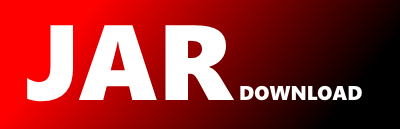
net.sf.saxon.dom.DOMSender Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of saxon9 Show documentation
Show all versions of saxon9 Show documentation
Provides a basic XSLT 2.0 and XQuery 1.0 processor (W3C Recommendations,
January 2007). Command line interfaces and implementations of several
Java APIs (DOM, XPath, s9api) are also included.
The newest version!
package net.sf.saxon.dom;
import net.sf.saxon.Configuration;
import net.sf.saxon.event.PipelineConfiguration;
import net.sf.saxon.event.Receiver;
import net.sf.saxon.event.SaxonLocator;
import net.sf.saxon.event.SourceLocationProvider;
import net.sf.saxon.om.NameChecker;
import net.sf.saxon.om.NamePool;
import net.sf.saxon.om.StandardNames;
import net.sf.saxon.trans.XPathException;
import org.w3c.dom.*;
import org.xml.sax.helpers.AttributesImpl;
import org.xml.sax.helpers.NamespaceSupport;
import java.util.HashMap;
import java.util.Iterator;
/**
* DOMSender.java: pseudo-SAX driver for a DOM source document.
* This class takes an existing
* DOM Document and walks around it in a depth-first traversal,
* calling a Receiver to process the nodes as it does so
*/
public class DOMSender implements SaxonLocator, SourceLocationProvider {
private Receiver receiver;
private PipelineConfiguration pipe;
private NamespaceSupport nsSupport = new NamespaceSupport();
private AttributesImpl attlist = new AttributesImpl();
private String[] parts = new String[3];
private String[] elparts = new String[3];
private HashMap nsDeclarations = new HashMap(10);
protected Node root = null;
protected String systemId;
/**
* Set the pipeline configuration
* @param pipe the pipeline configuration
*/
public void setPipelineConfiguration(PipelineConfiguration pipe) {
this.pipe = pipe;
}
/**
* Set the receiver.
* @param receiver The object to receive content events.
*/
public void setReceiver (Receiver receiver) {
this.receiver = receiver;
}
/**
* Set the DOM Document that will be walked
* @param start the root node from which the tree walk will start
*/
public void setStartNode(Node start) {
root = start;
}
/**
* Set the systemId of the source document (which will also be
* used for the destination)
* @param systemId the systemId of the source document
*/
public void setSystemId(String systemId) {
this.systemId = systemId;
}
/**
* Walk a document (traversing the nodes depth first)
* @exception net.sf.saxon.trans.XPathException On any error in the document
*/
public void send() throws XPathException {
if (root==null) {
throw new XPathException("DOMSender: no start node defined");
}
if (receiver==null) {
throw new XPathException("DOMSender: no receiver defined");
}
receiver.setSystemId(systemId);
pipe.setLocationProvider(this);
receiver.setPipelineConfiguration(pipe);
receiver.open();
if (root.getNodeType() == Node.ELEMENT_NODE) {
sendElement((Element)root);
} else {
// walk the root node
receiver.startDocument(0);
walkNode(root);
receiver.endDocument();
}
receiver.close();
}
/**
* Walk a document starting from a particular element node. This has to make
* sure that all the namespace declarations in scope for the element are
* treated as if they were namespace declarations on the element itself.
* @param startNode the start element node from which the walk will start
*/
private void sendElement(Element startNode) throws XPathException {
Element node = startNode;
NamedNodeMap topAtts = gatherNamespaces(node, false);
while (true) {
gatherNamespaces(node, true);
Node parent = node.getParentNode();
if (parent != null && parent.getNodeType() == Node.ELEMENT_NODE) {
node = (Element)parent;
} else {
break;
}
}
outputElement(startNode, topAtts);
}
/**
* Walk an element of a document (traversing the children depth first)
* @param node The DOM Element object to walk
* @exception net.sf.saxon.trans.XPathException On any error in the document
*
*/
private void walkNode (Node node) throws XPathException {
if (node.hasChildNodes()) {
NodeList nit = node.getChildNodes();
final int len = nit.getLength();
for (int i=0; i=0) {
try {
String prefix = att.getPrefix();
String uri = att.getNamespaceURI();
//System.err.println("Implicit Namespace: " + prefix + "=" + uri);
if (nsDeclarations.get(prefix)==null) {
nsSupport.declarePrefix(prefix, uri);
nsDeclarations.put(prefix, uri);
}
} catch (Throwable err) {
// it must be a level 1 DOM
}
}
}
return atts;
}
// Implement the SAX Locator interface. This is needed to pass the base URI of nodes
// to the receiver. We don't attempt to preserve the original base URI of each individual
// node as it is copied, only the base URI of the document as a whole.
public int getColumnNumber() {
return -1;
}
public int getLineNumber() {
return -1;
}
public String getPublicId() {
return null;
}
public String getSystemId() {
return systemId;
}
public String getSystemId(long locationId) {
return getSystemId();
}
public int getLineNumber(long locationId) {
return getLineNumber();
}
// public static void main(String[] args) throws Exception {
// Configuration config = new Configuration();
// StaticQueryContext sqc = new StaticQueryContext(config);
// DocumentInfo doc = sqc.buildDocument(new StreamSource("file:///MyJava/samples/styles/books.xsl"));
// Document dom = (Document)NodeOverNodeInfo.wrap(doc);
// DOMSender sender = new DOMSender();
// final PipelineConfiguration pipe = config.makePipelineConfiguration();
// sender.setPipelineConfiguration(pipe);
// sender.setStartNode(dom);
// Receiver r = (config.getSerializerFactory().getReceiver(
// new StreamResult(System.out), pipe, new Properties()
// ));
// NamespaceReducer nr = new NamespaceReducer();
// nr.setPipelineConfiguration(pipe);
// nr.setUnderlyingReceiver(r);
// sender.setReceiver(nr);
// sender.send();
//
// }
}
//
// The contents of this file are subject to the Mozilla Public License Version 1.0 (the "License");
// you may not use this file except in compliance with the License. You may obtain a copy of the
// License at http://www.mozilla.org/MPL/
//
// Software distributed under the License is distributed on an "AS IS" basis,
// WITHOUT WARRANTY OF ANY KIND, either express or implied.
// See the License for the specific language governing rights and limitations under the License.
//
// The Original Code is: all this file.
//
// The Initial Developer of the Original Code is Michael H. Kay.
//
// Portions created by (your name) are Copyright (C) (your legal entity). All Rights Reserved.
//
// Contributor(s): none.
//
© 2015 - 2025 Weber Informatics LLC | Privacy Policy