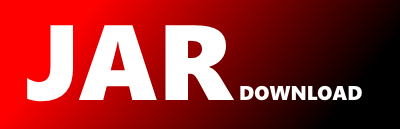
org.openforis.collect.datacleansing.DataCleansingMetadataView Maven / Gradle / Ivy
package org.openforis.collect.datacleansing;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Collections;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.UUID;
import org.openforis.collect.datacleansing.form.DataCleansingChainForm;
import org.openforis.collect.datacleansing.form.DataCleansingItemForm;
import org.openforis.collect.datacleansing.form.DataCleansingStepForm;
import org.openforis.collect.datacleansing.form.DataQueryForm;
import org.openforis.collect.datacleansing.form.DataQueryGroupForm;
import org.openforis.collect.datacleansing.form.DataQueryTypeForm;
import org.openforis.collect.model.CollectSurvey;
import org.openforis.commons.collection.CollectionUtils;
/**
*
* @author S. Ricci
*
*/
public class DataCleansingMetadataView {
private List dataQueryTypes;
private List dataQueries;
private List dataQueryGroups;
private List cleansingSteps;
private List cleansingChains;
public DataCleansingMetadataView() {
}
public static DataCleansingMetadataView fromMetadata(DataCleansingMetadata metadata) {
DataCleansingMetadataView view = new DataCleansingMetadataView();
view.dataQueryTypes = PersistedSurveyObjects.convert(metadata.getDataQueryTypes(), DataQueryTypeForm.class);
view.dataQueries = PersistedSurveyObjects.convert(metadata.getDataQueries(), DataQueryForm.class);
view.dataQueryGroups = PersistedSurveyObjects.convert(metadata.getDataQueryGroups(), DataQueryGroupForm.class);
view.cleansingSteps = PersistedSurveyObjects.convert(metadata.getCleansingSteps(), DataCleansingStepForm.class);
view.cleansingChains = PersistedSurveyObjects.convert(metadata.getCleansingChains(), DataCleansingChainForm.class);
return view;
}
public DataCleansingMetadata toMetadata(CollectSurvey survey) {
//convert view items to metadata items
List queryTypes = PersistedSurveyObjects.toItems(survey, this.dataQueryTypes, DataQueryType.class);
List queries = PersistedSurveyObjects.toItems(survey, this.dataQueries, DataQuery.class);
List errorQueryGroups = PersistedSurveyObjects.toItems(survey, this.dataQueryGroups, DataQueryGroup.class);
List cleansingSteps = PersistedSurveyObjects.toItems(survey, this.cleansingSteps, DataCleansingStep.class);
List cleansingChains = PersistedSurveyObjects.toItems(survey, this.cleansingChains, DataCleansingChain.class);
//create maps to facilitate object searching
Map queryTypeByOriginalId = PersistedSurveyObjects.createObjectFromIdMap(queryTypes);
Map queryByOriginalId = PersistedSurveyObjects.createObjectFromIdMap(queries);
Map cleansingStepByOriginalId = PersistedSurveyObjects.createObjectFromIdMap(cleansingSteps);
//replace objects with same instances
for (DataQuery query : queries) {
query.setType(queryTypeByOriginalId.get(query.getTypeId()));
}
for (DataQueryGroup queryGroup : errorQueryGroups) {
List originalQueryIds = queryGroup.getQueryIds();
List originalQueries = new ArrayList(originalQueryIds.size());
for (Integer originalQueryId : originalQueryIds) {
originalQueries.add(queryByOriginalId.get(originalQueryId));
}
queryGroup.removeAllQueries();
queryGroup.setQueries(originalQueries);
}
for (DataCleansingStep step : cleansingSteps) {
DataQuery query = queryByOriginalId.get(step.getQueryId());
step.setQuery(query);
}
for (DataCleansingChain chain : cleansingChains) {
DataCleansingChainForm viewItem = CollectionUtils.findItem(this.cleansingChains, chain.getId());
List stepIds = viewItem.getStepIds();
for (Integer stepId : stepIds) {
DataCleansingStep step = cleansingStepByOriginalId.get(stepId);
chain.addStep(step);
}
}
PersistedSurveyObjects.resetIds(queryTypes);
PersistedSurveyObjects.resetIds(queries);
PersistedSurveyObjects.resetIds(errorQueryGroups);
PersistedSurveyObjects.resetIds(cleansingSteps);
PersistedSurveyObjects.resetIds(cleansingChains);
DataCleansingMetadata metadata = new DataCleansingMetadata(survey,
queryTypes,
queries,
errorQueryGroups,
cleansingSteps,
cleansingChains);
return metadata;
}
private static class PersistedSurveyObjects {
public static , T extends DataCleansingItem> List convert(List items, Class resultType) {
List result = new ArrayList(items.size());
for (T i : items) {
try {
F o = resultType.getDeclaredConstructor(i.getClass()).newInstance(i);
result.add(o);
} catch (Exception e) {
String message = String.format("Error creating objects of type %s from type %s", resultType.getName(), i.getClass().getName());
throw new RuntimeException(message, e);
}
}
return result;
}
private static Map createObjectFromIdMap(Collection items) {
Map result = new HashMap(items.size());
for (T item : items) {
result.put(item.getId(), item);
}
return result;
}
private static void resetIds(Collection items) {
for (T item : items) {
item.setId(null);
}
}
private static > List toItems(
CollectSurvey survey, List formItems, Class itemType) {
if (org.apache.commons.collections.CollectionUtils.isEmpty(formItems)) {
return Collections.emptyList();
}
List items = new ArrayList(formItems.size());
for (F form : formItems) {
try {
I item = itemType.getDeclaredConstructor(CollectSurvey.class, UUID.class).newInstance(survey, form.getUuid());
form.copyTo(item, "uuid");
items.add(item);
} catch (Exception e) {
throw new RuntimeException("Error creating items from form items", e);
}
}
return items;
}
}
public List getDataQueryTypes() {
return dataQueryTypes;
}
public List getDataQueries() {
return dataQueries;
}
public List getDataQueryGroups() {
return dataQueryGroups;
}
public List getCleansingSteps() {
return cleansingSteps;
}
public List getCleansingChains() {
return cleansingChains;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy