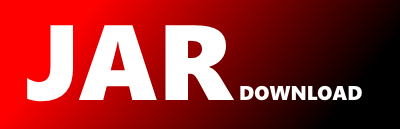
org.openforis.collect.datacleansing.DataQueryGroup Maven / Gradle / Ivy
package org.openforis.collect.datacleansing;
import java.util.ArrayList;
import java.util.List;
import java.util.UUID;
import org.openforis.collect.model.CollectSurvey;
import org.openforis.commons.collection.CollectionUtils;
/**
*
* @author S. Ricci
*
*/
public class DataQueryGroup extends DataCleansingItem {
private static final long serialVersionUID = 1L;
private String title;
private String description;
private List queryIds = new ArrayList();
private transient List queries = new ArrayList();
public DataQueryGroup(CollectSurvey survey) {
super(survey);
}
public DataQueryGroup(CollectSurvey survey, UUID uuid) {
super(survey, uuid);
}
public DataQuery getQuery(int id) {
DataQuery query = CollectionUtils.findItem(queries, id);
return query;
}
public void addQuery(DataQuery query) {
this.queries.add(query);
this.queryIds.add(query.getId());
}
public void removeAllQueries() {
this.queries.clear();
this.queryIds.clear();
}
public void allAllQueries(List queries) {
for (DataQuery query : queries) {
addQuery(query);
}
}
public String getTitle() {
return title;
}
public void setTitle(String title) {
this.title = title;
}
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public List getQueries() {
return queries;
}
public void setQueries(List queries) {
this.queries = queries;
}
public List getQueryIds() {
return queryIds;
}
public void setQueryIds(List queryIds) {
this.queryIds = queryIds;
}
@Override
public boolean deepEquals(Object obj, boolean ignoreId) {
if (this == obj)
return true;
if (!super.deepEquals(obj, ignoreId))
return false;
if (getClass() != obj.getClass())
return false;
DataQueryGroup other = (DataQueryGroup) obj;
if (! CollectionUtils.deepEquals(queries, other.queries, true))
return false;
return true;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy