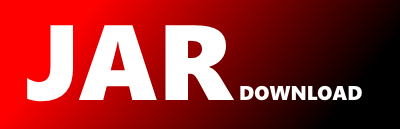
net.sf.saxon.event.XML10ContentChecker Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of saxon9 Show documentation
Show all versions of saxon9 Show documentation
Provides a basic XSLT 2.0 and XQuery 1.0 processor (W3C Recommendations,
January 2007). Command line interfaces and implementations of several
Java APIs (DOM, XPath, s9api) are also included.
The newest version!
package net.sf.saxon.event;
import net.sf.saxon.Err;
import net.sf.saxon.charcode.UTF16;
import net.sf.saxon.charcode.XMLCharacterData;
import net.sf.saxon.expr.ExpressionLocation;
import net.sf.saxon.om.Name10Checker;
import net.sf.saxon.om.NameChecker;
import net.sf.saxon.om.NamePool;
import net.sf.saxon.sort.IntHashSet;
import net.sf.saxon.trans.XPathException;
/**
* This class is used on the serialization pipeline to check that the document conforms
* to XML 1.0 rules. It is placed on the pipeline only when the configuration permits
* XML 1.1 constructs, but the particular output document is being serialized as XML 1.0
*/
public class XML10ContentChecker extends ProxyReceiver {
private NameChecker checker = Name10Checker.getInstance();
private NamePool pool;
private IntHashSet cache = new IntHashSet(100);
public void setPipelineConfiguration(PipelineConfiguration pipe) {
pool = pipe.getConfiguration().getNamePool();
super.setPipelineConfiguration(pipe);
}
/**
* Notify the start of an element
*
* @param nameCode integer code identifying the name of the element within the name pool.
* @param typeCode integer code identifying the element's type within the name pool.
* @param properties properties of the element node
*/
public void startElement(int nameCode, int typeCode, int locationId, int properties) throws XPathException {
if (!cache.contains(nameCode)) {
if (!checker.isValidNCName(pool.getLocalName(nameCode))) {
XPathException err = new XPathException("Invalid XML 1.0 element name " +
Err.wrap(pool.getLocalName(nameCode), Err.ELEMENT));
err.setErrorCode("SERE0005");
err.setLocator(new ExpressionLocation(getPipelineConfiguration().getLocationProvider(), locationId));
throw err;
}
cache.add(nameCode);
}
nextReceiver.startElement(nameCode, typeCode, locationId, properties);
}
/**
* Notify an attribute. Attributes are notified after the startElement event, and before any
* children. Namespaces and attributes may be intermingled.
*
* @param nameCode The name of the attribute, as held in the name pool
* @param typeCode The type of the attribute, as held in the name pool
* @param properties Bit significant value. The following bits are defined:
* DISABLE_ESCAPING Disable escaping for this attribute
* NO_SPECIAL_CHARACTERS Attribute value contains no special characters
* @throws IllegalStateException: attempt to output an attribute when there is no open element
* start tag
*/
public void attribute(int nameCode, int typeCode, CharSequence value, int locationId, int properties) throws XPathException {
if (!cache.contains(nameCode)) {
if (!checker.isValidNCName(pool.getLocalName(nameCode))) {
XPathException err = new XPathException("Invalid XML 1.0 attribute name " +
Err.wrap(pool.getLocalName(nameCode), Err.ATTRIBUTE));
err.setErrorCode("SERE0005");
err.setLocator(new ExpressionLocation(getPipelineConfiguration().getLocationProvider(), locationId));
throw err;
}
cache.add(nameCode);
}
checkString(value, locationId);
nextReceiver.attribute(nameCode, typeCode, value, locationId, properties);
}
/**
* Character data
*/
public void characters(CharSequence chars, int locationId, int properties) throws XPathException {
checkString(chars, locationId);
nextReceiver.characters(chars, locationId, properties);
}
/**
* Output a comment
*/
public void comment(CharSequence chars, int locationId, int properties) throws XPathException {
checkString(chars, locationId);
nextReceiver.comment(chars, locationId, properties);
}
/**
* Processing Instruction
*/
public void processingInstruction(String target, CharSequence data, int locationId, int properties) throws XPathException {
if (!checker.isValidNCName(target)) {
XPathException err = new XPathException("Invalid XML 1.0 processing instruction name " +
Err.wrap(target));
err.setErrorCode("SERE0005");
err.setLocator(new ExpressionLocation(getPipelineConfiguration().getLocationProvider(), locationId));
throw err;
}
checkString(data, locationId);
nextReceiver.processingInstruction(target, data, locationId, properties);
}
/**
* Check that a string consists of valid XML 1.0 characters (UTF-16 encoded)
* @param in the string to be checked
* @param locationId the location of the string
*/
private void checkString(CharSequence in, long locationId) throws XPathException {
final int len = in.length();
for (int c=0; c
© 2015 - 2025 Weber Informatics LLC | Privacy Policy