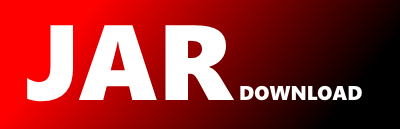
org.openforis.collect.utils.Proxies Maven / Gradle / Ivy
package org.openforis.collect.utils;
import java.beans.Expression;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Collections;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
import org.openforis.collect.Proxy;
public class Proxies {
public static P fromObject(T obj, Class
proxyType) {
if (obj == null) {
return null;
}
try {
@SuppressWarnings("unchecked")
P proxy = (P) new Expression(proxyType, "new", new Object[] {obj}).getValue();
return proxy;
} catch (Exception e) {
throw new RuntimeException("Error creating proxy", e);
}
}
public static
List
fromList(List objects, Class proxyType) {
if (objects == null) {
return Collections.emptyList();
}
List
result = new ArrayList
(objects.size());
fillWithProxies(result, objects, proxyType);
return result;
}
public static
Set
fromSet(Set objects, Class proxyType) {
if (objects == null) {
return Collections.emptySet();
}
Set
result = new HashSet
(objects.size());
fillWithProxies(result, objects, proxyType);
return result;
}
private static
void fillWithProxies(Collection
result, Collection objects, Class proxyType) {
for (T obj : objects) {
result.add(fromObject(obj, proxyType));
}
}
}