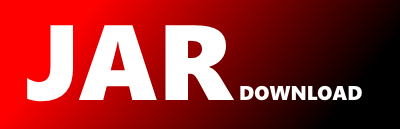
org.openfeed.VolumeAtPrice Maven / Gradle / Ivy
The newest version!
// Generated by the protocol buffer compiler. DO NOT EDIT!
// NO CHECKED-IN PROTOBUF GENCODE
// source: openfeed.proto
// Protobuf Java Version: 4.28.3
package org.openfeed;
/**
*
* / The VolumeAtPrice class encapsulates all of the trades throughout the day,
* / and organizes a table of volume at prices.
*
*
* Protobuf type {@code org.openfeed.VolumeAtPrice}
*/
public final class VolumeAtPrice extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:org.openfeed.VolumeAtPrice)
VolumeAtPriceOrBuilder {
private static final long serialVersionUID = 0L;
static {
com.google.protobuf.RuntimeVersion.validateProtobufGencodeVersion(
com.google.protobuf.RuntimeVersion.RuntimeDomain.PUBLIC,
/* major= */ 4,
/* minor= */ 28,
/* patch= */ 3,
/* suffix= */ "",
VolumeAtPrice.class.getName());
}
// Use VolumeAtPrice.newBuilder() to construct.
private VolumeAtPrice(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private VolumeAtPrice() {
symbol_ = "";
priceVolumes_ = java.util.Collections.emptyList();
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.openfeed.Openfeed.internal_static_org_openfeed_VolumeAtPrice_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.openfeed.Openfeed.internal_static_org_openfeed_VolumeAtPrice_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.openfeed.VolumeAtPrice.class, org.openfeed.VolumeAtPrice.Builder.class);
}
public interface PriceLevelVolumeOrBuilder extends
// @@protoc_insertion_point(interface_extends:org.openfeed.VolumeAtPrice.PriceLevelVolume)
com.google.protobuf.MessageOrBuilder {
/**
* sint64 price = 1;
* @return The price.
*/
long getPrice();
/**
* sint64 volume = 2;
* @return The volume.
*/
long getVolume();
}
/**
* Protobuf type {@code org.openfeed.VolumeAtPrice.PriceLevelVolume}
*/
public static final class PriceLevelVolume extends
com.google.protobuf.GeneratedMessage implements
// @@protoc_insertion_point(message_implements:org.openfeed.VolumeAtPrice.PriceLevelVolume)
PriceLevelVolumeOrBuilder {
private static final long serialVersionUID = 0L;
static {
com.google.protobuf.RuntimeVersion.validateProtobufGencodeVersion(
com.google.protobuf.RuntimeVersion.RuntimeDomain.PUBLIC,
/* major= */ 4,
/* minor= */ 28,
/* patch= */ 3,
/* suffix= */ "",
PriceLevelVolume.class.getName());
}
// Use PriceLevelVolume.newBuilder() to construct.
private PriceLevelVolume(com.google.protobuf.GeneratedMessage.Builder> builder) {
super(builder);
}
private PriceLevelVolume() {
}
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.openfeed.Openfeed.internal_static_org_openfeed_VolumeAtPrice_PriceLevelVolume_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.openfeed.Openfeed.internal_static_org_openfeed_VolumeAtPrice_PriceLevelVolume_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.openfeed.VolumeAtPrice.PriceLevelVolume.class, org.openfeed.VolumeAtPrice.PriceLevelVolume.Builder.class);
}
public static final int PRICE_FIELD_NUMBER = 1;
private long price_ = 0L;
/**
* sint64 price = 1;
* @return The price.
*/
@java.lang.Override
public long getPrice() {
return price_;
}
public static final int VOLUME_FIELD_NUMBER = 2;
private long volume_ = 0L;
/**
* sint64 volume = 2;
* @return The volume.
*/
@java.lang.Override
public long getVolume() {
return volume_;
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (price_ != 0L) {
output.writeSInt64(1, price_);
}
if (volume_ != 0L) {
output.writeSInt64(2, volume_);
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (price_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeSInt64Size(1, price_);
}
if (volume_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeSInt64Size(2, volume_);
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.openfeed.VolumeAtPrice.PriceLevelVolume)) {
return super.equals(obj);
}
org.openfeed.VolumeAtPrice.PriceLevelVolume other = (org.openfeed.VolumeAtPrice.PriceLevelVolume) obj;
if (getPrice()
!= other.getPrice()) return false;
if (getVolume()
!= other.getVolume()) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + PRICE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getPrice());
hash = (37 * hash) + VOLUME_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getVolume());
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.openfeed.VolumeAtPrice.PriceLevelVolume parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.openfeed.VolumeAtPrice.PriceLevelVolume parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.openfeed.VolumeAtPrice.PriceLevelVolume parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.openfeed.VolumeAtPrice.PriceLevelVolume parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.openfeed.VolumeAtPrice.PriceLevelVolume parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.openfeed.VolumeAtPrice.PriceLevelVolume parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.openfeed.VolumeAtPrice.PriceLevelVolume parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static org.openfeed.VolumeAtPrice.PriceLevelVolume parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.openfeed.VolumeAtPrice.PriceLevelVolume parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input);
}
public static org.openfeed.VolumeAtPrice.PriceLevelVolume parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.openfeed.VolumeAtPrice.PriceLevelVolume parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static org.openfeed.VolumeAtPrice.PriceLevelVolume parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.openfeed.VolumeAtPrice.PriceLevelVolume prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
* Protobuf type {@code org.openfeed.VolumeAtPrice.PriceLevelVolume}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:org.openfeed.VolumeAtPrice.PriceLevelVolume)
org.openfeed.VolumeAtPrice.PriceLevelVolumeOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.openfeed.Openfeed.internal_static_org_openfeed_VolumeAtPrice_PriceLevelVolume_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.openfeed.Openfeed.internal_static_org_openfeed_VolumeAtPrice_PriceLevelVolume_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.openfeed.VolumeAtPrice.PriceLevelVolume.class, org.openfeed.VolumeAtPrice.PriceLevelVolume.Builder.class);
}
// Construct using org.openfeed.VolumeAtPrice.PriceLevelVolume.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
price_ = 0L;
volume_ = 0L;
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.openfeed.Openfeed.internal_static_org_openfeed_VolumeAtPrice_PriceLevelVolume_descriptor;
}
@java.lang.Override
public org.openfeed.VolumeAtPrice.PriceLevelVolume getDefaultInstanceForType() {
return org.openfeed.VolumeAtPrice.PriceLevelVolume.getDefaultInstance();
}
@java.lang.Override
public org.openfeed.VolumeAtPrice.PriceLevelVolume build() {
org.openfeed.VolumeAtPrice.PriceLevelVolume result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.openfeed.VolumeAtPrice.PriceLevelVolume buildPartial() {
org.openfeed.VolumeAtPrice.PriceLevelVolume result = new org.openfeed.VolumeAtPrice.PriceLevelVolume(this);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartial0(org.openfeed.VolumeAtPrice.PriceLevelVolume result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.price_ = price_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.volume_ = volume_;
}
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.openfeed.VolumeAtPrice.PriceLevelVolume) {
return mergeFrom((org.openfeed.VolumeAtPrice.PriceLevelVolume)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.openfeed.VolumeAtPrice.PriceLevelVolume other) {
if (other == org.openfeed.VolumeAtPrice.PriceLevelVolume.getDefaultInstance()) return this;
if (other.getPrice() != 0L) {
setPrice(other.getPrice());
}
if (other.getVolume() != 0L) {
setVolume(other.getVolume());
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
price_ = input.readSInt64();
bitField0_ |= 0x00000001;
break;
} // case 8
case 16: {
volume_ = input.readSInt64();
bitField0_ |= 0x00000002;
break;
} // case 16
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private long price_ ;
/**
* sint64 price = 1;
* @return The price.
*/
@java.lang.Override
public long getPrice() {
return price_;
}
/**
* sint64 price = 1;
* @param value The price to set.
* @return This builder for chaining.
*/
public Builder setPrice(long value) {
price_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* sint64 price = 1;
* @return This builder for chaining.
*/
public Builder clearPrice() {
bitField0_ = (bitField0_ & ~0x00000001);
price_ = 0L;
onChanged();
return this;
}
private long volume_ ;
/**
* sint64 volume = 2;
* @return The volume.
*/
@java.lang.Override
public long getVolume() {
return volume_;
}
/**
* sint64 volume = 2;
* @param value The volume to set.
* @return This builder for chaining.
*/
public Builder setVolume(long value) {
volume_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* sint64 volume = 2;
* @return This builder for chaining.
*/
public Builder clearVolume() {
bitField0_ = (bitField0_ & ~0x00000002);
volume_ = 0L;
onChanged();
return this;
}
// @@protoc_insertion_point(builder_scope:org.openfeed.VolumeAtPrice.PriceLevelVolume)
}
// @@protoc_insertion_point(class_scope:org.openfeed.VolumeAtPrice.PriceLevelVolume)
private static final org.openfeed.VolumeAtPrice.PriceLevelVolume DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.openfeed.VolumeAtPrice.PriceLevelVolume();
}
public static org.openfeed.VolumeAtPrice.PriceLevelVolume getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public PriceLevelVolume parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.openfeed.VolumeAtPrice.PriceLevelVolume getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
public static final int MARKETID_FIELD_NUMBER = 1;
private long marketId_ = 0L;
/**
* sint64 marketId = 1;
* @return The marketId.
*/
@java.lang.Override
public long getMarketId() {
return marketId_;
}
public static final int SYMBOL_FIELD_NUMBER = 2;
@SuppressWarnings("serial")
private volatile java.lang.Object symbol_ = "";
/**
* string symbol = 2;
* @return The symbol.
*/
@java.lang.Override
public java.lang.String getSymbol() {
java.lang.Object ref = symbol_;
if (ref instanceof java.lang.String) {
return (java.lang.String) ref;
} else {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
symbol_ = s;
return s;
}
}
/**
* string symbol = 2;
* @return The bytes for symbol.
*/
@java.lang.Override
public com.google.protobuf.ByteString
getSymbolBytes() {
java.lang.Object ref = symbol_;
if (ref instanceof java.lang.String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
symbol_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
public static final int TRANSACTIONTIME_FIELD_NUMBER = 3;
private long transactionTime_ = 0L;
/**
*
* / UTC Timestamp of transaction, nano seconds since Unix epoch
*
*
* sint64 transactionTime = 3;
* @return The transactionTime.
*/
@java.lang.Override
public long getTransactionTime() {
return transactionTime_;
}
public static final int LASTPRICE_FIELD_NUMBER = 4;
private long lastPrice_ = 0L;
/**
* sint64 lastPrice = 4;
* @return The lastPrice.
*/
@java.lang.Override
public long getLastPrice() {
return lastPrice_;
}
public static final int LASTQUANTITY_FIELD_NUMBER = 5;
private long lastQuantity_ = 0L;
/**
* sint64 lastQuantity = 5;
* @return The lastQuantity.
*/
@java.lang.Override
public long getLastQuantity() {
return lastQuantity_;
}
public static final int LASTCUMULATIVEVOLUME_FIELD_NUMBER = 6;
private long lastCumulativeVolume_ = 0L;
/**
* sint64 lastCumulativeVolume = 6;
* @return The lastCumulativeVolume.
*/
@java.lang.Override
public long getLastCumulativeVolume() {
return lastCumulativeVolume_;
}
public static final int TRADEDATE_FIELD_NUMBER = 7;
private int tradeDate_ = 0;
/**
*
* / Date only, format 2012-07-04 -> 20120704
*
*
* sint32 tradeDate = 7;
* @return The tradeDate.
*/
@java.lang.Override
public int getTradeDate() {
return tradeDate_;
}
public static final int PRICEVOLUMES_FIELD_NUMBER = 8;
@SuppressWarnings("serial")
private java.util.List priceVolumes_;
/**
* repeated .org.openfeed.VolumeAtPrice.PriceLevelVolume priceVolumes = 8;
*/
@java.lang.Override
public java.util.List getPriceVolumesList() {
return priceVolumes_;
}
/**
* repeated .org.openfeed.VolumeAtPrice.PriceLevelVolume priceVolumes = 8;
*/
@java.lang.Override
public java.util.List extends org.openfeed.VolumeAtPrice.PriceLevelVolumeOrBuilder>
getPriceVolumesOrBuilderList() {
return priceVolumes_;
}
/**
* repeated .org.openfeed.VolumeAtPrice.PriceLevelVolume priceVolumes = 8;
*/
@java.lang.Override
public int getPriceVolumesCount() {
return priceVolumes_.size();
}
/**
* repeated .org.openfeed.VolumeAtPrice.PriceLevelVolume priceVolumes = 8;
*/
@java.lang.Override
public org.openfeed.VolumeAtPrice.PriceLevelVolume getPriceVolumes(int index) {
return priceVolumes_.get(index);
}
/**
* repeated .org.openfeed.VolumeAtPrice.PriceLevelVolume priceVolumes = 8;
*/
@java.lang.Override
public org.openfeed.VolumeAtPrice.PriceLevelVolumeOrBuilder getPriceVolumesOrBuilder(
int index) {
return priceVolumes_.get(index);
}
private byte memoizedIsInitialized = -1;
@java.lang.Override
public final boolean isInitialized() {
byte isInitialized = memoizedIsInitialized;
if (isInitialized == 1) return true;
if (isInitialized == 0) return false;
memoizedIsInitialized = 1;
return true;
}
@java.lang.Override
public void writeTo(com.google.protobuf.CodedOutputStream output)
throws java.io.IOException {
if (marketId_ != 0L) {
output.writeSInt64(1, marketId_);
}
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(symbol_)) {
com.google.protobuf.GeneratedMessage.writeString(output, 2, symbol_);
}
if (transactionTime_ != 0L) {
output.writeSInt64(3, transactionTime_);
}
if (lastPrice_ != 0L) {
output.writeSInt64(4, lastPrice_);
}
if (lastQuantity_ != 0L) {
output.writeSInt64(5, lastQuantity_);
}
if (lastCumulativeVolume_ != 0L) {
output.writeSInt64(6, lastCumulativeVolume_);
}
if (tradeDate_ != 0) {
output.writeSInt32(7, tradeDate_);
}
for (int i = 0; i < priceVolumes_.size(); i++) {
output.writeMessage(8, priceVolumes_.get(i));
}
getUnknownFields().writeTo(output);
}
@java.lang.Override
public int getSerializedSize() {
int size = memoizedSize;
if (size != -1) return size;
size = 0;
if (marketId_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeSInt64Size(1, marketId_);
}
if (!com.google.protobuf.GeneratedMessage.isStringEmpty(symbol_)) {
size += com.google.protobuf.GeneratedMessage.computeStringSize(2, symbol_);
}
if (transactionTime_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeSInt64Size(3, transactionTime_);
}
if (lastPrice_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeSInt64Size(4, lastPrice_);
}
if (lastQuantity_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeSInt64Size(5, lastQuantity_);
}
if (lastCumulativeVolume_ != 0L) {
size += com.google.protobuf.CodedOutputStream
.computeSInt64Size(6, lastCumulativeVolume_);
}
if (tradeDate_ != 0) {
size += com.google.protobuf.CodedOutputStream
.computeSInt32Size(7, tradeDate_);
}
for (int i = 0; i < priceVolumes_.size(); i++) {
size += com.google.protobuf.CodedOutputStream
.computeMessageSize(8, priceVolumes_.get(i));
}
size += getUnknownFields().getSerializedSize();
memoizedSize = size;
return size;
}
@java.lang.Override
public boolean equals(final java.lang.Object obj) {
if (obj == this) {
return true;
}
if (!(obj instanceof org.openfeed.VolumeAtPrice)) {
return super.equals(obj);
}
org.openfeed.VolumeAtPrice other = (org.openfeed.VolumeAtPrice) obj;
if (getMarketId()
!= other.getMarketId()) return false;
if (!getSymbol()
.equals(other.getSymbol())) return false;
if (getTransactionTime()
!= other.getTransactionTime()) return false;
if (getLastPrice()
!= other.getLastPrice()) return false;
if (getLastQuantity()
!= other.getLastQuantity()) return false;
if (getLastCumulativeVolume()
!= other.getLastCumulativeVolume()) return false;
if (getTradeDate()
!= other.getTradeDate()) return false;
if (!getPriceVolumesList()
.equals(other.getPriceVolumesList())) return false;
if (!getUnknownFields().equals(other.getUnknownFields())) return false;
return true;
}
@java.lang.Override
public int hashCode() {
if (memoizedHashCode != 0) {
return memoizedHashCode;
}
int hash = 41;
hash = (19 * hash) + getDescriptor().hashCode();
hash = (37 * hash) + MARKETID_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getMarketId());
hash = (37 * hash) + SYMBOL_FIELD_NUMBER;
hash = (53 * hash) + getSymbol().hashCode();
hash = (37 * hash) + TRANSACTIONTIME_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getTransactionTime());
hash = (37 * hash) + LASTPRICE_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getLastPrice());
hash = (37 * hash) + LASTQUANTITY_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getLastQuantity());
hash = (37 * hash) + LASTCUMULATIVEVOLUME_FIELD_NUMBER;
hash = (53 * hash) + com.google.protobuf.Internal.hashLong(
getLastCumulativeVolume());
hash = (37 * hash) + TRADEDATE_FIELD_NUMBER;
hash = (53 * hash) + getTradeDate();
if (getPriceVolumesCount() > 0) {
hash = (37 * hash) + PRICEVOLUMES_FIELD_NUMBER;
hash = (53 * hash) + getPriceVolumesList().hashCode();
}
hash = (29 * hash) + getUnknownFields().hashCode();
memoizedHashCode = hash;
return hash;
}
public static org.openfeed.VolumeAtPrice parseFrom(
java.nio.ByteBuffer data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.openfeed.VolumeAtPrice parseFrom(
java.nio.ByteBuffer data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.openfeed.VolumeAtPrice parseFrom(
com.google.protobuf.ByteString data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.openfeed.VolumeAtPrice parseFrom(
com.google.protobuf.ByteString data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.openfeed.VolumeAtPrice parseFrom(byte[] data)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data);
}
public static org.openfeed.VolumeAtPrice parseFrom(
byte[] data,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
return PARSER.parseFrom(data, extensionRegistry);
}
public static org.openfeed.VolumeAtPrice parseFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static org.openfeed.VolumeAtPrice parseFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
public static org.openfeed.VolumeAtPrice parseDelimitedFrom(java.io.InputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input);
}
public static org.openfeed.VolumeAtPrice parseDelimitedFrom(
java.io.InputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseDelimitedWithIOException(PARSER, input, extensionRegistry);
}
public static org.openfeed.VolumeAtPrice parseFrom(
com.google.protobuf.CodedInputStream input)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input);
}
public static org.openfeed.VolumeAtPrice parseFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
return com.google.protobuf.GeneratedMessage
.parseWithIOException(PARSER, input, extensionRegistry);
}
@java.lang.Override
public Builder newBuilderForType() { return newBuilder(); }
public static Builder newBuilder() {
return DEFAULT_INSTANCE.toBuilder();
}
public static Builder newBuilder(org.openfeed.VolumeAtPrice prototype) {
return DEFAULT_INSTANCE.toBuilder().mergeFrom(prototype);
}
@java.lang.Override
public Builder toBuilder() {
return this == DEFAULT_INSTANCE
? new Builder() : new Builder().mergeFrom(this);
}
@java.lang.Override
protected Builder newBuilderForType(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
Builder builder = new Builder(parent);
return builder;
}
/**
*
* / The VolumeAtPrice class encapsulates all of the trades throughout the day,
* / and organizes a table of volume at prices.
*
*
* Protobuf type {@code org.openfeed.VolumeAtPrice}
*/
public static final class Builder extends
com.google.protobuf.GeneratedMessage.Builder implements
// @@protoc_insertion_point(builder_implements:org.openfeed.VolumeAtPrice)
org.openfeed.VolumeAtPriceOrBuilder {
public static final com.google.protobuf.Descriptors.Descriptor
getDescriptor() {
return org.openfeed.Openfeed.internal_static_org_openfeed_VolumeAtPrice_descriptor;
}
@java.lang.Override
protected com.google.protobuf.GeneratedMessage.FieldAccessorTable
internalGetFieldAccessorTable() {
return org.openfeed.Openfeed.internal_static_org_openfeed_VolumeAtPrice_fieldAccessorTable
.ensureFieldAccessorsInitialized(
org.openfeed.VolumeAtPrice.class, org.openfeed.VolumeAtPrice.Builder.class);
}
// Construct using org.openfeed.VolumeAtPrice.newBuilder()
private Builder() {
}
private Builder(
com.google.protobuf.GeneratedMessage.BuilderParent parent) {
super(parent);
}
@java.lang.Override
public Builder clear() {
super.clear();
bitField0_ = 0;
marketId_ = 0L;
symbol_ = "";
transactionTime_ = 0L;
lastPrice_ = 0L;
lastQuantity_ = 0L;
lastCumulativeVolume_ = 0L;
tradeDate_ = 0;
if (priceVolumesBuilder_ == null) {
priceVolumes_ = java.util.Collections.emptyList();
} else {
priceVolumes_ = null;
priceVolumesBuilder_.clear();
}
bitField0_ = (bitField0_ & ~0x00000080);
return this;
}
@java.lang.Override
public com.google.protobuf.Descriptors.Descriptor
getDescriptorForType() {
return org.openfeed.Openfeed.internal_static_org_openfeed_VolumeAtPrice_descriptor;
}
@java.lang.Override
public org.openfeed.VolumeAtPrice getDefaultInstanceForType() {
return org.openfeed.VolumeAtPrice.getDefaultInstance();
}
@java.lang.Override
public org.openfeed.VolumeAtPrice build() {
org.openfeed.VolumeAtPrice result = buildPartial();
if (!result.isInitialized()) {
throw newUninitializedMessageException(result);
}
return result;
}
@java.lang.Override
public org.openfeed.VolumeAtPrice buildPartial() {
org.openfeed.VolumeAtPrice result = new org.openfeed.VolumeAtPrice(this);
buildPartialRepeatedFields(result);
if (bitField0_ != 0) { buildPartial0(result); }
onBuilt();
return result;
}
private void buildPartialRepeatedFields(org.openfeed.VolumeAtPrice result) {
if (priceVolumesBuilder_ == null) {
if (((bitField0_ & 0x00000080) != 0)) {
priceVolumes_ = java.util.Collections.unmodifiableList(priceVolumes_);
bitField0_ = (bitField0_ & ~0x00000080);
}
result.priceVolumes_ = priceVolumes_;
} else {
result.priceVolumes_ = priceVolumesBuilder_.build();
}
}
private void buildPartial0(org.openfeed.VolumeAtPrice result) {
int from_bitField0_ = bitField0_;
if (((from_bitField0_ & 0x00000001) != 0)) {
result.marketId_ = marketId_;
}
if (((from_bitField0_ & 0x00000002) != 0)) {
result.symbol_ = symbol_;
}
if (((from_bitField0_ & 0x00000004) != 0)) {
result.transactionTime_ = transactionTime_;
}
if (((from_bitField0_ & 0x00000008) != 0)) {
result.lastPrice_ = lastPrice_;
}
if (((from_bitField0_ & 0x00000010) != 0)) {
result.lastQuantity_ = lastQuantity_;
}
if (((from_bitField0_ & 0x00000020) != 0)) {
result.lastCumulativeVolume_ = lastCumulativeVolume_;
}
if (((from_bitField0_ & 0x00000040) != 0)) {
result.tradeDate_ = tradeDate_;
}
}
@java.lang.Override
public Builder mergeFrom(com.google.protobuf.Message other) {
if (other instanceof org.openfeed.VolumeAtPrice) {
return mergeFrom((org.openfeed.VolumeAtPrice)other);
} else {
super.mergeFrom(other);
return this;
}
}
public Builder mergeFrom(org.openfeed.VolumeAtPrice other) {
if (other == org.openfeed.VolumeAtPrice.getDefaultInstance()) return this;
if (other.getMarketId() != 0L) {
setMarketId(other.getMarketId());
}
if (!other.getSymbol().isEmpty()) {
symbol_ = other.symbol_;
bitField0_ |= 0x00000002;
onChanged();
}
if (other.getTransactionTime() != 0L) {
setTransactionTime(other.getTransactionTime());
}
if (other.getLastPrice() != 0L) {
setLastPrice(other.getLastPrice());
}
if (other.getLastQuantity() != 0L) {
setLastQuantity(other.getLastQuantity());
}
if (other.getLastCumulativeVolume() != 0L) {
setLastCumulativeVolume(other.getLastCumulativeVolume());
}
if (other.getTradeDate() != 0) {
setTradeDate(other.getTradeDate());
}
if (priceVolumesBuilder_ == null) {
if (!other.priceVolumes_.isEmpty()) {
if (priceVolumes_.isEmpty()) {
priceVolumes_ = other.priceVolumes_;
bitField0_ = (bitField0_ & ~0x00000080);
} else {
ensurePriceVolumesIsMutable();
priceVolumes_.addAll(other.priceVolumes_);
}
onChanged();
}
} else {
if (!other.priceVolumes_.isEmpty()) {
if (priceVolumesBuilder_.isEmpty()) {
priceVolumesBuilder_.dispose();
priceVolumesBuilder_ = null;
priceVolumes_ = other.priceVolumes_;
bitField0_ = (bitField0_ & ~0x00000080);
priceVolumesBuilder_ =
com.google.protobuf.GeneratedMessage.alwaysUseFieldBuilders ?
getPriceVolumesFieldBuilder() : null;
} else {
priceVolumesBuilder_.addAllMessages(other.priceVolumes_);
}
}
}
this.mergeUnknownFields(other.getUnknownFields());
onChanged();
return this;
}
@java.lang.Override
public final boolean isInitialized() {
return true;
}
@java.lang.Override
public Builder mergeFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws java.io.IOException {
if (extensionRegistry == null) {
throw new java.lang.NullPointerException();
}
try {
boolean done = false;
while (!done) {
int tag = input.readTag();
switch (tag) {
case 0:
done = true;
break;
case 8: {
marketId_ = input.readSInt64();
bitField0_ |= 0x00000001;
break;
} // case 8
case 18: {
symbol_ = input.readStringRequireUtf8();
bitField0_ |= 0x00000002;
break;
} // case 18
case 24: {
transactionTime_ = input.readSInt64();
bitField0_ |= 0x00000004;
break;
} // case 24
case 32: {
lastPrice_ = input.readSInt64();
bitField0_ |= 0x00000008;
break;
} // case 32
case 40: {
lastQuantity_ = input.readSInt64();
bitField0_ |= 0x00000010;
break;
} // case 40
case 48: {
lastCumulativeVolume_ = input.readSInt64();
bitField0_ |= 0x00000020;
break;
} // case 48
case 56: {
tradeDate_ = input.readSInt32();
bitField0_ |= 0x00000040;
break;
} // case 56
case 66: {
org.openfeed.VolumeAtPrice.PriceLevelVolume m =
input.readMessage(
org.openfeed.VolumeAtPrice.PriceLevelVolume.parser(),
extensionRegistry);
if (priceVolumesBuilder_ == null) {
ensurePriceVolumesIsMutable();
priceVolumes_.add(m);
} else {
priceVolumesBuilder_.addMessage(m);
}
break;
} // case 66
default: {
if (!super.parseUnknownField(input, extensionRegistry, tag)) {
done = true; // was an endgroup tag
}
break;
} // default:
} // switch (tag)
} // while (!done)
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.unwrapIOException();
} finally {
onChanged();
} // finally
return this;
}
private int bitField0_;
private long marketId_ ;
/**
* sint64 marketId = 1;
* @return The marketId.
*/
@java.lang.Override
public long getMarketId() {
return marketId_;
}
/**
* sint64 marketId = 1;
* @param value The marketId to set.
* @return This builder for chaining.
*/
public Builder setMarketId(long value) {
marketId_ = value;
bitField0_ |= 0x00000001;
onChanged();
return this;
}
/**
* sint64 marketId = 1;
* @return This builder for chaining.
*/
public Builder clearMarketId() {
bitField0_ = (bitField0_ & ~0x00000001);
marketId_ = 0L;
onChanged();
return this;
}
private java.lang.Object symbol_ = "";
/**
* string symbol = 2;
* @return The symbol.
*/
public java.lang.String getSymbol() {
java.lang.Object ref = symbol_;
if (!(ref instanceof java.lang.String)) {
com.google.protobuf.ByteString bs =
(com.google.protobuf.ByteString) ref;
java.lang.String s = bs.toStringUtf8();
symbol_ = s;
return s;
} else {
return (java.lang.String) ref;
}
}
/**
* string symbol = 2;
* @return The bytes for symbol.
*/
public com.google.protobuf.ByteString
getSymbolBytes() {
java.lang.Object ref = symbol_;
if (ref instanceof String) {
com.google.protobuf.ByteString b =
com.google.protobuf.ByteString.copyFromUtf8(
(java.lang.String) ref);
symbol_ = b;
return b;
} else {
return (com.google.protobuf.ByteString) ref;
}
}
/**
* string symbol = 2;
* @param value The symbol to set.
* @return This builder for chaining.
*/
public Builder setSymbol(
java.lang.String value) {
if (value == null) { throw new NullPointerException(); }
symbol_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
/**
* string symbol = 2;
* @return This builder for chaining.
*/
public Builder clearSymbol() {
symbol_ = getDefaultInstance().getSymbol();
bitField0_ = (bitField0_ & ~0x00000002);
onChanged();
return this;
}
/**
* string symbol = 2;
* @param value The bytes for symbol to set.
* @return This builder for chaining.
*/
public Builder setSymbolBytes(
com.google.protobuf.ByteString value) {
if (value == null) { throw new NullPointerException(); }
checkByteStringIsUtf8(value);
symbol_ = value;
bitField0_ |= 0x00000002;
onChanged();
return this;
}
private long transactionTime_ ;
/**
*
* / UTC Timestamp of transaction, nano seconds since Unix epoch
*
*
* sint64 transactionTime = 3;
* @return The transactionTime.
*/
@java.lang.Override
public long getTransactionTime() {
return transactionTime_;
}
/**
*
* / UTC Timestamp of transaction, nano seconds since Unix epoch
*
*
* sint64 transactionTime = 3;
* @param value The transactionTime to set.
* @return This builder for chaining.
*/
public Builder setTransactionTime(long value) {
transactionTime_ = value;
bitField0_ |= 0x00000004;
onChanged();
return this;
}
/**
*
* / UTC Timestamp of transaction, nano seconds since Unix epoch
*
*
* sint64 transactionTime = 3;
* @return This builder for chaining.
*/
public Builder clearTransactionTime() {
bitField0_ = (bitField0_ & ~0x00000004);
transactionTime_ = 0L;
onChanged();
return this;
}
private long lastPrice_ ;
/**
* sint64 lastPrice = 4;
* @return The lastPrice.
*/
@java.lang.Override
public long getLastPrice() {
return lastPrice_;
}
/**
* sint64 lastPrice = 4;
* @param value The lastPrice to set.
* @return This builder for chaining.
*/
public Builder setLastPrice(long value) {
lastPrice_ = value;
bitField0_ |= 0x00000008;
onChanged();
return this;
}
/**
* sint64 lastPrice = 4;
* @return This builder for chaining.
*/
public Builder clearLastPrice() {
bitField0_ = (bitField0_ & ~0x00000008);
lastPrice_ = 0L;
onChanged();
return this;
}
private long lastQuantity_ ;
/**
* sint64 lastQuantity = 5;
* @return The lastQuantity.
*/
@java.lang.Override
public long getLastQuantity() {
return lastQuantity_;
}
/**
* sint64 lastQuantity = 5;
* @param value The lastQuantity to set.
* @return This builder for chaining.
*/
public Builder setLastQuantity(long value) {
lastQuantity_ = value;
bitField0_ |= 0x00000010;
onChanged();
return this;
}
/**
* sint64 lastQuantity = 5;
* @return This builder for chaining.
*/
public Builder clearLastQuantity() {
bitField0_ = (bitField0_ & ~0x00000010);
lastQuantity_ = 0L;
onChanged();
return this;
}
private long lastCumulativeVolume_ ;
/**
* sint64 lastCumulativeVolume = 6;
* @return The lastCumulativeVolume.
*/
@java.lang.Override
public long getLastCumulativeVolume() {
return lastCumulativeVolume_;
}
/**
* sint64 lastCumulativeVolume = 6;
* @param value The lastCumulativeVolume to set.
* @return This builder for chaining.
*/
public Builder setLastCumulativeVolume(long value) {
lastCumulativeVolume_ = value;
bitField0_ |= 0x00000020;
onChanged();
return this;
}
/**
* sint64 lastCumulativeVolume = 6;
* @return This builder for chaining.
*/
public Builder clearLastCumulativeVolume() {
bitField0_ = (bitField0_ & ~0x00000020);
lastCumulativeVolume_ = 0L;
onChanged();
return this;
}
private int tradeDate_ ;
/**
*
* / Date only, format 2012-07-04 -> 20120704
*
*
* sint32 tradeDate = 7;
* @return The tradeDate.
*/
@java.lang.Override
public int getTradeDate() {
return tradeDate_;
}
/**
*
* / Date only, format 2012-07-04 -> 20120704
*
*
* sint32 tradeDate = 7;
* @param value The tradeDate to set.
* @return This builder for chaining.
*/
public Builder setTradeDate(int value) {
tradeDate_ = value;
bitField0_ |= 0x00000040;
onChanged();
return this;
}
/**
*
* / Date only, format 2012-07-04 -> 20120704
*
*
* sint32 tradeDate = 7;
* @return This builder for chaining.
*/
public Builder clearTradeDate() {
bitField0_ = (bitField0_ & ~0x00000040);
tradeDate_ = 0;
onChanged();
return this;
}
private java.util.List priceVolumes_ =
java.util.Collections.emptyList();
private void ensurePriceVolumesIsMutable() {
if (!((bitField0_ & 0x00000080) != 0)) {
priceVolumes_ = new java.util.ArrayList(priceVolumes_);
bitField0_ |= 0x00000080;
}
}
private com.google.protobuf.RepeatedFieldBuilder<
org.openfeed.VolumeAtPrice.PriceLevelVolume, org.openfeed.VolumeAtPrice.PriceLevelVolume.Builder, org.openfeed.VolumeAtPrice.PriceLevelVolumeOrBuilder> priceVolumesBuilder_;
/**
* repeated .org.openfeed.VolumeAtPrice.PriceLevelVolume priceVolumes = 8;
*/
public java.util.List getPriceVolumesList() {
if (priceVolumesBuilder_ == null) {
return java.util.Collections.unmodifiableList(priceVolumes_);
} else {
return priceVolumesBuilder_.getMessageList();
}
}
/**
* repeated .org.openfeed.VolumeAtPrice.PriceLevelVolume priceVolumes = 8;
*/
public int getPriceVolumesCount() {
if (priceVolumesBuilder_ == null) {
return priceVolumes_.size();
} else {
return priceVolumesBuilder_.getCount();
}
}
/**
* repeated .org.openfeed.VolumeAtPrice.PriceLevelVolume priceVolumes = 8;
*/
public org.openfeed.VolumeAtPrice.PriceLevelVolume getPriceVolumes(int index) {
if (priceVolumesBuilder_ == null) {
return priceVolumes_.get(index);
} else {
return priceVolumesBuilder_.getMessage(index);
}
}
/**
* repeated .org.openfeed.VolumeAtPrice.PriceLevelVolume priceVolumes = 8;
*/
public Builder setPriceVolumes(
int index, org.openfeed.VolumeAtPrice.PriceLevelVolume value) {
if (priceVolumesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensurePriceVolumesIsMutable();
priceVolumes_.set(index, value);
onChanged();
} else {
priceVolumesBuilder_.setMessage(index, value);
}
return this;
}
/**
* repeated .org.openfeed.VolumeAtPrice.PriceLevelVolume priceVolumes = 8;
*/
public Builder setPriceVolumes(
int index, org.openfeed.VolumeAtPrice.PriceLevelVolume.Builder builderForValue) {
if (priceVolumesBuilder_ == null) {
ensurePriceVolumesIsMutable();
priceVolumes_.set(index, builderForValue.build());
onChanged();
} else {
priceVolumesBuilder_.setMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .org.openfeed.VolumeAtPrice.PriceLevelVolume priceVolumes = 8;
*/
public Builder addPriceVolumes(org.openfeed.VolumeAtPrice.PriceLevelVolume value) {
if (priceVolumesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensurePriceVolumesIsMutable();
priceVolumes_.add(value);
onChanged();
} else {
priceVolumesBuilder_.addMessage(value);
}
return this;
}
/**
* repeated .org.openfeed.VolumeAtPrice.PriceLevelVolume priceVolumes = 8;
*/
public Builder addPriceVolumes(
int index, org.openfeed.VolumeAtPrice.PriceLevelVolume value) {
if (priceVolumesBuilder_ == null) {
if (value == null) {
throw new NullPointerException();
}
ensurePriceVolumesIsMutable();
priceVolumes_.add(index, value);
onChanged();
} else {
priceVolumesBuilder_.addMessage(index, value);
}
return this;
}
/**
* repeated .org.openfeed.VolumeAtPrice.PriceLevelVolume priceVolumes = 8;
*/
public Builder addPriceVolumes(
org.openfeed.VolumeAtPrice.PriceLevelVolume.Builder builderForValue) {
if (priceVolumesBuilder_ == null) {
ensurePriceVolumesIsMutable();
priceVolumes_.add(builderForValue.build());
onChanged();
} else {
priceVolumesBuilder_.addMessage(builderForValue.build());
}
return this;
}
/**
* repeated .org.openfeed.VolumeAtPrice.PriceLevelVolume priceVolumes = 8;
*/
public Builder addPriceVolumes(
int index, org.openfeed.VolumeAtPrice.PriceLevelVolume.Builder builderForValue) {
if (priceVolumesBuilder_ == null) {
ensurePriceVolumesIsMutable();
priceVolumes_.add(index, builderForValue.build());
onChanged();
} else {
priceVolumesBuilder_.addMessage(index, builderForValue.build());
}
return this;
}
/**
* repeated .org.openfeed.VolumeAtPrice.PriceLevelVolume priceVolumes = 8;
*/
public Builder addAllPriceVolumes(
java.lang.Iterable extends org.openfeed.VolumeAtPrice.PriceLevelVolume> values) {
if (priceVolumesBuilder_ == null) {
ensurePriceVolumesIsMutable();
com.google.protobuf.AbstractMessageLite.Builder.addAll(
values, priceVolumes_);
onChanged();
} else {
priceVolumesBuilder_.addAllMessages(values);
}
return this;
}
/**
* repeated .org.openfeed.VolumeAtPrice.PriceLevelVolume priceVolumes = 8;
*/
public Builder clearPriceVolumes() {
if (priceVolumesBuilder_ == null) {
priceVolumes_ = java.util.Collections.emptyList();
bitField0_ = (bitField0_ & ~0x00000080);
onChanged();
} else {
priceVolumesBuilder_.clear();
}
return this;
}
/**
* repeated .org.openfeed.VolumeAtPrice.PriceLevelVolume priceVolumes = 8;
*/
public Builder removePriceVolumes(int index) {
if (priceVolumesBuilder_ == null) {
ensurePriceVolumesIsMutable();
priceVolumes_.remove(index);
onChanged();
} else {
priceVolumesBuilder_.remove(index);
}
return this;
}
/**
* repeated .org.openfeed.VolumeAtPrice.PriceLevelVolume priceVolumes = 8;
*/
public org.openfeed.VolumeAtPrice.PriceLevelVolume.Builder getPriceVolumesBuilder(
int index) {
return getPriceVolumesFieldBuilder().getBuilder(index);
}
/**
* repeated .org.openfeed.VolumeAtPrice.PriceLevelVolume priceVolumes = 8;
*/
public org.openfeed.VolumeAtPrice.PriceLevelVolumeOrBuilder getPriceVolumesOrBuilder(
int index) {
if (priceVolumesBuilder_ == null) {
return priceVolumes_.get(index); } else {
return priceVolumesBuilder_.getMessageOrBuilder(index);
}
}
/**
* repeated .org.openfeed.VolumeAtPrice.PriceLevelVolume priceVolumes = 8;
*/
public java.util.List extends org.openfeed.VolumeAtPrice.PriceLevelVolumeOrBuilder>
getPriceVolumesOrBuilderList() {
if (priceVolumesBuilder_ != null) {
return priceVolumesBuilder_.getMessageOrBuilderList();
} else {
return java.util.Collections.unmodifiableList(priceVolumes_);
}
}
/**
* repeated .org.openfeed.VolumeAtPrice.PriceLevelVolume priceVolumes = 8;
*/
public org.openfeed.VolumeAtPrice.PriceLevelVolume.Builder addPriceVolumesBuilder() {
return getPriceVolumesFieldBuilder().addBuilder(
org.openfeed.VolumeAtPrice.PriceLevelVolume.getDefaultInstance());
}
/**
* repeated .org.openfeed.VolumeAtPrice.PriceLevelVolume priceVolumes = 8;
*/
public org.openfeed.VolumeAtPrice.PriceLevelVolume.Builder addPriceVolumesBuilder(
int index) {
return getPriceVolumesFieldBuilder().addBuilder(
index, org.openfeed.VolumeAtPrice.PriceLevelVolume.getDefaultInstance());
}
/**
* repeated .org.openfeed.VolumeAtPrice.PriceLevelVolume priceVolumes = 8;
*/
public java.util.List
getPriceVolumesBuilderList() {
return getPriceVolumesFieldBuilder().getBuilderList();
}
private com.google.protobuf.RepeatedFieldBuilder<
org.openfeed.VolumeAtPrice.PriceLevelVolume, org.openfeed.VolumeAtPrice.PriceLevelVolume.Builder, org.openfeed.VolumeAtPrice.PriceLevelVolumeOrBuilder>
getPriceVolumesFieldBuilder() {
if (priceVolumesBuilder_ == null) {
priceVolumesBuilder_ = new com.google.protobuf.RepeatedFieldBuilder<
org.openfeed.VolumeAtPrice.PriceLevelVolume, org.openfeed.VolumeAtPrice.PriceLevelVolume.Builder, org.openfeed.VolumeAtPrice.PriceLevelVolumeOrBuilder>(
priceVolumes_,
((bitField0_ & 0x00000080) != 0),
getParentForChildren(),
isClean());
priceVolumes_ = null;
}
return priceVolumesBuilder_;
}
// @@protoc_insertion_point(builder_scope:org.openfeed.VolumeAtPrice)
}
// @@protoc_insertion_point(class_scope:org.openfeed.VolumeAtPrice)
private static final org.openfeed.VolumeAtPrice DEFAULT_INSTANCE;
static {
DEFAULT_INSTANCE = new org.openfeed.VolumeAtPrice();
}
public static org.openfeed.VolumeAtPrice getDefaultInstance() {
return DEFAULT_INSTANCE;
}
private static final com.google.protobuf.Parser
PARSER = new com.google.protobuf.AbstractParser() {
@java.lang.Override
public VolumeAtPrice parsePartialFrom(
com.google.protobuf.CodedInputStream input,
com.google.protobuf.ExtensionRegistryLite extensionRegistry)
throws com.google.protobuf.InvalidProtocolBufferException {
Builder builder = newBuilder();
try {
builder.mergeFrom(input, extensionRegistry);
} catch (com.google.protobuf.InvalidProtocolBufferException e) {
throw e.setUnfinishedMessage(builder.buildPartial());
} catch (com.google.protobuf.UninitializedMessageException e) {
throw e.asInvalidProtocolBufferException().setUnfinishedMessage(builder.buildPartial());
} catch (java.io.IOException e) {
throw new com.google.protobuf.InvalidProtocolBufferException(e)
.setUnfinishedMessage(builder.buildPartial());
}
return builder.buildPartial();
}
};
public static com.google.protobuf.Parser parser() {
return PARSER;
}
@java.lang.Override
public com.google.protobuf.Parser getParserForType() {
return PARSER;
}
@java.lang.Override
public org.openfeed.VolumeAtPrice getDefaultInstanceForType() {
return DEFAULT_INSTANCE;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy