerms getSettlementTerms() {
org.openfeed.SettlementTerms result = org.openfeed.SettlementTerms.forNumber(settlementTerms_);
return result == null ? org.openfeed.SettlementTerms.UNRECOGNIZED : result;
}
public static final int CROSSTYPE_FIELD_NUMBER = 22;
private int crossType_ = 0;
/**
* .org.openfeed.CrossType crossType = 22;
* @return The enum numeric value on the wire for crossType.
*/
@java.lang.Override public int getCrossTypeValue() {
return crossType_;
}
/**
* .org.openfeed.CrossType crossType = 22;
* @return The crossType.
*/
@java.lang.Override public org.openfeed.CrossType getCrossType() {
org.openfeed.CrossType result = org.openfeed.CrossType.forNumber(crossType_);
return result == null ? org.openfeed.CrossType.UNRECOGNIZED : result;
}
public static final int BYPASS_FIELD_NUMBER = 23;
private boolean byPass_ = false;
/**
* bool byPass = 23;
* @return The byPass.
*/
@java.lang.Override
public boolean getByPass() {
return byPass_;
}
public static final int ORIGINALTRADEID_FIELD_NUMBER = 24;
private com.google.protobuf.ByteString originalTradeId_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes originalTradeId = 24;
* @return The originalTradeId.
*/
@java.lang.Override
public com.google.protobuf.ByteString getOriginalTradeId() {
return originalTradeId_;
}
public static final int SALECONDITION_FIELD_NUMBER = 25;
private com.google.protobuf.ByteString saleCondition_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes saleCondition = 25;
* @return The saleCondition.
*/
@java.lang.Override
public com.google.protobuf.ByteString getSaleCondition() {
return saleCondition_;
}
public static final int CURRENCY_FIELD_NUMBER = 26;
@SuppressWarnings("serial")
private volatile java.lang.Object currency_ = "";
/**
* string currency = 26;
* @return The currency.
*/
@java.lang.Override
public java.lang.String getCurrency() {
java.lang.Object ref = currency_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
currency_ = s;
return s;
}
}
/**
* string currency = 26;
* @return The bytes for currency.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getCurrencyBytes() {
java.lang.Object ref = currency_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
currency_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int DISTRIBUTIONTIME_FIELD_NUMBER = 27;
private long distributionTime_ = 0L;
/**
*
* / Distribution time in nano seconds since epoch.
*
*
* sint64 distributionTime = 27;
* @return The distributionTime.
*/
@java.lang.Override
public long getDistributionTime() {
return distributionTime_;
}
public static final int TRANSACTIONTIME2_FIELD_NUMBER = 28;
private long transactionTime2_ = 0L;
/**
*
* / time in nano seconds since epoch.
*
*
* sint64 transactionTime2 = 28;
* @return The transactionTime2.
*/
@java.lang.Override
public long getTransactionTime2() {
return transactionTime2_;
}
public static final int ORIGINALTRADEPRICE_FIELD_NUMBER = 29;
private long originalTradePrice_ = 0L;
/**
*
* Original Price
*
*
* sint64 originalTradePrice = 29;
* @return The originalTradePrice.
*/
@java.lang.Override
public long getOriginalTradePrice() {
return originalTradePrice_;
}
public static final int ORIGINALTRADEQUANTITY_FIELD_NUMBER = 30;
private long originalTradeQuantity_ = 0L;
/**
*
* Original Quantity
*
*
* sint64 originalTradeQuantity = 30;
* @return The originalTradeQuantity.
*/
@java.lang.Override
public long getOriginalTradeQuantity() {
return originalTradeQuantity_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (!originatorId_.isEmpty()) {
output.writeBytes(8, originatorId_);
}
if (transactionTime_ != 0L) {
output.writeSInt64(9, transactionTime_);
}
if (price_ != 0L) {
output.writeSInt64(10, price_);
}
if (quantity_ != 0L) {
output.writeSInt64(11, quantity_);
}
if (!tradeId_.isEmpty()) {
output.writeBytes(12, tradeId_);
}
if (side_ != org.openfeed.BookSide.UNKNOWN_BOOK_SIDE.getNumber()) {
output.writeEnum(13, side_);
}
if (tradeDate_ != 0) {
output.writeSInt32(14, tradeDate_);
}
if (!buyerId_.isEmpty()) {
output.writeBytes(15, buyerId_);
}
if (!sellerId_.isEmpty()) {
output.writeBytes(16, sellerId_);
}
if (openingTrade_ != false) {
output.writeBool(17, openingTrade_);
}
if (systemPriced_ != false) {
output.writeBool(18, systemPriced_);
}
if (marketOnClose_ != false) {
output.writeBool(19, marketOnClose_);
}
if (oddLot_ != false) {
output.writeBool(20, oddLot_);
}
if (settlementTerms_ != org.openfeed.SettlementTerms.UNKNOWN_SETTLEMENT_TERMS.getNumber()) {
output.writeEnum(21, settlementTerms_);
}
if (crossType_ != org.openfeed.CrossType.UNKNOWN_CROSS_TYPE.getNumber()) {
output.writeEnum(22, crossType_);
}
if (byPass_ != false) {
output.writeBool(23, byPass_);
}
if (!originalTradeId_.isEmpty()) {
output.writeBytes(24, originalTradeId_);
}
if (!saleCondition_.isEmpty()) {
output.writeBytes(25, saleCondition_);
}
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(currency_)) {
com.google.protobuf.GeneratedMessage.writeString(output, 26, currency_);
}
if (distributionTime_ != 0L) {
output.writeSInt64(27, distributionTime_);
}
if (transactionTime2_ != 0L) {
output.writeSInt64(28, transactionTime2_);
}
if (originalTradePrice_ != 0L) {
output.writeSInt64(29, originalTradePrice_);
}
if (originalTradeQuantity_ != 0L) {
output.writeSInt64(30, originalTradeQuantity_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (!originatorId_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(8, originatorId_);
}
if (transactionTime_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeSInt64Size(9, transactionTime_);
}
if (price_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeSInt64Size(10, price_);
}
if (quantity_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeSInt64Size(11, quantity_);
}
if (!tradeId_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(12, tradeId_);
}
if (side_ != org.openfeed.BookSide.UNKNOWN_BOOK_SIDE.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(13, side_);
}
if (tradeDate_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeSInt32Size(14, tradeDate_);
}
if (!buyerId_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(15, buyerId_);
}
if (!sellerId_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(16, sellerId_);
}
if (openingTrade_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(17, openingTrade_);
}
if (systemPriced_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(18, systemPriced_);
}
if (marketOnClose_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(19, marketOnClose_);
}
if (oddLot_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(20, oddLot_);
}
if (settlementTerms_ != org.openfeed.SettlementTerms.UNKNOWN_SETTLEMENT_TERMS.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(21, settlementTerms_);
}
if (crossType_ != org.openfeed.CrossType.UNKNOWN_CROSS_TYPE.getNumber()) {
size += com.google.protobuf.CodedOutputStream
.computeEnumSize(22, crossType_);
}
if (byPass_ != false) {
size += com.google.protobuf.CodedOutputStream
.computeBoolSize(23, byPass_);
}
if (!originalTradeId_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(24, originalTradeId_);
}
if (!saleCondition_.isEmpty()) {
size += com.google.protobuf.CodedOutputStream
.computeBytesSize(25, saleCondition_);
}
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(currency_)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(26, currency_);
}
if (distributionTime_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeSInt64Size(27, distributionTime_);
}
if (transactionTime2_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeSInt64Size(28, transactionTime2_);
}
if (originalTradePrice_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeSInt64Size(29, originalTradePrice_);
}
if (originalTradeQuantity_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeSInt64Size(30, originalTradeQuantity_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.openfeed.TradeCorrection)) {
return super.equals(obj);
}
org.openfeed.TradeCorrection other = (org.openfeed.TradeCorrection) obj;
if (!getOriginatorId()
.equals(other.getOriginatorId())) return false;
if (getTransactionTime()
!= other.getTransactionTime()) return false;
if (getPrice()
!= other.getPrice()) return false;
if (getQuantity()
!= other.getQuantity()) return false;
if (!getTradeId()
.equals(other.getTradeId())) return false;
if (side_ != other.side_) return false;
if (getTradeDate()
!= other.getTradeDate()) return false;
if (!getBuyerId()
.equals(other.getBuyerId())) return false;
if (!getSellerId()
.equals(other.getSellerId())) return false;
if (getOpeningTrade()
!= other.getOpeningTrade()) return false;
if (getSystemPriced()
!= other.getSystemPriced()) return false;
if (getMarketOnClose()
!= other.getMarketOnClose()) return false;
if (getOddLot()
!= other.getOddLot()) return false;
if (settlementTerms_ != other.settlementTerms_) return false;
if (crossType_ != other.crossType_) return false;
if (getByPass()
!= other.getByPass()) return false;
if (!getOriginalTradeId()
.equals(other.getOriginalTradeId())) return false;
if (!getSaleCondition()
.equals(other.getSaleCondition())) return false;
if (!getCurrency()
.equals(other.getCurrency())) return false;
if (getDistributionTime()
!= other.getDistributionTime()) return false;
if (getTransactionTime2()
!= other.getTransactionTime2()) return false;
if (getOriginalTradePrice()
!= other.getOriginalTradePrice()) return false;
if (getOriginalTradeQuantity()
!= other.getOriginalTradeQuantity()) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + ORIGINATORID_FIELD_NUMBER;
hash = (53 * hash) + getOriginatorId().hashCode();
hash = (37 * hash) + TRANSACTIONTIME_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getTransactionTime());
hash = (37 * hash) + PRICE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getPrice());
hash = (37 * hash) + QUANTITY_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getQuantity());
hash = (37 * hash) + TRADEID_FIELD_NUMBER;
hash = (53 * hash) + getTradeId().hashCode();
hash = (37 * hash) + SIDE_FIELD_NUMBER;
hash = (53 * hash) + side_;
hash = (37 * hash) + TRADEDATE_FIELD_NUMBER;
hash = (53 * hash) + getTradeDate();
hash = (37 * hash) + BUYERID_FIELD_NUMBER;
hash = (53 * hash) + getBuyerId().hashCode();
hash = (37 * hash) + SELLERID_FIELD_NUMBER;
hash = (53 * hash) + getSellerId().hashCode();
hash = (37 * hash) + OPENINGTRADE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getOpeningTrade());
hash = (37 * hash) + SYSTEMPRICED_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getSystemPriced());
hash = (37 * hash) + MARKETONCLOSE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getMarketOnClose());
hash = (37 * hash) + ODDLOT_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getOddLot());
hash = (37 * hash) + SETTLEMENTTERMS_FIELD_NUMBER;
hash = (53 * hash) + settlementTerms_;
hash = (37 * hash) + CROSSTYPE_FIELD_NUMBER;
hash = (53 * hash) + crossType_;
hash = (37 * hash) + BYPASS_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashBoolean(
getByPass());
hash = (37 * hash) + ORIGINALTRADEID_FIELD_NUMBER;
hash = (53 * hash) + getOriginalTradeId().hashCode();
hash = (37 * hash) + SALECONDITION_FIELD_NUMBER;
hash = (53 * hash) + getSaleCondition().hashCode();
hash = (37 * hash) + CURRENCY_FIELD_NUMBER;
hash = (53 * hash) + getCurrency().hashCode();
hash = (37 * hash) + DISTRIBUTIONTIME_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getDistributionTime());
hash = (37 * hash) + TRANSACTIONTIME2_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getTransactionTime2());
hash = (37 * hash) + ORIGINALTRADEPRICE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getOriginalTradePrice());
hash = (37 * hash) + ORIGINALTRADEQUANTITY_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getOriginalTradeQuantity());
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.openfeed.TradeCorrection parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.openfeed.TradeCorrection parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.openfeed.TradeCorrection parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.openfeed.TradeCorrection parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.openfeed.TradeCorrection parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.openfeed.TradeCorrection parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.openfeed.TradeCorrection parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static org.openfeed.TradeCorrection parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.openfeed.TradeCorrection parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input);
}
public static org.openfeed.TradeCorrection parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.openfeed.TradeCorrection parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static org.openfeed.TradeCorrection parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.openfeed.TradeCorrection prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* / Trade Correction
*
*
* Protobuf type {@code org.openfeed.TradeCorrection}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:org.openfeed.TradeCorrection)
org.openfeed.TradeCorrectionOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.openfeed.Openfeed.internal_static_org_openfeed_TradeCorrection_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.openfeed.Openfeed.internal_static_org_openfeed_TradeCorrection_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.openfeed.TradeCorrection.class, org.openfeed.TradeCorrection.Builder.class);
}
// Construct using org.openfeed.TradeCorrection.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
originatorId_ = com.google.protobuf.ByteString.EMPTY;
transactionTime_ = 0L;
price_ = 0L;
quantity_ = 0L;
tradeId_ = com.google.protobuf.ByteString.EMPTY;
side_ = 0;
tradeDate_ = 0;
buyerId_ = com.google.protobuf.ByteString.EMPTY;
sellerId_ = com.google.protobuf.ByteString.EMPTY;
openingTrade_ = false;
systemPriced_ = false;
marketOnClose_ = false;
oddLot_ = false;
settlementTerms_ = 0;
crossType_ = 0;
byPass_ = false;
originalTradeId_ = com.google.protobuf.ByteString.EMPTY;
saleCondition_ = com.google.protobuf.ByteString.EMPTY;
currency_ = "";
distributionTime_ = 0L;
transactionTime2_ = 0L;
originalTradePrice_ = 0L;
originalTradeQuantity_ = 0L;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.openfeed.Openfeed.internal_static_org_openfeed_TradeCorrection_descriptor;
}
@java.lang.Override
public org.openfeed.TradeCorrection getDefaultInstanceForType() {
return org.openfeed.TradeCorrection.getDefaultInstance();
}
@java.lang.Override
public org.openfeed.TradeCorrection build() {
org.openfeed.TradeCorrection result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.openfeed.TradeCorrection buildPartial() {
org.openfeed.TradeCorrection result = new org.openfeed.TradeCorrection(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(org.openfeed.TradeCorrection result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.originatorId_ = originatorId_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.transactionTime_ = transactionTime_;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.price_ = price_;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.quantity_ = quantity_;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.tradeId_ = tradeId_;
}
if (((from_bitField0_ & 0x00000020) != 0)) {
result.side_ = side_;
}
if (((from_bitField0_ & 0x00000040) != 0)) {
result.tradeDate_ = tradeDate_;
}
if (((from_bitField0_ & 0x00000080) != 0)) {
result.buyerId_ = buyerId_;
}
if (((from_bitField0_ & 0x00000100) != 0)) {
result.sellerId_ = sellerId_;
}
if (((from_bitField0_ & 0x00000200) != 0)) {
result.openingTrade_ = openingTrade_;
}
if (((from_bitField0_ & 0x00000400) != 0)) {
result.systemPriced_ = systemPriced_;
}
if (((from_bitField0_ & 0x00000800) != 0)) {
result.marketOnClose_ = marketOnClose_;
}
if (((from_bitField0_ & 0x00001000) != 0)) {
result.oddLot_ = oddLot_;
}
if (((from_bitField0_ & 0x00002000) != 0)) {
result.settlementTerms_ = settlementTerms_;
}
if (((from_bitField0_ & 0x00004000) != 0)) {
result.crossType_ = crossType_;
}
if (((from_bitField0_ & 0x00008000) != 0)) {
result.byPass_ = byPass_;
}
if (((from_bitField0_ & 0x00010000) != 0)) {
result.originalTradeId_ = originalTradeId_;
}
if (((from_bitField0_ & 0x00020000) != 0)) {
result.saleCondition_ = saleCondition_;
}
if (((from_bitField0_ & 0x00040000) != 0)) {
result.currency_ = currency_;
}
if (((from_bitField0_ & 0x00080000) != 0)) {
result.distributionTime_ = distributionTime_;
}
if (((from_bitField0_ & 0x00100000) != 0)) {
result.transactionTime2_ = transactionTime2_;
}
if (((from_bitField0_ & 0x00200000) != 0)) {
result.originalTradePrice_ = originalTradePrice_;
}
if (((from_bitField0_ & 0x00400000) != 0)) {
result.originalTradeQuantity_ = originalTradeQuantity_;
}
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.openfeed.TradeCorrection) {
return mergeFrom((org.openfeed.TradeCorrection)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.openfeed.TradeCorrection other) {
if (other == org.openfeed.TradeCorrection.getDefaultInstance()) return this;
if (other.getOriginatorId() != com.google.protobuf.ByteString.EMPTY) {
setOriginatorId(other.getOriginatorId());
}
if (other.getTransactionTime() != 0L) {
setTransactionTime(other.getTransactionTime());
}
if (other.getPrice() != 0L) {
setPrice(other.getPrice());
}
if (other.getQuantity() != 0L) {
setQuantity(other.getQuantity());
}
if (other.getTradeId() != com.google.protobuf.ByteString.EMPTY) {
setTradeId(other.getTradeId());
}
if (other.side_ != 0) {
setSideValue(other.getSideValue());
}
if (other.getTradeDate() != 0) {
setTradeDate(other.getTradeDate());
}
if (other.getBuyerId() != com.google.protobuf.ByteString.EMPTY) {
setBuyerId(other.getBuyerId());
}
if (other.getSellerId() != com.google.protobuf.ByteString.EMPTY) {
setSellerId(other.getSellerId());
}
if (other.getOpeningTrade() != false) {
setOpeningTrade(other.getOpeningTrade());
}
if (other.getSystemPriced() != false) {
setSystemPriced(other.getSystemPriced());
}
if (other.getMarketOnClose() != false) {
setMarketOnClose(other.getMarketOnClose());
}
if (other.getOddLot() != false) {
setOddLot(other.getOddLot());
}
if (other.settlementTerms_ != 0) {
setSettlementTermsValue(other.getSettlementTermsValue());
}
if (other.crossType_ != 0) {
setCrossTypeValue(other.getCrossTypeValue());
}
if (other.getByPass() != false) {
setByPass(other.getByPass());
}
if (other.getOriginalTradeId() != com.google.protobuf.ByteString.EMPTY) {
setOriginalTradeId(other.getOriginalTradeId());
}
if (other.getSaleCondition() != com.google.protobuf.ByteString.EMPTY) {
setSaleCondition(other.getSaleCondition());
}
if (!other.getCurrency().isEmpty()) {
currency_ = other.currency_;
bitField0_ |= 0x00040000;
onChanged();
}
if (other.getDistributionTime() != 0L) {
setDistributionTime(other.getDistributionTime());
}
if (other.getTransactionTime2() != 0L) {
setTransactionTime2(other.getTransactionTime2());
}
if (other.getOriginalTradePrice() != 0L) {
setOriginalTradePrice(other.getOriginalTradePrice());
}
if (other.getOriginalTradeQuantity() != 0L) {
setOriginalTradeQuantity(other.getOriginalTradeQuantity());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 66: {
originatorId_ = input.readBytes();
bitField0_ |= 0x00000001;
break;
} // case 66
case 72: {
transactionTime_ = input.readSInt64();
bitField0_ |= 0x00000002;
break;
} // case 72
case 80: {
price_ = input.readSInt64();
bitField0_ |= 0x00000004;
break;
} // case 80
case 88: {
quantity_ = input.readSInt64();
bitField0_ |= 0x00000008;
break;
} // case 88
case 98: {
tradeId_ = input.readBytes();
bitField0_ |= 0x00000010;
break;
} // case 98
case 104: {
side_ = input.readEnum();
bitField0_ |= 0x00000020;
break;
} // case 104
case 112: {
tradeDate_ = input.readSInt32();
bitField0_ |= 0x00000040;
break;
} // case 112
case 122: {
buyerId_ = input.readBytes();
bitField0_ |= 0x00000080;
break;
} // case 122
case 130: {
sellerId_ = input.readBytes();
bitField0_ |= 0x00000100;
break;
} // case 130
case 136: {
openingTrade_ = input.readBool();
bitField0_ |= 0x00000200;
break;
} // case 136
case 144: {
systemPriced_ = input.readBool();
bitField0_ |= 0x00000400;
break;
} // case 144
case 152: {
marketOnClose_ = input.readBool();
bitField0_ |= 0x00000800;
break;
} // case 152
case 160: {
oddLot_ = input.readBool();
bitField0_ |= 0x00001000;
break;
} // case 160
case 168: {
settlementTerms_ = input.readEnum();
bitField0_ |= 0x00002000;
break;
} // case 168
case 176: {
crossType_ = input.readEnum();
bitField0_ |= 0x00004000;
break;
} // case 176
case 184: {
byPass_ = input.readBool();
bitField0_ |= 0x00008000;
break;
} // case 184
case 194: {
originalTradeId_ = input.readBytes();
bitField0_ |= 0x00010000;
break;
} // case 194
case 202: {
saleCondition_ = input.readBytes();
bitField0_ |= 0x00020000;
break;
} // case 202
case 210: {
currency_ = input.readStringRequireUtf8();
bitField0_ |= 0x00040000;
break;
} // case 210
case 216: {
distributionTime_ = input.readSInt64();
bitField0_ |= 0x00080000;
break;
} // case 216
case 224: {
transactionTime2_ = input.readSInt64();
bitField0_ |= 0x00100000;
break;
} // case 224
case 232: {
originalTradePrice_ = input.readSInt64();
bitField0_ |= 0x00200000;
break;
} // case 232
case 240: {
originalTradeQuantity_ = input.readSInt64();
bitField0_ |= 0x00400000;
break;
} // case 240
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private com.google.protobuf.ByteString originatorId_ = com.google.protobuf.ByteString.EMPTY;
/**
*
* Market participant/originator
*
*
* bytes originatorId = 8;
* @return The originatorId.
*/
@java.lang.Override
public com.google.protobuf.ByteString getOriginatorId() {
return originatorId_;
}
/**
*
* Market participant/originator
*
*
* bytes originatorId = 8;
* @param value The originatorId to set.
* @return This builder for chaining.
*/
public Builder setOriginatorId(com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
originatorId_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
*
* Market participant/originator
*
*
* bytes originatorId = 8;
* @return This builder for chaining.
*/
public Builder clearOriginatorId() {
bitField0_ = (bitField0_ & ~0x00000001);
originatorId_ = getDefaultInstance().getOriginatorId();
onChanged();
return this;
}
private long transactionTime_ ;
/**
* sint64 transactionTime = 9;
* @return The transactionTime.
*/
@java.lang.Override
public long getTransactionTime() {
return transactionTime_;
}
/**
* sint64 transactionTime = 9;
* @param value The transactionTime to set.
* @return This builder for chaining.
*/
public Builder setTransactionTime(long value) {
transactionTime_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* sint64 transactionTime = 9;
* @return This builder for chaining.
*/
public Builder clearTransactionTime() {
bitField0_ = (bitField0_ & ~0x00000002);
transactionTime_ = 0L;
onChanged();
return this;
}
private long price_ ;
/**
*
* Corrected Price
*
*
* sint64 price = 10;
* @return The price.
*/
@java.lang.Override
public long getPrice() {
return price_;
}
/**
*
* Corrected Price
*
*
* sint64 price = 10;
* @param value The price to set.
* @return This builder for chaining.
*/
public Builder setPrice(long value) {
price_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
* Corrected Price
*
*
* sint64 price = 10;
* @return This builder for chaining.
*/
public Builder clearPrice() {
bitField0_ = (bitField0_ & ~0x00000004);
price_ = 0L;
onChanged();
return this;
}
private long quantity_ ;
/**
*
* Corrected Quantity
*
*
* sint64 quantity = 11;
* @return The quantity.
*/
@java.lang.Override
public long getQuantity() {
return quantity_;
}
/**
*
* Corrected Quantity
*
*
* sint64 quantity = 11;
* @param value The quantity to set.
* @return This builder for chaining.
*/
public Builder setQuantity(long value) {
quantity_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
*
* Corrected Quantity
*
*
* sint64 quantity = 11;
* @return This builder for chaining.
*/
public Builder clearQuantity() {
bitField0_ = (bitField0_ & ~0x00000008);
quantity_ = 0L;
onChanged();
return this;
}
private com.google.protobuf.ByteString tradeId_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes tradeId = 12;
* @return The tradeId.
*/
@java.lang.Override
public com.google.protobuf.ByteString getTradeId() {
return tradeId_;
}
/**
* bytes tradeId = 12;
* @param value The tradeId to set.
* @return This builder for chaining.
*/
public Builder setTradeId(com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
tradeId_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
* bytes tradeId = 12;
* @return This builder for chaining.
*/
public Builder clearTradeId() {
bitField0_ = (bitField0_ & ~0x00000010);
tradeId_ = getDefaultInstance().getTradeId();
onChanged();
return this;
}
private int side_ = 0;
/**
* .org.openfeed.BookSide side = 13;
* @return The enum numeric value on the wire for side.
*/
@java.lang.Override public int getSideValue() {
return side_;
}
/**
* .org.openfeed.BookSide side = 13;
* @param value The enum numeric value on the wire for side to set.
* @return This builder for chaining.
*/
public Builder setSideValue(int value) {
side_ = value;
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
* .org.openfeed.BookSide side = 13;
* @return The side.
*/
@java.lang.Override
public org.openfeed.BookSide getSide() {
org.openfeed.BookSide result = org.openfeed.BookSide.forNumber(side_);
return result == null ? org.openfeed.BookSide.UNRECOGNIZED : result;
}
/**
* .org.openfeed.BookSide side = 13;
* @param value The side to set.
* @return This builder for chaining.
*/
public Builder setSide(org.openfeed.BookSide value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00000020;
side_ = value.getNumber();
onChanged();
return this;
}
/**
* .org.openfeed.BookSide side = 13;
* @return This builder for chaining.
*/
public Builder clearSide() {
bitField0_ = (bitField0_ & ~0x00000020);
side_ = 0;
onChanged();
return this;
}
private int tradeDate_ ;
/**
*
* Date only, format 2012-07-04 -> 20120704
*
*
* sint32 tradeDate = 14;
* @return The tradeDate.
*/
@java.lang.Override
public int getTradeDate() {
return tradeDate_;
}
/**
*
* Date only, format 2012-07-04 -> 20120704
*
*
* sint32 tradeDate = 14;
* @param value The tradeDate to set.
* @return This builder for chaining.
*/
public Builder setTradeDate(int value) {
tradeDate_ = value;
bitField0_ |= 0x00000040;
onChanged();
return this;
}
/**
*
* Date only, format 2012-07-04 -> 20120704
*
*
* sint32 tradeDate = 14;
* @return This builder for chaining.
*/
public Builder clearTradeDate() {
bitField0_ = (bitField0_ & ~0x00000040);
tradeDate_ = 0;
onChanged();
return this;
}
private com.google.protobuf.ByteString buyerId_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes buyerId = 15;
* @return The buyerId.
*/
@java.lang.Override
public com.google.protobuf.ByteString getBuyerId() {
return buyerId_;
}
/**
* bytes buyerId = 15;
* @param value The buyerId to set.
* @return This builder for chaining.
*/
public Builder setBuyerId(com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
buyerId_ = value;
bitField0_ |= 0x00000080;
onChanged();
return this;
}
/**
* bytes buyerId = 15;
* @return This builder for chaining.
*/
public Builder clearBuyerId() {
bitField0_ = (bitField0_ & ~0x00000080);
buyerId_ = getDefaultInstance().getBuyerId();
onChanged();
return this;
}
private com.google.protobuf.ByteString sellerId_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes sellerId = 16;
* @return The sellerId.
*/
@java.lang.Override
public com.google.protobuf.ByteString getSellerId() {
return sellerId_;
}
/**
* bytes sellerId = 16;
* @param value The sellerId to set.
* @return This builder for chaining.
*/
public Builder setSellerId(com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
sellerId_ = value;
bitField0_ |= 0x00000100;
onChanged();
return this;
}
/**
* bytes sellerId = 16;
* @return This builder for chaining.
*/
public Builder clearSellerId() {
bitField0_ = (bitField0_ & ~0x00000100);
sellerId_ = getDefaultInstance().getSellerId();
onChanged();
return this;
}
private boolean openingTrade_ ;
/**
* bool openingTrade = 17;
* @return The openingTrade.
*/
@java.lang.Override
public boolean getOpeningTrade() {
return openingTrade_;
}
/**
* bool openingTrade = 17;
* @param value The openingTrade to set.
* @return This builder for chaining.
*/
public Builder setOpeningTrade(boolean value) {
openingTrade_ = value;
bitField0_ |= 0x00000200;
onChanged();
return this;
}
/**
* bool openingTrade = 17;
* @return This builder for chaining.
*/
public Builder clearOpeningTrade() {
bitField0_ = (bitField0_ & ~0x00000200);
openingTrade_ = false;
onChanged();
return this;
}
private boolean systemPriced_ ;
/**
* bool systemPriced = 18;
* @return The systemPriced.
*/
@java.lang.Override
public boolean getSystemPriced() {
return systemPriced_;
}
/**
* bool systemPriced = 18;
* @param value The systemPriced to set.
* @return This builder for chaining.
*/
public Builder setSystemPriced(boolean value) {
systemPriced_ = value;
bitField0_ |= 0x00000400;
onChanged();
return this;
}
/**
* bool systemPriced = 18;
* @return This builder for chaining.
*/
public Builder clearSystemPriced() {
bitField0_ = (bitField0_ & ~0x00000400);
systemPriced_ = false;
onChanged();
return this;
}
private boolean marketOnClose_ ;
/**
* bool marketOnClose = 19;
* @return The marketOnClose.
*/
@java.lang.Override
public boolean getMarketOnClose() {
return marketOnClose_;
}
/**
* bool marketOnClose = 19;
* @param value The marketOnClose to set.
* @return This builder for chaining.
*/
public Builder setMarketOnClose(boolean value) {
marketOnClose_ = value;
bitField0_ |= 0x00000800;
onChanged();
return this;
}
/**
* bool marketOnClose = 19;
* @return This builder for chaining.
*/
public Builder clearMarketOnClose() {
bitField0_ = (bitField0_ & ~0x00000800);
marketOnClose_ = false;
onChanged();
return this;
}
private boolean oddLot_ ;
/**
* bool oddLot = 20;
* @return The oddLot.
*/
@java.lang.Override
public boolean getOddLot() {
return oddLot_;
}
/**
* bool oddLot = 20;
* @param value The oddLot to set.
* @return This builder for chaining.
*/
public Builder setOddLot(boolean value) {
oddLot_ = value;
bitField0_ |= 0x00001000;
onChanged();
return this;
}
/**
* bool oddLot = 20;
* @return This builder for chaining.
*/
public Builder clearOddLot() {
bitField0_ = (bitField0_ & ~0x00001000);
oddLot_ = false;
onChanged();
return this;
}
private int settlementTerms_ = 0;
/**
* .org.openfeed.SettlementTerms settlementTerms = 21;
* @return The enum numeric value on the wire for settlementTerms.
*/
@java.lang.Override public int getSettlementTermsValue() {
return settlementTerms_;
}
/**
* .org.openfeed.SettlementTerms settlementTerms = 21;
* @param value The enum numeric value on the wire for settlementTerms to set.
* @return This builder for chaining.
*/
public Builder setSettlementTermsValue(int value) {
settlementTerms_ = value;
bitField0_ |= 0x00002000;
onChanged();
return this;
}
/**
* .org.openfeed.SettlementTerms settlementTerms = 21;
* @return The settlementTerms.
*/
@java.lang.Override
public org.openfeed.SettlementTerms getSettlementTerms() {
org.openfeed.SettlementTerms result = org.openfeed.SettlementTerms.forNumber(settlementTerms_);
return result == null ? org.openfeed.SettlementTerms.UNRECOGNIZED : result;
}
/**
* .org.openfeed.SettlementTerms settlementTerms = 21;
* @param value The settlementTerms to set.
* @return This builder for chaining.
*/
public Builder setSettlementTerms(org.openfeed.SettlementTerms value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00002000;
settlementTerms_ = value.getNumber();
onChanged();
return this;
}
/**
* .org.openfeed.SettlementTerms settlementTerms = 21;
* @return This builder for chaining.
*/
public Builder clearSettlementTerms() {
bitField0_ = (bitField0_ & ~0x00002000);
settlementTerms_ = 0;
onChanged();
return this;
}
private int crossType_ = 0;
/**
* .org.openfeed.CrossType crossType = 22;
* @return The enum numeric value on the wire for crossType.
*/
@java.lang.Override public int getCrossTypeValue() {
return crossType_;
}
/**
* .org.openfeed.CrossType crossType = 22;
* @param value The enum numeric value on the wire for crossType to set.
* @return This builder for chaining.
*/
public Builder setCrossTypeValue(int value) {
crossType_ = value;
bitField0_ |= 0x00004000;
onChanged();
return this;
}
/**
* .org.openfeed.CrossType crossType = 22;
* @return The crossType.
*/
@java.lang.Override
public org.openfeed.CrossType getCrossType() {
org.openfeed.CrossType result = org.openfeed.CrossType.forNumber(crossType_);
return result == null ? org.openfeed.CrossType.UNRECOGNIZED : result;
}
/**
* .org.openfeed.CrossType crossType = 22;
* @param value The crossType to set.
* @return This builder for chaining.
*/
public Builder setCrossType(org.openfeed.CrossType value) {
if (value == null) {
throw new NullPointerException();
}
bitField0_ |= 0x00004000;
crossType_ = value.getNumber();
onChanged();
return this;
}
/**
* .org.openfeed.CrossType crossType = 22;
* @return This builder for chaining.
*/
public Builder clearCrossType() {
bitField0_ = (bitField0_ & ~0x00004000);
crossType_ = 0;
onChanged();
return this;
}
private boolean byPass_ ;
/**
* bool byPass = 23;
* @return The byPass.
*/
@java.lang.Override
public boolean getByPass() {
return byPass_;
}
/**
* bool byPass = 23;
* @param value The byPass to set.
* @return This builder for chaining.
*/
public Builder setByPass(boolean value) {
byPass_ = value;
bitField0_ |= 0x00008000;
onChanged();
return this;
}
/**
* bool byPass = 23;
* @return This builder for chaining.
*/
public Builder clearByPass() {
bitField0_ = (bitField0_ & ~0x00008000);
byPass_ = false;
onChanged();
return this;
}
private com.google.protobuf.ByteString originalTradeId_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes originalTradeId = 24;
* @return The originalTradeId.
*/
@java.lang.Override
public com.google.protobuf.ByteString getOriginalTradeId() {
return originalTradeId_;
}
/**
* bytes originalTradeId = 24;
* @param value The originalTradeId to set.
* @return This builder for chaining.
*/
public Builder setOriginalTradeId(com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
originalTradeId_ = value;
bitField0_ |= 0x00010000;
onChanged();
return this;
}
/**
* bytes originalTradeId = 24;
* @return This builder for chaining.
*/
public Builder clearOriginalTradeId() {
bitField0_ = (bitField0_ & ~0x00010000);
originalTradeId_ = getDefaultInstance().getOriginalTradeId();
onChanged();
return this;
}
private com.google.protobuf.ByteString saleCondition_ = com.google.protobuf.ByteString.EMPTY;
/**
* bytes saleCondition = 25;
* @return The saleCondition.
*/
@java.lang.Override
public com.google.protobuf.ByteString getSaleCondition() {
return saleCondition_;
}
/**
* bytes saleCondition = 25;
* @param value The saleCondition to set.
* @return This builder for chaining.
*/
public Builder setSaleCondition(com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
saleCondition_ = value;
bitField0_ |= 0x00020000;
onChanged();
return this;
}
/**
* bytes saleCondition = 25;
* @return This builder for chaining.
*/
public Builder clearSaleCondition() {
bitField0_ = (bitField0_ & ~0x00020000);
saleCondition_ = getDefaultInstance().getSaleCondition();
onChanged();
return this;
}
private java.lang.Object currency_ = "";
/**
* string currency = 26;
* @return The currency.
*/
public java.lang.String getCurrency() {
java.lang.Object ref = currency_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
currency_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string currency = 26;
* @return The bytes for currency.
*/
public com.google.protobuf.ByteString
getCurrencyBytes() {
java.lang.Object ref = currency_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
currency_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string currency = 26;
* @param value The currency to set.
* @return This builder for chaining.
*/
public Builder setCurrency(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
currency_ = value;
bitField0_ |= 0x00040000;
onChanged();
return this;
}
/**
* string currency = 26;
* @return This builder for chaining.
*/
public Builder clearCurrency() {
currency_ = getDefaultInstance().getCurrency();
bitField0_ = (bitField0_ & ~0x00040000);
onChanged();
return this;
}
/**
* string currency = 26;
* @param value The bytes for currency to set.
* @return This builder for chaining.
*/
public Builder setCurrencyBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
currency_ = value;
bitField0_ |= 0x00040000;
onChanged();
return this;
}
private long distributionTime_ ;
/**
*
* / Distribution time in nano seconds since epoch.
*
*
* sint64 distributionTime = 27;
* @return The distributionTime.
*/
@java.lang.Override
public long getDistributionTime() {
return distributionTime_;
}
/**
*
* / Distribution time in nano seconds since epoch.
*
*
* sint64 distributionTime = 27;
* @param value The distributionTime to set.
* @return This builder for chaining.
*/
public Builder setDistributionTime(long value) {
distributionTime_ = value;
bitField0_ |= 0x00080000;
onChanged();
return this;
}
/**
*
* / Distribution time in nano seconds since epoch.
*
*
* sint64 distributionTime = 27;
* @return This builder for chaining.
*/
public Builder clearDistributionTime() {
bitField0_ = (bitField0_ & ~0x00080000);
distributionTime_ = 0L;
onChanged();
return this;
}
private long transactionTime2_ ;
/**
*
* / time in nano seconds since epoch.
*
*
* sint64 transactionTime2 = 28;
* @return The transactionTime2.
*/
@java.lang.Override
public long getTransactionTime2() {
return transactionTime2_;
}
/**
*
* / time in nano seconds since epoch.
*
*
* sint64 transactionTime2 = 28;
* @param value The transactionTime2 to set.
* @return This builder for chaining.
*/
public Builder setTransactionTime2(long value) {
transactionTime2_ = value;
bitField0_ |= 0x00100000;
onChanged();
return this;
}
/**
*
* / time in nano seconds since epoch.
*
*
* sint64 transactionTime2 = 28;
* @return This builder for chaining.
*/
public Builder clearTransactionTime2() {
bitField0_ = (bitField0_ & ~0x00100000);
transactionTime2_ = 0L;
onChanged();
return this;
}
private long originalTradePrice_ ;
/**
*
* Original Price
*
*
* sint64 originalTradePrice = 29;
* @return The originalTradePrice.
*/
@java.lang.Override
public long getOriginalTradePrice() {
return originalTradePrice_;
}
/**
*
* Original Price
*
*
* sint64 originalTradePrice = 29;
* @param value The originalTradePrice to set.
* @return This builder for chaining.
*/
public Builder setOriginalTradePrice(long value) {
originalTradePrice_ = value;
bitField0_ |= 0x00200000;
onChanged();
return this;
}
/**
*
* Original Price
*
*
* sint64 originalTradePrice = 29;
* @return This builder for chaining.
*/
public Builder clearOriginalTradePrice() {
bitField0_ = (bitField0_ & ~0x00200000);
originalTradePrice_ = 0L;
onChanged();
return this;
}
private long originalTradeQuantity_ ;
/**
*
* Original Quantity
*
*
* sint64 originalTradeQuantity = 30;
* @return The originalTradeQuantity.
*/
@java.lang.Override
public long getOriginalTradeQuantity() {
return originalTradeQuantity_;
}
/**
*
* Original Quantity
*
*
* sint64 originalTradeQuantity = 30;
* @param value The originalTradeQuantity to set.
* @return This builder for chaining.
*/
public Builder setOriginalTradeQuantity(long value) {
originalTradeQuantity_ = value;
bitField0_ |= 0x00400000;
onChanged();
return this;
}
/**
*
* Original Quantity
*
*
* sint64 originalTradeQuantity = 30;
* @return This builder for chaining.
*/
public Builder clearOriginalTradeQuantity() {
bitField0_ = (bitField0_ & ~0x00400000);
originalTradeQuantity_ = 0L;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:org.openfeed.TradeCorrection)
}
// @@protoc_insertion_point(class_scope:org.openfeed.TradeCorrection)
private static final org.openfeed.TradeCorrection DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.openfeed.TradeCorrection();
}
public static org.openfeed.TradeCorrection getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public TradeCorrection parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.openfeed.TradeCorrection getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy